Writing a Web Application
- I. Introduction
- What is a Web Application?
- II. Guidelines for Developing a Web Application
- Directory Structure
- Deploying on WebLogic Server
- Using a Default Web Application
- The ServletContext
- URLs and Web Applications
- Using Initialization Parameters for Deployment-time Configuration
- III. Creating the Deployment Descriptor
- Steps to Create a Deployment Descriptor file (web.xml)
- Mapping External Resources: Creating the weblogic.xml file
- Steps to Create the weblogic.xml File
- IV. Packaging a Web Application
- Steps to create a .war archive
- Related documents
- Using WebLogic HTTP Servlets
- Using WebLogic JSP
I. Introduction
This document describes how to write a Java 2 Enterprise Edition
(J2EE) Web Application for WebLogic Server. Deploying your web-based applications as Web Applications offers many advantages, including:
- A compact, consistent, and portable directory structure.
- You have the ability to segregate applications and provide a
unique environment for each application.
- EJBs and other resources can be tied together with web-based components.
What Is a Web Application?
"Web Application" is a concept defined by the Java Servlet
Specification v2.2 that describes a set of server-side
resources that make up an online application. These resources include:
- Servlets
- JavaServer Pages (JSP)
- Static HTML pages
- Server side classes
- Enterprise JavaBeans (EJB)
- Other resources specific to the Web Application
The specification defines how to package these resources into a
portable Web Application aRchive (.war) file so that they can be deployed on an
application server (such as WebLogic Server) that supports J2EE Web
Applications. A Web Application operates within a servlet context that
defines an environment that is separate from other Web Applications
running on the same server. Each Web Application is accompanied by a
deployment descriptor that ties its resources together and describes
how the resources are deployed on an application server.
WebLogic Server supports the Servlet v2.2 specification and can
host multiple Web Applications.
II. Guidelines for Developing a Web Application
A Web Application must follow certain guidelines in order to be
archived into a .war file, or be deployed on a Servlet 2.2 compliant
server. The scope of a Web Application defines the HTTP interface and
logical presentation of the application.
Each Web Application is accompanied by a deployment descriptor
that describes how the application is deployed on the host server. A
well-designed Web Application can be implemented in such a way that it
is abstracted from the physical location of resources on the Web
server. You can then use the deployment descriptor to adapt the
application to the environment of a host server at deployment time
without recompiling or changing any source code.
Directory Structure
You develop your Web Application within a specified directory
structure so that it can be archived and deployed on WebLogic Server,
or another Servlet 2.2 compliant server. All servlets, classes,
static files, and other resources belonging to a Web Application are
organized under a directory hierarchy. The root of this hierarchy
defines the document root of your Web Application. All files under
this root directory can be served to the client, except for files
under the special directories WEB-INF and META-INF located in the root directory.
Private files should be located in the WEB-INF directory, under the root
directory. All files under WEB-INF are private, and are not be served to a
client. Make sure the WEB-INF directory
contains the following files:
- WEB-INF/web.xml
- The Web Application deployment descriptor that configures the web
application.
- WEB-INF/weblogic.xml
-
A WebLogic Server-specific file that defines how named resources in
the web.xml file are mapped to
resources residing elsewhere in WebLogic Server.
- WEB-INF/classes
- Contains server-side classes such as HTTP servlets and utility classes
- WEB-INF/lib
- Contains .jar files used by the Web Application
Deploying on WebLogic Server
You can deploy the Web Application as an expanded directory
hierarchy (as described under Directory
Structure above), or in an archived format as a .war file. It is
useful to deploy the Web Application as an expanded set of directories
during development, then deploy it as an archived .war file in a production environment.
To deploy a Web Application on WebLogic Server, add the
following property to the weblogic.properties file:
weblogic.httpd.webApp.myapp=location
Where:
- myapp
- Is the context name given to the Web Application. The context name is
included in the initial part of any URL request to the Web Application.
- location
- Is the root directory of the Web Application, or the location of the .war file that contains the Web Application
archive.
All other configuration for your Web Application is defined in
the individual deployment descriptors (the web.xml file) for each Web Application. Deployment descriptors are described in detail
later in this document.
Default Web Application
A default Web Application handles all requests that do not match a Web
Application that is defined in the weblogic.properties file. Define a default Web
Application by specifying the following property in the weblogic.properties file: weblogic.httpd.defaultWebApp=DefaultWebApp
Where:
- DefaultWebApp
- Is the fully qualified path name to the
.war file you want as the default Web Application. An example of this would be:
weblogic.httpd.defaultWebApp=c:\webapp\mywebapp
You would request pages in this default Web Application with this URL:
http://host:port/somefile.ext
If you do not explicitly define a default Web Application as
shown above, WebLogic Server will implicitly define a default
Web Application. This implicitly-defined Web Application will have as
its document root the directory defined in the weblogic.properties file with the property weblogic.httpd.documentRoot . If you
do not explicitly define a default Web Application, and you do not
define a document root, WebLogic Server will use the myserver/public_html directory in
the WebLogic Server distribution as the document root.
