Mail Classes Examples
This section provides examples for creating and authenticating email messages.
The following code example creates and sends a very simple email, then tests the results.
import PT_MCF_MAIL:*;
/*-- Create an outbound email object --*/
Local PT_MCF_MAIL:MCFOutboundEmail &eMail =
create PT_MCF_MAIL:MCFOutboundEmail();
/*-- Initialize the usual fields of an email --*/
&eMail.From = &FromAddress;
&eMail.Recipients = &ToList;
&eMail.Subject = &Subject;
&eMail.Text = &MailBody;
/*-- The send method uses the default SMTP parameters as set in the app server configuration file.
This send method makes a connection to the SMTP server, sends the mail and then disconnects.
The result are returned as a number corresponding to the possible values.
The list of ValidSent, InvalidSent and Invalid addresses are returned in the email object itself
----*/
Local integer &resp = &eMail.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following code example creates a text email and overrides the SMTP settings as found in the application server configuration file.
import PT_MCF_MAIL:*;
/*-- Create an email object by setting individual parameters ---*/
Local PT_MCF_MAIL:MCFOutboundEmail &eMail =
create PT_MCF_MAIL:MCFOutboundEmail();
&eMail.Recipients = &ToList; /* comma separated list of email addresses */
&eMail.CC = &CCList; /* comma separated list of email addresses */
&eMail.BCC = &BCCList; /* comma separated list of email addresses */
&eMail.From = &FromAddress; /* from email address */
&eMail.ReplyTo = &ReplyToAddress; /* in case the reply is to be sent to a different email address */
&eMail.Sender = &SenderAddress; /* If different from the "from" address */
&eMail.Subject = &Subject; /* email subject line */
&eMail.Text = &MailBody; /* email body text */
/*-- Override the default SMTP parameters specified in app server configuration file ----*/
&eMail.SMTPServer = "psp-smtpg-01";
&eMail.SMTPPort = 10266; /*-- Usually this is 25 by default */
Local integer &resp = &eMail.Send();
/* Now check the &resp for the result */
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following example creates an HTML email.
import PT_MCF_MAIL:*;
/*-- Create an email object by setting individual parameters
---*/
Local PT_MCF_MAIL:MCFOutboundEmail &email =
create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
&email.Text =
"<html><body><H1><b>Hi there!</b></H1><P>We are ready.</body></html>";
&email.ContentType = "text/html";
Local integer &res = &email.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following code example creates an email with both HTML and text sections.
The email client on the target host must be able to detect the HTML and text parts in the email and display the part that the client is configured to display.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email =
create PT_MCF_MAIL:MCFOutboundEmail();
Local string &TestName = "Text and its alternate html body";
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
Local PT_MCF_MAIL:MCFBodyPart &text = create PT_MCF_MAIL:MCFBodyPart();
&text.Text = "Hi There";
Local PT_MCF_MAIL:MCFBodyPart &html = create PT_MCF_MAIL:MCFBodyPart();
&html.Text =
"<html><BODY><H1>EMail test with HTML content</H1><b>Hi There</b>" |
"<A href='http://www.peoplesoft.com'>Check this out!</A>" |
"<P></BODY></html>";
&html.ContentType = "text/html";
Local PT_MCF_MAIL:MCFMultipart &mp = create PT_MCF_MAIL:MCFMultipart();
&mp.SubType = "alternative; differences=Content-type";
&mp.AddBodyPart(&text);
&mp.AddBodyPart(&html);
&email.MultiPart = ∓
Local integer &res = &email.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following code example creates an HTML email with embedded images. The content ID for an image can be a reference to a part in the email. In the following code example, the images become a part of the email and are transmitted as attachments to the email.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email =
create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
Local string &htmlText =
"<html>" |
" <head><title></title></head>" |
" <body>" |
" <b>A sample jpg</b><br>" |
" <IMG SRC=cid:23abc@pc27 width=566 height=424><br>" |
" <b>End of jpg</b>" |
" </body>" |
"</html>";
/* In this sample, the htmlText is assembled using | operator
only for the readability.
