Using WebLogic Enterprise JavaBeans
- I. Introduction
- Overview of WebLogic Enterprise JavaBeans (EJB)
- Introduction to Enterprise JavaBeans
- II. The API
- WebLogic EJB API reference
- III. Implementing with WebLogic EJB
- Developing an Enterprise JavaBean
- Deploying Enterprise JavaBeans in WebLogic
- EJB and persistence
- EJB and finders
- EJB and transactions
- Pooling and instantiating EJBeans on the server
- EJB and JDBC
- EJB and security
- Retrieving EJBean properties at runtime
- WebLogic EJB utilities
- ejbc
- DDCreator
- ComplianceChecker
- EJB Deployment Wizard
- IV. Change history
- Other related documents
- Installing WebLogic (non-Windows)
- Installing WebLogic (Windows)
- Overview of WebLogic Enterprise JavaBeans
- Designing a WebLogic EJB application
- Using the WebLogic EJB
Deployment Wizard
- Troubleshooting your WebLogic EJB application
- Writing a T3Client application
- Code examples
- Glossary
The
icons link to the glossary entry for the word preceding the icon.
I. Introduction
Overview of WebLogic Enterprise JavaBeans
WebLogic Enterprise JavaBeans (EJB) is one of the services that
operate within WebLogic .
As a service, it has access to all of the
WebLogic facilities (like logging, instrumentation, and Workspaces), and
like other WebLogic component-based services, it is an integrated part
of WebLogic. The APIs for all of the WebLogic services and facilities share
many common aspects that make building a complex networked application
easier; your application may use several services, all of which
can share access to objects and client resources. EJB offers another
design choice -- along with
JavaServer Pages (JSP),
Java Message Service (JMS),
Remote Method Invocation (RMI) ,
Java Naming and Directory Interface (JNDI) ,
WebLogic Events and
WebLogic Beans
-- for building distributed applications in WebLogic.
This document covers aspects specific to writing applications with
the EJB API. You should also read and be familiar with:
WebLogic Enterprise JavaBeans (EJB) is an implementation of the
Enterprise JavaBeans specification (version 1.0) from
JavaSoft and supports these EJB features:
- Entity beans (with either container- or self-managed persistence
)
- Session beans (either stateful or stateless)
- JTS
implementation for distributed transactions
- Support for client-demarcated transactions
Introduction to EJB
What are Enterprise JavaBeans?
The JavaSoft Enterprise JavaBeans (EJB) specification defines a
component architecture for building distributed, object-oriented
business applications in Java. The EJB architecture addresses the
development, deployment, and runtime aspects of an enterprise
application's lifecycle.
An Enterprise JavaBean (EJBean) encapsulates business logic
inside a component framework that manages the details of security,
transaction, and state management. Low-level details such as
multithreading ,
resource pooling, clustering, distributed naming,
automatic persistence, remote invocation, transaction boundary
management, and distributed transaction management are
handled by the EJB "container." This built-in low-level support allows
the EJB developer to focus on the business problem to be solved.
An Enterprise JavaBean is, in essence, a transactional and secure
RMI
(or CORBA )
object, some of whose runtime properties are specified
at deployment time using special classes called "deployment
descriptors."
With the EJB model, you can write or buy
business components (such as invoices, bank accounts and shipping
routes) and, during deployment into a certain project, specify how the
component should be used -- which users have access to which methods,
whether the framework should automatically start a transaction or
whether it should inherit the caller's transaction, and so on.
In this scenario, an EJBean contains the business logic (methods)
and the customization needed for a particular application (deployment
descriptor), and the EJBean will run within any standard
implementation of the EJB framework. The spirit of "write once,
run anywhere" carries through into EJB: any vendor's EJB
framework can run any third-party EJBeans to create an
application. Nuances of the security mechanisms and specific
distributed transaction monitors are entirely abstracted out of the
application code (unless the bean author chooses to make such calls
explicitly).
II. The WebLogic EJB API
WebLogic EJB API Reference
The EJB API reference is available via ftp from JavaSoft in the
Enterprise JavaBeans specification (version 1.0).
There are three packages shipped as part of WebLogic EJB:
- Package javax.ejb
- Package javax.ejb.deployment
- Package javax.jts
WebLogic's implementation includes these utilities:
Note:
- WebLogic EJB uses WebLogic's own RMI implementation in EJB.
We import
java.rmi.RemoteException in our EJB examples only because
javax.ejb.* requires it.
See Using WebLogic RMI
for additional information on WebLogic's RMI implementation.
- WebLogic EJB includes these enhancements in addition to the specification:
III. Implementing with WebLogic EJB
Before starting to write your own Enterprise JavaBeans, we highly
recommend that you go through all of the
example EJBeans
included in the WebLogic distribution, compiling, installing and running them.
There are three parts to using WebLogic EJB:
- Develop an EJBean or obtain one from a third-party supplier.
- Deploy the EJBean. Adjust its deployment descriptor as required
for your particular installation, and edit the
weblogic.properties file
for your WebLogic Server so that the EJBean is loaded at startup.
- Write an application that uses the bean.
In this section, we'll cover the basics of developing, deploying
and accessing EJBeans, along these topics:
- Developing an Enterprise JavaBean
- Deploying Enterprise JavaBeans in WebLogic
- EJB and persistence
- EJB and finders
- EJB and transactions
- EJB and JDBC
- Setting up a server-side connection pool for EJB
- Configuring the pool in the weblogic.properties file
- Using the pool in your EJBean
- Retrieving EJBean properties at runtime
- WebLogic EJB utilities
Developing an Enterprise JavaBean
Here are the steps to developing an EJBean:
- Write or obtain an EJBean
- Create a serialized Deployment Descriptor
- Package the EJB components into a .jar file. (optional)
You can then deploy and use the EJBean.
Step 1. Write or obtain an EJBean
The EJB specification outlines
the different responsibilities of the EJBean writer and EJB framework,
and WebLogic EJB expects EJBeans to conform to those responsibilities.
In writing your own EJBeans, pay careful attention to these responsibilities.
