
The application uses a simple Java class to store the data and displays a list of employees on one page and a graph of salaries on another. As you build the application, you will add ADF layout components to further control and customize the behavior of the application.
In Part 2 of the tutorial, you add a call to a web service that accepts arguments and upgrades the salary of an employee. In Part 3, you add specific device integration to your application.
Assumptions: |
---|
To complete this tutorial you must have installed JDeveloper 11.1.2.4 and have installed the ADF Mobile Extension. You must also have the Android SDK version 4.2.2 API level 17 installed. If you choose, you can deploy the application to a connected Android device. The tutorial demonstrates deploying the application to an Android emulator. You launch the emulator using the Android Virtual Device (AVD) Manager, which is part of Android SDK Tools. If you are using an iOS environment, you can use the following tutorial to set up the development environment: Set Up and Configure an iOS Environment. If you are using an Android environment, you can use the following tutorial: Set Up and Configure an Android Environment. |
When you work in JDeveloper, you organize your work in projects within an application. JDeveloper provides several templates that you can use to create an application and projects. The templates are pre-configured with a basic set of technologies that are needed for developing various types of applications, and you create a working environment by selecting the template that fits your needs. You can then configure it to add any other technologies you plan to use.
In this first section, you create an application by using the ADF Mobile application template. You then create a couple of mobile features that will hold the application you are be building.
-
Open JDeveloper 11.1.2.4.
-
In the Application Navigator, click New Application.
-
In the New Gallery, select General > Applications > Mobile Application (ADF) and click OK.
-
In the Create Mobile Application (ADF) wizard, name the application Employees, and set the Application Package Prefix to mycomp. Click Next.
-
Click Next to accept the default Project Name (ApplicationController).
-
Notice the default package and click Next to accept the default value and continue.
-
Click Next to accept the Project Name for the second project.
-
On the last step of the wizard, notice the Default Package name and click Finish to create the application.
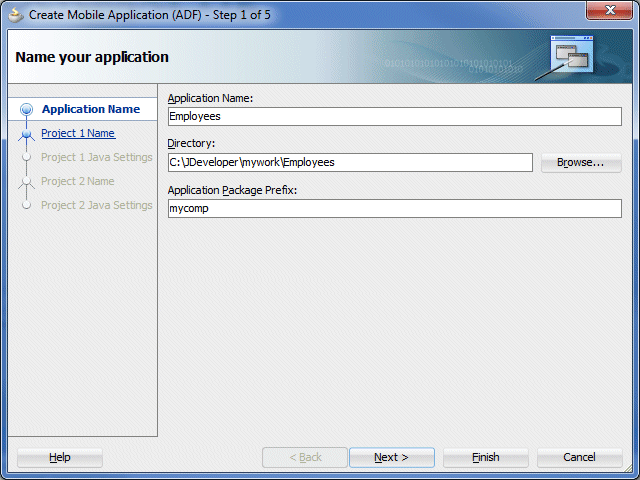
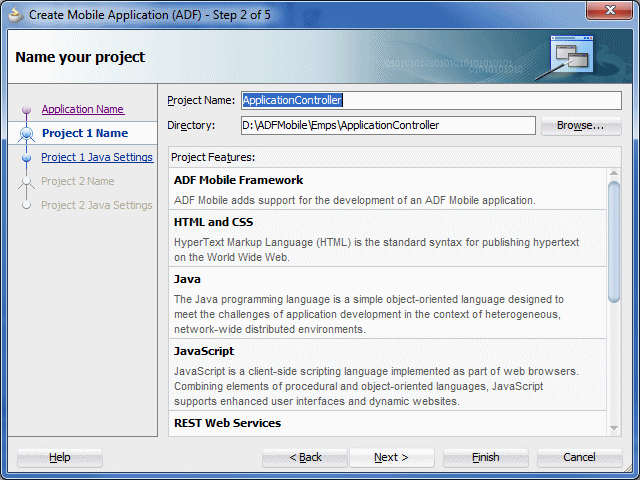
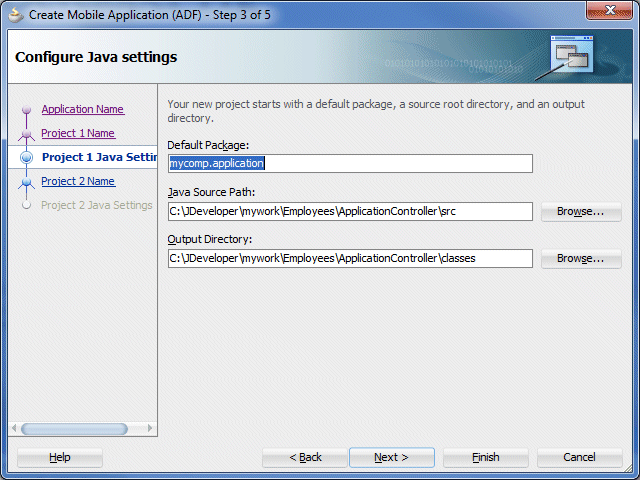
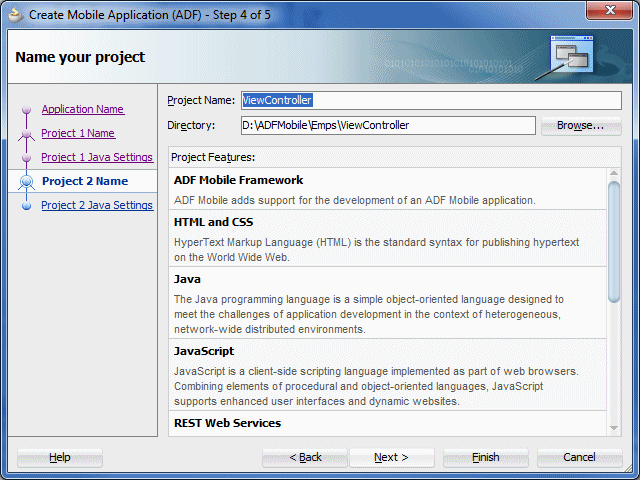
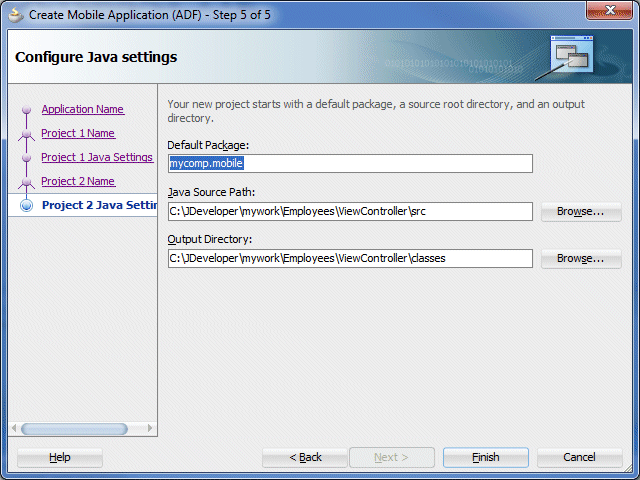
JDeveloper creates the application and opens it in the Application Navigator. The Application Navigator should look something like the following:
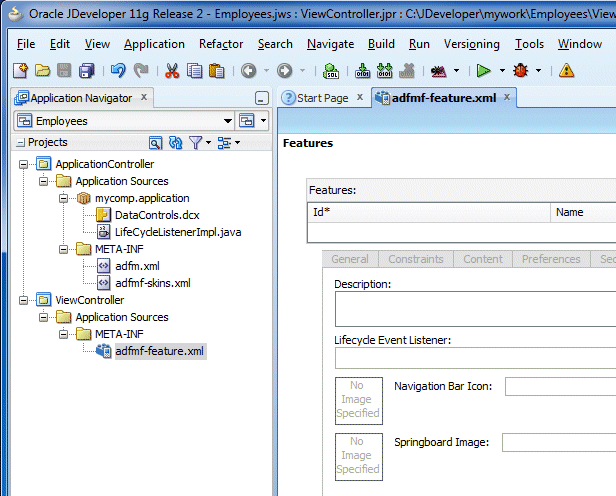
When you deploy an Oracle ADF Mobile application, the application will be a single application on the mobile device. The application may consist of several parts. For example, there may be a search component, a document (like a help page), a data entry form, or even a list. Each of these parts is defined as a feature within the application. In this section of the tutorial, you create the features that you will deploy as part of your application.
-
The features of an ADF Mobile application are defined in the adfmf-feature.xml file. If the file is not already open, double-click the file to open it in the editor.
-
In adfmf-features.xml, click the green plus sign
in the Features table to create a new feature.
-
Name the new feature Employees and click OK.
-
Click the green plus sign
in the Features table to create another new feature. Set the Feature Name to Help, then click OK.
-
In the Features table, select the Help feature. Under the Features table, click the Content tab. Set the Type to Local HTML.
-
Next to the URL property, click the Create (green plus sign
) icon to create the local HTML file.
-
Set the File Name to help.html and click OK.
-
JDeveloper creates the file and opens it in the editor. Enter "This document will be the Help System" in the help.html file.
-
Back in adfmf-feature.xml, select Employees in the Features table, and in the Content tab make sure that the Type is set to ADF Mobile AMX.
-
Save your work.
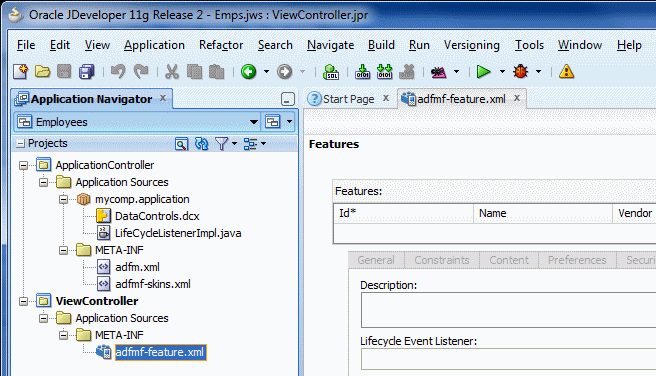
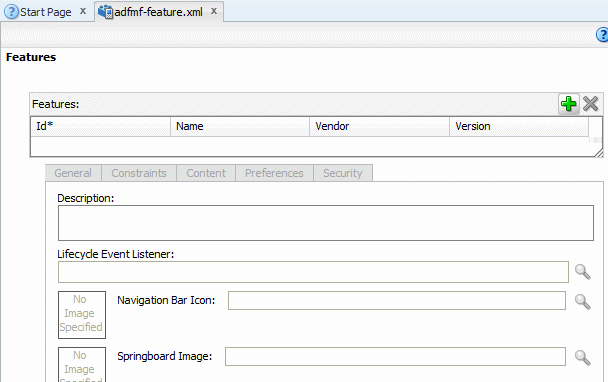
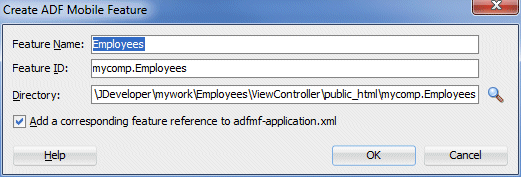
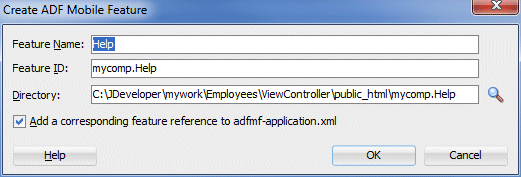
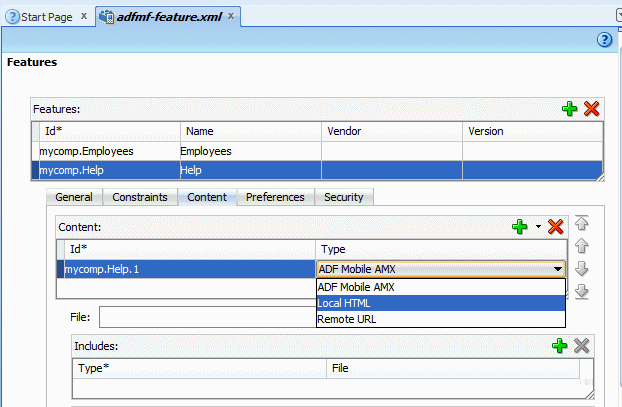
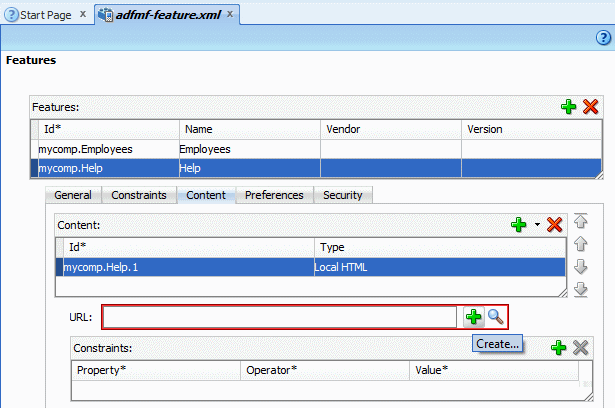
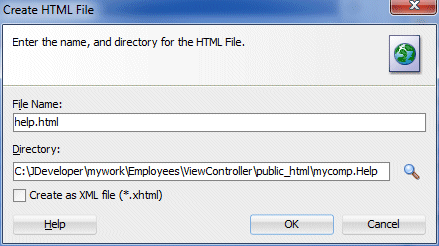
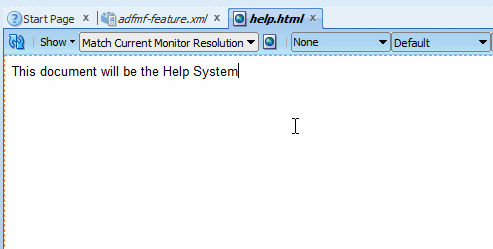
In this section you create an ADF task flow that will be consumed by the Employees feature. An ADF task flow contains multiple pages that interact with one another. The task flow provides structure to the interaction.
