オーダー明細の延長
オーダー管理拡張を使用して、既存のオーダー明細とまだ関連していない新規オーダー明細を作成できます。
明細のステータスの確認
出荷済、請求済、クローズ済または取消済ステータスのオーダー明細の属性を更新できない可能性があるため、明細を更新する前にオーダー明細のステータスを確認してください。
拡張機能でShipping Method(出荷方法)属性を更新し、明細がすでに履行されてクローズされているかどうかを判断する条件を拡張の最初に追加します。 その条件がtrueの場合、出荷方法属性を更新しても意味がなく、拡張を終了できます。
新規オーダー明細の作成
createNewLineメソッドを使用して新しいオーダー明細を作成するコード・スニペットの例を次に示します。
header.createNewLine
ノート
- CreateLineParams()パラメータを、createNewLineメソッドで使用できます。
- 既存の明細の値に従って条件を指定して、新しい明細を追加するかどうかを決定することもできます。
- createNewLineメソッドをオーダー・ヘッダーで使用します。
- 明細の作成時に、オーダー明細属性の値を設定するには、オーダー明細のsetAttributeメソッドを使用します。 これを実行しないと、オーダー管理拡張によって、オーダー・ヘッダーからオーダー明細に値がカスケードされます。
- オーダー管理拡張を使用して明細を追加すると、AddedByExtensionFlag属性がYに設定されるので、拡張によって明細が追加されたかどうかをこの属性で判断できます。
createNewLineメソッドは次の場合にのみ使用します。
- Oracle Pricingで価格設定する明細。 ソース・システムで価格設定する明細でcreateNewLineを使用すると、エラーが発生します。
- 「保存時」イベント。 他のイベントでcreateNewLineを使用すると、エラーが発生します。
- 標準品目を含む明細を作成する場合。 createNewLineを使用してサブスクリプションまたはカバレッジを含む明細を作成すると、エラーが発生します。
CustomerPONumber属性に値CreateStandaloneLine
が含まれ、行にAS54888品目が含まれるオーダーの新規行を作成する例を次に示します。
//---
import oracle.apps.scm.doo.common.extensions.CreateLineParams;
def poNumber = header.getAttribute("CustomerPONumber");
if(poNumber != "CreateStandaloneLine") return;
def createLineParams = new CreateLineParams(); // Initialize the new variable so we can send the required attributes.
createLineParams.setProductNumber("AS54888"); // Add the AS54888 item to the new line.
createLineParams.setOrderedUOM("Each"); // Set the unit of measure to Each.
createLineParams.setOrderedQuantity(10); // Set the ordered quantity to 10.
header.createNewLine(createLineParams); // Use the attribute values from the above lines to create the line. The extension will cascade the other ship to and bill to attribute values from the order header.
--//
AS54888の製品識別子が2157で、番号のかわりに識別子を使用する必要があるとします。 番号のかわりに識別子を指定するには、次の行を置換します:
createLineParams.setProductNumber("AS54888");
次の行で:
createLineParams.setProductIdentifier(2157);
別のオーダー明細に関連付けられていないオーダー明細の作成
標準品目またはサービス品目があり、既存の明細を参照する必要がないオーダー明細を作成、読取り、更新または取消できますが、オーダー管理では販売オーダーの価格を設定する必要があります。
拡張機能により、AddedByExtensionFlag属性が自動的に更新され、行が追加されたことが示されます。
この例では、条件に従って運送費明細を追加します:
- オーダー明細にAS54888品目が含まれ、明細の数量が5未満の場合は、運送費明細を追加します。
また、元のline.Youに運送明細の番号が追加され、そのデータを使用して、後続の保存または改訂中に別の運送費明細が追加されないようにできます。
運送費明細の追加後にAS54888を含む明細をコピーすると、Order Managementでは拡張可能フレックスフィールドもコピーされます。 コードでは、コピーした明細の運送費明細は追加されません。
// Import the objects that you need to add an order line.
import oracle.apps.scm.doo.common.extensions.CreateLineParams;
// This code is for testing purposes. It makes sure the extension doesn't affect other users.
// It looks at the customer's purchase order number:
if (!"SNADDFREIGHT".equals(header.getAttribute("CustomerPONumber"))) return;
def lines = header.getAttribute("Lines");
while(lines.hasNext()){
def line = lines.next();
def float orderQuantity = line.getAttribute("OrderedQuantity")
def product = line.getAttribute("ProductNumber");
def linenumber = line.getAttribute("LineNumber");
// Use this code to debug the extension:
debug(" Orig Line " + linenumber + " " + orderQuantity.toString() + product + line.getAttribute("AddedByExtensionFlag"));
def MinimumOrdQty = 5
if (product == "AS54888") {
//If the ordered quantity is more than 5, then continue to next code line.
if (orderQuantity > MinimumOrdQty) continue;
else {
/* Get the extensible flexfield's context for the line. If the context isn't available, then it means the extension didn't add the new line, so you use getContext instead of getOrCreate.
*/
def effContext = line.getContextRow("FulfillLineContext3");
//If the extensible flexfield's context is available, and if the segment contains data, then go to next code line.
if (effContext != null && effContext.getAttribute("_FL3AttributeChar1") != null) continue;
//If your code reaches this point, then the extension didn't add the freight line.
def createLineParams = new CreateLineParams();
createLineParams.setProductNumber("Freight_GST_QPMapper");
createLineParams.setOrderedUOM("Each");
createLineParams.setOrderedQuantity(1);
/* The extension will automatically use header.createNewLine to create the new line.
