Quick Start
You can make many types of HTTP requests using Oracle Applications Cloud REST APIs. You can easily make requests to view, create, update, or delete records. As an example, let's look at how to send a simple REST HTTP request to find out the structure of an Announcement object.
Step 1: Consider Before You Start
Review the basics. If you're new to REST APIs, make sure you understand the basics of REST and JSON, and scan our list of important terms.
Review opt-in requirements. Some resources or their attributes may be associated with features that require opt-in before you can use them. You must make sure that you enable opt-in features before you start.
Choose a client. REST APIs connect software programs over the HTTP protocol. You need a software client to send the HTTP requests. In our examples, we use cURL. But, cURL isn't the only tool you can use. To help you choose one, see Work with your REST API Client.
Step 2: Get Your Oracle Applications Cloud Account Info
To make a REST HTTP request, you need to gather a few bits of information:
- REST Server URL. Typically, the URL of your Oracle Cloud service. For example,
https://servername.fa.us2.oraclecloud.com
. - User name and password. An Oracle Cloud service user with permissions to access the resources you're using.
You can find the REST Server URL, user name, and password in the welcome email sent to your Oracle Cloud service administrator.
Step 3: Configure Your Client
With the information gathered so far, you're ready to configure your client to send a REST HTTP request.
-
Construct the request URL. The URL consists of the server name and the resource path:
https://<server>/<resource-path>
The <server> is the REST Server URL from Step 2, as in:
https://servername.fa.us2.oraclecloud.com
The <resource-path> is the relative path or endpoint to the REST resource you're working with. You can pick any endpoint in All REST Endpoints. However, some resources or their attributes may be associated with features that require opt-in before you can use them. In our example, we're interested in the Announcements resource or its attributes, which requires us to opt in the feature associated with it.
/fscmRestApi/resources/11.13.18.05/announcements
Combine the REST Server URL and, in this example, the Announcements REST resource path and your request URL is complete. For more information, see URL Paths.
https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements
-
Provide your account information. Include your user name and password (from Step 2) in the client. For example, if you are using cURL, you can specify your account information using the
-u cURL
command as follows:-u <username:password>
In a REST API client such as Postman, you enter the user name and password on the Authorization tab. This screenshot shows how to specify this information in Postman:
You must also select the appropriate authorization type, such as basic, for your server. See Step 4 for details.
-
Set the media type. Media type defines the structure of the HTTP payloads exchanged between the server and the client. For example, if you're using cURL, you can specify a resource item media type using the header -H command as follows:
-H 'Content-Type: application/vnd.oracle.adf.resourceitem+json'
For any request that has a request body (like POST or PATCH), you must include the Content-Type request header. For more information on media types, see Supported Media Types.
When you're done, the complete cURL command should look like this:
curl -u <username:password> \
-X GET https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe \
-H 'Content-Type: application/vnd.oracle.adf.resourceitem+json' | json_pp
If you're not familiar with any of the syntax used in the example, check out Work with your REST API Client.
If you're an advanced REST user, but are reading our Quick Start anyway, you might want to set the REST Framework or configure Cross-Origin Resource Sharing (CORS) now. Otherwise, you're ready to move on to Step 4.
Step 4: Authenticate and Authorize
Now that you've configured the client with a complete request URL, it's time to authenticate and authorize yourself. Authentication proves that your credentials are genuine, and authorization allows you to apply your access privileges.
Authentication
To make sure data access over a network is secure, Oracle Applications Cloud REST APIs use a global Oracle Web Services Manager (OWSM) security policy called Multi Token Over SSL RESTful Service Policy (oracle/multi_token_over_ssl_rest_service_policy
). This security policy enforces the following authentication standards:
- Basic authentication over SSL (Secure Socket Layer), which extracts the user name and password credentials from the HTTP header.
- SAML 2.0 bearer token in the HTTP header over SSL , which extracts a SAML 2.0 bearer assertion (XML security token).
- JWT in the HTTP header over SSL, which extracts the user name from the JWT.