-
If it is not already open, double-click adfmf-feature.xml in the Application Navigator to open it in the editor. If it is already open, you can click the tab named adfmf-feature.xml.
-
In the editor, select the Employees feature, and click the Content tab.
-
Click the Add (green plus sign
) icon next to the File field on the Content table and select Task Flow. This will create a task flow and associate it with the Employees feature.
-
In the Create dialog box, set the File Name property to EmpsTaskFlow.xml and click OK.
-
Notice the Component Palette located in the upper-right corner of JDeveloper. From that palette, drag a view activity to the task flow.
-
Notice that the name of the view activity is highlighted in the task flow diagram. Change the name the view activity to empList.
-
Drag a second view activity to the task flow and name this one graph.
-
Click Control Flow Case on the Component Palette.
-
After you have selected the control flow case, click the empList view activity and then click the graph view activity.
-
Set the name of the control flow case to showGraph.
-
Select the showGraph control flow case and in the Property Inspector under Behavior, set the Transition property to flipRight.
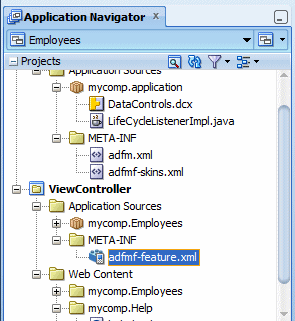
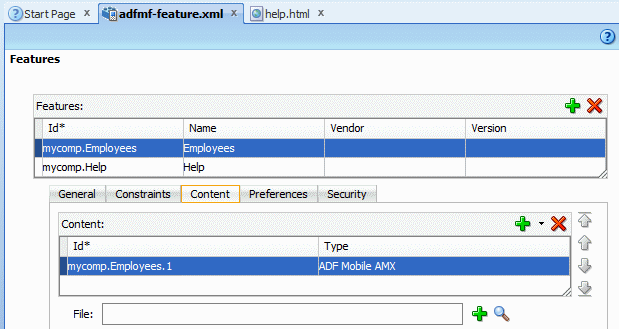
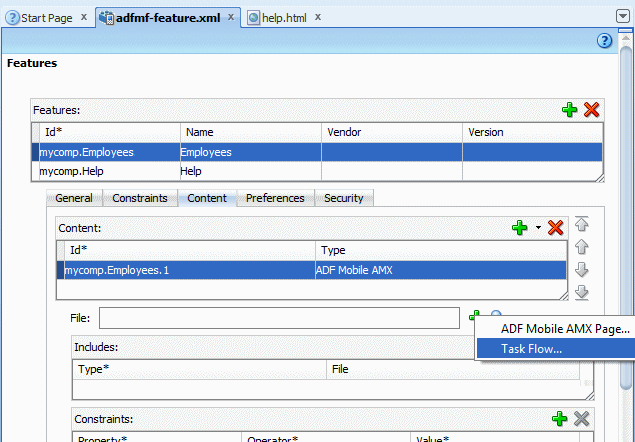
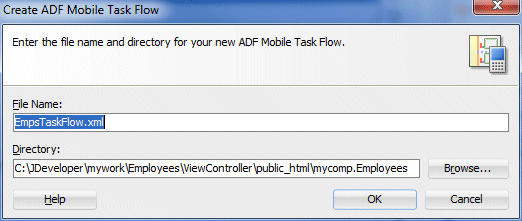
JDeveloper creates the task flow, and opens it in the editor.
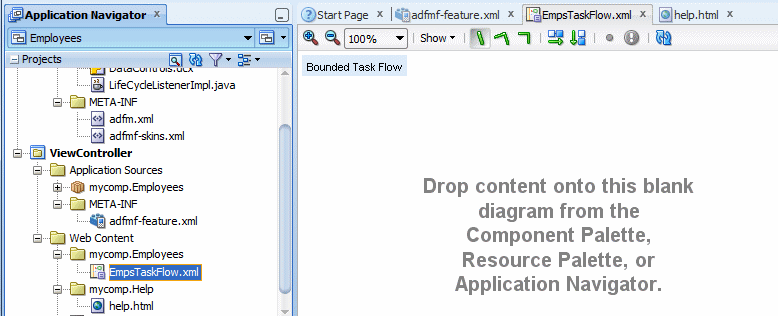
You can now add content to the task flow. The content will consist of view activities (pointers to pages), and control flow cases (navigation controls).
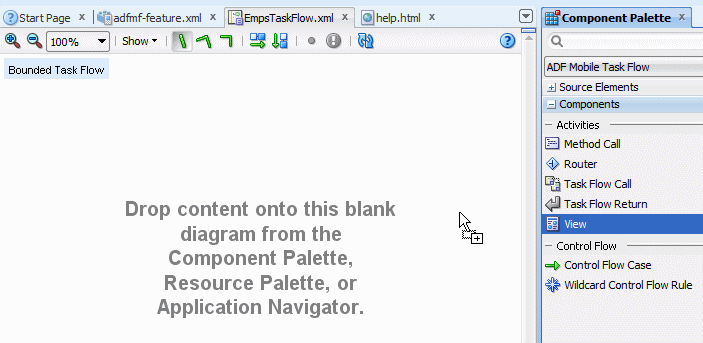
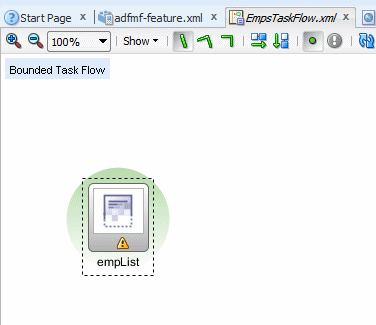
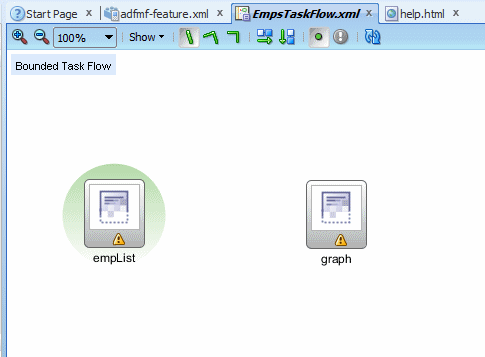
You now have two view activities on the task flow. The next step is to create navigation rules, or control flow cases, between the view activities.
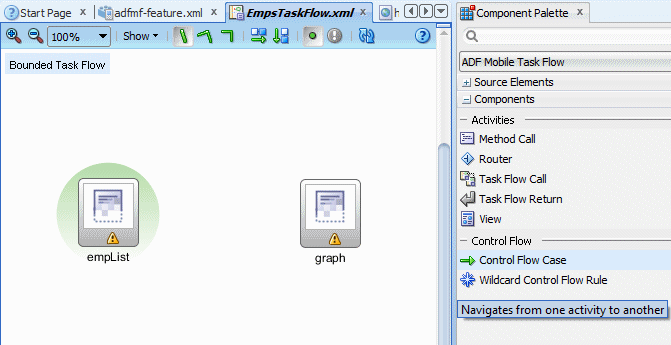
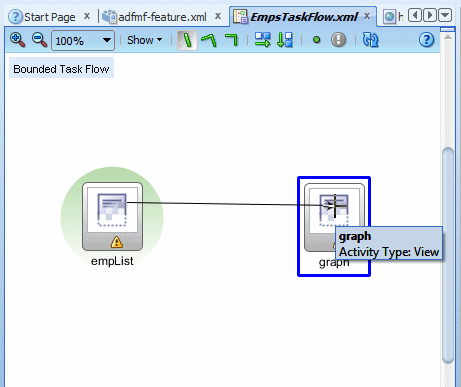
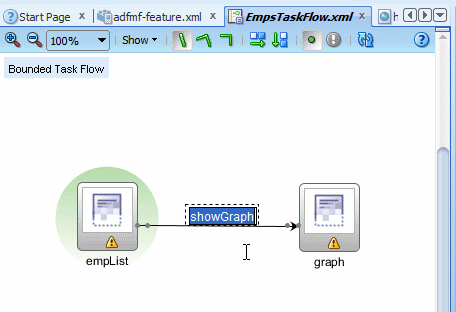
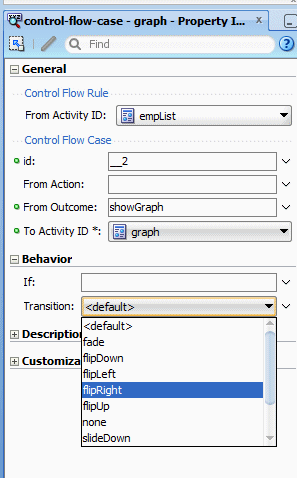
The EmpsTaskFlow should look something like the following:
Remember that the view activities you have created are merely pointers to pages. You create the underlying pages later in this tutorial.
ADF provides an application layer that manages data so that the UI can consume the data without concern about where or how the data is stored. This layer is called the data control layer.
This tutorial uses POJOs (Plain Old Java Objects) for the application data source. In this section, you learn how to create Java classes and then generate data controls that are based on the classes. The user interface portion of the application uses the data controls to present data to the user.
-
Right-click the ViewController project and select New.
-
In the New Gallery, select Java Class and click OK.
-
Name the class Emp and click OK to accept the other default values.
-
Add the following private variables. When you add Date hiredate, JDeveloper will prompt you to add an import. Choose java.util.Date for the import.
private String name;
private String email;
private int salary;
private Date hireDate; -
Add two constructors as follows:
public Emp(String name, String email) {
super();
this.name = name;
this.email = email;
}
public Emp(String name, String email, int salary, Date hireDate) {
super();
this.name = name;
this.email = email;
this.salary = salary;
this.hireDate = hireDate;
} -
Right-click inside the code editor and select Generate Accessors. This will create the getters and setters for the variables you choose.
-
Select Emp to select all the included methods, select the Notify listeners when property changes check box, and click OK.
-
Just as you did a few steps earlier, create a Java class and name it Emps. (HINT: Right-click ViewController and select New; choose Java Class.)
