You can use the Fire Event action in an action chain to trigger a custom event, which in turn starts a different action chain to do things like display a notification, transform data, and so on. You could also trigger a custom event by using an event helper's fireCustomEvent()
method (see Module Function Event Helper) in a module function (JavaScript function):
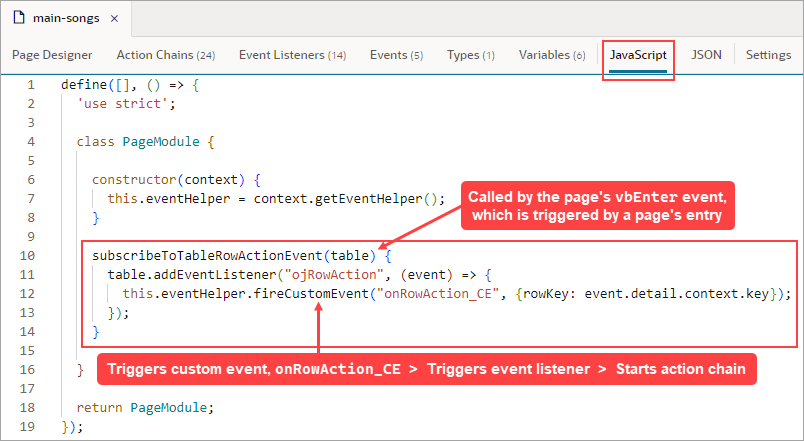
Description of the illustration jsac-add-listener-component.png
Each custom event has a Behavior property, which sets whether an action chain runs serially or in parallel. The default behavior is "Notify", which runs the action chain in parallel. For more about this property, see Choose How Custom Events Call Event Listeners.
After creating a custom event, you create an event listener for it to start one or more action chains.
In this example, we'll use a module function to subscribe to a table's ojRowAction
event to trigger a custom event that starts an action chain. The action chain then saves the selected row's data to a page variable. To begin:
- Create a page variable of type Object to hold the selected row's data:
For this part, you create a JavaScript function (module function) to subscribe to the table's ojRowAction
event, which is triggered when a user clicks a table's row. You'll use this event to trigger your custom event, which will start the action chain that saves the row's data to the rowData
page variable.
- To create the function, select the JavaScript tab. Use the context's
eventHelper
object's fireCustomEvent()
method to trigger your custom event and to pass it the required payload. The event
parameter contains the row's data (event.detail.context.data
).
Here's the example code:
constructor(context) {
this.eventHelper = context.getEventHelper();
}
subscribeToTableRowActionEvent(table) {
table.addEventListener("ojRowAction", (event) => {
this.eventHelper.fireCustomEvent("onRowAction_CustomEvent", {rowData: event.detail.context.data});
});
};
Next, you need to create the event listener for the page's vbEnter
event, which is triggered when the page starts. You'll use this event listener to start an action chain that calls the function to subscribe to the table's ojRowAction
event.
- To create the event listener for the page's
vbEnter
event, select the Event Listeners tab, and click the + Event Listener button to create it.
- For the wizard's Select Event step, in the Lifecycle Events section, select
vbEnter
and click Next.
- For the Select Action Chain step, click the Page Action Chains section's Add icon to create an action chain for the listener to initiate.
If the custom event for this listener has input parameters, which this one doesn't, the action chain would be created with an event
input parameter that contains the custom event's input parameters.
You are taken to the Action Chain editor, where you can create the action chain to call the function that subscribes to the table's ojRowAction
event.
- Add the Call Function action to the canvas. Set its
Function Name
property to the JavaScript function, and pass the table to the function using the table
parameter:
For this next part, you'll create the custom event that will be triggered by the table's ojRowAction
event. You'll also create the action chain that assigns the row's data to the rowData
page variable.
- On the page's Events tab, click the + Custom Event button to create a custom event. In the Properties pane, click the Payload property's Add Parameter link and define an input parameter of type Object. This input parameter will be used to pass the row's data to the action chain that assigns the data to the
rowData
page variable.
- For the custom event's Behavior property, set whether the action chain runs serially or in parallel. The default,
notify
, is in parallel. For details about each option, see Choose How Custom Events Call Event Listeners.
We now need to create an event listener for the event to specify which action chains to start when the event occurs (more than one action chain can be started by an event listener).
- On the page's Event Listeners tab, click the + Event Listener button to create a listener.
- For the wizard's Select Event step, scroll down to the Page Events section and select the custom event that you created. Click Next.
- For the Select Action Chain step, click the Add icon for the Page Action Chains section to create an action chain for the listener to initiate.
When a listener’s action chain is created here, if the listener's custom event has input parameters, the action chain is created with an event
input parameter. This event
object contains the custom event's input parameters (example: event.param1
, event.param2...
), and the event
object is automatically passed to the new action chain.
- In the Action Chain editor, note that the action chain has the
event
input parameter, which contains the custom event's input parameter. Add the Assign Variable action, and set its Variable
property to the page variable that will contain the row's data. Lastly, set it's Value
property to the relevant value in the event
object that was passed to the action chain: