*
01 TPTRXDEF-REC.
COPY TPTRXDEF.
*
01
TPSTATUS-REC.
COPY TPSTATUS.
*
CALL "TPBEGIN" USING
TPTRXDEF-REC TPSTATUS-REC.
Table 9‑1 describes the
TPTRXDEF-REC structure fields
|
|
|
The use of 0 or an unrealistically large value for the T-OUT parameter delays system detection and reporting of errors. The system uses the T-OUT parameter to ensure that responses to service requests are sent within a reasonable time, and to terminate transactions that encounter problems such as network failures before executing a commit.
In a production system, you should set T-OUT to a value large enough to accommodate expected delays due to system load and database contention. A small multiple of the expected average response time is often an appropriate choice.
Note:
|
The value assigned to the T-OUT parameter should be consistent with that of the SCANUNIT parameter set by the Oracle Tuxedo application administrator in the configuration file. The SCANUNIT parameter specifies the frequency with which the system checks, or scans, for timed-out transactions and blocked calls in service requests. The value of this parameter represents the interval of time between these periodic scans, referred to as the scanning unit. You should set the T-OUT parameter to a value that is greater than the scanning unit. If you set the T-OUT parameter to a value smaller than the scanning unit, there will be a discrepancy between the time at which a transaction times out and the time at which this timeout is discovered by the system. The default value for SCANUNIT is 10 seconds. You may need to discuss the setting of the T-OUT parameter with your application administrator to make sure the value you assign to the T-OUT parameter is compatible with the values assigned to your system parameters.
|
|
|
|
Any process may call TPBEGIN unless the process is already in transaction mode. If
TPBEGIN is called in transaction mode, the call fails due to a protocol error and
TP-STATUS is set to
TPEPROTO. If the process is in transaction mode, the transaction is unaffected by the failure.
. . .
MOVE 0 TO T-OUT.
CALL "TPBEGIN" USING
TPTRXDEF-REC
TPSTATUS-REC.
IF NOT TPOK
error processing
. . .
program statements
. . .
CALL "TPCOMMIT" USING
TPTRXDEF-REC
TPSTATUS-REC.
IF NOT TPOK
error processing
. . .
MOVE "BUY" TO SERVICE-NAME.
SET TPBLOCK TO TRUE.
SET TPNOTRAN TO TRUE.
SET TPREPLY TO TRUE.
SET TPNOTIME TO TRUE.
SET TPSIGRSTRT TO TRUE.
CALL "TPACALL" USING
TPSVCDEF-REC
TPTYPE-REC
BUY-REC
TPSTATUS-REC.
IF NOT TPOK
error processing
. . .
MOVE 0 TO T-OUT.
CALL "TPBEGIN" USING
TPTRXDEF-REC
TPSTATUS-REC.
vIF NOT TPOK
error processing
* ERROR TP-STATUS is set to TPEPROTO
. . .
program statements
. . .
SET TPBLOCK TO TRUE.
SET TPNOTRAN TO TRUE.
SET TPCHANGE TO TRUE.
SET TPNOTIME TO TRUE.
SET TPSIGRSTRT TO TRUE.
SET TPGETANY TO TRUE.
CALL "TPGETRPLY" USING
TPSVCDEF-REC
TPTYPE-REC
WK-AREA
TPSTATUS-REC.
IF NOT TPOK
error processing
. . .
MOVE 30 TO T-OUT.
CALL "TPBEGIN" USING TPTRXDEF-REC TPSTATUS-REC.
IF NOT TPOK
MOVE "Failed to BEGIN a transaction" TO LOG-REC-TEXT.
MOVE 29 to LOG-REC-LEN
CALL "USERLOG" USING
LOG-REC-TEXT
LOG-REC-LEN
TPSTATUS-REC
CALL "TPTERM" USING
TPSTATUS-REC
PERFORM A-999-EXIT.
. . .
communication CALL statements
. . .
IF TPETIME
CALL "TPABORT" USING
TPTRXDEF-REC
TPSTATUS-REC
IF NOT TPOK
error processing
ELSE
CALL "TPCOMMIT" USING
TPTRXDEF-REC
TPSTATUS-REC
IF NOT TPOK
error processing
Note:
|
When a process is in transaction mode and makes a communication call with TPNOTRAN, it prohibits the called service from becoming a participant in the current transaction. Whether the service request succeeds or fails has no impact on the outcome of the transaction. The transaction can still timeout while waiting for a reply that is due from a service, whether it is part of the transaction or not. Refer to “Managing Errors” in Programming Oracle Tuxedo ATMI Applications Using C for more information on the effects of the TPNOTRAN flag.
|
DATA DIVISION.