-
The following code is the complete code for the Emps class. Replace all of the code in the editor.
-
Select Emps.java in the Application Navigator (HINT: You can also press ALT+Home.)
-
Click Save All on the Toolbar to save your work.
-
From the Build menu, select Rebuild ViewController.jpr. This rebuilds all of the class files for the project.
-
In the Application Navigator, right-click Emps.java and select Create Data Control.
-
Save your work.
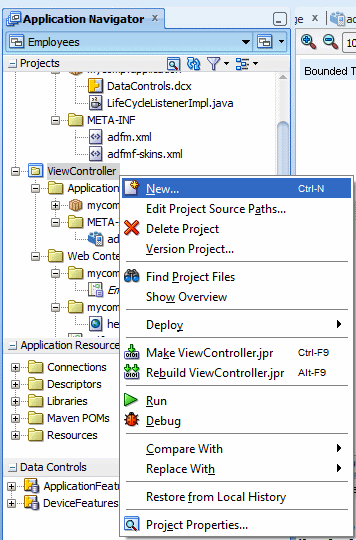
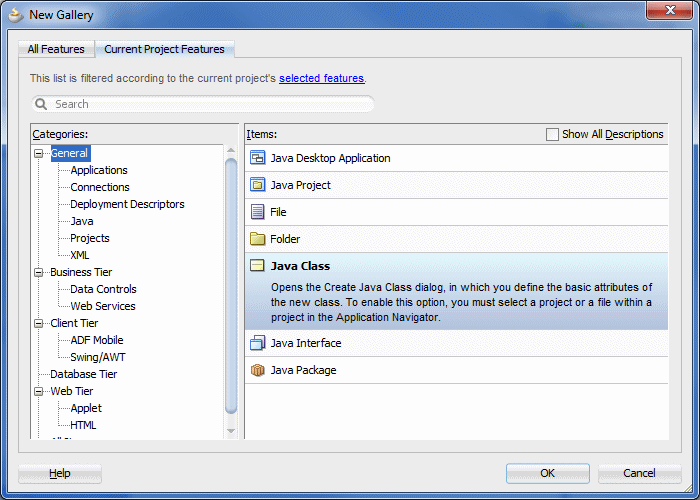
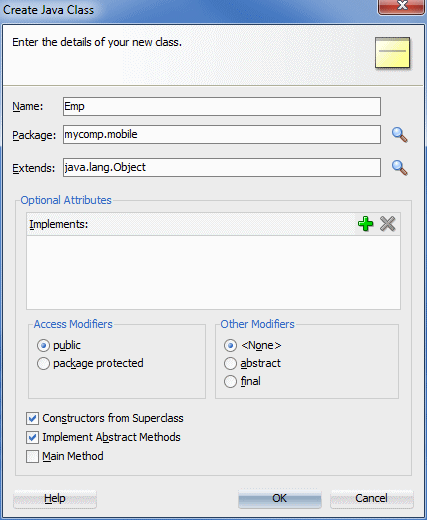
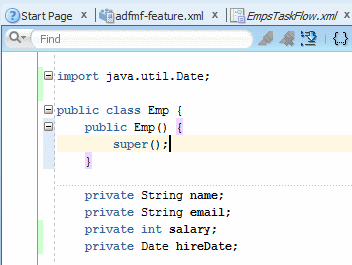
The code should look something like:
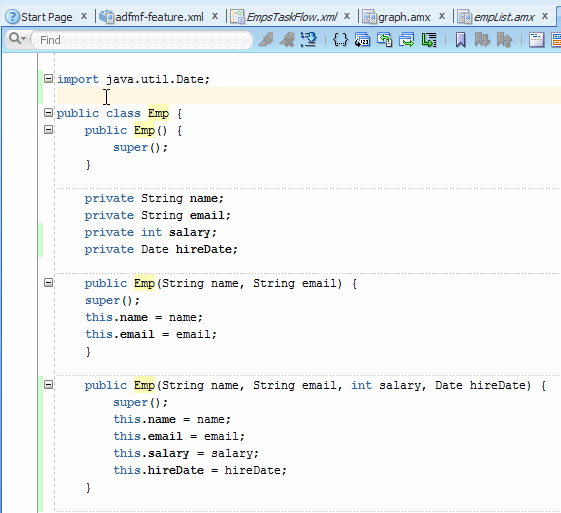
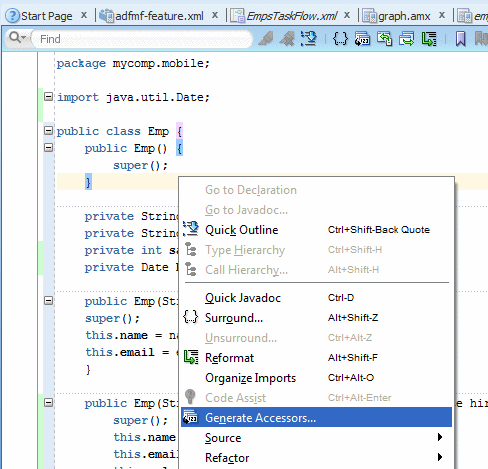
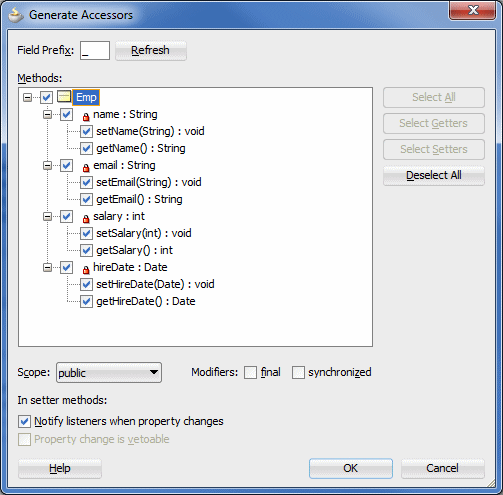
JDeveloper generates all of the getters and setters (accessors) for your class.
In the next few steps you create a class needed that contains the employee data.
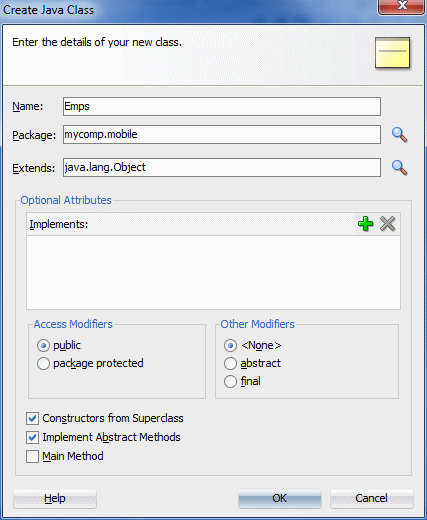
The class will look like:
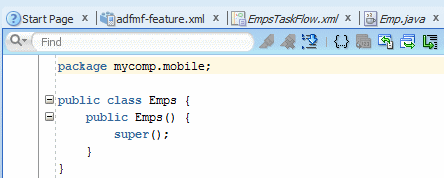
package mycomp.mobile;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
public class Emps {
private List s_emps = null;
public Emps() {
super();
}
public Emp[] getEmps() {
//This Method gets a list of the employees and their emails
Emp[] emps = null;
s_emps = new ArrayList();
s_emps.add(new Emp("Bill", "bill@oracle.com",4000, getADate(2011,3,24,9,0) )) ;
s_emps.add(new Emp("Gary", "gary@oracle.com", 5000, getADate(2007,2,24,9,0) )) ;
s_emps.add(new Emp("Jeff", "jeff@oracle.com", 5500, getADate(2003,2,19,9,0) )) ;
s_emps.add(new Emp("Joe", "joe@oracle.com", 4000, getADate(2012,2,13,9,0) )) ;
s_emps.add(new Emp("Shay", "shay@oracle.com",6000, getADate(2002,2,21,9,0) )) ;
emps = (Emp[]) s_emps.toArray(new Emp[s_emps.size()]);
return emps;
}
private Date getADate(int y,int m, int d,int h, int mi) {
Calendar c1 = Calendar.getInstance();
c1.set(y, m, d, h, mi);
Date retDate = c1.getTime();
return retDate;
}
}
The code should look like:
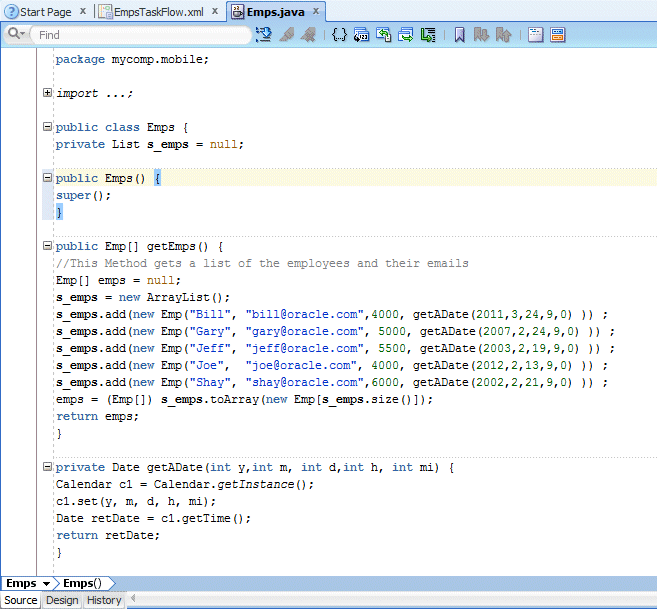
You now have a Java class that is the data source. Next you create an ADF data control that the application will use to consume the data.
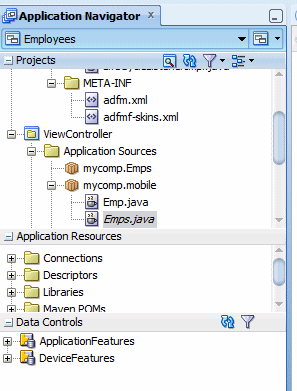
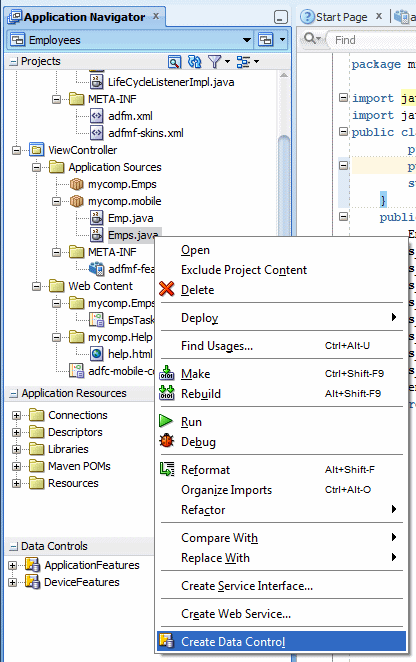
Now that you have a data control defined, you can begin creating the UI portion of the application.
In this section of the tutorial, you create the pages that are associated with the view activities you created on the task flow. You have already created the navigation rules, so you will now create the pages and add buttons for the application navigation.
-
Open EmpsTaskFlow.xml in the editor. (HINT: The tab should still be open, but if not, double-click EmpsTaskFlow.xml in the Application Navigator.)
-
Double-click empList and select all of the page facets. Click OK to create the page.
-
Use the drag bar to split the editor into two sections. The drag bar is the small vertical bar to the right of the bottom scroll bar in the editor.
-
In the editor, click the Preview tab at the bottom of the right pane to preview the UI.
-
In the code section, within the header facet, click the outputText component and set the value property to Emp List.
-
In the source editor, select the button in the primary facet and in the Property Inspector, set the Text property to Graph. When the user clicks this button, the application will display a graph of employee data.
-
Set the Action property to showGraph, which is the navigation case to navigate to the Graph page.
-
Expand the Data Controls palette to see the Emps data control.
