Creating Calculated Mapping Application Class Objects
This section discusses how to:
Create an application package.
Create an application class.
Access staged field values.
Access file field values.
It is assumed that the person who will be responsible for creating and managing the application classes is a seasoned engineer who is comfortable with working with PeopleTools Application Designer, is well versed in writing PeopleCode, and has detailed knowledge of the referential integrity associated with the tables referenced by File Parser.
Warning! The examples presented in this section have not been exposed to any rigorous testing scenarios. If you decide to use these examples in any form, it is strongly recommended that a complete and thorough set of test cycles be run before moving to production.
To create an application package:
Open PeopleTools Application Designer.
File/New/Application Package.
Save the empty application package with a meaningful name.
At the root level of the application package, insert a new application package. Repeat as necessary to produce a meaningful application package structure.
To create an application class:
At the appropriate level within your application package, insert an application class.
Save it with a meaningful name, reflective of what the application class is doing and is intuitive for the end user to select when defining the File Parser Mapping Definitions.
The following shows an example:
This example illustrates the fields and controls on the Application package example. You can find definitions for the fields and controls later on this page.
The following application class code illustrates how to reference data elements that reside at the Staging level.
Note: In the following code, only the code formatted in bold can be changed by the developer. Rest of the code is required to successfully run or reference staging field values.
import SCC_FILE_PARSER:UTIL:FieldCalculationAbstract;
import SCC_FILE_PARSER:MODEL:Results:ResultsField;
import SCC_FILE_PARSER:MODEL:Results:ResultsRecord;
import SCC_FILE_PARSER:MODEL:Results:ResultsCollection;
import SCC_FILE_PARSER:UTIL:Exception:FileParserException;
/**
* class FetchDegreeDescr
*
* @version 1.0
* @author Your Institution
*
* Module: Your Application
* Description: Your Description
*/
/**
* This Application Class is designed to read the incoming Degree value
* residing on the mapped/staged Record Definition: SCC_STG_EXTDEGR, Field: DEGREE,
* and then obtain the description from the table PS_DEGREE_TBL.
*
* If found, pass back the description, else pass back the string ’NOT FOUND’.
*/
class FetchDegreeDescr extends SCC_FILE_PARSER:UTIL:FieldCalculationAbstract
/* public methods */
method FetchDegreeDescr();
method calculateValue(&ResultsFieldIn As SCC_FILE_PARSER:MODEL:Results:ResultsField,
&ResultsCollectionIn As SCC_FILE_PARSER:MODEL:Results:ResultsCollection) Returns any;
private
method GetFileData();
method GetFieldReferences();
instance string &Degree;
instance string &DegreeDescrOut;
instance SCC_FILE_PARSER:MODEL:Results:ResultsField &ResultsField;
instance SCC_FILE_PARSER:MODEL:Results:ResultsCollection &ResultsCollection;
instance SCC_FILE_PARSER:MODEL:Results:ResultsField &DegreeField;
end-class;
/**
* Instantiate the App. Class.
*/
method FetchDegreeDescr
%Super = create SCC_FILE_PARSER:UTIL:FieldCalculationAbstract();
end-method;
/**
* This method is the main driver that passes back the derived value.
*/
method calculateValue
/+ &ResultsFieldIn as SCC_FILE_PARSER:MODEL:Results:ResultsField, +/
/+ &ResultsCollectionIn as SCC_FILE_PARSER:MODEL:Results:ResultsCollection +/
/+ Returns Any +/
/+ Extends/implements SCC_FILE_PARSER:UTIL:FieldCalculationAbstract.CalculateValue +/
&ResultsCollection = &ResultsCollectionIn;
&ResultsField = &ResultsFieldIn;
%This.GetFileData();
/**
* Pass back the derived value to the field calling this Calculated Application Class.
*/
Return &DegreeDescrOut;
end-method;
/**
* This method uses the obtained degree value as the key in the criteria to obtain the
* description from the table PS_DEGREE_TBL.
*/
method GetFileData
Local string &DegreeDescr;
/**
* Call the method GetFieldReferences to obtain the incoming degree value.
*/
If &DegreeField = Null Then
%This.GetFieldReferences();
End-If;
&Degree = "";
/**
* Test to see if we found a degree value. If so, execute the SQL statement to
* obtain the description.
*/
If &DegreeField <> Null Then
&Degree = &DegreeField.FieldValue;
SQLExec("SELECT A.DESCR FROM PS_DEGREE_TBL A WHERE A.DEGREE =:1 AND A.EFF_STATUS =
’A’ AND A.EFFDT = (SELECT MAX(A1.EFFDT) FROM PS_DEGREE_TBL A1 WHERE A1.DEGREE =
A.DEGREE AND A1.EFFDT <= %DateIn(:2))", &Degree, %Date, &DegreeDescr);
/**
* Test to see if we found a degree description. If so, assign the value to be
* passed back.