Specifying just one string can be more efficient
*/
Local PT_MCF_MAIL:MCFMultipart &mp = create PT_MCF_MAIL:MCFMultipart();
&mp.SubType = "related";
Local PT_MCF_MAIL:MCFBodyPart &html = create PT_MCF_MAIL:MCFBodyPart();
&html.Text = &htmlText;
&html.ContentType = "text/html";
Local PT_MCF_MAIL:MCFBodyPart &image = create PT_MCF_MAIL:MCFBodyPart();
&image.SetAttachmentContent("///file:C:/User/Documentum/XML%20Applications/proddoc/peoplebook_upc/peoplebook_upc.dtd",
%FilePath_Absolute, "sample.jpg", "This is a sample image!", "", "");
&image.AddHeader("Content-ID", "<23abc@pc27>");
&mp.AddBodyPart(&html);
&mp.AddBodyPart(&image);
&email.MultiPart = ∓
Local integer &res = &email.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following example creates an email message using output from a long edit box enabled with the rich text editor. Using the ParseRichTextHtml method, rich text output of the long edit box is split into an array of MCFBodyPart objects.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email = create PT_MCF_MAIL:MCFOutboundEmail();
Local PT_MCF_MAIL:MCFMultipart &mp = create PT_MCF_MAIL:MCFMultipart();
Local PT_MCF_MAIL:MCFMailUtil &mu = create PT_MCF_MAIL:MCFMailUtil();
Local string &htmlText = RECORD_NAME.FIELD_NAME.Value;
&mp.SubType = "related";
&email.Recipients = &recipients;
&email.From = &from;
&email.Subject = "Your Order";
Local array of PT_MCF_MAIL:MCFBodyPart &bp;
&mu.imagesLocation = "c:\temp\images";
&bp = &mu.ParseRichTextHtml(&htmlText);
If (&bp = Null) Then
MessageBox(0, "", 0, 0, &mu.ErrorDescription);
Return;
End-If;
&mp.AddBodyPart(&bp [1]);
For &i = 2 To &bp.Len
&mp.AddBodyPart(&bp [&i]);
End-For;
&email.MultiPart = ∓
Local integer &resp = &email.Send();
/* Exec code to check the &resp for the result */
/* Exec code to delete temporary image files from the imagesLocation directory */
For example, the rich text editor is used to create a message containing text and two images as shown in this example:
Image: Rich text editor displaying an example email
This example illustrates the fields and controls on the Rich text editor displaying an example email. You can find definitions for the fields and controls later on this page.
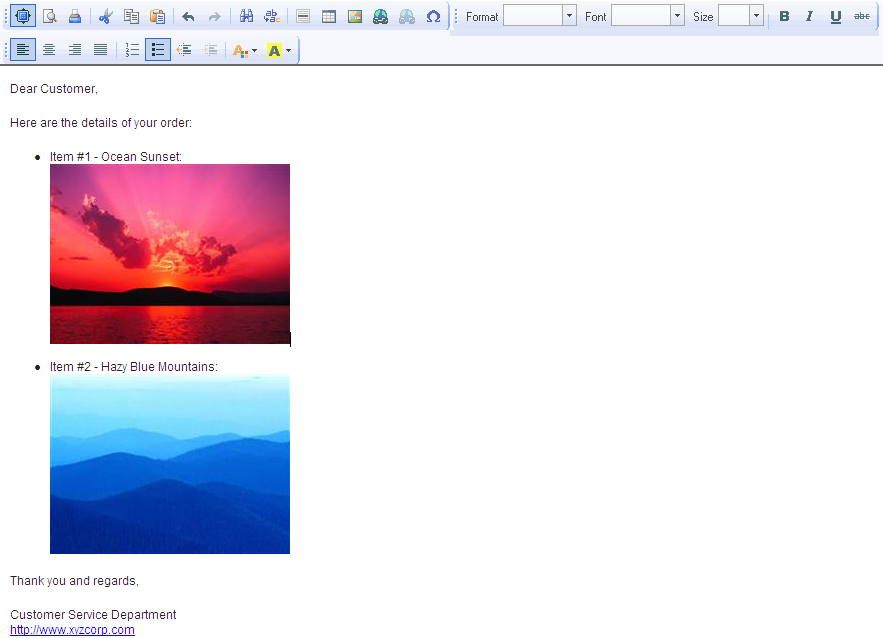
The HTML for this email is as follows:
<p>Dear Customer,</p>