Example EJBeans
are included in the WebLogic distribution to demonstrate these relationships.
A utility called
ComplianceChecker
is provided with WebLogic EJB to examine
packaged EJBeans and determine if they follow specified relationships.
Step 2. Create a serialized Deployment Descriptor
The Deployment Descriptor ties together the different classes and
interfaces, and is used to build the code-generated class files.
It also allows you to specify some aspects of the EJBean's deployment at runtime.
The Deployment Descriptor is an instance of either a session
descriptor or an entity descriptor, serialized and stored in a
file. To facilitate the preparation of this file, WebLogic EJB includes
a utility application
DDCreator
that takes a
text file specification and
creates the appropriate serialized deployment descriptor.
Step 3. Package the EJBean components into a .jar file (optional)
This is optional because if you are going to immediately deploy the EJBean, you
can skip this step because you will need to do it again
at the end of the deployment process.
However, if you are going to send the EJBean to someone else for deployment, you can
package the output of the previous steps into a .jar file and send it to them
for deployment.
Deploying Enterprise JavaBeans in WebLogic
Here are the steps to deploying an EJBean, assuming you have completed the steps of the
previous section:
- Set your CLASSPATH for your development
- Adjust the serialized Deployment Descriptor (optional)
- Generate the EJB container classes using ejbc
- Package the EJB components into a .jar file
- Deploy the EJBean in a WebLogic Server
If you have completed the development process and created a .jar file, you can use the
WebLogic EJB Deployment Wizard to perform all of these steps.
You can then use the EJBean.
Step 1. Set your CLASSPATH for your development
We cover this in our TechStart Guide Setting your development environment.
Step 2. Adjust the serialized Deployment Descriptor (optional)
Check the deployment descriptor and modify any of its properties
for your particular deployment (if required). To do so, you can either use the
WebLogic EJB Deployment Wizard
or look at the text file
that was used to generate the deployment descriptor.
If you change any of the properties, you will need to create a new serialized
deployment descriptor to replace the existing one packaged with the bean.
Step 3. Generate the EJB container classes using ejbc
Generate the wrapper classes using the WebLogic EJB compiler
(ejbc)
with this command (typed on one line), referencing the serialized deployment descriptor:
$ java weblogic.ejbc -d /weblogic/myserver/temp AccountBeanDD.ser
or, if using a .jar file (type this command on one line):
$ java weblogic.ejbc -d /weblogic/myserver/temp AccountBean.jar
This will create the appropriate files for the bean, and place them in a
temporary directory, in this case
/weblogic/myserver/temp.
(The classes will have been passed through the RMI compiler automatically.)
Step 4. Package the EJB components into a .jar file.
Package the outputs of the previous steps -- the serialized Deployment Descriptor,
the compiled files for the EJBean classes, all generated classes, and
any additional required classes -- into a .jar file, using the Jar tool included in
your Java JDK.
The Enterprise JavaBeans specification (version 1.0) from JavaSoft
describes in section 15.3: ejb-jar Manifest (page 116) how to construct
the manifest file to be included in the .jar file. Sample
manifests are included with each of the
example EJBeans.
A manifest file points to the serialized deployment descriptors that
describes the EJBeans in the .jar file.
Multiple beans may be packaged together, provided that there is a deployment
descriptor for each one. It's a good idea to ship the text file used to create
the deployment descriptor, in the event that at deployment,
properties in the deployment descriptor need alteration.
If there are multiple beans packaged together, there is a single manifest file that lists
all the serialized deployment descriptors that are found in the .jar file.
Note: If you are upgrading from an earlier version of WebLogic, you will need to
re-run ejbc on any existing EJBeans to regenerate
the wrapper classes so that they're compatible with the WebLogic Server version.
The .jar file should be placed in a directory that's not on the CLASSPATH of your WebLogic Server.
(Our examples use the /myserver
directory for placing EJBeans.)
Step 5. Deploy the EJBean in a WebLogic Server
Deploy the EJBean either by:
- Adding the path of the .jar file to the
weblogic.ejb.deploy property of the
weblogic.properties file of the WebLogic Server:
weblogic.ejb.deploy=c:/weblogic/classes/AccountBean.jar
and then (re)starting the Server.
Additional bean classes can be added to the property as a comma-delimited list, referencing the .jar files.
- Using the hot deploy feature of WebLogic Server. This method enables you to deploy an EJBean into a running server. However, EJBeans that are hot deployed are not automatically deployed when you next boot WebLogic Server. If you require that the EJBean be deployed automatically, edit the weblogic.properties file.
You can then use the EJBean.
Note: Beginning with version 4.5.1, deploying an EJB using the
.ser file in the
weblogic.ejb.deploy property has been deprecated.
Use .jars instead, even when using the Microsoft JDK for Java.
If you are using EJBeans and the Microsoft SDK for Java, you will need to:
- Download and install a Java SDK from Sun to provide access to a jar tool
- As we have deprecated support for .ser files for deploying EJBeans, use the
jar tool to package your EJBeans into .jar file for deployment as shown in our Example Guide
Building Enterprise JavaBean examples
- You will need to add the path Java SDK bin and classes.zip
to your PATH and classpath respectively for the jar tool to work
- Add the EJBean .jar files that are being deployed to the classpath of the WebLogic Server
before you start the Server. You cannot use the hot deploy feature with Microsoft SDK for Java.
EJB and persistence
An entity EJBean can save its state in any transactional or
non-transactional persistent storage, or it can ask the framework to
save its non-transient instance variables automatically. WebLogic EJB
allows both choices -- even a mixture of the two.
You can either manually control persistence, or use the automatic persistence
available for entity beans.
Persistence is controlled by specifying one or more environment properties
in the deployment descriptor:
- containerManagedFields
This is a standard property which lists the public non-transient instance variables
that the EJBean expects to be made automatically persistent.
Even if there are no managed fields, the bean's object reference and the primary
key are remembered by the framework.
- persistentStoreType (such as "jdbc")
Note: File persistence has been deprecated as of WebLogic version 4.5.1.