* Otherwise, assign the text string ’NOT FOUND’.
*/
If &DegreeDescr = "" Then
&DegreeDescrOut = "NOT FOUND";
Else
&DegreeDescrOut = &DegreeDescr;
End-If;
End-If;
end-method;
method GetFieldReferences
Local integer &SegmentNbr;
Local integer &RecordRow;
Local SCC_FILE_PARSER:MODEL:Results:ResultsRecord &obj_SCC_STG_EXTDEGR;
&SegmentNbr = &ResultsField.ResultsRecord.SegmentNbr;
&RecordRow = &ResultsField.ResultsRecord.RecordRow;
/**
* Establish a reference to the staging record definition
* containing the incoming mapped field.
*/
&obj_SCC_STG_EXTDEGR = &ResultsCollection.GetResultsRecord(&SegmentNbr,
"SCC_STG_EXTDEGR", &RecordRow);
/**
* If the reference exists, establish a reference to the staging mapped field.
*/
If &obj_SCC_STG_EXTDEGR <> Null Then
&DegreeField = &obj_SCC_STG_EXTDEGR.GetResultsField("DEGREE");
End-If;
end-method;
To reference an incoming File Field value, you need to obtain two key values associated with that specific field. These key values are Segment Number and the Sort Order Number.
To obtain the required keys, follow these steps:
Step 1: Identify the File Fields you wish to access.
In this example, we want to access the incoming Home Phone Area Code and the Home Phone.
Step 2: Identify the Segment Number. This value is derived based upon the File Definition. If the File Definition does not contain Multiple Row Types (that is, you have not selected the Multiple Row Type check box), then the Segment Number will always be a value of 1 (one).
This example illustrates the fields and controls on the File Definition page - Multiple Row Types is not selected. You can find definitions for the fields and controls later on this page.
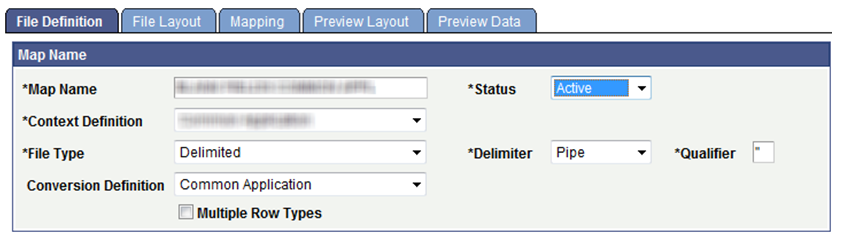
In this example, a Segment Number of 2 (two) would be used if fields contained within DTL_NAME are to be referenced:
This example illustrates the fields and controls on the File Definition page - Multiple Row Types is selected. You can find definitions for the fields and controls later on this page.
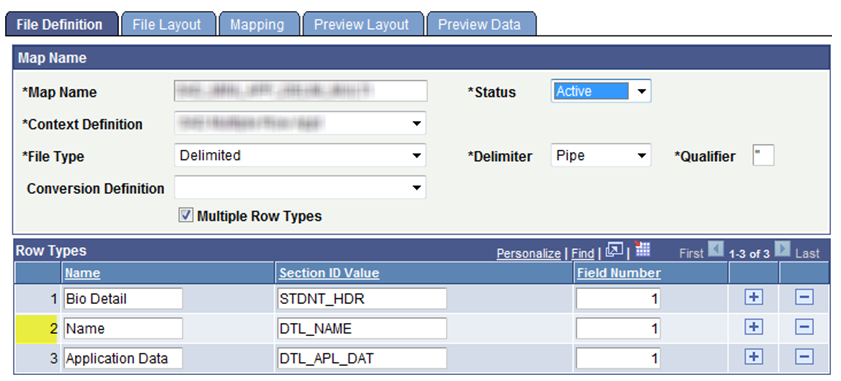
Step 3: Obtain the Sort Order Number(s). The Sort Order Number can be found on the File Layout page:
This example illustrates the fields and controls on the File Layout - Location tab. You can find definitions for the fields and controls later on this page.