<p>Here are the details of your order:</p>
<ul>
<li> Item #1 - Ocean Sunset:
<br /> <img height="180" id="PT_RTE_IMG_DB_LOC###20091111142435187Sunset_thumbnail.jpg" src="/cs/QEDMO/cache/PTRTDB_JPG_GIYDAOJRGEYTCMJUGI1DGNJRHA2VG4LOONSXIX2UNB1W1YTOMFUWYLTKOBTQ=_GA==_GE==_2000000000.JPG" width="240" /><br /> </li>
<li> Item #2 - Hazy Blue Mountains:
<br /> <img height="180" id="PT_RTE_IMG_DB_LOC###20091111142508395Mountains_thumbnail.jpg" src="/cs/QEDMO/cache/PTRTDB_JPG_GIYDAOJRGEYTCMJUGI1TAOBTHE1U122VNZ1GC1LOONPXI1DVNVRG3YLJNQXGU3DH_GA==_GE==_2000000000.JPG" width="240" /></li>
</ul>
<p>Thank you and regards,</p>
<p>Customer Service Department
<br /><a href="http://www.xyzcorp.com">http://www.xyzcorp.com</a></p>
When this HTML is parsed by the ParseRichTextHtml method, an array with three MCFBodyPart elements is created. The first MCFBodyPart is array element &bp [1] with the following properties:
&bp [1].ContentType =
text/html
&bp [1].Text =
<p>Dear Customer,</p> <p>Here are the details of your order:</p> <ul> <li> Item #1 - Ocean Sunset: <br /> <img height="180" id="PT_RTE_IMG_DB_LOC###20091111142435187Sunset_thumbnail.jpg" src="cid:2205@pc27" width="240" /> <br /> </li> <li> Item #2 - Hazy Blue Mountains: <br /> <img height="180" id="PT_RTE_IMG_DB_LOC###20091111142508395Mountains_thumbnail.jpg" src="cid:8160@pc27" width="240" /></li> </ul> <p>Thank you and regards,</p> <p>Customer Service Department <br /><a href="http://www.xyzcorp.com">http://www.xyzcorp.com</a></p>
The second MCFBodyPart is array element &bp [2] with the following properties:
&bp [2].ContentType =
image/gif
&bp [2].Description =
Image1
&bp [2].Disposition =
inline
&bp [2].AttachmentURL =
c:\temp\images/20091111142435187Sunset_thumbnail.jpg
The final MCFBodyPart is array element &bp [3] with the following properties:
&bp [3].ContentType =
image/gif
&bp [3].Description =
Image2
&bp [3].Disposition =
inline
&bp [3].AttachmentURL =
c:\temp\images/20091111142508395Mountains_thumbnail.jpg
Observe that the email received by the recipient is formatted the way that it appeared in the rich text editor as shown in the following example:
Image: Email as received in Microsoft Outlook
This example illustrates the fields and controls on the Email as received in Microsoft Outlook. You can find definitions for the fields and controls later on this page.
