Component Interface Examples
The following are examples of some of the most usual actions you're likely to perform using a Component Interface.
The following is an example of how to create a new instance of a Component Interface.
To create a new instance of data:
In this example, you are creating a new instance of data for the EXPRESS Component Interface, which is based on the EXPRESS_ISSUE_INV component. The following is the complete code sample: the steps explain each line.
Local ApiObject &MYSESSION;
Local ApiObject &MYCI;
&MYSESSION = %Session;
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EXPRESS);
&MYCI.BUSINESS_UNIT = "H01B";
&MYCI.INTERNAL_FLG = "Y";
&MYCI.ORDER_NO = "NEXT’;
&MYCI.Create();
&MYCI.CUSTOMER = "John’s Chicken Shack";
&MYCI.LOCATION = "H10B6987";
.
.
.
If NOT(&MYCI.Save()) Then
/* save didn’t complete */
&COLL = &MYSESSION.PSMessages;
For &I = 1 to &COLL.Count
&ERROR = &COLL.Item(&I);
&TEXT = &ERROR.Text;
/* do error processing */
End-For;
&COLL.DeleteAll();
End-if;
Get a session object.
Before you can get a Component Interface, you have to get a session object. The session controls access to the Component Interface, provides error tracing, enables you to set the runtime environment, and so on.
&MYSESSION = %Session;
Get a Component Interface.
Use the GetCompIntfc method with a session object to get the Component Interface. You must specify a Component Interface definition that has already been created. You receive a runtime error if you specify a Component Interface that doesn’t exist.
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EXPRESS);
After you execute the GetCompIntfc method, you have only the structure of the Component Interface. You haven’t populated the Component Interface with data yet.
Set the CREATEKEYS.
These key values are required to open a new instance of the data. If the values you specify aren’t unique, that is, if an instance of the data already exists in the database with those key values, you receive a runtime error.
&MYCI.BUSINESS_UNIT = "H01B"; &MYCI.INTERNAL_FLG = "Y"; &MYCI.ORDER_NO = "NEXT’;
Create the instance of data for the Component Interface.
After you set the key values, you must use the Create method to populate the Component Interface with the key values you set.
&MYCI.Create();
This creates an instance of this data. However, it hasn’t been saved to the database. You must use the Save method before the instance of data is committed to the database.
Set the rest of the fields.
Assign values to the other fields.
&MYCI.CUSTOMER = "John’s Chicken Shack"; &MYCI.LOCATION = "H10B6987"; . . .
If you have specified InteractiveMode as True, every time you assign a value or use the InsertItem or DeleteItem methods, any PeopleCode programs associated with that field (either with record field or the component record field) fires (FieldEdit, FieldChange, RowInsert, and so on.)
Save the data.
When you execute the Save method, the new instance of the data is saved to the database.
If NOT(&MYCI.Save()) Then
The Save method returns a Boolean value: True if the save was successful, False otherwise. You can use this value to do error checking.
The standard PeopleSoft save business rules (that is, any PeopleCode programs associated with SaveEdit, SavePreChange, WorkFlow, and so on.) are executed. If you didn’t specify the Component Interface to run in interactive mode, FieldEdit, FieldChange, and so on, also run at this time for all fields that had values set.
Note: If you’re running a Component Interface from an Application Engine program, the data won’t actually be committed to the database until the Application Engine program performs a COMMIT.
Check Errors.
You can check if there were any errors using the PSMessages property on the session object.
If NOT(&MYCI.Save()) Then /* save didn’t complete */ &COLL = &MYSESSION.PSMessages; For &I = 1 to &COLL.Count &ERROR = &COLL.Item(&I); &TEXT = &ERROR.Text; /* do error processing */ End-For; &COLL.DeleteAll(); End-if;
If there are multiple errors, all errors are logged to the PSMessages collection, not just the first occurrence of an error. As you correct each error, delete it from the PSMessages collection.
The Text property of the PSMessage returns the text of the error message. At the end of this text is a contextual string that contains the name of the field that generated the error. The contextual string has the following syntax:
{BusinessComponentName.[CollectionName(Row).[CollectionName(Row).[CollectionName(Row)]]].PropertyName}
For example, if you specified the incorrect format for a date field of the Component Interface named ABS_HIST, the Text property would contain the following string:
Invalid Date {ABS_HIST.BEGIN_DT} (90), (1)
The contextual string (by itself) is available using the Source property of the PSMessage.
