Business Interlink Class Methods
In this section, we discuss the Business Interlink class methods. The methods are discussed in alphabetical order.
Syntax
AddDoc(docname)
Description
The AddDoc method adds a document to an input structure. The added document is an input parameter for a Business Interlink object that is not of simple type (such as integer or string). You must add the document to the input structure before you can add values to its members with AddValue.
Parameters
Field or Control |
Definition |
---|---|
docname |
The name of the document that AddDoc adds to the structure. |
Returns
The document added to the BIDocs input document.
Example
In the following example, the input structure for Calculate Cost, or the root level document, is created with the GetInputDocs method. (To create, or add, more input structures, use AddNextDoc.) The Calculate Cost input parameter From is a document, so the AddDoc method adds it to the input document.
Local Interlink &QE_COST;
Local BIDocs &CalcCostIn;
Local BIDocs &FromDoc, &ToDoc, &PackageDoc, &AccountDoc;
Local number &ret, &retinput;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
&CalcCostIn = &QE_COST.GetInputDocs("");
&ret = &CalcCostIn.AddValue("input_param1","value");
&FromDoc = &CalcCostIn.AddDoc("From");
&ret = &FromDoc.AddValue("OriginCountry", "United States");
&ret = &FromDoc.AddValue("OriginPostal_Code", &ORIGIN);
&ret = &FromDoc.AddValue("Ship_Date", &SHIPDATE);
/* Call AddDoc and AddValue for To, Package_Info, and Account_Info_List (code not shown) */
Image: Example input structure
The following shows the input structure for this example. It contains five input parameters: input_param1, From, To, Package_Info, and Account_Info_List. This is a version of the Federal Express plug-in that was modified for this example (input_param1 was added, Account_Info was modified to be a list).
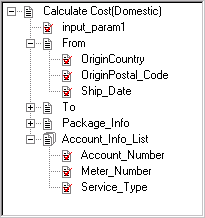
Syntax
AddInputRow(inputname, value)
where inputname and value are in matched pairs, in the form:
inputname1, value1 [, inputname2, value2] . . .
Description
The AddInputRow method adds a row of input data (value) from PeopleCode variables or record fields for the Business Interlink object executing the method. These must be entered in matched pairs, that is, every input name must be followed by its matching value.
Note: The input name, not the input path, of the interface definition is used for this method.
There must be an input name for every input parameter defined in the interface definition used to instantiate the Business Interlink object.
If you specify a record field that is not part of the record the PeopleCode program is associated with, you must use recname..fieldname for that value.
You can specify default values for every input name in the interface definition (created in Application Designer.) These values are used if you create a PeopleCode "template" by dragging the interface definition from the Project window in Application Designer to an open PeopleCode editor window.
Parameters
Field or Control |
Definition |
---|---|
inputname |
Specify the input name. There must be one inputname for every input name defined in the interface definition used to instantiate the Business Interlink object. |
value |
Specify the value for the input name. This can be a constant, a variable, or a record field. Each value must be paired with an inputname. |
Returns
A Boolean value: True if the input values were successfully added. Otherwise, it returns False.
Example
In the following example, the Business Interlink object name is SRA_ALL_1, and the input name, such as ship_site_name, are being bound to record fields, such as QE_RP_SITENAME or VENDOR_INFO.QE_RP_PROMISEDATE, to variables like &PARTNAME, or to literals, like 10 for quantity.
&SRA_ALL_1.AddInputRow("ship_site_name", QE_RP_SITENAME,
"promise_date", VENDOR_INFO.QE_RP_PROMISEDATE,
"request_date", QE_RP_ORDERREQDATE,
"subline_site_name", QE_RP_SITENAME,
"quantity", 10,
"part_name", &PARTNAME,
"site_name", INV_LOCATIONS.QE_RP_SITENAME);
Syntax
AddNextDoc()
Description
The AddNextDoc method adds a document to one of the following levels:
The root level of the input structure for a Business Interlink object. You create a reference to this level with the GetInputDocs method.
When a document within the input structure is a list, AddNextDoc adds another document to the list. If you use AddNextDoc on a document that is not a list, AddNextDoc fails and returns an error.
Parameters
None.
Returns
This method returns an integer. The values are:
Value |
Description |
---|---|
0 |
eNoError. The method succeeded. |
1 |
eNO_DOCUMENT. The document referenced by this method does not exist. |
2 |
eDOCLIST_OUT_RANGE. You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
eDOCUMENT_UNINITIALIZED. Internal error. |
4 |
eNOT_DOCUMENTTYPE. You tried to perform an operation upon a parameter that is not a document type. |
5 |
eNOT_LISTTYPE. You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
7 |
eNOT_BASICTYPE. You tried to perform a GetValue or AddValue upon a parameter that is not of basic type: Integer, Float, String, Time, Date, DateTime. |
8 |
eNO_DATA. You tried to retrieve data from a document that contained no data. |
Example
In this example, the input structure for Calculate Cost, or the root level document, is accessed with the GetInputDocs method. The AddNextDoc method adds another BIDocs input document. The Calculate Cost input parameter Account_Info_List is a document, so the AddDoc method adds it to the BIDocs input document. Account_Info_List is also a document list, so AddNextDoc method adds another Account_Info_List document.
Local Interlink &QE_COST;
Local BIDocs &CalcCostIn;
Local BIDocs &FromDoc, &ToDoc, &PackageDoc, &AccountDoc;
Local number &ret, &retinput;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
&CalcCostIn = &QE_COST.GetInputDocs("");
For &n = 1 to &number_of_input_sets
/* Get values for inputs, such as &ORIGIN (code not shown) */
&ret = &CalcCostIn.AddValue("input_param1","value");
&FromDoc = &CalcCostIn.AddDoc("From");
&ret = &FromDoc.AddValue("OriginCountry", "United States");
&ret = &FromDoc.AddValue("OriginPostal_Code", &ORIGIN);
&ret = &FromDoc.AddValue("Ship_Date", &SHIPDATE);
/* Call AddDoc and AddValue for To and Package_Info
(code not shown) */
/* Call AddDoc and AddNextDoc for the AccountDoc document list */
&AccountDoc = &CalcCostIn.AddDoc("Account_Info_List");
For &m = 1 to &number_of_AccountDocs
&ret = &AccountDoc.AddValue("Account_Number", &ACCOUNT);
&ret = &AccountDoc.AddValue("Meter_Number", &METER);
&ret = &AccountDoc.AddValue("Service_Type", &SVCTYPE);
&retinput = &AccountDoc.AddNextDoc();
End-For; &retinput = &CalcCostIn.AddNextDoc();
End-For;
&retinput = &To.AddNextDoc();
Image: Example input structure
The following shows the input structure for this example. It contains five input parameters: input_param1, From, To, Package_Info, and Account_Info_List.
From, To, and Package_Info are not lists, so if you try to use AddNextDoc with these parameters, such as the following line of code, AddNextDoc will fail and return an error message.
