Charting Examples
This section includes example of charts, with the PeopleCode used to create them.
This section demonstrates how to create a simple chart based on the Chart class. This chart is meant to be used to demonstrate basic principles of creating charts and give an opportunity to experiment with different chart types. It is not typical of how a chart is used in PeopleSoft applications.
The first six steps also apply to creating charts using the Gantt class, the OrgChart class, and the RatingBoxChart class.
The steps to create a chart are:
Place a chart control on a page.
Associate the chart control with a record field.
Create the chart data record.
Add PeopleCode to instantiate a chart object.
Set the data source.
View the page in the browser.
Add data for the chart.
Specify the series data.
Specify chart colors.
Add text elements.
Review the complete program.
Placing a Chart Control on a Page
In Application Designer, create a new page.
Select Insert, Chart and draw a rectangle to represent the area the chart will occupy. The chart can be any size, and you can have more than one chart on a page.
Place the page on a component and register the component.
Associating the Chart Control with a Record Field
Image: Chart Properties dialog box
Each chart on a component must be associated with a unique field. Double-click the chart control to access the Chart Properties dialog box and enter a record name and field name.
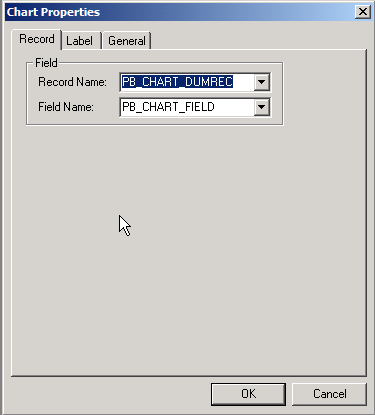
The chart field has no special requirements. Its only purpose is to provide a reference to the field in PeopleCode.
This sample chart uses a derived/work record named DOC_CHRT_WRK that is dedicated to this purpose.
Creating the Chart Data Record
The chart needs either a record or a rowset to serve as the data source. This sample chart uses a record. See the Chart class SetData method for details about using a rowset.
See SetData.
Image: Example of a chart data record
At a minimum, the record must have fields for the x-axis data and the y-axis data. Optionally, the record can have fields for series data, chart color, and glyph size.
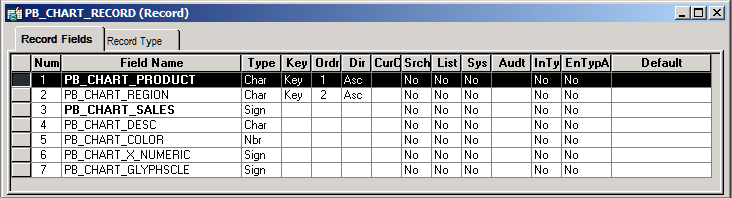
The record must be available in the component buffer at runtime. You can use a database record that is already part of your application or you can use a derived/work record or a component rowset that you populate at runtime. For greatest control, you will normally populate your record or rowset at runtime.
For the sample chart, place the record on a grid on a second page on the sample component. This placement gives you the opportunity to experiment with the data.
Image: Example of a page definition with chart data grid
Your chart data record must be placed at level 1 on the same component as the chart so that the data is available in the component buffer at runtime. In your application, you can hide the grids or scroll areas that hold the records. For the sample chart, you will keep the grid visible so you can enter sample data.
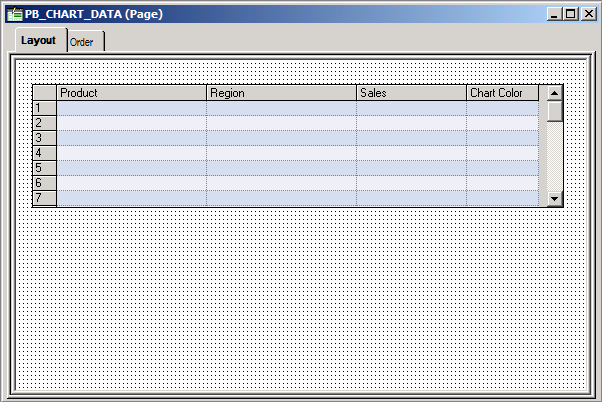
Adding PeopleCode to Instantiate a Chart Object
This example instantiates a chart object using the Chart class, so it can be used to create bar charts, line charts, pie charts, and so on. Other examples in this section demonstrate how to create charts based on the Gantt class, OrgChart class, and RatingBoxChart class.
See Creating a Gantt ChartCreating a Gantt Chart, Creating a Rating Box Chart.
Add this PeopleCode to the Activate event on the page:
/* Declare and instantiate a chart object */
Component Chart &cChart;
&cChart = GetChart(DOC_CHRT_WRK.DOC_CHRT_FLD);
Chart PeopleCode is commonly placed on the Activate event, but it can be placed on any appropriate event. You could, for instance, execute the chart PeopleCode from a FieldChange event associated with a push button.
Setting the Data Source
The SetData method takes either a record or a rowset as an argument. In general, specify a record or component rowset when building a chart from page data and a standalone rowset when building a chart using an iScript.
In this example, the PeopleCode sets Product as the series data and sets Region to the x-axis, so that in a bar chart products will be grouped along the x-axis by region. In a line chart, each series is represented by a line. Sales figures are charted on the y-axis.
Note: SetData, SetDataXAxis, and SetDataYAxis are Chart class methods. The other charting classes provide equivalent methods to specify data sources.
/* Set the chart data record and specify x-axis, y-axis, and series data */
&cChart.SetData(Record.DOC_CHRT_SLSREC);
&cChart.SetDataXAxis(DOC_CHRT_SLSREC.DOC_CHRT_RGN);
&cChart.SetDataYAxis(DOC_CHRT_SLSREC.DOC_CHRT_SALES);
See SetData, SetDataSeries, SetDataXAxis, SetDataYAxis, Creating a Chart Using an iScript.
Viewing the Page in the Browser
Image: Chart class chart with no data
This is the most basic Chart class chart. It has no data and only the default y-axis.
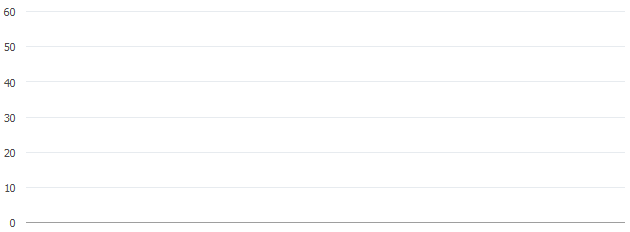
Adding Data for the Chart
Add the following data to the chart:
Region |
Sales |
---|---|
Arizona |
60 |
California |
55 |
Oregon |
50 |
Washington |
45 |
Image: A simple bar chart
This bar chart is generated by the example code and data:
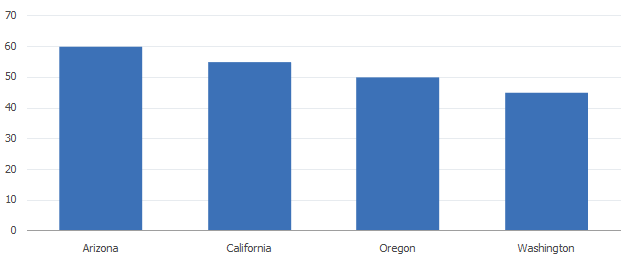
This chart graphs sales on the y-axis (the vertical axis) and regions on the x-axis (the horizontal axis).
When the data for the chart is grouped according to values, a set of data representing a single value is called a series. For instance, if a chart shows sales for different products in each region, then the set of data for each product is a series.
At this point, the chart has only one series, so you do not need to set the series record field.
Specify the Series Data
If you want to graph more than one product on your chart, you need to specify the source of the series data.