-
Drag the emps collection from within the Emps data control to the Panel Page node in the Structure window and drop the collection as an ADF Mobile List View. The Structure window provides an easy to read hierarchical view of the current page. The hierarchical view makes it easier for you to select components and move them around on the page. Because the structure window and editor operate on the same code, the are always in sync.
-
Under List Formats, select Simple, then under Variations, select the variation with text items grouped by dividers. Under Styles, verify that the first style in the list is selected and click OK.
-
In the Edit List View dialog box, set the Divider Mode to First Letter and click OK.
-
Save your work.
-
Just as you did before, double-click the graph view activity on the EmpsTaskFlow.
-
Make sure that all the page facets are selected and click OK.
-
Use the drag bar and split the editor window to show two views. Remember, the drag bar is the small vertical bar to the right of the bottom scroll bar in the editor.
-
In editor, click the Preview tab at the bottom of the right pane to preview the UI.
-
In the code section, select the commandButton in the primary facet and in the Property Inspector, set the Text to Back and the Action to __back.
-
Just as you did for the empList page, drag the emps collection from within the Emps data control to the Panel Page node in the Structure window, but this time, drop it as an ADF Mobile Chart.
-
In the Component Gallery, select Bar and the first Quick Start Layout, and click OK.
-
In the Create Mobile Bar Chart wizard, drag salary to the Bars field and then drag name to the X Axis field. Click OK.
-
Save your work.
-
In Application Resources, expand Descriptors > ADF META-INF and navigate to adfmf-application.xml.
-
Double-click adfmf-application.xml to open it in the editor view.
-
On the Application tab, notice that you can specify the application name and set application menu properties. Click the Feature References tab to see the features that are part of this application.
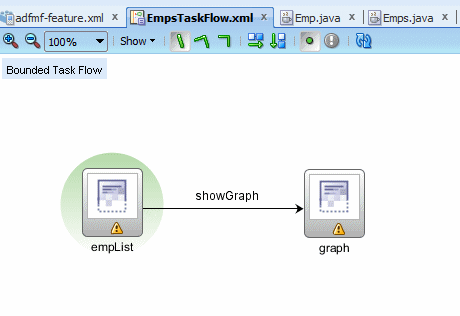
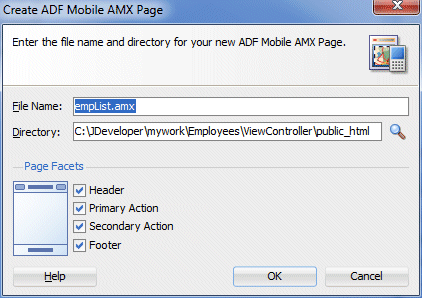
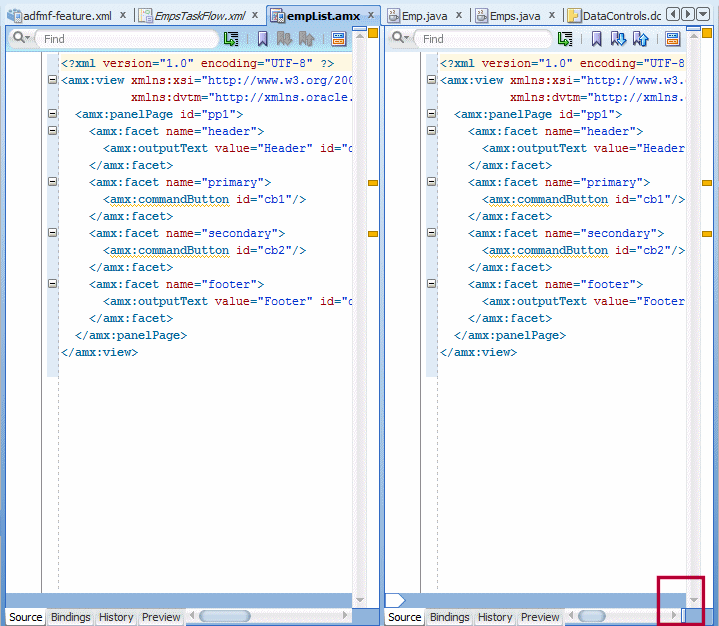
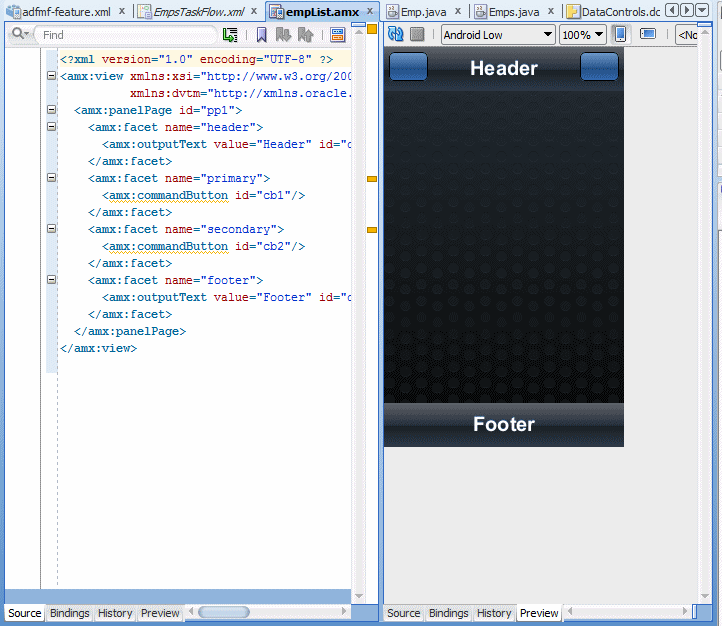
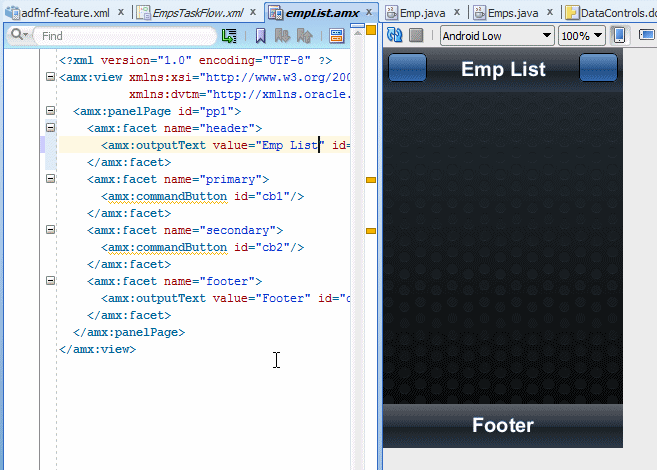
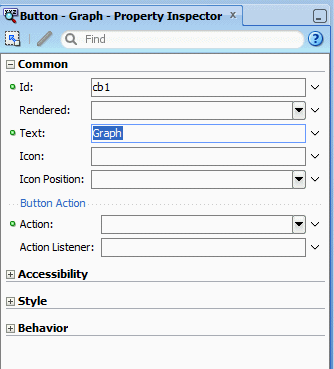
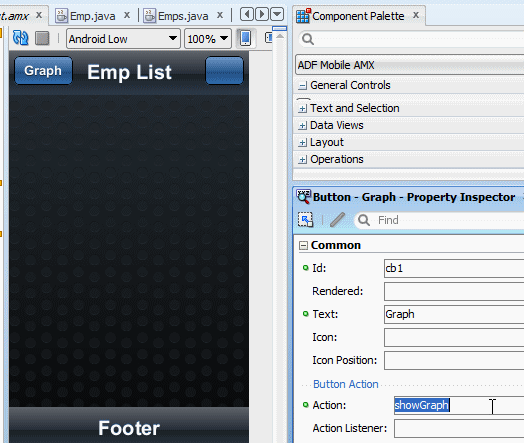
In the next few steps, you add data components to the page from the data controls you created earlier.
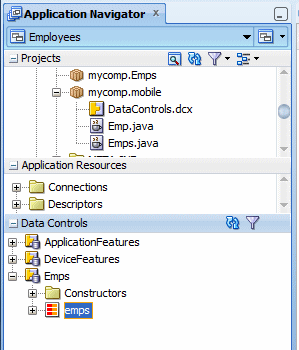
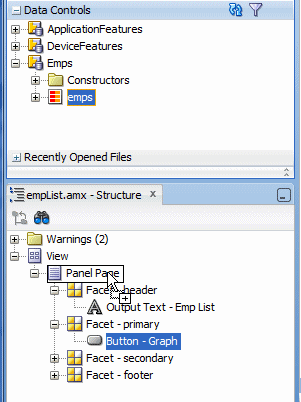
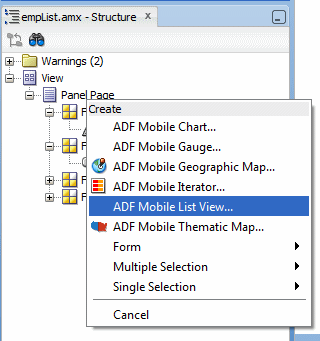
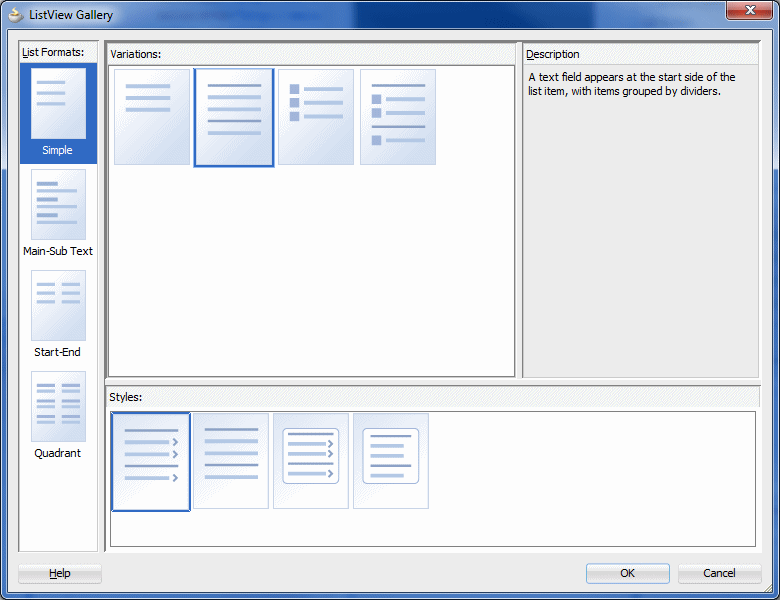
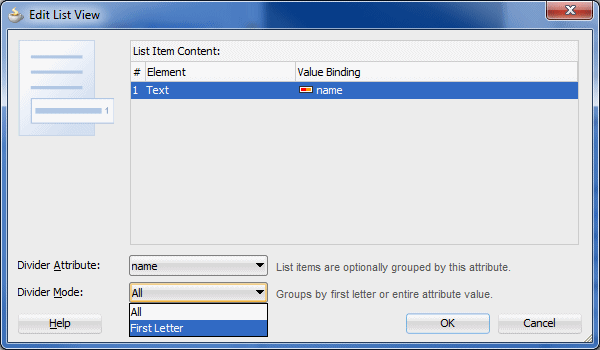
That finishes the first page for now. Next, you create the page that will house the graph.