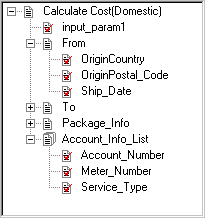
Syntax
AddValue(paramname, value)
Description
The AddValue method adds a value to a member of a document within the input structure for a Business Interlink object.
Parameters
Field or Control |
Definition |
---|---|
paramname |
The name of the member of the document that is having a value added to it. |
value |
The value that is added. This can be a constant, a variable, or a record field. The data type can be String, Integer, Double, Float, Time, Date, or DateTime. |
Returns
This method returns an integer. The values are:
Value |
Description |
---|---|
0 |
eNoError. The method succeeded. |
1 |
eNO_DOCUMENT. The document referenced by this method does not exist. |
2 |
eDOCLIST_OUT_RANGE. You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
eDOCUMENT_UNINITIALIZED. Internal error. |
4 |
eNOT_DOCUMENTTYPE. You tried to perform an operation upon a parameter that is not a document type. |
5 |
eNOT_LISTTYPE. You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
7 |
eNOT_BASICTYPE. You tried to perform a GetValue or AddValue upon a parameter that is not of basic type: Integer, Float, String, Time, Date, DateTime. |
8 |
eNO_DATA. You tried to retrieve data from a document that contained no data. |
Example
In the following example, the Business Interlink object name is QE_COST.
In the following example, the input structure for Calculate Cost, or the root level document, is accessed with the GetInputDocs method. (To create, or add, more input structures, use AddNextDoc.) The Calculate Cost input parameter From is a document, so the AddDoc method adds it to the input structure. Then the AddValue method adds values to each of the From document members.
Local Interlink &QE_COST;
Local BIDocs &CalcCostIn;
Local BIDocs &FromDoc, &ToDoc, &PackageDoc, &AccountDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
/* Get some values for input, such as &ORIGIN (code not shown) */
&CalcCostIn = &QE_COST.GetInputDocs("");
&ret = &CalcCostIn.AddValue("input_param1","value");
&FromDoc = &CalcCostIn.AddDoc("From");
&ret = &FromDoc.AddValue("OriginCountry", "United States");
&ret = &FromDoc.AddValue("OriginPostal_Code", &ORIGIN);
&ret = &FromDoc.AddValue("Ship_Date", &SHIPDATE);
/* Call AddDoc, AddValue for Package, Account_Info_List, and also call AddNextDoc for Account_Info_List (code not shown) */
Image: Example input structure
The following shows the input structure for this example. It contains five input parameters: input_param1, From, To, Package_Info, and Account_Info_List.
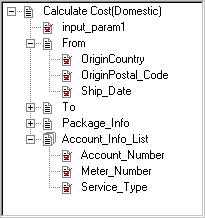
Syntax
BulkExecute(RECORD.inputrecname [, RECORD.outputrecname] [, {user_process_inst | user_operid}])
Description
The BulkExecute method uses the data in the specified record to populate the input buffer, copying like-named fields. Then the method executes, and, optionally, fills the record specified by outputrecname with data from the Business Interlink output buffer. BulkExecute results in significantly faster performance for transactions that process large amounts of data. Instead of adding one input row at a time, then fetching the values one at a time, you might write the data to a staging table, use the BulkExecute method and then read the data from the output table. This would be especially effective in Application Engine programs that process sets of data rather than individual rows.
This method assumes that the names of the fields in the record match the names of the inputs (or outputs) defined in the Business Interlink Definition. If there is no field in the inputrecname for a Business Interlink input parameter, the parameter’s default value is used. If no default is specified, an empty string is passed. You must ensure that this default value is legitimate.
Fields in the output buffer are matched against the fields specified in the output record. You do not have to specify an output record. If you don’t specify an output record, the output buffers are not be populated.
If you specify an output record, and if you have fields in the output record that are not specified as output parameters, they aren't populated with the BulkExecute method. In addition, they shouldn’t be set as NOT NULL, otherwise inserts fail.
Note: Before you use this method, you should flush the record used for output and remove any residual data that might exist in it.
If you specify an output record, and you want to use the output record for error checking, you must add the following fields to your output record:
RETURN_STATUS
RETURN_STATUS_MSG
Note: The field names in the output record must match these names exactly.
Then you must mark one or more input parameters as "key fields" in the Business Interlink definition (using the BulkExecute ID check box.) The value of these key fields are copied to the output record, and you can use these fields to match error messages with input (or output) rows.
To order your input (and output) rows, you must add the BI_SEQ_NUM column to both the input and output table:
Note: The field name in your input and output records must match this name exactly.
The input rows are read in the order of numbers in BI_SEQ_NUM, and the output rows are generated using the same order number.
This method automatically executes, so you don’t need to use the Execute method with this method.
Whether this method halts on execution depends on the setting of the StopAtError configuration parameter. The default value is True, that is, stop if the error number returned is something other than a 1 or 2. This configuration parameter must be set before using the BulkExecute method.
Parameters
Field or Control |
Definition |
---|---|
RECORD.inputrecname |
Specify a record (SQL table) that contains the data to populate the input buffer of the Business Interlink. |
RECORD .outputrecname |
Specify a record (SQL table) that will hold the data that populates the output buffer of the Business Interlink. This is optional; it is often used to error check the input. |
user_process_inst | user_operid |
This is an optional parameter that allows either different Application Engine programs or different clients to populate the same RECORD. outputrecname at the same time. |
For user_process_inst, the parameter takes an integer.RECORD. outputrecname must have a PROCESS_INSTANCE field. The PROCESS_INSTANCE field is used to identify the Application Engine program that is using this Business Interlink. You can use the %PROCESS_INSTANCE variable to populate user_process_inst. |
|
For user_operid, the parameter takes a string.RECORD. outputrecname must have an OPERID field. The OPERID field is used to identify the client who is using this Business Interlink. You can use the %OPERID variable to populate user_operid. |
Returns
The following are the valid returns:
Value |
Description |
---|---|
1 |
The Business Interlink object executes successfully |
2 |
The Business Interlink object failed to execute |
3 |
Transaction failed |
4 |
Query failed |
5 |
Missing criteria |
6 |
Input mismatch |
7 |
Output mismatch |
8 |
No response from server |
9 |
Missing parameter |
10 |
Invalid username |
11 |
Invalid password |
12 |
Invalid server name |
13 |
Connection error |
14 |
Connection refused |
15 |
Timeout reached |
16 |
Unequal lists |
17 |
No data for output |
18 |
Output parameters empty |
19 |
Driver not found |
20 |
Internet connect error |
21 |
XML parser error |
22 |
XML deserialize |
Example
Image: Example input record for BulkExecute
This image is an example of input record for BulkExecute. Here are two PeopleSoft records that could be used as input and output records for BulkExecute. The key fields in the input record, which need to have the BulkExecute ID check box checked in Application Designer, are QE_RP_PO_NUMBER and QE_RP_SITENAME. These key fields are used both for input and output.