It will be removed in a later version of WebLogic.
If the persistentStoreType is "file",
all instances of an EJBean are stored in a single file
under the persistentDirectoryRoot
with the name of the EJBean converted to a directory name. This defaults
to a directory "pstore" in the directory where WebLogic was started.
File persistence is intended only for development, prototyping
and building demonstration applications; because it lacks transactional
integrity, it is not supported for production environments. For that, you
need to use a database and JDBC persistence.
(Stateful session beans are stored using file persistence when they are
passivated, in a location specified by the
persistentDirectoryRoot property.
See
Using
the WebLogic EJB Deployment Wizard: Session-specific properties.)
- persistentDirectoryRoot (file persistence)
Note: File persistence has been deprecated as of WebLogic version 4.5.1.
It will be removed in a later version of WebLogic.
If the persistentStoreType
is "file", the serialized files
are created in this directory. The default file is "/pstore/example_bean.dat",
where the directory "pstore" is located in the directory
which the WebLogic Server was launched, and example_bean
is the fully-qualified name of the EJBean with underscores ("_") replacing the
periods (".") in the name.
For example, if the persistentDirectoryRoot
is "c:\weblogic\mystore", all instances of the EJBean
"examples.ejb.AccountBean" will be stored in a single file
"c:\weblogic\mystore\examples_ejb_AccountBean.dat".
If the persistentStoreType
is "jdbc", the
container looks for the "tableName", "poolName", and "attributeMap" properties:
- tableName (jdbc persistence)
The name of the table; it defaults to the bean's classname.
- poolname (jdbc persistence)
The connection pool used for database persistence. Alternatively, you may
specify the JDBC driver URL and driver name.
- attributeMap (jdbc persistence)
This is a hashtable which contains the name of the database
column for each container-managed attribute.
No automatic type conversion
between types is performed on primary key columns, so the mapping
between these columns must be between equivalent types.
- isModifiedMethodName (environment properties)
By default, WebLogic Server calls ejbStore() for a container-managed entity EJBean at
the successful completion (commit) of each transaction. ejbStore() is called at commit time
regardless of whether or not the EJBean's persistent fields were
actually updated. WebLogic Server provides the
isModifiedMethodName
deployment property for cases
where unnecessary calls to ejbStore() may result in poor performance.
To use isModifiedMethodName,
the EJBean developer must first create an EJB method that "cues"
WebLogic Server when persistent data has been updated. The method must
return "false" to indicate that no EJB fields were updated, or "true"
to indicate that some fields were modified.
The EJBean deployer then identifies the name of this method using the
isModifiedMethodName
property. WebLogic Server calls the specified method name when a
transaction commits, and calls ejbStore() only if the method returns "true."
Note: isModifiedMethodName can improve performance by
avoiding unnecessary calls to ejbStore(). However, it places a greater burden on the
EJBean developer to correctly identify when updates have occurred. If the
specified isModifiedMethodName
returns an incorrect flag to WebLogic Server, data integrity problems
can occur, and they may be difficult to track down.
If container-managed entity EJBean updates appear to become "lost" in
your system, start by ensuring that all isModifiedMethodName methods return "true" under every
circumstance. In this way, you can revert to WebLogic Server's default
ejbStore() behavior and
possibly correct the problem.
This fragment from a deployment descriptor specifies
automatic saving using file persistence of an EJBean to a file in a directory "c:\myobjects":
(environmentProperties
(persistentStoreProperties
persistentStoreType file
persistentDirectoryRoot c:\myobjects
); end persistentStoreProperties
); end environmentProperties
This fragment from a deployment descriptor specifies
automatic saving using jdbc persistence of two attributes of an EJBean
("accountId", "balance") to a database table ("ejbAccounts")
using a connection pool ("ejbPool"):
(environmentProperties
(persistentStoreProperties
persistentStoreType jdbc
(jdbc
tableName ejbAccounts
; Ensure that table "ejbAccounts" is
; already created in the database using
; an appropriate SQL statement such as:
; "create table ejbAccounts (id varchar(15), bal float, type varchar(15))"
; Otherwise, a run-time exception will be thrown.
dbIsShared false
; Either "true" or "false".
; If this is true, caching is turned off, because
; WebLogic knows it doesn't "own" the table.
poolName ejbPool
(attributeMap
; Maps the EJBean fields to database column names
; EJBean fields Database column name
; -----------------------------------------
accountId id
balance bal
); end attributeMap
); end jdbc
); end persistentStoreProperties
); end environmentProperties
Note that the connection pool must be established in the
weblogic.properties file,
and that the table and columns must already
exist in the database before the EJBean is called.
EJB and finders
If you are using container-managed JDBC persistence, you can specify
finder methods of the form findMethod()
to find either an individual or collection of EJBeans.
If you need to do complicated SQL lookups -- such a dynamically set 'where' clause --
you will not be able to do that with the current syntax provided for
container-managed persistence. Instead, you will need to use bean-managed persistence,
which allows you to write custom finders.
You specify a method signature in the EJBHome interface for the EJBean and
specify the method's expressions in the deployment descriptor using a custom syntax.
You use method parameters and EJBean attributes in the expressions; the EJB container
will automatically map the attributes to the appropriate columns in the persistent store.
The EJBean compiler follows the syntax to generate code that automatically does the lookup
in the JDBC persistent store.
Here is an interface for the
findAccount method
from the container-managed JDBC persistence
example:
public interface AccountHome extends EJBHome {
// ...
public Account findAccount(double balanceEqual)
throws FinderException, RemoteException;
// ...
}
Here is an interface for the
findBigAccounts method
from the container-managed JDBC persistence
example:
public interface AccountHome extends EJBHome {
// ...
public Enumeration findBigAccounts(double balanceGreaterThan)
throws FinderException, RemoteException;
// ...
}
The expression defined in the deployment descriptor for this finder could be:
(> balance $balanceGreaterThan)
where balance is an attribute (field) of the EJBean and
$balanceGreaterThan is the symbol for the method parameter
balanceGreaterThan.
