Pricing Algorithm Steps
Set up logic for your pricing algorithm steps.
Here are the types of steps that you can add.
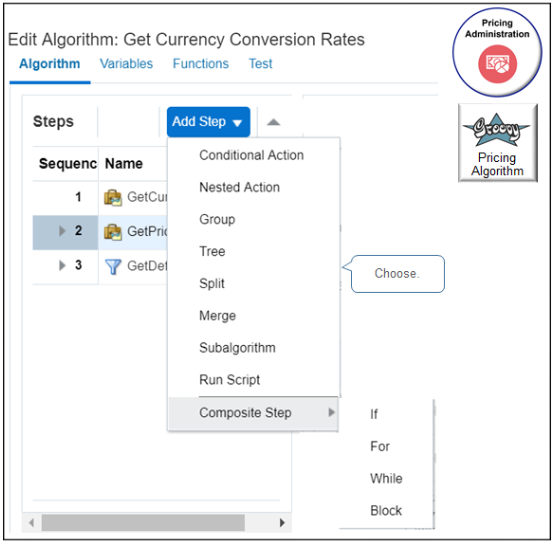
You typically use Conditional Action, Nested Action, Subalgorithm, or Group.
Conditional Action Step
If the primary data set meets the condition you specify, then a conditional action step does an action on all rows in the set. Here's the structure a conditional action step uses.
If (condition 1) { . . . }
else if (condition 2) { . . . }
else if (condition 3) { . . . }
. . .
else { default }
Here's some example conditions from the Copy Basis Value to Candidate step of the predefined Calculate Covered Item Charges algorithm.
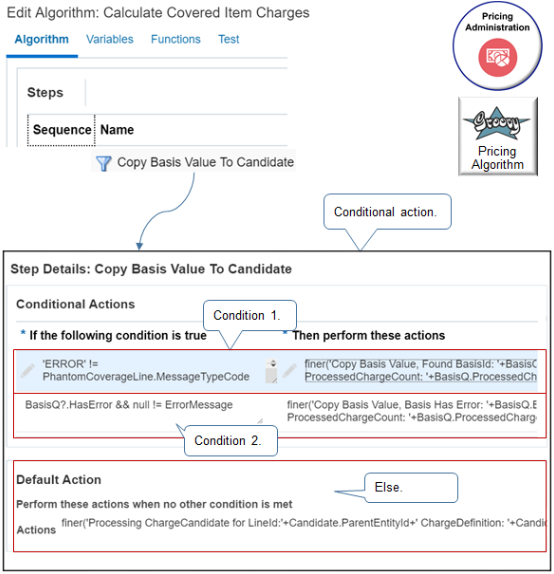
In the next example, examine a step that calculates a rollup charge for a configured item. Here's what it does.
-
Evaluates each row of the primary data set.
-
Iterates through the charge for the root item of the configured item, and the charge for each child item.
-
Creates a rollup charge for each charge definition, as necessary.
Here's a more detailed description of what it does.
-
Gets charges from the PriceRequest payload.
-
If
Charge.RollupFlag = true
, which indicates that the payload contains at least one rolled up charge, then create a RollupCharge variable in the payload. -
Populate the RollupCharge variable with rolled up charges for each child item of the configured item.
-
Set the OutputStatus to SUCCESS on the ServiceParam data set to indicate that this step successfully created the rollup charges.
Examine a step that includes a conditional action.
-
Go to the Pricing Administration work area.
-
On the Overview page, click Tasks > Manage Algorithms.
-
On the Manage Algorithms page, click Calculate Rollup Charges for Prepriced Orders, then click Actions > Create Version.
-
In the Name column, click the new version of Calculate Rollup Charges for Prepriced Orders that you just created.
-
On the Edit Algorithm page, click the Create Rollup Charge step.
-
In the Step Details area, in the Condition window, examine the condition.
Condition
Description
!Charge.RollupFlag && 'LINE' == Charge.ParentEntityCode && ('ROOT' == Line.ItemType || 'COMPONENT' == Line.ItemType)
Set the exit criteria for this step.
In this example, the condition sets the OutputStatus to SUCCESS in the ServiceParam data set to indicate that the step successfully created the rollup charges.
-
In the Step Details area, notice the parts of the Execute Condition area.
Part
Description
Local Variable
Declare variables that apply only to the code you write in the Conditional Actions and Default Action areas.
Conditional Actions
Set up conditional logic that Pricing applies for the step.
Default Action
If the conditional action doesn't evaluate to true, then set up the logic to use for the default action step.
-
In the Local Variables area, notice the variables.
Variable Name
Description
Rollup
Identifies each charge when Pricing iterates through the code in the Action window in the Conditional Actions area.
RollupChargeCounter
Counts the iterations.
-
In the Conditional Actions area, notice the condition.
True Condition
Description
RollupCharge.size() = 0
Specifies the condition.