This line will include the price just as it would if you added it in the Order Management work area. The extension sets the AddedByExtensionFlag attribute to Y on the new line.
If you want to add a free item, then use line.createNewLine instead of header.createNewLine. You can't edit the free line, the free line won't contain a price, and the extension won't set the AddedByExtensionFlag attribute on the free line to Y.*/
fline = header.createNewLine(createLineParams);
// Use code line to debug this extension:
debug("Freight " + fline.getAttribute("LineNumber"));
// Now that you added the freight line, you can add those details to the extensible flexfield on the original line:
def effContext1 = line.getOrCreateContextRow("FulfillLineContext3");
effContext1.setAttribute("_FL3AttributeChar1",fline.getAttribute("LineNumber"));
}
}
}
void debug(String msg) {
header.setAttribute("ShippingInstructions", header.getAttribute("ShippingInstructions") + msg + "\n");
}
社内資材転送の管理
社内資材転送の価格設定は凍結されているため、社内資材転送を含む販売オーダーではcreateNewLineメソッドを使用できません。
オーダーに社内資材転送が含まれる場合は、拡張で次の条件を使用して明細の追加をスキップします。
If (header.getAttribute("TransactionDocumentTypeCode") == "TO") return;
拡張機能によって品目が自動的に追加されたかどうかを示します
Order Managementの作業領域をカスタマイズして、Order Management Extensionで品目が自動的に追加されたかどうかを示すことができます。 オーダー明細の品目の摘要に「Automatically added by system」というテキストを表示するとします。
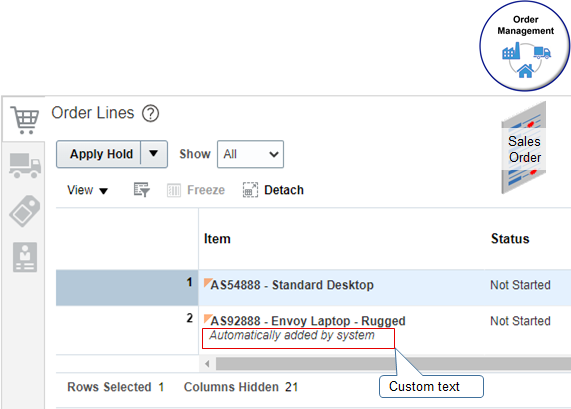
設定に使用する一般的なフローを次に示します。
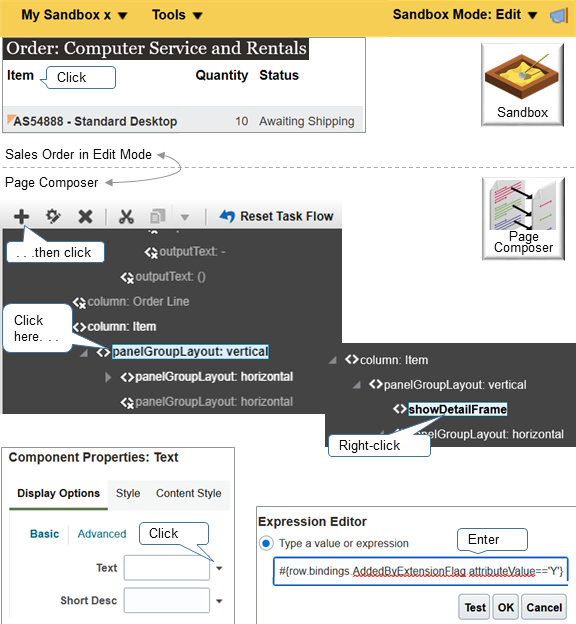
演習
- ページ・コンポーザを開きます。
- サンドボックスを作成し、ページ・コンポーザを有効にします。 詳細は、オーダー管理での属性の表示方法の変更を参照してください。
- 「オーダー管理」作業領域に移動し、「タスク」→「オーダーの管理」をクリックします。
- 「オーダーの管理」ページで、任意の販売オーダーを検索して開きます。
- オーダー・ページの右上隅にある「ログイン名」をクリックします。
- 設定およびアクション・メニューで、「ページを編集」をクリックします。
ページ・コンポーザは、別のユーザー・インタフェースで開き、オーダー管理作業領域の外側のフレームのように配置されます。 ページ・コンポーザを使用して、オーダー管理作業領域のユーザー・インタフェースをリアルタイムで変更できるようになりました。
- 販売オーダーの構成にアクセスします。
- ページ・コンポーザのStructure(構造)をクリックtab.
