Adding HTML Content to JavaFX Applications
Beta Draft: 2013-09-17
4 Adding a WebView Component to the Application Scene
This chapter introduces the WebViewSample application and explains how to implement the task of adding a WebView
component to the scene of a JavaFX application.
The WebViewSample application creates the Browser
class that encapsulates both the WebView
object and the toolbar with UI controls. The WebViewSample
class of the application creates the scene and adds a Browser
object to the scene.
Creating an Embedded Browser
Example 4-1 shows how to add the WebView
component to the application scene.
Example 4-1 Creating a Browser by Using the WebView and WebEngine Classes
import javafx.application.Application; import javafx.geometry.HPos; import javafx.geometry.VPos; import javafx.scene.Node; import javafx.scene.Scene; import javafx.scene.layout.HBox; import javafx.scene.layout.Priority; import javafx.scene.layout.Region; import javafx.scene.paint.Color; import javafx.scene.web.WebEngine; import javafx.scene.web.WebView; import javafx.stage.Stage; public class WebViewSample extends Application { private Scene scene; @Override public void start(Stage stage) { // create the scene stage.setTitle("Web View"); scene = new Scene(new Browser(),900,600, Color.web("#666970")); stage.setScene(scene); scene.getStylesheets().add("webviewsample/BrowserToolbar.css"); stage.show(); } public static void main(String[] args){ launch(args); } } class Browser extends Region { final WebView browser = new WebView(); final WebEngine webEngine = browser.getEngine(); public Browser() { //apply the styles getStyleClass().add("browser"); // load the web page webEngine.load("http://www.oracle.com/products/index.html"); //add the web view to the scene getChildren().add(browser); } private Node createSpacer() { Region spacer = new Region(); HBox.setHgrow(spacer, Priority.ALWAYS); return spacer; } @Override protected void layoutChildren() { double w = getWidth(); double h = getHeight(); layoutInArea(browser,0,0,w,h,0, HPos.CENTER, VPos.CENTER); } @Override protected double computePrefWidth(double height) { return 900; } @Override protected double computePrefHeight(double width) { return 600; } }
In this code, the web engine loads a URL that points to the Oracle corporate web site. The WebView
object that contains this web engine is added to the application scene by using the getChildren
and add
methods.
When you add, compile, and run this code fragment, it produces the application window shown in Figure 4-1.
Figure 4-1 WebView Object in an Application Scene
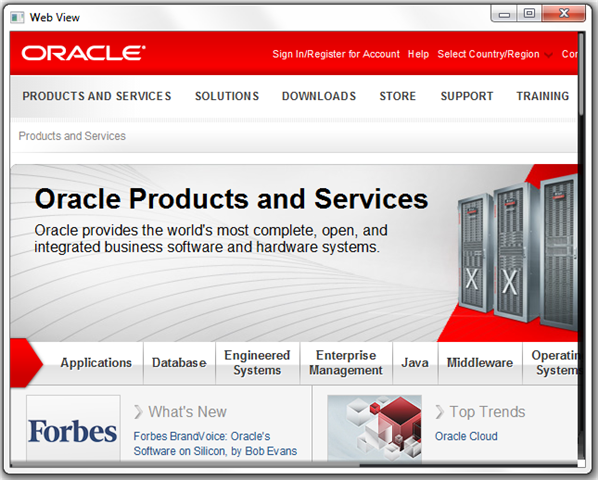
Description of "Figure 4-1 WebView Object in an Application Scene "
Creating an Application Toolbar
Add a toolbar with four Hyperlink
objects to switch between different Oracle web resources. Study the modified code of the Browser
class shown in Example 4-2. It adds URLs for alternative web resources including Oracle products, blogs, Java documentation, and the partner network. The code fragment also creates a toolbar and adds the hyperlinks to it.