WORKING-STORAGE SECTION.
*
01 TPTYPE-REC.
COPY TPTYPE.
*
01 TPSTATUS-REC.
COPY TPSTATUS.
*
01 TPINFDEF-REC.
COPY TPINFDEF.
*
01 TPSVCDEF-REC.
COPY TPSVCDEF.
*
01 TPTRXDEF-REC.
COPY TPTRXDEF.
*
01 LOG-REC PIC X(30) VALUE " ".
01 LOG-REC-LEN PIC S9(9) COMP-5.
*
01 USR-DATA-REC PIC X(16).
*
01 AUDV-REC.
05 AUDV-BRANCH-ID PIC S9(9) COMP-5.
05 AUDV-BALANCE PIC S9(9) COMP-5.
05 AUDV-ERRMSG PIC X(60).
*
PROCEDURE DIVISION.
*
A-000.
. . .
* Get Command Line Options set Variables (Q-BRANCH)
MOVE SPACES TO USRNAME.
MOVE SPACES TO CLTNAME.
MOVE SPACES TO PASSWD.
MOVE SPACES TO GRPNAME.
CALL "TPINITIALIZE" USING TPINFDEF-REC
USR-DATA-REC
TPSTATUS-REC.
IF NOT TPOK
MOVE "Failed to join application" TO LOG-REC
MOVE 26 TO LOG-REC-LEN
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC
PERFORM A-999-EXIT.
* Start global transaction
MOVE 30 TO T-OUT.
CALL "TPBEGIN" USING TPTRXDEF-REC TPSTATUS-REC.
IF NOT TPOK
MOVE 29 to LOG-REC-LEN
MOVE "Failed to begin a transaction" TO LOG-REC
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC
PERFORM DO-TPTERM.
* Set up record
MOVE Q-BRANCH TO AUDV-BRANCH-ID.
MOVE ZEROS TO AUDV-BALANCE.
MOVE SPACES TO AUDV-ERRMSG.
* Set up TPCALL records
MOVE "GETBALANCE" TO SERVICE-NAME.
MOVE "VIEW" TO REC-TYPE.
MOVE LENGTH OF AUDV-REC TO LEN.
SET TPBLOCK TO TRUE.
SET TPTRAN IN TPSVCDEF-REC TO TRUE.
SET TPNOTIME TO TRUE.
SET TPSIGRSTRT TO TRUE.
SET TPCHANGE TO TRUE.
*
CALL "TPCALL" USING TPSVCDEF-REC
TPTYPE-REC
AUDV-REC
TPTYPE-REC
AUDV-REC
TPSTATUS-REC.
IF NOT TPOK
MOVE 19 to LOG-REC-LEN
MOVE "Service call failed" TO LOG-REC
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC
PERFORM DO-TPABORT
PERFORM DO-TPTERM.
* Commit global transaction
CALL "TPCOMMIT" USING TPTRXDEF-REC
TPSTATUS-REC
IF NOT TPOK
MOVE 16 to LOG-REC-LEN
MOVE "Failed to commit" TO LOG-REC
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC
PERFORM DO-TPTERM.
* Show results only when transaction has completed successfully
DISPLAY "BRANCH=" Q-BRANCH.
DISPLAY "BALANCE=" AUDV-BALANCE.
PERFORM DO-TPTERM.
* Abort the transaction
DO-TPABORT.
CALL "TPABORT" USING TPTRXDEF-REC
TPSTATUS-REC
IF NOT TPOK
MOVE 26 to LOG-REC-LEN
MOVE "Failed to abort transaction" TO LOG-REC
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC.
* Leave the application
DO-TPTERM.
CALL "TPTERM" USING TPSTATUS-REC.
IF NOT TPOK
MOVE 27 to LOG-REC-LEN
MOVE "Failed to leave application" TO LOG-REC
CALL "USERLOG" USING LOG-REC
LOG-REC-LEN
TPSTATUS-REC.
EXIT PROGRAM.
*
A-999-EXIT.
*
EXIT PROGRAM.