- 構造の表示/非表示、構造の表示ボタンを切り替えて、構造ウィンドウの位置を確認できます。 通常はページの下部にあります。
- 構造ウィンドウで、「ドック>右」をクリックします。
- 構造ウィンドウを展開して、その内容を確認します。
- 販売オーダーのオーダー明細で、「品目列ヘッダー」をクリックします。
- 構造ウィンドウが自動的に
column: Item
コンポーネントにスクロールされることに注意してください。
- 販売オーダーにテキスト・コンポーネントを追加します。
panelGroupLayout: vertical
コンポーネント内にテキストを追加するため、「panelGroupLayout : 垂直」をクリックします。- 構造ウィンドウの上部で、「プラス・アイコン」 ( +)をクリックします。
- コンテンツの追加ダイアログで、「コンポーネント」をクリックします。
- テキスト行の「追加」をクリックします。
- ページ・コンポーザによって、構造ウィンドウの
panelGroupLayout: vertical
コンポーネントのすぐ下に新しいshowDetailFrame: Text
コンポーネントが追加されたことに注意してください。 - コンテンツの追加ダイアログで、「クローズ」をクリックします。
- オーダー管理拡張を追加します。
- 新しい「showDetailFrame: テキスト」コンポーネントを右クリックし、「編集」をクリックします。
- 「コンポーネント・プロパティ」ダイアログの「表示オプション」タブの基本セクションで、テキスト属性の横にある「下向き矢印」をクリックし、「式ビルダー」をクリックします。
- 「式エディタ」ダイアログで、「値または式の入力」オプションを選択し、値を入力します。
#{row.bindings.AddedByExtensionFlag.attributeValue=='Y'}
- 「OK」をクリックします。
- 「コンポーネント・プロパティ」ダイアログで、「適用> OK」をクリックします。
- display.
にするテキストを追加
- 販売オーダーのItem(品目)列に、テキストを入力できる領域が含まれるようになりました。
- 「テキストの編集」をクリックし、表示するテキスト(「システムにより自動的に追加」など)を追加します。
アイコンの表示
アイコン名coverageinclude_ena.pngがあり、品目の一部として表示するとします。 ページ・コンポーザでオーダー管理拡張を使用して、次のものを追加できます:
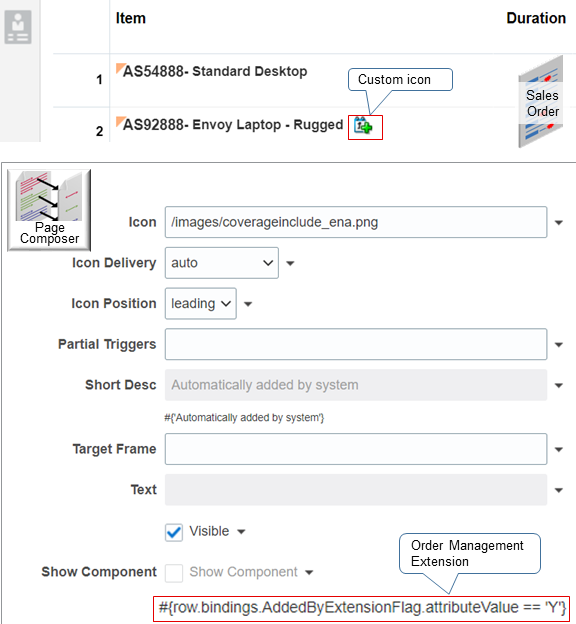
無料の品目を追加
条件に従って、フリー品目を既存の品目に追加できます。 明細の価格は0.00となり、更新できません。 拡張機能により、tranformedFrom属性が自動的に更新され、この行が既存の品目に追加されたことが示されます。 たとえば:
- オーダー明細にAS54888品目が含まれ、明細の数量が10を超える場合は、数量1の無料品目を追加します。
保存時イベントを使用します:
// import the objects that you need to add an order line
import oracle.apps.scm.doo.common.extensions.CreateLineParams;
// This code is for testing purposes. It makes sure the extension doesn't affect other users.
// It looks at the customer's purchase order number:
if (!"SNADDFREE".equals(header.getAttribute("CustomerPONumber"))) return;
def lines = header.getAttribute("Lines");
while(lines.hasNext()){
def line = lines.next();
def float orderQuantity = line.getAttribute("OrderedQuantity")
def product = line.getAttribute("ProductNumber");
def linenumber = line.getAttribute("LineNumber");
// Use this code to debug the extension:
debug(" Orig Line " + linenumber + " " + orderQuantity.toString() + product + line.getAttribute("AddedByExtensionFlag"));
if (product == "AS54888") {
//If the ordered quantity is less than or equal to 10, then continue to the next code line.
if (orderQuantity <= 10) continue;
else {
def isClosed = line.isClosed()
def isCanceled = line.isCanceled()
def isTransformed = line.isTransformed();
debug("closed " + isClosed + " canceled " + isCanceled + " transformed " + isTransformed);
//Make sure the current order line isn't closed, cancelled, or transformed.
if (isClosed || isCanceled || isTransformed) continue;
//Make sure you haven't already added a free item to the order line.
def freeLines = line.getTransformedLines();
// The above code line gives you a list of lines that you have added for the order line.
debug(" associated lines " + freeLines);
//If the above code returns an empty list, then the freeLines variable will be false
// If you need to check the count of items in the list, then use the size()method.
if (freeLines && freeLines.size() > 0) continue;
//If your code reaches this point, then the extension didn't add the free order line.
def createLineParams = new CreateLineParams();
createLineParams.setProductNumber("AS54888"); //Freight_GST_QPMapper");
createLineParams.setOrderedUOM("Each");
createLineParams.setOrderedQuantity(orderQuantity);
/* The extension will automatically use line.createNewLine to add the order line that has the free item.
This order line will have a price of 0 and you can't update it.
If you want to add an independent line that's priced and that you can update, then use header.createNewLine instead of line.createNewLine.
