Invoking View Trees From Application Pages
This section outlines the development steps and provides some sample code to view trees from an application page. It provides two methods of how to view trees from application pages:
Without multi-node selection.
With multi-node selection.
To view a tree from an application page:
Add the PSTREEVIEWERWRK page to your component as a hidden page.
Add a field to a work record that will then be used as a Command Button or Hyperlink to your secondary page.
The user selects this button or link to invoke the Tree Viewer page. You also need to add the following sample code to the FieldChange event for this field.
Add the Command Button or Hyperlink to the application page.
Add a Secondary Page control to the application page, and set the secondary page to PSTREEVIEWER.
The Secondary Page control must be placed on the page at a level higher than level 3.
In addition, the Command button or Hyperlink to invoke the PSTREEVIEWER secondary page must be placed on the same level as a secondary page control.
Add the application PeopleCode, which should do the following:
Set the values of the Tree’s key fields on the PSTREEVIEWWRK record.
Determine whether a node has been previously selected, and if so, setting the Tree_Node field to be the ID of the selected node:
If a leaf has been previously selected, your code should do the following:
— Populate the Range_From and Range_To fields with the selected leaf values
— Set the Tree_Node field to the parent node
— Set the Leaf_Selected field to "Y"
Set any of the display options that you want to use.
Display the Tree Viewer secondary page (PSTREEVIEWER) by calling the DoModal PeopleCode function.
Optionally, check the return code value and storing the ID of the selected node if the user selected a node and clicked the OK or Select button.
/* Note: Keys of Tree are stored in &SetId,&TreeName variables.
Assume that application has Leaf selected with values stored in variables &RangeFrom; &RangeTo and has parent node name stored in &TreeNode variable. QE_TREETEST_WRK Record holds input and received output values. */
/*Tree to open specification */
PSTREEVIEWWRK.SETID = &SETID;
PSTREEVIEWWRK.SETCNTRLVALUE = " ";
PSTREEVIEWWRK.TREE_NAME = &TREENAME;
PSTREEVIEWWRK.TREE_BRANCH = " ";
PSTREEVIEWWRK.EFFDT = %DATE; /* Get Latest Tree as of Today */
/* Tree appearance specification */
PSTREEVIEWWRK.PAGE_SIZE = 60;
PSTREEVIEWWRK.SHOW_LEAVES = "Y";
PSTREEVIEWWRK.SHOW_LEVELS = "Y";
PSTREEVIEWWRK.MULTINODESELECTION = "N";
/* Leaf input specification */
/* (Assuming QE_TREETEST_WK.LEAF_SELECTED ="Y"; */
PSTREEVIEWWRK.LEAF_SELECTED = QE_TREETEST_WRK.LEAF_SELECTED;
PSTREEVIEWWRK.TREE_NODE = &TreeNode;
PSTREEVIEWWRK.RANGE_FROM = &RangeFrom;
PSTREEVIEWWRK.RANGE_TO = &RangeTo;
/* Opening the PSTREEVIEWER secondary page */
&rslt = DoModal(Page.PSTREEVIEWER, " ", - 1, - 1);
/* populating the application Record (QE_TREETEST_WRK) with output values from user selection in Tree */
If &rslt = 1 Then
QE_TREETEST_WRK.TREE_NODE = PSTREEVIEWWRK.TREE_NODE;
QE_TREETEST_WRK.TREE_LEVEL_NUM = PSTREEVIEWWRK.TREE_LEVEL_NUM;
QE_TREETEST_WRK.TREE_LEVEL = PSTREEVIEWWRK.TREE_LEVEL;
QE_TREETEST_WRK.TREE_LEVEL_DESCR = PSTREEVIEWWRK.TREE_LEVEL_DESCR;
QE_TREETEST_WRK.RANGE_FROM = PSTREEVIEWWRK.RANGE_FROM;
QE_TREETEST_WRK.RANGE_TO = PSTREEVIEWWRK.RANGE_TO;
QE_TREETEST_WRK.DYNAMIC_FLAG = PSTREEVIEWWRK.DYNAMIC_FLAG;
QE_TREETEST_WRK.MESSAGE_SET_NBR = PSTREEVIEWWRK.MESSAGE_SET_NBR;
QE_TREETEST_WRK.MESSAGE_NBR = PSTREEVIEWWRK.MESSAGE_NBR;
End-If;
The following is the sample PeopleCode (Method A), which would be part of the FieldChange event triggered from a Command Button or Hyperlink command on the application page:
In some cases, you may need to use the Component Level Record variable &cPSTREEVIEWWRK to set values for the tree. For example, if the application added the Tree Viewer secondary page to the application’s secondary page and cannot reach the record from the component buffer. The following is the sample PeopleCode illustrating the use of the variable:
Component Record &cPSTREEVIEWWRK;
Component boolean &gbShowTreeLeaves;
Local number &rslt;
/* opening the Tree Viewer secondary page */
&cPSTREEVIEWWRK = CreateRecord(Record.PSTREEVIEWWRK);
&cPSTREEVIEWWRK.SETID.Value = &SETID;
&cPSTREEVIEWWRK.SETCNTRLVALUE.Value = " ";
&cPSTREEVIEWWRK.TREE_NAME.Value = &TREENAME;
&cPSTREEVIEWWRK.TREE_BRANCH.Value = " ";
&cPSTREEVIEWWRK.EFFDT.Value = %DATE; /* Get Latest Tree as of Today */;
&cPSTREEVIEWWRK.PAGE_SIZE.Value = 60;
&cPSTREEVIEWWRK.SHOW_LEVELS.Value = "Y";
&cPSTREEVIEWWRK.MULTINODESELECTION.Value = "N";
If &gbShowTreeLeaves Then
&cPSTREEVIEWWRK.SHOW_LEAVES.Value = "Y";
Else
&cPSTREEVIEWWRK.SHOW_LEAVES.Value = "N";
End-If;
&rslt = DoModal(Page.PSTREEVIEWER, "", - 1, - 1);
/* reading output value in a case when Component Level Record variable &cPSTREEVIEWERWRK is used. */
If &rslt = 1 Then
QE_TREETEST_WRK.TREE_NODE = &cPSTREEVIEWWRK.TREE_NODE.value;
QE_TREETEST_WRK.TREE_LEVEL_NUM = &cPSTREEVIEWWRK.TREE_LEVEL_NUM.value;
QE_TREETEST_WRK.TREE_LEVEL = &cPSTREEVIEWWRK.TREE_LEVEL.value;
QE_TREETEST_WRK.TREE_LEVEL_DESCR = &cPSTREEVIEWWRK.TREE_LEVEL_DESCR.value;
QE_TREETEST_WRK.RANGE_FROM = &cPSTREEVIEWWRK.RANGE_FROM.value;
QE_TREETEST_WRK.RANGE_TO = &cPSTREEVIEWWRK.RANGE_TO.value;
QE_TREETEST_WRK.DYNAMIC_FLAG = &cPSTREEVIEWWRK.DYNAMIC_FLAG.value;
QE_TREETEST_WRK.MESSAGE_SET_NBR = &cPSTREEVIEWWRK.MESSAGE_SET_NBR.value;
QE_TREETEST_WRK.MESSAGE_NBR = &cPSTREEVIEWWRK.MESSAGE_NBR.value;
EndModal(1);
Else
EndModal(0);
End-If;
Note: The name of the variable &cPSTREEVIEWWRK is hard-coded and should not be changed.
The segment of code in italics reads the results that came from the tree. (Node or leaf selected).
An example of an application that uses the Tree Viewer secondary page (PSTREEVIEWER) with the Multinodeselection flag set to "N" is the Copy/Delete Tree (PSTREEMAINT) component.
To view the Tree Viewer secondary page from the Copy/Delete Tree page:
Select
Select a tree.
Click the View button.
Image: Tree Viewer secondary page without multi-node selection
The following example shows the QE_PERS_DATA tree as viewed on the Tree Viewer secondary page without multi-node selection:
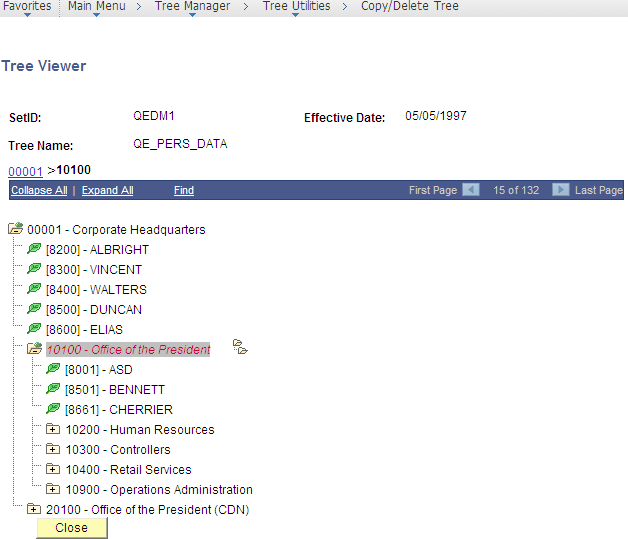
An example of an application that uses the PSTREEVIEWER secondary page with the Multinodeselection flag set to "Y" is the Query Manager component.
To view the Tree Viewer secondary page from Query Manager:
Select
Click the Edit link for any query.
Click the Add Criteria icon button.
Select Field as the first expression.
Select in tree as the condition type.
Click the New Node List link.
Select a tree to display.
Use the page fields and tree widgets to select multiple tree nodes.
Once all tree nodes have been selected, click the OK button.
Image: Tree Viewer secondary page with multi-node selection
The following example shows the QE_PERS_DATA tree as viewed on the Tree Viewer secondary page with multi-node selection:
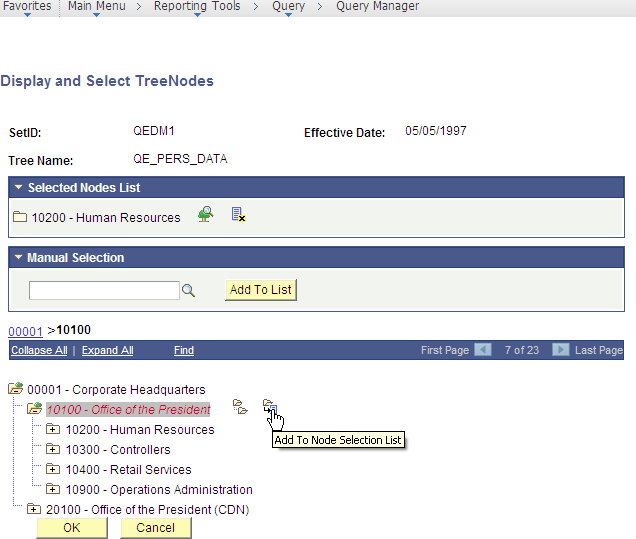
The Tree Viewer secondary page (PSTREEVIEWER) in Method B has a frame that holds the Selected Nodes List with action buttons associated with each selected node. This page is used to select the set of nodes, return them to the calling application (Query Manager), and use the list of nodes as Query criteria.
Once all nodes have been selected, the selected tree setID, tree name, effective date, and selected nodes display in the Select Tree Node List group box. The list of selected nodes can also be read from the Multinode field of the PSTREEVIEWWRK work record as a comma-separated string. The string can be parsed to get the node names.