Creating Push Notification Events
When creating push notification events, you:
Define the event.
An event is associated with a status change on the server. When you define an event, you specify its data type and data structure.
(Optional) Add the event to an event collection.
You can group a set of events that exhibit a common behavior as a collection. When an endpoint is subscribed to a collection, it receives notifications for each event included in the collection whenever they are published from the server.
Publish the event using PeopleCode.
Subscribe endpoints to the event or event collection.
Use the Define Server Side Events page to define the data type and data structure for state changes that occur on the server. Examples of state changes include:
Process Scheduler process or job status change.
Approval notification for a workflow.
A custom state change such as adding or updating a row in an application data table.
Navigation
Image: Define Server Side Events Page
The following example shows the Server Side Events grid where you add server side events.
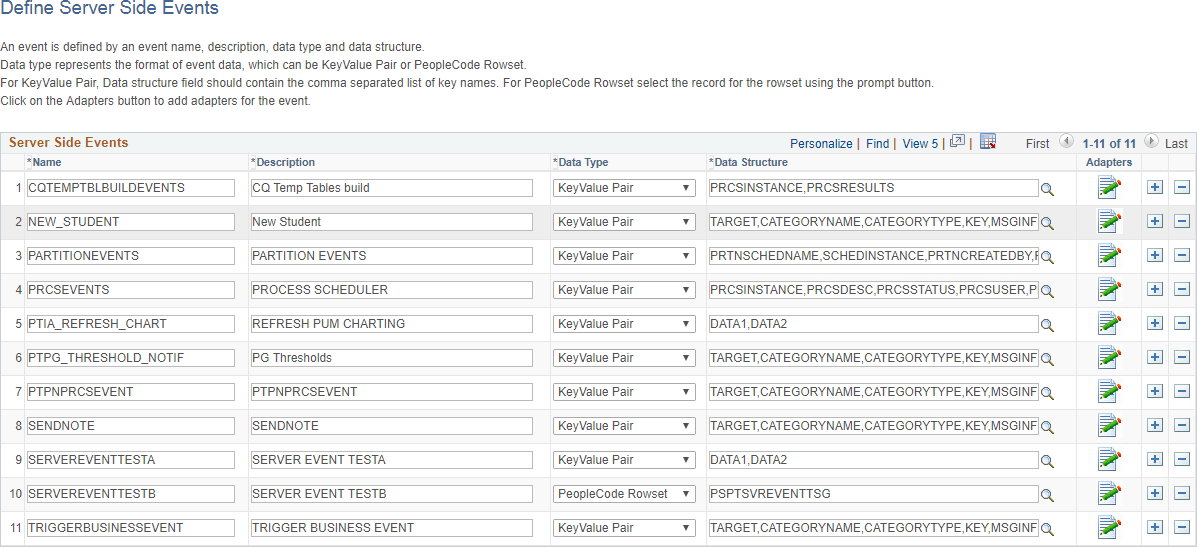
Field or Control |
Definition |
---|---|
Name |
Enter a unique name for the event. |
Description |
Enter a description of the event. |
Data Type |
Select between KeyValue Pair or PeopleCode Rowset. The data type defines how the system structures the event data while publishing the event. The value you assign determines what you enter for a data structure. |
Data Structure |
Enter the Data Structure value, depending on the Data Type selected.
Use delivered PeopleCode
application classes to set event data (in the form of key-value pairs
or record field data) when publishing the event.
|
Adapters |
Adapters are used for sending push notifications to mobile applications running in the Oracle Mobile Application Framework (MAF). An adapter is associated with a server side event. For more information on adapters and working with MAF, see Creating an Adapter. |
Use the Define Server Event Collections page to define event collections and add defined events to a collection.
Navigation
.
Image: Define Server Event Collections Page
This example illustrates the fields and controls on the Define Server Event Collections Page. You can find definitions for the fields and controls later on this page.

Field or Control |
Definition |
---|---|
Collection Name |
Enter the unique name for the event collection. |
Description |
Enter a description of the event collection. |
Root Package ID |
Select the PeopleCode application package that contains the application class that is the handler for this collection. |
Application Class ID |
Select the PeopleCode application class that handles the events that are part of the event collection when they are published. A valid handler class implements the PT_PNCOLL_HANDLER:Handler interface. |
Events |
Click the Events link to maintain the events for the event collection using the Add Events page. If an endpoint is subscribed to the collection, then it will receive the push notifications for each of the events listed in this page. |
Add Events Page
Use the Add Events page to maintain the events associated with an event collection.
Image: Add Events page
This example illustrates the fields and controls on the Add Events page.
