Get and Post the Resources Consumed to Fulfill Work Orders
You can use a REST API to post the resources that the plant consumed to fulfill one work order operation. You can use a REST API to scan the serial of an assembly to get the details of the work order and work order resources, and then post the transaction for the resources that the work order operation consumes.
Let's discuss these scenarios:
-
Get work order details according to assembly serial.
-
Get the resources required to fulfill one work order operation.
-
Post resource consumption for one work order operation.
Get Work Order Details According to Assembly Serial
Assume you create a solution that allows John Brown, a discrete manufacturer, to use a mobile client to connect to your partner application, and then use a REST API. An application on the client scans the serial of assembly PRE5712, and gets the serial details.
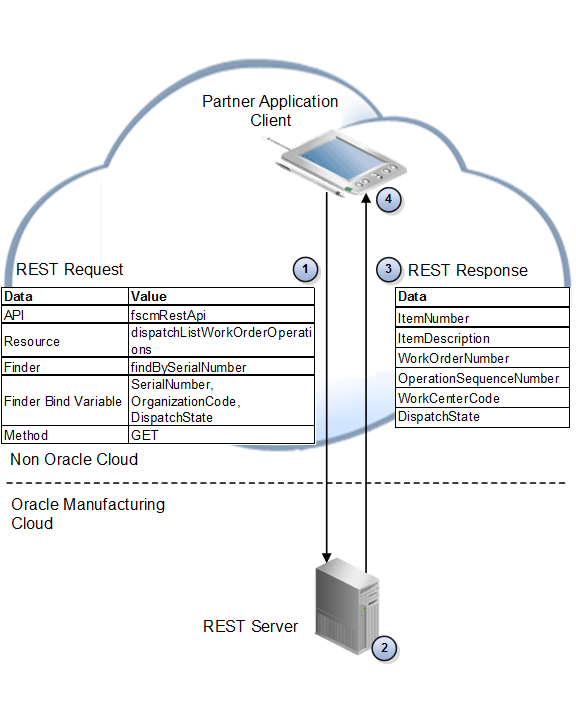
Here's a typical application processing flow for the scenarios:
-
You send a REST request from the client to the server to get work order details for assembly serial PRE5712.
-
The response payload includes details that describe the results of the request.
-
Your partner application extracts the values for the following attributes from the response payload, and then displays them in the client.
Attribute
Description
ItemNumber
Value that uniquely identifies the Assembly Number. For example, MI-1234.
ItemDescription
Text description of the Assembly. For example, 5.5" mobile.
WorkOrderNumber
Number that identifies the work order. For example, M1-1208.
OperationSequenceNumber
Number that identifies the work order operation. For example, 20.
WorkCenterCode
Code that uniquely identifies the work center. For example, YLX_WC_02.
DispatchState
Dispatch state of the assembly. For example, READY.
Example URL
Use this resource URL format.
GET
curl -u username:password "https://servername/fscmRestApi/resources/version/dispatchListWorkOrderOperations?finder=findBySerialNumber;SerialNumber=PRE5712,OrganizationCode=M1,DispatchState=READY"
Example Response
Here's an example of the response body in JSON format.
{ "items" : [ { "OrganizationId" : 207, "OrganizationCode" : "M1", "OrganizationName" : "Tall Manufacturing", "WorkAreaId" : 300100091177813, "WorkAreaCode" : "YLX_WA_02", "WorkAreaName" : "YLX_WA_02", "WorkCenterId" : 300100091177814, "WorkCenterCode" : "YLX_WC_02", "WorkCenterName" : "YLX_WC_02", "WorkCenterDescription" : "YLX_WC_02", "WorkOrderId" : 300100093180128, "WorkOrderNumber" : "M1-1208", "WorkOrderDescription" : "WO for prototype", "InventoryItemId" : 300100039626650, "ItemNumber" : "MI-1234", "ItemDescription" : "5.5" mobile", "OperationId" : 300100093180130, "OperationSequenceNumber" : 20, "OperationName" : "OP20", "OperationDescription" : "Assemble", "DispatchState" : "READY", "Quantity" : 10, "UnitOfMeasure" : "Each", "PlannedStartDate" : "2016-10-27T04:38:00-07:00", "PlannedCompletionDate" : "2016-10-27T04:38:00-07:00", "WorkOrderPriority" : null, "PurchaseOrderId" : null, "PurchaseOrder" : null, "PurchaseOrderLineId" : null, "PurchaseOrderLine" : null, "CustomerId" : null, "CustomerName" : null, } ] }
Get the Resources Required to Fulfill One Work Order Operation
John gets work order number M1-1208 and operation sequence number 20, and then gets the list of resources that operation 20 requires.