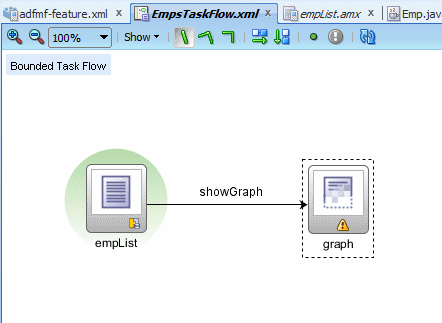
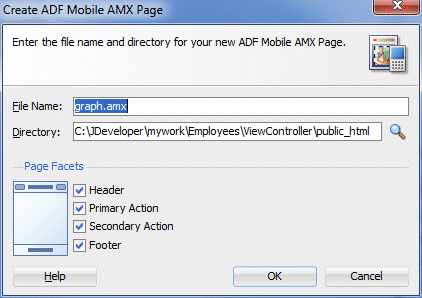
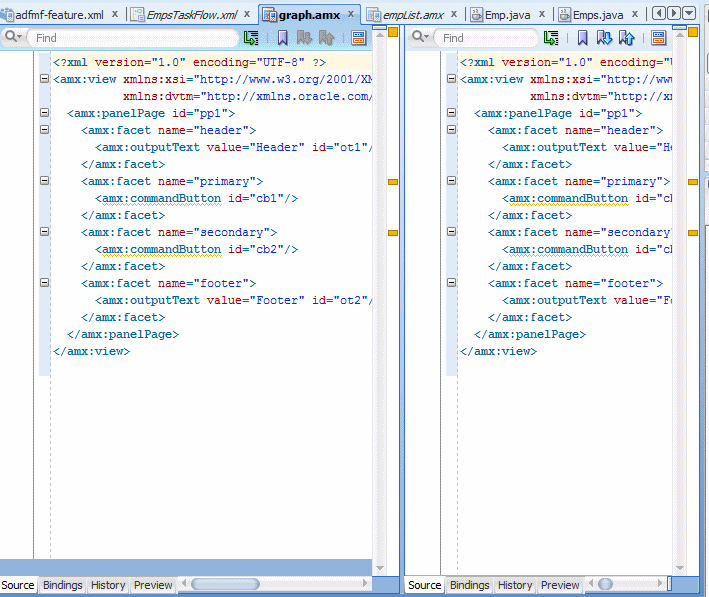
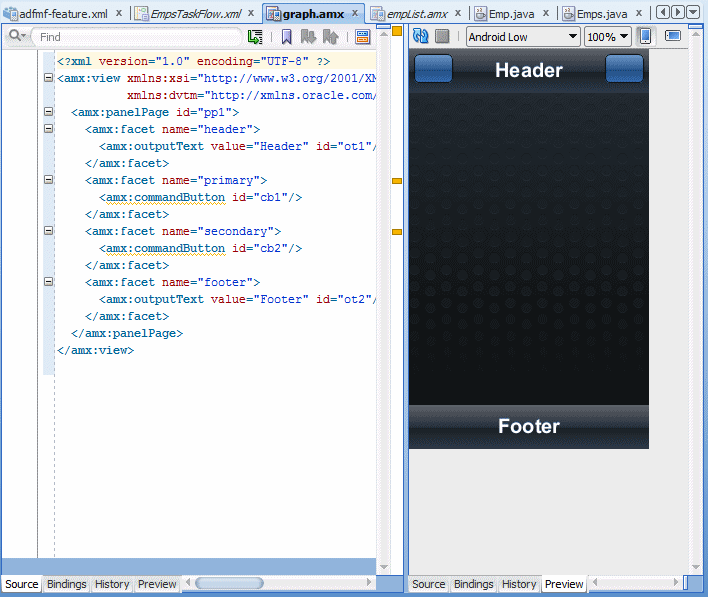
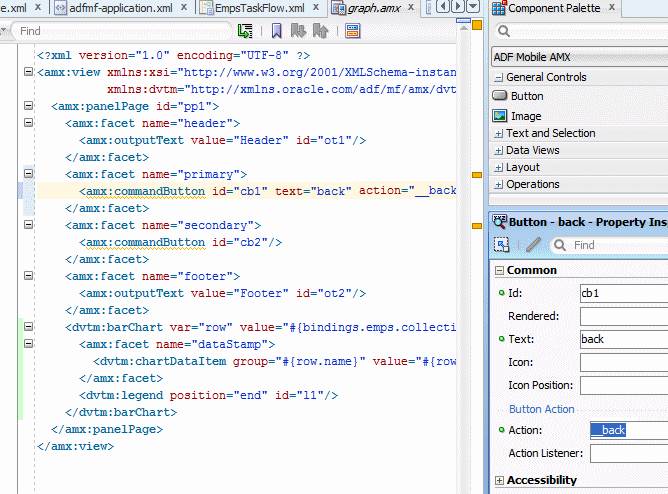
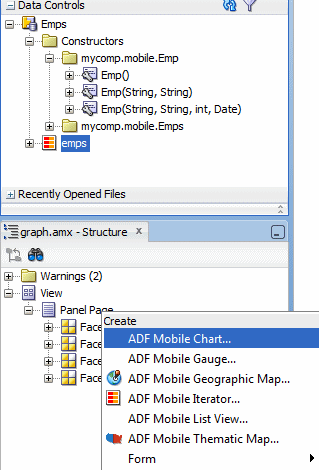
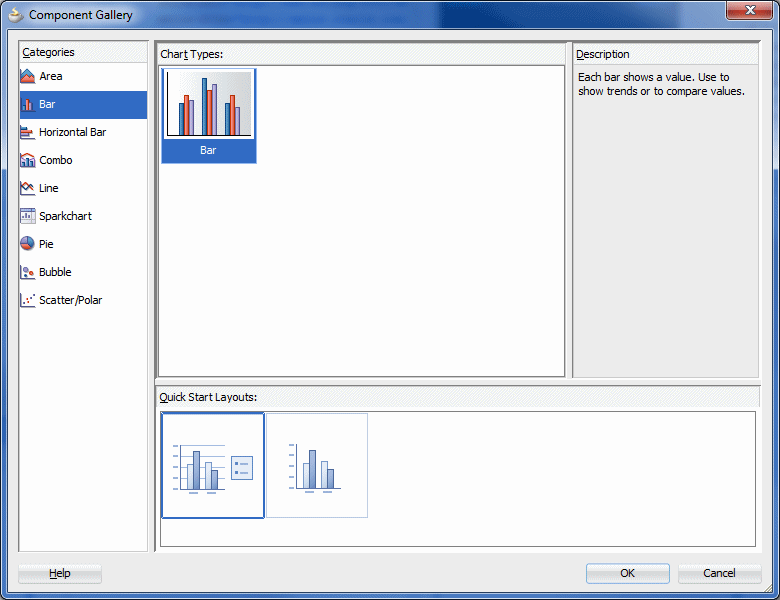
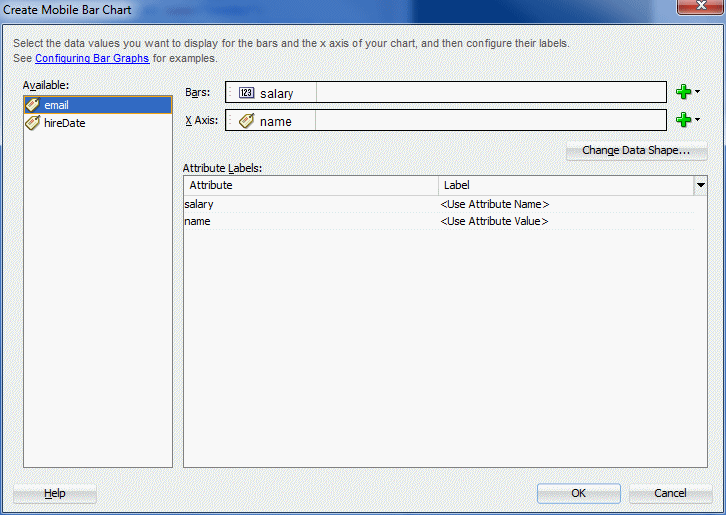
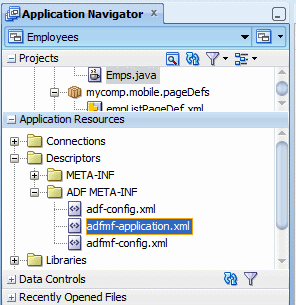
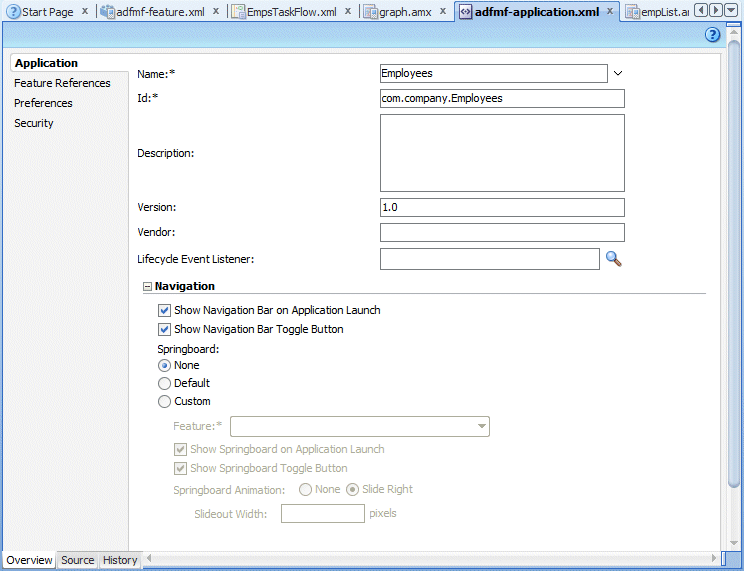
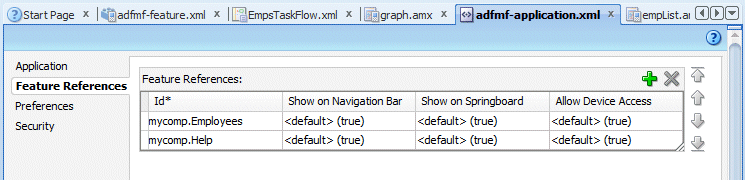
You now have a working application. In the next section you deploy the application, and then you test it.
When you deploy an ADF Mobile application, you can improve performance by using Release mode. This mode causes the application to be deployed with an optimized JVM and minimal JavaScript libraries, thereby significantly improving the performance of the application.
For iOS, you select Release mode when you create the deployment profile, which you will do shortly. If you are using an iOS environment, you can skip these steps and continue at step 8 (beginning of deployment steps.)
For Android, you have to create a local keystore before you can use Release mode. The next few steps set up the keystore for Android deployment.
-
Open a command window. Navigate to the JDK\bin directory used by JDeveloper. If you are not sure where to find this directory, use Help > About > Properties > java.home to see the JDK or JRE that JDeveloper is using. For Windows 7 make sure you open the command window as Administrator. To open the command window as Administrator Click Start, in the Start Search box, type cmd and then press CTRL + SHIFT + ENTER.
-
Enter the following command but substitute a name of your choosing for the Keystore Name and Alias Name. In the screenshot below, we used employees for both. After you type the command, press enter. NOTE: You will need to type the command into the a command window instead of using copy and paste.
keytool –genkey –v –keystore <Keystore Name>.keystore –alias <Alias Name> -keyalg RSA –keysize 2048 –validity 10000
-
You will be prompted for several values including your name, city, State, and so on. Enter values for those variables.
-
Enter a password as the last prompt:
-
In the JDeveloper menu, select Tools > Preferences.
-
Expand ADF Mobile and select Platforms. In the Signing Credentials section, click the Release tab.
-
In the Keystore Location property, enter the location of the keystore you just created. Enter the Keystore Password, Key Alias,and Key Password and then click OK.
-
From the Application menu, select Deploy > New Deployment Profile.
-
Select ADF Mobile for Android or ADF Mobile for iOS and click OK.
-
Click Android Options or iOS options, select Release for the Build Mode, and click OK.
-
To deploy the application, from the Application menu, select Deploy > ADROID_MOBILE_NATIVE_archive1.
-
Select Deploy application to emulator and click Next.
-
Click Finish to begin the deployment.
-
In the Log window, click the Deployment tab to see the deployment messages.
-
After the deployment is complete, switch windows to the Android emulator.
-
Click the Apps icon at the bottom middle of the emulator screen. This will show the Applications that are deployed to the device, or emulator.
-
In the Apps page, click the Employees application to open the application you just built and deployed. Notice the Emp List which shows all of the employees from your data source. NOTE: The emulator may be slower than an actual device and it may take a while for the application to load.
-
Click the Graph button to navigate to the Graph page.