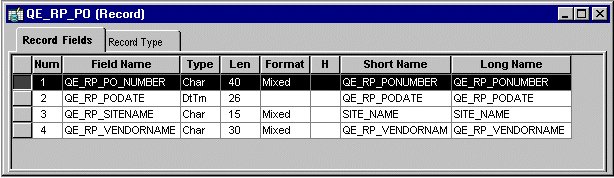
Image: Example output record for BulkExecute
This image is an example of output record for BulkExecute.
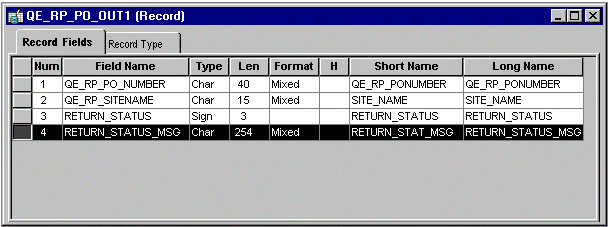
Image: Example Business Interlink inputs for BulkExecute
The following is an example of Business Interlink inputs for BulkExecute. Here are the corresponding inputs and outputs for a Business Interlink that has had its inputs and outputs renamed to match the field names in the records. The inputs and outputs have been renamed where necessary to match the record field names.
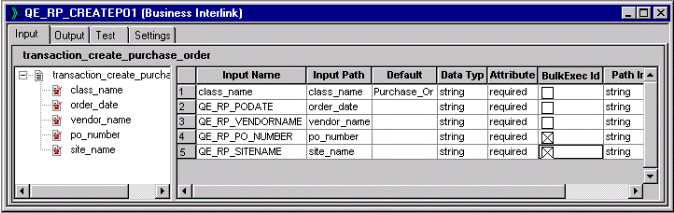
Image: Example Business Interlink inputs for BulkExecute
The following image is an example of Business Interlink inputs for BulkExecute.
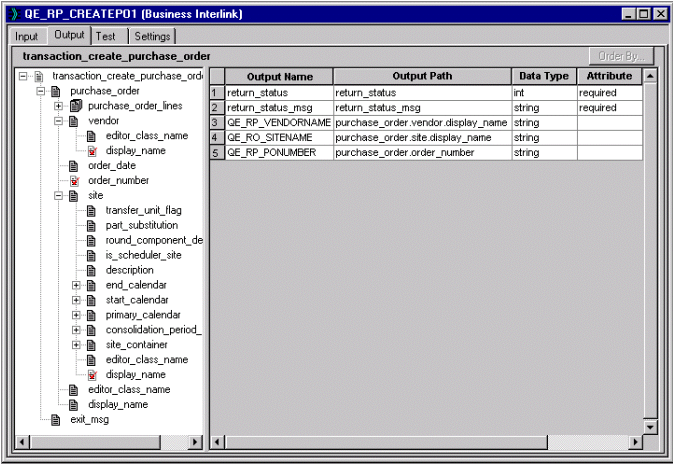
The PeopleCode program using these records could contain the following code to run BulkExecute.
Local Interlink &CREATE_PO;
Local number &EXECRSLT;
&CREATE_PO = GetInterlink(INTERLINK.QE_RP_CREATEPO1);
/* The next three lines set configuration parameters, which are not shown in the previous examples. */
&CREATE_PO.SERVER_NAME = "bc1";
&CREATE_PO.RSERVER_HOST = "192.0.2.1";
&CREATE_PO.RSERVER_PORT = "2200";
&EXECRSLT = &CREATE_PO.BulkExecute(RECORD.QE_RP_PO, RECORD.QE_RP_PO_OUT1);
Syntax
Clear()
Description
The Clear method clears the input and output buffers. If you're using Business Interlinks in a batch program, every time after you use the Execute method, use the Clear method to flush out the input and output buffers.
Parameters
None.
Returns
None.
Example
For &n = 1 to x
&myinterlink.AddInputRow("input1", value1, "input2", value2);
&myinterlink.Execute();
&myinterlink.FetchNextRow("output1", value1, "output2", value2);
/* do processing on data */
&myinterlink.Clear();
&n = &n + 1;
End-for;
Syntax
Execute()
Description
When an Interlink object executes the Execute method, the transaction associated with the Interlink object is executed.
If there is only one row, after appropriate substitution is made, the transaction is executed only once. Otherwise, the data is "batched up" and sent once. You have to call Execute only once to execute all the rows of the input buffer.
Generally, you would only use the Execute method after using the AddInputRow method. If you create a PeopleCode "template" by dragging the Business Interlink definition from the Project window in Application Designer to an open PeopleCode editor window, the usual order of the execution of methods is shown in the template.
If you're using Business Interlinks in a batch program, every time after you use the Execute method, use the Clear method to flush out the input and output buffers.
Whether this method halts on execution depends on the setting of the StopAtError configuration parameter. The default value is True, that is, stop if the error number returned is something other than a 1 or 2. This configuration parameter must be set before using the Execute method.
Parameters
None.
Returns
The following are the valid returns:
Value |
Description |
---|---|
1 |
The Business Interlink object executes successfully |
2 |
The Business Interlink object failed to execute |
3 |
Transaction failed |
4 |
Query failed |
5 |
Missing criteria |
6 |
Input mismatch |
7 |
Output mismatch |
8 |
No response from server |
9 |
Missing parameter |
10 |
Invalid username |
11 |
Invalid password |
12 |
Invalid server name |
13 |
Connection error |
14 |
Connection refused |
15 |
Timeout reached |
16 |
Unequal lists |
17 |
No data for output |
18 |
Output parameters empty |
19 |
Driver not found |
20 |
Internet connect error |
21 |
XML parser error |
22 |
XML deserialize |
Example
Local number &EXECRSLT;
. . .
&EXECRSLT = &SRA_ALL_1.Execute();
If (&EXECRSLT <> 1) Then
/* The instance failed to execute */
/* Do Error Processing */
End-If;
Syntax
FetchIntoRecord(RECORD.recname, [, {user_process_inst | user_operid}])
Description
The FetchIntoRecord method copies the data from the output buffer into the specified record (SQL table) copying like-named fields. This method assumes that the names of the fields in the record match the names of the outputs defined in the Business Interlink definition.
You can use the FetchIntoRecord method to perform a transaction on a large amount of data that you want to receive from your system. Instead of executing your Business Interlink and then fetching one output row at a time, you might execute your Business Interlink, then use the FetchIntoRecord method to write the data returned from the Business Interlink to a staging table.
Note: Before you use this method, you should flush the record used for output and remove any residual data that might exist in it.
If your data is hierarchical, that is, in a rowset, and you want to preserve the hierarchy, use FetchIntoRowset instead of this method.
To order your output rows, you must add the BI_SEQ_NUM column to the record.
This column is populated with a sequence number that corresponds to the order in which each row of the record was written to by the method.