A call in the client such as
myEJBean.findBigAccounts(amount) will return an list
of all EJBeans whose balance attribute is greater than
the value of amount.
Details of using finders are described in Using the WebLogic EJB
Deployment Wizard: JDBC Finders (finderDescriptors).
An example using a finder is the
containerManaged
example.
EJB and transactions
Note that in all cases, transactions must be restricted to
a single persistent store. You can't, for instance, mix
JDBC persistence from two different sources in the same transaction, because
JDBC 1.2 does not support the 2-phase commit protocol.
A future version of JDBC may support this.
Transaction isolation level
The isolation level for transactions set in the control descriptor
property of the
Deployment Descriptor
is passed to the
underlying database. The behavior of an EJBean depends on
the isolation level passed and the concurrency control of the
underlying persistent store.
If you would like the behavior of previous versions of WebLogic EJB, you
should use an isolation level consistent with the default isolation level
for your installation. You should consult with your DBMS administrator for
details.
You should write your client following
standard design principles for client-server systems, avoiding
long updates and locking rows indefinitely.
Special note for Oracle databases
In particular, you should be aware that Oracle uses optimistic
concurrency. As a consequence, even with a setting of
TRANSACTION_SERIALIZABLE,
it does not detect serialization
problems until commit time. The message returned is:
java.sql.SQLException: ORA-08177: can't serialize access for this transaction
Even if you use TRANSACTION_SERIALIZABLE,
you will get exceptions and rollbacks in the client if contention occurs between
clients for the same rows. You will need to
write your client to catch any SQL exceptions that occur, examine them and
take appropriate action, such as attempting the transaction again.
Multiple EJBeans in a single manual transaction
In this first code fragment, a client calls two different beans, and
shows what is required to begin and commit a transaction.
The transactionAttribute
property in the bean's deployment descriptor has been set
to TX_REQUIRED:
import javax.ejb.*;
import javax.naming.*;
import javax.jts.*;
// To begin a transaction,
// get a UserTransaction object from JNDI
UserTransaction u = null;
// First, get initial jndi context
jndiContext = .... ;
// Then, get a reference to a UserTransaction object
u = (UserTransaction)
jndiContext.lookup("javax.jts.UserTransaction");
u.begin();
// make EJB calls
account1.withdraw(100);
account2.deposit(100);
u.commit(); // or u.rollback.
To the client, the transaction is bracketed across two separate calls
to two different EJ beans.
Multiple EJBeans in automatic transactions
In this second code fragment, we'll let WebLogic EJB generate
transactions. The transactionAttribute
property in the bean's deployment descriptor can be set
to either TX_REQUIRED or
TX_REQUIRES_NEW:
// make EJB calls
account1.withdraw(100);
account2.deposit(100);
In this case, each method call is automatically in its own transaction.
Encapsulating a multi-operation transaction
A third possibility is to use a "wrapper" EJBean that encapsulates a
transaction. The client would call the wrapper EJBean to perform an action
such as a bank transfer. The wrapper EJBean would respond by starting a new
transaction that depended on the successful completion of both an
increment of one account and the decrement of another before committing.
To the client, this would appear as a single operation, conducted as a
single transaction; to the wrapper EJBean, it would appear as two separate
operations that are bracketed by a transaction.
This would be a matter of taking the first case above and turning it into a session EJBean.
Further information on transactions can be found in the packages
javax.jts
and
javax.ejb.deployment.
Pooling and instantiating EJBeans on the server
WebLogic EJB uses both a free pool and a cache of bean instances to improve performance and
throughput.
Free pool
The free pool contains instantiated (but uninitialized or referenced) instances
of a bean class. Having beans in the free pool allows the Server to quickly allocate a bean with
values when required instead of having to both create the object and set its attributes.
The pool size starts at zero and grows as needed up to the maximum size specified in
the deployment descriptor by the
maxBeansInFreePool
property.
EJBean cache
Referenced EJBeans are stored in a cache.
The size of the cache is set by the
maxBeansInCache
property which is the maximum number of objects
(active plus inactive) of this class that are allowed in memory.
Active objects are beans that are in use by a client in a transaction;
inactive objects are beans that are either not in a transaction
nor currently engaged in a method call, but have not yet timed out.
Once a bean has timed out, it may be passivated.
The timeout is based on the
idleTimeoutSeconds
setting in the deployment descriptor.
If the maxBeansInCache limit is reached, and all beans are active,
the next call for a new bean will get a CacheFullException.
If some beans are inactive,
they may be passivated before the idle time out is reached in order to fulfill
the request for a new bean.
For stateful session beans and entity EJBeans
When the server starts, the pool is empty.
When clients lookup and obtain a reference to an EJBean,
new instances are created, but with only one instance per primary key.
(Stateful session beans have an implicit primary key.)
This means:
- If two clients are accessing the same EJBean using the same primary key
(as is the case when more than one client uses the serialized handle to access
the same entity EJBean), they are both using the same instance of the EJBean.
We implement the pessimistic concurrency model
- If two clients are accessing the same EJBean but with different
primary keys, there are two separate instances of the EJBean, each bound to its
primary key.
- When all the references to an EJB are released, the EJBean
instance is not deleted, but is unbound from its primary key and is saved
in the free pool of available EJBean instances for that class.
For stateless session beans
For stateless session beans there are, of course, no primary keys. The EJBean
is returned to the pool as soon as the invocation is complete -- not when the
references are freed. Hence each client accesses its own instance of the EJBean
and the instance may be different from invocation to invocation.
EJB and JDBC
Setting up a server-side connection pool for EJB
When writing EJBeans that use bean-managed persistence and JDBC,
you will need to be familiar with WebLogic JDBC
server-side connection pools. WebLogic's JDBC pool drivers give
server-side Java programs, including EJBeans, access to connection
pools on the WebLogic Server.
Using a connection pool means that
databases can be changed by editing entries in the WebLogic properties
file and that you do not need to know in advance which database your
EJB will use. The driver only works with server-side Java classes
running on the same WebLogic Server with which the connection pool was
registered.