Example 4-2 Creating a Toolbar
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.HPos; import javafx.geometry.VPos; import javafx.scene.Node; import javafx.scene.Scene; import javafx.scene.control.Hyperlink; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.layout.HBox; import javafx.scene.layout.Priority; import javafx.scene.layout.Region; import javafx.scene.paint.Color; import javafx.scene.web.WebEngine; import javafx.scene.web.WebView; import javafx.stage.Stage; public class WebViewSample extends Application { private Scene scene; @Override public void start(Stage stage) { // create scene stage.setTitle("Web View"); scene = new Scene(new Browser(),900,600, Color.web("#666970")); stage.setScene(scene); scene.getStylesheets().add("webviewsample/BrowserToolbar.css"); // show stage stage.show(); } public static void main(String[] args){ launch(args); } } class Browser extends Region { private HBox toolBar; final private static String[] imageFiles = new String[]{ "product.png", "blog.png", "documentation.png", "partners.png" }; final private static String[] captions = new String[]{ "Products", "Blogs", "Documentation", "Partners" }; final private static String[] urls = new String[]{ "http://www.oracle.com/products/index.html", "http://blogs.oracle.com/", "http://docs.oracle.com/javase/index.html", "http://www.oracle.com/partners/index.html" }; final ImageView selectedImage = new ImageView(); final Hyperlink[] hpls = new Hyperlink[captions.length]; final Image[] images = new Image[imageFiles.length]; final WebView browser = new WebView(); final WebEngine webEngine = browser.getEngine(); public Browser() { //apply the styles getStyleClass().add("browser"); for (int i = 0; i < captions.length; i++) { final Hyperlink hpl = hpls[i] = new Hyperlink(captions[i]); Image image = images[i] = new Image(getClass().getResourceAsStream(imageFiles[i])); hpl.setGraphic(new ImageView (image)); final String url = urls[i]; hpl.setOnAction(new EventHandler<ActionEvent>() { @Override public void handle(ActionEvent e) { webEngine.load(url); } }); } // load the home page webEngine.load("http://www.oracle.com/products/index.html"); // create the toolbar toolBar = new HBox(); toolBar.getStyleClass().add("browser-toolbar"); toolBar.getChildren().addAll(hpls); //add components getChildren().add(toolBar); getChildren().add(browser); } private Node createSpacer() { Region spacer = new Region(); HBox.setHgrow(spacer, Priority.ALWAYS); return spacer; } @Override protected void layoutChildren() { double w = getWidth(); double h = getHeight(); double tbHeight = toolBar.prefHeight(w); layoutInArea(browser,0,0,w,h-tbHeight,0, HPos.CENTER, VPos.CENTER); layoutInArea(toolBar,0,h-tbHeight,w,tbHeight,0,HPos.CENTER,VPos.CENTER); } @Override protected double computePrefWidth(double height) { return 900; } @Override protected double computePrefHeight(double width) { return 600; } }
This code uses a for
loop to create the hyperlinks. The setOnAction
method defines the behavior of the hyperlinks. When a user clicks a link, the corresponding URL value is passed to the load
method of the webEngine
. When you compile and run the modified application, the application window changes as shown in Figure 4-2.
Figure 4-2 Embedded Browser with the Navigation Toolbar
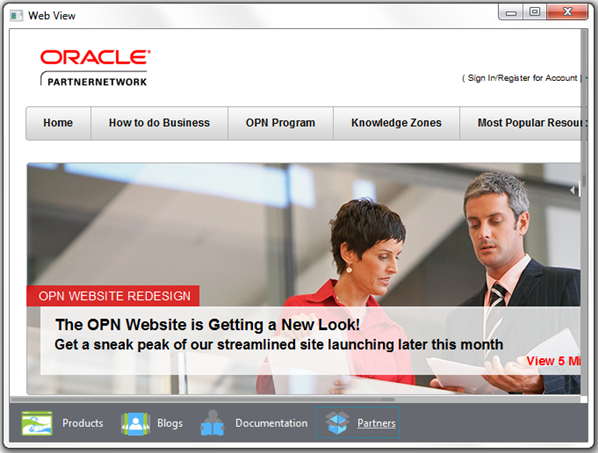
Description of "Figure 4-2 Embedded Browser with the Navigation Toolbar"