Calculate Dates in Business Rules
You can calculate a date in a business rule.
-
A business rule doesn't come predefined with functions that do date arithmetic, such as
Scheduled Ship Date minus 1
. -
To avoid data type and object hierarchy problems, you can't use a business rule to do date arithmetic with a fact. However, you can create a rule that does date arithmetic in an orchestration process.
-
A date attribute use the time stamp data type.
Here's an example that uses an If statement to set
up fline
as the alias for the fulfillment
line. It compares the time of the scheduled ship date to the current
date, and uses milliseconds in the comparison conversion.
If fline.scheduleShipDate.time>=CurrentDate.date.timeInMillis
In another example, here's some code that calculates the scheduled arrival date to happen three days after the current date.
If fline.scheduleArivalDate.time>=
CurrentDate.date.timeInMillis+3*24*60*60*1000
where
-
3 is the number of days.
-
24 is the number of hours in one day.
-
60 is the number of minutes in one hour.
-
60 is the number of seconds in one hour.
-
1000 is the number of milliseconds in one second.
Here's a dialog that sets up a business rule for the line selection criteria in an orchestration process.
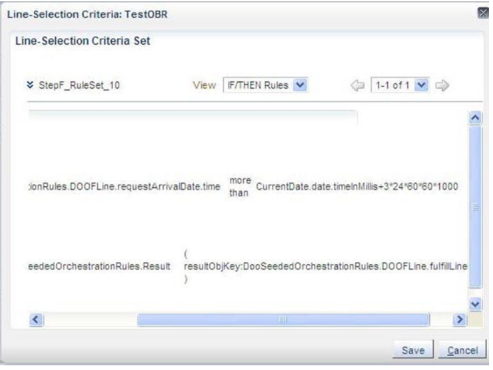
Note
Rule Part |
Code |
---|---|
If the requested arrival date happens earlier than three days after the current date, then skip this step. |
|
Action |
Assert New
|
If you use this kind of calculation in the THEN clause, then make sure your rule doesn't modify or overwrite the original value. You might need to assign a temporary attribute to hold the calculation before your rule populates the result object.
Create Temporary Attributes
Use a Timestamp object to create a temporary attribute, such as to provide an offset to a data attribute. For example, three days before the scheduled ship date. The Assign New action creates a new instance of the attribute, provides an alias, and sets the initial value.
Here's an example of a lead time expression in a
business rule on an orchestration process. fline
represents the fulfillment line object and DateTime is the temporary
attribute.
Action |
Code |
---|---|
Assign New |
|
Modify |
|
Assert New |
|
Use Date Functions in Advanced Mode
Function |
Example |
---|---|
|
|
|
|
|
|
|
|
Note
-
Use these functions for each attribute that's a Timestamp data type, including a temporary attribute that you declare with the Timestamp object.
-
You can manually enter the function after you select the attribute. You can also open the Expression Builder, click the Functions tab, then open the folder next to the date attribute.
-
The format for each function, except compareTo, is true or false, and this format doesn't require quotation marks.
-
Here's the format that compareTo uses.
-
-1
. The object to the left of compareTo happens before the object to the right of compareTo. -
0
. The object to the left of compareTo happens at the same time as the object to the right of compareTo. -
1. The object to the left of compareTo happens after the object to the right of compareTo.
-