For example, add the products shown in this table to your data:
Product |
Region |
Sales |
---|---|---|
Footballs |
Arizona |
60 |
Rackets |
Arizona |
50 |
Shoes |
Arizona |
42 |
Tents |
Arizona |
35 |
Footballs |
California |
55 |
Rackets |
California |
46 |
Shoes |
California |
30 |
Tents |
California |
35 |
Footballs |
Oregon |
50 |
Rackets |
Oregon |
40 |
Shoes |
Oregon |
30 |
Tents |
Oregon |
25 |
Footballs |
Washington |
45 |
Rackets |
Washington |
30 |
Shoes |
Washington |
20 |
Tents |
Washington |
15 |
Add this PeopleCode to set the series record field:
&cChart.SetDataSeries(DOC_CHRT_SLSREC.DOC_CHRT_PRDCT);
Image: Default 2D bar chart with products as series
The resulting chart is a default 2D bar chart with bars for each region grouped by series:
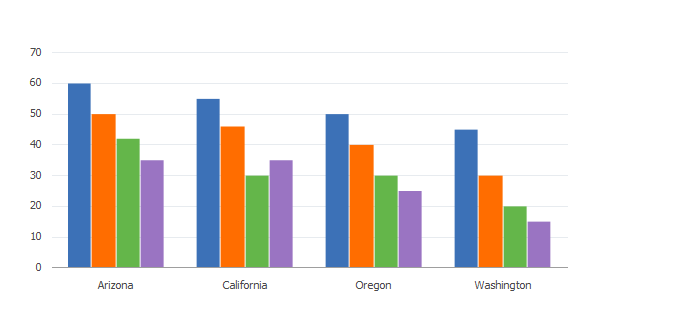
The chart has four series:
Footballs
Rackets
Shoes
Tents
Each series shows four regions along the x-axis:
Arizona
California
Oregon
Washington
Sales values appear against the y-axis as bar height.
The chart uses the default colors.
Specifying Chart Colors
If you do not want to use the default colors, you need a column containing the colors for each bar. Typically, the bars in a series are colored alike.
Product |
Region |
Sales |
Chart Color |
---|---|---|---|
Footballs |
Arizona |
60 |
1 |
Footballs |
California |
55 |
1 |
Footballs |
Oregon |
50 |
1 |
Footballs |
Washington |
45 |
1 |
Rackets |
Arizona |
50 |
13 |
Rackets |
Oregon |
46 |
13 |
Rackets |
Washington |
40 |
13 |
Rackets |
California |
30 |
13 |
Shoes |
Arizona |
42 |
5 |
Shoes |
California |
30 |
5 |
Shoes |
Oregon |
30 |
5 |
Shoes |
Washington |
20 |
5 |
Tents |
Arizona |
35 |
15 |
Tents |
California |
35 |
15 |
Tents |
Oregon |
25 |
15 |
Tents |
Washington |
15 |
15 |
Add the following PeopleCode to your program to set the colors for your chart:
/* Associate color field with chart */
&cChart.SetDataColor(DOC_CHRT_SLSREC.DOC_CHRT_COLOR);
Adding Text Elements
The following PeopleCode adds these elements to your chart:
Title
Legend
Y-axis title
X-axis title
/* Set title and legend properties */
&cChart.MainTitle = "Recreational Product Sales by Region";
&cChart.HasLegend = True;
&cChart.LegendPosition = %ChartLegend_Right;
/* Set axis text properties */
&cChart.XAxisTitle = "Region";
&cChart.YAxisTitle = "Sales";
Image: Sample bar chart with custom colors, a main title, axis titles, and a legend
This is the resulting chart:
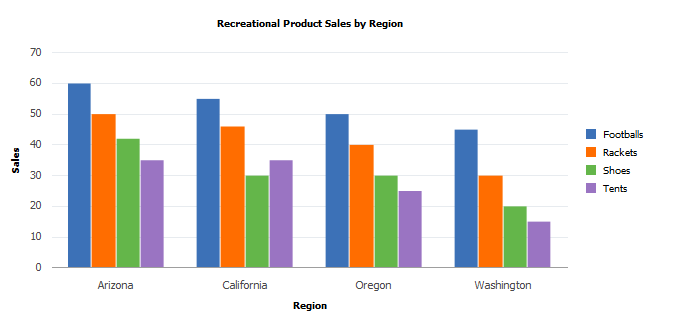
Reviewing the Complete Program
The PeopleCode for this bar chart has been presented in snippets. Here is the complete program that produced the chart:
/* Declare and instantiate a chart object */
Component Chart &cChart;
&cChart = GetChart(DOC_CHRT_WRK.DOC_CHRT_FLD);
/* Set the chart data record and specify x-axis, y-axis, and series
data */
&cChart.SetData(Record.DOC_CHRT_SLSREC);
&cChart.SetDataXAxis(DOC_CHRT_SLSREC.DOC_CHRT_RGN);
&cChart.SetDataYAxis(DOC_CHRT_SLSREC.DOC_CHRT_SALES);
&cChart.SetDataSeries(DOC_CHRT_SLSREC.DOC_CHRT_PRDCT);
/* Associate color field with chart */
&cChart.SetDataColor(DOC_CHRT_SLSREC.DOC_CHRT_COLOR);
/* Set title and legend properties */
&cChart.MainTitle = "Recreational Product Sales by Region";
&cChart.HasLegend = True;
&cChart.LegendPosition = %ChartLegend_Right;
/* Set axis text properties */
&cChart.XAxisTitle = "Region";
&cChart.YAxisTitle = "Sales";
Other Modifications
The remainder of this section shows the effects of modifying other aspects of your chart.
Changing X-Axis and Series Values
&cChart2.SetDataXAxis(DOC_CHRT_SLSREC.DOC_CHRT_PRDCT);
&cChart2.SetDataSeries(DOC_CHRT_SLSREC.DOC_CHRT_RGN);
&cChart2.MainTitle = "Recreational Product Sales by Product";
&cChart2.XAxisTitle = "Product";
Image: Bar chart with series set to region
To group sales by product instead of by region, switch the series and the x-axis. You will also need to reorder colors to correspond to regions instead of products:
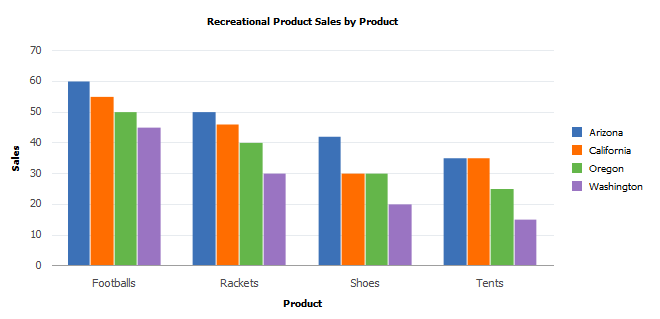
Axis Properties
Image: Bar chart with negative values
If your chart has negative values, the bars automatically extend below the x-axis:
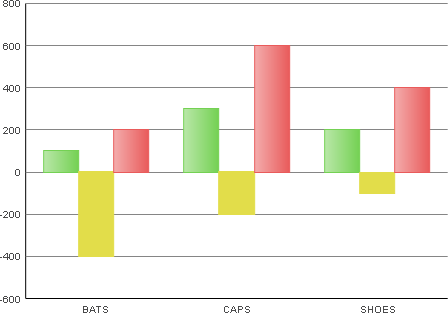
If you want to remove the tick marks from the x- or y-axis, you need to specify an empty array for the SetXAxisLabels or SetYAxisLabels methods.
The following example removes the tick marks from the y-axis:
Local array of string &Arr;
&Arr = CreateArray("");
&Chart.SetYAxisLabels(&Arr);
If you set the x-axis labels to an empty array to remove the ticks, you must be plotting a single series on the chart because labels are automatically generated for each series if you do not provide them.
