Web Services Development: Starting Points for JAX-WS
This document describes the following starting points of developing JAX-WS-enabled Web services:
- Generating a Web Service from a WSDL File
- Customizing a Web Service
- Using the Customizations Editor
- Generating a Web Service from Java
- Starting from a Java Class
- Starting from Scratch
- Generating a WSDL File by Starting from a Java Class
- Related Information
- Contents of a WSDL File
- Imported WSDL Files
- Creating a new WSDL File
- Testing Web Services
1. Generating a Web Service From a WSDL File
Using OEPE, you can generate a Web service from a WSDL file. The resulting Web
service class will contain the public endpoint interface described by the
WSDL without the implementation. After
the Web service has been generated, you have to fill in the Web service
implementation details.
To generate a Web service from a WSDL, follow this procedure:
You can test your Web service using the Test Client. For more information, see Testing Web Services.
You can also customize your Web service.
1.1 Customizing a Web Service
You can customize your Web Service using the JAX-WS bindings file that points to an appropriate WSDL file.
A JAX-WS bindings file lets you change the shape of the Java Web service artifacts generated through WSDLC. The bindings allow you to modify class and method names, method signatures (wrapper versus non-wrapper), add Javadoc, and attach handlers.
Sources of information on the bindings file specifications with examples can be found in the references section.
To create a bindings file, use the JAX-WS Bindings File wizard as follows:
-
Right-click in the Project Explorer and select New > Other from the drop-down menu. This opens the New dialog that lets you select a wizard to use.
-
Select WebLogic Web Services > JAX-WS Bindings Customization, as Figure 2 shows, and then click Next.
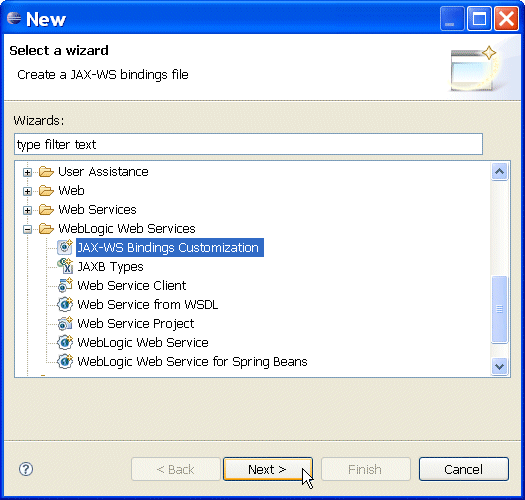
Figure 2. New WebLogic Web Services - JAX-WS Bindings Customization Selection
This opens the New JAX-WS Bindings File wizard, as Figure 3 shows.
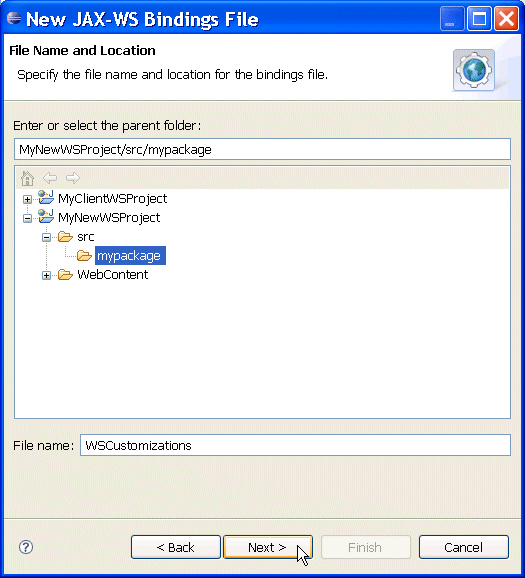
Figure 3. New JAX-WS Bindings File Wizard
-
Specify the name and location for your bindings file, and click Next. This opens the New JAX-WS Bindings File > dialog, as Figure 4 shows. Select the file from the list of WSDL files available in the project in which the bindings file will be generated.
