Order Management拡張のテスト
オーダー管理拡張をテストします。
コードの検証
「拡張機能の編集」ページの定義領域にコードを入力し、検証をクリックして設計時コードを検証します。 オーダー管理では、カッコ、セミコロンなどの正しい使用方法など、コードが正しく書式設定されていることを確認します。
-
無効な属性またはメソッドへの参照
-
メッセージ名とトークンの間の参照が正しくありません
-
webサービス名への参照が正しくありません
ロギングを使用したコードのテスト
開発中にデバッグ・テストを含めて、コードをテストし、期待どおりに動作することを確認します。
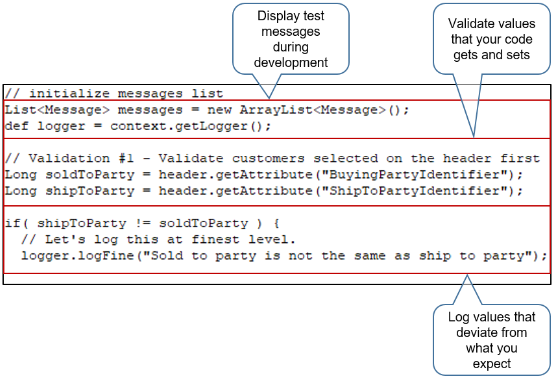
ノート
-
テストが終了し、拡張機能を本番環境にデプロイする準備ができたら、テスト・コードをコメントするかわりに、デバッグ・ロジックを変更してログ・ファイルに書き込みます。 ログ・ファイルへの書込みは、今後のトラブルシューティングに役立ちます。 本番環境のログ・ファイルにデータを書き込む場合は、Oracle Supportまたは顧客管理者に連絡して、アプリケーション診断データを含むログ・ファイルへの表示アクセス権を取得する必要があります。
-
ログ・ファイルを使用してパフォーマンスを評価します。 たとえば、多くの拡張コードを記述する場合は、パフォーマンスを調べてモニターし、コードがパフォーマンスに悪影響を及ぼさないようにします。
たとえば、拡張コードは通常、オーダー入力担当者が発行をクリックした直後に大量の検証を実行します。 オーダー管理で送信の完了に長い時間(5分など)が必要な場合、コードを詳細に調べて、合理化できるロジックが含まれているかどうかを判断する必要があります。
デバッグ・メソッドの使用
開発中にコードの様々なポイントでDebugメソッドを使用して、作業領域オーダー管理で確認できる属性に変数およびその他の値を記述します。 この例では、debug
を3回コールし、デバッグの内容をShippingInstructions属性に書き込みます。
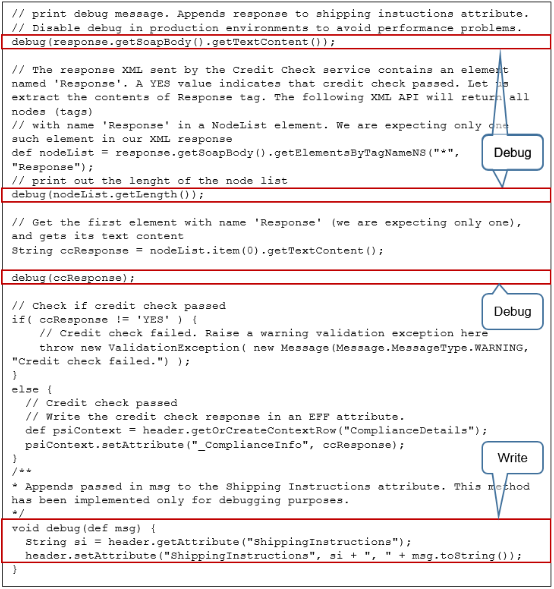
次に、これらの内容がオーダー管理作業領域の出荷指示属性に表示されるようにコードを記述し、この表示出力を確認して、コード内の様々なポイントに存在する様々な属性および変数の値を決定できます。
本番にデプロイする準備ができたら、void debug
を変更します。 たとえば、このコードを使用してデバッグの内容を記述するとします。
void debug(def msg)
{String si = header.getAttribute("ShippingInstructions");
header.setAttribute("ShippingInstructions", si + ", " + msg.toString());
このコードをログに書き込むように変更します。 header.setAttribute
をlogger.logFine
に置き換えます。 たとえば:
void debug(def msg)
{String si = header.getAttribute("ShippingInstructions");
logger.logFine("ShippingInstructions", si + ", " + msg.toString());
この方法を使用すると、コード内の1行のみを変更できます。これは、debug
をコールする10行の場合に役立ちます。 また、debugメソッドを呼び出す各行をコメント化することもできますが、コメント化によって、debugを呼び出す行がないか、debugをコールしないがコード・ロジックにとって重要な行を誤ってコメントするリスクが生じます。
この例の完全なコードを次に示します。
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
def poNumber = header.getAttribute("CustomerPONumber");
if( poNumber != "CreditCheck" ) return;
// get attribute to populate in the payload
String customer = header.getAttribute("BillToCustomerName");
Long accountId = header.getAttribute("BillToCustomerIdentifier");
BigDecimal amount = new BigDecimal(1000);
// prepare the payload
String payLoad = "<ns1:creditChecking xmlns:ns1=\"http://xmlns.oracle.com/apps/financials/receivables/creditManagement/creditChecking/creditCheckingService/types/\">" +
"<ns1:request xmlns:ns2=\"http://xmlns.oracle.com/apps/financials/receivables/creditManagement/creditChecking/creditCheckingService/\">" +
"<ns2:CustomerName>" + customer + "</ns2:CustomerName>" +
"<ns2:CustomerAccountNumber>" + accountId + "</ns2:CustomerAccountNumber>" +