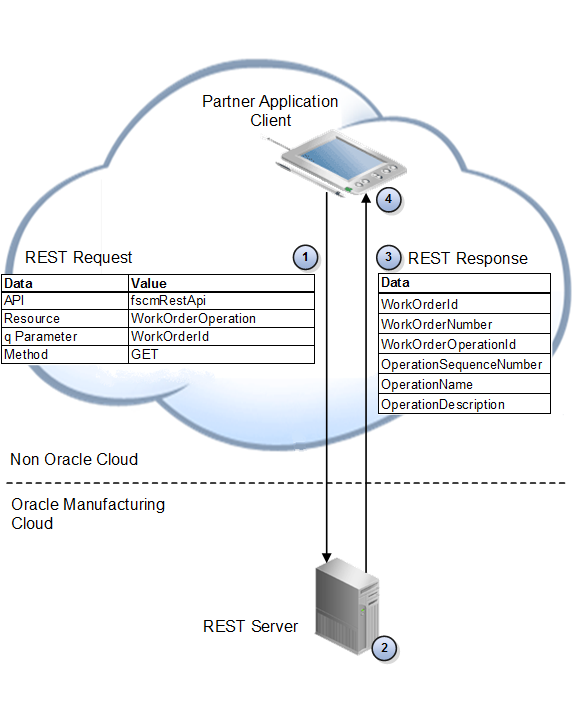
Here's a typical application processing flow for the scenarios:
-
Send a REST request to get the component details.
You use the values of these attributes that you received in the response payload when you searched for work order details according to assembly serial:
Attribute
Description
WorkOrderId
Unique value that Oracle Manufacturing Cloud creates when it creates a work order. For example, 300100093180128.
WorkOrderOperationId
Unique value that Oracle Manufacturing Cloud creates when it creates a work order operation. For example, 300100093180130.
-
The response payload includes details that describe the results of the request.
-
Your partner application extracts the values for these attributes from the response payload, and then displays them in the client.
Attribute
Description
ResourceSequenceNumber
Value that uniquely identifies the sequence of the resource in the work order operation. For example, 10.
ResourceCode
Code that uniquely identifies the resource. For example, RT-A-R9.
ResourceDescription
Description of the resource. For example, Test Workbench.
ResourceType
Type of resource. For example, EQUIPMENT or LABOR.
UsageRate
Resource usage required to make one unit of the assembly.
BasisType
Contains 1 or 2. 1: the resource consumption is fixed. It does not change according to work order quantity. 2: the resource consumption is not fixed. It might change according to work order quantity.
RequiredUsage
Resource usage that the plant requires to make the work order quantity. The application calculates this value depending on whether the Basis type is fixed or variable. Fixed: the Required Usage equals the Usage.
UnitOfMeasure
Unit of measure of the resource usage.
AssignedUnits
Number of resource units the application assigns to perform the operation.
Example URL
Use this resource URL format.
GET
curl -u username:password "https://servername/fscmRestApi/resources/version/workOrders/WorkOrderId/child/WorkOrderMaterial?q=WorkOrderOperationId=WorkOrderOperationId"
For example, the following command gets resource details for work order M1-1208:
curl -u username:password "https://servername/fscmRestApi/resources/version/workOrders/300100093180128/child/WorkOrderResource?q=WorkOrderOperationId=300100093180130"
Example Response
Here's an example of the response body in JSON format.
{ "items": [ { "OrganizationId": 207, "ResourceSequenceNumber": 10, "WorkOrderOperationResourceId": 300100071643550, "ResourceId": 300100071643444, "ResourceCode": "RT-A-R9", "ResourceName": "RT-A-R9", "ResourceDescription": "Test Workbench", "ResourceType": "EQUIPMENT", "WorkAreaId": 300100071643435, "WorkAreaName": "RT-A-WA1", "WorkAreaCode": "RT-A-WA1", "WorkAreaDescription": "RT-A-WA1", "WorkCenterId": 300100071643453, "WorkCenterName": "RT-A-WC1", "WorkCenterCode": "RT-A-WC1", "WorkCenterDescription": "RT-A-WC1", "WorkOrderId": 300100093180128, "WorkOrderOperationId": 300100093180130, "OperationSequenceNumber": 20, "OperationName": "Test", "StandardOperationId": null, "StandardOperationCode": null, "PrincipalFlag": false, "UsageRate": 5, "BasisType": "1", "RequiredUsage": 75, "InverseRequiredUsage": 0.2, "UnitOfMeasure": "HRS", "ChargeType": "MANUAL", "AssignedUnits": 1, "PlannedStartDate": "2016-10-04T17:27:00-08:00", "PlannedCompletionDate": "2016-10-07T20:27:00-08:00", "ResourceActivityCode": null, "ScheduledFlag": false, "PhantomFlag": false, "ActualResourceUsage": null, }, { "OrganizationId": 207, "ResourceSequenceNumber": 20, "WorkOrderOperationResourceId": 300100071643551, "ResourceId": 300100071643445, "ResourceCode": "RT-A-R10", "ResourceName": "RT-A-R10", "ResourceDescription": "Test Engineer", "ResourceType": "LABOR", "WorkAreaId": 300100071643435, "WorkAreaName": "RT-A-WA1", "WorkAreaCode": "RT-A-WA1", "WorkAreaDescription": "RT-A-WA1", "WorkCenterId": 300100071643453, "WorkCenterName": "RT-A-WC1", "WorkCenterCode": "RT-A-WC1", "WorkCenterDescription": "RT-A-WC1", "WorkOrderId": 300100093180128, "WorkOrderOperationId": 300100093180130 "OperationSequenceNumber": 20, "OperationName": "Test", "StandardOperationId": null, "StandardOperationCode": null, "PrincipalFlag": false, "UsageRate": 5.98802, "BasisType": "1", "RequiredUsage": 89.8203, "InverseRequiredUsage": 0.16700011022007274, "UnitOfMeasure": "HRS", "ChargeType": "MANUAL", "AssignedUnits": 1, "PlannedStartDate": "2016-10-07T20:27:00-08:00", "PlannedCompletionDate": "2016-10-11T14:16:13-08:00", "ResourceActivityCode": null, "ScheduledFlag": false, "PhantomFlag": false, "ActualResourceUsage": null, } ] }
Post Resource Consumption for One Work Order Operation
The operator on the shop floor records the hours consumed for one RT-A-R9 resource, and then posts the resource transaction in Oracle Manufacturing Cloud.