When the command finishes, the keystore is ready to use. Next you configure JDeveloper to use the keystore.
Now that JDeveloper knows about the keystore, you can use Release mode in the deployment profiles.
In the next few steps, you create a deployment profile.
Remember that before you can deploy the application, you need to have started the emulator. Instructions are in the the tutorial Set Up and Configure an Android Environment.
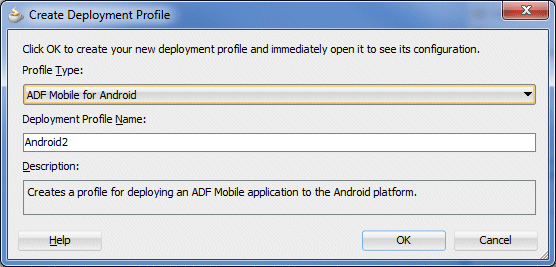
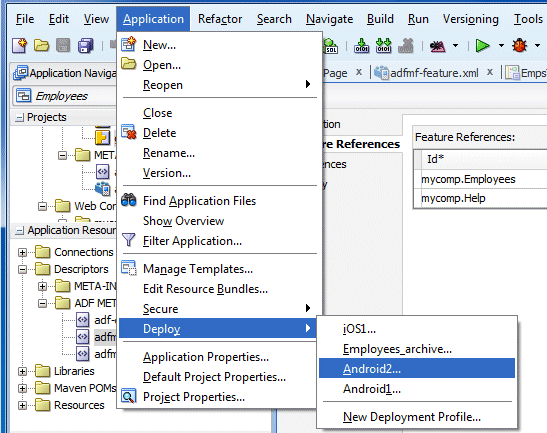
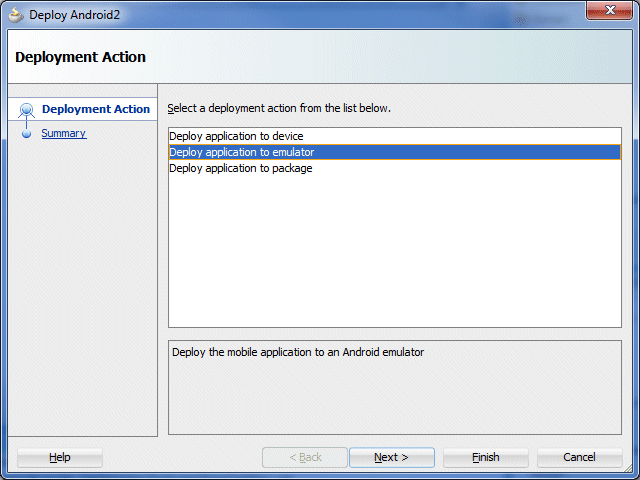
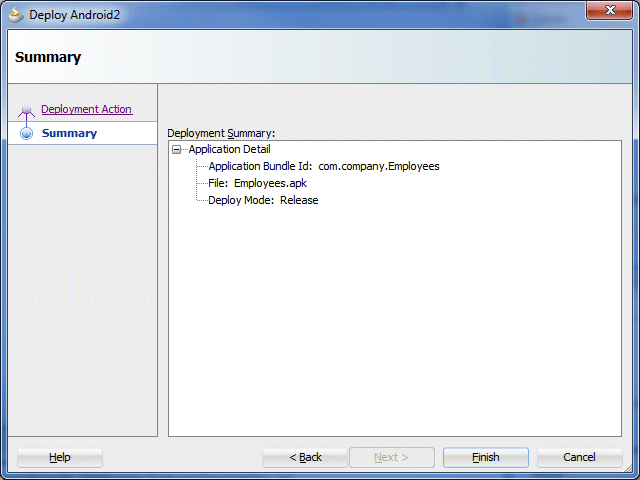
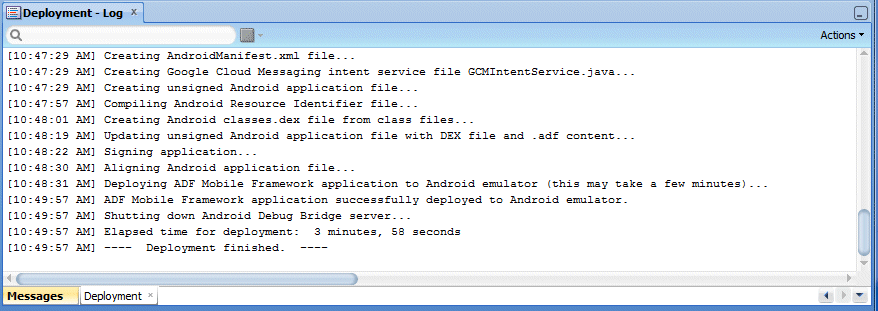
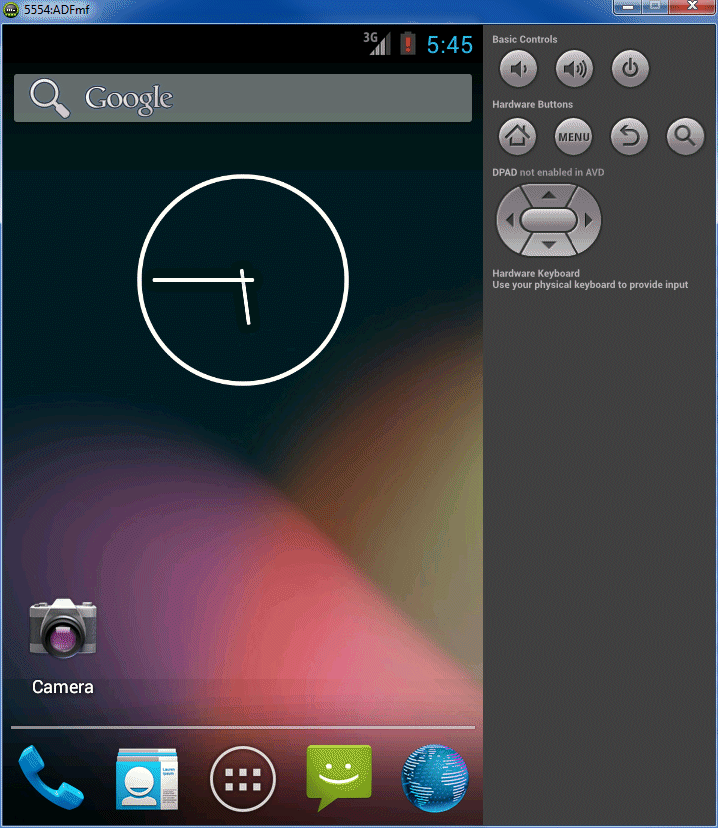
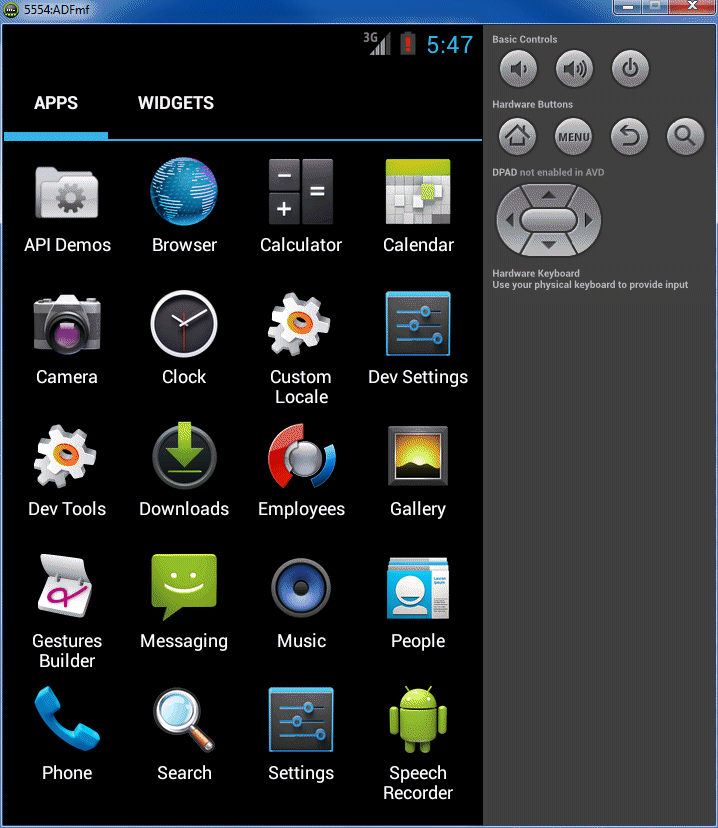
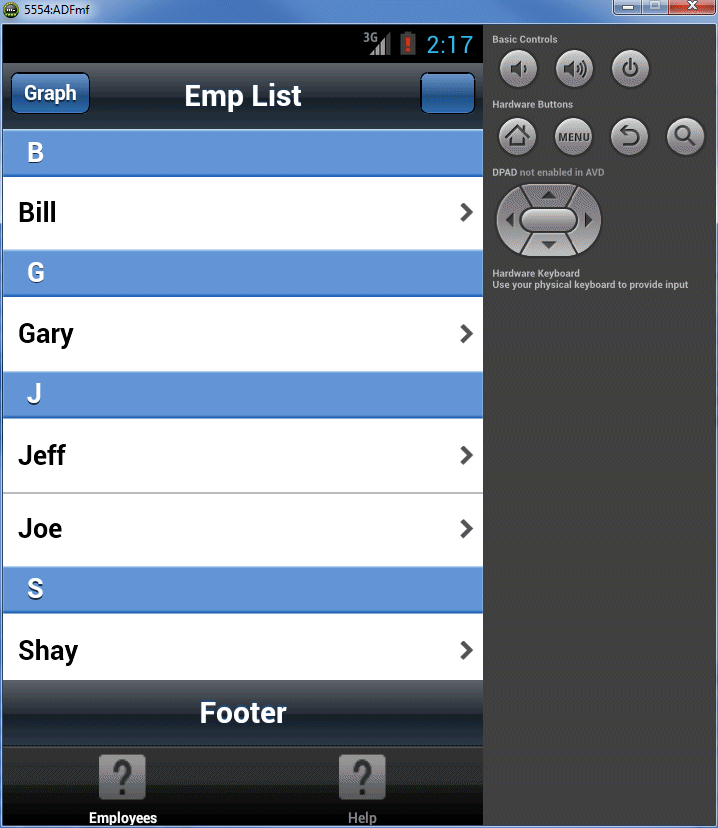
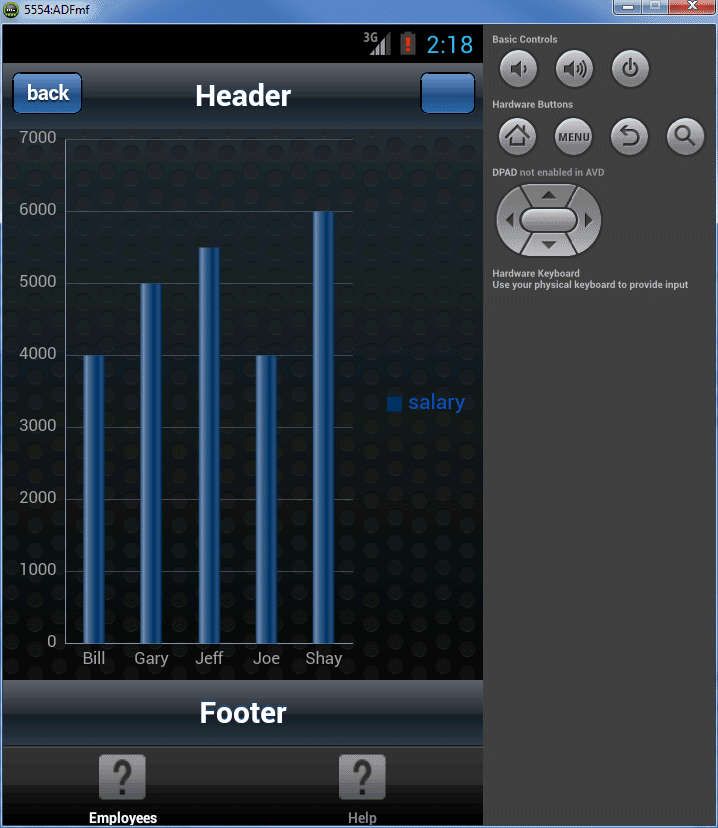
You now have a working ADF Mobile application that you have deployed to an Android emulator. In the next part of this tutorial, you modify the UI.