"<ns2:RequestType>Authorization</ns2:RequestType>" +
"<ns2:PriceType>ONE_TIME</ns2:PriceType>" +
"<ns2:RecurrencePeriod></ns2:RecurrencePeriod>" +
"<ns2:RequestAuthorizationAmount currencyCode=\"USD\">" + amount + "</ns2:RequestAuthorizationAmount>" +
"<ns2:RequestAuthorizationCurrency>USD</ns2:RequestAuthorizationCurrency>" +
"<ns2:ExistingAuthorizationNumber></ns2:ExistingAuthorizationNumber>" +
"<ns2:Requestor>ar_super_user</ns2:Requestor>" +
"</ns1:request>" +
"</ns1:creditChecking>";
// invoke the Check Check service using web service connector name 'CreditCheckService'. The connector is set up using task 'Manage External Interface Web Service Details'. Since this is an external service that is secured
// using message protection policy, we have registered the the https URL of the service
def response = context.invokeSoapService("CreditCheckService", payLoad);
// print a debug message. This appends the entire response to the shipping instuctions attribute.
// To avoid performance problems, you must comment out all debug statements in your production environment.
debug(response.getSoapBody().getTextContent());
// The response XML sent by the Credit Check service contains an element named 'Response'. A YES value indicates that credit check passed. Let us extract the contents of Response tag. The following XML API will return all nodes (tags)
// with name 'Response' in a NodeList element. We are expecting only one such element in our XML response
def nodeList = response.getSoapBody().getElementsByTagNameNS("*", "Response");
// print out the lenght of the node list
debug(nodeList.getLength());
// Get the first element with name 'Response' (we are expecting only one), and gets its text content
String ccResponse = nodeList.item(0).getTextContent();
debug(ccResponse);
// Check if credit check passed
if( ccResponse != 'YES' ) {
// Credit check failed. Use a warning validation exception here.
throw new ValidationException( new Message(Message.MessageType.WARNING, "Credit check failed.") );
}
else {
// Credit check passed
// Write the credit check response in an EFF attribute.
def psiContext = header.getOrCreateContextRow("ComplianceDetails");
psiContext.setAttribute("_ComplianceInfo", ccResponse);
}
/**
* Appends passed in msg to the Shipping Instructions attribute. This method has been implemented only for debugging purposes.
*/
void debug(def msg) {
String si = header.getAttribute("ShippingInstructions");
header.setAttribute("ShippingInstructions", si + ", " + msg.toString());
}