Executing Unit Tests for Entities
This section discusses how to:
Create an entity unit test.
Run entity unit test cases.
Page Name |
Definition Name |
Navigation |
Usage |
---|---|---|---|
Entity Unit Tests (Setup) |
SCC_ENTITY_UT |
Select Unit Test in the Action field on the Entity Registry page. |
Attach the unit test application classes to an entity and run the unit tests. |
Entity Unit Tester |
SCC_ENT_MASS_UT |
|
Run all the unit tests associated with a specified entity. Unit tests are defined on the Entity Unit Tests (Setup) page. |
Entity Unit Tests |
SCC_ENTITY_UT |
Click the View Unit Tests link on the Entity Unit Tester page. |
View the unit tests tied to an entity name, the application class used and the latest run status for a specific unit test. |
Unit tests are small focused tests that academic institutions can use to ensure that each unit of code in an entity works properly. This is done to find errors in the smallest segments of code during development and allow them to be fixed early, resulting in higher quality code.
Because the Entity Registry component is highly extensible, the system integrates unit testing with the Entity Registry, by delivering a unit test framework.
Coding a Unit Test
Each unit test must extend the class TestBase.
There are three methods that the unit test can override in TestBase:
Setup(): Is the first method that your unit test must call. This method should be used to set up the environment for the test. If environment setup is not required, the unit test does not have to implement this method.
Run(): Is the main testing method. A unit test must run all the code relevant to executing the test in this method. If the test fails, the property status should equal the property FAILED. If the test runs successfully, the property status should be set to PASSED. This method must be overridden.
Teardown(): Is the method that does the cleanup. In theory, this method should reset all the data, as if the test had never been run, therefore, if required the test can be run again successfully. This method is optional, depending on your testing requirements.
The full class reference for TestBase is presented in the SCC_COMMON:Testing:TestBase application class. This application class is described later in this section.
The following code shows an example where a unit test retrieves a constituent that is based on TestBase:
import SCC_COMMON:Testing:*; import SCC_COMMON:ENTITY:IEntity; import SCC_SL_TRANSACTION:INTFC:Constituent; class GetConstituent extends SCC_COMMON:Testing:TestBase method GetConstituent(); method setup(); method teardown(); method run(); end-class; method GetConstituent %Super = create SCC_COMMON:Testing:TestBase("GetConstituent"); end-method; method run /+ Extends/implements SCC_COMMON:Testing:TestBase.Run +/ Local SCC_SL_TRANSACTION:INTFC:Constituent &constituent = create SCC_SL_TRANSACTION:INTFC:Constituent( Null); /*Retrieves the constituent*/ &constituent.setStageMode( True); &constituent.EMPLID = "KU0004"; &constituent.fromProduction(); /*Prints in the message area the complete consitutent xml for the constituent received*/ Local XmlDoc &responseDoc = CreateXmlDoc(""); Local XmlNode &rootNode = &responseDoc.CreateDocumentElement("CONSTITUENT"); &constituent.toXmlNode(&rootNode); %This.Msg(&responseDoc.GenFormattedXmlString()); end-method; method setup /+ Extends/implements SCC_COMMON:Testing:TestBase.Setup +/ end-method; method teardown /+ Extends/implements SCC_COMMON:Testing:TestBase.Teardown +/ end-method;
Attaching a Unit Test
You attach a unit test to a specific entity. To attach a unit test to an entity:
Navigate to the Entity Registry page for the entity to which you want to attach a unit test (Set Up SACR, System Administration, Entity, Entity Registry). Continuing with the previous code example, the following shows that the Constituent entity is selected.
Image: Example of the Entity Registry page for the Constituent entity
This example illustrates the fields and controls on the Example of the Entity Registry page for the Constituent entity. You can find definitions for the fields and controls later on this page.
Select Unit Test in the Action field to access the Entity Unit Tests (setup) page.
Image: Entity Unit Tests (setup) page (for the Constituent entity)
This example illustrates the fields and controls on the Entity Unit Tests (setup) page (for the Constituent entity). You can find definitions for the fields and controls later on this page.
Field or Control
Definition
Active Select to allow the system to run the unit test.
If you clear this check box, the system ignores the unit test.
Type Select a categorical classification of the unit test, this is mostly for description.
Application Class Select the class that you have created for the unit test. In the previous exhibit example — Entity Unit Tests (setup) page for the Constituent entity — the MessageGenerator class is selected for the Constituent entity.
Run Unit Tests Click to run the unit test.
You can add any number of unit tests on this page and click this button once to run all the tests simultaneously.
OK Click to save this unit test setup.
The other fields on this page are similar to the Entity Unit Tests (view-only) page that is described in the next section “Running Entity Unit Test Cases”.
class SCC_COMMON:Testing:TestBase
This is the base class which developers must extend in order to code their tests. The methods to be overridden are Setup(), Run(), and Teardown(). The system always calls these methods in that order for each test class. In the final phase of code execution, the system attempts to call Teardown(), even when there are errors.
In addition, the system delivers helper methods for asserting various invariant conditions. The syntax is: Assert (&condition_which_must_be_true, "The expected condition was not met!"); In other words, the first argument to Assert() is a condition which you expect to be true. The second argument is an error message to display if the condition is not met. The other Assert*() methods are more of syntactic sugar.
Summary:
Property Summary:
public string FAILED
public string NOT_RUN
public string PASSED
public string status
public string UnitTestName
Constructor Summary:
Method Modifier and Return Type
Method Name and Parameters
Method Description
public void
TestBase(string UnitTestName_in)
This is the constructor.
Developers should pass in a test name as the sole argument. For now, the system does not use this value.