Note: If you’ve called your Component Interface from an Application Engine program, all errors are also logged in the Application Engine error log tables.
See Error Handling, Source.
The following is an example of how to get an existing instance of a Component Interface.
In this example, you are getting an existing instance of data for the EMPL_CHKLST_BC Component Interface, which is based on the EMPLOYEE_CHECKLIST component. The following is the complete code sample: the steps explain each line.
Local ApiObject &MYSESSION;
Local ApiObject &MYCI;
&MYSESSION = %Session;
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EMPL_CHKLST_BC);
&MYCI.EMPLID= "8001";
&MYCI.Get();
/* Get checklist Code */
&CHECKLIST_CD = &MYCI.CHECKLIST_CD;
/* Set Effective date */
&MYCI.EFFDT = "05-01-1990";
.
.
.
If NOT(&MYCI.Save()) Then
/* save didn’t complete */
&COLL = &MYSESSION.PSMessages;
For &I = 1 to &COLL.Count
&ERROR = &COLL.Item(&I);
&TEXT = &ERROR.Text;
/* do error processing */
End-For;
&COLL.DeleteAll();
End-if;
Get a session object.
Before you can get a Component Interface, you have to get a session object. The session controls access to the Component Interface, provides error tracing, enables you to set the runtime environment, and so on.
&MYSESSION = %Session;
Get a Component Interface.
Use the GetCompIntfc method with a session object to get the Component Interface. You must specify a Component Interface definition that has already been created. You receive a runtime error if you specify a Component Interface that doesn’t exist.
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EMPL_CHKLST_BC);
After you execute the GetCompIntfc method, you have only the structure of the Component Interface. You haven’t populated the Component Interface with data yet.
Set the GETKEYS.
These are the key values required to return a unique instance of existing data. If the keys you specify allow for more than one instance of the data to be returned, or if no instance of the data matching the key values is found, you receive a runtime error.
&MYCI.EMPLID = "8001";
Get the instance of data for the Component Interface.
After you set the key values, you have to use the Get method.
&MYCI.Get();
This populates the Component Interface with data, based on the key values you set.
Get field values or set field values.
At this point, you can either get values or set values.
&CHECKLIST_CD = &MYCI.CHECKLIST_CD; /* OR */ &MYCI.EFFDT = "05-01-1990";
If you have specified InteractiveMode as True, every time you assign a value, any PeopleCode programs associated with that field (either with record field or the component record field) fires (FieldEdit, FieldChange, and so on.)
Save or Cancel the Component Interface, as appropriate.
If you’ve changed values and you want to save your changes to the database, you must use the Save method.
If NOT(&MYCI.Save()) Then
The Save method returns a Boolean value: True if the save was successful, False otherwise. Use this value to do error checking.
The standard PeopleSoft save business rules (that is, any PeopleCode programs associated with SaveEdit, SavePreChange, WorkFlow, and so on.) are executed. If you didn’t specify the Component Interface to run in interactive mode, FieldEdit, FieldChange, and so on, also run at this time.
Note: If you’re running a Component Interface from an Application Engine program, the data won’t actually be committed to the database until the Application Engine program performs a COMMIT.
If you don’t want to save any changes to the data, use the Cancel method. The Component Interface is reset to the state it was in just after you used the GetCompIntfc method.
&MYCI.Cancel();
This is similar to a user pressing ESC while in a component, and choosing to not save any of their changes.
Check Errors.
You can check if there were any errors using the PSMessages property on the session object.
If NOT(&MYCI.Save()) Then /* save didn’t complete */ &COLL = &MYSESSION.PSMessages; For &I = 1 to &COLL.Count &ERROR = &COLL.Item(&I); &TEXT = &ERROR.Text; /* do error processing */ End-For; &COLL.DeleteAll(); End-if;
If there are multiple errors, all errors are logged to the PSMessages collection, not just the first occurrence of an error. As you correct each error, delete it from the PSMessages collection.
