Optionally create a new Customer PO when editing a project
This example allows a customer to streamline their business processes by quickly creating customer POs as a part of saving a project.
-
Saves ~7 mouse clicks
-
Can be used on a per-project basis (not required)
-
Can be used multiple times if many POs are required on one project
A new custom Quick Customer PO section has been added to the project form. A user script is triggered as the project saves to create the specified customer PO.
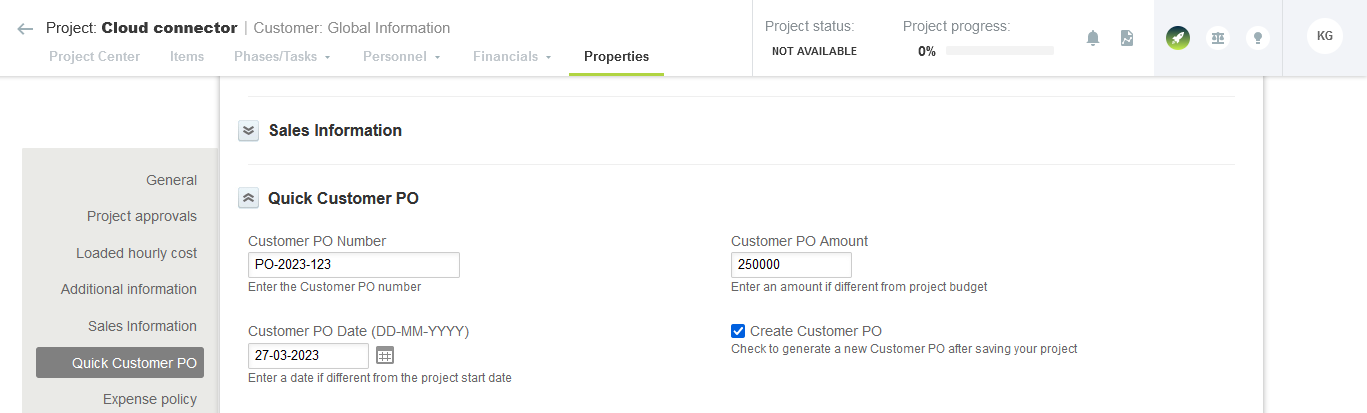
The Create Customer PO check box signals that a new customer PO record is to be created and the customer PO fields cleared allowing the user to quickly create additional customer POs. When the project is saved the specified Customer PO is then created.
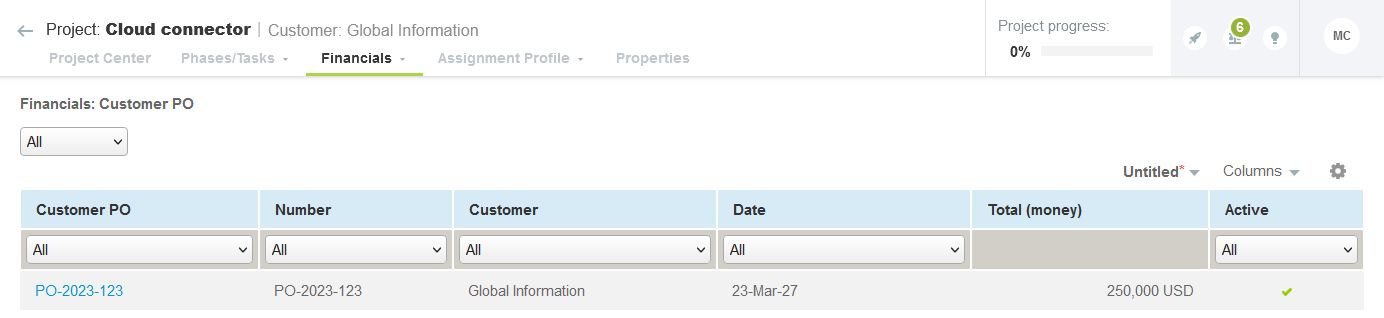
Follow the steps below or download the solutions file, see Creating Solutions for details.
Setup
-
Create a new Project form script deployment.
-
Enter a Filename and click SAVE. The extension ‘.js’ is automatically appended if not supplied.
-
Click on the script link to launch the Scripting Studio.
-
(1) Copy the Program Listing below into the editor, (2) set the After save event, and set createCustomerPO as the Entrance Function.
-
Set up the following custom fields for Project. You can set the Divider text for the first custom field to Quick Customer PO to place the custom fields in their own section.
Add the following hints:
-
prj_custpo_num — Hint: Enter the customer's PO number.
