To test your EJBs you need to run a client program that can create or find EJB instances and call their remote interface methods. JDeveloper provides a sample client utility that will help you create clients quickly. You can run and test EJBs using either the integrated server or a remote server;
-
Click the Red button and select the IntegratedWebLogicServer to stop WebLogicServer.
-
Collapse the ViewController project and expand the Model project.
-
Double-click Employees.java to open the source code for the class and expand the @Entity node to display the hidden code.
-
Add a comma at the end of the last @NamedQuery statement, then add a query to the class that retrieves employees by name. Add the following statement:
@NamedQuery(name = "Employees.findByName", query = "select o from Employees o where o.firstName like :p_name")
So that the code looks like the following:
@Entity
@NamedQueries( {
@NamedQuery(name = "Employees.findAll", query = "select o from Employees o"),
@NamedQuery(name = "Employees.findBySal", query = "select o from Employees o where o.salary > :p_sal"),
@NamedQuery(name = "Employees.findByName", query = "select o from Employees o where o.firstName like :p_name")
})
What makes these objects different from other Java files are the annotations that identify them as EJB entities.Read more...
A key feature of EJB 3.0 and JPA is the ability to create entities that contain object-relational mappings by using metadata annotations rather than deployment descriptors as in earlier versions.
-
Click the Make icon to compile the Employees.java class.
Make sure that the Message - Log window does not report any errors.
-
Add the new method to the session bean as follows:
Right-click the HRFacadeBean node in the Applications window and select Edit Session Facade from the context menu.
-
Expand the Employees node of the dialog. Notice that the new named query getEmployeesFindByName appears as an exposable method. Select getEmployeesFindByName, deselect getEmployeesFindBySal and click OK.
-
In the Applications window, right-click the HRFacadeBean.java and select New Sample Java Client...
-
In the Create Sample Java Client pane, leave the values at their default and click OK.
-
Two things need to be fixed.
First since the method uses a parameter, we need to add a value for the parameter.
In the code, scroll to the "/* FIXME: Pass parameters here */". In the getEmployeesFindByName method, add "p%" as the parameter. When you're finished, your code should look like the code below.
(List<Employees>) hRFacade.getEmployeesFindByName("p%") /* FIXME: Pass parameters here */)
-
Second, copy the following code and paste it at the end of the class.
Some import statements will also be included into your code
private static Context getInitialContext() throws NamingException {
Hashtable env = new Hashtable();
// WebLogic Server 10.x connection details
env.put( Context.INITIAL_CONTEXT_FACTORY, "weblogic.jndi.WLInitialContextFactory" );
env.put(Context.PROVIDER_URL, "t3://127.0.0.1:7101");
return new InitialContext( env );
}
-
Click the Save All
icon to save your work.
-
Right click the HRFacadeBean in the Applications window and select Run from the context menu to launch the facade bean in WebLogicServer.
Wait until the WebLogicServer is started.
The integrated Oracle WebLogic Server runs within JDeveloper. You can run and test EJBs quickly and easily using this server, and then deploy your EJBs with no changes to them. -
Then, right click HRFacadeClient and select Run from context.
-
To better display the results of the findByName() method, in the HRFacadeClient.java class, comment out the for loop corresponding to the getEmployeesFindAll() method, and comment out the for loop corresponding to the getDepartmentsFindAll() method. Your code should look something like this:
-
Click the Make button
to recompile the class, and ensure that no errors are returned.
-
Right click the HRFacadeClient class and select Run from context.
-
The Log window should now display the returned rows retrieved by your ' P%' clause.
The server is stopped when you see the following message in the log window.
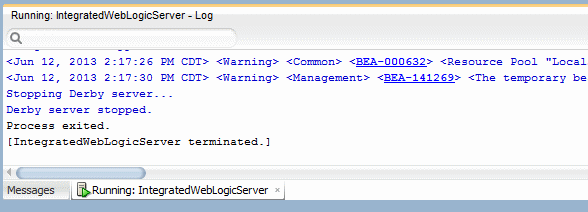
JDeveloper provides a way to test the EJB by creating a sample client. Normally, you would right click HRFacadeBean and select New Sample Java Client from the context menu. HOWEVER, due to a bug in the current version of JDeveloper, the option to generate a sample client from the context menu will not function properly. The following steps help you fix this problem. For more details on this issue, refer to the release notes..
The Log window returns the database data based on the three methods the client contains (getDepartmentsFindAll(), getEmployeesFindAll(), and getEmployeesFindByName().)
In this section, you create a session bean that implements a method to find employee and department records.
-
Create a new persistence unit to run the java service outside the Java EE container.
Right-click the META-INF > persistence.xml and select New Java Service Facade from the context menu.
-
In the Java Service Class panel, you can choose to create a new persistence unit (in the next panel) or use an existing unit. Select Choose a Persistence Unit or Create one in the next Panel, check the Generate a Sample Java Client checkbox and click Next.
-
Name the the Persistence Unit outside. Choose JDBC Connection and make sure the JDBC connection is set to HR. Click Next.
-
All methods should be selected by default. Select only the following methods and click OK.
In the source editor window, for the JavaServiceFacadeClient class, add "P%" as a parameter to the getEmployeesFindByName method so that the statement is:
(List<Employees>)javaServiceFacade.getEmployeesFindByName("P%")
-
Click the Make
button to compile the class and save your work.
-
Right-click the JavaServiceFacadeClient node in the Applications window and select Run from context.
-
The Log window displays the result of the execution of the class running outside Java EE container, returning the lastName of the first of the retrieved records (Pat Fay).
-
Double-click the META-INF > persistence.xml node to display the contents of the file.
-
The persistence editor opens showing the Model - Persistence Unit by default. Click the bread crumb navigation button to show both persistence units.
-
Click the Source tab to show the code for both persistence units that have been created. The Model one and the outside one.
Click Next then Finish.
- Build the data model with EJB 3.0 Using the EJB diagrammer
- Build the view project
- Add and expose a new method to the UI
- Test the facade bean inside and outside the Java EE container

