Guidelines for Using Extensions to Manage Messages
Use an extension to define a message.
Set Up Your Message
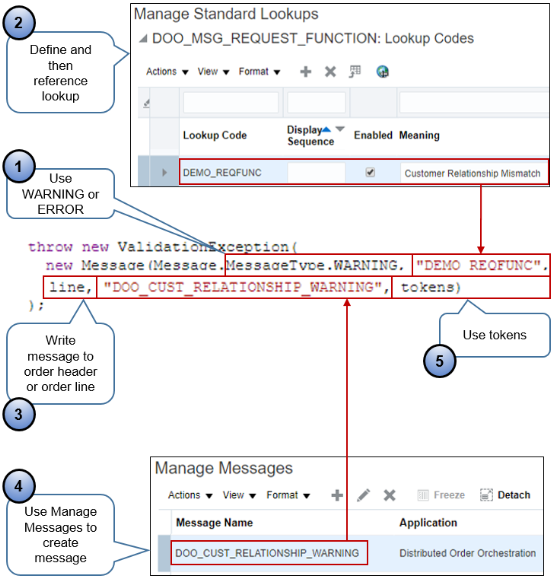
Note
-
messageType. Define the messageType as WARNING or ERROR. The Order Management work area uses a different icon for each type.
-
Lookup. Use the Manage Order Lookups page in the Setup and Maintenance work area to categorize your messages, then reference them from your code.
Use predefined lookups or create your own. Categorizing helps the Order Entry Specialist search for messages and view them in analytic charts.
Use Error to improve search and display because errors display in work area Order Management in attribute Error Type.
In this example, assume you define the Meaning as Customer Relationship Mismatch.
-
Header or line. Indicate where the error happens. Use one of these values.
-
header. Apply the message to an error that happens on the order header.
-
line. Apply the message to an error that happens on an order line.
The example in the screen print uses
line
. This example includes code (not visible, for brevity) abovethrow new ValidationException
that definesline
as a variable, uses it to iterate through all the lines in the sales order, then saves the order line number where the error happens, such as line 3. At run time, Order Management displays an icon on line 3 that the Order Entry Specialist can click to examine message details.
-
-
Manage Messages. Use the Manage Messages page in the Setup and Maintenance work area.
-
Referencing a message provides advantages over hard coding the message.
-
Reference the message from different extensions, manage the message independently of the extension, and translate the message to some other language besides English.
-
Using the Manage Messages page can simplify message management because other developers and administrators can then use Manage Messages instead of modifying
hard coded
messages in your extension, which requires knowledge of writing in Groovy. -
You can also define a message to help you troubleshoot your extension during development. You can include details in the message that display the state of various objects to help you pinpoint problem areas. Remove the message when you're ready to deploy your extension to your production environment.
-
-
Use tokens as placeholders for variable content.
The Order Entry Specialist can view and search data in the Order Management work area according to Error Type or Message Type. In this example, notice that the Overview page displays Customer Relationship Mismatch as a label in the Draft Orders in Error diagram.
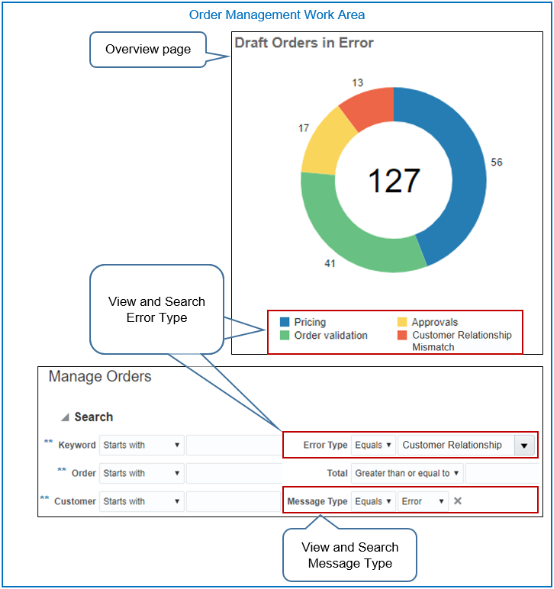
The Order Entry Specialist can take action.
-
Click on the orange part of the circle to drill into sales orders that are in error because of the mismatch.
-
Search for the Error Type attribute on the Manage Orders for Customer Relationship Mismatch page.
Accumulate Error Messages and Display Them
Accumulate messages, then display them in the Order Management work area all together at one time.
For example, assume you write an extension that contains three lines that check for error conditions, x, y, and z. At run time, assume x, y and z all meet their error conditions, but the extension stops immediately after it encounters x and displays the message for error x.
