Using CDATA in an XML File Generated Through XML File Layout
Utilizing BI Publisher’s built-in support for Rich Text data (using html2fo function in form field) requires the use of CDATA sections for RTE data elements in an XML file. However, when using XML File Layout to create the XML file, there is currently no native support for creating these CDATA sections. As a result, the CDATA sections should be added manually prior to writing the XML file.
The following example illustrates how to manually add the CDATA sections.
Consider the following record QE_APPL_RESUME, having character fields IDENTIFIER and QE_RESUME_TEXT, the latter containing RTE data.
select * from PS_QE_APPL_RESUME;
IDENTIFIER QE_RESUME_TEXT
------------- --------------------------------------------------------------
12231 <p><strong>RTE Text</strong><br />containing <,>,", and &</p>
The following screenshot shows XML File Layout defined for this record.
Image: XML File Layout
This example illustrates the XML File Layout defined for the sample record.
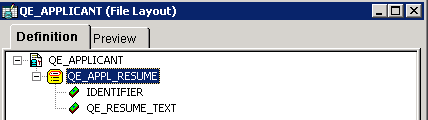
The following PeopleCode is used to generate an XML file using the WriteRecord() method in XML File Layout:
Local Record &Rec;
Local File &fXML;
Local SQL &SQL;
&fXML = GetFile("c:\temp\QE_APPLICANT1.xml", "W", "UTF-8", %FilePath_Absolute);
If &fXML.IsOpen Then
If &fXML.SetFileLayout(FileLayout.QE_APPLICANT) Then
&Rec = CreateRecord(Record.QE_APPL_RESUME);
&SQL = CreateSQL("%Selectall(:1)", &Rec);
While &SQL.Fetch(&Rec)
&fXML.WriteRecord(&Rec);
&fXML.WriteLine("</QE_APPL_RESUME>");
End-While;
End-If;
End-If;
&fXML.Close();
The following is a snippet from the generated XML file:
<QE_APPL_RESUME>
<IDENTIFIER>12231</IDENTIFIER>
<QE_RESUME_TEXT><p><strong>RTE Text<
/strong><br />containing <,>,", and &</p>
</QE_RESUME_TEXT>
</QE_APPL_RESUME>
BI Publisher’s built-in support for Rich Text data requires that RTE text must not be escaped with entity reference characters, but rather the RTE text must be in a CDATA block for the BIP Reporting Engine to be able to process it.
The way around this is to use WriteString() method instead of WriteRecord() and manually write the CDATA section for each individual field that contains RTE data:
The following snippet illustrates the modified PeopleCode:
Local Record &Rec;
Local File &fXML;
Local SQL &SQL;
&fXML = GetFile("c:\temp\QE_APPLICANT2.xml", "W", "UTF-8", %FilePath_Absolute);
If &fXML.IsOpen Then
If &fXML.SetFileLayout(FileLayout.QE_APPLICANT) Then
&Rec = CreateRecord(Record.QE_APPL_RESUME);
&SQL = CreateSQL("%Selectall(:1)", &Rec);
While &SQL.Fetch(&Rec)
rem &fXML.WriteRecord(&Rec);
&fXML.WriteLine("<QE_APPL_RESUME>");
&fXML.WriteString("<IDENTIFIER>");
&fXML.WriteString(&Rec.IDENTIFIER.Value);
&fXML.WriteString("</IDENTIFIER>");
&fXML.WriteString("<QE_RESUME_TEXT><![CDATA[");
&fXML.WriteString(&Rec.QE_RESUME_TEXT.Value);
&fXML.WriteString("]]></QE_RESUME_TEXT>");
&fXML.WriteLine("</QE_APPL_RESUME>");
End-While;
End-If;
End-If;
&fXML.Close();
It is essentially the same as the original code, but uses WriteString() to individually write out all fields from the record that contains the RTE field. This is necessary to be able to write the CDATA section string around the RTE field contents.
The following snippet is from the generated XML file:
<QE_APPL_RESUME>
<IDENTIFIER>12231</IDENTIFIER><QE_RESUME_TEXT>
<![CDATA[<p><strong>RTE Text</strong><br />containing <,>,", and &</p>]]>
</QE_RESUME_TEXT></QE_APPL_RESUME>
<QE_APPL_RESUME>