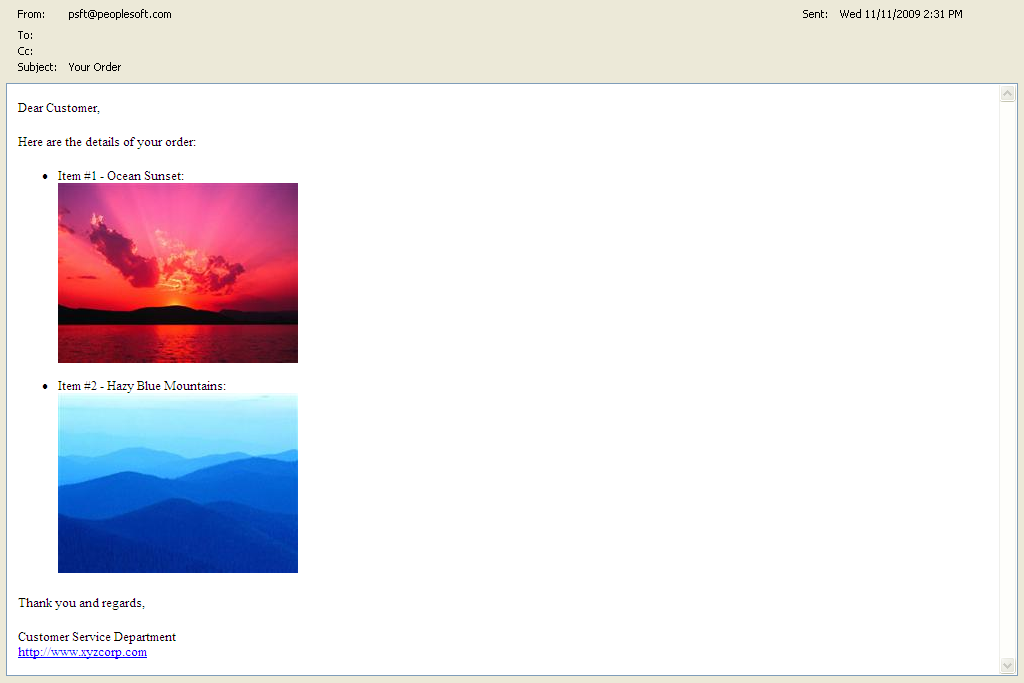
Finally, the XML code that represents this email message is as follows:
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<SOAP-ENV:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:cms="http://cms.ocs.oracle/" xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xs="http://www.w3.org/2001/XMLSchema">
<SOAP-ENV:Body>
<cms:GetEntitySnapshotResponse>
<return xsi:type="EmailMessageType_DEBUG" SnapshotId="0000000000000001000009E2607E2B4200000124E528DD580000000000000001" CEN="334B:3BF0:emsg:38893C00F42F38A1E0404498C8A6612B0000FEE32642">
<CreatedOn>2009-11-11T21:31:07Z</CreatedOn>
<Creator EID="334B:3BF0:user:CB3F97695BD24114B65149D2E4CC842E0000000112AE"/>
<Deleted>false</Deleted>
<ModifiedBy EID="334B:3BF0:user:CB3F97695BD24114B65149D2E4CC842E0000000112AE"/>
<ModifiedOn>2009-11-11T21:31:07Z</ModifiedOn>
<Name>your order</Name>
<Parent EID="334B:3BF0:afrh:38893C00F42F38A1E0404498C8A6612B00001EF9C00A"/>
<New>false</New>
<ReadFlag>true</ReadFlag>
<UserCreatedOn>2009-11-11T21:31:06Z</UserCreatedOn>
<Viewer EID="334B:3BF0:user:CB3F97695BD24114B65149D2E4CC842E0000000112AE"/>
<Content>
<ContentId>24316678.11257950022359.JavaMail.admin@XXXXX</ContentId>
<MediaType>message/rfc822</MediaType>
<Body xsi:type="MultiContentType">
<MediaType>multipart/related</MediaType>
<Parts>
<Item xsi:type="SimpleContentType">
<MediaType>text/html</MediaType>
<CharacterEncoding>us-ascii</CharacterEncoding>
<Language>en</Language>
<ContentStream>
<Id>T0RNMU5ESTFOVFpEUmtKQ05FWTJNemhETmpWQ1JqWXdRelZDT0VJek9EUXdNREF3TURBd01EWTVRVUU9JCQjIyQkN2JpdCQkIyMkJDg3NyQkIyMkJDU2Mg==</Id>
<Length>640</Length>
</ContentStream>
</Item>
<Item xsi:type="SimpleContentType">
<Disposition>INLINE</Disposition>
<ContentId><2205@pc27></ContentId>
<MediaType>image/gif</MediaType>
<CharacterEncoding>ascii</CharacterEncoding>
<Language>en</Language>
<ContentStream>
<Id>T0RNMU5ESTFOVFpEUmtKQ05FWTJNemhETmpWQ1JqWXdRelZDT0VJek9EUXdNREF3TURBd01EWTVRVUU9JCQjIyQkYmFzZTY0JCQjIyQkMTY3NCQkIyMkJDExNzI4</Id>
<Length>11923</Length>
</ContentStream>
<Name>20091111142435187Sunset_thumbnail.jpg</Name>
</Item>
<Item xsi:type="SimpleContentType">
<Disposition>INLINE</Disposition>
<ContentId><8160@pc27></ContentId>
<MediaType>image/gif</MediaType>
<CharacterEncoding>ascii</CharacterEncoding>
<Language>en</Language>
<ContentStream>
<Id>T0RNMU5ESTFOVFpEUmtKQ05FWTJNemhETmpWQ1JqWXdRelZDT0VJek9EUXdNREF3TURBd01EWTVRVUU9JCQjIyQkYmFzZTY0JCQjIyQkMTM2NDMkJCMjJCQ3MzUy</Id>
<Length>7553</Length>
</ContentStream>