The Text property of the PSMessage returns the text of the error message. At the end of this text is a contextual string that contains the name of the field that generated the error. The contextual string has the following syntax:
{ComponentInterfaceName.[CollectionName(Row).[CollectionName(Row).[CollectionName(Row)]]].PropertyName}
For example, if you specified the incorrect format for a date field of the Component Interface named ABS_HIST, the Text property would contain the following string:
Invalid Date {ABS_HIST.BEGIN_DT} (90), (1)
The contextual string (by itself) is available using the Source property of the PSMessage.
Note: If you’ve called your Component Interface from an Application Engine program, all errors are also logged in the Application Engine error log tables.
See Error Handling, Source.
The following is an example of how to retrieve a list of instances of data.
To retrieve a list of instances of data:
In this example, you are getting a list of existing instances of data for the EMPL_CHKLST_CI Component Interface, which is based on the EMPLOYEE_CHECKLIST component. The following is the complete code sample: the steps break it up and explain each line.
Local ApiObject &MYSESSION;
Local ApiObject &MYCI;
Local ApiObject &MYNEWCI;
&MYSESSION = %Session;
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EMPL_CHKLST_CI);
&MYCI.EMPLID= "8";
&MYCI.LAST_NAME_SRCH = "S";
&MYLIST = &MYCI.Find();
For &I = 1 to &MYLIST.Count;
/* note: do not reuse the CI used to create the list, or the list will be destroyed */
&MYNEWCI = &MYLIST.Item(&I);
/* CI from list still must be instantiated to use it */
&MYNEWCI.Get();
/* do some processing */
End-For;
Get a session object.
Before you can get a Component Interface, you have to get a session object. The session controls access to the Component Interface, provides error tracing, enables you to set the runtime environment, and so on.
&MYSESSION = %Session;
Get a Component Interface.
Use the GetCompIntfc method with a session object to get the Component Interface. You must specify a Component Interface definition that has already been created. You receive a runtime error if you specify a Component Interface that doesn’t exist.
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.EMPL_CHKLST_CI);
After you execute the GetCompIntfc method, you have only the structure of the Component Interface. You haven’t populated the Component Interface with data yet.
Set the FINDKEYS values.
These can be alternate key values or partial key values. If no instance of the data matching the key values is found, you receive a runtime error.
&MYCI.EMPLID = "8"; &MYCI.LAST_NAME_SRCH = "S";
Get a list of data instances for the Component Interface.
After you set the alternate or partial key values, use the Find method to return a list of data instances for the Component Interface.
&MYLIST = &MYCI.Find();
Note: The Find method can be executed only at level zero in a Component Interface.
Select an instance of the data.
To select an instance of the data, you can use any of the following standard data collection methods:
First
Item
Next
For example, you could loop through every instance of the data to do some processing:
For &I = 1 to &MYLIST.Count;
/* note: do not reuse the Component Interface used to */
/* create the list here, or the list will be destroyed */
&MYNEWCI = &MYLIST.Item(&I);
/* CI from list must still be instantiated to use it */
&MYNEWCI.Get();
/* do some processing */
End-For;
After you have a specific instance of the data, you can get values, set values, and so on.
You can rename a collection in a Component Interface. For example, suppose you had the same record at level zero and at level one. You may want to rename the level one data collection to reflect this. The data in a data collection is associated with the primary database record of a scroll, not with the name you supply.
Here is an example of using a Component Interface that has the same record at level zero and level one. The Component Interface is based on the CURRENCY_CD_TBL component.
Image: Example of CI with same record at level zero and level one
This example illustrates the fields and controls on the Example of CI with same record at level zero and level one. You can find definitions for the fields and controls later on this page.
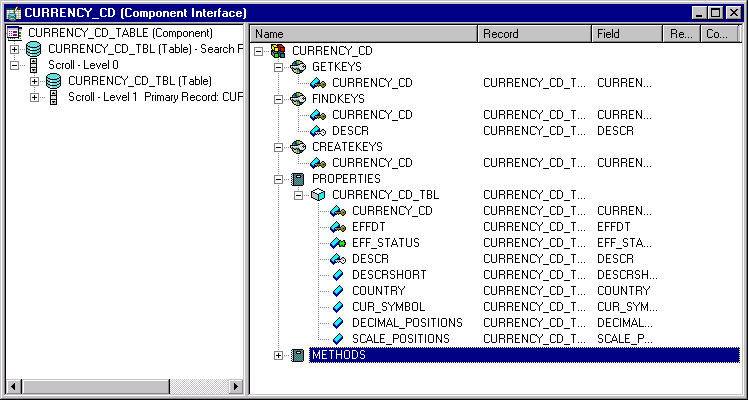
The following code example is based on this Component Interface and does the following:
Gets an existing Component Interface.