Method Summary:
Method Modifier and Return Type
Method Name and Parameters
Method Description
public void
Assert (boolean isTrue, string onFail)
The first argument must be a Boolean expression, which should evaluate to true. If false, the system throws an exception to indicate that the test failed. The system catches the exception, marks the test as having failed, and continues on to the next test.
public void
AssertNumbersDiffer(number num1, number num2, string onFail)
NA
public void
AssertNumbersEqual(number num1, number num2, string onFail)
NA
public void
AssertStringsDiffer(string str1, string str2, string onFail)
NA
public void
AssertStringsEqual(string str1, string str2, string onFail)
NA
public void
Msg(string msg)
NA
public void
Run()
This method must be overridden to implement the actual test that developers want to write.
Warning! If you do not override this method, the system throws an error.
public void
Setup()
The first method of the test class to be called during a run.
public void
Teardown()
The last method of the test class to be called during a run. The framework tries to call this method even after an error. Due to inconsistent exception handling by the PeopleCode runtime, a PeopleCode "catch" statement does not catch all exceptions, and so the system cannot guarantee this method will be run after an error.
Examples of untrappable errors include calls to the Error() builtin, SQL errors, and PeopleCode compile errors.
Access the Entity Unit Tester page (
).Image: Entity Unit Tester page
This example illustrates the fields and controls on the Entity Unit Tester page. You can find definitions for the fields and controls later on this page.
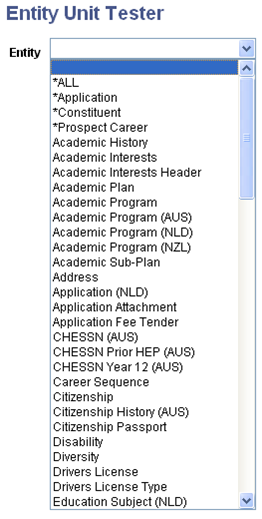
This component is purely for running unit tests that you associate with entities on the Entity Unit Tests (setup) page. The unit tests themselves are based on the Campus Solutions delivered unit test framework.
First, select an entity. This will load the unit tests specified in the Entity Registry for that entity and all child entities in the grid below it. Except for the entity called ‘All’, the prompt dynamically marks with an * the entities that have no parent entity. Those can be considered top level entities. ALL is a special case as it will run unit tests for all entities in the registry.
Image: Example of the Entity Unit Tester page for a selected entity
This example illustrates the fields and controls on the Example of the Entity Unit Tester page for a selected entity. You can find definitions for the fields and controls later on this page.
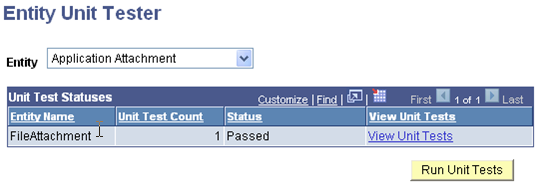
Once an entity is selected, any unit test for that entity or any children show up. If an entity has no unit tests, it will not be in the grid.
Clicking the Run Unit Tests button will start running unit tests entity by entity. Each time all the unit tests for an entity are completed the status is returned and the next entity is set for running. This is all done automatically.
The statuses will be set to either Passed or Failed - Passed telling you all the unit tests passed and Failed meaning at least one unit test failed.
Warning! Once the unit tests start, wait until statuses are set for all unit tests. Changing pages and other such actions will interrupt the unit test process.
Note: When unit tests are run from the Entity Registry page directly it will perform a similar process, however instead of doing it on a per entity basis it will be on a per unit test basis.
Click the View Unit Tests link on the Entity Unit Tester page to access the Entity Unit Tests page. The Entity Unit Tests page displays the individual unit tests for an entity.
Image: Entity Unit Tests page
This example illustrates the fields and controls on the Entity Unit Tests page. You can find definitions for the fields and controls later on this page.
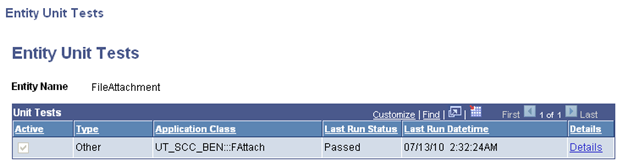
Note: The fields on the Entity Unit Tests page are display only. Their setup is defined by selecting Unit Test in the Action field on the Entity Registry page for the entity.
Field or Control |
Definition |
---|---|
Active |
Specifies if the unit test is set to active and should be run. Any unit test set to not active will not be run. |
Type |
A categorical classification of the unit test, this is mostly for description. |
Application Class |
The application class of the unit test you created for this entity. |
Last Run Status |
The status the last time this unit test was run (Passed or Failed). |
Last Run Datetime |
The last time this unit test was run. |
Details |
Click to access the Entity Unit Test Details page. The page displays the details of the unit test, including all messages output. If a unit test fails, the information provided could help you identify the reasons of the failure. |
The following is an example of the Entity Unit Test Details page:
Image: Entity Unit Test Details page
This example illustrates the fields and controls on the Entity Unit Test Details page. You can find definitions for the fields and controls later on this page.
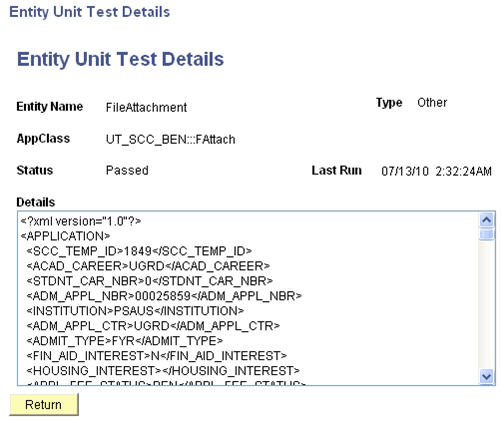