See SetXAxisLabels, SetYAxisLabels.
You can also use the XAxisMin, XAxisMax, YAxisMin, YAxisMax, and other axis properties to control the appearance of the axes.
Chart Class Chart Types
Use the Type property to set the chart type.
2D Bar Chart
&cChart.Type=%ChartType_2DBar;
Image: 2D bar chart
The default Chart class chart type is a 2D bar chart:
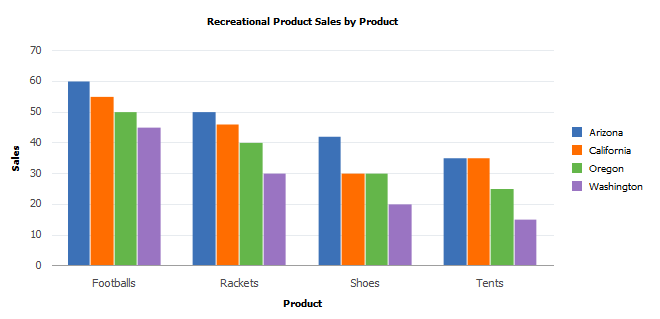
The following examples show the various chart types using the same data. Each example gives the PeopleCode snippet that creates that chart type.
For a complete list of all Chart class chart types, see Chart class Type property.
See Type.
The Charting Examples section provides other examples of PeopleCode for charts using Gantt class, OrgChart class, and RatingBoxChart class at the end of the chapter.
See Creating a Gantt ChartCreating a Gantt Chart, Creating a Rating Box Chart.
Horizontal Bar Chart
Use this line of code to specify a horizontal bar chart:
&cChart.Type = %ChartType_2DHorizBar;
Image: Horizontal bar chart
This example illustrates a horizontal bar chart:
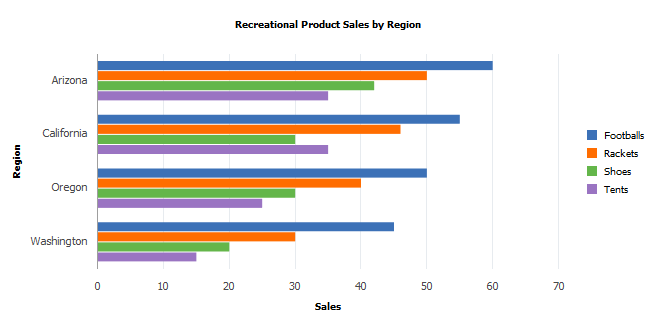
Stacked Bar Chart
With a the stacked bar chart, you can place a different emphasis on the same data. The stacked column shows total sales per region as well as the product segments that contribute to the total.
Use this line of code to specify a stacked bar chart:
&cChart.Type = %ChartType_2DStackedBar;
Image: Stacked bar chart
This example illustrates a stacked bar chart:
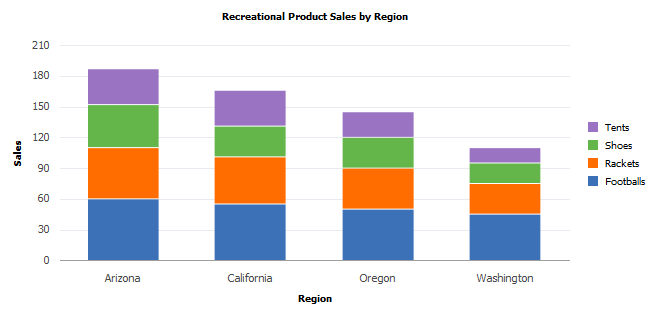
Horizontal Stacked Bar Chart
Use this line of code to specify a horizontal stacked bar chart:
&cChart.Type = %ChartType_2DHorizStackedBar;
Image: Horizontal stacked bar chart
This example illustrates a horizontal stacked bar chart:
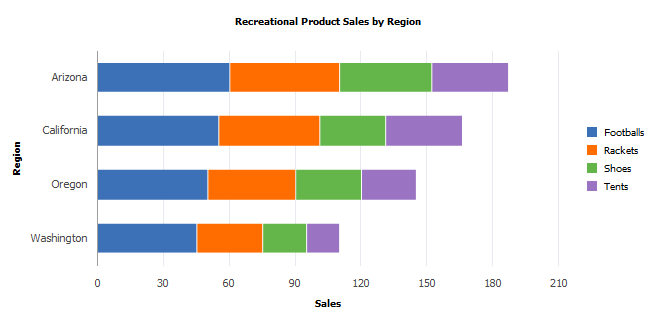
Percent Bar Chart
A percent bar is similar to a stacked bar, but the segments of each bar add up to 100. The segments show the proportional contribution of each product to total sales.
Note: Negative values cannot be displayed in any type of percent bar chart.
Use this line of code to specify a stacked percent bar chart:
&cChart.Type = %ChartType_2DPercentBar;
Image: Percent bar chart
This example illustrates a percent bar chart:
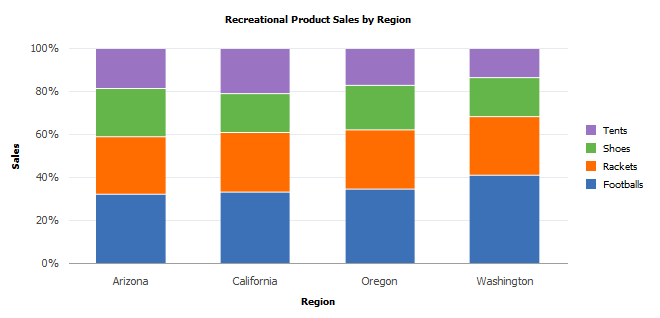
Horizontal Percent Bar Chart
Note: Negative values cannot be displayed in any type of percent bar chart.
Use this line of code to specify a horizontal percent bar chart:
&cChart.Type = %ChartType_2DHorizPercentBar;
Image: Horizontal percent bar chart
This example illustrates the fields and controls on a horizontal percent bar chart:
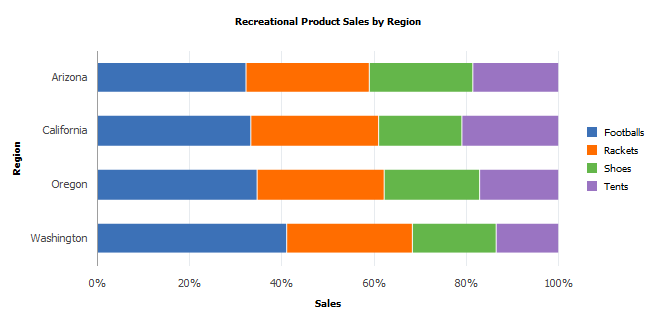
Line Chart
A line chart is useful for showing changes and trends over time or across categories.
Use this line of code to specify a line chart:
&cChart.Type = %ChartType_2DLine;
Image: Line chart
This example illustrates a line chart:
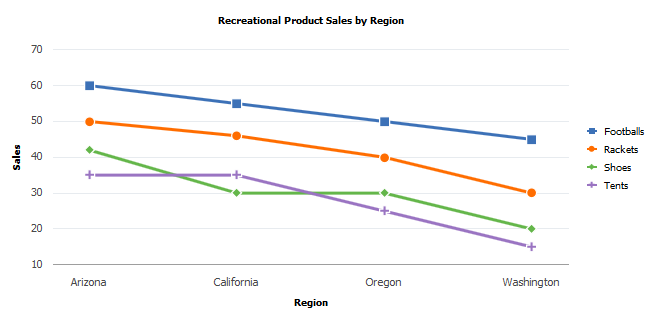
Overlay Chart
You can overlay one chart on top of another to compare two different but related sets of data. The following chart uses a second set of data, showing average temperature for each region.