Instead, write your extension so it continues to run through y and z, saves each message to a temporary list, then displays messages for x, y and z together in a single dialog. This technique allows the Order Entry Specialist to examine all errors together, correct them, then resubmit the sales order instead of correcting x, submit, correct y, submit, correct z, and submit.
For example:
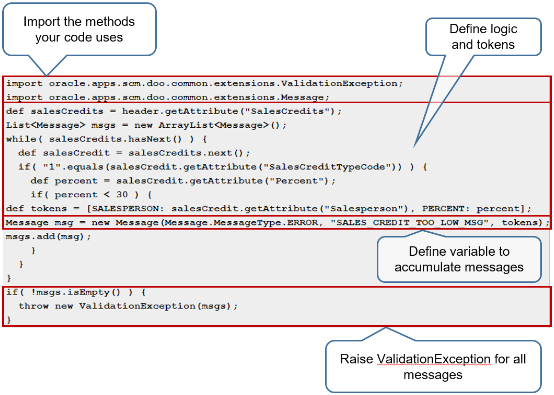
If the revenue percentage is less than 30% for the sales credit, then the extension creates a message and stores it in a local list. The extension processes all sales credits. If the local list contains any messages after it finishes processing sales credits, then the extension displays them in a dialog in the Order Management work area.
Here is the code that this example uses to examine each sales credit.
Code |
Description |
---|---|
|
Import the ValidationException method so your code can use it. |
|
Import the Message method so your code can use it. |
|
Define a local variable named salesCredits, use the getAttribute method to get the value of the SalesCredits attribute from the order header, then set the value of this variable to the value that getAttribute returns. getAttrbute("SalesCredits") returns an iterator you can use to access sales credits rows. For example, the code references salesCredits later in this topic to get SalesCreditTypeCode, Percent, and Salesperson. |
|
Use this code.
|
The |
Iterate through each sales credit until it finishes processing all of them. Examine attributes SalesCreditTypeCode, Percent, and Salesperson for each sales credit. |
|
Define a local variable named |
|
If the condition is True, then create a message,
and add it to the local |
|
Define these tokens.
|
|
Define a local variable named |
|
Use this code.
|
|
Add the contents of local variable |
|
If local list Perform this check after the while loop finishes processing all sales credits. |
Here's the complete code without comments.
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
def salesCredits = header.getAttribute("SalesCredits");
List<Message> msgs = new ArrayList<Message>();
while( salesCredits.hasNext() ) {
def salesCredit = salesCredits.next();
if( "1".equals(salesCredit.getAttribute("SalesCreditTypeCode")) ) {
def percent = salesCredit.getAttribute("Percent");
if ( percent < 30 ) {
def tokens = [SALESPERSON: salesCredit.getAttribute("Salesperson"), PERCENT: percent];
Message msg = new Message(Message.MessageType.ERROR, "SALES_CREDIT_TOO_LOW_MSG", tokens);
msgs.add(msg);
}
}
}
if( !msgs.isEmpty() ) {
throw new ValidationException(msgs);
}
Consider Order Import Behavior
Import behavior it different depending on whether your extension creates a warning message or error message.
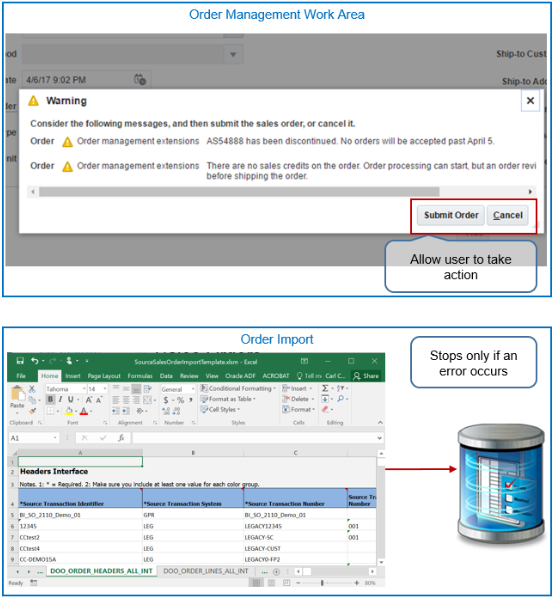
Note
-
Sales orders entered in the Order Management work area. The Warning dialog in work area Order Management allows the Order Entry Specialist to examine the warning, then submit the sales order or cancel.
-
Source orders imported from a source system. Order processing stops only if an error happens. If only warnings happen, then you can view the messages but the import submits the sales order to order fulfillment. It doesn't allow user intervention.