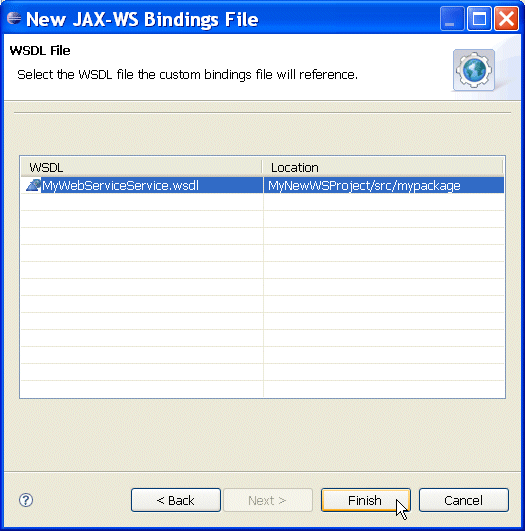
Figure 4. New JAX-WS Bindings File - WSDL File
- Click Finish on the New JAX-WS Bindings File dialog to complete generation of the bindings file in the specified location.
Upon the completion, the New JAX-WS Bindings File wizard opens the created bindings file in an appropriate editor. The contents of the file is a basic bindings file shell with a wsdlLocation element pointing to the identified WSDL file, as the following example shows:
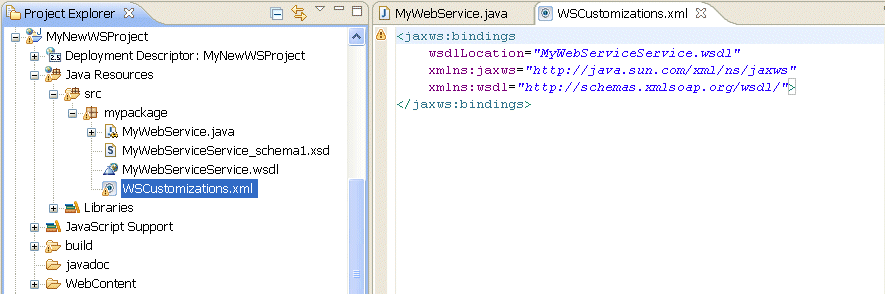
Once created, you can customize the bindings file using the customizations editor.
1.1.1 Using the Customizations Editor
The customizations editor lets you define the contents of a JAX-WS external bindings file (not embedded in the WSLD file), while providing information on the possible XML elements within the file. Using the editor, you can specify a variety of customization options based on the shape of the generated Java code. Note that the editor allows you to define both server-side and client-side customization options.
The customizations editor shows which classes, methods, and parameters will be generated when you apply a particular bindings file's customizations.
You can use the customizations editor to modify the following types of files:
- A single WSDL file: the editor supports having all WSDL information defined within a single WSDL file. The editor will not load a secondary WSDL file imported through WSDL import or include mechanisms.
- External bindings files: even though you can define JAX-WS bindings elements either within a WSDL file, or in an external file, the customizations editor only supports external bindings files, ignoring the JAX-WS bindings elements within the WSDL file. Note that, although ignored by the editor, those elements will not be ignored by the artifact generation tooling. Also note that you can use the editor to modify only one external bindings file at one time.
You can use the external bindings file to customize the shape of the generated Web service, customize specifics of the generated Web service client (for example, enable asynchronous clients), and add JAX-WS handlers.
- Schema configurations: JAX-WS uses W3C Schema (XSD) elements to define the JAXB types that will be used by the generated Service Endpoint Interface-based Java Web service. You can include the schema information in the WSDL file either inline, or through different import or include mechanisms. The customization editor supports having WSDL type information defined through inline schemas, as well as external schemas imported using the schema import mechanism.
This example shows how inline types define the JAXB type information in an embedded schema element in the types section of the WSDL file.

This example demonstrates the case of imported schemas, where the customizations editor supports importing schemas directly from the WSDL file using the schema import mechanism. The import element must define a namespace and a schemaLocation attribute. Note that imports in the imported schemas will not be followed.

The customization editor consists of the following parts:
- A tree control on the left - its nodes represent a combination of the WSDL and the Java artifacts that will be generated.
- An edit page on the right - when you select a node in the tree, the editor will display an appropriate edit page where you can edit the properties (supported customization options), as follows:
- The Global node and the corresponding page, shown in Figure 5, let you specify the following settings that apply to the editor in global scope (for example, to all generated Java artifacts):
- WSDL URI - the URI to the WSDL file that this bindings file customizes. Note that, even though the JAX-WS 2.1 specification states that the URI can specify a local WSDL or a remote WSDL file, this editor supports only local WSDL files.