Use the OLType property to specify whether the overlay is a line chart or a histogram.
Add this PeopleCode to the PeopleCode for your bar chart to create 2D line chart overlay:
/* Create Overlay */
&cChart3.SetOLData(Record.DOC_CHRT_OLREC);
rem &cChart.SetOLDataSeries(DOC_CHRT_OLREC.SC_CHART_SERIES);
&cChart3.SetOLDataXAxis(DOC_CHRT_OLREC.DOC_CHRT_RGN);
&cChart3.SetOLDataYAxis(DOC_CHRT_OLREC.DOC_CHRT_SALES);
&cChart3.OLType = %ChartType_2DLine;
Image: Overlay chart
This example illustrates a 2D line chart overlay on a vertical bar chart:
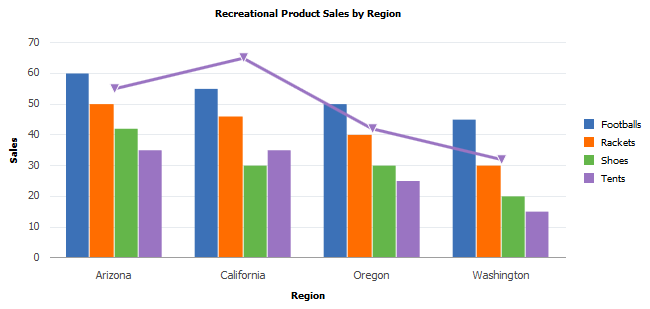
Scatter Chart
Use this line of code to specify a scatter chart:
&cChart.Type = %ChartType_2DScatter;
A scatter chart compares number pairs. Each pair is plotted against the horizontal x-axis and the vertical y-axis. The data values are scattered across the chart. XY scatter charts are good for showing comparisons of numbers, such as scientific or statistical data, where several measurements need to be plotted on a single chart.
A scatter chart is a true XY chart, meaning that the x-axis is numeric. The IsTrueXY property is automatically set to True.
For example, this sample data could be used to make an XY scatter chart:
Tenure |
Salary |
---|---|
11 |
88000 |
12.2 |
96200 |
4.6 |
101300 |
6.6 |
83000 |
14 |
111200 |
8.5 |
118000 |
You use this code snippet to make a scatter chart:
&cChart5.Type = %ChartType_2DScatter;
&cChart5.SetData(Record.DOC_CHRT_XYDATA);
&cChart5.SetDataXAxis(DOC_CHRT_XYDATA.DOC_CHRT_X);
&cChart5.SetDataYAxis(DOC_CHRT_XYDATA.DOC_CHRT_Y);
Image: Scatter chart
This example illustrates the scatter chart for the preceding data:
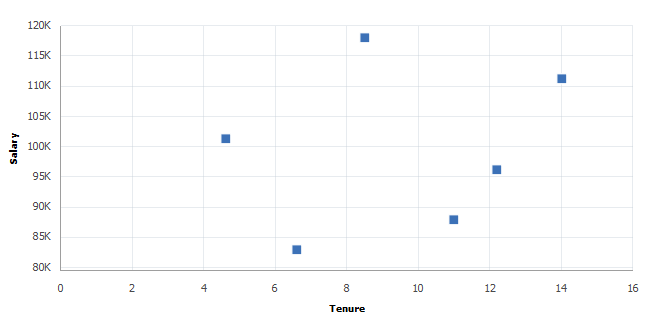
Note: If you have only one point of data, that is, only one x and y pair, use a scatter chart. You may have unexpected results if you use a line chart with only one point.
Bubble Chart
A bubble chart is a special type of scatter chart.
If you add a third data set, the z-axis, to a scatter chart, you can create a bubble chart, which uses the size of the markers to represent a third dimension of numeric data.
You can also set annotations for scatter charts and bubble charts.
This chart example uses Age to determine the size of the bubble markers on the chart and Name to set the annotations:
Tenure |
Salary |
Age |
Name |
---|---|---|---|
11 |
88000 |
39 |
Joyce |
12.2 |
96200 |
55 |
Tolstoy |
4.6 |
101300 |
32 |
Shelley |
6.6 |
83000 |
48 |
Tolkien |
14 |
111200 |
59 |
Dickens |
8.5 |
118000 |
49 |
Hemingway |
&cChart6.Type = %ChartType_2DBubble;
&cChart6.SetData(Record.DOC_CHRT_XYDATA);
&cChart6.SetDataXAxis(DOC_CHRT_XYDATA.DOC_CHRT_X);
&cChart6.SetDataYAxis(DOC_CHRT_XYDATA.DOC_CHRT_Y);
&cChart6.SetDataZAxis(DOC_CHRT_XYDATA.DOC_CHRT_AGE);
&cChart6.SetDataAnnotations(DOC_CHRT_XYDATA.DOC_CHRT_LASTNAME);
Image: Bubble chart
This example illustrates the bubble chart for the preceding data:
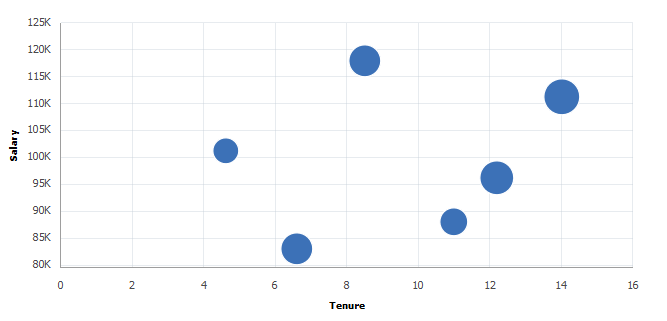
Pie Chart
A pie chart shows comparisons within a single set of values, and it shows how parts contribute to a whole. It is an ideal chart type to display the contribution of each region to an annual sales total.
A pie chart ignores the series and only plots x-axis data against y-axis values. Our original bar chart has four regions times four products, resulting in 16 XY pairs. A pie chart with all of those slices would be confusing and not helpful. Instead, suppose you want to show just the data for tents, which are in rows 13–16. You can use a combination of the DataWidth and DataStartRow properties to select which rows to display.
You can make a pie chart showing only rows 13–16 by adding this snippet of code:
&cChart.DataWidth = 4;
&cChart.DataStartRow = 13;
&cChart.Type = %ChartType_2DPie;
Image: 2D pie chart
This example illustrates a 2D pie chart generated from a portion of the rowset:
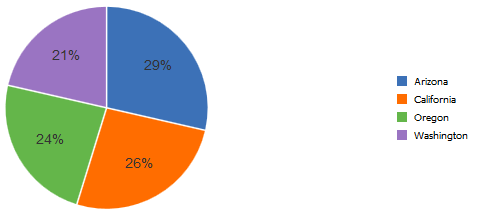
Exploded Pie Chart
Use the SetExplodedSectorsArray Chart class method to specify the sectors to explode. Sectors correspond to rows of data used by the pie chart. Sector 1 is the first row of data, or the row specified with the DataStartRow chart class property, if it is used, and so on.
The following snippet of code explodes sectors 1 and 3:
Local array of number &ExplodedArray;
&ExplodedArray = CreateArray(1,3);
&cChart.SetExplodedSectorsArray(&ExplodedArray);
Image: Exploded pie chart
This example illustrates an exploded pie chart:
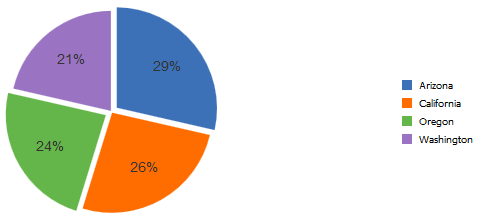
See the SetExplodedSectorsArray property.