Finds the current effective-dated item index.
Inserts a new row before the current effective-dated item using the InsertItem method.
Local ApiObject &Session; Local ApiObject &CURRENCY_CD; Local ApiObject &CURRENCY_CD_TBLCol; Local ApiObject &CURRENCY_CD_TBLItm; Local ApiObject &PSMessages; Local string &ErrorText, &ErrorType; Local number &ErrorCount; Local Boolean &Error; Function CheckErrorCodes() &PSMessages = &Session.PSMessages; &ErrorCount = &PSMessages.Count; For &i = 1 To &ErrorCount &ErrorText = &PSMessages.Item(&i).Text; &ErrorType = &PSMessages.Item(&i).Type; End-For; End-Function; /* Initialize Flags */ &Error = False; &Session = %Session; If &Session <> Null Then CheckErrorCodes(); /* Application Specific Error Processing */ Else &CURRENCY_CD = &Session.GetCompIntfc(CompIntfc.CURRENCY_CD); If &CURRENCY_CD = Null Then CheckErrorCodes(); /* Application Specific Error Processing */ Else /* Set Component Interface Get Keys */ &CURRENCY_CD.CURRENCY_CD = "USD"; If Not &CURRENCY_CD.Get() Then CheckErrorCodes(); /* Application Specific Error Processing */ &Error = True; End-If; If Not &Error Then &CURRENCY_CD_TBLCol = &CURRENCY_CD.CURRENCY_CD_TBL; &CURRENCY_CD_TBLItm = &CURRENCY_CD_TBLCol.InsertItem(&CURRENCY_CD_TBLCol.CurrentItemNum()); &CURRENCY_CD_TBLItm.EFFDT = %Date; &CURRENCY_CD_TBLItm.EFF_STATUS = "A"; &CURRENCY_CD_TBLItm.DESCR = "NewCurrencyCode"; &CURRENCY_CD_TBLItm.DESCRSHORT = "New"; &CURRENCY_CD_TBLItm.COUNTRY = "USA"; &CURRENCY_CD_TBLItm.CUR_SYMBOL = "?"; &CURRENCY_CD_TBLItm.DECIMAL_POSITIONS = 4; &CURRENCY_CD_TBLItm.SCALE_POSITIONS = 0; /* Save Instance of Component Interface */ If Not &CURRENCY_CD.Save() Then CheckErrorCodes(); /* Application Specific Error Processing */ End-If; End-If; /* End: Set Component Interface Properties */ /* Cancel Instance of Component Interface */ &CURRENCY_CD.Cancel(); End-If;
End-If;
Here's a code example in Visual Basic that does the same thing as the previous code example.
Private Sub CURRENCY_CD()
On Error GoTo eMessage
'***** Set Object References *****
Dim oCISession As Object
Dim oCURRENCY_CD As Object
Dim oCURRENCY_CD_TBL As Object
Dim oCURRENCY_CD_TBLItem As Object
'***** Set Connect Parameters *****
strAppSeverPath = "//PSOFT101999:9000"
strOperatorID = "PTDMO"
strPassword = "PTDMO"
'***** Create PeopleSoft Session Object *****
Set oCISession = CreateObject("PeopleSoft.Session")
'***** Connect to the App Sever *****
oCISession.Connect 1, strAppSeverPath, strOperatorID, strPassword, 0
'***** Get the Component *****
Set oCURRENCY_CD = oCISession.GetCompIntfc("CURRENCY_CD")
'***** Set the Component Interface Mode *****
oCURRENCY_CD.InteractiveMode = False
oCURRENCY_CD.GetHistoryItems = True
'***** Set Component Get/Create Keys *****
oCURRENCY_CD.CURRENCY_CD = "USD"
'***** Execute Create *****
oCURRENCY_CD.Get
'Set CURRENCY_CD_TBL Collection Field Properties --
'Parent: PS_ROOT Collection
Set oCURRENCY_CD_TBL = oCURRENCY_CD.CURRENCY_CD_TBL
Set oCURRENCY_CD_TBLItem = oCURRENCY_CD_TBL.InsertItem(oCURRENCY_CD_TBL.CurrentItemNum())
oCURRENCY_CD_TBLItem.EFFDT = Date
oCURRENCY_CD_TBLItem.EFF_STATUS = "A"
oCURRENCY_CD_TBLItem.DESCR = "NewCurrencyCode"
oCURRENCY_CD_TBLItem.DESCRSHORT = "New"
oCURRENCY_CD_TBLItem.COUNTRY = "USA"
oCURRENCY_CD_TBLItem.CUR_SYMBOL = "?"