A WebLogic JDBC connection pool provides a pool of JDBC connections that
are created when the WebLogic Server is started up. Then, when an
authorized user needs a connection to a database, the user requests
and uses an already existing connection from the pool, rather than
creating a direct, specific connection to the database.
You can configure a pool to set:
- the number of connections in the pool
- how fast it grows incrementally when demand increases and shrinks as
demand decreases
- which users can borrow connections from the pool
When a user calls close()
on a pool connection, it is merely returned to
the pool. Connection pools, because they're efficient and
configurable, provide performance benefits, and they also allow you to
manage database connections within your application.
For more information on connection pools, read Using connection pools in the
WebLogic JDBC Developers Guide.
WebLogic has special server-side pool drivers that allow you to set up
connection pools for objects that operate within WebLogic, rather than
within a WebLogic client. One of the server-side pool drivers is a
JTS-based driver that understands how to handle EJB-related
requests. Because EJBeans are essentially contained within the WebLogic
Server's VM ,
you do not need a multitier connection.
There are several pieces of information that you will use, either in
your source code or in the deployment descriptor, and in the
weblogic.properties file. You will
need to know:
- Name of the connection pool. You can name the connection pool
anything you want. The pool name is part of the pool registration and
the access control list that you set up in the
weblogic.properties file. You will also use
the pool name in your source code, or, optionally, in the deployment
descriptor, from which you can retrieve the information for use in
your EJBean.
- The URL
and package name of the two-tier driver that you will
use to make the JDBC connection between the WebLogic Server and your
database. For example, if you are using an Oracle database with the
jdbcKona/Oracle
two-tier driver, the URL is "jdbc:weblogic:oracle" and
the package name is "weblogic.jdbc.oci.Driver." This information goes
in connection pool registration in the
weblogic.properties file. Note that the URL is
colon-delimited and the package name is period-delimited.
- Username and password for a valid use for the
database. This information is part of the connection pool registration
in the weblogic.properties
file.
- Other database-related or transaction-related information, such as
the name of the table, column names, row data, etc, you will use for EJB
data. You can identify such information directly in your source code,
or optionally, in the deployment descriptor for the EJBean, where you
can retrieve the information for use in your EJBean.
Configuring the pool in the weblogic.properties file
Here is an example of a registration that sets up a connection pool
named "ejbPool" for a Cloudscape database. (This pool registration
is shipped commented out in the properties file packaged with
the distribution.) To use this pool, just change the database-
and user-specific information to match your environment.
weblogic.jdbc.connectionPool.ejbPool=\
url=jdbc:cloudscape:ejbdemo,\
driver=COM.cloudscape.core.JDBCDriver,\
loginDelaySecs=1,\
initialCapacity=2,\
maxCapacity=2,\
capacityIncrement=1,\
props=user=none;password=none;server=none
weblogic.allow.reserve.weblogic.jdbc.connectionPool.ejbPool=\
guest,joe,jill
The "weblogic.allow" entry is the access control list for the
"ejbPool" connection pool. It lists those T3Users that are allowed
access to the connections in the pool. Each user in the list should be
registered with a password in the properties file. Read more about
setting up usernames, passwords, and access control lists in the
WebLogic Administrator Guide document, Setting WebLogic properties.
Using the pool in your EJBean
In your EJBean's source code, or optionally in the
deployment descriptor where you can use a named environment property
to retrieve the information, you will set up the JTS-pool-related
information, the name of the table for EJB data, and optionally other
database-related information.
Here is an example of how you might use a connection pool in your
EJBean. Here we have hard-coded the driver URL ("jdbc:weblogic:jts")
and package name ("weblogic.jdbc.jts.Driver") as well as the table
name ("tradingorders"), directly into the source code.
We have set up environment properties in the deployment descriptor
for the "jdbc" properties, which includes a sub-property "poolName"
set as "ejbPool", the name of a connection pool we have
set up in the weblogic.properties file.
We pass the pool name as
part of the driver URL ("jdbc:weblogic:jts:poolName").
You can place all such information directly in the source, or make it
all environment variables, depending on your application.
Class.forName("weblogic.jdbc.jts.Driver").newInstance();
Connection con =
DriverManager.getConnection("jdbc:weblogic:jts:ejbPool");
Statement stmt = con.createStatement();
str = "insert into tradingorders " +
"(traderName, account, stockSymbol, shares, price)"
+ " VALUES (" +
"'" + traderName + "'" + "," +
"'" + customerName + "'" + "," +
"'" + stockSymbol + "'" + "," +
shares + "," +
price + ")";
stmt.executeUpdate(str);
For more on using server-side connection pools, read the Developers
Guide Using WebLogic HTTP Servlets:
Using connection pools with server-side Java.
Using EJB with the JDBC-ODBC bridge
Using the JDBC-ODBC bridge -- for example, to access a Microsoft Access database with
Enterprise JavaBeans -- is not supported.
Because the JDBC-ODBC bridge is not thread-safe, it can
interfere with the operation of the Server.
EJB and security
This is a summary on implementing security
with WebLogic EJBeans. For more information on
security, read the Developers Guide
Using WebLogic ACLs (Access Control Lists).
WebLogic security has the notion of "groups" which can contain "users"
or other groups. Users can belong to multiple groups.
Users have a name and a credential (a certificate or a password).
WebLogic works with different security domains (also called "realms").
Examples are NT realm, NIS realm, UNIX realm. Each of these realms
can validate a given username/credential combination. WebLogic allows
custom realms to be plugged in, and defaults to the "weblogic realm",
which uses user names and passwords defined in the weblogic.properties file.
For information on custom realms,
see Using WebLogic ACLs:
Customizing the WebLogic realm with user-defined ACLs.
For information on setting up users and groups,
see Using WebLogic ACLs:
Setting up ACLs in the WebLogic realm.
As per the JavaSoft EJB specification, in EJB
you should not define a single user for access control;
instead, you should use a role. Roles map directly to groups.
You can then change the users in the
ACL (by changing the membership of the group) without having to recompile your EJBean.