3D Pie Chart
Use this line of code to specify a 3D pie chart:
&cChart.Type = %ChartType_3DPie;
Image: 3D Pie Chart
This example illustrates a 3D pie chart.
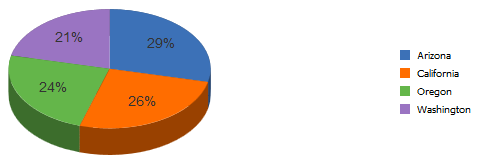
Adding Drilldown
If you set the property IsDrillable to True and add FieldChange PeopleCode to your chart, your user can get more information by clicking a chart data element—a bar, line segment, data point, or pie slice.
For a simple example, add this PeopleCode to the FieldChange event on the y-axis field:
MessageBox(0, "", 0, 0, "Sales for " | DOC_CHRT_SLSREC.DOC_CHRT_PRDCT | " in " | DOC_CHRT_SLSREC.DOC_CHRT_RGN | " = " | DOC_CHRT_SLSREC.DOC_CHRT_SALES | "");
And add this code to the page Activate event for your pie chart:
&cChart.IsDrillable = True;
Image: Example of drill-down triggering PeopleCode with MessageBox
When the user clicks the Arizona slice of the pie chart, this message appears:
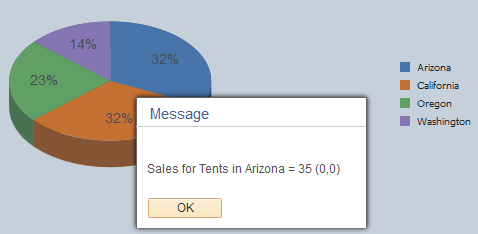
Current context provides the values for fields in the current row in the component buffer. You can use drilldown with your chart data fields along with any other data in the component buffer to accomplish anything FieldChange PeopleCode is capable of.
If the bars on a bar chart are too narrow, a user may not be able to click them. Sometimes, a few bars on a chart are affected, sometimes all of the bars cannot be clicked. The workaround is to put fewer bars on your chart or to make your chart bigger so that each bar is wider.
See IsDrillable.
Using the Other Charting Classes
In addition to the all the charts you can create with the Chart class, you can create specialized charts using the Gantt class, the OrgChart class, and the RatingBoxChart class.
You follow the same initial steps to create these specialized charts as you do for the Chart class chart types. The PeopleCode programs to set the data and properties are similar as well, but Gantt charts, organization charts, and rating box charts use data differently and interact with the user in different ways than do the Chart class charts.
See Creating a Gantt ChartCreating a Gantt Chart, Creating a Rating Box Chart.
Image: Gantt chart
The PeopleCode and data used in this example create the following Gantt chart:
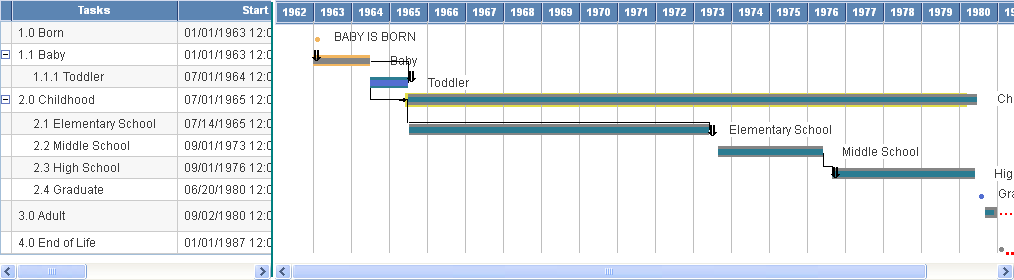
This example uses the following data in the record QE_GANTT_TASK:
Image: Example of Gantt task data
This example illustrates Gantt task data:
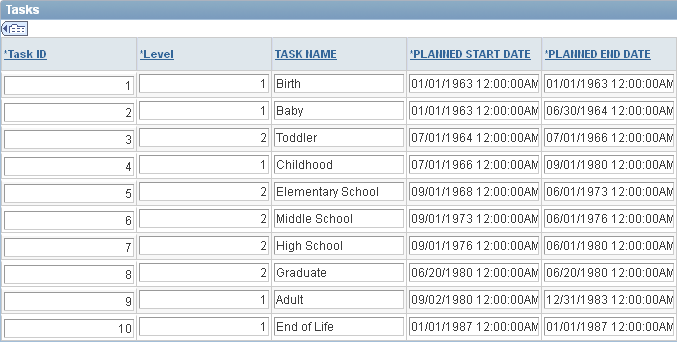
The chart also uses the following dependency data in the record QE_GANTT_TASKD:
Image: Example of Gantt dependency data
This example illustrates Gantt dependency data:
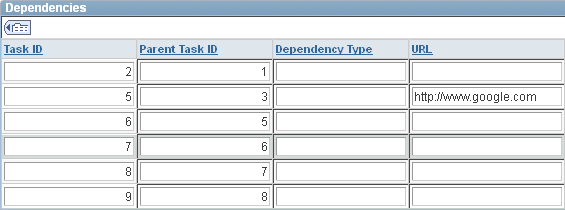
To create a Gantt chart:
In Application Designer, add a chart control to a page and associate the chart control with a record name and a field name.
Initialize a Gantt chart object.
Initialize the chart using the GetGanttChart function. The arguments of the function are the record and field name specified for the chart definition in Application Designer.
Local Gantt &gGantt; &gGantt = GetGanttChart(QE_CHART_DUMREC.QE_CHART_FIELD);
Specify values for the required methods.
At a minimum, specify the source of the task data and which fields contain task ID, start date, and end date.
&gGantt.SetTaskData(Record.QE_GANTT_TASK); &gGantt.SetTaskID(QE_GANTT_TASK.QE_TASK_ID); &gGantt.SetPlannedStartDate(QE_GANTT_TASK.QE_PLANNED_START); &gGantt.SetPlannedEndDate(QE_GANTT_TASK.QE_PLANNED_END);
Specify values for the additional methods controlling the appearance of the data.
After setting the required methods, you can set additional methods to control the appearance of the chart. The following code specifies the percentage of the entire chart that is taken up by the chart area, the width and height of the chart, if the user can click the chart area of the Gantt chart and either execute a function or a URL, and whether labels should appear with the tasks in the task section.
&gGantt.SetChartArea(50); &gGantt.Width = 700; &gGantt.Height = 380; &gGantt.IsDrillable = True; &gGantt.ShowTaskLabels = True;
Specify the appearance of grid lines.
Depending on the type of data that you are using, you can specify the type of grid lines that should appear in the chart section of the Gantt chart.
&gGantt.GridLineType = %ChartLine_Solid; &gGantt.GridLines = %ChartGrid_Vertical;
Specify the appearance of data.
The following methods control the appearance of the different parts of the task data, such as the label, which hints appear when you pass the mouse over the task data, and so on.
&gGantt.SetTaskName(QE_GANTT_TASK.QE_TASK_NAME); &gGantt.SetWBSNumbering(QE_GANTT_TASK.QE_HINTS); &gGantt.SetTaskLevel(QE_GANTT_TASK.QE_LEVEL); &gGantt.SetTaskLabel(QE_GANTT_TASK.QE_TASK_LABEL); &gGantt.SetTaskProgress(QE_GANTT_TASK.QE_TASK_PROGRESS); &gGantt.SetTaskBarURL(QE_GANTT_TASK.QE_URL); &gGantt.SetTaskMilestone(QE_GANTT_TASK.QE_TASK_MILESTONE); &gGantt.TaskMilestoneGlyph = QE_GANTT_PROJ.QE_MILESTONE_GLYPH; &gGantt.SetPlannedTaskBarColor(QE_GANTT_TASK.QE_PLANNED_COLOR); &gGantt.SetActualTaskBarColor(QE_GANTT_TASK.QE_ACTUAL_COLOR); &gGantt.SetTaskProgressBarColor(QE_GANTT_TASK.QE_PROGRESS_COLOR);
Specify the additional fields that will appear in the table section.