<Name>20091111142508395Mountains_thumbnail.jpg</Name>
</Item>
</Parts>
</Body>
<From>
<Address><psft@peoplesoft.com></Address>
</From>
<Priority>NONE</Priority>
<Sender>
<Address><psft@peoplesoft.com></Address>
</Sender>
<SentDate>2009-11-11T21:31:06Z</SentDate>
<Size>21039</Size>
<Subject>Your Order</Subject>
<TOReceivers>
<Item>
<Address><></Address>
<Participant EID="334B:3BF0:user:CB3F97695BD24114B65149D2E4CC842E0000000112AE"/>
<Directives/>
<Status>DELIVERED</Status>
</Item>
</TOReceivers>
</Content>
<DeliveredTime>
<DateOnly>false</DateOnly>
<FloatingTime>false</FloatingTime>
<Timestamp>2009-11-11T21:31:07Z</Timestamp>
</DeliveredTime>
<Modifiable>false</Modifiable>
<ReceiptRequested>false</ReceiptRequested>
<Type>EMAIL</Type>
<MimeHeaders>
<Item>
<Name>X-AUTH-TYPE</Name>
<Value>SW50ZXJuYWwgSVA=</Value>
</Item>
<Item>
<Name>Received</Name>
<Value>ZnJvbSByZ21pbmV0MTMub3JhY2xlLmNvbSAoLzE0OC44Ny4xMTMuMTI1KSBieSBkZWZhdWx0IChPcmFjbGUgQmVlaGl2ZSBHYXRld2F5IHY0LjApIHdpdGggRVNNVFAgOyBXZWQsIDExIE5vdiAyMDA5IDEzOjMxOjA2IC0wODAw</Value>
</Item>
<Item>
<Name>Received</Name>
<Value>ZnJvbSBCVUZGWSAoYnVmZnkudXMub3JhY2xlLmNvbSBbMTAuMTM4LjIyOC4xMzNdKSBieSByZ21pbmV0MTMub3JhY2xlLmNvbSAoU3dpdGNoLTMuMy4xL1N3aXRjaC0zLjMuMSkgd2l0aCBFU01UUCBpZCBuQUJMVjZVSjAxMzk0NiBmb3IgPGRhdmUucGllcmNlQG9yYWNsZS5jb20+OyBXZWQsIDExIE5vdiAyMDA5IDIxOjMxOjA2IEdNVA==</Value>
</Item>
<Item>
<Name>MIME-Version</Name>
<Value>MS4w</Value>
</Item>
<Item>
<Name>To</Name>
<Value>ZGF2ZS5waWVyY2VAb3JhY2xlLmNvbQ==</Value>
</Item>
<Item>
<Name>MESSAGE-ID</Name>
<Value>PDI0MzE2Njc4LjExMjU3OTUwMDIyMzU5LkphdmFNYWlsLmFkbWluQEJVRkZZPg==</Value>
</Item>
<Item>
<Name>Content-Type</Name>
<Value>bXVsdGlwYXJ0L3JlbGF0ZWQ7ICBib3VuZGFyeT0iLS0tLT1fUGFydF8xXzIzNzUyMTguMTI1Nzk1MDAyMjEwOSI=</Value>
</Item>
<Item>
<Name>From</Name>
<Value>cHNmdEBwZW9wbGVzb2Z0LmNvbQ==</Value>
</Item>
<Item>
<Name>X-SOURCE-IP</Name>
<Value>YnVmZnkudXMub3JhY2xlLmNvbSBbMTAuMTM4LjIyOC4xMzNd</Value>
</Item>
<Item>
<Name>X-CT-REFID</Name>
<Value>c3RyPTAwMDEuMEEwOTAyMDQuNEFGQjJEMTkuMDBGQSxzcz0xLGZncz0w</Value>
</Item>
<Item>
<Name>Subject</Name>
<Value>WW91ciBPcmRlcg==</Value>
</Item>
<Item>
<Name>Date</Name>
<Value>V2VkLCAxMSBOb3YgMjAwOSAyMTozMTowNiBHTVQ=</Value>
</Item>
</MimeHeaders>
</return>
</cms:GetEntitySnapshotResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
The following code example creates an email with two attachments.
Oracle recommends that you always provide the proper extension in the file name, otherwise the receiving email client may not be able to associate it with the appropriate application.