Parameters
Field or Control |
Definition |
---|---|
RECORD. recname |
Specify a record (SQL table) that you want to populate with data from the output buffers. |
user_process_inst | user_operid |
This is an optional parameter that enables either different Application Engine programs or different clients to populate the same RECORD. outputrecname at the same time. For user_process_inst, the parameter takes an integer.RECORD. outputrecname must have a PROCESS_INSTANCE field. The PROCESS_INSTANCE field is used to identify the Application Engine program that is using this Business Interlink. You can use the %PROCESS_INSTANCE variable to populate user_process_inst. For user_operid, the parameter takes a string.RECORD. outputrecname must have an OPERID field. The OPERID field is used to identify the client who is using this Business Interlink. You can use the %OPERID variable to populate user_operid. |
Returns
Number of rows fetched if one or more non-empty rows are returned.
If an empty row is returned, that is, every if field is empty except the return_status and return_status_msg fields, this method returns one of the following error messages:
Number |
Meaning |
---|---|
0 |
No error |
1 |
NoInterfaceObject |
2 |
ParamCountError |
3 |
IncorrectParameterType |
4 |
NoColumnForOutputParm |
5 |
NoColumnForInputParm |
6 |
BulkInsertStartFailed |
7 |
BulkInsertStopFailed |
8 |
CouldNotCreateSelectCursor |
9 |
CouldNotCreateInsertCursor |
10 |
CouldNotDestroySelectCursor |
11 |
CouldNotDestroyInsertCursor |
12 |
InputRecordDoesNotExist |
13 |
OutputRecordDoesNotExist |
14 |
ContainEmptyRow |
15 |
SQLExecError |
201 |
FieldTooLarge |
202 |
IgnoreSignNumber |
203 |
ConvertFloatToInt |
Example
&RSLT = &QE_SM_CONCAT.FetchIntoRecord(RECORD.PERSONAL__VENDR_DATA);
If &RSLT = 0 Then
/* no rows fetched */
Else
/* do processing */
End-If;
Syntax
FetchIntoRowset(&Rowset)
Description
The FetchIntoRowset method copies the data from the output buffer into the specified rowset, copying like-named fields. This method assumes that the names of the fields in the rowset match the names of the outputs defined in the Business Interlink definition, and that the structure is the same.
Note: Before you use this method, you should flush the rowset used for output and remove any residual data that might exist in it.
Use this method only if you have a hierarchical data structure and you want to preserve the hierarchy. Otherwise, use BulkExecute or FetchIntoRecord.
Parameters
Field or Control |
Definition |
---|---|
&Rowset |
Specify rowset object that you want to populate with data from the output buffers. This must be an existing, instantiated rowset. |
Returns
Number of rows fetched if one or more non-empty rows are returned.
If an empty row is returned, that is, every if field is empty except the return_status and return_status_msg fields, this method returns one of the following error messages:
Value |
Description |
---|---|
0 |
No error |
1 |
NoInterfaceObject |
2 |
ParamCountError |
3 |
IncorrectParameterType |
4 |
NoColumnForOutputParm |
5 |
NoColumnForInputParm |
6 |
BulkInsertStartFailed |
7 |
BulkInsertStopFailed |
8 |
CouldNotCreateSelectCursor |
9 |
CouldNotCreateInsertCursor |
10 |
CouldNotDestroySelectCursor |
11 |
CouldNotDestroyInsertCursor |
12 |
InputRecordDoesNotExist |
13 |
OutputRecordDoesNotExist |
14 |
ContainEmptyRow |
15 |
SQLExecError |
201 |
FieldTooLarge |
202 |
IgnoreSignNumber |
203 |
ConvertFloatToInt |
Example
Image: EMPLOYEE_CHECKLIST Page order
The following an image of EMPLOYEE_CHECKLIST Page order. The example uses the rowset on level one from the EMPLOYEE_CHECKLIST page. A PeopleCode program running in a field on level zero in that page can access the level one (child rowset).
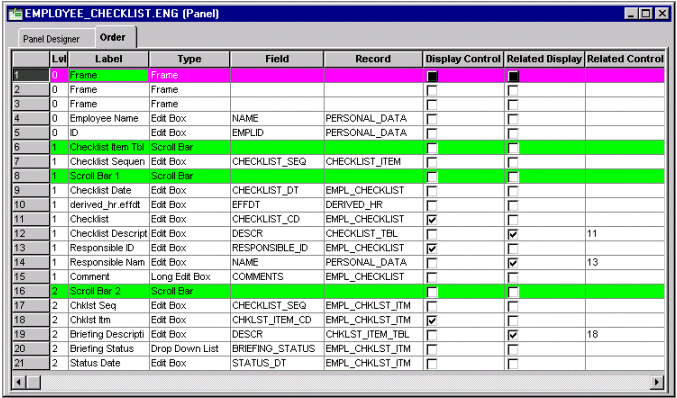
EMPL_CHECKLIST is the primary database record for the level one scrollbar on the EMPLOYEE_CHECKLIST page. The following PeopleCode accesses the level one rowset using EMPL_CHECKLIST. The Business Interlink object name is QE_BI_EMPL_CHECKLIST. This Business Interlink object uses the level one rowset as its input and its output.
The InputRowset method uses this rowset as input for QE_BI_EMPL_CHECKLIST. Then a blank duplicate of the rowset is created with CreateRowset, and then the output of QE_BI_EMPL_CHECKLIST is fetched into the blank rowset with FetchIntoRowset.
&MYROWSET = GetRowset(SCROLL.EMPL_CHECKLIST);
&ROWCOUNT = &QE_BI_EMPL_CHECKLIST.InputRowset(&MYROWSET);
&RSLT = &QE_BI_EMPL_CHECKLIST.Execute();
/* do some error processing */
&WorkRowset = CreateRowset(&MYROWSET);
&ROWCOUNT = &QE_BI_EMPL_CHECKLIST.FetchIntoRowset(&WorkRowset);
If &ROWCOUNT = 0 Then
/* do some error processing */
Else
/* Process the rowset from QE_BI_EMPL_CHECKLIST. Check it for errors. */
For &I = 1 to &WorkRowset.RowCount
For &K = 1 to &WorkRowset(&I).RecordCount
&REC = &WorkRowset(&I).GetRecord(&K);
&REC.ExecuteEdits();
For &M = 1 to &REC.FieldCount
If &REC.GetField(&M).EditError Then
/* there are errors */
/* do other processing */
End-If;
End-For;
End-For;
End-for;
End-if;
Syntax
FetchNextRow(outputname, value)
where outputname and value are in matched pairs, in the form:
outputname1, value1 [, outputname2, value2] . . .
Description
After the Business Interlink object executes the method Execute, the FetchNextRow method can be used to retrieve a row of output and store the values of the output name (outputname) to PeopleCode variables or record fields (value). These must be entered in matched pairs, that is, every output name must be followed by its matching value.
Note: The output name, not the output path of the interface definition is used for this method.
There must be an outputname for every output name defined in the interface definition used to instantiate the Business Interlink object.
If you specify a record field that is not part of the record the PeopleCode program is associated with, you must use recname.fieldname for that value.
You can specify default values for every output name in the interface definition (created in Application Designer.) These values are used if you create a PeopleCode "template" by dragging the interface definition from the Project window in Application Designer to an open PeopleCode Editor window.