You can also specify application data that will appear with the task data.
&gGantt.SetTaskAppData(QE_GANTT_TASK.QE_PLANNED_START, QE_GANTT_TASK.QE_PLANNED_END, QE_GANTT_TASK.QE_TASK_PROGRESS, QE_GANTT_TASK.QE_TASK_NAME);
Specify titles for the task bars.
&gGantt.TaskTitle = "Tasks"; &appDataArray = CreateArray("Start", "End", "Progress", "Name"); &gGantt.SetTaskAppDataTitles(&appDataArray);
Specify the fields for task dependency data.
You do not need to specify dependency data. However, if you do, you must also specify the parent ID and the child ID (where the dependency data starts and ends). You can also specify the type of line used between dependencies and a URL that the browser will open to if the user clicks a dependency line.
&gGantt.SetTaskDepenencyData(Record.QE_GANTT_TASKD); &gGantt.SetTaskDependencyParentID(QE_GANTT_TASKD.QE_PARENT_TASK_ID); &gGantt.SetTaskDependencyChildID(QE_GANTT_TASKD.QE_TASK_ID); &gGantt.TaskDependencyLineType = %ChartLine_Solid; &gGantt.SetTaskDependencyURL(QE_GANTT_TASKD.URL);
Specify the actual dates for the project.
If you know the actual start and end dates, in addition to the planned date, you can add those to your chart.
&gGantt.SetActualStartDate(QE_GANTT_TASK.QE_ACTUAL_START); &gGantt.SetActualEndDate(QE_GANTT_TASK.QE_ACTUAL_END);
Specify the time line axis values.
You can specify values for where the time line axis starts and ends.
&gGantt.AxisStartDateTime = DateTimeValue("01/01/1963 8:00:00"); &gGantt.AxisEndDateTime = DateTimeValue("01/01/1987 8:00:00");
Specify the format of the time line axis values.
You can specify the format of the time line elements, such as the appearance of the days of the week, whether as a number or using the full name, and so on.
&gGantt.SetMonthFormat(%Chart_MonthFormat_FullName); &gGantt.SetDayFormat(%Chart_DayFormat_2Digit);
Image: Organization chart
The PeopleCode used in this example creates the following organization chart:
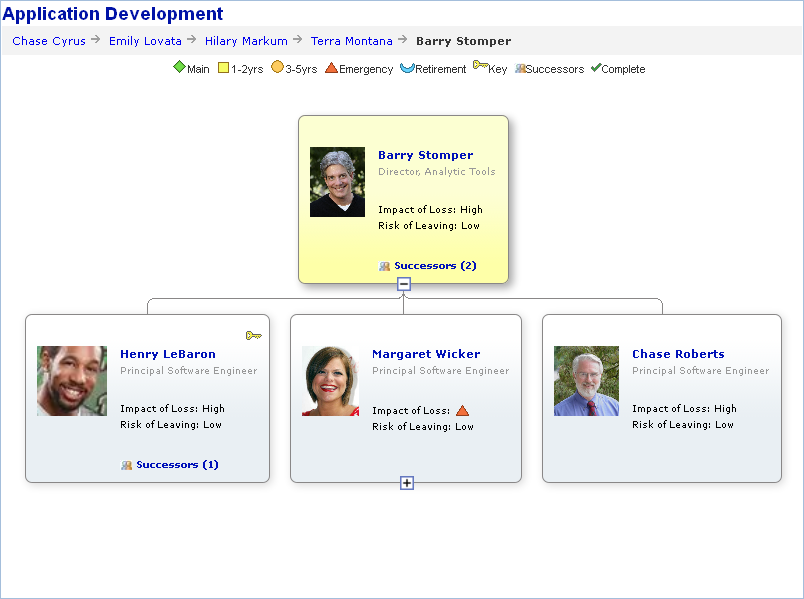
To create an organization chart:
Add a chart control to a page in Application Designer.
Associate the chart control with a record name and field name.
Add the organization node record and the pop-up node record to the component.
These records are derived/work records that contain clones of the subrecords PTORGNODE_SBR and PTPOPUPNODE_SBR. These records must be in the component buffer. You can add them to a hidden grid on the page, or you can place them on another page in the same component.
If the chart will have breadcrumbs, add the breadcrumb node record to the component.
This derived/work record contains a clone of the subrecord PTORGCRMB_SBR. The record must be in the component buffer.
Prepare the organization chart data.
In an event such as PreBuild, instantiate two rowset objects and populate them with the organization node data and the pop-up node data.
This example shows one possible way. How you do it depends on how your data is stored and how you intend to present it.
Component Rowset &rs, &rsP, &rsBC, &rsOrgNode, &rsOrgPopupND, &rsBreadCrumbs; Component OrgChart &ocOrgChart; Local Rowset &RSGridDR; Local Rowset &RSAGRID; /* The record QE_ORG_NODEDATA has the data for the org chart ** ** You will probably fill the rowset with some subset of the data** ** in the database. This example uses all the rows. */ &rs = CreateRowset(Record.QE_ORG_NODEDATA); &rs.Fill(); /* Get the grid on the page for the node data and initalize to nulls */ & rsOrgNode = GetLevel0()(1).GetRowset(Scroll.QE_ORG_NODE); & rsOrgNode.Flush(); /* Copy data from database table, QE_ORG_NODEDATA to the derived/work ** record QE_ORG_NODE_DR */ &rs.CopyTo(&rsOrgNode, Record.QE_ORG_NODEDATA, Record.QE_ORG_NODE_DR); /* The record QE_ORG_POPDATA has the data for the popup chart */ &rsP = CreateRowset(Record.QE_ORG_POPDATA); &rsP.Fill(); /* Get the grid on the page for the popup data and initalize to nulls */ & rsOrgPopupND = GetLevel0()(1).GetRowset(Scroll.QE_ORG_POPUP); & rsOrgPopupND.Flush(); /* Copy data from database table, QE_ORG_POPDATA to the derived/work ** record QE_ORG_POP_DR */ &rsP.CopyTo(&rsOrgPopupND, Record.QE_ORG_POPDATA, Record.QE_ORG_POP_DR); /* Fill in the breadcrumb information for this chart if the chart ** ** uses breadcrumbs. */ &rsBC = CreateRowset(Record.QE_ORG_CRMB); &rsBC.Fill(); /* Get the grid on the page for the pop data and initalize to nulls */ &rsBreadCrumbs = GetLevel0()(1).GetRowset(Scroll.QE_ORG_BCRMB); &rsBreadCrumbs.Flush(); /* Copy data from database table, QE_ORG_CRMB to the derived/work ** record QE_ORG_CRMB_DR */ &rsBC.CopyTo(&rsBreadCrumbs, Record.QE_ORG_CRMB, Record.QE_ORG_CRMB_DR);
Instantiate an OrgChart object.
In an event such as Activate, add PeopleCode to instantiate and define your organization chart.
Get the chart using the GetOrgChart function. The argument for this function is the record name and field name specified on the Records tab of the Chart Properties dialog box in Application Designer.
Component Rowset & rsOrgNode, & rsOrgPopupND, &rsBreadCrumbs; Component OrgChart &ocOrgChart; &ocOrgChart= GetOrgChart(QE_CHART_DUMREC.QE_CHART_FIELD);
Specify the organization node record and the pop-up node record.
These records contain the clones of the subrecords PTORGNODE_SBR and PTORGPOPUP_SBR, respectively.
&ocOrgChart.SetNodeRecord(Record.QE_ORG_NODE_DR); &ocOrgChart.SetPopUpNodeRecord(Record.QE_ORG_POP_DR);
Specify the node data and pop-up node data rowset objects.
