Prepare the Webhook
To prepare the webhook, you create a webhook channel in Oracle Digital Assistant, and then you create your webhook.
Overview of the Webhook
The webhook is an intermediary between the bot and the live agent system. It allows you to use the built-in system components System.AgentInitiation and System.AgentConversation that were originally designed for use with Oracle Service Cloud.
The webhook resides on a server that you control. It accepts HTTP POST requests from the bot for transmission to the live agent system. It accepts HTTP POST requests from the live agent system for transmission to the bot. And it converts the format of the message payload from one system's format to the other.
The bot sends one of the following types of message:
- A chat request message.
- A chat message.
- A chat terminate message.
The live agent system can send many types of message. Some types of message are intended for the bot user to read, and others are intended for the Digital Assistant system. For example, the live agent system can instruct the bot to transition to a specific state, and it can instruct the bot to add a new utterance to one of its existing intents.
These are the types of message that the Digital Assistant system understands:
- accepted - the human agent is on the line
- delayed - a human agent is not yet available
- rejected - no human agent is available nor is expected to be available
- agent - a message from the human agent to the user
- agentLeft - the human agent has returned control to the bot
- agentAction - the human agent has instructed the bot to either transistion to a specific state or to add an utterance to a specific existing intent
Create the Webhook Channel
Before creating your webhook, you should create a webhook channel in Oracle Digital Assistant.
When you create the channel, Digital Assistant generates a secret key and a URL for its webhook. Your webhook uses this information to access the Digital Assistant webhook.
- In the left navigation menu, under Development click Channels
- When the Channels page opens, click + Channel
- In the Create Channel dialog, enter a channel name. Use the channel name that you specified in the agentChannel field of the System.AgentInitiation component in the BotML for your chatbot skill.
- In the Channel Type field, select Webhook
- In the Outgoing Webhook URI field, enter the URL of your webhook. You can update this value later if you need to.
- Click Create. After the channel is created, further configuration is needed
- In the Route to field, select the Skill that you want to associate with this webhook channel.
- Click the Channel Enabled switch to enable the channel.
- In the Platform Version field, make sure that the version is 1.1(Conversation Model).
- Record the Secret Key and the Webhook URL and save them in a safe place. You'll need these values later when you create your webhook.
Create the Webhook
The webhook that you create manages the data flow between the chatbot and the live agent system. It makes it possible for chatbot users to chat directly with human agents.
The following project directory layout shows a minimal implementation of the webhook.
webhook
config
config.js
src
server.js
package.json
Conceptually, it's possible to have all functionality in server.js
, but you'll probably want to separate the tasks out into their own modules.
The following diagram shows a possible architecture of the webhook code.
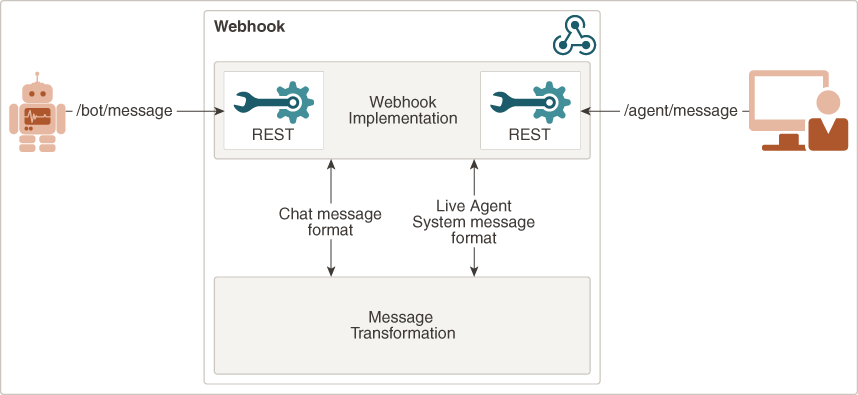
Description of the illustration webhook-architecture.png
The initial request to talk to an agent comes from the chatbot via an HTTP POST request to the /bot/message
endpoint of your webhook. The chatbot obtains this URL from the Outgoing Webhook URI field in the Webhook Channel that you set up in the ODA interface.
Your webhook then transforms the message payload from the format that the chatbot uses into a format that the live agent system can use. After the format is transformed, the webhook sends it to the live agent system's endpoint.
The agent system responds to the chatbot by sending an HTTP POST request to the /agent/message
endpoint of your webhook. Your webhook transforms the agent system format into the chatbot format, and then sends it to the chatbot. Your webhook needs the chatbot's secret key and webhook URL for this.
To allow handling more than one user, you must keep track of user info. The info should include the conversation history, the user id, channel name, and channel id.
Receive Message from Chatbot
When your webhook receives a message from the chatbot, it must determine the type of message and act accordingly.
The chatbot sends a JSON payload. The type field of the message payload contains one of the following strings:
- "agentRequest"
- "botConversationEnded"
- "botTextMessagePayload"
The following outline describes what your webhook must accomplish for each type:
- if "agentRequest" then
- store user info and web channel info which includes
user ID, user channel ID, channel name, and webchannel ID
- construct payload for live agent system
- POST chat request with payload to live agent system
- if "botTextMessagePayload" then
- construct payload for live agent system
- POST chat message to live agent system
- if "botConversationEnded" then
- construct payload for live agent system
- POST terminate chat request to live agent system
- delete user info
You must also consider the task of authenticating with the live agent system.
How you organize your code is up to you. You can refer to the sample code on GitHub for one method of doing that.
Note that the sample code uses an in-memory cache to store the user info. This is a convenience for the purposes of demonstration. In a production application you should use a more robust method, such as a database system.
Receive Message from Agent
When your webhook receives a message from the live agent system, it must determine the type of message and act accordingly.
Whatever format that your webhook receives from the live agent system, it must convert that format into the JSON that the chatbot system requires. For example, the format of the chat accepted message looks like the following JSON:
{
"type": "accepted",
"payload": {
"message": "Hello my name is Agent, how can I help you?",
"sessionId": "123456",
"botUser": {
"userId": "3246969"
}
}
}
Refer to the code sample README on GitHub for descriptions of the other message types.
The following outline describes what your webhook must accomplish for each type:
if "accepted" then
- get user info from storage
- generate payload
- POST payload to ODA
if "delayed" then
- transform payload
- POST payload to ODA
if "rejected" then
- transform payload
- POST payload to ODA
if "agent" then
- transform payload
- POST payload to ODA
if "agentLeft" then
- transform payload
- POST payload to ODA
if "agentAction" then
- transform payload
- POST payload to ODA // includes either a state for ODA to switch to,
// or a new utterance for an existing intent
How you organize your code is up to you. You can refer to the sample code on GitHub for one method of doing that.