If you are porting an application that was deployed on an earlier
version of WebLogic Server, using the implicitly defined default Web
Application allows you to retain the URLs you previously used to
request your servlets, JSP files, and static pages, for example:
http://host:port/somefile.jsp
If you move such an application into an explictly-defined
Web Application, you will be required to request the application by
including the name of the Web Application in the URL, for example:
http://host:port/myapp/somefile.jsp
Properties that describe the behavior of a Web Application are
defined in the web.xml file
for that Web Application (also called the deployment descriptor).
In contrast, the behavior of an implicitly defined Web
Application is defined only in the weblogic.properties file. Although some properties set
in the weblogic.properties
file affect the behavior of Web Applications, properties set in the
weblogic.properties file
are not necessarily inherited by Web Applications.
The diagrams below describe the relationship between Web
Applications and an implicitly or explicitly defined default Web
Application.
Explicitly Defined Web Applications
Implicitly Defined Default Web Application
The ServletContext
Each Web Application runs within a separate context that isolates
it from other Web Applications on the host server. All servlets within
a Web Application running in a WebLogic Server have access to shared
information and resources via the ServletContext object.
Note, however, that the ServletContext is only shared by a Web Application
running in the same virtual machine (VM). That is, the ServletContext is not shared
by servlets deployed from the same Web Application that are running on
a different server in a WebLogic Cluster. Web Applications running on
different servers in a WebLogic Cluster must use another mechanism,
such as a shared database, to share data.
URLs and Web Applications
The context of a Web Application is always reflected in the URL
used to access the Web Application. The Servlet 2.2 specification
breaks a URL into the following components:
requestURI = contextPath + servletPath + pathInfo
Where the contextPath is the
name of the Web Application and servletPath is the registered name of a servlet, and
pathInfo provides additional
parameters that can be parsed and used by the servlet represented by
the servletPath.
Constructing URLs in this manner requires careful attention when
creating hyperlinks between web pages within the same Web
Application. For example, consider a Web Application operating within
a context path named catalog
on a WebLogic Server running at the address http://www.beasys.com. A file called index.html in the Web Applications's
document root is accessed via the URL http://www.beasys.com/catalog/index.html. All absolute
requests to pages or servlets within the Web Application must contain
the contextPath.
Because the contextPath is
required when using absolute URLs, your web pages and servlets must
make careful reference to other pages within the Web Application. If
you want your Web Application to be truly portable, do not assume that
the name of the Web Application will remain the same. Therefore, all
static pages should only make relative references to other pages and
servlets within the Web Application.
Your dynamic pages (servlets or JSPs) can be more flexible because
they can obtain the servlet context name and the host address via the
Servlet 2.2 API, and then use them to build dynamic absolute links to
resources in the same Web Application.
Your servlets can obtain the contextPath portion of the URI using the getContextPath() method of the HttpServletRequest. Another useful
method is getRequestURI(),
which returns the URI that was used to request this servlet or JSP
page.
Using Initialization Parameters for Deployment-time Configuration
The J2EE programming model helps you to avoid hard coding the
addresses of the resources that your application uses by using the deployment descriptor to define deployment-time
variables that can be accessed and incorporated into the logic of the
servlets in your Web Application.
You can use initialization parameters in your Web Application to configure
its behavior from the deployment descriptor at deployment time. For
example, you could use initialization parameters to configure a
particular table in a database, or to specify the email address of a
Webmaster for the Web Application.
There are two scopes for accessing initialization parameters: a
the Web Application scope, and a per-servlet scope.
- Web Application Scope
- The ServletContext
provides access to initialization parameters that are shared by all
servlets in the Web Application. The ServletContext provides the following methods for
retrieving initialization parameters:
- public String getInitParameter(String parameterName);
- public Enumeration getInitParameterNames();
Configure initialization parameters in the deployment descriptor using
<context-param> elements.
The syntax for setting context parameters is described in more detail
later under Defining Context Parameters. Note that
all parameter names beginning with "weblogic." are reserved to configure WebLogic-specific
functionality. For more details, see Reserved Context
Parameter Names.