-
In the Conditional Actions area, examine the code in the Actions window.
This code gets charges for each child item of the configured item, then populates the RollupCharge variable with the rolled up charge for the child. Pricing iterates through the code for each child item until it finishes pricing the child.
-
Click Add Condition > True Condition.
Notice that Pricing added a new condition in the Conditional Actions area. If you add more than one condition, then Pricing evaluates each condition in the sequence that the Conditional Actions area displays them. Click Move Up or Move Down to modify the sequence.
-
In the Conditional Actions area, click Delete.
-
On the Manage Algorithms page, delete the In Progress version of pricing algorithm Calculate Rollup Charges for Prepriced Orders.
Nested Action Step
A nested action step does a different action on each row of the data set.
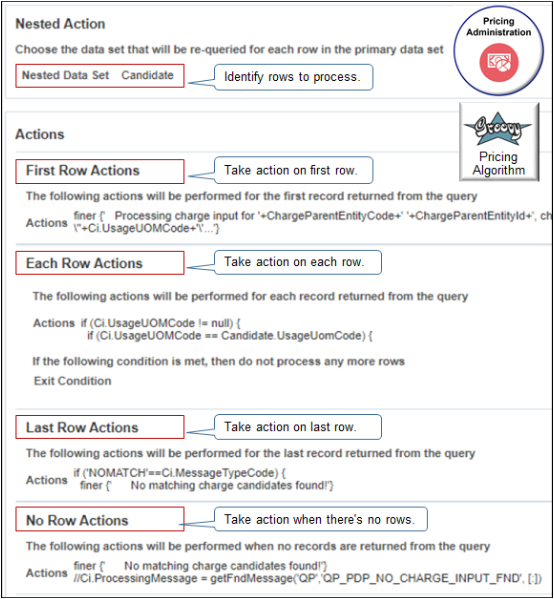
Note
-
Use the Nested Data Set to identify the rows that the nested action processes.
-
Here's what the actions do.
An Action on This Row
Applies the Action on This Record
First row action.
The first record that the query returns.
For each row action.
Each record that the query returns, including the first record.
Last row action.
The last record that the query returns.
No row action.
No record. Applies when the query doesn't return any records.
Here's the structure a nested action step uses.
For each Nested Set Row {
If First Row {. . .}
For Each Row {. . .}
If Last Row {. . .}
If No Row {. . .}
}
Assume you must implement a condition.
-
If more than one order line on a sales order references the same item, then apply a discount on the item.
You can write a pricing algorithm step that applies action x to the first row of the data set, and action y to the other rows of the data set.
Examine a step that uses a nested action to find a charge on the shipping charge list for each item on each order line, then writes the candidates to the output SDO.
-
Go to the Pricing Administration work area.
-
On the Overview page, click Tasks > Manage Algorithms.
-
On the Manage Algorithms page, click Calculate Shipping Charges, then click Actions > Create Version.
-
In the Name column, click the new version of Calculate Shipping Charges that you just created.
-
On the Edit Algorithm page, click the Find Shipping Charge Candidates step.
-
In the Step Details area, in the Condition window, examine the condition.
Condition
Description
'SUCCESS' == ServiceParam.OutputStatus && 'ERROR' != Line.MessageTypeCode && 'ORDER' == Line.LineCategoryCode && ('STANDARD' == Line.ItemType || 'ROOT' == Line.ItemType) && Line.AppliedShippingChargeListId != null && finer(AlgmName + ': finding charge candidates for line ' + Line.LineId + ' (' + Line.InventoryItemId + ')') == null
Set the exit criteria for this step.
The condition sets the OutputStatus to SUCCESS in the ServiceParam data set to indicate that this step successfully found a charge on the shipping charge list for each item on each order line, and then wrote the candidates to the output SDO.
-
In the Nested Action area, notice that you can choose the data set that this step will query each time it processes a row in the primary data set.
In this example, notice that the step comes predefined to use the Charge data set because it includes the data that the step requires to find a charge on the shipping charge list for each item, such as the PricingStrategyId to use for the order line, the SellingBusinessUnitId for the order header, and so on.
-
In the Actions area, click Add Action, then notice that you can specify a different action for each row that the query returns.
For example, choose First Row Actions to add an action that this step performs only on the first row that the query returns.
-
In the First Row Actions area, in the Actions window, examine the code, and notice that it finds a charge on the shipping charge list for each item that the first row references.
To simplify viewing, copy the code to the clipboard, then paste it into a word processing application.
-
In the No Row Actions area, in the Actions window, examine the code, and notice that it handles a situation where the step can't locate a shipping charge list for the item.
-
On the Manage Algorithms page, delete the In Progress version of the Calculate Shipping Charges pricing algorithm.
Subalgorithm Step
A subalgorithm step calls another pricing algorithm. Here's an example.