*/
fline = line.createNewLine(createLineParams);
// Use this code line to debug this extension:
debug("Free " + fline.getAttribute("LineNumber"));
}
}
}
オーダー明細からの手動価格調整の取得
Iimport oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
if (!"PMC TEST".equals(header.getAttribute("CustomerPONumber"))) return;
List < Message > messages = new ArrayList < Message > ();
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def MPAS = line.getAttribute("ManualPriceAdjustments");
while (MPAS.hasNext()) {
def mpa = MPAS.next();
messages.add(new Message(Message.MessageType.ERROR, "AdjustmentElementBasis is " + mpa.getAttribute("AdjustmentElementBasis")));
messages.add(new Message(Message.MessageType.ERROR, "AdjustmentTypeCode is " + mpa.getAttribute("AdjustmentTypeCode")));
messages.add(new Message(Message.MessageType.ERROR, "ChargeDefinitionCode is " + mpa.getAttribute("ChargeDefinitionCode")));
messages.add(new Message(Message.MessageType.ERROR, "ChargeRollupFlag is " + mpa.getAttribute("ChargeRollupFlag")));
messages.add(new Message(Message.MessageType.ERROR, "Comments is " + mpa.getAttribute("Comments")));
messages.add(new Message(Message.MessageType.ERROR, "ManualPriceAdjustmentId is " + mpa.getAttribute("ManualPriceAdjustmentId")));
messages.add(new Message(Message.MessageType.ERROR, "ParentEntityCode is " + mpa.getAttribute("ParentEntityCode")));
messages.add(new Message(Message.MessageType.ERROR, "ParentEntityId is " + mpa.getAttribute("ParentEntityId")));
messages.add(new Message(Message.MessageType.ERROR, "PricePeriodicityCode is " + mpa.getAttribute("PricePeriodicityCode")));
messages.add(new Message(Message.MessageType.ERROR, "ReasonCode is " + mpa.getAttribute("ReasonCode")));
messages.add(new Message(Message.MessageType.ERROR, "SequenceNumber is " + mpa.getAttribute("SequenceNumber")));
messages.add(new Message(Message.MessageType.ERROR, "SourceManualPriceAdjustmentIdentifier is " + mpa.getAttribute("SourceManualPriceAdjustmentIdentifier")));
messages.add(new Message(Message.MessageType.ERROR, "ValidationStatusCode is " + mpa.getAttribute("ValidationStatusCode")));
messages.add(new Message(Message.MessageType.ERROR, "ChargeDefinition is " + mpa.getAttribute("ChargeDefinition")));
messages.add(new Message(Message.MessageType.ERROR, "AdjustmentType is " + mpa.getAttribute("AdjustmentType")));
messages.add(new Message(Message.MessageType.ERROR, "AdjustmentElementBasisCode is " + mpa.getAttribute("AdjustmentElementBasisCode")));
}
}
ValidationException ex = new ValidationException(messages);
throw ex;
履行明細属性の取得
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
if (!"PMC TEST".equals(header.getAttribute("CustomerPONumber"))) return;
List < Message > messages = new ArrayList < Message > ();
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def flinedetails = line.getAttribute("FulfillLineDetails");
while (flinedetails.hasNext()) {
def flinedetail = flinedetails.next();
messages.add(new Message(Message.MessageType.ERROR, "ActualDeliveryDate is " + flinedetail.getAttribute("ActualDeliveryDate")));
messages.add(new Message(Message.MessageType.ERROR, "AvailabilityShipDate is " + flinedetail.getAttribute("AvailabilityShipDate")));
messages.add(new Message(Message.MessageType.ERROR, "BillOfLadingNumber is " + flinedetail.getAttribute("BillOfLadingNumber")));
messages.add(new Message(Message.MessageType.ERROR, "BillingTransactionNumber is " + flinedetail.getAttribute("BillingTransactionNumber")));
messages.add(new Message(Message.MessageType.ERROR, "DeliveryName is " + flinedetail.getAttribute("DeliveryName")));
messages.add(new Message(Message.MessageType.ERROR, "ExceptionFlag is " + flinedetail.getAttribute("ExceptionFlag")));
messages.add(new Message(Message.MessageType.ERROR, "Category is " + flinedetail.getAttribute("Category")));
messages.add(new Message(Message.MessageType.ERROR, "CreatedBy is " + flinedetail.getAttribute("CreatedBy")));
messages.add(new Message(Message.MessageType.ERROR, "CreationDate is " + flinedetail.getAttribute("CreationDate")));
messages.add(new Message(Message.MessageType.ERROR, "CustomerTrxLineId is " + flinedetail.getAttribute("CustomerTrxLineId")));
messages.add(new Message(Message.MessageType.ERROR, "FulfillLineDetailId is " + flinedetail.getAttribute("FulfillLineDetailId")));
messages.add(new Message(Message.MessageType.ERROR, "FulfillLineId is " + flinedetail.getAttribute("FulfillLineId")));
messages.add(new Message(Message.MessageType.ERROR, "LastUpdateDate is " + flinedetail.getAttribute("LastUpdateDate")));
messages.add(new Message(Message.MessageType.ERROR, "LastUpdateLogin is " + flinedetail.getAttribute("LastUpdateLogin")));
messages.add(new Message(Message.MessageType.ERROR, "LastUpdatedBy is " + flinedetail.getAttribute("LastUpdatedBy")));