- Servlet scope
- The base class GenericServlet
provides access to initialization parameters defined on a per-servlet
scope. Because the HttpServlet
class extends the GenericServlet class, you can access the parameters via
the following methods:
- public String getInitParameter(String parameterName);
- public Enumeration getInitParameterNames();
Configure initialization parameters in the deployment descriptor
using <init-param> elements nested within a <servlet> element.
III. Creating the Deployment Descriptor
To deploy a Web Application, you create a deployment descriptor
that describes how the application is to be deployed on WebLogic
Server. The deployment descriptor defines many properties similar to
those defined in the weblogic.properties file, except that the deployment
descriptor only defines properties for its associated Web
Application. The following sets of properties are defined in the deployment
descriptor:
- Servlet / JSP definitions and mappings
- Session configuration
- Initialization parameters
- MIME type mappings
- Welcome file list
- Error pages
- Security
The deployment descriptor is a simple text file, formatted using XML
notation. Follow the steps in this section to create a deployment
descriptor. Depending on your application, you may not need to include
all of the elements listed here to create your Web Application. Each
step is described in detail below, at its corresponding link. The elements in the web.xml file must be entered in the order they are presented in this document.
- Create a deployment descriptor file called
web.xml under the WEB-INF directory. Use any text editor.
Create the following header:
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 1.2//EN"
"http://java.sun.com/j2ee/dtds/web-app_2_2.dtd">
The header refers to the location and version of the Document Type
Descriptor (DTD) file for the deployment descriptor. Although this
header references an external URL at java.sun.com, WebLogic Server contains its own copy of
the DTD file, so your host server need not have access to the
Internet. However, you must still include this <!DOCTYPE...> element in your
web.xml file, and have it
reference the external URL because the version of the DTD contained in
this element is used to identify the version of this deployment
descriptor.
- Create the main body of the web.xml file
by wrapping it within a pair of opening and closing <web-app> tags, after the pattern:
<web-app>
... body defining the Web Application ...
</web-app>
- Add elements within the <web-app>
... </web-app> tags that define your Web
Application. The remainder of this document describes these elements and how they help define your Web Application.
In XML, properties are defined by surrounding a property
name or value with opening and closing tags as shown above. The
opening tag, the body (the property name or value), and the closing
tag are collectively called an element. Some elements do not
use the surrounding tags, but instead use a single tag that contains
attributes called an empty-tag.
The body of the <web-app> element itself contains additional
elements that determine how the Web Application will run on WebLogic
Server. The order of the tag elements within the file must follow the
order reflected in this document. This ordering is defined in the DTD
file. For more information, refer to the DTD, available on Sun's
website at:
http://java.sun.com/j2ee/dtds/web-app_2_2.dtd.
Where an element contains other nested elements, this document illustrates
the nested elements by indenting them with a vertical blue line.
- Define Deployment-time Properties
These tags provide information for the deployment tools or the application
server resource management tools. These values are not used by the WebLogic
Server in this release.
- <small-icon>
iconfile </small-icon>
- (Optional) Names a 16x16 pixel .gif or .jpg image to represent the
Web Application. Currently, this is not used by WebLogic.
- <large-icon>
iconfile.gif </large-icon>
- (Optional) Names a 32x32 pixel .gif or .jpg image to represent the
Web Application. Currently, this is not used by WebLogic.
- <display-name>
application-name </display-name>
- (Optional) Gives the Web Application a name.
- <description>
descriptive-text
</description>
- (Optional) Verbose description of the Web Application.
- <distributable>
- (Optional) Currently, this element is not
used by WebLogic Server and does not affect the deployment of a Web
Application.
- Set up HTTP properties.
To make your HTTP servlets accessible to a Web browser, you first declare them
in the deployment descriptor, then map them to a URL pattern.
The scope of the servlet declarations and mappings in the deployment
descriptor are specific to this Web Application.
In other words, properties configured in the weblogic.properties are not necessarily inherited
by Web Applications.
Note the relationship of the following properties
from weblogic.properties to
Web Applications:
- MIME types set up in the weblogic.properties file are inherited by all Web
Applications. Additional MIME types can also be defined for each Web
Application. For details on defining MIME types for a Web Application,
see Defining MIME Types. For details on setting
MIME types in the weblogic.properties file, see Setting standard MIME Types.
- The document root will be the root directory of the Web
Application, not the directory specified with the weblogic.httpd.documentRoot property in the weblogic.properties file.
- Parameters specified using reserved context
parameters will override properties set in the weblogic.properties file. Reserved
context parameters have a <param-name> beginning with weblogic.. These include:
- servlets and servlet mappings
- session timeout
- mime types
- welcome file
- error pages
- security constraints and roles
- initArgs registered
for the JSPServlet in the weblogic.properties file will not be used in a Web
Application unless they are specified with a reserved context
parameter. For example a JSP compiler specified with the compileCommand init argument must be
specified in a Web Application with the weblogic.jsp.compile context parameter in the
web.xml file.