You must select one of the standards. Let's look at our example using Basic authentication over SSL. To authenticate, you must submit the user name and password for your Oracle Cloud account. Typically, the user name and password are encoded in Base64 format, as in:
curl \
-X GET https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe \
-H 'Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=' \
-H 'Content-Type: application/vnd.oracle.adf.resourceitem+json'
Alternatively, you can use the -u cURL
option to pass the user name and password for your Oracle Cloud account, as in this example:
curl -u username:password \
-X GET https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe \
-H 'Content-Type: application/vnd.oracle.adf.resourceitem+json'
Your authorization and authentication information gets passed in the Authorization key of the request header. When passing tokens (SAML or JWT) in Postman, the Authorization key must include Bearer, followed by the token, as shown in this screenshot:
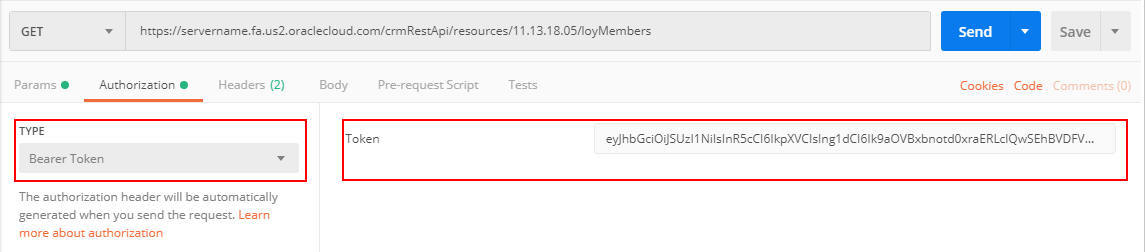
Authorization
Authorization enforces access privileges by service role. Access to an object determines access to a REST resource. So, make sure that your user has the proper role.
For additional details, including a list of specific roles for accessing a REST resource, see Security Reference for Common Features.
Step 5: Send an HTTP Request
You're almost done. Now that your authentication and authorization are set, you're ready to send a test HTTP request. Continuing with our example, you want to get all the information about the structure of the Announcements object in REST. You can do this using the describe
action in cURL:
curl -u username:password \
-X GET https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe \
-H 'Content-Type: application/vnd.oracle.adf.resourceitem+json'
This is how the request looks in Postman:
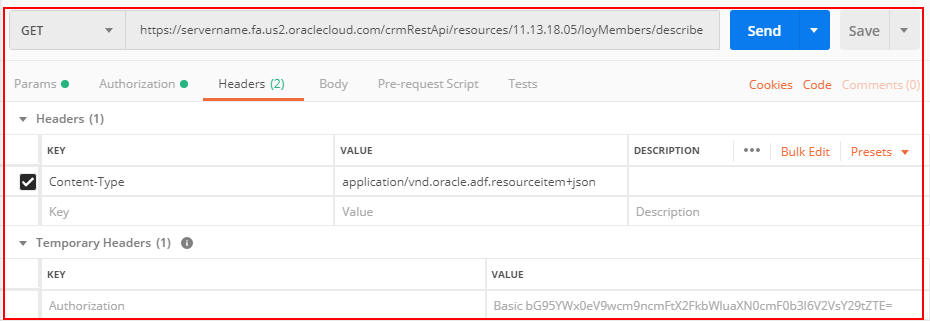
If your request for information about the Announcements object is successful, you receive a response with a body similar to the following abbreviated example. If your request fails, and you're using cURL, review the response comments, adjust your request, and then try again. If you're using other REST clients, review the failure Status Codes, and then try again.
{
"Resources": {
"announcements": {
"discrColumnType": false,
"attributes": [
{
"name": "AnnouncementId",
"type": "string",
"updatable": true,
"mandatory": true,
"queryable": true,
"allowChanges": "inCreate",
"precision": 18,
"hasDefaultValueExpression": true
},
...
],
"collection": {
"rangeSize": 25,
"finders": [
{
"name": "PrimaryKey",
"attributes": [
{
"name": "AnnouncementId",
"type": "string",
"updatable": true,
"mandatory": true,
"queryable": true,
"allowChanges": "inCreate",
"precision": 18,
"hasDefaultValueExpression": true
}
]
}
],
"links": [
{
"rel": "self",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements",
"name": "self",
"kind": "collection"
}
],
"actions": [
{
"name": "get",
"method": "GET",
"responseType": [
"application/vnd.oracle.adf.resourcecollection+json",
"application/json"
]
},
{
"name": "create",
"method": "POST",
"requestType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
],
"responseType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
]
},
{
"name": "upsert",
"header": "Upsert-Mode=true",
"method": "POST",
"requestType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
],
"responseType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
]
}
]
},
"item": {
"links": [
{
"rel": "self",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/{id}",
"name": "self",
"kind": "item"
},
{
"rel": "canonical",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/{id}",
"name": "canonical",
"kind": "item"
},
{
"rel": "enclosure",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/enclosure/DescriptionClob",
"name": "DescriptionClob",
"kind": "other"
}
],
"actions": [
{
"name": "get",
"method": "GET",
"responseType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
]
},
{
"name": "update",
"method": "PATCH",
"requestType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
],
"responseType": [
"application/vnd.oracle.adf.resourceitem+json",
"application/json"
]
},
{
"name": "delete",
"method": "DELETE"
}
]
},
"links": [
{
"rel": "self",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe",
"name": "self",
"kind": "describe"
},
{
"rel": "canonical",
"href": "https://servername.fa.us2.oraclecloud.com/fscmRestApi/resources/11.13.18.05/announcements/describe",
"name": "canonical",
"kind": "describe"
}
]
}
}
}
In a REST API client such as Postman, the results are formatted and displayed in the Response section. For example, Postman lets you view the output in multiple formats. This screenshot shows the response in JSON.
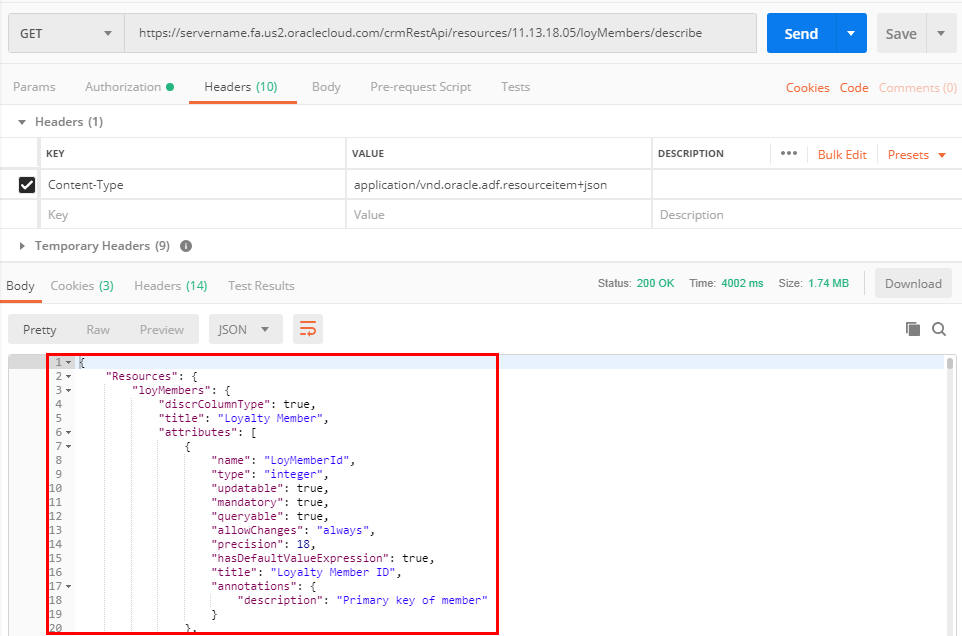
Note:
When working with a resource that uses both an auto-generated unique identifier and a user-defined identifier to expose an entity relationship (foreign key), you must use only one of these parameters in the request payload in any of the CRUD actions. If you include both, the behavior isn't specified and may change without notice. To understand this, let's assume that the calendarEvent resource includes a foreign key to an announcement resource. Suppose, the announcement resource has a unique identifierAnnouncementId (300100111705686)
and an alternative user-defined identifier called
AnnouncementNumber (ANN_332708)
. When you create a new calendarEvent, or update a calendarEvent's reference to an announcement, you must not include both the
AnnouncementId
and the
AnnouncementNumber
parameters in the request payload.
Congratulations! Now you're ready to do more with your REST APIs.
- Explore the Learn More section to better manage collections.
- Join the Oracle Developer Community, where you can share tips and advice with others.