Step 3. Create and Manage Entities
Time to complete this step: 30 minutes
The JPA ORM Generation wizard allows modification of existing O/R mappings and
the creation of new entity associations.
The tasks you will complete in this step are:
To Create a New One-to-One Association between CUSTOMER and CUSTOMERID
Workshop also supports the creation of new entity associations in case your
database lacks foreign key definitions (such as for performance reasons). It
can create Simple Associations (one to one, one to many, many to one) between
two tables and Many to Many Associations through an intermediate table.
- In the DbXplorer view, right-click SalesDBConnection and
select Generate
JPA Mapping.
- In the Web Application dialog, select workshop-jpa-tutorial and
click OK.
- In the JPA ORM Generation dialog, select all the database
tables except CONTACT and click Next.
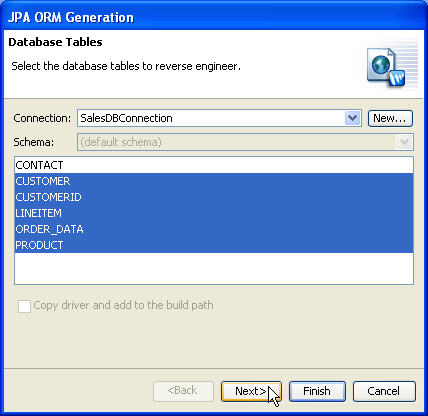
- In the JPA ORM Generation dialog, click the Create New Association link.
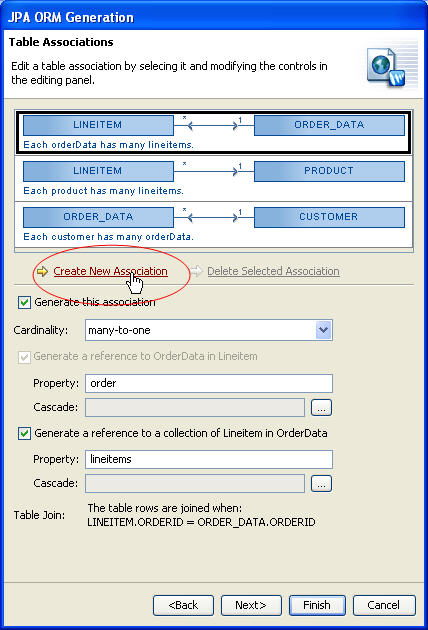
- In the Create New Association dialog, using the Table
1 drop down menu, select
CUSTOMER.
Using the Table 2 drop down menu, select CUSTOMERID.
Click Next.
This creates a simple one-to-one association between the CUSTOMER and
CUSTOMERID tables.
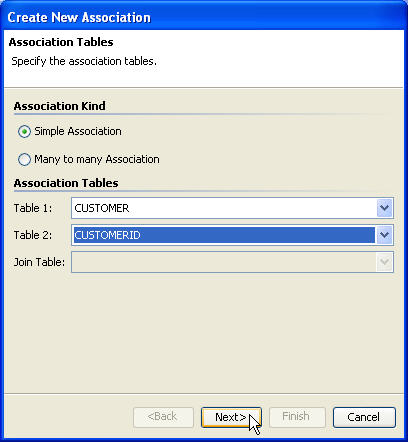
- Next we specify the join columns between the tables.
Using the CUSTOMER table drop down menu, select the CUSTOMERID column.
Using the
CUSTOMERID table drop down menu, select the CUSTOMERID column.
Click Next.
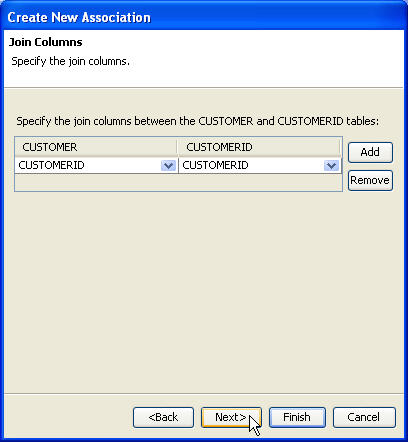
- Select One-to-One to specify one customer per customerid.
Click Finish.
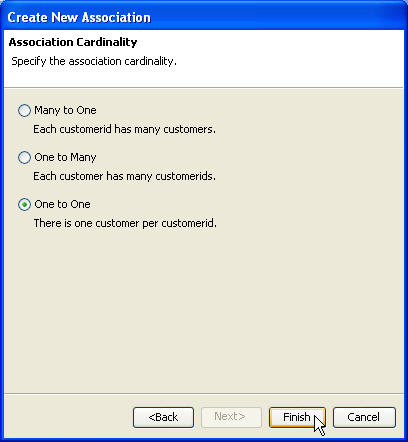
Since the new associations (shown in brown)
are not observed in the database schema, they will be annotated programmatically
in the respective entity beans.
