9 Create Login and Logout Functionality
The HR Web Application has two users, namely hradmin
and
hrstaff
.
Once you login to the HR Application, you see the landing page, with details of the
web application. The hradmin
and hrstaff
have different
privileges and access to different features.
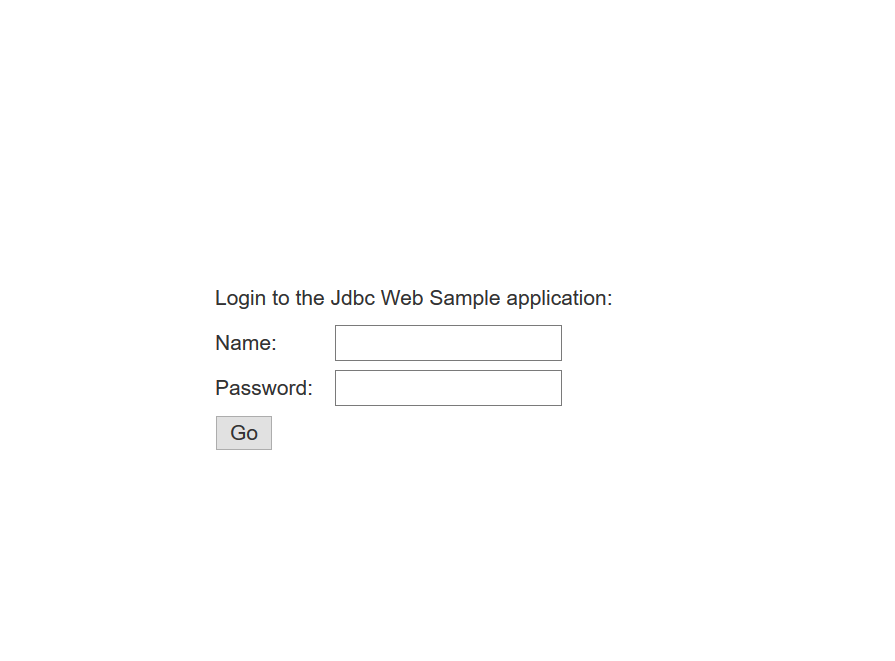
This chapter shows the required classes and additional code required to build the Login and Logout functionality in the application.
-
Create a XML file
tomcat-users.xml
for login functionality. -
Create a HTML page
login.html
to login the user. -
Create a HTML page
login-failed.html
to display the error message. -
Create a
web.xml
to authenticate the users during login. -
Create a HTML page
about.html
to show more details about the application. -
Create a landing page
index.html
and define the html pages for redirection. - Add code to the servlet
WebController.java
to process logout.
9.1 Create
tomcat-users.xml
Create an XML file tomcat-users.xml
and list down the users
you want to allow access to. Specify both the username and password for each one of the
users.
Class Name: /java/HRWebApp/tomcat-users.java
Github Location: tomcat-users.xml
Steps to create the xml file:
- Create the file
tomcat-users.xml
.<?xml version='1.0' encoding='utf-8'?> z <tomcat-users xmlns="http://tomcat.apache.org/xml" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://tomcat.apache.org/xml tomcat-users.xsd" version="1.0"> <role rolename="manager"/> <role rolename="staff"/> <user username="hradmin" password="welcome" roles="manager,staff"/> <user username="hrstaff" password="welcome" roles="staff"/> </tomcat-users>
- Place this file under
TOMCAT_HOME/conf/tomcat-users.xml
on your machine.
9.2 Create login.html
The login page is displayed when you invoke the main page of the web application. The login page displays the fields to capture the username and password.
Class Name: src/main/webapp/login.html
Github Location: login.html
Steps to create the HTML page:
- Create the title, head, and stylesheet for
login.html
.<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Login to Jdbc Web Sample application</title> <link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"> <style> #cent { position:absolute; top:50%; left:50%; margin-top:-50px; /* this is half the height of your div*/ margin-left:-100px; /*this is half of width of your div*/ } td { height: 30px; } </style> </head>
- Create the
<body>
and<form>
to submit the login credentials entered by the user.<body> <div id="cent"> <form method="POST" action="j_security_check"> <table> <tr> <td colspan="2">Login to the Jdbc Web Sample application:</td> </tr> <td>Name:</td> <td><input type="text" name="j_username" /></td> </tr> <tr> <td>Password:</td> <td><input type="password" name="j_password"/></td> </tr> <tr> <td colspan="2"><input type="submit" value="Go" /></td> </tr> </table> </form> </div> </body>
9.3 Create
login-failed.html
A html page to display the error messgae if the login is unsuccessful.
Class Name: src/main/webapp/login-failed.html
Github Location: login-failed.html
Steps to create the HTML page:
- Create the
login-failed.html
as shown below.<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Login Failed</title> </head> <body> <p> Sorry, login failed! </p> </body> </html>
9.4 Create web.xml
The web.xml
file consists of descriptors to authenticate
the users when the login page is displayed to the user.