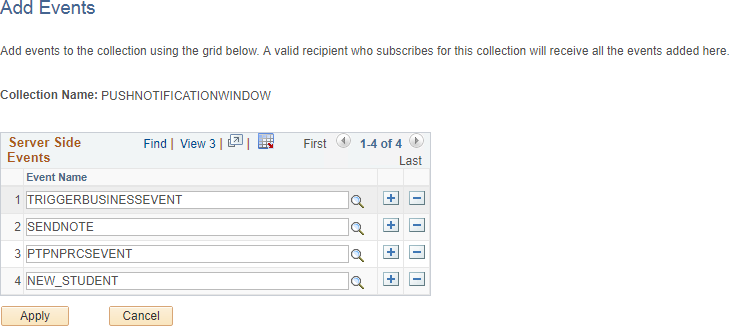
You use PeopleCode to publish an event:
For custom endpoint subscriptions, create an object of the PSEvent class to publish the event.
For certain delivered endpoint subscriptions, instantiate and use delivered applications classes to publish to that endpoint—for example, to the Notification window.
Publishing an Event for KeyValue Pairs
This example demonstrates the publishing of the event PROCESSSTATUSCHANGE with the keys PROCESSID and RUNSTATUS and recipient user, VP1.
Create the event object.
Local object &EventObject; &EventObject = CreateObject("PSEvent");
Set values for the keys defined in the event
&EventObject.SetKeyValuePair("PROCESSID", PSPRCSSTATUS.PROCESSID); &EventObject.SetKeyValuePair("STATUS", PSPRCSSTATUS.RUNSTATUS);
Add a recipient user for the event.
In this case, the event will be sent only to user VP1 (if VP1 has subscribed to the event).
&EventObject.AddRecipient("VP1", 1)
The the parameters for AddRecipient are:
Field or Control
Definition
recipient Specify the recipient using either a user name or a role name.
recipient type Use a value of 1 to indicate a user.
Use a value of 2 to indicate a role.
Publish the event.
&EventObject.Publish("PROCESSSTATUSCHANGE");
Publishing an Event for a PeopleCode Rowset
If the data type is PeopleCode rowset, you set the values for the fields in the record and add a recipient.
This example illustrates publishing an event for a PeopleCode rowset. In this example, WORKFLOWAPPROVAL is the server event for which name and status fields are defined. All users assigned the role of Workflow Administrator are added as recipients.
Create the event object.
Local object &EventObject; &EventObject = CreateObject("PSEvent");
Set the PeopleCode Rowset for the event.
Local object &EventObject; &rs = CreateRowset(Record.PSPTWKLAPPVL); &rs.InsertRow(&i); &rs(&i).PSPTWKLAPPVL.NAME.Value = "Test Workflow"; &rs(&i).PSPTWKLAPPVL.STATUS.Value = 1; &EventObject.SetRowsetData(&rs);
(Optional) Add a recipient role for the event.
In this case, the event will be sent only to users who have subscribed to the event and belong to the role: Workflow Administrator.
&EventObject.AddRecipient("Workflow Administrator", 2);
Publish the event.
&ret = &EventObject.publish("WORKFLOWAPPROVAL");
Publishing to the Notification Window
For example, the Notification window endpoint is delivered with a subscription to the PUSHNOTIFICATIONWINDOW event collection as seen previously on the Define Server Event Collections page. Once you have defined and added your custom event to the PUSHNOTIFICATIONWINDOW event collection, you can instantiate and use the PTPN_PUBLISH:PublishToWindow application class to publish your custom event to the Notification window.
The following PeopleCode excerpt illustrates the publication of a notification of type alert:
import PTPN_PUBLISH:PublishToWindow;
/* Creates and publishes an alert notification to the current user. */
Local string &KEY = "Student_ID" | PSU_STUDENT_TBL.STUDENT_ID.Value;
Local string &MSGINFO1;
&MSGINFO1 = "You added a new student named " | PSU_STUDENT_TBL.STUDENT_NAME.Value | " with ID " | PSU_STUDENT_TBL.STUDENT_ID.Value | ".";
Local PTPN_PUBLISH:PublishToWindow &newStdnt_alert = create PTPN_PUBLISH:PublishToWindow("NEW_STUDENT", "Student Data");
&newStdnt_alert.SetCategoryTypeFyi(); /* Type for alerts. */
&newStdnt_alert.AddRecipient(%UserId, 1);
&newStdnt_alert.SetMsgKey(&KEY);
&newStdnt_alert.SetMsgInfo(&MSGINFO1);
&newStdnt_alert.SetMsgStateNew();
&newStdnt_alert.SetPriority("3"); /* Low priority */
&newStdnt_alert.Publish("");
You can use one of several methodologies to subscribe an endpoint to an event or event collection:
Using JavaScript, the event data is published using the JavaScript Object Notation (JSON) format:
Subscribe to a specific event.
Subscribe to an event collection.
Using PeopleCode.
For custom endpoint subscriptions, create an object of the PSEvent class to subscribe to the event.
Using Interwindow Communication (IWC).
For a MAF application, creating and associating an adapter to an event.