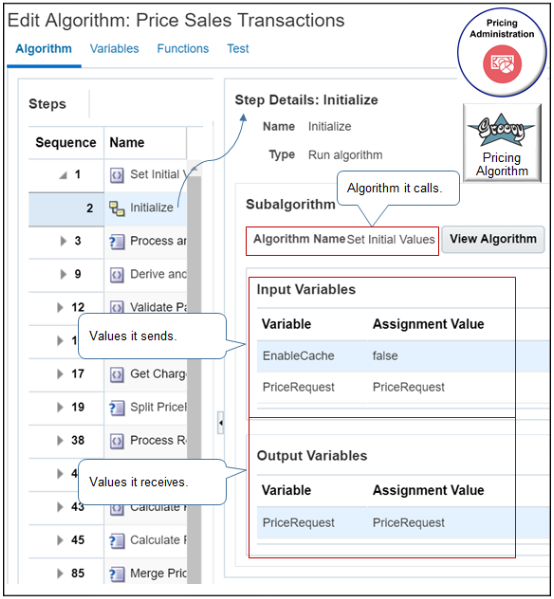
Note
-
The Initialize step of the predefined Price Sales Transactions algorithm calls the Set Initial Values algorithm.
-
Use the Algorithm Name in the Subalgorithm area to choose the subalgorithm.
-
Use input variables to specify values that the calling algorithm sends to the subalgorithm.
-
Use output variables to specify values that the subalgorithm sends to the calling algorithm.
-
The Step Details area adds variables that the subalgorithm contains when you set the Algorithm Name. If you require more, create them, then add them.
Group Step
A group step groups the rows in the primary data set according to the value of an attribute, then processes a different action for each group.
For example, the predefined Aggregate Roll Up Charge Components pricing algorithm rolls up charges so your customer can view one value for a configured item. This step creates an aggregate charge component that sums the individual charge components for a rollup charge.
Examine a step that finds a charge on the shipping charge list for each item on each order line, and then writes the candidates to the output SDO.
-
Go to the Pricing Administration work area.
-
On the Overview page, click Tasks > Manage Algorithms.
-
On the Manage Algorithms page, click Aggregate Roll Up Charge Components, then click Actions > Create Version.
-
In the Name column, click the new version of Aggregate Roll Up Charge Components that you just created.
-
On the Edit Algorithm page, click the Create Aggregate Charge Component by Element Code step.
This step examines charge components that aren't aggregated and adds them together according to the price element code for each rollup charge. It then creates the corresponding aggregate charge component and sets the RollupFlag for the component to true.
-
In the Step Details area, in the Condition window, examine the condition.
Condition
Description
'SUCCESS' == ServiceParam.OutputStatus && !(Comp.IsPassedIn ?: false) && Rollup != null && !(Rollup.IsPassedIn ?: false) && 'LINE' == Rollup.ParentEntityCode && (PriceElement == null || Comp.PriceElementCode == PriceElement) && !Comp.RollupFlag && Comp.AggregateChargeComponentId == null && Comp.SourceTypeCode!='MANUAL_ADJUSTMENT'
Sets the exit criteria for this step.
The condition sets the OutputStatus to SUCCESS in the ServiceParam data set to indicate that the step successfully added charge components according to the price element code for each rollup charge, then created the corresponding aggregate charge component.
-
In the Group by Attributes area, notice the predefined attributes. This step uses them to arrange rows in the primary data set into groups.
Attribute
Description
Rollup.ChargeId
Groups rows in the primary data set according to the ChargeId attribute in the Rollup data set.
Comp.PriceElementCode
Groups rows in the primary data set according to the PriceElementCode attribute of the
Comp
data set.Each attribute you add in the Group by Attributes area adds the potential for a separate group of rows in the primary data set. Pricing arranges them sequentially according to the attributes you add.
If you add more than one attribute, then Pricing places the group according to the sequence that the Group by Attributes area displays them. It places the first group first, the second group immediately after the first group. It places rows that aren't in any group immediately after the last group.
For example:
-
The ChargeId attribute in rows two, five, and seven of the Rollup data set each equal 123.
-
The PriceElementCode attribute in rows three, eight, and nine of the
Comp
data set each equal 456.
Here's the sequence the step uses to arrange rows in the
Comp
primary data set for this example.-
Row two of the Rollup data set
-
Row five of the Rollup data set
-
Row seven of the Rollup data set
-
Row three of the
Comp
data set -
Row eight of the
Comp
data set -
Row nine of the
Comp
data set -
All other rows that aren't in the first group or the second group
-
-
In the Actions area, click Add Action, then notice you can specify different actions for rows in each group.
Actions
Description
Group First Row Actions
Group actions for the first row.
Group Each Row Actions
Group actions for each row.
Group Last Row Actions
Group actions for the last row.
-
On the Manage Algorithms page, delete the In Progress version of the Aggregate Roll Up Charge Components pricing algorithm.