In the deployment descriptor, in the access control properties,
you can configure access control, in this case using
groups "administrator" and "managers":
(accessControlEntries
DEFAULT [administrator managers]
; applies to the entire bean unless overridden
; by a method-specific access control entry
buy [managers]
; override for method buy
"sell(int)" [managers]
"sell(int, double)" [managers]
; To distinguish between two methods with the same name
; and differing signatures, specify the signature
)
On the client side, you specify the user and credentials (in this
case, the password) while logging into JNDI. Your permissions
would be determined by the group that you belong to.
static public Context getInitialContext() throws Exception
{
Properties p = new Properties();
p.put(Context.INITIAL_CONTEXT_FACTORY,
"weblogic.jndi.T3InitialContextFactory");
p.put(Context.PROVIDER_URL, url);
if (user != null) {
System.out.println ("user: " + user);
p.put(Context.SECURITY_PRINCIPAL, user);
}
if (password != null) {
p.put(Context.SECURITY_CREDENTIALS, password);
}
return new InitialContext(p);
}
In summary:
- Create a list of users and credentials in the weblogic.properties file
- Specify access control entries in the deployment descriptor
- Specify the user and credential in the client to obtain access to the bean
The limitation with this approach is that any change in the user names, passwords or
membership in the groups will require restarting the server. This is fine for testing
and development; however, in production, you will probably want to store your
security credentials in a database and access them dynamically. This is described in
Using WebLogic ACLs:
Writing a custom realm.
Retrieving EJBean properties at runtime
At runtime, you can retrieve values from the deployment descriptor
of an EJBean. This is very useful for initializing values.
Here is a fragment of environment properties from a deployment descriptor:
(environmentProperties
(StockPrices
;StockPrices is a nested hash table
WEBL 100.0
INTL 150.0
); end StockPrices
); end environmentProperties
You can retrieve the value of the stock prices at runtime by using:
Properties props = context.getEnvironment();
stockPrices = (Hashtable) props.get("StockPrices");
You can retrieve all the properties (environment and otherwise) using an Enumeration:
public void propertyTest() {
Properties props = context.getEnvironment();
Enumeration enum = props.elements();
while (enum.hasMoreElements()) {
System.out.println("Property: " + enum.nextElement());
}
}
The output would look like this:
Property: {findBigAccounts(double amount)=(> balance $amount)}
Property: isModified
Property: {jdbc={tableName=ejbAccounts,
attributeMap={testInteger=testint, balance=bal, accountId=id},
dbIsShared=false, poolName=ejbPool},
persistentStoreType=jdbc}
Additional information on environment properties,
WebLogic-specific properties and their use can be found in
Using the WebLogic EJB Deployment Wizard: Environment (environmentProperties).
WebLogic EJB utilities
This section covers the utilities supplied with
WebLogic EJB to help you in development and deployment:
- ejbc: the EJB compiler
- DDCreator: generates deployment descriptors
- ComplianceChecker: checks serialized deployment descriptors
- EJB Deployment Wizard
ejbc: the EJB compiler
For each deployment descriptor either specified in the command line or
present in a .jar file, weblogic.ejbc
creates wrapper classes for the corresponding EJBean class.
It then runs these through the RMI compiler, which generates a client-side stub
and a server-side skeleton .
These files are not inserted back into the .jar file. They are left
under the directory specified by the -d option.
By default, ejbc uses
javac as a compiler. For faster performance,
specify a different compiler (such as Symantec's
sj) using the
-compiler flag.
Running WebLogic under Microsoft SDK for Java
If you are going to run WebLogic under Microsoft SDK for Java and use EJBeans, you need to be aware that
Microsoft SDK for Java serializes objects in a manner that is
different from what other JVMs expect, and which causes serialization problems when
using EJBeans. It means that you must use JView instead of Java
when running any EJB
utilities such as DDCreator or the EJB compiler (ejbc) if you intend to
run WebLogic under Microsoft SDK for Java and use EJBeans.
See the
example EJBeans
build script (using the -ms option) that demonstrates this.
Syntax
$ java weblogic.ejbc options .jar or .ser
Arguments
- options
Option
|
Description
|
-classpath <CLASSPATH>
|
Sets a CLASSPATH for the compiler. This
overrides the system or shell CLASSPATH. |
-d <dir>
|
Sets the destination directory for the generated files.
|
-compiler <javac>
|
Sets the compiler for ejbc to use.
|
-nodeploy
|
Don't unpack jar files into the target directory.
|
-keepgenerated
|
Saves the intermediate Java files generated during compilation.
|
-normi
|
Passed through to Symantec's sj to stop generation of RMI stubs.
|
-help
|
Prints a list of all options available for the compiler.
|
- .jar or .ser
- The name of the .jar file or serialized deployment descriptor that is to be compiled.
Additional options are available; use the -help
option for a list.
Examples
(To be entered each on a single line)
$ java weblogic.ejbc AccountBeanDD.ser
$ java weblogic.ejbc -classpath %CLASSPATH%
-compiler c:\VisualCafedbDE\Bin\sj.exe
-d c:\weblogic\classes Account.jar
DDCreator: Deployment descriptor generator
Given a text description of the deployment descriptor,
DDCreator
generates a serialized EntityDescriptor or SessionDescriptor.
The example EJB deployment descriptor template created by the
-example flag contains extensive comments describing the
different options for setting up a deployment descriptor.
Also, each deployment descriptor included with the
example EJBeans
included in the WebLogic distribution
contains comments explaining the different parts of a deployment descriptor.
See these examples and their deployment descriptors for additional information.
Running WebLogic under Microsoft SDK for Java
If you are going to run WebLogic under Microsoft SDK for Java and use EJBeans, you need to be aware that
Microsoft SDK for Java serializes objects in a manner that is
different from what other JVMs expect, and which causes serialization problems when
using EJBeans. It means that you must use JView instead of Java
when running any EJB
utilities such as DDCreator or the EJB compiler (ejbc) if you intend to
run WebLogic under Microsoft SDK for Java and use EJBeans.