oCURRENCY_CD_TBLItem.DECIMAL_POSITIONS = 4
oCURRENCY_CD_TBLItem.SCALE_POSITIONS = 0
'***** Save Component Interface *****
oCURRENCY_CD.Save
oCURRENCY_CD.Cancel
Exit Sub
eMessage:
'***** Display VB Runtime Errors *****
MsgBox Err.Description
'***** Display PeopleSoft Error Messages *****
If oCISession.PSMessages.Count > 0 Then
For i = 1 To oCISession.PSMessages.Count
MsgBox oCISession.PSMessages.Item(i).Text
Next i
End If
End Sub
Sub MAIN()
CURRENCY_CD
End Sub
The CopyRowset and CopyRowsetDelta methods use the primary database name of a scroll, not the name you may give a collection.
A data collection represents a row of data. You often insert or delete a row of data.
To insert or delete a row of data:
In this example, you are getting a existing instance of data for the BUS_EXP Component Interface, which is based on the BUSINESS_EXPENSES component, then inserting (or deleting) a row of data in the second level scroll.
Image: BUS_EXP Component Interface definition
This example illustrates the fields and controls on the BUS_EXP Component Interface definition. You can find definitions for the fields and controls later on this page.
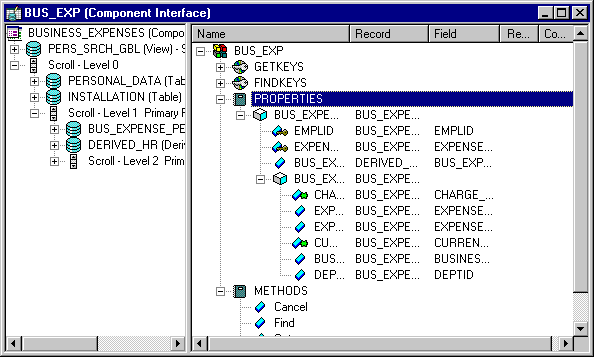
The following is the complete code sample: the steps explain each line.
Local ApiObject &MYSESSION;
Local ApiObject &MYCI;
&MYSESSION = %Session;
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.BUS_EXP);
&MYCI.EMPLID= "8001";
&MYCI.Get();
&MYLEVEL1 = &MYCI.BUS_EXPENSE_PER;
/* get appropriate item in lvl1 collection */
For &I = 1 to &MYLEVEL1.Count
&ITEM = &MYLEVEL1.Item(&I);
&MYLEVEL2 = &ITEM.BUS_EXPENSE_DTL;
&COUNT = &MYLEVEL2.Count
/* get appropriate item in lvl2 collection */
For &J = 1 to &COUNT
&LVL2ITEM = &MYLEVEL2.Item(&J);
&CIDATE = &LVL2ITEM.CHARGE_DT;
If &CIDATE <> %Date Then
/* insert row */
&NEWITEM = &MYLEVEL2.InsertItem(&COUNT);
/* set values for &NEWITEM */
Else
/* delete last row */
&MYLEVEL2.DeleteItem(&COUNT);
End-If;
End-For;
End-For;
If NOT(&MYCI.Save()) Then
/* save didn’t complete */
&COLL = &MYSESSION.PSMessages;
For &I = 1 to &COLL.Count
&ERROR = &COLL.Item(&I);
&TEXT = &ERROR.Text;
/* do error processing */
End-For;
&COLL.DeleteAll();
End-if;
Get a session object.