Class Name: src/main/webapp/WEB-INF/web.xml
Github Location: web.xml
Steps to create the xml file:
- Use the following code to create the
web.xml
file.<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd"> <web-app> <display-name>Jdbc Web Sample</display-name> <security-role> <role-name>manager</role-name> </security-role> <security-role> <role-name>staff</role-name> </security-role> <security-constraint> <web-resource-collection> <web-resource-name>Wildcard means whole app requires authentication</web-resource-name> <url-pattern>/*</url-pattern> <http-method>GET</http-method> <http-method>POST</http-method> </web-resource-collection> <auth-constraint> <role-name>manager</role-name> </auth-constraint> <user-data-constraint> <transport-guarantee>NONE</transport-guarantee> </user-data-constraint> </security-constraint> <security-constraint> <web-resource-collection> <web-resource-name>Wildcard means whole app requires authentication</web-resource-name> <url-pattern>/*</url-pattern> <http-method>GET</http-method> </web-resource-collection> <auth-constraint> <role-name>staff</role-name> </auth-constraint> <user-data-constraint> <transport-guarantee>NONE</transport-guarantee> </user-data-constraint> </security-constraint> <login-config> <auth-method>FORM</auth-method> <form-login-config> <form-login-page>/login.html</form-login-page> <form-error-page>/login-failed.html</form-error-page> </form-login-config> </login-config> </web-app>
9.5 Create about.html
The about.html
file displays information about the HR
Application, users and functionalities.
Class Name: src/main/webapp/about.html
Github Location: about.html
Steps to use the HTML page: Download the about.html and use it in your application.
9.6 Create index.html
The index.html
file consists of all details about the HR
Web Application. It describes in detail its users and functionalities.
Class Name: src/main/webapp/index.html
Github Location: index.html
Steps to create the HTML page:
- Create the title, head, and stylesheet for
index.html
.<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Employee table listing</title> <link rel="stylesheet" type="text/css" href="css/app.css" > <style> iframe:focus { outline: none; } iframe[seamless] { display: block; } </style> </head> <body>
- Create
<body>
and actions for the features through navigation links and logout.<body> <div id="sideNav" class="sidenav"> <a href="javascript:void(0)" class="closebtn" onclick="closeNav()" class="staff">×</a> <a href="javascript:switchSrc('listAll.html')" class="staff">List All</a> <a href="javascript:switchSrc('listById.html')" class="staff">Search By Id</a> <a href="javascript:switchSrc('listByName.html')" class="manager">Update Employee Record</a> <a href="javascript:switchSrc('incrementSalary.html')" class="manager">Increment Salary</a> <a href="javascript:switchSrc('about.html')">About</a> </div> <div id="main"> <div align="right"> <div id="myrole" style="display:inline; color:#393318; display: block; background-color:#eff0f1;position: absolute; top: 20px; right: 8%;" >myrole</div> <a href="javascript:void(0)" onclick="logout()" class="staff" style="display: block; position: absolute; top: 20px; right: 1%">Logout</a> </div> <div> <span style="font-size:30px;cursor:pointer" onclick="openNav()"> Java 2 Days HR Web Application </span> </div> <div> <iframe id="content" src="about.html" frameborder="0" style="overflow:hidden; height:100%; width:100%" height="100%" width="100%"></iframe> </div> </div> <script> function openNav() { document.getElementById("sideNav").style.width = "256px"; document.getElementById("main").style.marginLeft = "256px"; } function closeNav() { document.getElementById("sideNav").style.width = "0"; document.getElementById("main").style.marginLeft= "0"; } function switchSrc(src) { document.getElementById('content').src = src; } function logout() { var xmllogout = new XMLHttpRequest(); xmllogout.open("GET", "WebController?logout=true", true, "_", "_"); xmllogout.withCredentials = true; // Invlalid credentials to fake logout xmllogout.setRequestHeader("Authorization", "Basic 00001"); xmllogout.send(); xmllogout.onreadystatechange = function() { window.location.replace("index.html"); } return true; } var xmlhttp = new XMLHttpRequest(); var url = "getrole"; xmlhttp.onreadystatechange = function() { if (xmlhttp.readyState == 4 && xmlhttp.status == 200) { role = xmlhttp.responseText; console.log("role: " +role); if (role == "staff") { console.log ("disabling manager"); var x = document.getElementsByClassName('manager'); for(i = 0; i < x.length; ++i) { x[i].style.display = 'none'; } } document.getElementById('myrole').innerHTML = ' '+role+' '; } } xmlhttp.open("GET", url, true); xmlhttp.send(); </script> </body>
9.7 Add the code to a Servlet to process the request
Add the relevant code to WebController.java
to login and
logout of the application.
Class Name:
src/main/java/com/oracle/jdbc/samples/web/WebController.java
Github Location: WebController.java
Steps to add the code:
- Open the
WebController.java
class. To create theWebController.java
, refer to Creating a Servlet to Process the Request. Use the same class and add the required code. - Declare a variable
LOGOUT
to capture the status of the user. This is a global variable, hence, declare it outside the methodprocessRequest()
but within theWebController
class.private static final String LOGOUT = "logout";
- The method
processRequest()
is already created in the ListAll feature. Now, we add the code to implement Logout functionality. Create anif
block to verify the functionality you will invoke based on input. Check if the input value isLOGOUT
.if ((value = request.getParameter(LOGOUT)) != null) { /* Getting session and then invalidating it */ HttpSession session = request.getSession(false); if (request.isRequestedSessionIdValid() && session != null) { session.invalidate(); } handleLogOutResponse(request,response); response.setStatus(HttpServletResponse.SC_UNAUTHORIZED); return; }
- If the input value is
LOGOUT
, invoke the method to handle the logout of user.private void handleLogOutResponse(HttpServletRequest request, HttpServletResponse response) { Cookie[] cookies = request.getCookies(); for (Cookie cookie : cookies) { cookie.setMaxAge(0); cookie.setValue(null); cookie.setPath("/"); response.addCookie(cookie); } }