See the
example EJBeans
build script (using the -ms option) that demonstrates this.
Syntax
$ java weblogic.ejb.utils.DDCreator options textfile
Arguments
- options
Option
|
Description
|
-d <dir>
|
Sets the destination directory for the generated files.
|
-outputfile <filename>
|
Sets the name of the output file for the serialized deployment descriptor.
|
-example
|
Prints out an example EJB deployment descriptor template.
You can capture and edit this template to create a deployment
descriptor text file that can be then fed back into DDCreator
as textfile to generate
the deployment descriptor.
|
-help
|
Prints a list of all options available for the DDCreator.
|
- textfile
- The text description of a deployment descriptor.
Example
- Create a textual template for your deployment descriptor.
For a sample, use DDCreator and then edit the file for your specific case:
$ java weblogic.ejb.utils.DDCreator -example > myBeanDD.txt
(For an example, see the file DeploymentDescriptor.txt
in examples/ejb/beanManaged/deployment)
- Edit the myBeanDD.txt file.
- Now, run the modified file through DDCreator:
$ java weblogic.ejb.utils.DDCreator myBeanDD.txt
If your deployment descriptor describes an EJBean
myBean,
DDCreator will create the serialized deployment descriptor in a file
myBeanDD.ser.
ComplianceChecker: EJBean package checker
The utility program
weblogic.ejb.utils.ComplianceChecker
inspects a packaged EJBean and determines if it follows specified relationships.
The utility outputs to stdout.
Syntax
$ java weblogic.ejb.utils.ComplianceChecker .jar or .ser
Arguments
- .jar or .ser
- The name of the .jar file or serialized deployment descriptor to be checked.
Example
$ java weblogic.ejb.utils.ComplianceChecker beanManaged.jar
If the EJBean is correctly constructed, you will see a message such as:
File is compliant with EJB 1.0
If there is an error, you will see a message such as:
File: statefulSession.jar
==========================================================
Bean class: examples.ejb.statefulSession.server.TraderBean
----------------------------------------------------------
Session bean must have at least one valid create() method
EJB Deployment Wizard
An additional document describes the
EJB Deployment Wizard for
configuring and deploying EJBeans.
IV. Change history
- Release 4.0.3
-
We have added a new Deployment Descriptor environmental property called
"delayUpdatesUntilEndOfTx". This optional optimization affects when changes
to a bean's state are propogated to the persistent store. If the property
is set to "true" (the default), updates of the persistent store of
all beans in the transaction will be performed at the end of the
transaction. If this is set to "false", the update of a bean's persistent store will be
performed at the conclusion of each method invoke.
Previously, this optimization was enabled automatically which can
cause problems in applications that utilize integrity constraints
on the underlying database tables.
-
Fixed a problem with the passivation of stateful session beans not freeing
associated memory.
-
Fixed a bug in setting the transaction isolation
levels when using EJBeans and Oracle's type 4 driver.
-
Fixed a problem with using container-managed persistence for
BigDecimal fields in a DB2 database.
-
We have changed the embedding of stack traces in the exceptions thrown
by the WebLogic Server to make it easier to solve problems with EJB.
-
Fixed a bug which prevented char types
in remote method signatures from compiling with the EJB compiler.
-
Fixed a problem with container-managed JDBC persistence: when
home.create() is called with a primary key
of an existing EJBean, though a
DuplicateKeyException was thrown,
the existing EJBean was being overwritten by the new bean.
-
Fixed a problem with create()
being called twice for stateless session beans.
-
Fixed a problem with EJB Deployment Wizard passing the classpath to the EJB compiler.
-
Fixed a problem when the EJB Deployment Wizard opened a corrupted preferences file.
-
Fixed a problem with our extensions to the standard DeploymentDescriptors not implementing
equals() correctly.
-
We have changed the EJB examples to use a
Hashtable instead of a
Properties object when a T3Client application
creates an initial context, as that is the preferred method.
You should modify any code you have written that uses
Properties objects to instead use a
Hashtable.
-
Introduced a new commented-out connection pool called "demoPool" for use by examples such as EJB.
It uses the evaluation Cloudscape database called "demo" that's shipped with the
distribution. We have changed the EJB examples to use this database and connection pool,
as it is pre-configured with tables and data for the examples.
- Release 4.0.2
-
Fixed a problem with the exception thrown when an EJB transaction was rolled back
by the coordinator. The exception thrown should have been from the JTS JDBC call
in-progress rather than an exception raised by a database connection being closed
by another thread.
-
Fixed a problem with flagging transactions as either rolled back or
committed before they actually were rolled back or committed.
-
Fixed a problem with a container-managed EJBean state not being restored after a SQL exception
was thrown.
-
Fixed a problem with exceptions thrown while calling
ejbCreate() for a stateless session EJBean from another EJBean
being swallowed and not propogated to the calling bean.
-
Fixed a problem with EJBContext.getEJBObject()
returning null when called during
ejbCreate().
-
The EJBean compiler (ejbc) now alerts you to possible mismatches
between the exceptions in the signatures of Bean and Remote interface methods.
-
Fixed a problem with the EJB Deployment Wizard not setting JDBC environment properties correctly.
-
Added support in the EJB Deployment Wizard for the EJB finder keywords
isNull,
isNotNull,
orderBy.
- Release 4.0.1
-
Fixed a bug with resurrecting a session bean from a handle.
-
Fixed a bug with using EJBeans with a class in the root package.
-
Changed behavior so that problems parsing code causes the EJBC and
RMIC compilers to throw CodeGenerationExceptions.
-
Fixed a bug with the ordering of creates and removes of EJBeans.
-
EJB-based servlet sessions are now initialized during server startup to
avoid security restrictions on JNDI.
-
The EJB compiler (ejbc) now checks for mis-matches between the exceptions
thrown by the remote interface and the bean.
-
When TX_REQUIRED is set, all finds now start a JTS transaction if one
isn't already present.
-
Fixed a bug with persisting of EJB object references during stateful
session bean passivation.