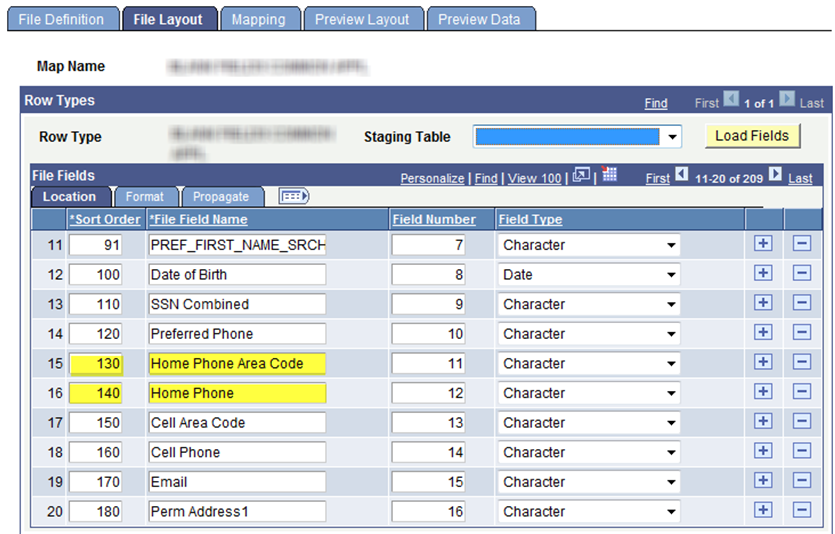
In the graphic we see that Home Phone Area Code has a Sort Order Number of 130, and the Home Phone Sort Order Number is 140.
Step 4: Write these values down to be referenced within your application class.
The following application class code illustrates how to reference data elements that reside at the incoming File Field level.
Note: In the following code, only the code formatted in bold can be changed by the developer. Rest of the code is required to successfully run or reference staging field values.
import SCC_FILE_PARSER:MODEL:Results:ResultsField;
import SCC_FILE_PARSER:UTIL:FieldCalculationAbstract;
import SCC_FILE_PARSER:MODEL:Results:ResultsFileFields;
import SCC_FILE_PARSER:MODEL:Results:ResultsCollection;
/**
* class BuildHomePhone
*
* @version 1.0
* @author Your Institution
*
* Module: Your Application
* Description: Your Description
*/
/**
* This Application Class is designed to read two values, Home Phone Area Code
* and Home Phone from the incoming File Fields. They will then be concatenated
* into one single field and passed back to the mapped field containing the
* Calculated mapping action/reference to this Application Class.
*/
class BuildHomePhone extends SCC_FILE_PARSER:UTIL:FieldCalculationAbstract
/* public methods */
method BuildHomePhone();
method calculateValue(&ResultsFieldIn As SCC_FILE_PARSER:MODEL:Results:ResultsField,
&ResultsCollectionIn As SCC_FILE_PARSER:MODEL:Results:ResultsCollection) Returns any;
private
instance string &FileFldValueOut;
instance string &FieldValueString01, &FieldValueString02;
instance SCC_FILE_PARSER:MODEL:Results:ResultsField &ResultsField;
instance SCC_FILE_PARSER:MODEL:Results:ResultsCollection &ResultsCollection;
instance SCC_FILE_PARSER:MODEL:Results:ResultsFileFields &FileFldValueField;
end-class;
/**
* Instantiate the App. Class.
*/
method BuildHomePhone
%Super = create SCC_FILE_PARSER:UTIL:FieldCalculationAbstract();
end-method;
/**
* This method is the main driver that passes back the derived value.
*/
method calculateValue
/+ &ResultsFieldIn as SCC_FILE_PARSER:MODEL:Results:ResultsField, +/
/+ &ResultsCollectionIn as SCC_FILE_PARSER:MODEL:Results:ResultsCollection +/
/+ Returns Any +/
/+ Extends/implements SCC_FILE_PARSER:UTIL:FieldCalculationAbstract.CalculateValue +/
Local integer &filesegout, &fldnumout;
&FileFldValueField = &ResultsCollectionIn.RFF;
/**
* Assign the appropriate value for the Segment Number to be passed to
* the Method FetchFileFieldValue.
*/
&filesegout = 1; /* File Segment Number will always be 1 for non-multiple row types. */
/**
* Assign the appropriate value for the File Field value to be passed to
* the Method FetchFileFieldValue.
*/
/* Home Phone Area Code */
&fldnumout = 130;
&FieldValueString01 = &FileFldValueField.FetchFileFieldValue(&filesegout, &fldnumout);
/* Home Phone */
&fldnumout = 140;
&FieldValueString02 = &FileFldValueField.FetchFileFieldValue(&filesegout, &fldnumout);
/**
* Test that we have values to build and pass back.
*/
If (&FieldValueString01 <> " " And
&FieldValueString02 <> " ") Then
/* Build the home phone number */
&FileFldValueOut = &FieldValueString01 | " " | &FieldValueString02;
Else
&FileFldValueOut = " ";
End-If;
Return &FileFldValueOut;
end-method;