messages.add(new Message(Message.MessageType.ERROR, "ObjectVersionNumber is " + flinedetail.getAttribute("ObjectVersionNumber")));
messages.add(new Message(Message.MessageType.ERROR, "Quantity is " + flinedetail.getAttribute("Quantity")));
messages.add(new Message(Message.MessageType.ERROR, "RmaReceiptDate is " + flinedetail.getAttribute("RmaReceiptDate")));
messages.add(new Message(Message.MessageType.ERROR, "RmaReceiptLineNumber is " + flinedetail.getAttribute("RmaReceiptLineNumber")));
messages.add(new Message(Message.MessageType.ERROR, "RmaReceiptNumber is " + flinedetail.getAttribute("RmaReceiptNumber")));
messages.add(new Message(Message.MessageType.ERROR, "RmaReceiptTransactionId is " + flinedetail.getAttribute("RmaReceiptTransactionId")));
messages.add(new Message(Message.MessageType.ERROR, "Status is " + flinedetail.getAttribute("Status")));
messages.add(new Message(Message.MessageType.ERROR, "StatusAsofDate is " + flinedetail.getAttribute("StatusAsofDate")));
messages.add(new Message(Message.MessageType.ERROR, "TaskType is " + flinedetail.getAttribute("TaskType")));
messages.add(new Message(Message.MessageType.ERROR, "TrackingNumber is " + flinedetail.getAttribute("TrackingNumber")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceCode is " + flinedetail.getAttribute("TradeComplianceCode")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceExplanation is " + flinedetail.getAttribute("TradeComplianceExplanation")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceResultCode is " + flinedetail.getAttribute("TradeComplianceResultCode")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceTypeCode is " + flinedetail.getAttribute("TradeComplianceTypeCode")));
messages.add(new Message(Message.MessageType.ERROR, "WaybillNumber is " + flinedetail.getAttribute("WaybillNumber")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceName is " + flinedetail.getAttribute("TradeComplianceName")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceCode is " + flinedetail.getAttribute("TradeComplianceCode")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceResultName is " + flinedetail.getAttribute("TradeComplianceResultName")));
messages.add(new Message(Message.MessageType.ERROR, "TradeComplianceTypeName is " + flinedetail.getAttribute("TradeComplianceTypeName")));
}
}
ValidationException ex = new ValidationException(messages);
throw ex;
オーダー明細の更新の防止
オーダー管理で、履行済、取消済、クローズ済、バックオーダー済または分割済のオーダー明細が更新されないようにします。 この拡張では、オーダー管理によって取り消された明細の更新は防止されません。
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
/*
def poNumber = header.getAttribute("OrderNumber");
if (poNumber == null || !poNumber.equals("520956"))
return;
*/
def reference = header.getAttribute("ReferenceHeaderId");
if(reference != null){
def lines = header.getAttribute("Lines");
if (!lines.hasNext()){
throw new ValidationException("We need more time to process the order.");
}
Set<Long> splitFlinesSet = null;
def vo = context.getViewObject("oracle.apps.scm.doo.processOrder.publicModel.partyMerge.view.FulfillLinePVO");
def vc1 = vo.createViewCriteria();
def vcrow1 = vc1.createViewCriteriaRow();
vcrow1.setAttribute("HeaderId", header.getAttribute("HeaderId"));
vcrow1.setAttribute("FulfillLineNumber", " > 1 ");
vcrow1.setAttribute("FulfillmentSplitRefId", " is not null ");
vcrow1.setAttribute("FulfilledQty", " is null ");
rowset1 = vo.findByViewCriteria(vc1, -1);
rowset1.reset();
while (rowset1.hasNext()) {
if (splitFlinesSet == null) {
splitFlinesSet = new TreeSet<Long>();
}
def line1 = rowset1.next();
splitFlinesSet.add(line1.getAttribute("FulfillLineId"));
}
if (splitFlinesSet == null) {
return;
}
while (lines.hasNext()) {
def line = lines.next();
Long fLineId = line.getAttribute("FulfillmentLineIdentifier");
if (!(splitFlinesSet.contains(fLineId))) {
continue;
}
if('Y'.equals(line.getAttribute("ModifiedFlag")) && line.getAttribute("ReferenceFulfillmentLineIdentifier") != null && !(0 == line.getAttribute("OrderedQuantity")) ){
throw new ValidationException("Backordered Split line can't be updated. DisplayLineNumber: " + line.getAttribute("DisplayLineNumber"));
}
}
}
バックオーダー中のオーダー明細の更新の防止
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
String orderType = header.getAttribute("TransactionTypeCode");
def excludeStatuses = ["CANCELED", "CLOSED", "PARTIAL_CLOSE"] as Set;
def forOrderTypes = ["DOMESTIC", "EXPORT", "DOMESTICPPD", "CONSIGN", "EXPORTC", "EXPORTD", "EXPORTF", "HWIPARTNER", "HWIPARTNERC", "HWIPARTNERD", "HWIPARTNERF", "RETURN", "VAS"] as Set;
if (orderType == null) return;
if (!forOrderTypes.contains(orderType)) return;
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
Long lineId = line.getAttribute("LineId");