-
Fixed a problem with ejbCreate()
not being called when a stateless session bean is recreated
after being removed.
-
Fixed a bug where the transaction context was not being restored after
invoking on a bean.
-
When an element of a deployment descriptor carries an illegal value, a
message is now printed in the server log while deploying the bean
showing which element of the deployment descriptor has the invalid
value.
-
Fixed a bug in the WebLogic EJB Deployment Wizard that was putting
persistence information incorrectly into the deployment descriptor.
-
The WebLogic EJB Deployment Wizard now determines all of the bean's
business methods, even if they come from a base class or an interface.
-
The EJB Compliance Checker (used by DDCreator) now checks that
stateless session beans do not implement the SessionSynchronization
interface.
-
Fixed problem with reentrant beans under TX_NOT_SUPPORTED.
-
Added a new Cluster EJB example:
This example has been revised to demonstrate failover and load
balancing in a robust manner using stateless session and entity beans.
- Release 4.0.0
-
Changed how EJB treats transaction isolation level; the isolation
level for transactions set in the control descriptors property of the
Deployment Descriptor is now respected and passed to the underlying
database. Oracle users: You should be aware that Oracle
databases use optimistic locking. As a consequence, if you use
TRANSACTION_SERIALIZABLE, you will get exceptions and rollbacks in the
client if contention occurs between clients for the same rows. As
mentioned above for a cluster, you will need to write your client to
catch any SQL exceptions that occur, examine them and take appropriate
action, such as attempting the transaction again.
-
Added a new operator, orderBy, to the JDBC finders that
WebLogic can generate for you when using container-managed
persistence. The finder expression syntax is described in Using the WebLogic EJB
Deployment Wizard; an example using the finder syntax is included in the
distribution.
-
Added support in the EJB Deployment Wizard for the new cluster-related
EJBC flags.
-
Fixed a bug that was causing corruption of the state of stateful
session beans when running multiple instances from a multithreaded
client (with each thread creating its own stateful session bean
instance), under very high client traffic.
-
Changed the behavior of a bean so that it immediately rolls back upon
time-out, rather than merely changing to a rollback state.
-
Fixed a bug where, after every five cycles, only one bean was being
passivated instead of the entire set of idle beans in the cache.
-
Fixed a problem with loading jar files for EJB in the WebLogic Server with an
error message indicating an unexpected end of the ZLIB input stream.
-
Behavior now that refresh() on
find is called if needsRefresh() is true, rather than just if isNew() is true.
-
Solved several interaction problems with third-party products.
-
Fixed a problem with generated JDBC code and the INTERSOLV SequeLink
JDBC Driver.
-
Rewrote the script for building examples under JView to not require
the jar tool; only the RMI and WebLogic classes are required.
-
Fixed a ClassCastException that occurred when trying to run EJBs with
WebLogic and Symantec Cafe 3.
-
Added support to the WebLogic EJB compiler (EJBC) for the -normi flag to be passed through
to the Java compiler. This can be be used with the Symantec 3.0 JIT to
prevent it from generating RMI stubs.
-
Fixed a bug where EJBeans received errors when using Sybase JDBC's ResultSet.next() method.
-
Fixed performance problems with the setup() method in PSJDBC to prevent the return of any
rows when soliciting metadata from a database.
- Added new examples demonstrating sequences,
using EJBeans with Jolt for WebLogic to access a Jolt
Server, using WebLogic's isModified extension to
EJB. Added an EJB example "quick guide" to help you find EJBean examples
relevant to the problem you are working on.
- Release 3.1
- First release compliant with the EJB 1.0 spec. WebLogic EJB now
complies with the JavaSoft EJB 1.0 specification, available at the
Javasoft website. The specification is an important part of the
documentation of this service, and you should download and refer to it
during your development.
-
When running under jview, WebLogic can't load EJBeans serialized with
the Java JDK. This is due to a bug in the Microsoft serialization that
has been reported to Microsoft. We have received verification that the
bug (the removal of a class initializer resulted in a different
serialization ID) has been fixed and will be in the final SDK 3.0 VM.
-
Added restrictions per the spec on session beans; they have no primary
key and cannot be cached.
-
Restrict the scope of EJBContext.getUserTransaction() to TX_BEAN_MANAGED
only. A client should not be able to initiate a transaction except in
a container with bean-managed persistence.
-
Fixed a synchronicity problem with create and remove.
-
Added a PersistenceDescriptor property to the
WLSessionDescriptor.
You must recompile your beans with this release.
-
Fixed problem in Console with updating display of
EJB transactions.
-
Fixed problems associated with memory leaks when
methods of session beans were called repeatedly.
-
Fixed concurrency problems with create/remove.
-
Made startup process more robust if registered beans
are not properly deployed.
-
Corrected problem related to not calling ejbActivate().
- Release 3.0.4
- Fixed an error in the weblogic.properties file that was the indirect cause of
security exceptions with EJBeans. If you are using an older properties
file, be sure that there is an entry for the "ejbPool" (formerly
"tradingPool") connection pool, that can be used for any EJB examples,
and that it looks like this:
weblogic.jdbc.connectionPool.ejbPool=\
url=jdbc:weblogic:oracle,\
driver=weblogic.jdbc.oci.Driver,\
loginDelaySecs=1,\
initialCapacity=1,\
maxCapacity=10,\
capacityIncrement=2,\
props=user=SCOTT;password=tiger;server=demo
weblogic.allow.reserve.weblogic.jdbc.connectionPool.ejbPool=\
guest
-
The line "props=user=SCOTT;password=tiger;server=demo" should not
have a trailing comma-backslash (,\).
-
Made the EJB compiler add a target jar file to its classpath
without an explicit "-classpath" compiler flag.
- Release 3.0.3 -- 3/24/98
- Fixed a problem that caused some classes from a .jar file to be
loaded incorrectly.
- Release 3.0.1 -- 3/10/98
- Second release, compliant with the EJB 0.8 spec.
- Release 3.0 -- 1/29/98
- First release, compliant with the EJB 0.5 spec.
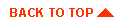
|