Now that you have a working application, you refine the user interface to take advantage of some of the ADF Mobile layout components.
In the next few steps, you move the list of employees to a navigator pane. You also add a read-only form that shows employee details that you did not show in the employee list. As you recall, the employee list shows only the employee name. You also split the page in to two parts: an employee list, and an employee form.
-
In JDeveloper open the empList.amx page. (HINT: Either find the page in the Application Navigator and double-click it, or use the EmpsTaskFlow and double-click the empList view component)
-
From the Layout section of the Component Palette, drag a Panel Splitter component to the Panel Page in the Structure window.
-
In the Structure window, expand the Panel Splitter to expose the Facet - navigator and the Panel Item component.
-
You want the list to display as part of the new Panel Splitter so within the Structure window, drag the List View component down to the Facet - navigator.
-
From the Data Controls palette, drag the emps data control to the the Panel Item in the Structure window and drop it as an ADF Read Only Form.
-
Click OK to accept the default labels and components for the form.
-
In the Structure window, expand the List View node to show the List Item component.
-
From the Operations section of the Component Palette, drag a Set Property Listener component to the List Item component in the Structure window.
-
The Set Property Listener has three properties, From, To, and Type. Select the Set Property Listener component and set the From parameter to #{row.rowKey}. Set the To parameter to #{pageFlowScope.empId}. Set the Type to action. The expression row.rowKey returns the id of the current row. The pageFlowScope is a predefined managed bean where you can store values. In this case you are storing the Id of the current row as the variable empId. Setting the Type to action specifies that when the user selects an item from the list, the current row Id is stored in pageFlowScope.empId.
-
Expand Emps > Operations in the Data Controls palette to show all of the available operations.
-
Drag the setCurrentRowWithKey operation to the List Item in the Structure window and drop the operation as an ADF Mobile Button.
-
Under Parameters in the Edit Action Binding dialog box, set the Value of the Parameter to the same value you used as the To value on the Set Property Listener, which was #{pageFlowScope.empId}.
-
Find the button component in the code editor.
-
Select the actionListener code and either drag it to the listItem component above, or copy and paste it into the listItem.
-
Because you don't want the button, delete the commandButton.
-
Save your work
-
From the Application menu in JDeveloper, select Application > Deploy > ANDROID_MOBILE_NATIVE_archive1 to Android emulator.
-
After the application is deployed, run it as you did before and notice that the page shows employee details and that there is an arrow on the left side of the page.
-
Click the arrow to expand the splitter and show the employee list.
-
Click any other employee to show that employee's details.
-
The page flips back to show the employee details.
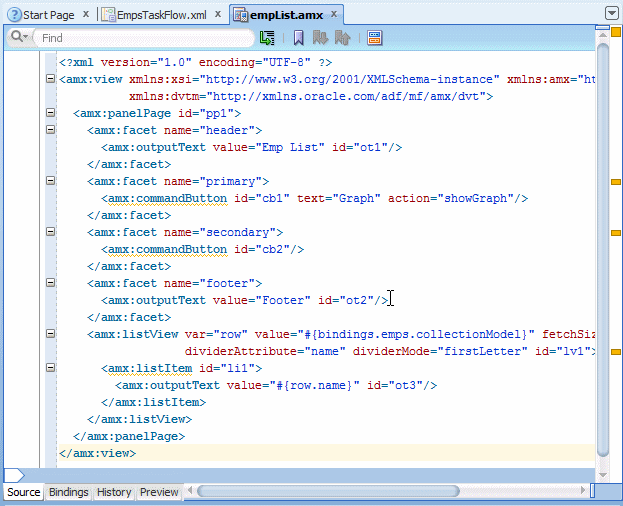
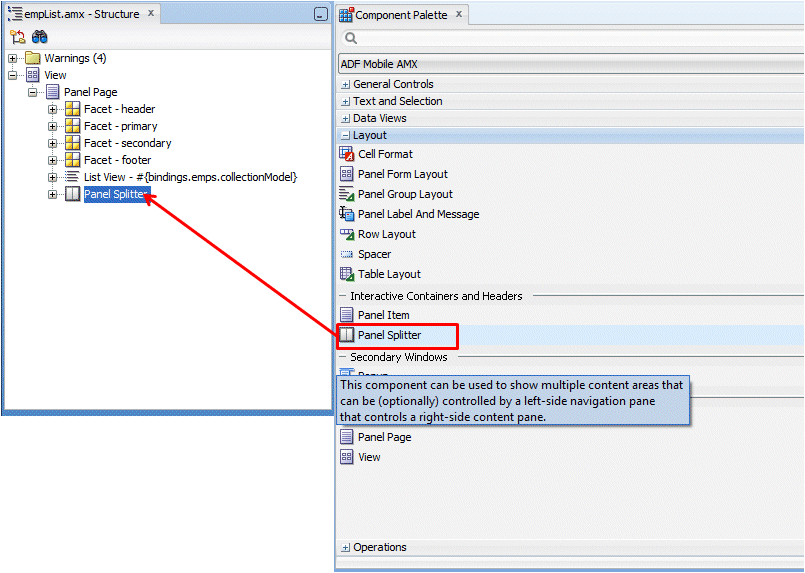
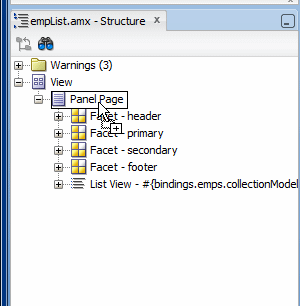
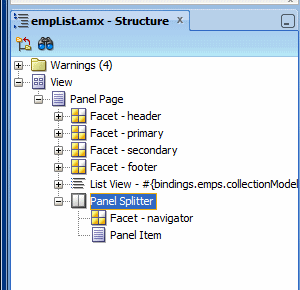
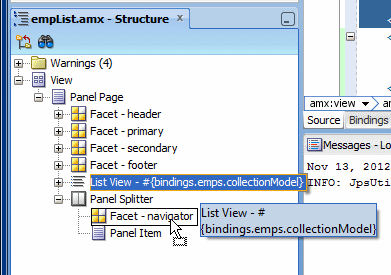
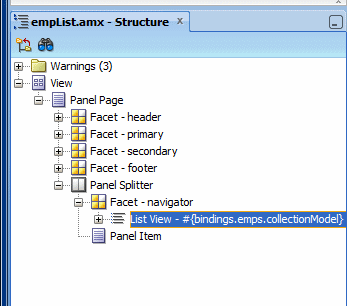
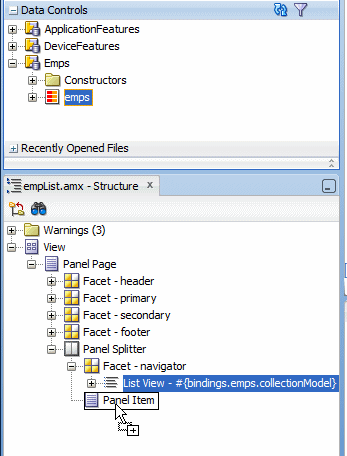
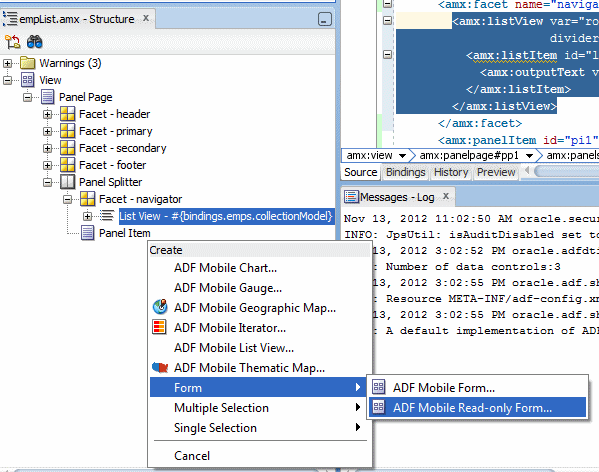
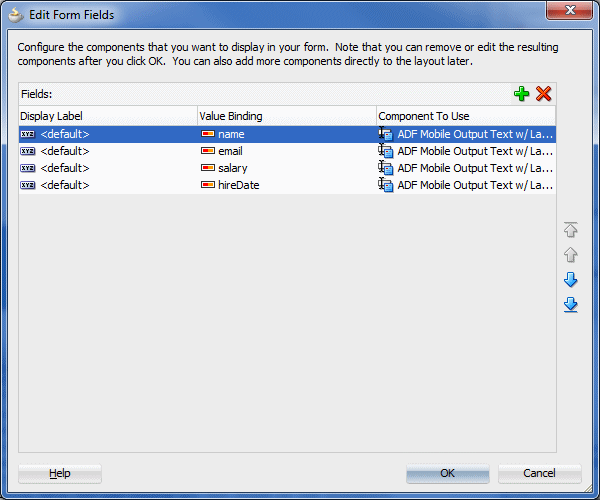
Now you have two sections on the page: the employee list and the employee form. At run time, you want to make sure that whatever employee the user selects is displayed on the form. In the next few steps, you add a component to keep track of that.
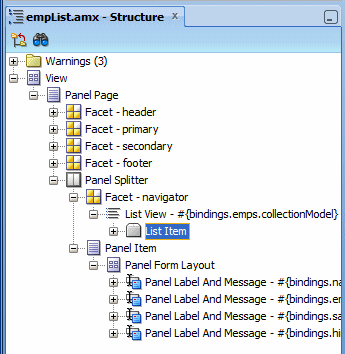
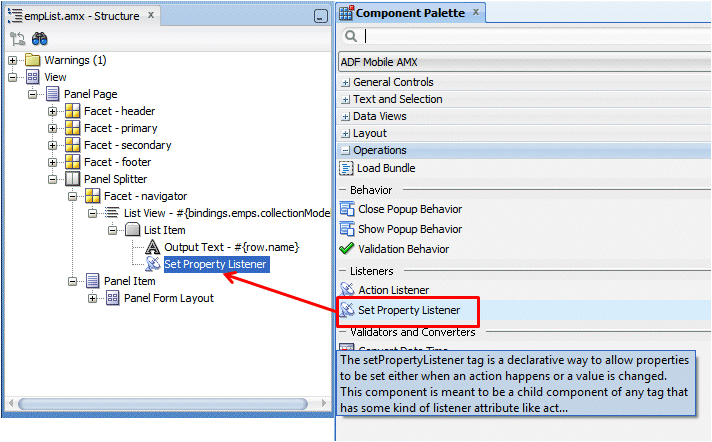
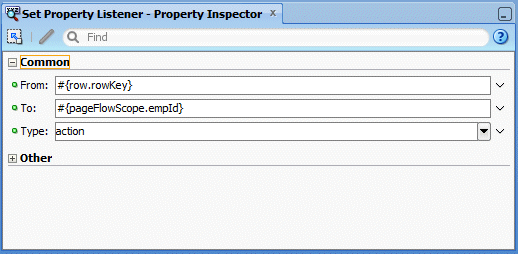
Next, you are going to add an operation from the Emps data control that will accept a parameter and set the current row of the iterator to the row that the user selects.