/*&RSORGND and &RSORGPOPUPND are level 1 component rowsets that contain the ** organization node data and pop-up node data respectively.*/ &ocOrgChart.SetNodeData(&rsOrgNode); &ocOrgChart.SetPopUpNodeData(&RSORGPOPUPND);
(Optional) Specify values for the chart display properties.
&ocOrgChart.MainTitle = "Application Development"; &ocOrgChart.MainTitleStyle = "PT_ORGCHART_TITLE"; &ocOrgChart.Direction = 2; &ocOrgChart.Height = 380; &ocOrgChart.Width = 700; &ocOrgChart.VerticalSpace = 15; &ocOrgChart.ImageLocation = 1; &ocOrgChart.Style= "PTORGCHART"; &ocOrgChart.HasLegend = True; &ocOrgChart.NodeMaxDisplayDescLength =30; &ocOrgChart.CollapsedImage ="PT_COLLAPSED_NODE"; &ocOrgChart.ExpandedImage ="PT_EXPANDED_NODE"; &Expanded_Msg=MsgGetText(110, 100); &Collapsed_Msg=MsgGetText(110, 101); &ocOrgChart.Expanded_Msg =&Expanded_Msg &ocOrgChart.Collapsed_Msg =&Collapsed_Msg
Specify values for the legend. This is required only if the chart has a legend.
&ocOrgChart.LegendStyle = PT_ORGCHART_LEGEND; &LegendArray = CreateArray("Main", "1-2yrs", "3-5yrs", "Emergency", "Retirement", "Key", "Successors"); &LegendImgArray = CreateArray("QE_NOWORGCHART", "QE_12ORGCHART", "QE_34ORGCHART", "QE_EMRORGCHART", "QE_RETIRORG", "QE_KPERSON", "QE_SUCCSORGCHART"); &ocOrgChart.SetLegend(&LegendArray); &ocOrgChart.SetLegendImg(&LegendImgArray); &ocOrgChart.LegendPosition = %ChartLegend_Top; &ocOrgChart.LegendStyle = "PT_ORGCHART_LEGEND";
(Optional) Specify image values.
&ocOrgChart.ImageLocation =1; &ocOrgChart.ImageHeight =150; &ocOrgChart.ImageMouseoverMagnificationFactor =150; &ocOrgChart.DefaultImage=PT_CHART_DEFAULTIMG;
Specify the breadcrumb data and the rowset and display properties. These are required only if your chart uses breadcrumbs.
&ocOrgChart.SetCrumbRecord(Record.QE_ORG_CRMB_DR); &ocOrgChart.SetCrumbData(&rsBreadCrumbs); &ocOrgChart.CrumbMaxDisplayLength = 24; &ocOrgChart.CrumbSeparatorImage = "PT_ORG_BRCRM_SEP"; &ocOrgChart.CrumbDescrStyle = "PT_ORGCHART_BRDCRM";
(Optional) Specify the maximum number of nodes that will appear in the pop-up without a vertical scroll bar.
&ocOrgChart.MaxPopUpDisplayNode = 2;
(Optional) Assign style class names to control the styles of each node descriptor.
If you do not specify a style class name, then the PeopleTools default style class is used.
This example uses the default style class names, so this segment of code could be omitted. It is shown for demonstration purposes only.
&ocOrgChart.NodeDescr1Style = "PT_ORGNODE_DESC1"; &ocOrgChart.NodeDescr2Style = "PT_ORGNODE_DESC2"; &ocOrgChart.NodeDescr3Style = "PT_ORGNODE_DESC3"; &ocOrgChart.NodeDescr4Style = "PT_ORGNODE_DESC4"; &ocOrgChart.NodeDescr5Style = "PT_ORGNODE_DESC5"; &ocOrgChart.NodeDescr6Style = "PT_ORGNODE_DESC6"; &ocOrgChart.NodeDescr7Style = "PT_ORGNODE_DESC7";
(Optional) Assign style class names to control the styles of each pop-up node descriptor.
If you do not specify a style class name, then the PeopleTools default style sheet is used.
This example uses the default style class names, so this segment of code could be omitted. It is shown for demonstration purposes only.
&ocOrgChart.PopupNodeDescr1Style = "PT_POPNODE_DESC1"; &ocOrgChart.PopupNodeDescr2Style = "PT_POPNODE_DESC2"; &ocOrgChart.PopupNodeDescr3Style = "PT_POPNODE_DESC3"; &ocOrgChart.PopupNodeDescr4Style = "PT_POPNODE_DESC4"; &ocOrgChart.PopupNodeDescr5Style = "PT_POPNODE_DESC5"; &ocOrgChart.PopupNodeDescr6Style = "PT_POPNODE_DESC6"; &ocOrgChart.PopupNodeDescr7Style = "PT_POPNODE_DESC7"; &ocOrgChart.PopupNodeDescr8Style = "PT_POPNODE_DESC8"; &ocOrgChart.PopupHeaderStyle = "PT_POPNODE_HEADER";
Image: Rating box chart
The PeopleCode used in the following example creates this rating box chart:
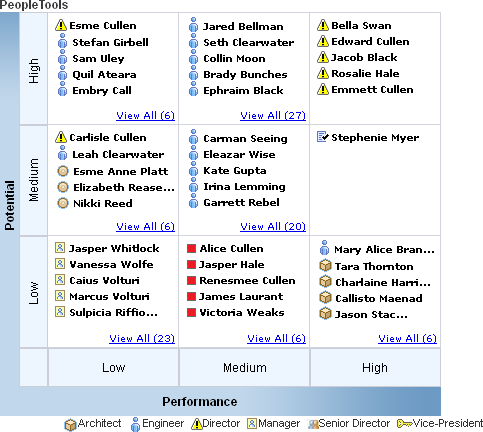
Follow these steps to create a rating box chart.
Create the rating box chart node record.
Clone the PTRATINGBOX_SBR subrecord to create an application-specific subrecord.
Create a new application-specific record and insert the new subrecord.
Add a chart control to a page in Application Designer.
Add the rating box chart node record to the component.
This record must be in the component buffer. If you do not want to give the user access to the data you can add the record to a hidden grid on the page. Alternatively, you can place it on another page in the same component.
Add PeopleCode.
In an event such as PreBuild, add PeopleCode to create a component buffer rowset that references the rating box chart node record.
Add PeopleCode, probably in the page Activate event, to populate the rowset and define the chart.
Add FieldChange PeopleCode that will execute when drag-and-drop events occur.
PeopleCode for the Rating Box Chart Example
Complete these steps to create a rating box chart:
Prepare the data for the chart.
Typically, write PeopleCode in an event such as PreBuild to populate a rowset with the data you want to display in the rating box chart.
Component Rowset &rs, &rsRatingBox; Component RatingBoxChart &rbRatingBoxChart; /* the record QE_RATEBOX_DATA has the data for the RATING BOX chart */ &rs = CreateRowset(Record.QE_RATEBOX_DATA); &rs.Fill("Where QE_RATEBOX_TC=:1", QE_RATEBOX_TC.QE_RATEBOX_TC.Value); /* Get the grid on the page for the node data and initalize to nulls */ &rsGrid = GetLevel0()(1).GetRowset(Scroll.QE_RATEBOX_TC); &rsGrid.Flush(); /* Copy data from database table, QE_RATEBOX_DATA, to the level 1 component rowset associated with the component derived working record QE_RATEBOX_DR */ &rs.CopyTo(&rsGrid, Record.QE_RATEBOX_DATA, Record.QE_RATEBOX_DR);
Define the RatingBoxChart.
Add PeopleCode in an event such as Activate.
Instantiate a RatingBoxChart object using the GetRatingBoxChart built-in function to reference the page control field of the chart.