Parameters
Field or Control |
Definition |
---|---|
outputname |
Specify the output name. There must be one outputname for every output name defined in the interface definition used to instantiate the Business Interlink object. |
value |
Specify the value for the output name. This can be a constant, a variable, or a record field. Each value must be paired with an outputname. |
Returns
A Boolean value: True if the row of output parameters was fetched. Otherwise, it returns False.
Example
In the following example, the Business Interlink object name is SRA_ALL_1, and the output names, such as costs, are being bound to record fields, such as STR_COST.
&RSLT = &SRA_ALL_1.FetchNextRow("costs", &STR_COST,
"unit_costs", &STR_UNIT_COST,
"customer_ship_dates", &STR_SHIP_DATE,
"quantities", &STR_QUANTITY,
"so_numbers", &STR_SO_NUM,
"so_names", &STR_SO_NAME,
"line_numbers", &STR_LINE_NUM,
"ship_sets", &STR_SHIP_SET,
"customer_receipt_dates", &STR_RECPT_DATE);
Syntax
GetCount(docname)
Description
The GetCount method returns the number of documents within a document list contained within an output structure for a Business Interlink object.
Parameters
Field or Control |
Definition |
---|---|
docname |
The name of the document list. |
Returns
The number of documents in the list.
Example
In the following example, the output structure for Calculate Cost, or the root level document, is accessed with the GetOutputDocs method. The Calculate Cost output parameter output_param2_List is a document, so the GetDoc method gets it from the output structure. Because output_param2_List is a document list, GetCount gets the number of documents in the list.
Local Interlink &QE_COST;
Local number &count;
Local BIDocs &CalcCostOut;
Local BIDocs &OutlistDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
/* Get inputs, execute. (code not shown) */
&CalcCostOut = &QE_COST.GetOutputDocs("");
/* Call GetValue for output_param1, call GetDoc, GetValue for
Service_Rate (code not shown) */
&OutlistDoc = &CalcCostOut.GetDoc("output_param2_List");
&count = &CalcCostOut.GetCount("output_param2_List");
&I = 0;
While (&I < &count)
&ret = &OutlistDoc.GetValue("output_member1", &VALUE1);
&ret = &OutlistDoc.GetValue("output_member2", &VALUE2);
If &ret = 0 Then
/* Process output values */
&I = &I + 1;
&retoutput = &OutlistDoc.GetNextDoc();
End-If;
End-While;
Image: Example output structure
The following example shows the output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List.
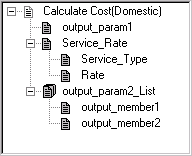
Syntax
GetDoc(docname)
Description
The GetDoc method gets a document from an output structure. The document is an output parameter for a Business Interlink object that is not of simple type (such as integer or string). You must get the document from the output structure before you can get values from its members with GetValue.
You can call GetDoc using dot notation, to access documents that are "nested" within other documents. For example, the following accesses a document nested three levels deep:
&Docs3 = &CalcCostOut.GetDoc("Doc1.Doc2.Doc3");
Parameters
Field or Control |
Definition |
---|---|
docname |
The name of the document that GetDoc should get from the output structure. |
Returns
A reference to the document received from the output structure.
Example
In the following example, the Output structure for Calculate Cost(Domestic), or the root level document, is created with the GetOutputDocs method. (To create, or get, more Output structures, call GetNextDoc.) The Calculate Cost output parameter Service_Rate is a document, so the GetDoc method gets it from the Output structure.
Local Interlink &QE_COST;
Local number &count;
Local BIDocs &CalcCostOut;
Local BIDocs &ServiceRateDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
&ret = &CalcCostOut.GetValue("output_param1",&VALUE);
&ServiceRateDoc = &CalcCostOut.GetDoc("Service_Rate");
&ret = &ServiceRateDoc.GetValue("Service_Type", &SERVICE_TYPE);
&ret = &ServiceRateDoc.GetValue("Rate", &RATE);
/* Call GetDoc, GetValue, GetNextDoc for output_param2_List (code not shown) */
If &ret = 0 Then
/* Process output values */
End-If;
Image: Example output structure
The following illustration shows the Output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List. This is a version of the Federal Express plug-in that was modified for this example (output_param1 and output_param2_List were added).
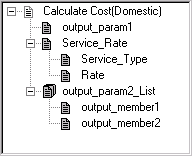
In the following example, GetDoc is used to access a document that is nested more deeply. To access a document that is more deeply nested, you can either call GetDoc for each nesting, or you can call GetDoc once using the nesting feature.
Calling GetDoc with the nesting feature:
Local Interlink &QE_4GETDOC;
Local BIDocs &CalcCostOut, &Docs3;
Local number &ret;
&QE_4GETDOC = GetInterlink(Interlink.QE_4GETDOC_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_4GETDOC.GetOutputDocs("");
&Docs3 = &CalcCostOut.GetDoc("Doc1.Doc2.Doc3");
&ret = &Docs3.GetValue("output_member3", &VALUE);
Calling GetDoc without the nesting feature:
Local Interlink &QE_4GETDOC;
Local BIDocs &CalcCostOut, &Docs1, &Docs2, &Docs3;
Local number &ret;
&QE_4GETDOC = GetInterlink(Interlink.QE_4GETDOC_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_4GETDOC.GetOutputDocs("");
&Docs1 = &CalcCostOut.GetDoc("Doc1");
&Docs2 = &Docs1.GetDoc("Doc2");
&Docs3 = &Docs2.GetDoc("Doc3");
&ret = &Docs3.GetValue("output_member3", &VALUE);
Image: Example output structure with nested documents
The following image is an example of output structure with nested documents.
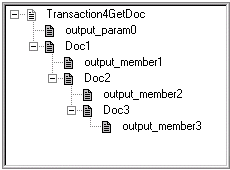
Syntax
GetFieldCount()
Description
Use the GetFieldCount method to support dynamic output. It returns the number of columns in the output buffer, which is the same as the number of outputs in each row of the output buffer.
Note: This method can also be used with hierarchical doc data.
Parameters
None.
Returns
The number of columns in the output buffer, which is the same as the number of outputs in each row of the output buffer.
Example
The following example moves to the first column, first field of the output buffer. The Repeat loop goes through every row in the output buffer. The For loop processes every field in every row.
If (&MYBI.MoveFirst()) Then
Repeat
For &I = 1 to &MYBI.GetFieldCount
&TYPE = &MYBI.GetFieldType(&I);
Evaluate &TYPE
Where = 1
/* do processing */
. . .
End-Evaluate;
End-For;
Until Not (&MYBI.MoveNext());
Else
/* do error processing - output buffer not returned */
End-If;
Syntax
GetFieldType(index)
Description
Use the GetFieldType method to support dynamic output. It returns the type of field specified by index. You can use this method only after you have used the MoveFirst method: otherwise the system doesn’t know where to start.
Note: This method can also be used with hierarchical doc data.