- The fileServlet is automatically registered for *.html and *.htm files and is the default servlet.
- The JSPServlet will be automatically registered for *.jsp files.

- Declare Servlets
Deploying a servlet involves two steps:
- Declare the
servlet. In this step, you give the servlet a name, declare the class
file (or jsp file) used to implement its behavior, and set other
servlet-specific properties.
- Map your named servlet to one or more URL patterns.
In the deployment descriptor, declare a <servlet> to represent each HTTP servlet class or
JSP file. The servlet declaration configures several properties
specific to that servlet resource. As mentioned above, you use a
different set of tags to map a <servlet> declaration to a URL pattern for an
HTTP request.
You can request JSP files using their file name. You do not need
to map the JSPServlet explicitly in a Web Application as you would in the
weblogic.properties file. All
files with the extension .jsp are
implicitly handled by the JSPServlet -- the Servlet 2.2
specification states that JSP files should be handled automatically. However,
an explicit JSP mapping in web.xml
will take precedence over the implicit mapping.
Use the following opening and closing tags to declare a servlet:
<servlet>
 |
 |
 |
Use a <servlet> tag to declare
each servlet in your Web Application. The servlet tag body is defined by the
following nested tags:
- <servlet-name>name</servlet-name>
- (Required) Defines the canonical name of the servlet, used to
reference the servlet definition elsewhere in the deployment descriptor.
- <servlet-class>package.name.MyClass</servlet-class>
-or-
<jsp-file>/foo/bar/myFile.jsp</jsp-file>
- (Required) The full package name of the HTTP servlet class, or the
name of a JSP file. You must use only one of these tags in your servlet body.
If you specify the <jsp-file>,
its location should be relative to the Web Application root directory.
- <init-param>
 |
 |
 |
- (Optional) Defines an initialization parameter for the
servlet at this scope. Use a separate set of <init-param> tags for each parameter. The body is
constructed of the following tags:
- <param-name>name</param-name>
- (Required) Defines the name of this parameter.
- <param-value>value</param-value>
- (Required) Defines a String value for this parameter.
- <description>...text...</description>
- (Optional) A verbose description of the initialization
parameter.
Here is an example of an initialization parameter, within some <servlet> tags.
<servlet>
<init-param>
<param-name> feedbackEmail </param-name>
<param-value> feedback123@beasys.com </param-value>
<description>
The email for web-site feedback.
</description>
</init-param>
</servlet>
|
- </init-param>
- <load-on-startup>loadOrder</load-on-startup>
- (Optional) This property is not honored by WebLogic Server in this
release.
- <security-role-ref>
- </security-role-ref>
- <small-icon>iconfile</small-icon>
- (Optional) Names a 16x16 pixel .gif or .jpg image to represent the
servlet. Currently, this is not used by WebLogic Server.
- <large-icon>iconfile</large-icon>
- (Optional) Names a 32x32 pixel .gif or .jpg image to represent the
servlet. Currently, this is not used by WebLogic Server.
- <display-name>Servlet Name</display-name>
- (Optional) Gives the servlet a display name, where Servlet Name is the name of your servlet.
- <description>...text...</description>
- (Optional) Verbose description of the servlet.
|
</servlet>
Here is an example servlet declaration using the above tags:
<servlet>
<servlet-name>LoginServlet</servlet-name>
<servlet-class>admin.servlets.Login</servlet-class>
<init-param>
<param-name>webmaster</param-name>
<param-value>webhelp@myco.com</param-value>
</init-param>
</servlet>

- Map a servlet or JSP to a URL
Once you declare your servlet or JSP
using a <servlet>
element, map it to one or more URL patterns to make it a public HTTP
resource. For each mapping, use a <servlet-mapping> element.
- <servlet-mapping>
 |
 |
 |
(Optional) A servlet-mapping element defines the mapping between a
servlet and a URL pattern. The body of the element contains the following
nested elements:
- <servlet-name>name</servlet-name>
- (Required) Declare the name of the servlet to which you are
mapping a URL pattern. This name corresponds to the name you assigned
a servlet in a <servlet>
declaration tag.
- <url-pattern>pattern</url-pattern>
- (Required) Describes a pattern used to resolve URLs. The
portion of the URL after the http://host:port + contextPath is compared to the <url-pattern> by WebLogic Server. If the
patterns match, the servlet mapped in this element will be called.