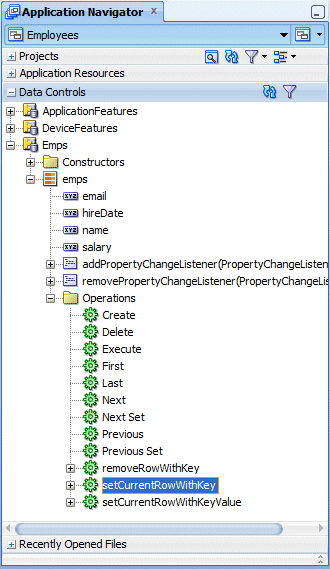
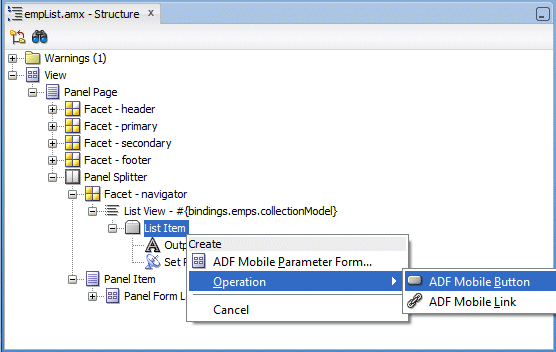
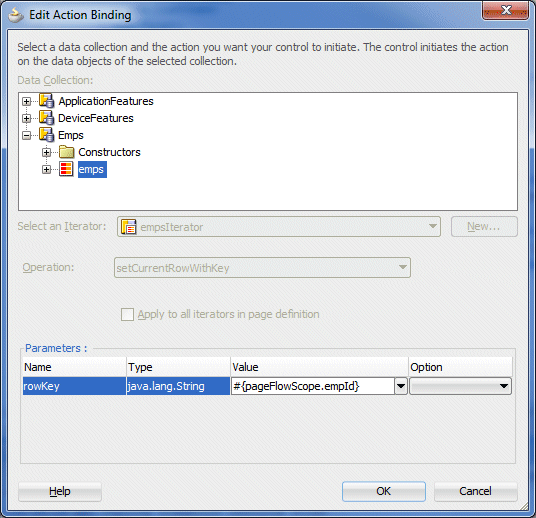
Two things happen when you add the button: First, JDeveloper adds the button to the Emp List, and then it creates an action listener that will set the current row key when the button is clicked. In reality, a button displayed within the list is not the look you really want. Instead you want the current row key to be captured and stored whenever a row is selected.
In the next few steps, you copy the code from the button, add it to the listItem, and remove the button.
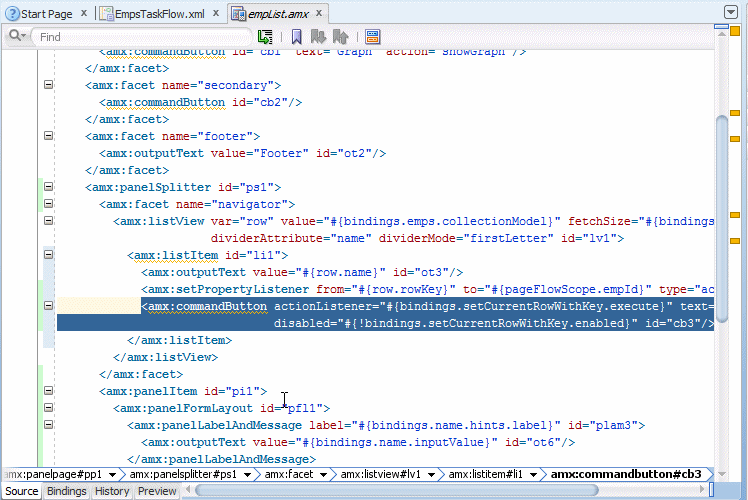
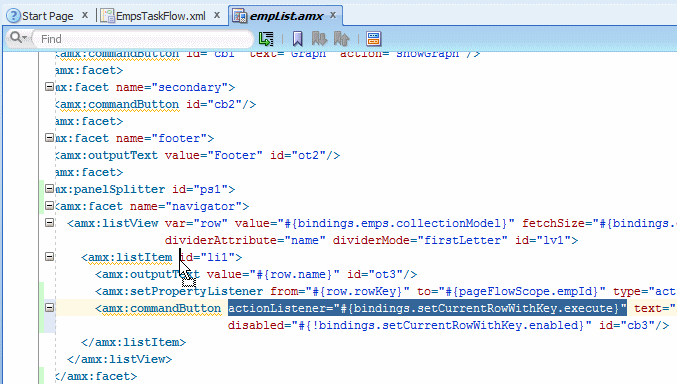
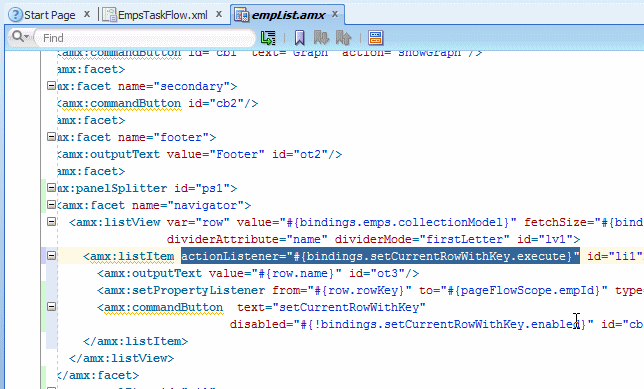
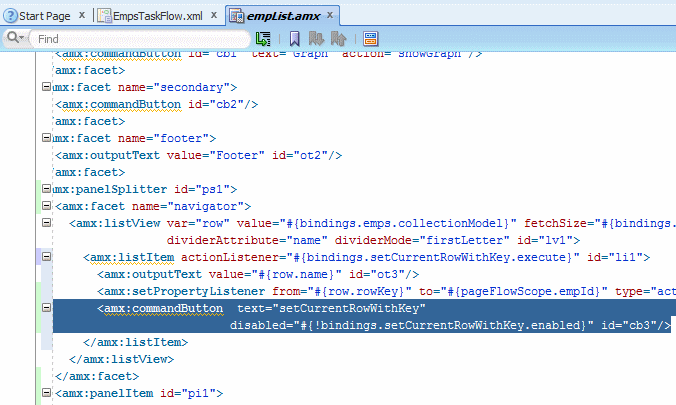
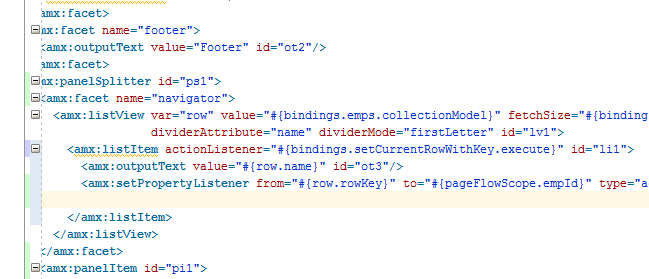
Now you can deploy the application to show your work.
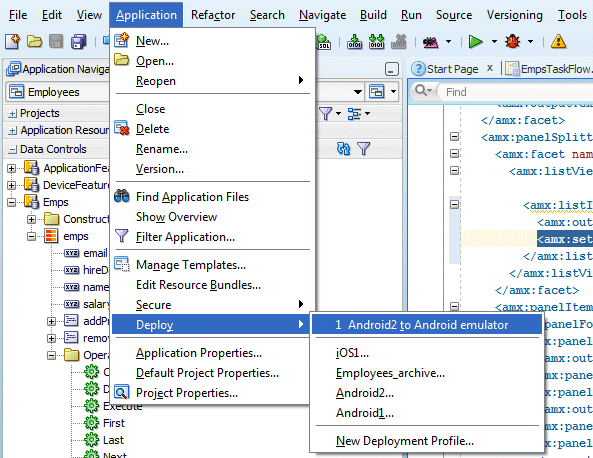
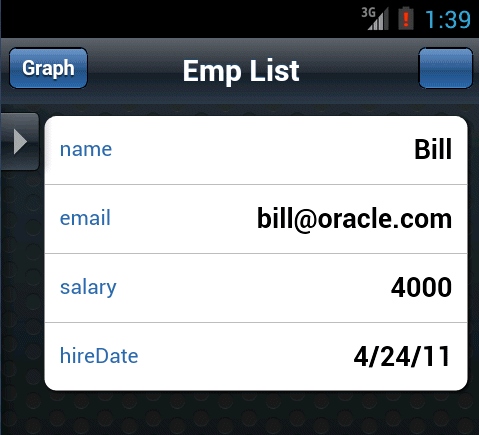
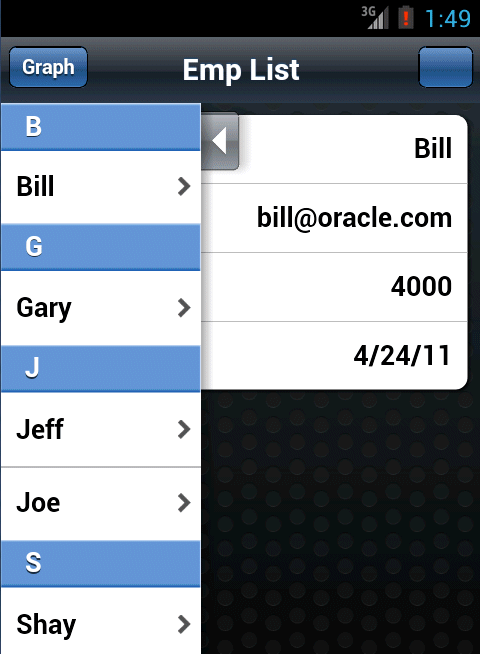
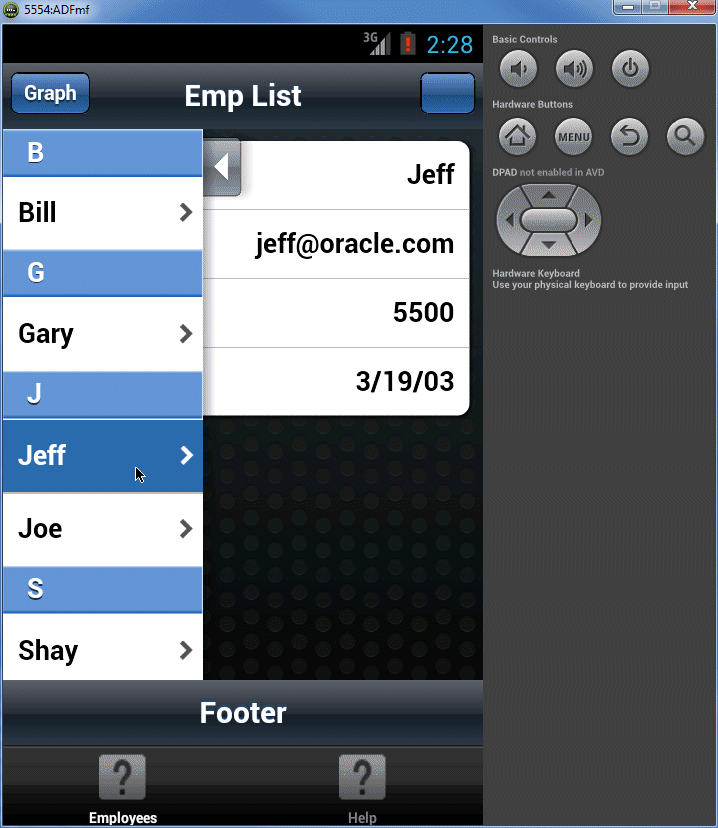
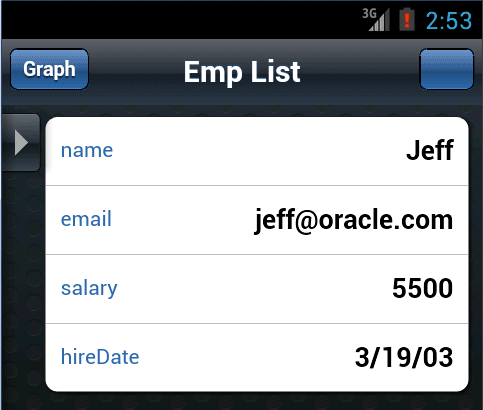
You have now completed the first part of this tutorial. In the next part, you add a call to a web service that will update the salary of an employee.