Note: There is no automatic restriction of attachment size using the mail classes. To restrict an email based on attachment size, your application must check the size of an attachment before composing and sending the email.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email =
create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
Local string &plain_text = "Hi there!";
Local PT_MCF_MAIL:MCFBodyPart &text = create PT_MCF_MAIL:MCFBodyPart();
&text.Text = &plain_text;
Local PT_MCF_MAIL:MCFBodyPart &attach1 = create PT_MCF_MAIL:MCFBodyPart();
&attach1.SetAttachmentContent("Ocean Wave.jpg", %FilePath_Relative,
"Ocean Wave.jpg", "Ocean Wave", "", "");
/* %FilePath_Relative indicates the file is available at Appserver's FILES dierctory */
Local PT_MCF_MAIL:MCFBodyPart &attach2 = create PT_MCF_MAIL:MCFBodyPart();
&attach2.SetAttachmentContent("///file:C:/User/Documentum/XML%20Applications/proddoc/peoplebook_upc/peoplebook_upc.dtd",
%FilePath_Absolute, "Sample.jpg", "Sample", "", "");
/* The Sample.jpg is available in the "public" folder of my-server machine*/
Local PT_MCF_MAIL:MCFMultipart &mp = create PT_MCF_MAIL:MCFMultipart();
&mp.AddBodyPart(&text);
&mp.AddBodyPart(&attach1);
&mp.AddBodyPart(&attach2);
&email.MultiPart = ∓
Local integer &res = &email.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
The following code example creates two email attachments by specifying a URL for each file location instead of an absolute or relative path to the file.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email = create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
Local string &plain_text = "Hi there!";
Local PT_MCF_MAIL:MCFBodyPart &text = create PT_MCF_MAIL:MCFBodyPart();
&text.Text = &plain_text;
Local PT_MCF_MAIL:MCFBodyPart &attach1 = create PT_MCF_MAIL:MCFBodyPart();
&attach1.SetAttachmentContent("http://example.com/index.htm", %FilePath_Absolute, "example.html", "An Example", "", "");
Local PT_MCF_MAIL:MCFBodyPart &attach2 = create PT_MCF_MAIL:MCFBodyPart();
&attach2.SetAttachmentContent("ftp://www.w3c/docs/somedoc.htm", %FilePath_Absolute, "somedoc.htm", "somedoc from w3c", "", "");
Local PT_MCF_MAIL:MCFMultipart &mp = create PT_MCF_MAIL:MCFMultipart();
&mp.AddBodyPart(&text);
&mp.AddBodyPart(&attach1);
&mp.AddBodyPart(&attach2);
&email.MultiPart = ∓
Local integer &res = &email.Send();
Local boolean &done;
Evaluate &resp
When %ObEmail_Delivered
/* every thing ok */
&done = True;
Break;
When %ObEmail_NotDelivered
&done = False;