Before you can get a Component Interface, you have to get a session object. The session controls access to the Component Interface, provides error tracing, enables you to set the runtime environment, and so on.
&MYSESSION = %Session;
Get a Component Interface.
Use the GetCompIntfc method with a session object to get the Component Interface. You must specify a Component Interface definition that has already been created. You receive a runtime error if you specify a Component Interface that doesn’t exist.
&MYCI = &MYSESSION.GetCompIntfc(COMPINTFC.BUS_EXP);
After you execute the GetCompIntfc method, you have only the structure of the Component Interface. You haven’t populated the Component Interface with data yet.
Set the GETKEYS.
These are the key values required to return a unique instance of existing data. If the keys you specify allow for more than one instance of the data to be returned, or if no instance of the data matching the key values is found, you receive a runtime error.
&MYCI.EMPLID = "8001";
Get the instance of data for the Component Interface.
After you set the key values, you must use the Get method.
&MYCI.Get();
This populates the Component Interface with data, based on the key values you set.
Get the level one scroll.
The name of the scroll can be treated like a property. It returns a data collection that contains all the level one data.
&MYLEVEL1 = &MYCI.BUS_EXPENSE_PER
Get the appropriate item in the level one data collection.
Remember, scroll data is hierarchical. You must get the appropriate level one row before you can access the level two data.
For &I = 1 to &MYLEVEL1.Count &ITEM = &MYLEVEL1.Item(&I);
Get the level two scroll.
This is done the same way as you accessed the level one scroll, by using the scroll name as a property.
&MYLEVEL2 = &ITEM.BUS_EXPENSE_DTL;
Get the appropriate item in the level two data collection.
A data collection is made up of a series of items (rows of data.) You have to access the appropriate item (row) in this level also.
&COUNT = &MYLEVEL2.Count /* get appropriate item in lvl2 collection */ For &J = 1 to &COUNT &LVL2ITEM = &MYLEVEL2.Item(&J);
Insert or delete a row of data.
You can insert or delete a row of data from a data collection. The following example finds the last item (row of data) in the second level scroll. If it matches the value of %Date, the last item is deleted. If it doesn’t match, a new row is inserted.
&CIDATE = &LVL2ITEM.CHARGE_DT; If &CIDATE <> %Date Then /* insert row */ &NEWITEM = &MYLEVEL2.InsertItem(&COUNT); /* set values for &NEWITEM */ Else /* delete last row */ &MYLEVEL2.DeleteItem(&COUNT); End-If;
Save the data.
When you execute the Save method, the new instance of the data is saved to the database.
If NOT(&MYCI.Save()) Then
The Save method returns a Boolean value: True if the save was successful, False otherwise. Use this value to do error checking.
The standard PeopleSoft save business rules (that is, any PeopleCode programs associated with SaveEdit, SavePreChange, WorkFlow, and so on) are executed. If you did not specify the Component Interface to run in interactive mode, FieldEdit, FieldChange, and so on, also run at this time.
Note: If you’re running a Component Interface from an Application Engine program, the data won’t actually be committed to the database until the Application Engine program performs a COMMIT.
Check Errors.
You can check if there were any errors using the PSMessages property on the session object.
If NOT(&MYCI.Save()) Then /* save didn’t complete */ &COLL = &SESSION.PSMessages; For &I = 1 to &COLL.Count &ERROR = &COLL.Item(&I); &TEXT = &ERROR.Text; /* do error processing */ End-For; &COLL.DeleteAll(); End-if;
If there are multiple errors, all errors are logged to the PSMessages collection, not just the first occurrence of an error. As you correct each error, delete it from the PSMessages collection.
The Text property of the PSMessage returns the text of the error message. At the end of this text is a contextual string that contains the name of the field that generated the error. The contextual string has the following syntax:
{BusinessComponentName.[CollectionName(Row).[CollectionName(Row).[CollectionName(Row)]]].PropertyName}
For example, if you specified the incorrect format for a date field of the Component Interface named ABS_HIST, the Text property contains the following string:
Invalid Date {ABS_HIST.BEGIN_DT} (90), (1)
The contextual string (by itself) is available using the Source property of the PSMessage.
Note: If you’ve called the Component Interface from an Application Engine program, all errors are also logged in the Application Engine error log tables.
See Error Handling, Source.