2.1 Simple Example: Inserting, Indexing, and Querying Spatial Data
This topic presents a simple example of creating a spatial table, inserting data, creating the spatial index, and performing spatial queries
It refers to concepts that were explained in Spatial Concepts and that will be explained in other sections of this chapter.
The scenario is a soft drink manufacturer that has identified geographical areas of marketing interest for several products (colas). The colas could be those produced by the company or by its competitors, or some combination. Each area of interest could represent any user-defined criterion: for example, an area where that cola has the majority market share, or where the cola is under competitive pressure, or where the cola is believed to have significant growth potential. Each area could be a neighborhood in a city, or a part of a state, province, or country.
The following figure shows the areas of interest for four colas.
Figure 2-1 Areas of Interest for the Simple Example
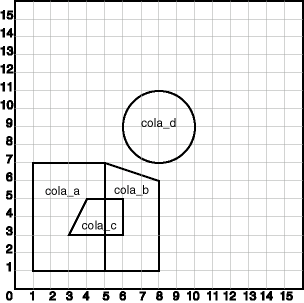
Description of "Figure 2-1 Areas of Interest for the Simple Example"
The example in this topic performs the following operations:
-
Creates a table (COLA_MARKETS) to hold the spatial data
-
Inserts rows for four areas of interest (
cola_a
,cola_b
,cola_c
,cola_d
) -
Updates the USER_SDO_GEOM_METADATA view to reflect the dimensional information for the areas
-
Creates a spatial index (COLA_SPATIAL_IDX)
-
Performs some spatial queries
Many concepts and techniques in the following example are explained in detail in other sections of this chapter.
Example 2-1 Example: Inserting, Indexing, and Querying Spatial Data
-- Create a table for cola (soft drink) markets in a -- given geography (such as city or state). -- Each row will be an area of interest for a specific -- cola (for example, where the cola is most preferred -- by residents, where the manufacturer believes the -- cola has growth potential, and so on). -- (For restrictions on spatial table and column names, see -- TABLE_NAME and COLUMN_NAME.) CREATE TABLE cola_markets ( mkt_id NUMBER PRIMARY KEY, name VARCHAR2(32), shape SDO_GEOMETRY); -- The next INSERT statement creates an area of interest for -- Cola A. This area happens to be a rectangle. -- The area could represent any user-defined criterion: for -- example, where Cola A is the preferred drink, where -- Cola A is under competitive pressure, where Cola A -- has strong growth potential, and so on. INSERT INTO cola_markets VALUES( 1, 'cola_a', SDO_GEOMETRY( 2003, -- two-dimensional polygon NULL, NULL, SDO_ELEM_INFO_ARRAY(1,1003,3), -- one rectangle (1003 = exterior) SDO_ORDINATE_ARRAY(1,1, 5,7) -- only 2 points needed to -- define rectangle (lower left and upper right) with -- Cartesian-coordinate data ) ); -- The next two INSERT statements create areas of interest for -- Cola B and Cola C. These areas are simple polygons (but not -- rectangles). INSERT INTO cola_markets VALUES( 2, 'cola_b', SDO_GEOMETRY( 2003, -- two-dimensional polygon NULL, NULL, SDO_ELEM_INFO_ARRAY(1,1003,1), -- one polygon (exterior polygon ring) SDO_ORDINATE_ARRAY(5,1, 8,1, 8,6, 5,7, 5,1) ) ); INSERT INTO cola_markets VALUES( 3, 'cola_c', SDO_GEOMETRY( 2003, -- two-dimensional polygon NULL, NULL, SDO_ELEM_INFO_ARRAY(1,1003,1), -- one polygon (exterior polygon ring) SDO_ORDINATE_ARRAY(3,3, 6,3, 6,5, 4,5, 3,3) ) ); -- Now insert an area of interest for Cola D. This is a -- circle with a radius of 2. It is completely outside the -- first three areas of interest. INSERT INTO cola_markets VALUES( 4, 'cola_d', SDO_GEOMETRY( 2003, -- two-dimensional polygon NULL, NULL, SDO_ELEM_INFO_ARRAY(1,1003,4), -- one circle SDO_ORDINATE_ARRAY(8,7, 10,9, 8,11) ) ); --------------------------------------------------------------------------- -- UPDATE METADATA VIEW -- --------------------------------------------------------------------------- -- Update the USER_SDO_GEOM_METADATA view. This is required -- before the spatial index can be created. Do this only once for each -- layer (that is, table-column combination; here: COLA_MARKETS and SHAPE). INSERT INTO user_sdo_geom_metadata (TABLE_NAME, COLUMN_NAME, DIMINFO, SRID) VALUES ( 'cola_markets', 'shape', SDO_DIM_ARRAY( -- 20X20 grid SDO_DIM_ELEMENT('X', 0, 20, 0.005), SDO_DIM_ELEMENT('Y', 0, 20, 0.005) ), NULL -- SRID ); ------------------------------------------------------------------- -- CREATE THE SPATIAL INDEX -- ------------------------------------------------------------------- CREATE INDEX cola_spatial_idx ON cola_markets(shape) INDEXTYPE IS MDSYS.SPATIAL_INDEX_V2; -- Preceding statement created an R-tree index. ------------------------------------------------------------------- -- PERFORM SOME SPATIAL QUERIES -- ------------------------------------------------------------------- -- Return the topological intersection of two geometries. SELECT SDO_GEOM.SDO_INTERSECTION(c_a.shape, c_c.shape, 0.005) FROM cola_markets c_a, cola_markets c_c WHERE c_a.name = 'cola_a' AND c_c.name = 'cola_c'; -- Do two geometries have any spatial relationship? SELECT SDO_GEOM.RELATE(c_b.shape, 'anyinteract', c_d.shape, 0.005) FROM cola_markets c_b, cola_markets c_d WHERE c_b.name = 'cola_b' AND c_d.name = 'cola_d'; -- Return the areas of all cola markets. SELECT name, SDO_GEOM.SDO_AREA(shape, 0.005) FROM cola_markets; -- Return the area of just cola_a. SELECT c.name, SDO_GEOM.SDO_AREA(c.shape, 0.005) FROM cola_markets c WHERE c.name = 'cola_a'; -- Return the distance between two geometries. SELECT SDO_GEOM.SDO_DISTANCE(c_b.shape, c_d.shape, 0.005) FROM cola_markets c_b, cola_markets c_d WHERE c_b.name = 'cola_b' AND c_d.name = 'cola_d'; -- Is a geometry valid? SELECT c.name, SDO_GEOM.VALIDATE_GEOMETRY_WITH_CONTEXT(c.shape, 0.005) FROM cola_markets c WHERE c.name = 'cola_c'; -- Is a layer valid? (First, create the results table.) CREATE TABLE val_results (sdo_rowid ROWID, result VARCHAR2(2000)); CALL SDO_GEOM.VALIDATE_LAYER_WITH_CONTEXT('COLA_MARKETS', 'SHAPE', 'VAL_RESULTS', 2); SELECT * from val_results;
Parent topic: Spatial Data Types and Metadata