- Package name and JavaDoc - the package name for the generated Java artifacts. A light-gray value in the name setting means that the default package name will be used. This default value is determined by the WSDL file's target namespace. The JavaDoc can only be specified if a package name other than the defaul is specified:
- Wrapper Style (
enableWrapperStyle customization option) - the wrapper style setting for Java method generation. Accepting the default value of true will result in wrapper style method generation only if the rules are satisfied, as defined in section 2.3.1.2 of the JAX-WS 2.1 specification. This value applies to all endpoint interfaces and methods, however, each endpoint interface or method can override this setting.
- Async Mapping (
enableAsyncMapping customization option) - the async mapping setting for asynchronous method generation on the endpoint interfaces. The default value is true . The generated asynchronous methods can only be used on the client side. This value applies to all endpoint interfaces and methods, however, each endpoint interface or method can override this setting. For more information, see section 2.3.4.2 of the JAX-WS 2.1 specification.
- MIME content (
enableMIMEContent customizaition option) - the MIME content setting for the use of the mime:content information. The default value is true . The client- and server-side settings must match. This value applies to all endpoint interfaces and methods, however, each endpoint interface or method can override this setting. For more information, see section 2.6.3.1 of the JAX-WS 2.1 specification.
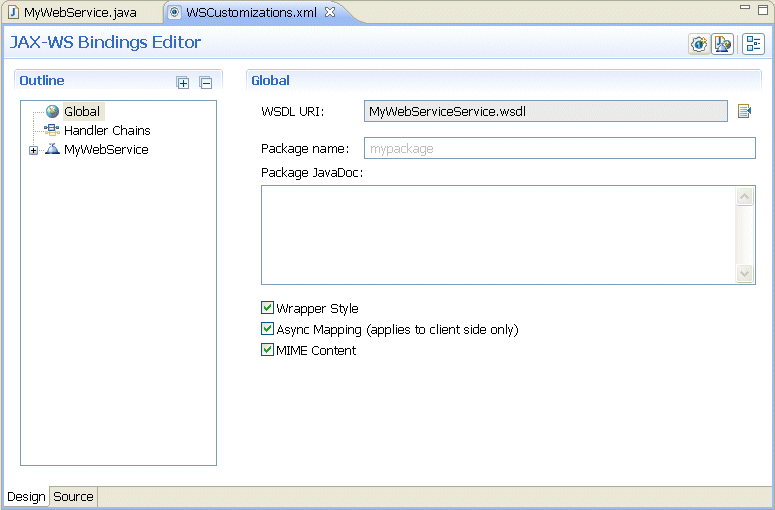
Figure 5. Customizations Editor - Global Node
- The Handler Chains node and the corresponding page, shown in Figure 6, display existing, or let you define new handler chains consisting of either logical handlers, SOAP handlers, or a combination of both types. Note that Handler Chains sections on each page (if applicable) provide the same options. You can specify the following:
- Type - A handler chain can apply to everything (Global), Services, Ports, or Protocol bindings. While this field is not editable in the editor design view, it can be changed in the source view, and the source changes will be reflected in the editor.
- Applies To - the specific pattern (Services, Ports), bindings (Protocol), or global (All) to which this handler chain applies. While this field is not editable in the editor design view, you can modify it in the source view, and the source changes will be reflected in the editor.
- Class name - Java class that implements
LogicalHandler interface (for logical handlers), or SOAPHandler interface (for SOAP handlers).
- Handler name - the name of the Handler that is unique within the module.
- Initialization parameters for the Handler with editable names and values
- Connection and/or binding setting
- SOAP handler settings:
- SOAP headers - information to identify which SOAP headers will be processed by the SOAP Handler.
- SOAP roles - SOAP roles for the SOAP Handler. For more information, see section 10.1.1.1 of the JAX-WS 2.1 specification.