Component Rowset &rs, &rsGrid; Component RatingBoxChart &rbRatingBoxChart; &rbRatingBoxChart = GetRatingBoxChart(QE_CHART_DUMREC.QE_CHART_FIELD);
Set the required display properties for the chart.
&rbRatingBoxChart.XaxisBoxNum = 3; &rbRatingBoxChart.YaxisBoxNum = 3; &rbRatingBoxChart.PopUpWidth = 200; &rbRatingBoxChart.PopUpHeight = 200; &XAxisArray = CreateArray("Low", "Medium", "High"); &YAxisArray = CreateArray("Low", "Medium", "High"); &rbRatingBoxChart.SetXAxisLabels(&XAxisArray); &rbRatingBoxChart.SetYAxisLabels(&YAxisArray);
(Optional) Specify other values for the chart display properties.
If you do not specify these properties, the following default value will be used:
&rbRatingBoxChart.IsDragable = True; &rbRatingBoxChart.BoxMaxDisplayItems = 3; &rbRatingBoxChart.NDMaxDisplayDescLength = 25; &rbRatingBoxChart.ShowNodeDescription = True; &rbRatingBoxChart.GridLineType = %ChartLine_Solid; &rbRatingBoxChart.XAxisTitle = "Performance"; &rbRatingBoxChart.YAxisTitle = "Potential"; &rbRatingBoxChart.NDMaxDisplayDescLength = 15; &rbRatingBoxChart.BoxMaxDisplayItems = 5; &rbRatingBoxChart.Height = 400; &rbRatingBoxChart.Width = 400; &rbRatingBoxChart.ShowNodeDescription = true; &rbRatingBoxChart.GridLineType=%ChartLine_Solid; &rbRatingBoxChart.IsDragable = True; &rbRatingBoxChart. BoxMaxDisplayItems= 1; &rbRatingBoxChart. NDMaxDisplayDescLength = 50;
(Optional) Assign style class names for the chart and x- and y-axis title and labels.
If you do not specify a style class name, the PeopleTools default style sheet is used.
&rbRatingBoxChart.Style = "PSCHARTDEFAULT"; &rbRatingBoxChart.XAxisTitleStyle = "PT_RATBOX_XTTL"; &rbRatingBoxChart.YAxisTitleStyle = "PT_RATBOY_YTTL"; &rbRatingBoxChart.XAxisLabelStyle = "PT_RATBOX_XAXIS"; &rbRatingBoxChart.YAxisLabelStyle = "PT_RATBOX_YAXIS";
(Optional) Specify title and legend properties.
&rbRatingBoxChart.MainTitle = "Development Division"; &rbRatingBoxChart.MainTitleStyle="PT_RATBOX_TITLE" &rbRatingBoxChart.HasLegend = True; &rbRatingBoxChart.LegendPosition = %ChartLegend_Bottom; &LegendArray = CreateArray("Architect", "Engineer", "Director", "Manager", "Senior Director", "Vice-President"); &LegendImgArray = CreateArray("PT_ACECUBE", "PT_WF_PERSON","PT_STATUS_ALERT_ICN", "PT_STATUS_ASSIGNED_ICN", "QE_SUCCSORGCHART", "QE_KPERSON"); &rbRatingBoxChart.SetLegend(&LegendArray); &rbRatingBoxChart.SetLegendImg(&LegendImgArray);
Set the node data rowset and record.
The chart refreshes when the SetRBNodeData method is called.
/* Set the node data rowset and the node record. */ &rbRatingBoxChart.SetRBNodeData(&rsGrid); &rbRatingBoxChart.SetRBNodeRecord(Record.QE_RATEBOX_DR);
Add FieldChange PeopleCode on the fields PTXASISRATINGS and PTYAXISRATINGS if you want to perform other logic after the drag and drop operation.
This example shows a call to the SetRBNodeData function to redraw the chart the user changes the values in either of these two fields in the component:
/* FieldChange PeopleCode for fields PTXAXISRATINGS and PTYAXISRATINGS*/ &rbRatingBoxChart.SetRBNodeData(&rsGrid);
You can also create a Chart class chart using an iScript. The following example creates a chart, populates it, and then sends the chart URL to a Response object. The complete code sample is shown.
Note: You cannot create a chart from gauge classes, the Gantt class, the OrgChart class, or the RatingBoxChart class using an iScript.
Function IScript_GetChartURL()
Local Chart &cChart;
Local Rowset &rsRowset;
Local string &sMap;
Local string &sURL;
&cChart = CreateObject("Chart");
&rsRowset = CreateRowset(Record.QE_CHART_RECORD);
&rsRowset.Fill("where QE_CHART_REGION= :1", "MIDWEST");
&cChart.SetData(&rsRowset);
&cChart.Width = 400;
&cChart.Height = 300;
&cChart.SetDataYAxis(QE_CHART_RECORD.QE_CHART_SALES);
&cChart.SetDataXAxis(QE_CHART_RECORD.QE_CHART_PRODUCT);
&cChart.SetDataSeries(QE_CHART_RECORD.QE_CHART_REGION);
&cChart.HasLegend = True;
&cChart.LegendPosition = %ChartLegend_Right;
&sURL = %Response.GetChartURL(&cChart);
&sMap = &cChart.ImageMap;
%Response.Write("<HTML><IMG SRC=");
%Response.Write(&sURL);
%Response.Write(" USEMAP=#THEMAP></IMG><MAP NAME=THEMAP>");
%Response.Write(&sMap);
%Response.Write("</MAP></HTML>");
End-Function;
See CreateObject.
Complete these steps to create a chart using an iScript:
Create the chart object.
For this example, no chart control is available on a page to be referenced. To create the chart object, use the CreateObject function. The string passed in to the function must be the name of the class you are instantiating. For instance, to instantiate a chart from the Chart class, pass the string “Chart”.
&cChart = CreateObject("Chart");
Create a rowset for the chart data and set the chart data.
The CreateRowset function creates a standalone rowset data structure. Use the Fill method to populate the empty rowset with data. The SetData method associates the rowset data with the chart.
&rsRowset = CreateRowset(Record.QE_CHART_RECORD); &rsRowset.Fill("where QE_CHART_REGION= :1", "MIDWEST"); &cChart.SetData(&rsRowset);
Set the height and width of the chart.
Because the chart is not associated with a chart control on a page, you have to specify the size of the chart image to be generated using the Height and Width properties. The unit of measurement for both of these properties is pixels.
&cChart.Width = 400; &cChart.Height = 300;
Set the data axes, series, and legend for the chart.
As with all charts, you must set the data axes. If necessary for your data, also set the data series and the legend.
&cChart.SetDataYAxis(QE_CHART_RECORD.QE_CHART_SALES); &cChart.SetDataXAxis(QE_CHART_RECORD.QE_CHART_PRODUCT); &cChart.SetDataSeries(QE_CHART_RECORD.QE_CHART_REGION); &cChart.HasLegend = True; &cChart.LegendPosition = %ChartLegend_Right;
Generate the URL for the chart.
Use the GetChartURL method of the Response class to generate the URL that will reference the chart data.
&sURL = %Response.GetChartURL(&cChart);
Generate the image map.
Use the ImageMap property to generate the image map for the chart. The Response object uses this value to draw the map.
&sMap = &cChart.ImageMap;
Create the response chart.
Use the Write Response class method to generate the chart. Note that first, the data is set using the URL generated with GetChartURL, and then the image map is used.
%Response.Write("<HTML><IMG SRC="); %Response.Write(&sURL); %Response.Write(" USEMAP=#THEMAP></IMG><MAP NAME=THEMAP>"); %Response.Write(&sMap); %Response.Write("</MAP></HTML>");
Note: If one or more labels does not render in the chart, increase the width of the chart, use shorter label text, or reduce the data set being charted.