-
prj_custpo_amt — Hint: Enter an amount if different from the project budget.
-
prj_custpo_date — Hint: Enter a date if different from the project start date.
-
prj_create_po — Hint: Check to create a new Customer PO after saving your project.
-
Program Listing
function createCustomerPO(type) {
try {
var FLD_CUSTPO_NUM = 'prj_custpo_num__c',
FLD_CUSTPO_AMT = 'prj_custpo_amt__c',
FLD_CUSTPO_DATE = 'prj_custpo_date__c',
FLD_CREATE_PO = 'prj_create_po__c';
// get updated project record fields
var updPrj = NSOA.form.getNewRecord();
// if the "Create PO" checkbox is checked and a PO number is entered, create a PO
if (updPrj[FLD_CREATE_PO] == '1' && updPrj[FLD_CUSTPO_NUM]) {
var recCustPO = new NSOA.record.oaCustomerpo();
recCustPO.number = updPrj[FLD_CUSTPO_NUM];
recCustPO.name = updPrj[FLD_CUSTPO_NUM] + ' ' + updPrj.name;
// use the PO date if available, otherwise use project start date
if (updPrj[FLD_CUSTPO_DATE] != '0000-00-00') {
recCustPO.date = updPrj[FLD_CUSTPO_DATE];
} else {
recCustPO.date = updPrj.start_date;
}
// currency custom fields return ISO-#.##; remove the ISO code and dash
var cleanAmt = updPrj[FLD_CUSTPO_AMT].replace(/-\w{3}/, '');
// use the PO amt if available, otherwise use project budget
if (cleanAmt && cleanAmt != '0.00') {
recCustPO.total = cleanAmt;
} else if (updPrj.budget && updPrj.budget > 0.00) {
recCustPO.total = updPrj.budget;
}
recCustPO.currency = updPrj.currency;
recCustPO.customerid = updPrj.customerid;
recCustPO.active = 1;
// disable the current user's filter set while script runs
NSOA.wsapi.disableFilterSet(true);
// add the new customer po to the project
var custPOResults = NSOA.wsapi.add(
[recCustPO]
);
if (!custPOResults || !custPOResults[0]) {
NSOA.meta.log('error', 'Unexpected error! Customer PO was not created.');
} else if (custPOResults[0].errors) {
custPOResults[0].errors.forEach(function(err) {
NSOA.meta.log('error', 'Error: ' + err.code + ' - ' + err.comment);
});
} else {
// new customer po to project link object
var recCustPOtoProj = new NSOA.record.oaCustomerpo_to_project();
recCustPOtoProj.customerpoid = custPOResults[0].id;
recCustPOtoProj.customerid = updPrj.customerid;
recCustPOtoProj.projectid = updPrj.id;
recCustPOtoProj.active = '1';
// disable the current user's filter set while script runs
NSOA.wsapi.disableFilterSet(true);
// add the new customer po to the project
var custPOtoProjResults = NSOA.wsapi.add(
[recCustPOtoProj]
);
if (!custPOtoProjResults || !custPOtoProjResults[0]) {
NSOA.meta.log('error',
'Unexpected error! Customer PO was not linked to the project.');
} else if (custPOtoProjResults[0].errors) {
custPOtoProjResults[0].errors.forEach(function(err) {
NSOA.meta.log('error', 'Error: ' + err.code + ' - ' + err.comment);
});
}
}
// create project object to hold update information and clear quick po
var recProj = new NSOA.record.oaProject(updPrj.id);
recProj[FLD_CUSTPO_NUM] = '';
recProj[FLD_CREATE_PO] = '';
recProj[FLD_CUSTPO_AMT] = '';
recProj[FLD_CUSTPO_DATE] = '';
// disable the current user's filter set while script runs
NSOA.wsapi.disableFilterSet(true);
// enable custom field editing
var update_custom = {
name: 'update_custom',
value: '1'
};
// update the project to clear quick customer po create information
var projResults = NSOA.wsapi.modify(
[update_custom], [recProj]
);
if (!projResults || !projResults[0]) {
NSOA.meta.log('error', 'Unexpected error! Project was not updated.');
} else if (projResults[0].errors) {
projResults[0].errors.forEach(function(err) {
NSOA.meta.log('error', 'Error: ' + err.code + ' - ' + err.comment);
});
}
} else {
NSOA.meta.log('debug', 'Customer po creation was skipped.');
}
} catch (e) {
NSOA.meta.log('error', 'Uncaught error: ' + e);
}
}