TopBlend:
Here is the
first difference.
There are 35 differences.
is old.
is new.
javax.swing.undo
Class UndoManager
java.lang.Object
javax.swing.undo.AbstractUndoableEdit
javax.swing.undo.CompoundEdit
javax.swing.undo.UndoManager
-
All Implemented Interfaces:
-
Serializable
,
EventListener
,
UndoableEditListener
,
UndoableEdit
-
public class UndoManager
- extends CompoundEdit
- implements UndoableEditListener
UndoManager manages a list of UndoableEdits, providing a way to undo or redo the appropriate edits. There are two ways to add edits to an UndoManager. Add the edit directly using the addEdit method, or add the UndoManager to a bean that supports UndoableEditListener. The following examples creates an UndoManager and adds it as an UndoableEditListener to a JTextField:
Concrete subclass of CompoundEdit which can serve as a UndoableEditListener, consolidating the UndoableEditEvents from a variety of sources, and undoing or redoing them one at a time. Unlike AbstractUndoableEdit and CompoundEdit, the public methods of this class are synchronized, and should be safe to call from multiple threads. This should make UndoManager a convenient marshall for sets of undoable JavaBeans.
Warning: Serialized objects of this class will not be compatible with future Swing releases. The current serialization support is appropriate for short term storage or RMI between applications running the same version of Swing. As of 1.4, support for long term storage of all JavaBeans
TM
has been added to the java.beans package. Please see
XMLEncoder
.
Field Summary
|
Fields inherited from class javax.swing.undo.
CompoundEdit
|
edits
|
Fields inherited from class javax.swing.undo.
AbstractUndoableEdit
|
RedoName
,
UndoName
|
Method Summary
|
boolean
|
addEdit
(
UndoableEdit
If inProgress, inserts anEdit at indexOfNextAdd, and removes any old edits that were at indexOfNextAdd or later.
|
boolean
|
canRedo
()
Overridden to preserve usual semantics: returns true if a redo operation would be successful now, false otherwise
|
boolean
|
canUndo
()
Overridden to preserve usual semantics: returns true if an undo operation would be successful now, false otherwise
|
boolean
|
canUndoOrRedo
()
Return true if calling undoOrRedo will undo or redo.
|
void
|
discardAllEdits
()
Empty the undo manager, sending each edit a die message in the process.
|
protected
UndoableEdit
|
editToBeRedone
()
Returns the the next significant edit to be redone if redo is called.
|
protected
UndoableEdit
|
editToBeUndone
()
Returns the the next significant edit to be undone if undo is called.
|
void
|
end
()
Sending end() to an UndoManager turns it into a plain old (ended) CompoundEdit.
|
int
|
getLimit
()
Returns the maximum number of edits this UndoManager will hold.
|
String
|
getRedoPresentationName
()
If inProgress, returns getRedoPresentationName of the significant edit that will be redone when redo() is invoked.
|
String
|
getUndoOrRedoPresentationName
()
Return the appropriate name for a command that toggles between undo and redo.
|
String
|
getUndoPresentationName
()
If inProgress, returns getUndoPresentationName of the significant edit that will be undone when undo() is invoked.
|
void
|
redo
()
If this UndoManager is inProgress, redoes the last significant UndoableEdit at indexOfNextAdd or after, and all insignificant edits up to it.
|
protected void
|
redoTo
(
UndoableEdit
Redoes all changes from indexOfNextAdd to edit.
|
void
|
setLimit
(int l)
Set the maximum number of edits this UndoManager will hold.
|
String
|
toString
()
Returns a string that displays and identifies this object's properties.
|
protected void
|
trimEdits
(int from, int to)
Tell the edits in the given range (inclusive) to die, and remove them from edits.
|
protected void
|
trimForLimit
()
Reduce the number of queued edits to a range of size limit, centered on indexOfNextAdd.
|
void
|
undo
()
If this UndoManager is inProgress, undo the last significant UndoableEdit before indexOfNextAdd, and all insignificant edits back to it.
|
void
|
undoableEditHappened
(
UndoableEditEvent
Called by the UndoabledEdit sources this UndoManager listens to.
|
void
|
undoOrRedo
()
Undo or redo as appropriate.
|
protected void
|
undoTo
(
UndoableEdit
Undoes all changes from indexOfNextAdd to edit.
|
Methods inherited from class javax.swing.undo.
CompoundEdit
|
die
,
getPresentationName
,
isInProgress
,
isSignificant
,
lastEdit
|
Methods inherited from class javax.swing.undo.
AbstractUndoableEdit
|
replaceEdit
|
Constructor Detail
|
UndoManager
UndoManager undoManager = new UndoManager();
JTextField tf = ...;
tf.getDocument().addUndoableEditListener(undoManager);

public 
UndoManager 
()
Method Detail
|
getLimit

public int 
getLimit 
()
-
Returns the maximum number of edits this UndoManager will hold. Default value is 100.
-
-
-
See Also:
-
addEdit(javax.swing.undo.UndoableEdit)
,
setLimit(int)
discardAllEdits

public void 
discardAllEdits 
()
-
Empty the undo manager, sending each edit a die message in the process.
-
-
trimForLimit

protected void 
trimForLimit 
()
-
Reduce the number of queued edits to a range of size limit, centered on indexOfNextAdd.
-
-
trimEdits

protected void 
trimEdits 
(int from,
int to)
-
Tell the edits in the given range (inclusive) to die, and remove them from edits. from > to is a no-op.
-
-
setLimit

public void 
setLimit 
(int l)
-
Set the maximum number of edits this UndoManager will hold. If edits need to be discarded to shrink the limit, they will be told to die in the reverse of the order that they were added.
-
-
-
See Also:
-
addEdit(javax.swing.undo.UndoableEdit)
,
getLimit()
editToBeUndone

protected 
UndoableEdit
editToBeUndone 
()
-
Returns the the next significant edit to be undone if undo is called. May return null
-
-
editToBeRedone

protected 
UndoableEdit
editToBeRedone 
()
-
Returns the the next significant edit to be redone if redo is called. May return null
-
-
undoTo

protected void 
undoTo 
( 
UndoableEdit
edit)
throws 
CannotUndoException
-
Undoes all changes from indexOfNextAdd to edit. Updates indexOfNextAdd accordingly.
-
-
-
Throws:
-
CannotUndoException
redoTo

protected void 
redoTo 
( 
UndoableEdit
edit)
throws 
CannotRedoException
-
Redoes all changes from indexOfNextAdd to edit. Updates indexOfNextAdd accordingly.
-
-
-
Throws:
-
CannotRedoException
undoOrRedo

public void 
undoOrRedo 
()
throws 
CannotRedoException
,

CannotUndoException
-
Undo or redo as appropriate. Suitable for binding to an action that toggles between these two functions. Only makes sense to send this if limit == 1.
-
-
-
Throws:
-
CannotRedoException
-
CannotUndoException
-
See Also:
-
canUndoOrRedo()
,
getUndoOrRedoPresentationName()
canUndoOrRedo

public boolean 
canUndoOrRedo 
()
-
Return true if calling undoOrRedo will undo or redo. Suitable for deciding to enable a command that toggles between the two functions, which only makes sense to use if limit == 1.
-
-
-
See Also:
-
undoOrRedo()
undo

public void 
undo 
()
throws 
CannotUndoException
-
If this UndoManager is inProgress, undo the last significant UndoableEdit before indexOfNextAdd, and all insignificant edits back to it. Updates indexOfNextAdd accordingly.
If not inProgress, indexOfNextAdd is ignored and super's routine is called.
-
-
Specified by:
-
undo
in interface
UndoableEdit
-
Overrides:
-
undo
in class
CompoundEdit
-
-
Throws:
-
CannotUndoException
- if canUndo returns false
-
See Also:
-
CompoundEdit.end()
canUndo

public boolean 
canUndo 
()
-
Overridden to preserve usual semantics: returns true if an undo operation would be successful now, false otherwise
-
-
Specified by:
-
canUndo
in interface
UndoableEdit
-
Overrides:
-
canUndo
in class
CompoundEdit
-
-
Returns:
-
true if this edit is alive and hasBeenDone is true
-
See Also:
-
CompoundEdit.isInProgress()
redo

public void 
redo 
()
throws 
CannotRedoException
-
If this UndoManager is inProgress, redoes the last significant UndoableEdit at indexOfNextAdd or after, and all insignificant edits up to it. Updates indexOfNextAdd accordingly.
If not inProgress, indexOfNextAdd is ignored and super's routine is called.
-
-
Specified by:
-
redo
in interface
UndoableEdit
-
Overrides:
-
redo
in class
CompoundEdit
-
-
Throws:
-
CannotRedoException
- if canRedo returns false
-
See Also:
-
CompoundEdit.end()
canRedo

public boolean 
canRedo 
()
-
Overridden to preserve usual semantics: returns true if a redo operation would be successful now, false otherwise
-
-
Specified by:
-
canRedo
in interface
UndoableEdit
-
Overrides:
-
canRedo
in class
CompoundEdit
-
-
Returns:
-
true if this edit is alive and hasBeenDone is false
-
See Also:
-
CompoundEdit.isInProgress()
addEdit

public boolean 
addEdit 
( 
UndoableEdit
anEdit)
-
If inProgress, inserts anEdit at indexOfNextAdd, and removes any old edits that were at indexOfNextAdd or later. The die method is called on each edit that is removed is sent, in the reverse of the order the edits were added. Updates indexOfNextAdd.
If not inProgress, acts as a CompoundEdit.
-
-
Specified by:
-
addEdit
in interface
UndoableEdit
-
Overrides:
-
addEdit
in class
CompoundEdit
-
-
Parameters:
-
anEdit - the edit to be added
-
Returns:
-
true if the edit is inProgress; otherwise returns false
-
See Also:
-
CompoundEdit.end()
,
CompoundEdit.addEdit(javax.swing.undo.UndoableEdit)
end

public void 
end 
()
-
Sending end() to an UndoManager turns it into a plain old (ended) CompoundEdit.
Calls super's end() method (making inProgress false), then sends die() to the unreachable edits at indexOfNextAdd and beyond, in the reverse of the order in which they were added.
-
-
Overrides:
-
end
in class
CompoundEdit
-
-
See Also:
-
CompoundEdit.end()
getUndoOrRedoPresentationName

public 
String
getUndoOrRedoPresentationName 
()
-
Return the appropriate name for a command that toggles between undo and redo. Only makes sense to use such a command if limit == 1 and we're not in progress.
-
-
getUndoPresentationName

public 
String
getUndoPresentationName 
()
-
If inProgress, returns getUndoPresentationName of the significant edit that will be undone when undo() is invoked. If there is none, returns AbstractUndoableEdit.undoText from the defaults table.
If not inProgress, acts as a CompoundEdit
-
-
Specified by:
-
getUndoPresentationName
in interface
UndoableEdit
-
Overrides:
-
getUndoPresentationName
in class
CompoundEdit
-
-
Returns:
-
the value from the defaults table with key AbstractUndoableEdit.undoText, followed by a space, followed by getPresentationName unless getPresentationName is "" in which case, the defaults value is returned alone.
-
See Also:
-
undo()
,
CompoundEdit.getUndoPresentationName()
getRedoPresentationName

public 
String
getRedoPresentationName 
()
-
If inProgress, returns getRedoPresentationName of the significant edit that will be redone when redo() is invoked. If there is none, returns AbstractUndoableEdit.redoText from the defaults table.
If not inProgress, acts as a CompoundEdit
UndoManager maintains an ordered list of edits and the index of the next edit in that list. The index of the next edit is either the size of the current list of edits, or if undo has been invoked it corresponds to the index of the last significant edit that was undone. When undo is invoked all edits from the index of the next edit to the last significant edit are undone, in reverse order. For example, consider an UndoManager consisting of the following edits:
-
-
A
Specified by:
b
c
-
getRedoPresentationName
in interface
UndoableEdit
-
D
Overrides:
. Edits with a upper-case letter in bold are significant, those in lower-case and italicized are insignificant.
-
getRedoPresentationName
|
Figure 1
|
As shown in
figure 1
in class
CompoundEdit
, if
-
-
D
Returns:
was just added, the index of the next edit will be 4. Invoking undo results in invoking undo on
-
the value from the defaults table with key AbstractUndoableEdit.redoText, followed by a space, followed by getPresentationName unless getPresentationName is "" in which case, the defaults value is returned alone.
-
D
See Also:
and setting the index of the next edit to 3 (edit
c
), as shown in the following figure.
-
redo()
|
Figure 2
|
The last significant edit is
,
CompoundEdit.getUndoPresentationName()
undoableEditHappened

public void
A 
undoableEditHappened
, so that invoking undo again invokes undo on
c
,
b
, and
A
, in that order, setting the index of the next edit to 0, as shown in the following figure. 

( 
UndoableEditEvent
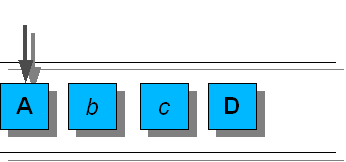 |
Figure 3 |

e)
-
Called by the UndoabledEdit sources this UndoManager listens to. Calls addEdit with e.getEdit().
Invoking redo results in invoking redo on all edits between the index of the next edit and the next significant edit (or the end of the list). Continuing with the previous example if redo were invoked, redo would in turn be invoked on
-
-
A
Specified by:
,
b
and
c
. In addition the index of the next edit is set to 3 (as shown in
figure 2
-
undoableEditHappened
).
Adding an edit to an UndoManager results in removing all edits from the index of the next edit to the end of the list. Continuing with the previous example, if a new edit,
e
, is added the edit
in interface
UndoableEditListener
-
-
D
See Also:
is removed from the list (after having die invoked on it). If
c
is not incorporated by the next edit (
c
.addEdit(
e
) returns true), or replaced by it (
e
.replaceEdit(
c
) returns true), the new edit is added after
c
, as shown in the following figure.
-
addEdit(javax.swing.undo.UndoableEdit)
|
Figure 4
|
Once end has been invoked on an UndoManager the superclass behavior is used for all UndoableEdit methods. Refer to CompoundEdit for more details on its behavior.
Unlike the rest of Swing, this class is thread safe.
Warning: Serialized objects of this class will not be compatible with future Swing releases. The current serialization support is appropriate for short term storage or RMI between applications running the same version of Swing. As of 1.4, support for long term storage of all JavaBeans
TM
has been added to the java.beans package. Please see
XMLEncoder
.
Field Summary
|
Fields inherited from class javax.swing.undo.
CompoundEdit
|
edits
|
Fields inherited from class javax.swing.undo.
AbstractUndoableEdit
|
RedoName
,
UndoName
|
Constructor Summary
|
UndoManager
()
Creates a new UndoManager.
|
Method Summary
|
boolean
|
addEdit
(
UndoableEdit
Adds an UndoableEdit to this UndoManager, if it's possible.
|
boolean
|
canRedo
()
Returns true if edits may be redone.
|
boolean
|
canUndo
()
Returns true if edits may be undone.
|
boolean
|
canUndoOrRedo
()
Returns true if it is possible to invoke undo or redo.
|
void
|
discardAllEdits
()
Empties the undo manager sending each edit a die message in the process.
|
protected
UndoableEdit
|
editToBeRedone
()
Returns the the next significant edit to be redone if redo is invoked.
|
protected
UndoableEdit
|
editToBeUndone
()
Returns the the next significant edit to be undone if undo is invoked.
|
void
|
end
()
Turns this UndoManager into a normal CompoundEdit.
|
int
|
getLimit
()
Returns the maximum number of edits this UndoManager will hold.
|
String
|
getRedoPresentationName
()
Returns a description of the redoable form of this edit.
|
String
|
getUndoOrRedoPresentationName
()
Convenience method that returns either getUndoPresentationName or getRedoPresentationName.
|
String
|
getUndoPresentationName
()
Returns a description of the undoable form of this edit.
|
void
|
redo
()
Redoes the appropriate edits.
|
protected void
|
redoTo
(
UndoableEdit
Redoes all changes from the index of the next edit to edit, updating the index of the next edit appropriately.
|
void
|
setLimit
(int l)
Set the maximum number of edits this UndoManager holds.
|
String
|
toString
()
Returns a string that displays and identifies this object's properties.
|
protected void
|
trimEdits
(int from, int to)
Removes edits in the specified range.
|
protected void
|
trimForLimit
()
Reduces the number of queued edits to a range of size limit, centered on the index of the next edit.
|
void
|
undo
()
Undoes the appropriate edits.
|
void
|
undoableEditHappened
(
UndoableEditEvent
An UndoableEditListener method.
|
void
|
undoOrRedo
()
Convenience method that invokes one of undo or redo.
|
protected void
|
undoTo
(
UndoableEdit
Undoes all changes from the index of the next edit to edit, updating the index of the next edit appropriately.
|
Methods inherited from class javax.swing.undo.
CompoundEdit
|
die
,
getPresentationName
,
isInProgress
,
isSignificant
,
lastEdit
|
Methods inherited from class javax.swing.undo.
AbstractUndoableEdit
|
replaceEdit
|
Constructor Detail
|
UndoManager
toString
public 
public 
String
UndoManager 
toString ()
-
Creates a new UndoManager.
Returns a string that displays and identifies this object's properties.
-
-
Overrides:
-
toString
in class
CompoundEdit
-
-
Returns:
-
a String representation of this object
Method Detail
|
getLimit
public int
getLimit
()
-
Returns the maximum number of edits this UndoManager will hold.
-
-
-
See Also:
-
addEdit(javax.swing.undo.UndoableEdit)
,
setLimit(int)
discardAllEdits
public void
discardAllEdits
()
-
Empties the undo manager sending each edit a die message in the process.
-
-
-
See Also:
-
AbstractUndoableEdit.die()
trimForLimit
protected void
trimForLimit
()
-
Reduces the number of queued edits to a range of size limit, centered on the index of the next edit.
-
-
trimEdits
protected void
trimEdits
(int from,
int to)
-
Removes edits in the specified range. All edits in the given range (inclusive, and in reverse order) will have die invoked on them and are removed from the list of edits. This has no effect if from > to.
-
-
-
Parameters:
-
from - the minimum index to remove
-
to - the maximum index to remove
setLimit
public void
setLimit
(int l)
-
Set the maximum number of edits this UndoManager holds. If edits need to be discarded to shrink the limit, die will be invoked on them in the reverse order they were added. The default is 100.
-
-
-
Parameters:
-
l - the new limit
-
See Also:
-
addEdit(javax.swing.undo.UndoableEdit)
,
getLimit()
editToBeUndone
protected
UndoableEdit
editToBeUndone
()
-
Returns the the next significant edit to be undone if undo is invoked. This returns null if there are no edits to be undone.
-
-
-
Returns:
-
the next significant edit to be undone
editToBeRedone
protected
UndoableEdit
editToBeRedone
()
-
Returns the the next significant edit to be redone if redo is invoked. This returns null if there are no edits to be redone.
-
-
-
Returns:
-
the next significant edit to be redone
undoTo
protected void
undoTo
(
UndoableEdit
edit)
throws
CannotUndoException
-
Undoes all changes from the index of the next edit to edit, updating the index of the next edit appropriately.
-
-
-
Throws:
-
CannotUndoException
- if one of the edits throws CannotUndoException
redoTo
protected void
redoTo
(
UndoableEdit
edit)
throws
CannotRedoException
-
Redoes all changes from the index of the next edit to edit, updating the index of the next edit appropriately.
-
-
-
Throws:
-
CannotRedoException
- if one of the edits throws CannotRedoException
undoOrRedo
public void
undoOrRedo
()
throws
CannotRedoException
,
CannotUndoException
-
Convenience method that invokes one of undo or redo. If any edits have been undone (the index of the next edit is less than the length of the edits list) this invokes redo, otherwise it invokes undo.
-
-
-
Throws:
-
CannotUndoException
- if one of the edits throws CannotUndoException
-
CannotRedoException
- if one of the edits throws CannotRedoException
-
See Also:
-
canUndoOrRedo()
,
getUndoOrRedoPresentationName()
canUndoOrRedo
public boolean
canUndoOrRedo
()
-
Returns true if it is possible to invoke undo or redo.
-
-
-
Returns:
-
true if invoking canUndoOrRedo is valid
-
See Also:
-
undoOrRedo()
undo
public void
undo
()
throws
CannotUndoException
-
Undoes the appropriate edits. If end has been invoked this calls through to the superclass, otherwise this invokes undo on all edits between the index of the next edit and the last significant edit, updating the index of the next edit appropriately.
-
-
Specified by:
-
undo
in interface
UndoableEdit
-
Overrides:
-
undo
in class
CompoundEdit
-
-
Throws:
-
CannotUndoException
- if one of the edits throws CannotUndoException or there are no edits to be undone
-
See Also:
-
CompoundEdit.end()
,
canUndo()
,
editToBeUndone()
canUndo
public boolean
canUndo
()
-
Returns true if edits may be undone. If end has been invoked, this returns the value from super. Otherwise this returns true if there are any edits to be undone (editToBeUndone returns non-null).
-
-
Specified by:
-
canUndo
in interface
UndoableEdit
-
Overrides:
-
canUndo
in class
CompoundEdit
-
-
Returns:
-
true if there are edits to be undone
-
See Also:
-
CompoundEdit.canUndo()
,
editToBeUndone()
redo
public void
redo
()
throws
CannotRedoException
-
Redoes the appropriate edits. If end has been invoked this calls through to the superclass. Otherwise this invokes redo on all edits between the index of the next edit and the next significant edit, updating the index of the next edit appropriately.
-
-
Specified by:
-
redo
in interface
UndoableEdit
-
Overrides:
-
redo
in class
CompoundEdit
-
-
Throws:
-
CannotRedoException
- if one of the edits throws CannotRedoException or there are no edits to be redone
-
See Also:
-
CompoundEdit.end()
,
canRedo()
,
editToBeRedone()
canRedo
public boolean
canRedo
()
-
Returns true if edits may be redone. If end has been invoked, this returns the value from super. Otherwise, this returns true if there are any edits to be redone (editToBeRedone returns non-null).
-
-
Specified by:
-
canRedo
in interface
UndoableEdit
-
Overrides:
-
canRedo
in class
CompoundEdit
-
-
Returns:
-
true if there are edits to be redone
-
See Also:
-
CompoundEdit.canRedo()
,
editToBeRedone()
addEdit
public boolean
addEdit
(
UndoableEdit
anEdit)
-
Adds an UndoableEdit to this UndoManager, if it's possible. This removes all edits from the index of the next edit to the end of the edits list. If end has been invoked the edit is not added and false is returned. If end hasn't been invoked this returns true.
-
-
Specified by:
-
addEdit
in interface
UndoableEdit
-
Overrides:
-
addEdit
in class
CompoundEdit
-
-
Parameters:
-
anEdit - the edit to be added
-
Returns:
-
true if anEdit can be incorporated into this edit
-
See Also:
-
CompoundEdit.end()
,
CompoundEdit.addEdit(javax.swing.undo.UndoableEdit)
end
public void
end
()
-
Turns this UndoManager into a normal CompoundEdit. This removes all edits that have been undone.
-
-
Overrides:
-
end
in class
CompoundEdit
-
-
See Also:
-
CompoundEdit.end()
getUndoOrRedoPresentationName
public
String
getUndoOrRedoPresentationName
()
-
Convenience method that returns either getUndoPresentationName or getRedoPresentationName. If the index of the next edit equals the size of the edits list, getUndoPresentationName is returned, otherwise getRedoPresentationName is returned.
-
-
-
Returns:
-
undo or redo name
getUndoPresentationName
public
String
getUndoPresentationName
()
-
Returns a description of the undoable form of this edit. If end has been invoked this calls into super. Otherwise if there are edits to be undone, this returns the value from the next significant edit that will be undone. If there are no edits to be undone and end has not been invoked this returns the value from the UIManager property "AbstractUndoableEdit.undoText".
-
-
Specified by:
-
getUndoPresentationName
in interface
UndoableEdit
-
Overrides:
-
getUndoPresentationName
in class
CompoundEdit
-
-
Returns:
-
a description of the undoable form of this edit
-
See Also:
-
undo()
,
CompoundEdit.getUndoPresentationName()
getRedoPresentationName
public
String
getRedoPresentationName
()
-
Returns a description of the redoable form of this edit. If end has been invoked this calls into super. Otherwise if there are edits to be redone, this returns the value from the next significant edit that will be redone. If there are no edits to be redone and end has not been invoked this returns the value from the UIManager property "AbstractUndoableEdit.redoText".
-
-
Specified by:
-
getRedoPresentationName
in interface
UndoableEdit
-
Overrides:
-
getRedoPresentationName
in class
CompoundEdit
-
-
Returns:
-
a description of the redoable form of this edit
-
See Also:
-
redo()
,
CompoundEdit.getRedoPresentationName()
undoableEditHappened
public void
undoableEditHappened
(
UndoableEditEvent
e)
-
An UndoableEditListener method. This invokes addEdit with e.getEdit().
-
-
Specified by:
-
undoableEditHappened
in interface
UndoableEditListener
-
-
Parameters:
-
e - the UndoableEditEvent the UndoableEditEvent will be added from
-
See Also:
-
addEdit(javax.swing.undo.UndoableEdit)
toString
public
String
toString
()
-
Returns a string that displays and identifies this object's properties.
-
-
Overrides:
-
toString
in class
CompoundEdit
-
-
Returns:
-
a String representation of this object