Here are some sample patterns:
/soda/grape/*
/foo/*
/contents
*.foo
|
- </servlet-mapping>
Here is an example of a <servlet-mapping> for the <servlet> declaration example used earlier:
<servlet-mapping>
<servlet-name>LoginServlet </servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>

- Configure HTTP Session Behavior
<session-config>
 |
 |
 |
(Optional) Configures the behavior of HTTP sessions in your Web
Application. Takes the following nested element:
- <session-timeout>time</session-timeout>
- (Optional) Defines the time that an inactive session remains valid
in minutes.
|
</session-config>

- Define MIME Types
MIME types tell the web browser how to handle a page's
content. You use a <mime-type> element to map a file extension to a
well known MIME type. You can use this to alter the way browsers see a
particular type of file in your Web Application. For instance, you
may want to serve .java source
files as plain text.
Your Web Application also inherits any MIME types defined in the
weblogic.properties file. For
information on setting up default MIME types, see the Administrators
Guide Setting up WebLogic as an
HTTP server.
<mime-mapping>
 |
 |
 |
(Optional) Defines a mapping between a file extension and a MIME type.
The body of this element must contain the following nested elements:
- <extension>ext</extension>
- (Required) Where ext is the
extension of the file. The extension specified is not preceded with a dot.
- <mime-type>type</mime-type>
- (Required) Where type is
the well known MIME type assigned to files ending with the extension ext.
|
</mime-mapping>

- Define Welcome and Error Pages
These elements define what the Web Application should do when
the requested URL does not map to a specific file.
<welcome-file-list>
 |
 |
 |
(Optional) A welcome-file is served when a browser requests a URL
that resolves to a directory, but cannot be resolved to a servlet. If a file
matching the filename of a welcome-file exists in the requested directory,
that file is served back to the client. A typical welcome-file name is
index.html, and might be included
in some directories to prevent the client a from receiving a directory
listing.
The <welcome-file-list>
defines all welcome files for this Web Application.
- <welcome-file>filename</welcome-file>
- (Required) You use one or more <welcome-file> elements to list each filename that the
Web Application recognizes as a welcome-file.
|
</welcome-file-list>
<error-page>
 |
 |
 |
(Optional) Allows you to configure the Web Application to respond with
a given file in the event of an error. You may configure error pages that
respond to either Java exceptions (such as java.lang.exception) or HTTP error codes (such as #404 File-not-found).
- <error-code>number</error-code>
- (Required or use <exception-type>) Sends the
- <exception-type>exception</exception-type>
- (Required or use <error-code>)
- <location>URL</location>
- (Required)
|
</error-page>
Note: Use either <error-code> or <exception-type>, inside an <error-page>
element but not both.
- Define context parameters. Context parameters affect the entire Web Application.
<context-param>
 |
 |
 |
(Optional) Specifies a parameter that may be accessed by any
servlet within the Web Application servlet context (see Initialization Parameters, above). Context
parameters allows you to configure parts of your Web Application at
deployment time. You can use multiple <context-param> blocks, each defining a single
parameter.
- <param-name>MyParamName</param-name>
- (Required) The name of the parameter.
- <param-value>MyParamValue</param-value>
- (Required) The String value of the parameter.
- <description>...text...</description>
- (Optional) A description of the parameter purpose.
|
</context-param>
Here is an example definition of a context parameter:
<context-param>
<description>
This parameter defines the email address of the webmaster
</description>
<param-name>Webmaster</param-name>
<param-value>scoobie@snacks.com</param-value>
</context-param>
Reserved Context Parameter Names
All context parameter names beginning with "weblogic." are reserved for WebLogic Server related
properties. For example:
<context-param>
<param-name>weblogic.httpd.servlet.reloadCheckSecs</param-name>
<param-value>10</param-value>
</context-param>
Setting the context parameter shown in the example above configures
WebLogic Server to wait the given number of seconds between checking
for modified servlet classes. This reserved context parameter is
available to your servlet code with the ServletContext.getInitParameter() method (as are all
context parameters). For details on accessing context parameters and
servlet parameters from your servlets and JSP pages, see the section
Using Deployment-time Configuration.
These are the reserved WebLogic context parameter names and their
function:
- weblogic.httpd.servlet.reloadCheckSecs
- Where the <param-value> gives the interval in seconds that
WebLogic checks for modified servlet classes. For more details on this
property, see
Reloading servlet
classes in the Setting WebLogic Properties document.
- weblogic.httpd.defaultServlet
- Where the <param-value> specifies the fully qualified class
name of a servlet that handles a URL request that cannot be resolved
to any other servlet. For more details on the default servlet, see Setting a default
servlet in the Setting WebLogic Properties document.