boolean backUpdateFlag = readFlineStatus(lineId);
if (backUpdateFlag) //if not back order line
{
if (!excludeStatuses.contains(line.getAttribute("StatusCode"))) {
def BillingTransactionTypeId_Current = line.getAttribute("BillingTransactionTypeIdentifier");
def BillingTransactionTypeId_New = line.getAttribute("BillingTransactionTypeIdentifier");
if (orderType.equals("DOMESTIC")) {
BillingTransactionTypeId_New = 300000034980382;
}
if (orderType.equals("EXPORT")) {
BillingTransactionTypeId_New = 300000034980386;
}
if (orderType.equals("DOMESTICPPD")) {
BillingTransactionTypeId_New = 300000039619347;
}
if (orderType.equals("CONSIGN")) {
BillingTransactionTypeId_New = 300000034980380;
}
if (orderType.equals("EXPORTC")) {
BillingTransactionTypeId_New = 300000034988180;
}
if (orderType.equals("EXPORTD")) {
BillingTransactionTypeId_New = 300000034988182;
}
if (orderType.equals("EXPORTF")) {
BillingTransactionTypeId_New = 300000034989759;
}
if (orderType.equals("HWIPARTNER")) {
BillingTransactionTypeId_New = 300000084209774;
}
if (orderType.equals("HWIPARTNERC")) {
BillingTransactionTypeId_New = 300000036925861;
}
if (orderType.equals("HWIPARTNERD")) {
BillingTransactionTypeId_New = 300000036925863;
}
if (orderType.equals("HWIPARTNERF")) {
BillingTransactionTypeId_New = 300000036925870;
}
if (orderType.equals("RETURN")) {
BillingTransactionTypeId_New = 300000034980378;
}
if (orderType.equals("VAS")) {
BillingTransactionTypeId_New = 300000034980370;
}
if (BillingTransactionTypeId_Current != BillingTransactionTypeId_New) {
line.setAttribute("BillingTransactionTypeIdentifier", BillingTransactionTypeId_New);
}
}//Condition skipping lines of particular status codes
}//Back order line check condition
}
/*Method that determines whether the line is shipped or backordered. If the line shipped, the method returns true. If the line is backordered, the method returns false.
*/
def readFlineStatus(Long lineId) {
def lines = header.getAttribute("Lines");
if (!lines.hasNext()) {
throw new ValidationException("In readFlineStatus failing to read lines");
}
while (lines.hasNext()) {
def line = lines.next();
def eachLineId = line.getAttribute("LineId");
def quantity = line.getAttribute("OrderedQuantity");
if (lineId.equals(eachLineId)) {
def flineDetails = line.getAttribute("FulfillLineDetails");
if (!flineDetails.hasNext()) {
continue;
}
while (flineDetails.hasNext()) {
def eachFlineDetails = flineDetails.next();
def status = eachFlineDetails.getAttribute("Status");
if ("BACKORDERED".equals(status) || "SHIPPED".equals(status)) {
return false;
}
}
}
}
return true;
}
ステータスに従ってオーダー明細をスキップし、リクエスト出荷日を設定
履行済、クローズ済または取消済ステータスのオーダー明細をスキップし、オーダー明細のリクエスト出荷日をオーダー・ヘッダーにあるRequestShipDate属性と同じ値に設定します。
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
/* Exclude orchestration processes that you have set up where the statuses are equivalent to SHIPPED or FULFILLED.*/
Set excludeStatusesSet = ["CANCELED", "CLOSED", "SHIPPED", "BACKORDERED", "AWAIT_BILLING", "BILLED" ];
/*
def poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null || !poNumber.equals("CSR_2720"))
return;
*/
def requestedDate = header.getAttribute("RequestShipDate");
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def statusCode = line.getAttribute("StatusCode");
if (statusCode != null && excludeStatusesSet.contains(statusCode)) {
continue;
}
/*
def comments = line.getAttribute("Comments");
if (comments == null)
comments = "TEST:";
line.setAttribute("Comments", comments + header.getAttribute("ChangeVersionNumber"));
*/
/*set the RequestedShipDate attribute on the order line to the value of the requestedDate variable. requestedDate contains the value of the RequestShipDate order header attribute.
line.setAttribute("RequestedShipDate",requestedDate);
line.setAttribute("ScheduleShipDate",requestedDate);
}
クローズ済オーダー明細のModifiedFlag属性の更新
if(!header.getAttribute("OrderNumber").equals("100002054")) {
return;
}
def lines = header.getAttribute("Lines");
while(lines.hasNext()) {
def line = lines.next();
if(line.getAttribute("ModifiedFlag").equals("Y") && line.getAttribute("StatusCode").equals("CLOSED")) {
line.setAttribute("ModifiedFlag", "N");
}
}
オーダー明細の日付の操作
延長に日付を含める方法を示す例を次に示します。 この拡張では、保存時拡張ポイントを使用します。
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import java.sql.Date;
import java.sql.Timestamp;
if( !"SNDATE".equals( header.getAttribute("CustomerPONumber" ) ) ) return;
def pattern = "MM/dd/YYYY hh:mm:ss a";
def date = header.getAttribute("TransactionOn");
/* You can use these formats:
Letter Date or Time Type Example
G Era designator Text AD
y Year Year 1996; 96
Y year Year 2009; 09
M Month in year Month Jul
MMMM Month in year Month July
w Week in year Number 27
W Week in month Number 2
D Day in year Number 189