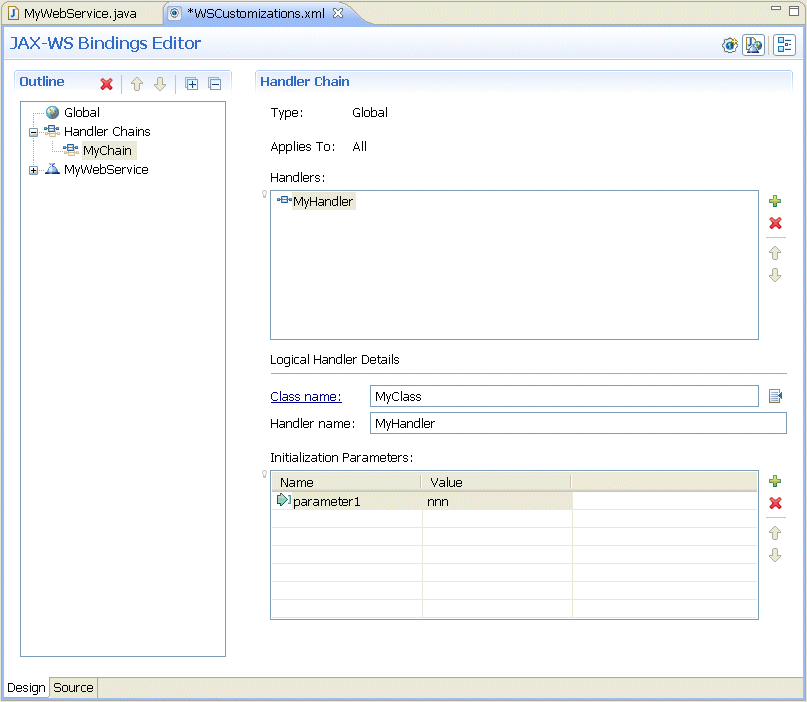
Figure 6. Customizations Editor - Handler Chains Node
- The Endpoint Interface node and the corresponding page, shown in Figure 7, represent the endpoint interface that will be generated from a WSDL Port Type after running WSDLC. This node lets you specify:
- Interface name and JavaDoc - the name for the generated Java interface. A light-gray value in the name setting means the default interface name is being used. This default value is determined by a WSDL Port Type. You can only specify the JavaDoc if the specified interface name is other than the default.
- Wrapper Style (
enableWrapperStyle customization option) - the wrapper style setting for Java method generation on a specific endpoint interface. The default value is determined by the Wrapper Style setting on the Global node. This value applies to a particular endpoint interface and its methods, however, each method can override this setting.
- Async Mapping (
enableAsyncMapping customization option) - the async mapping setting for asynchronous method generation on a specific endpoint interfaces. The default value is determined by the Async Mapping setting on the Global node. This value applies to a particular endpoint interface and its methods, however, each method can override this setting.
- Services - represents the implementing class that will be generated from a WSDL Port after running WSDLC:
- Service class name and JavaDoc - the name for the generated Java class. A light-gray value in the name setting means the default class name is being used. This default value is determined by a WSDL Port Type. You can only specify the JavaDoc if the specified interface name is other thatn the default.
- MIME content (
enableMIMEContent customizaition option) - the MIME content setting for the use of the mime:content information on the specific Service. The default value is determined by the MIME Content setting on the Global node. The value in the editor screen applies to the particular Service only.