- weblogic.httpd.documentRoot
- Where the <param-value>
redefines the location of the document root for this servlet context. The
default document root is the root directory of the Web Application.
- weblogic.httpd.servlet.classpath
- Where the <param-value> redefines the servlet
classpath--the location from which the Web Application loads its
servlet classes. In a Web Application, the servlet classpath defaults
to the WEB-INF/classes
directory. WebLogic Server treats this classpath as the equivalent of
the classpath established by the servlet.classpath
property that is specified in the weblogic.properties file.
- weblogic.jsp.precompile
- If set to true, all JSP files in the Web Application will be
pre-compiled when WebLogic Server starts up. If set to false, JSP
files will be compiled when they are first requested. The default is
false.
- weblogic.jsp.compileCommand
- Specifies the full pathname of the standard Java compiler used to
compile the generated JSP servlets. For example, to use the standard
Java compiler, specify its location on your system as the
<param-value>:
<param-value>/jdk117/bin/javac.exe</param-value>
- weblogic.jsp.verbose
- When set to true, debugging information is printed out to the browser, the
command prompt, and WebLogic Server log file.
- weblogic.jsp.packagePrefix
- Specifies the package into which all compiled JSP pages will be
placed.
- weblogic.jsp.keepgenerated
- Keeps the Java files that are created as an intermediary
step in the compilation process.
- weblogic.jsp.pageCheckSeconds
- Sets the interval at which WebLogic Server checks to see if JSP files
have changed and need recompiling. Dependencies are also checked
and recursively reloaded if changed. If set to 0, pages are checked on
every request. If set to -1, page checking and recompiling is
disabled.
- weblogic.jsp.encoding
- (Optional) Specifies the character set used in the JSP page. If not
specified, the default encoding for your platform is used.

- Define a JSP Tag Library Descriptor
<taglib>
 |
 |
 |
(Optional) Associates the location of a JSP Tag Library Descriptor (TLD) with a URI
pattern. Although you can specify a TLD in your JSPs that is relative
to the WEB-INF directory, you can also use the <taglib> tag to configure the TLD
when deploying your Web Application. Use a separate element for each
TLD. The URI pattern and the TLD location are defined by the following
elements:
- <taglib-uri>string_pattern</taglib-uri>
- (Required) Gives a string pattern. This pattern is matched against the URI
string used in the taglib directive on the JSP page. For example:
<%@ taglib uri="string_pattern" prefix="taglib" %>
For more details, see the Developers Guide,
"Writing JSP extensions".
- <taglib-location>filename</taglib-location>
- (Required) Gives the filename of the tag library descriptor
relative to the document root. That is, the root directory of the Web
Application. It is wise to store the tag library descriptor file under the
WEB-INF directory so it is not
available over HTTP request.
|
</taglib>

- Identify external resources
<resource-ref>
 |
 |
 |
(Optional) Defines a reference lookup name to an external resource.
This allows the servlet code to look up a resource by a 'virtual' name that is
wired to the actual location at deployment time. Use a separate <resource-ref> element to define each
external resource name. The external resource name is mapped to the actual
location name of the resource at deployment time in the WebLogic-specific
deployment descriptor weblogic.xml .
- <res-ref-name>name</res-ref-name>
- (Required) The name of the resource used in the JNDI Tree.
Servlets in the Web Application use this name to look-up a reference to the
resource.
- <res-type>Java class</res-type>
- (Required) The Java type of the resource that corresponds to the
reference name. Use the full package name of the Java type.
- <res-auth> CONTAINER | SERVLET</res-auth>
- (Required) Used to control the resource sign-on for security.
|
</resource-ref>


- Set up security constraints
A Web Application that uses security requires the user to login in order to
access its resources. The user's credentials are verified against a security
realm, and once authorized, the user will have access only to specified resources
within the Web Application.
Security in a Web Application is configured using three
elements:
- The <login-config> element specifies how the user is
prompted to login and the location of the security realm. If this
element is present, the user must be authenticated in order to access
any resource that is constrained by a <security-constraint>
defined in the in the Web Application.
- A <security-constraint> is used to define the
access privileges to a collection of resources via their URL
mapping.
- A <security-role> element represents a group or
principal in the realm. This security role name is used in the <security-constraint> element
and can be linked to an alternative role name used in servlet code via
the <security-role-ref>
element.
<security-constraint>
 |
 |
 |
(Optional) This element contains the following nested elements. You may
use one or more of these elements to define access privileges on certain
resources in the Web Application.
<web-resource-collection>
 |
 |
 |
(Required) Each <security-constraint>
element must have one or more
<web-resource-collection>
elements. These define the area of the Web
Application that this security constraint is applied to.