Parameters
Field or Control |
Definition |
---|---|
index |
Specify the number of the field you want to find the type of. |
Returns
A number indicating the type of the field. The values are:
Value |
Description |
---|---|
1 |
String |
2 |
Integer |
3 |
Float |
4 |
Boolean |
5 |
Date |
6 |
Time |
7 |
DateTime |
8 |
Binary |
9 |
Object |
Example
In the following example, the Business Interlink object name is &MYBI. The example uses MoveFirst to move to the first row of the output buffer, and to the first column, or first field, in that row. The Repeat loop uses MoveNext to go through every row in the output buffer. The For loop processes every field in every row, using the GetFieldCount method to get the number of fields, or outputs, in the row. Within the For loop, GetFieldType gets the type of the field data (string, integer, and so on.)
/* Add inputs to the Business Interlink object, then call Execute
to execute the Business Interlink object.
You are then ready to get the outputs using the following code. */
If (&MYBI.MoveFirst()) Then
Repeat
For &I = 1 to &MYBI.GetFieldCount
&TYPE = &MYBI.GetFieldType(&I);
Evaluate &TYPE
Where = 1
&STRING_VARIABLE = &MYBI.GetFieldValue(&I);
/* test for and process other field types */
End-Evaluate;
End-For;
Until Not(&MYBI.MoveNext());
Else
/* Process error - no output buffer */
End-If;
Syntax
GetFieldValue(index)
Description
Use the GetFieldValue method to support dynamic output. It returns the value of the field specified by index. You can use this method only after you have used the MoveFirst method: otherwise the system doesn’t know where to start.
Note: This method can also be used with hierarchical doc data.
Parameters
Field or Control |
Definition |
---|---|
index |
Specify the number of the field you want to find the value of. |
Returns
A string containing the value of the field.
Example
In the following example, the Business Interlink object name is &MYBI. The example uses MoveFirst to move to the first row of the output buffer, and to the first column, or first field, in that row. The Repeat loop uses MoveNext to go through every row in the output buffer. The For loop processes every field in every row, using the GetFieldCount method to get the number of fields, or outputs, in the row. Within the For loop, GetFieldValue gets the value of the field data.
/* Add inputs to the Business Interlink object, then call Execute to execute
the Business Interlink object. You are then ready to get the
outputs using the following code. */
If (&MYBI.MoveFirst()) Then
Repeat
For &I = 1 to &MYBI.GetFieldCount
&TYPE = &MYBI.GetFieldType(&I);
Evaluate &TYPE
Where = 1
&STRING_VARIABLE = &MYBI.GetFieldValue(&I);
/* test for and process other field types */
End-Evaluate;
End-For;
Until Not(&MYBI.MoveNext());
Else
/* Process error - no output buffer */
End-If;
Syntax
GetInputDocs("")
Description
The GetInputDocs method gets the top input document at the root level for a Business Interlink object. This is a hierarchical structure that contains the values for the inputs for this Business Interlink object. The methods that you use to put the input values into this document are the hierarchical data methods.
Parameters
A null string.
Returns
An input document. This is the document at the top of the root level of the input document for a Business Interlink object.
Example
Local Interlink &QE_COST;
Local BIDocs &CalcCostOut, &CalcCostIn;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
&CalcCostIn = &QE_COST.GetInputDocs("");
/* You can now insert the input values and execute the BI object */
Syntax
GetNextDoc()
Description
The GetNextDoc method gets a document from one of the following levels:
The root level of the Output structure for a Business Interlink object. This level was accessed with the GetOutputDocs method.
When a document within the Output structure is a list, GetNextDoc gets another document from the list. If you use GetNextDoc on a document that is not a list, GetNextDoc fails and returns an error.
Parameters
None.
Returns
The following are the valid returns:
Number |
Enum Value |
Description |
---|---|---|
0 |
eNoError |
The method succeeded. |
1 |
eNO_DOCUMENT |
The document referenced by this method does not exist. |
2 |
eDOCLIST_OUT_RANGE |
You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
eDOCUMENT_UNINITIALIZED |
Internal error |
4 |
eNOT_DOCUMENTTYPE |
You tried to perform an operation upon a parameter that is not a document type. |
5 |
eNOT_LISTTYPE |
You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
7 |
eNOT_BASICTYPE |
You tried to perform a GetValue or AddValue upon a parameter that is not of basic type: Integer, Float, String, Time, Date, DateTime. |
8 |
eNO_DATA |
You tried to retrieve data from a document that contained no data. |
Example
In this example, the Output structure for Calculate Cost, or the root level document, is accessed with the GetOutputDocs method. The GetNextDoc method gets another Output structure, assuming that there is more than one of them. The Calculate Cost output parameter output_param2_List is a document, so the GetDoc method gets it to the Output structure. output_param2_List is also a document list, so GetNextDoc method gets the next output_param2_List document.
Local Interlink &QE_COST;
Local number &count1, &count2;
Local BIDocs &CalcCostOut;
Local BIDocs &OutlistDoc;
Local number &ret1, &ret2;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
&ret1 = 0;
While (&ret1)
&ret1 = &CalcCostOut.GetValue("output_param1",&VALUE);
&ServiceRateDoc = &CalcCostOut.GetDoc("Service_Rate");
&ret1 = &ServiceRateDoc.GetValue("Service_Type", &SERVICE_TYPE);
&ret1 = &ServiceRateDoc.GetValue("Rate", &RATE);
/* Process output values */
&OutlistDoc = &CalcCostOut.GetDoc("output_param2_List");
&count2 = &CalcCostOut.GetCount("output_param2_List");
While (&I < &count2)
&ret2 = &OutlistDoc.GetValue("output_member1", &VALUE1);
&ret2 = &OutlistDoc.GetValue("output_member2", &VALUE2);
If &ret2 = 0 Then
/* Process output values */
&I = &I + 1;
&ret2 = &OutlistDoc.GetNextDoc();
End-If; &ret1 = &CalcCostOut.GetNextDoc();
End-While;
Image: Example output structure
The following shows the Output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List.
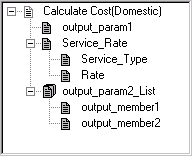
Syntax
GetOutputDocs("")
Description
The GetOutputDocs method gets the top output document at the root level for a Business Interlink object. This is a hierarchical structure that contains the values for the outputs for this Business Interlink object. The methods that you use to get output values from this document are the hierarchical data methods.
Parameters
A null string.
Returns
An output document. This is the document at the top of the root level of the output document for a Business Interlink object.
Example
Local Interlink &QE_COST;
Local BIDocs &CalcCostIn, &CalcCostOut;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
&CalcCostOut = &QE_COST.GetOutputDocs("");
/* You can now execute the Business Interlink object and get the output values. */
Syntax
GetPreviousDoc()
Description
The GetPreviousDoc method gets the previous document from either the root level of the Output structure for a Business Interlink object, or from a document within the Output structure. When these documents are in a list, GetPreviousDoc gets the previous document in the list. You must get the document before you can get its values with GetValue.
Parameters
None.