Note:
|
If TPCALL, TPACALL, or TPCONNECT is called by a process that has explicitly set TPNOTRAN, the operations performed by the called service do not become part of the current transaction. In other words, when you call the TPABORT routine, the operations performed by these services are not rolled back.
|
The TPCOMMIT(3cbl) routine commits the current transaction. When
TPCOMMIT returns successfully, all changes to resources as a result of the current transaction become permanent.
*
01 TPTRXDEF-REC.
COPY TPTRXDEF.
*
01
TPSTATUS-REC.
COPY TPSTATUS.
*
CALL "TPCOMMIT" USING
TPTRXDEF-REC TPSTATUS-REC.
For TPCOMMIT to succeed, the following conditions must be true:
If the first condition is false, the call fails and TP-STATUS is set to
TPEPROTO, indicating a protocol error. If the second or third condition is false, the call fails and
TP-STATUS is set to
TPEABORT, indicating that the transaction has been rolled back. If
TPCOMMIT is called by the initiator with outstanding transaction replies, the transaction is aborted and those reply descriptors associated with the transaction become invalid. If a participant calls
TPCOMMIT or
TPABORT, the transaction is unaffected.
A transaction is placed in a rollback-only state if any service call returns TPFAIL or indicates a service error. If
TPCOMMIT is called for a rollback-only transaction, the routine cancels the transaction, returns -1, and sets
TP-STATUS to
TPEABORT. The results are the same if
TPCOMMIT is called for a transaction that has already timed out:
TPCOMMIT returns -1 and sets
TP-STATUS to
TPEABORT. Refer to
“Managing Errors” in
Programming Oracle Tuxedo ATMI Applications Using C for more information on transaction errors.
When the TPCOMMIT routine is called, it initiates the
two-phase commit protocol. This protocol, as the name suggests, consists of two steps:
The commit sequence begins when the transaction initiator calls the TPCOMMIT routine. The Oracle Tuxedo TMS server process in the designated coordinator group contacts the TMS in each participant group that is to perform the first phase of the commit protocol. The TMS in each group then instructs the resource manager (RM) in that group to commit using the XA protocol that is defined for communications between the Transaction Managers and RMs. The RM writes, to stable storage, the states of the transaction before and after the commit sequence, and indicates success or failure to the TMS. The TMS then passes the response back to the coordinating TMS.
•
|
LOGGED—to require completion of phase 1
|
•
|
COMPLETE—to require completion of phase 2
|
Use the TPABORT(3cbl) routine to indicate an abnormal condition and explicitly abort a transaction. This function invalidates the call descriptors of any outstanding transactional replies. None of the changes produced by the transaction are applied to the resource. Use the following signature to call the
TPABORT routine:
*
01 TPTRXDEF-REC.
COPY TPTRXDEF.
*
01
TPSTATUS-REC.
COPY TPSTATUS.
*
CALL "TPABORT" USING
TPTRXDEF-REC TPSTATUS-REC.
Figure 9‑1 illustrates a conversational connection hierarchy that includes a global transaction.
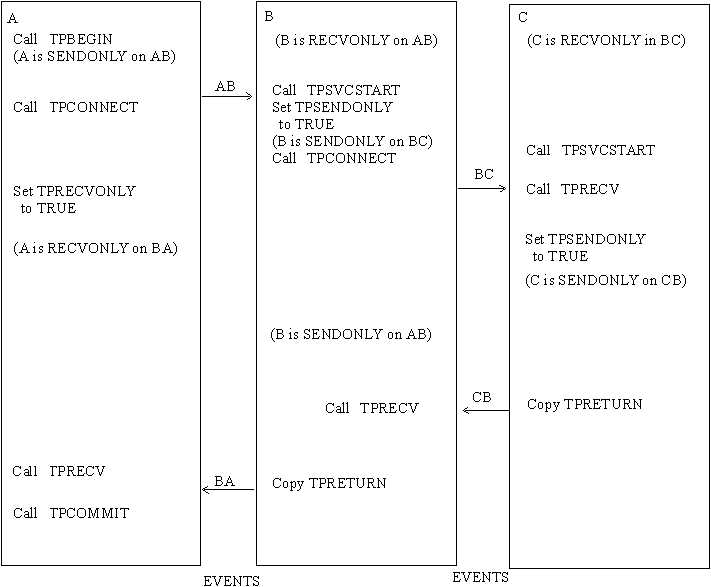
01 . . .