- Click Next.
- If necessary, on the Default Table Generation page, next
to the Java
Package field, click Browse. In the Choose
Java Package dialog, select com.bea.beans and
click OK.
- On the Default Table Generation page, click Finish.
To Review Generated Classes with Annotations
Review the following annotations in the class Customer and CustomerId.
- One-to-One Properties - A one-to-one property designates a relationship
in which an entity A references a single entity B, and no other As can
reference the same B. This is a one-to-one relationship between A and B.
The following shows the one-to-one mapping association between Customer
and CustomerId in the Customer class.
@OneToOne(fetch=FetchType.EAGER)
@JoinColumn(name="CUSTOMERID", referencedColumnName="CUSTOMERID",
nullable=false, insertable=false, updatable=false)
public Customerid getCustomeridBean() {
return this.customeridBean;
}
public void setCustomeridBean(Customerid customeridBean) {
this.customeridBean = customeridBean;
}
|
- The following shows the one-to-one mapping association between CustomerId
and Customer in the CustomerId class.
@OneToOne(mappedBy="customeridBean", fetch=FetchType.EAGER)
public Customer getCustomer() {
return this.customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
}
|
Note: The annotation attributes for one-to-one mappings are similar to
one-to-many and many-to-one annotation attributes as explained before in Step 2 of this tutorial: Review Generated Classes with
Annotations.
To Add Annotations to an Existing Java Class
In this step, you will create a Java class Contact
and use Workshop to annotate existing Java classes (POJOs) to make them into
JPA entity beans. This way, we will follow a "top-down"
development scenario.
- Select File > New > Class.
- In the Package field, enter com.bea.beans.
In the Name field, enter Contact.
In the Interfaces field, enter java.io.Serializable.
(Click the Add button to fill in this field.)
Select Constructors from superclass to add a no argument
constructor.
Click Finish.
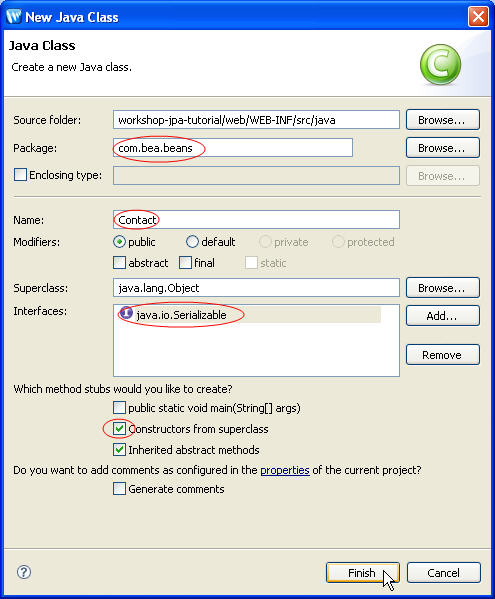
- Add the following set of private variables of type String
to the Contact class. Note: We are defining properties that
map to columns in the CONTACT database table.
private String contactId;
private String address;
private String city;
private String phone; |
- Add getter and setter methods for each property by right-clicking within
the source view
and selecting Source > Generate Getters and Setters. In
the Generate Getters and Setters dialog, click Select
All and click OK.
- Add the following property.
private static final long serialVersionUID = 1L;
|
- Override the equals( ) and hashCode( ) methods
as we are going to use Contact instances in Set.
public boolean equals(Object other) { if
( (this == other ) ) return true; if ( !(other instanceof
Contact) ) return false; Contact castOther = (Contact)
other; if( this.getContactId( ).equals(castOther.getContactId(
)) ) { return true; } else
{ return false; }
}
public int hashCode() { return this.getContactId( ).hashCode(
);
} |
- Save the Contact class. Now, we have the object representation
for CONTACT persistence data. Next, we will annotate the Contact
object.
- In the AppXplorer view, right-click on the project workshop-jpa-tutorial.
Choose New > Other. Expand JPA
and select Annotate Java Class, then click Next.
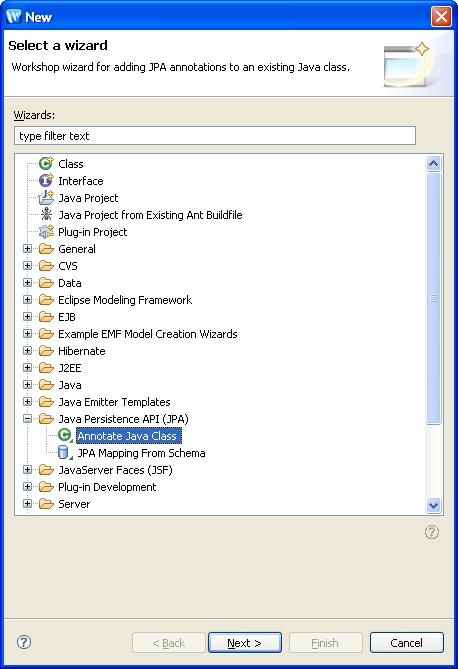
- Click Browse to select a Java Class. Type contact to view and select the
com.bea.beans.Contact object to be mapped.