Returns
The following are the possible returns:
Number |
Enum Value |
Description |
---|---|---|
0 |
eNoError |
The method succeeded |
1 |
eNO_DOCUMENT |
The document referenced by this method does not exist. |
2 |
eDOCLIST_OUT_RANGE |
You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
eDOCUMENT_UNINITIALIZED |
Internal error |
4 |
eNOT_DOCUMENTTYPE |
You tried to perform an operation upon a parameter that is not a document type. |
5 |
eNOT_LISTTYPE |
You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
7 |
eNOT_BASICTYPE |
You tried to perform a GetValue or AddValue upon a parameter that is not of basic type: Integer, Float, String, Time, Date, DateTime. |
8 |
eNO_DATA |
You tried to retrieve data from a document that contained no data. |
Example
In this example, the Output structure for Calculate Cost, or the root level document, is accessed with the GetOutputDocs method. It contains one output parameter, output_param2_List, which is also a list. The GetCount and MoveToDoc methods point to the last output_param2_List document in the list. The GetPreviousDoc method is used in a loop to cycle through the output_param2_List list, starting with the last and ending with the first in the list, and get each output_param2_List document and its values.
Local Interlink &QE_COST;
Local number &count;
Local BIDocs &CalcCostOut;
Local BIDocs &OutlistDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
/* Call GetValue for output_param1, call GetDoc, GetValue for Service_Rate (code not shown) */
&OutlistDoc = &CalcCostOut.GetDoc("output_param2_List");
&count = &CalcCostOut.GetCount("output_param2_List");
&ret = &OutlistDoc.MoveToDoc(&count-1);
&I = &count;
While (&I > 0)
&ret = &OutlistDoc.GetValue("output_member1", &VALUE1);
&ret = &OutlistDoc.GetValue("output_member2", &VALUE2);
If &ret = 0 Then
/* Process output values */
&I = &I - 1;
&retoutput = &OutlistDoc.GetPreviousDoc("");
End-If;
End-While;
Image: Example output structure
The following shows the Output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List.
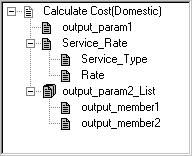
Syntax
GetStatus()
Description
The GetStatus method tests the BIDocs document. To find the status for any BIDocs method that does not return a status value, call GetStatus just after you call that BIDocs method.
Parameters
None.
Returns
The following are the possible returns:
Number |
Enum Value |
Description |
---|---|---|
0 |
NoError |
The method succeeded. |
1 |
NO_DOCUMENT |
The document referenced by this method does not exist. |
2 |
LIST_OUT_RANGE |
You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
DOCUMENT_UNINITIALIZED |
Internal error. |
4 |
NOT_DOCUMENTTYPE |
You tried to perform an operation upon a parameter that is not a document type. |
5 |
NOT_DOCUMENTLISTTYPE |
You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
6 |
NOT_LISTTYPE |
You tried to perform a list operation using GetValue, AddValue, on a non-list. |
7 |
NOT_SINGLEBASICTYPE |
You tried to perform a GetValue or AddValue upon a list that does not use a single basic type: Integer, Float, String, Time, Date, DateTime. |
9 |
NO_DATA |
You tried to retrieve data from a document that contained no data. |
10 |
GENERIC_ERROR |
There was an error with the transaction. |
Syntax
GetValue(paramname, value)
Description
The GetValue method gets a value from an output parameter within a document of the Output structure for a Business Interlink object.
Parameters
Field or Control |
Definition |
---|---|
Paramname |
The name of the member of the document that is having a value retrieved from it. |
Value |
The value that is retrieved. This can be a variable or a record field. The data type can be String, Integer, Double, Float, Time, Date, or DateTime. |
Returns
The following are the possible return values:
Return Status for Integer
Number |
Enum value and Meaning |
---|---|
0 |
NoError. The method succeeded. |
1 |
NO_DOCUMENT. The document referenced by this method does not exist. |
9 |
NO_DATA. You tried to retrieve data from a document that contained no data. |
10 |
GENERIC_ERROR. There was an error with the transaction. |
Example
In the following example, the Business Interlink object name is QE_COST.
In the following example, the Output structure for Calculate Cost, or the root level document, is created with the GetOutputDocs method. (If you wanted to create, or get, more Output structures, you would call GetNextDoc.) The Calculate Cost output parameter Service_Rate is a document, so the GetDoc method gets it from the Output structure. Then the GetValue method gets values from each of the Service_Rate document members.
Local Interlink &QE_COST;
Local BIDocs &CalcCostOut;
Local BIDocs &ServiceRateDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
&ret = &CalcCostOut.GetValue("output_param1",&PARAM1);
&ServiceRateDoc = &CalcCostOut.GetDoc("Service_Rate");
&ret = &ServiceRateDoc.GetValue("Service_Type", &SERVICE_TYPE);
&ret = &ServiceRateDoc.GetValue("Rate", &RATE);
/* Call GetDoc, GetValue, GetNextDoc for output_param2_List (code not shown) */
Image: Example output structure
The following illustration shows the Output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List. This is a version of the Federal Express plug-in that was modified for this example (output_param1 and output_param2_List were added).
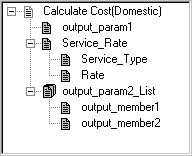
Syntax
InputRowset(&Rowset)
Description
The InputRowset method uses the data in the specified rowset to populate the input buffer, copying like-named fields in the appropriate structure. This method assumes that the names of the fields in the rowset match the names of the inputs defined in the Business Interlink definition, and that the structure of both rowsets are the same.
Use this method only if you have a hierarchical data structure and you want to preserve the hierarchy. Otherwise, use BulkExecute or AddInputRow.
Parameters
Field or Control |
Definition |
---|---|
&Rowset |
Specify an existing, instantiated rowset object. |
Returns
An optional value: the number of rows inserted into the output buffer.
Example
Image: EMPLOYEE_CHECKLIST Page structure
The example uses the rowset on level one from the EMPLOYEE_CHECKLIST page. A PeopleCode program running in a field on level zero in that panel can access the child rowset (level one), shown below from Scroll Bar 1 to Scroll Bar 2.
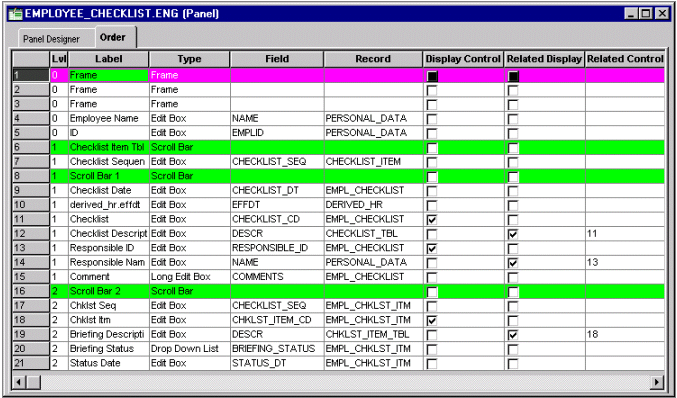
EMPL_CHECKLIST is the primary database record for the level one scrollbar on the EMPLOYEE_CHECKLIST page. The following PeopleCode access the level one rowset using EMPL_CHECKLIST. The Business Interlink object name is QE_BI_EMPL_CHECKLIST. This Business Interlink object uses the level one rowset as its input and its output.