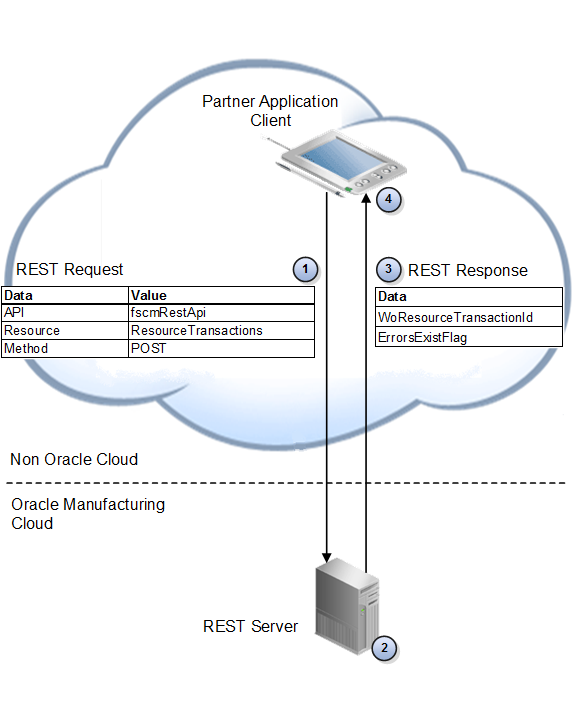
Here's a typical application processing flow for the scenarios:
-
Store the resource usage for one serial of the work order operation.
Note:
The request payload does not contain the serial.
-
The response payload includes details that describe the results of the request.
-
Your partner application extracts the values for these attributes from the response payload, and then displays them in the client. This table includes only some of the attributes of the Material Transaction resource.
Note:
Some resources contain only a few attributes. Others might contain several hundred attributes.
Attribute
Description
WoResourceTransactionId
Value that uniquely identifies the material transaction number. For example, 23826.
ErrorsExistFlag
A flag that indicates whether the transaction succeeded or failed.
Example URL
Use this resource URL format to post one resource transaction in plant M1, for work order M1-1208, operation sequence 20, and resource RT-A-R9.
POST
curl -u username:password -X POST -H "Content-Type:application/vnd.oracle.adf.resourceitem+json" -d 'request payload' "https://servername/fscmRestApi/resources/version/resourceTransactions"
Example Request
Here's an example of the request body in JSON format.
{ "SourceSystemCode" : "Client", "SourceSystemType" : "EXTERNAL", "ResourceTransactionDetail" : [ { "OrganizationCode" : "M1", "OrganizationId" : 207, "WorkOrderNumber":"M1-1208", "OperationSeqNumber":"20", "ResourceSeqNumber":"10", "ResourceCode":" RT-A-R9", "TransactionQuantity":"1", "TransactionUomCode":"HRS", "TransactionDate" : "2016-10-31T23:16:22-07:00", "TransactionTypeCode":"RESOURCE_CHARGE", } ] }
Example Response
Here's an example of the response body in JSON format.
{ "SourceSystemType" : "EXTERNAL", "SourceSystemCode" : "Client", "ErrorsExistFlag" : "false", "ResourceTransactionDetail" : [ { "WoResourceTransactionId" : 23826, "SourceSystemCode" : null, "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "OperationSequenceNumber" : 20, "ResourceSequenceNumber" : 10, "ResourceCode" : "RT-A-R9", "ResourceActivityCode" : null, "TransactionTypeCode" : "RESOURCE_CHARGE", "TransactionQuantity" : 1, "TransactionUnitOfMeasure" : "HRS", "TransactionDate" : "2016-10-31T23:16:22-07:00", "SourceLineReference" : null, "SourceHeaderReference" : null, "SourceLineReferenceId" : null, "SourceHeaderReferenceId" : null, "ErrorMessages" : "", "ErrorMessageNames" : "", }