- <web-resource-name>name</web-resource-name>
- (Required) You must give this group of web resources a name so that it
may be identified.
- <description>...text...</description>
- (Optional) A verbose description of this security constraint.
- <url-pattern>pattern</url-pattern>
- (Optional) You may use one or more of these elements to declare
which URI patterns this security constraint applies to. Please see the
Servlet 2.2 specification for a description of how to construct the URI
pattern. If you do not use at least one of these elements, this
<web-resource-collection>
is ignored by WebLogic Server.
- <http-method>GET | POST</http-method>
- (Optional) You may use one or more of these elements to declare
which HTTP methods are subject to the authorization constraint. If you omit
this element, the default behavior is to apply the security constraint to all
HTTP methods.
|
</web-resource-collection>
<auth-constraint>
</auth-constraint>
<user-data-constraint>
 |
 |
 |
(Optional) This element defines how the client should communicate with
the server.
- <description>...text...</description>
- (Optional) A verbose description.
- <transport-guarantee>NONE | INTEGRAL | CONFIDENTIAL</transport-guarantee>
- (Optional)
Possible Values:
- NONE
- Transport guarantees are not used.
- INTEGRAL
- Requires
data to be transmitted in a way that does not allow the data to be
altered. WebLogic Server establishes a Secure Sockets Layer (SSL) connection when the user is
authenticated using this constraint.
- CONFIDENTIAL
- Requires that the data be transmitted in a way that prevents the
data from being observed during transit. WebLogic Server establishes a
Secure Sockets Layer (SSL) connection when the user is authenticated using this constraint.
|
</user-data-constraint>
|
</security-constraint>
<login-config>
 |
 |
 |
(Optional) This element configures how the user is
authenticated. If this element is present, the user must be
authenticated in order to access any resource
that is constrained by a <security-constraint> defined in the in the Web
Application. Once authenticated, they may be authorized to access
other resources with access privileges.
- <auth-method>BASIC | FORM | CLIENT-CERT</auth-method>
- (Optional) Specifies the method used to authenticate the user.
The CLIENT-CERT option is
only available in WebLogic Server 5.1, with service pack 5 or later.
For more information on certificates and two-way authentication see Using SSL in the WebLogic Environment.
To use a
client certificate for authentication, implement the
weblogic.security.acl.CertAuthenticator interface. For more
information see the SimpleCertAuthenticator code
example.
- <realm-name>realmname</realm-name>
- (Optional) The name of the realm that is referenced to authenticate
the user credentials. If omitted, the WebLogic realm is used by default.
- <form-login-config>
</form-login-config>
|
</login-config>
<security-role>
 |
 |
 |
(Optional) Use one or more of these elements to declare user roles.
- <description>...text...</description>
- (Optional) A verbose description of this security role
- <role-name>rolename</role-name>
- (Optional) The role name. The name you use here must then
be map directly to principals or groups belonging to a security realm
defined in the weblogic.properties file. As of WebLogic Server 5.1,
Service Pack 3, you can also map role names used in your Web Application to
principals in a realm (with different names) using the weblogic.xml file.
|
</security-role>

- Set environment entries
<env-entry>
 |
 |
 |
(Optional) Used to declare an environment entry for an application. Use
a separate element for each environment entry.
- <description>...text...</description>
- (Optional) A textural description.
- <env-entry-name>name</env-entry-name>
- (Required) The name of the environment entry.
- <env-entry-value>value</env-entry-value>
- (Required) The value of the environment entry
- <env-entry-type>type</env-entry-type>
- (Required) The type of the environment entry. Can be set to one of
the following standard Java types:
- java.lang.Boolean
- java.lang.String
- java.lang.Integer
- java.lang.Double
- java.lang.Float
|
</env-entry>

- Identify Enterprise JavaBean (EJB) resources
<ejb-ref>
 |
 |
 |
(Optional) Defines a reference to an EJB
resource. This reference is mapped to the actual location of the EJB
at deployment time by defining the mapping in the WebLogic-specific
deployment descriptor file, weblogic.xml. Use a separate <resource-ref> element to define each
reference EJB name.
- <description>...text...</description>
- (Optional) A textual description.
- <ejb-ref-name>name</ejb-ref-name>
- (Required) The name of the EJB used in the JNDI Tree.
Servlets in the Web Application use this name to look-up a reference to the EJB.
- <ejb-ref-type>Entity | Session</ejb-ref-type>
- (Required) The type of the EJB. Use either
Entity or
Session.
- <home>mycom.ejb.AccountHome</home>
- (Required) The fully qualified class name of the EJB home
interface.