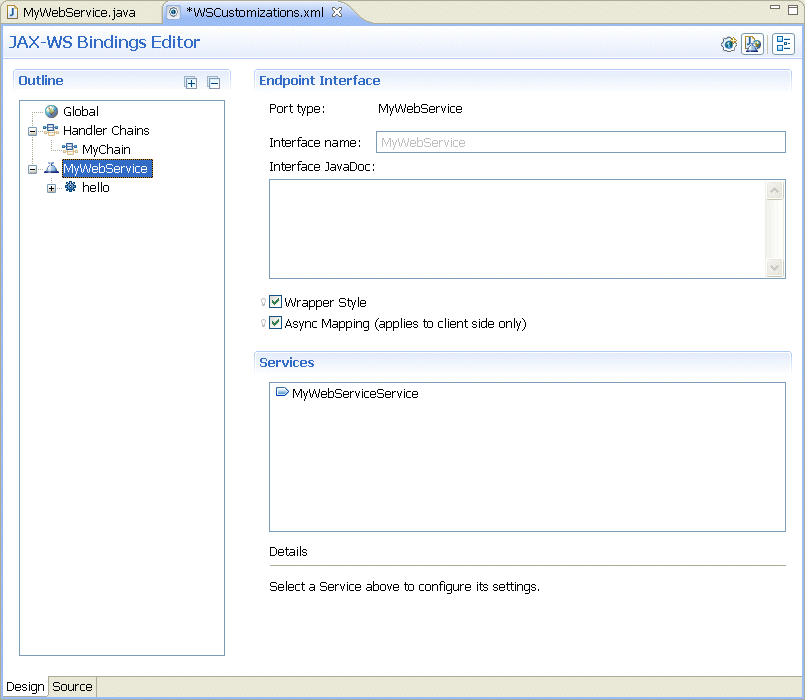
Figure 7. Customizations Editor - Endpoint Interface Node
- The Method node and the corresponding page, shown in Figure 8, represent the method that will be generated from a WSDL operation after running WSDLC. This node lets you specify:
- Method name and JavaDoc - the name for the generated Java method. A light-gray value in the name setting means the default method name is being used. This default value is determined by a WSDL operation. You can only specify the JavaDoc if the specified method name is other than the default.
- Wrapper Style (
enableWrapperStyle customization option) - the wrapper style setting for Java method generation on a specific method. The default value is determined by the Wrapper Style setting on a parent Endpoint Interface node. This value applies to a particular method only.
- Async Mapping (
enableAsyncMapping customization option) - the async mapping setting for asynchronous method generation on a specific method. The default value is determined by the Async Mapping setting on a parent Endpoint Interface node. This value applies to a particular method only.
- Method parameters - represents the method's parameters and the customization that will apply on the parameter name. Only the name is editable.
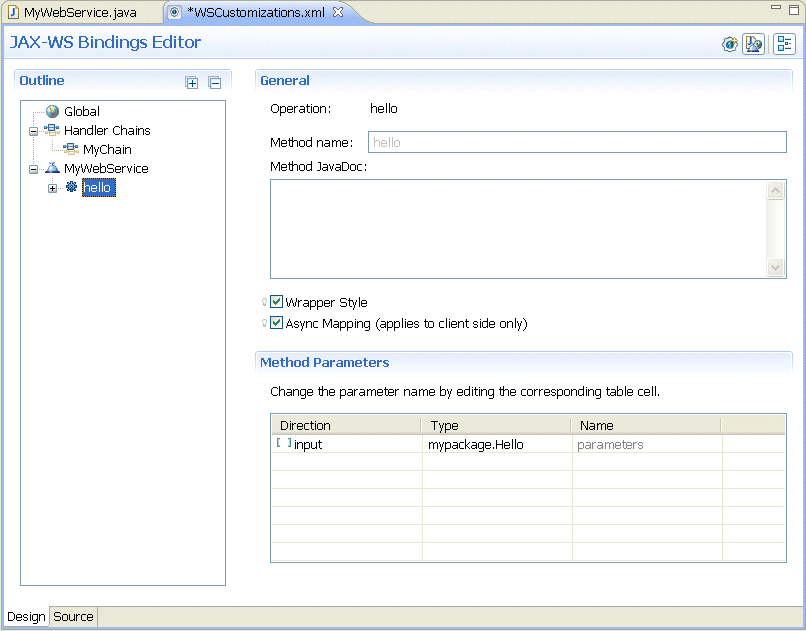
Figure 8. Customizations Editor - Method Node
- The Faults node and the corresponding page will only appear if there are Faults on the WSDL operation. This node serves as a grouping node to show a list of all the defined Faults.This node lets you specify:
- Fault Class name and JavaDoc - the name for the generated Java class. A light-gray value in the name setting means the default class name is being used. This default value is determined by a WSDL fault. You can only specify the JavaDoc if the specified class name is other than the default.
Notice that the editor pages open with default values already specified.
2. Generating a Web Service From Java
OEPE lets you generate Web services from Java using the following starting points:
2.1 Starting From Java Class
You can develop a JAX-WS-enabled Web service as a Java class, with methods becoming Web service operations, and method parameters and return types being Java beans. To indicate what methods should be exposed and to set other properties for the service, you use annotations.
To create a Web service from a Java class, do the following:
- Create a new Web service project.