MessageBox(0, "", 18036, 11013, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
When %ObEmail_PartiallyDelivered
&done = True;
MessageBox(0, "", 18036, 11015, "Message not found");
Break;
When %ObEmail_FailedBeforeSending
&done = False;
MessageBox(0, "", 18036, 11014, "Message not found");
MessageBox(0, "", &email.MessageSetNumber, &email.MessageNumber, "PeopleTools error not found", &email.GetErrorMsgParam(1), &email.GetErrorMsgParam(2));
Break;
End-Evaluate;
You can create several emails and send them all in a single connection to the SMTP server. However, your system administrator may also need to configure the SMTP server with longer connection time-outs if you are sending multiple emails.
import PT_MCF_MAIL:*;
/*-- Create an email object by setting individual parameters
---*/
Local array of PT_MCF_MAIL:MCFOutboundEmail &mails;
Local PT_MCF_MAIL:MCFOutboundEmail &email;
Local PT_MCF_MAIL:SMTPSession &commonSession =
create PT_MCF_MAIL:SMTPSession();
&email = &commonSession.CreateOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList1;
&email.Subject = &Subject;
&email.Text = &MailBody1;
&mails = CreateArray(&email);
&email = &commonSession.CreateOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList2;
&email.Subject = &Subject;
&email.Text = &MailBody2;
&mails [2] = &email;
&email = &commonSession.CreateOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList3;
&email.Subject = &Subject;
&email.Text = &MailBody3;
&mails [3] = &email;
Local array of integer &allRes = &commonSession.SendAll(&mails);
The following code example includes a user name and password to be used by the SMTP server.
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email =
create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
&email.Text = &MailBody;
&email.SMTPServer = "MySMTPServer";
&email.IsAuthenticationReqd = True;
&email.SMTPUserName = "SomeLoginName";
&email.SMTPUserPassword = "SomeLoginPassword";
Local integer &res = &email.Send();
The following code example uses an SSL connection to send an email message:
import PT_MCF_MAIL:*;
Local PT_MCF_MAIL:MCFOutboundEmail &email = create PT_MCF_MAIL:MCFOutboundEmail();
&email.From = &FromAddress;
&email.Recipients = &ToList;
&email.Subject = &Subject;
&email.Text = &MailBody;
&email.SMTPServer = "MySMTPServer";
&email.IsAuthenticationReqd = True;
&email.SMTPUserName = "SomeLoginName";
&email.SMTPUserPassword = "SomeLoginPassword";
&email.SMTPUseSSL = "Y";
&email.SMTPSSLClientCertAlias = &cAlias;
&email.SMTPSSLPort = &SSLPort;
Local integer &res = &email.Send();