- <remote>mycom.ejb.Account</remote>
- (Required) The fully qualified class name of the EJB remote
interface.
- <ejb-link>ejb.name</ejb-link>
- (Optional) The ejb-name of an EJB in an encompassing J2EE
application package.
|
</ejb-ref>

Creating the
weblogic.xml File:
Mapping External Resources
If you define external resources such as DataSources, EJBs, or a
Security realm (mapping of Security realms is available only in
WebLogic Server 5.1, Service Pack 3 or higher) in the web.xml deployment descriptor, you
can use any descriptive name to define the resource. To access the
resource, you then map this resource name to the actual name of the
resource in the JNDI tree using a file called weblogic.xml. Place this file in the WEB-INF
directory of your Web Application.
If your application does not contain references to external
resources, you do not need to create a weblogic.xml file.
A pair of sample web.xml
and weblogic.xml files are
available to demonstrate how these two deployment descriptor files
work together to define your Web Application. See the following links:
- web.xml
- weblogic.xml
Steps to Create the weblogic.xml File
- Begin the weblogic.xml
file with the following header:
<!DOCTYPE weblogic-web-app PUBLIC
"-//BEA Systems, Inc.//DTD Web Application 2.2//EN"
"http://www.beasys.com/j2ee/dtds/weblogic-web-jar.dtd">
This header refers to the location and version of the DTD file for the
deployment descriptor. Although this header references an external URL
at www.beasys.com, WebLogic
Server has its own copy of the DTD file, so your host server need
not have access to the Internet. However, you must still include this
DOCTYPE element in your weblogic.xml file, and have it
reference the external URL since the version of the DTD is used to
identify the version of this deployment descriptor.
Note that the order of the tag elements within the file must
follow the order specified in the DTD file. This ordering is reflected
in this document, but you should refer to the DTD for the exact
specification. The DTD is located in your WebLogic Server distribution
at:
//weblogic/classes/weblogic/servlet/internal/dd/weblogic-web-jar.dtd
- Wrap the main body of the weblogic.xml file within a pair of opening and closing
<weblogic-web-app> tags, for example:
<weblogic-web-app>
...
</weblogic-web-app>
The body of the <weblogic-web-app> element may contain the following tags:
- <description> ... </description>
- descriptive text describing the element
- <security-role-assignment> ... </security-role-assignment>
- maps security roles to principals in a realm defined in the weblogic.properties file
- <reference-descriptor> ... </reference-descriptor>
- maps resource names (as defined in the web.xml file) to JNDI names
- Map Security Role Names to a Security Realm
Note: Securitly Role mapping is only available in WebLogic Server 5.1, Service Pack 3 or higher.
<security-role-assignment>
</resource-description>
If you need to define multiple roles, define each additional pair of <role-name> and <principal-name> tags within separate <security-role-assignment> elements.
- Map non-EJB Resources
<resource-description>
 |
 |
 |
- <res-ref-name>name</res-ref-name>
- (Required) The name of the resource defined in your web.xml deployment descriptor.
- <jndi-name>JNDI name of resource</jndi-name>
- (Required) The JDNI name of the resource. For a DataSource, this will be the name of the DataSource as defined in your weblogic.properties file.
|
</resource-description>

- Map EJB Resources
<ejb-reference-description>
 |
 |
 |
- <ejb-ref-name>name</res-ref-name>
- (Required) The name of the EJB as defined in your web.xml deployment descriptor.
- <jndi-name>JNDI name of EJB</jndi-name>
- (Required) The JDNI name of the EJB as described in the weblogic-ejb-jar.xml file.
|
</ejb-reference-description>

IV. Packaging a Web Application
Packaging a Web Application involves creating an archive .war file. .war files can be served by any J2EE-compliant application server.
WebLogic Server can host a Web Application from a .war file or from an unpacked directory structure from a
.war file. The ability to run the Web
Application from the unpacked directory structure is useful during
development. The ability to create a .war archive is useful for transferring your Web Application
from a development environment to a live production environment.
Steps to create a .war archive:
- Structure your Web Application as described in the section Directory structure.
- Open a command shell, and change to the root directory of your Web
Application. In this example, we assume that your Web Application is called
myapp for this example, and that it is
located in the directory /weblogic/myserver/myapp.
- Make sure that you have a JDK available in the command shell's path.
- Run the following commands (the example show here is for Windows NT):
$ cd C:\weblogic\myserver\myapp
$ jar cf myapp.war *
The manifest file created as part of the JAR file and any other files
under the META-INF directory will not
be accessible when deployed on WebLogic Server. For more information on
packaging and signing JAR files, see the Java Tutorial section on
JAR files.
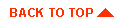