Subscribing to an Event Using JavaScript
Use the Subscribe JavaScript function to subscribe an endpoint to an event:
Subscribe(EVENT_NAME, Callback_Function_Name)
Field or Control |
Definition |
---|---|
EVENT_NAME |
The name of the event that the endpoint is being subscribed to. |
Callback_Function_Name |
JavaScript function invoked when the event is published on the server. |
For example:
Subscribe("PRCSEVENTS", ProcessHandler);
The ProcessHandler JavaScript function is then defined in the code:
function ProcessHandler(EventName, EventData)
{
if(EventName == "PRCSEVENTS")
// Actual code omitted here ...
}
Subscribing to an Event Collection Using JavaScript
Use the SubscribeCollection JavaScript function to subscribe an endpoint to an event collection:
SubscribeCollection(COLLECTION_NAME, Callback_Function_Name)
Field or Control |
Definition |
---|---|
COLLECTION_NAME |
The name of the event collection that the endpoint is being subscribed to. |
Callback_Function_Name |
JavaScript function invoked when the event is published on the server. |
For example, the Notification window has been subscribed to the PUSHNOTIFICATIONWINDOW event collection using this JavaScript code:
SubscribeCollection("PUSHNOTIFICATIONWINDOW",UpdatePNUI);
The UpdatePNUI JavaScript function is defined as follows:
function UpdatePNUI(EventName, EventData) {
try {
var parentNode = document.getElementById('PT_NOTIFY');
var parentNode_classic=top.document.getElementById('pthdr2notify_div');
if (parentNode != null || parentNode_classic!=null) {
NewNotification(EventData);
}
} catch (e) {}
}
Subscribing to an Event Using PeopleCode
For custom endpoint subscriptions, create an object of the PSEvent class to subscribe to the event. Use the Subscribe method, which requires an application class method be defined as the callback:
APPLICATION_PACKAGE:ApplicationClass:MethodName
For example, the following code creates a subscription for the PRCSEVENTS event:
Local object &EventObject = CreateObject("PSEvent");
Local boolean &SubscriptionHandle = &EventObject.Subscribe("PRCSEVENTS", "PT_CONQRS:CQTEMPTABLES:OnEvent");
Then, implement an application class that defines the callback method for the event subscription:
method OnEvent
/+ &EventObject as Object +/
Local object &EventNotifObject = CreateObject("PSEvent");
/* Actual code omitted here */
end-method;
Subscribing to an Event Using Interwindow Communication (IWC)
Use IWC to subscribe a PeopleSoft page to a published event, where the event model is mapped to page elements, such as fields and grids. Creating a subscription does not require any changes to the page. When the event is published from the server, the page is updated automatically with the event data.
To subscribe to an event through IWC:
In the browser, select
.Navigate through the portal registry folders and select the content reference definition for the component.
Click theIWC Message Events link on the Content Ref Administration page.
Select the Add a New Value tab, and click Add.
Add a new row on the IWC Message Events page, specifying these values:
Event Name: Enter the name of a defined event.
Message Event Type: Select Server Sub.
Click the Map to display the Map Events Data to Page Elements page.
Image: Map Events Data To Page Elements Page
On the Map Events Data To Page Elements page you can map the data of a defined event to a page element.
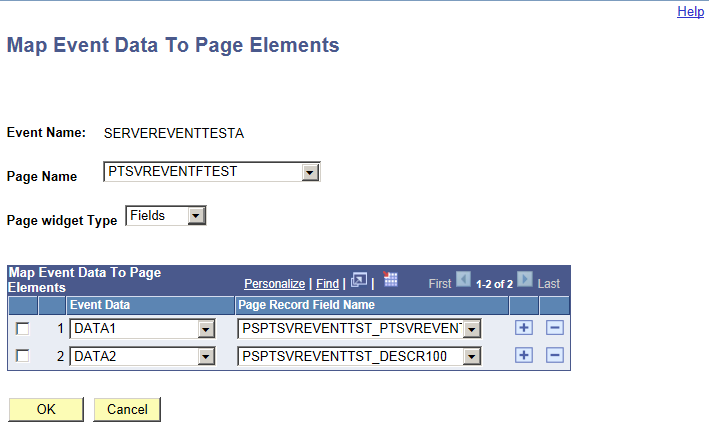
Field or Control |
Definition |
---|---|
Event Name |
Displays the event name that was entered on the Content Reference Message Events page. |
Page Name |
Select the page where you want the event subscription added. |
Page widget Type |
Select either Fields or Grid. |
Event Data |
Select one of the data elements defined for the event. The drop-down will display key values if the event data type is KeyValue Pair and record fields if the event data type is PeopleCode Rowset. |
Page Record Field Name |
Select the page record field name for the page element to be updated. |
Use these Application Data Set (ADS) definitions to copy event and collection definitions between databases.
Field or Control |
Definition |
---|---|
PTSVR_EVENTS |
ADS definition for events for copying event definitions and their corresponding IWC subscription configurations. |
PTPN_EVENT_COLLECTION |
ADS definition for event collections for copying both collection definitions as well as all the associated event definitions for that collection. |