Click OK.
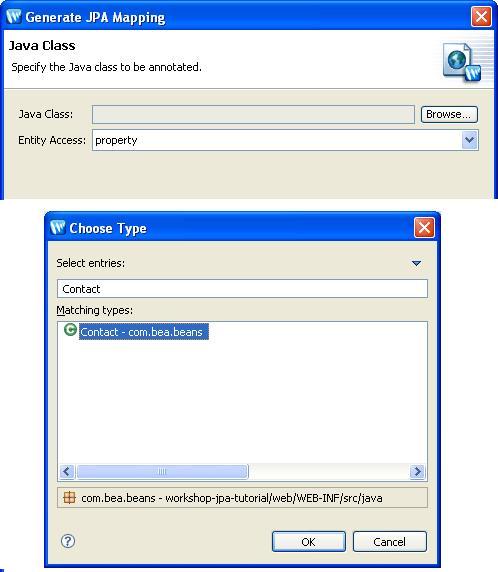
- In the Entity Access drop-down list, choose property and
click Next. (Note by choosing property,
you are instructing Workshop to annotate your class's accessors; if you
choose field,
Workshop annotates your class's fields.)
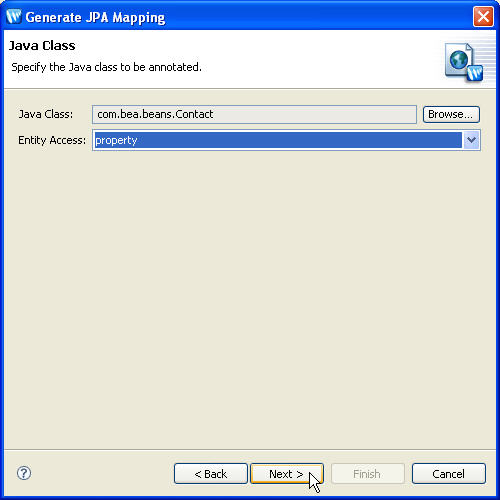
- In the Class Mapping Properties dialog, confirm that
the Database Table field has the value CONTACT and the Primary
Key Property field has the value contactId.
Click Next.
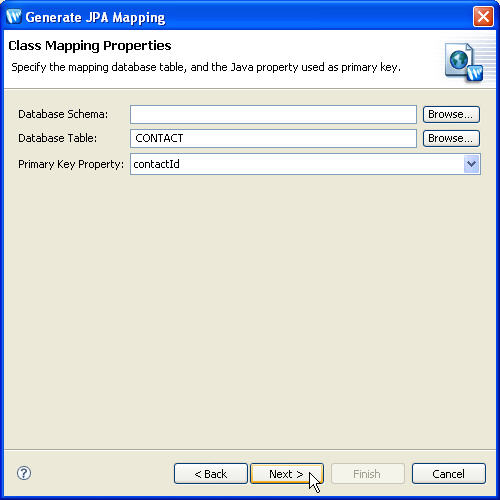
-
In the Bean Properties Mapping dialog, you can verify the mappings
between fields and database columns that are being
annotated in the Contact java class. Note that you can select a property mapping and click Edit to modify a selected property
mapping. In this tutorial, we do not need to change any mapping information.
Click Finish.
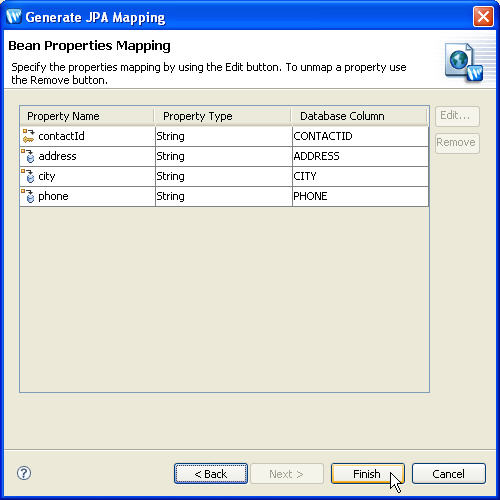
- Edit the annotated class Contact to add a bi-directional many-to-one mapping
association with Customer.
- Import the following javax.persistence classes for adding a new many-to-one
column join.
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne; |
- Add a property customer of type Customer.
private Customer customer; |
- Add the following annotation to the Contact entity for adding a many-to-one
association with Customer. The @JoinColumn annotation defines the attributes
Column Name which is the name of the column to which the property is bound
and the Referenced column which is the name of the primary key column
being joined to.