- Expand your new project tree and select Java Resources > src. Right-click the src node and select New > Class from the drop-down menu. This will open the New Java Class dialog, as Figure 9 shows.
- Enter the package name in the Package field, and a class name in the Name field, and then click Finish.
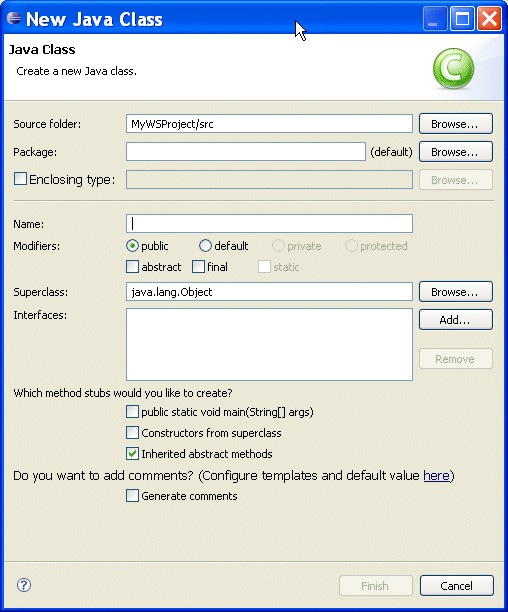
Figure 9. New Java Class Dialog
- When your new class definition opens in the source editor pane, add the following to the code:
- import
javax.jws.*
- annotate your class with the
@WebService annotation
- annotate your method with the
@WebMethod annotation
The source code will look similar to the following:
package pkg;
import javax.jws.*;
@WebService
public class MyClass {
@WebMethod
public void myMethod() {
}
}
Having followed the preceding procedure, you created a Web service from a Java class. You can run it by right-clicking the new class, and then selecting Run As > Run on Server from the drop-down menu.
You can test your Web service using the Test Client. For more information, see Testing Web Services.
2.2. Starting From Scratch
Using OEPE, you can create a JAX-WS-enabled Web service for your Java project without taking any prior steps.
To create a Web service from scratch using Java, do the following:
- Create a new Web service project.
- Right-click the project name in the Project Explorer and select New > Other from the drop-down menu. This will open the New > Select wizard dialog. Select WebLogic Web Service > WebLogic Web Service, and then click Next to open the New Web Service dialog, as Figure 10 shows.
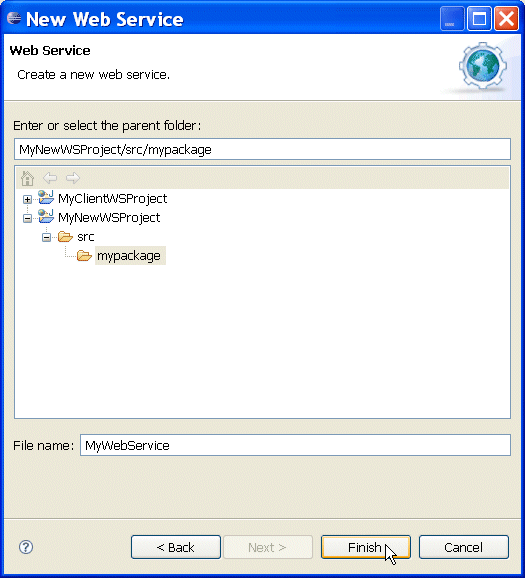
Figure 10. New Web Service Dialog
- On the New Web Service dialog, select the package and enter the file name for your new Web service, and then click Finish. This will generate a new Web service Java class template that you can use to develop your Web service.
Figure 11 shows and example of a newly generated MyWebService.java .
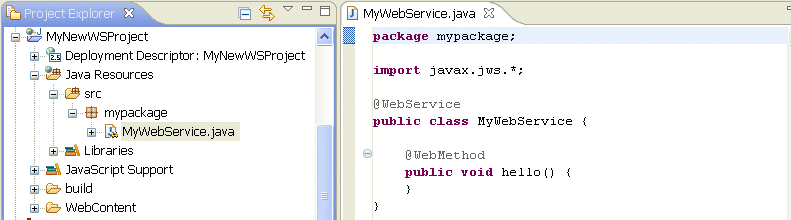
Figure 11. Web Service Class
3. Starting From a Java Class and Generating a WSDL File
Using OEPE, you can generate a WSDL file from a Java class for your project by following this procedure:
- Create new or use an existing Web service project.