The InputRowset method uses this rowset as input for QE_BI_EMPL_CHECKLIST. Then a blank duplicate of the rowset is created with CreateRowset, and then the output of QE_BI_EMPL_CHECKLIST is fetched into the blank rowset with FetchIntoRowset.
&MYROWSET = GetRowset(SCROLL.EMPL_CHECKLIST);
&ROWCOUNT = &QE_BI_EMPL_CHECKLIST.InputRowset(&MYROWSET);
&RSLT = &QE_BI_EMPL_CHECKLIST.Execute();
/* do some error processing */
&WorkRowset = CreateRowset(&MYROWSET);
&ROWCOUNT = &QE_BI_EMPL_CHECKLIST.FetchIntoRowset(&WorkRowset);
If &ROWCOUNT = 0 Then
/* do some error processing */
Else
/* Process the rowset from QE_BI_EMPL_CHECKLIST. Check it for errors. */
For &I = 1 to &WorkRowset.RowCount
For &K = 1 to &WorkRowset(&I).RecordCount
&REC = &WorkRowset(&I).GetRecord(&K);
&REC.ExecuteEdits();
For &M = 1 to &REC.FieldCount
If &REC.GetField(&M).EditError Then
/* there are errors */
/* do other processing */
End-If;
End-For;
End-For;
End-for;
End-if;
Syntax
MoveFirst()
Description
Use the MoveFirst method to support dynamic output. This method moves your cursor to the first column, first row of the output buffer. You must use this method before you try to access any data.
Parameters
None.
Returns
Boolean: True if successfully positioned cursor at the first column, first row of the output buffer, False otherwise.
Example
In the following example, the Business Interlink object name is &MYBI. The example uses MoveFirst to move to the first row of the output buffer, and to the first column, or first field, in that row. The Repeat loop uses MoveNext to go through every row in the output buffer. The For loop processes every field in every row, using the GetFieldCount method to get the number of fields, or outputs, in the row.
/* Add inputs to the Business Interlink object, then call Execute to execute the Business Interlink object. You are then ready to get the outputs using the following code. */
If (&MYBI.MoveFirst()) Then
Repeat
For &I = 1 to &MYBI.GetFieldCount
&TYPE = &MYBI.GetFieldType(&I);
Evaluate &TYPE
Where = 1
&STRING_VARIABLE = &MYBI.GetFieldValue(&I);
/* test for and process other field types */
End-Evaluate;
End-For;
Until Not(&MYBI.MoveNext());
Else
/* Process error - no output buffer */
End-If;
Syntax
MoveNext()
Description
Use the MoveNext method to support dynamic output. This method moves your cursor to the first column of the next row of the output buffer. You can use this method only after you have used the MoveFirst method: otherwise, the system doesn’t know where to start.
Parameters
None.
Returns
Boolean: True if successfully positioned cursor at the next row of the output buffer, False otherwise.
Example
In the following example, the Business Interlink object name is &MYBI. The example uses MoveFirst to move to the first row of the output buffer, and to the first column, or first field, in that row. The Repeat loop uses MoveNext to go through every row in the output buffer. The For loop processes every field in every row, using the GetFieldCount method to get the number of fields, or outputs, in the row.
/* Add inputs to the Business Interlink object, then call Execute to execute the Business Interlink object. You are then ready to get the outputs using the following code. */
If (&MYBI.MoveFirst()) Then
Repeat
For &I = 1 to &MYBI.GetFieldCount
&TYPE = &MYBI.GetFieldType(&I);
Evaluate &TYPE
Where = 1
&STRING_VARIABLE = &MYBI.GetFieldValue(&I);
/* test for and process other field types */
End-Evaluate;
End-For;
Until Not(&MYBI.MoveNext());
Else
/* Process error - no output buffer */
End-If;
Syntax
MoveToDoc(list_number)
Description
Within a list of documents in the Output structure, the MoveToDoc method moves to the documents given by the parameter list_number. After using MoveToDoc, the GetValue method gets the values of the document that is in the list_number+1 location in the list.
Parameters
Field or Control |
Definition |
---|---|
list_number |
The number indicating the document that MoveToDoc moves to. After using MoveToDoc, the GetValue method gets the values of the document that is in the list_number+1 location in the list. For example, if list_number is zero, then MoveToDoc moves to the first document in the list. |
Returns
This method returns an integer. The values are:
Value |
Description |
---|---|
0 |
eNoError. The method succeeded. |
1 |
eNO_DOCUMENT. The document referenced by this method does not exist. |
2 |
eDOCLIST_OUT_RANGE. You tried to access a document in a list, but the document list is out of range. For example, if a document list contains five documents, and then you call GetDoc/GetOutputDocs once, you can call GetNextDoc four times; the fifth time results in this error. |
3 |
eDOCUMENT_UNINITIALIZED. Internal error. |
4 |
eNOT_DOCUMENTTYPE. You tried to perform an operation upon a parameter that is not a document type. |
5 |
eNOT_LISTTYPE. You tried to perform a GetNextDoc or AddNextDoc upon a document that is not a list. |
7 |
eNOT_BASICTYPE. You tried to perform a GetValue or AddValue upon a parameter that is not of basic type: Integer, Float, String, Time, Date, DateTime. |
8 |
eNO_DATA. You tried to retrieve data from a document that contained no data. |
Example
The following example gets the values of the last document in the output_param2_List list. It uses GetCount to get the number of documents in the list, and then uses MoveToDoc to move to the last document in the list.
Local Interlink &QE_COST;
Local number &count;
Local BIDocs &CalcCostOut;
Local BIDocs &OutlistDoc;
Local number &ret;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
&OutlistDoc = &CalcCostOut.GetDoc("output_param2_List");
&count = &CalcCostOut.GetCount("output_param2_List");
&ret = &OutlistDoc.MoveToDoc(&count-1);
&ret = &OutlistDoc.GetValue("output_member1", &VALUE1);
&ret = &OutlistDoc.GetValue("output_member2", &VALUE2);
Image: Example output structure
The following illustration shows the Output structure for this example. It contains three output parameters: output_param1, Service_Rate, and output_param2_List. This is a version of the Federal Express plug-in that was modified for this example (output_param1 and output_param2_List were added).
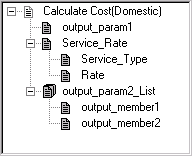
Syntax
ResetCursor()
Description
The ResetCursor method resets the cursor in the Output structure for a Business Interlink object to the top. After you call this method, the next time you call GetValue, you get an output value from the first document of the Output structures for a Business Interlink object.
Parameters
None.
Returns
None.
Example
The following code uses ResetCursor to reset the cursor in the Output structure to the top.
Local Interlink &QE_COST;
Local BIDocs &CalcCostOut;
&QE_COST = GetInterlink(Interlink.QE_COST_EX);
// Get inputs, execute. (code not shown)
&CalcCostOut = &QE_COST.GetOutputDocs("");
// Perform actions on the output documents (code not shown)
&CalcCostOut.ResetCursor();