@ManyToOne()
@JoinColumn(name="CUSTOMERID", referencedColumnName="CUSTOMERID")
public Customer getCustomer() {
return this.customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
} |
- For the above many-to-one customer property in the Contact entity there
should be a one-to-many contact property in the Customer entity.
- Open the class Customer.
- Add a property contacts of type java.util.Set
private java.util.Set<Contact> contacts;
|
- Add the following annotation to the Customer entity for adding a one-to-many
association with Contact. The @OnetoMany annotation defines the attribute
MappedBy which is the name of the many-to-one field in the Contact entity
that maps this bidirectional relation.
@OneToMany(mappedBy="customer")
public java.util.Set<Contact> getContacts() {
return this.contacts;
}
public void setContacts(java.util.Set<Contact> contacts) {
this.contacts = contacts;
} |
- Save the class Customer.
To Run EJBQL to Verify that the New Mappings are Correct and Function Properly
In this step we have created a new association between Customer and CustomerId
Entities using the JPA ORM Mapping tool. Also we have annotated an existing
Java class Contact to make it into a JPA entity bean. Use the EJBQL editor to
test the runtime behaviors.
- Test the bi-directional one-to-one mapping between Customer
and CustomerId. Execute the following query in the EJBQL
Editor:
select c from Customer c where c.customeridBean.customerid = 101
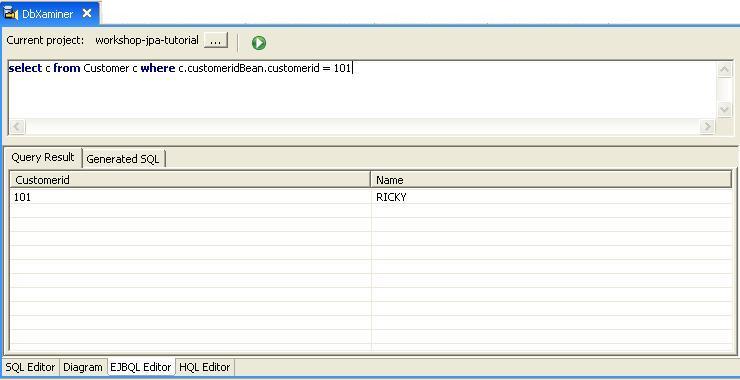
- Test the bi-directional one-to-one mapping between CustomerId
and Customer. Execute the following query in the EJBQL Editor:
select c from Customerid c where c.customer.customerid = 101
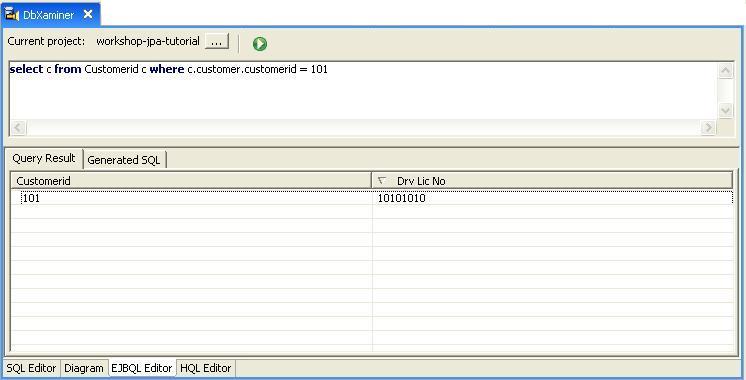
- Test bi-directional many-to-one mapping between Contact
and Customer. Execute the following query in the EJBQL Editor:
select c from Contact c where c.customer.customerid = 101
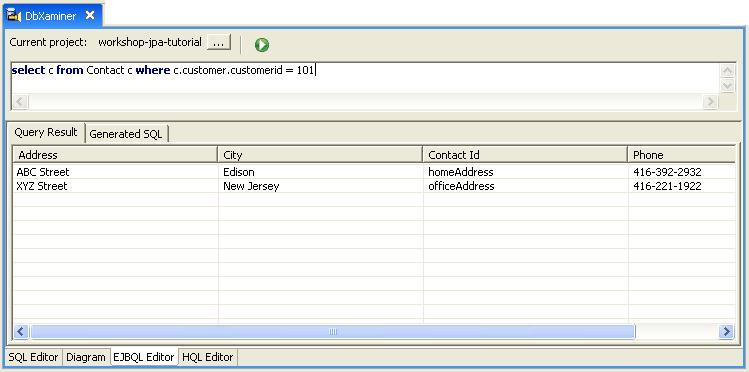
Click one of the following arrows to navigate through the tutorial:
Still need help? Post a question on the Workshop
newsgroup.