Ensure value of multiple commissions fields equals 100%
This script checks to ensure that sales commission amounts equal 100% (1.00) before allowing the project to be saved. It can be modified to support any number of sales rep commissions fields.
-
Enrich records with additional sales management information.
-
Easily reusable/extendible with minimal effort.
-
Might solve this case using allocation grid custom field, but this solution allows user pick lists and retains a more detailed audit trail.
A new custom Commission section has been added to the project form. A user script is triggered as the project saves to validate the commission values entered.
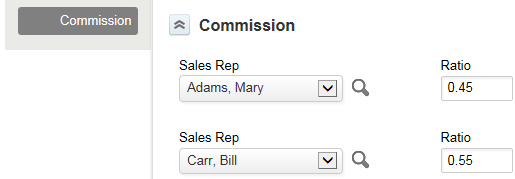
Follow the steps below or download the solutions file, see Creating Solutions for details.
Setup
-
Create a new Project form script deployment.
-
Enter a Filename and click SAVE. The extension ‘.js’ is automatically appended if not supplied.
-
Click on the script link to launch the Scripting Studio.
-
(1) Copy the Program Listing below into the editor, (2) set the Before save event, and set checkCommish as the Entrance Function.
-
Set up the required number of custom field pairs for Project. The first in each pair is a Pick List with a List source of User. The second in each pair is a Ratio. You can set the Divider text for the first custom field to Commission to place the custom fields in their own section.
-
Use the Form schema to identify the correct param names for the custom fields and change the array at the top of the script accordingly.
Tip:If the new custom fields are not listed in the Form schema, navigate to Projects, open a project form (this with will refresh the custom field list), and then open the Scripting Studio again.
Program Listing
// ADD YOUR REP AND RATIO CUSTOM FORM FIELD NAMES TO THE ARRAY BELOW
var repCompFlds = [
'prj_sales_rep_1__c', 'prj_sales_rep_ratio_1__c', // Use Form schema to find param names
'prj_sales_rep_2__c', 'prj_sales_rep_ratio_2__c'
];
function checkCommish(type) {
try {
var _len = repCompFlds.length,
_i = 1, // Skip over sales rep name
_j = 0,
totalComp = 0;
while (_i < _len) {
totalComp += parseFloat(NSOA.form.getValue(repCompFlds[_i]));
_i += 2; // Skip over sales rep name
}
var totalCompRound = round(totalComp, 2),
totalCompPercent = totalCompRound * 100;
if (totalCompRound !== 0 && totalCompRound != 1) {
NSOA.form.error(
'',
'The total sales commission ' + totalCompRound +
' (' + round(totalCompPercent, 2) + '%) must equal 100%!'
);
for (_j; _j < _len; _j++) {
NSOA.form.error(repCompFlds[_j], 'Please check and re-save.');
}
}
} catch (e) {
NSOA.meta.log('fatal', "Error running the script: " + e);
}
}
function round(number, decimals) {
return (Math.round(number * Math.pow(10, 2)) / Math.pow(10, 2)).toFixed(decimals);
}