d Day in month Number 10
E Day name in week Text Tue
EEEE Day name in week Text Tuesday
u Day number of week Number !1 (1 = Monday, ..., 7 = Sunday)
a Am/pm marker Text PM
H Hour in day (0-23) Number 0
k Hour in day (1-24) Number 24
K Hour in am/pm (0-11) Number 0
h Hour in am/pm (1-12) Number 12
m Minute in hour Number 30
s Second in minute Number 55
S Millisecond Number 978
z Time zone Text PST
zzzz Time zone Text Pacific Standard Time;
Z Time zone RFC 822 time zone -0800
X Time zone ISO 8601 time zone -08; -0800; -08:00
*/
debug( "Order Date "+ date.format(pattern));
def headerRSD = header.getAttribute("RequestShipDate");
def headerRSD_Time = headerRSD.getTime();
def vPSD = new java.sql.Date(headerRSD_Time - getTimeInMillis(2));
def vPAD = new java.sql.Date(headerRSD_Time + getTimeInMillis(7));
def lines = header.getAttribute("Lines");
while( lines.hasNext() ) {
def line = lines.next();
line.setAttribute("PromiseShipDate", vPSD);
line.setAttribute("PromiseArrivalDate", vPAD);
}
public static long getTimeInMillis(long days) {
return days * 24 * 60 * 60 * 1000;
}
void debug(String msg) {
String text = header.getAttribute("ShippingInstructions");
header.setAttribute("ShippingInstructions", text + ". " + msg);
}
オーダー明細の契約開始日からシステム日付を削除してください
販売オーダーをコピーすると、オーダー管理によって契約開始日属性がシステム日付に設定されます。 この拡張を使用して、契約開始日からシステム日付を削除します。
def lines = header.getAttribute("Lines");
while( lines.hasNext() ) {
def line = lines.next();
def VARTotalContractAmount = line.getAttribute("TotalContractAmount");
def refFlineId=line.getAttribute("ReferenceFulfillmentLineIdentifier");
if( VARTotalContractAmount == null && refFlineId==null) {
line.setAttribute("ContractStartDate1",null);
}
}
オーダー明細の単位のデフォルト値の設定
import oracle.apps.scm.doo.common.extensions.ValidationException;
def poNumber = header.getAttribute("CustomerPONumber");;
def reference = header.getAttribute("ReferenceHeaderId");
def headerId = header.getAttribute("HeaderId");
def sourceOrderId = header.getAttribute("SourceTransactionIdentifier");
//If you use the Order Management work area to create the sales order, then don't run this extension.
if (headerId == sourceOrderId)
return;
//If its an order revision, then don't run this extension. Remove this condition to run the extension for revisions.
if (reference != null)
return;
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def inventoryItemId = line.getAttribute("ProductIdentifier");
def orgId = line.getAttribute("InventoryOrganizationIdentifier");
def parentFLineId = line.getAttribute("RootParentLineReference");
if (parentFLineId != null) {
def item = getItem(inventoryItemId, orgId);
def uomCode = item.getAttribute("PrimaryUomCode");
if (uomCode != null) {
line.setAttribute("OrderedUOMCode", uomCode);
}
}
}
Object getItem(Long itemId, Long orgId) {
def itemPVO = context.getViewObject("oracle.apps.scm.productModel.items.publicView.ItemPVO");
def vc = itemPVO.createViewCriteria();
def vcrow = vc.createViewCriteriaRow();
vcrow.setAttribute("InventoryItemId", itemId);
vcrow.setAttribute("OrganizationId", orgId);
def rowset = itemPVO.findByViewCriteria(vc, -1);
def item = rowset.first();
return item;
}
単位のレートの取得
import oracle.apps.scm.doo.common.extensions.ValidationException;
def serviceInvoker = context.getServiceInvoker();
String payLoad = "<ns1:invUomConvert xmlns:ns1=\"http://xmlns.oracle.com/apps/scm/inventory/uom/uomPublicService/types/\">"+
"<ns1:pInventoryItemId>141</ns1:pInventoryItemId>"+
"<ns1:pFromQuantity>1</ns1:pFromQuantity>"+
"<ns1:pFromUomCode>Ea</ns1:pFromUomCode>"+
"<ns1:pToUomCode>Ea</ns1:pToUomCode>"+
"<ns1:pFromUnitOfMeasure/>"+
"<ns1:pToUnitOfMeasure/>"+
"</ns1:invUomConvert>";
def responseBody = serviceInvoker.invokeSoapService("UOM_RATE", payLoad).getSoapBody().getTextContent();
//throw new ValidationException("response final_Uom_Rate : "+responseBody);
一部出荷したオーダー明細の識別
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
String customerPONumber = header.getAttribute("CustomerPONumber");
if (customerPONumber != "SNPART") return;
List<Message> messages = new ArrayList<Message>();
// Get all of the sales order's order lines.
def Lines = header.getAttribute("Lines");
while (Lines.hasNext()) {
def line = Lines.next();
def flineID = line.getAttribute("FulfillmentLineIdentifier");
debug("Fulfill Line Id " + flineID.toString());
// Find out whether the FulfillmentSplitReferenceId attribute contains a value.
def splitFrom = line.getAttribute("FulfillmentSplitReferenceId")
if (splitFrom != null) {
// Find out whether the order line is split because of a partial shipment.
if (line.getAttribute("ShippedQuantity")== null) {
//This is the open order line.
debug("Line " + flineID + " is split from " + splitFrom);
//Add your logic here.
}
}
}
void debug(String msg) {
//messages.add(new Message(Message.MessageType.WARNING,msg));
header.setAttribute("PackingInstructions", header.getAttribute("PackingInstructions") + "" + msg);
}
構成モデルのオーダー明細間での値のカスケード
この例では、構成モデルの最上位の親からその親のすべての子明細に属性値をカスケードします。
import oracle.apps.scm.doo.common.extensions.ValidationException;
def poNumber = header.getAttribute("CustomerPONumber");;
//Condition that specifies to call the extension.
if(poNumber != null && poNumber.equals("CASCADE_CONTRACT_SDATE")){
def lines=header.getAttribute("Lines");
long currentTime = new Date().getTime();
def date = new java.sql.Date(currentTime);
//Iteration all lines of the order
while( lines.hasNext() ) {
def line=lines.next();
def inventoryItemId = line.getAttribute("ProductIdentifier");
def orgId = line.getAttribute("InventoryOrganizationIdentifier");
def parentLineReference=line.getAttribute("ParentLineReference");
def rootParentLineReference=line.getAttribute("RootParentLineReference");
def item = getItem(inventoryItemId, orgId); // Getting item details for the line
def itemTypeCode=item.getAttribute("BomItemType");
def pickComponentsFlag=item.getAttribute("PickComponentsFlag");
def parentstartdate=line.getAttribute("ContractStartDate1");
def flineId=line.getAttribute("FulfillmentLineIdentifier");
//Passing root line for PTO ,Hybrid and ATO Items
if(1==itemTypeCode && flineId==rootParentLineReference && rootParentLineReference== parentLineReference && parentstartdate!=null){
updateChildLines(line);
}
//Passing root line For KIT Items
else if(4==itemTypeCode && "Y".equals(pickComponentsFlag) && parentstartdate!=null){
updateChildLines(line);
}
}
}
Object getItem(Long itemId, Long orgId) {
def itemPVO = context.getViewObject("oracle.apps.scm.productModel.items.publicView.ItemPVO");
def vc = itemPVO.createViewCriteria();
def vcrow = vc.createViewCriteriaRow();
vcrow.setAttribute("InventoryItemId", itemId);
vcrow.setAttribute("OrganizationId", orgId);
def rowset = itemPVO.findByViewCriteria(vc, -1);
def item = rowset.first();
return item;
}
//Iterate through all lines. Set the child lines's contract start date and end date to the parent's contract start date and end date.
void updateChildLines(def rootfline){
def lines=header.getAttribute("Lines");
def flineId=rootfline.getAttribute("FulfillmentLineIdentifier");
def parentstartdate=rootfline.getAttribute("ContractStartDate1");
def parentenddate=rootfline.getAttribute("ContractEndDate1");
while( lines.hasNext() ) {
def line=lines.next();
def rootFlineId=line.getAttribute("RootParentLineReference");
if(flineId==rootFlineId){
line.setAttribute("ContractStartDate1",parentstartdate);
line.setAttribute("ContractEndDate1",parentenddate);
}
}
}
履行組織および出荷指示の設定
品目が出荷可能な場合は、オーダー明細の履行組織および明細の出荷指示を、品目が参照するデフォルトの出荷組織に設定します。
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
def poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("DefaultShippingOrg")) return;
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def inventoryItemId = line.getAttribute("ProductIdentifier");
def orgId = line.getAttribute("InventoryOrganizationIdentifier");
def item = getItem(inventoryItemId, orgId);
String shippable = item.getAttribute("ShippableItemFlag");
if ("Y".equals(shippable)) {
Long defaultOrgId = item.getAttribute("DefaultShippingOrg");
//msg = new Message(Message.MessageType.WARNING, "default: " + inventoryItemId + " - " + orgId + " - " + shippable+ " - " + defaultOrgId);
//throw new ValidationException(msg);
line.setAttribute("FulfillmentOrganizationIdentifier", defaultOrgId);
line.setAttribute("FulfillmentOrganizationIdentifier", 1234);
line.setAttribute("ShippingInstructions", "Ship From Org :" + 999);
}
}
Object getItem(Long itemId, Long orgId) {
def itemPVO = context.getViewObject("oracle.apps.scm.productModel.items.publicView.ItemPVO");
def vc = itemPVO.createViewCriteria();
def vcrow = vc.createViewCriteriaRow();
vcrow.setAttribute("InventoryItemId", itemId);
vcrow.setAttribute("OrganizationId", orgId);
vc.add(vcrow);
def rowset = itemPVO.findByViewCriteriaWithBindVars(vc, -1, new String[0], new String[0]);
def item = rowset.first();
return item;
}
危険品目の梱包指示の追加
品目が危険である場合は、オーダー明細に梱包指示を設定します。
def poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("HazardousMaterial")) return;
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def inventoryItemId = line.getAttribute("ProductIdentifier");
def orgId = line.getAttribute("InventoryOrganizationIdentifier");
def item = getItem(inventoryItemId, orgId);
String hazardous = item.getAttribute("HazardousMaterialFlag");
if ("Y".equals(hazardous)) {
//get row for fulfill line context PackShipInstruction
line.setAttribute("PackingInstructions", "Hazardous Handling Required.");
}
}
Object getItem(Long itemId, Long orgId) {
def itemPVO = context.getViewObject("oracle.apps.scm.productModel.items.publicView.ItemPVO");
def vc = itemPVO.createViewCriteria();
def vcrow = vc.createViewCriteriaRow();
vcrow.setAttribute("InventoryItemId", itemId);
vcrow.setAttribute("OrganizationId", orgId);
vc.add(vcrow);
def rowset = itemPVO.findByViewCriteriaWithBindVars(vc, -1, new String[0], new String[0]);
def item = rowset.first();
return item;
}