- Right-click a Java class in your Web service project in the Project Explorer, and select WebLogic Web Service > Generate WSDL from the drop-down menu.
This generates a WSDL file in the same package as the Java class that you used as a starting point.
4. Related Information
W3C
WSDL Specification
Ant Task Reference in the Oracle WebLogic Server documentation
JAX-WS Documenation
JAX-WS 2.0 Customizations
Handlers in JAX-WS
JAX-WS Bindings schema
JAX-WS handlers schema
4.1 Contents of a WSDL File
Files with the WSDL extension contain Web service interfaces expressed in
the Web Service Description Language (WSDL). WSDL is a standard XML document
type specified by the World Wide Web Consortium (W3C). For more information, see www.w3.org
WSDL files communicate interface information between Web
service producers and consumers. A WSDL description allows a client to utilize
a Web service's capabilities without knowledge of the implementation details
of the Web service.
A WSDL file contains the following information necessary for a client to
invoke the methods of a Web service:
-
The data types used as method parameters or return values.
-
The individual methods names and signatures (WSDL refers to methods as operations).
-
The protocols and message formats allowed for each method.
-
The URLs used to access the Web service.
4.2 Imported WSDL Files
When you want to use an external Web service from within Eclipse,
you should first obtain the WSDL file for the service you want to use, as follows:
- For
public Web services, the WSDL file will typically be available on the Web site
of the organization that publishes the Web service.
- For private Web services,
contact the organization that supports the Web service to obtain the WSDL
file.
WSDL files can also be found through both public and private UDDI
registries. For more information about UDDI, see www.uddi.org
Once you have the WSDL file, you may use Eclipse to create a Web service.
Some Web service tools produce WSDL files that do not contain an XML
declaration. The XML declaration is just the first line of an XML file of the
following form:
<?xml version="1.0" encoding="utf-8"
?>
If you receive a WSDL file that does not contain an XML declaration, you
must add a declaration to the file using a text editor before you can use
the WSDL file in Eclipse.
Note that the encoding attribute is not required. If an encoding attribute
is not present, the default encoding is utf-8.
4.3 Creating a New WSDL File
To create a WSDL file to use in your project, follow this procedure:
Note that for a JAX-WS Web service project, you enable the Standard Annotated Web Services project facet option by right-clicking the project in the Project Explorer, selecting Properties from the drop-down menu, and then selecting Project Facets > WebLogic Web Services.
4.4 Testing Web Services
As you develop a Web service, you can test it directly by using the test client. The test client provides a user interface through which you can test Web service operations with parameter values you choose.
Using the test client, you can do the following:
- Test a Web service from the project tree: When you test Web services with Eclipse, consider the following steps that launch the test client with a visual interface for invoking the Web service's operations:
- Expand the project tree to display the Web service source file.
- Right-click the source file, then click Run > Run on Server.
- When the test client is displayed, choose the operation you want to test by clicking the button labeled with the operation's name. If the operation has parameters, the test client provides boxes for you to enter the values to test with. If an operation includes complex types as parameters, the test client will display an XML template with placeholders for your test values.
- Examine the result of the test by looking at generated messages. When you execute an operation, the test client refreshes to display information about the message exchanged by the operation. The user interface provides a summary of message values, as well as the message XML itself. When an exception occurs, a fault message is displayed.
- Use the Message Log list to view the results of multiple tests.
- Click Show Operations to begin another test.
- View the WSDL file for the Web service you are currently testing by clicking the WSDL URL provided at the top of the test client window.
- Choose another Web service to test: You can test another Web service without closing the test client by clicking the Choose Another WSDL link at the top of the test client window. The test client will display a page with a box where you enter the WSDL URL, then click Test to display the test form for that Web service.
Alternatively, you can launch the test client without using Eclipse IDE by launching the client through a Web browser, as follows:
- With the Oracle WebLogic Server running, open a browser window and navigate to the http://localhost:7001/wls_utc URL to start the test client.
- In the Enter WSDL URL box, enter the URL for the WSDL of the Web service you want to test, and then click Test.
|