02 CALL "TPINITIALIZE" USING TPINFDEF-REC
03 USR-DATA-REC
04 TPSTATUS-REC.
05 IF NOT TPOK
06 error message,
07 EXIT PROGRAM .
08 MOVE 30 TO T-OUT.
09 CALL "TPBEGIN" USING TPTRXDEF-REC TPSTATUS-REC.
10 IF NOT TPOK
11
error message,
12 PERFORM DO-TPTERM.
13 * Set up record
14 MOVE "REPORT=accrcv DBNAME=accounts" TP-RECORD.
15 MOVE 27 TO LEN.
16 MOVE "REPORTS" TO SERVICE-NAME.
17 MOVE "STRING" TO REC-TYPE.
18 SET TPBLOCK TO TRUE.
19 SET TPTRAN IN TPSVCDEF-REC TO TRUE.
20 SET TPNOTIME TO TRUE.
21 SET TPSIGRSTRT TO TRUE.
22 SET TPCHANGE TO TRUE.
23 *
24 CALL "TPCALL" USING TPSVCDEF-REC
25 TPTYPE-REC
26 TP-RECORD
27 TPTYPE-REC
28 TP-RECORD
29 TPSTATUS-REC.
30 IF TPOK
31 PERFORM DO-TPCOMMIT
32 PERFORM DO-TPTERM.
33 * Check return status
34 IF TPESVCERR
35 DISPLAY "REPORT service's TPRETURN encountered problems"
36 ELSE IF TPESVCFAIL
37 DISPLAY "REPORT service FAILED with return code=" APPL-RETURN-CODE
38 ELSE IF TPEOTYPE
39 DISPLAY "REPORT service's reply is not of any known REC-TYPE"
40 *
41 PERFORM DO-TPABORT
42 PERFORM DO-TPTERM.
43 * Commit global transaction
44 DO-TPCOMMIT.
45 CALL "TPCOMMIT" USING TPTRXDEF-REC
46 TPSTATUS-REC
47 IF NOT TPOK
48 error message
49 * Abort the transaction
50 DO-TPABORT.
51 CALL "TPABORT" USING TPTRXDEF-REC
52 TPSTATUS-REC
53 IF NOT TPOK
54 error message
55 * Leave the application
56 DO-TPTERM.
57 CALL "TPTERM" USING TPSTATUS-REC.
58 IF NOT TPOK
59 error message
60 EXIT PROGRAM.
If not set to TPNOTRAN, then the system places the called process in transaction mode through the “rule of propagation.” The system does not check the
AUTOTRAN parameter.
If TPTRN-FLAG IN TPSVCDEF-REC is set to
TPNOTRAN, the services performed by the called process are not included in the current transaction (that is, the propagation rule is suppressed). The system checks the
AUTOTRAN parameter.
•
|
If AUTOTRAN is set to N (or if it is not set), the system does not place the called process in transaction mode.
|
•
|
If AUTOTRAN is set to Y, the system places the called process in transaction mode, but treats it as a new transaction.
|
01 TPTRXLEV-REC.
COPY TPTRXLEV.
01
TPSTATUS-REC.
COPY TPSTATUS.
CALL "TPGETLEV" USING
TPTRXLEV-REC TPSTATUS-REC.
TPGETLEV returns
TP-NOT-IN-TRAN if the caller is not in a transaction and
TP-IN-TRAN if the caller is.
The following code sample shows how to test for transaction level using the TPGETLEV routine (line 3). If the process is not already in transaction mode, the application starts a transaction (line 5). If
TPBEGIN fails, a message is returned to the status line (line 9) and
APPL-CODE IN TPSVCRET-REC of
TPRETURN is set to a code that can be retrieved in
APL-RETURN-CODE IN TPSTATUS-REC (lines 1 and 11).
. . . Application defined codes
001 77 BEG-FAILED PIC S9(9) VALUE 3.
. . .
002 PROCEDURE DIVISION.
. . .
003 CALL "TPGETLEV" USING TPTRCLEV-REC
TPSTATUS-REC.
004 IF NOT TPOK
error processing EXIT PROGRAM
005 IF TP-NOT-IN-TRAN
006 MOVE 30 TO T-OUT.
007 CALL "TPBEGIN" USING
TPTRXDEF-REC
TPSTATUS-REC.
008 IF NOT TPOK
009 MOVE "Attempt to TPBEGIN within service failed"
TO USER-MESSAGE.
010 SET TPFAIL TO TRUE.
011 MOVE BEG-FAILED TO APPL-CODE.
012 COPY TPRETURN REPLACING
013 DATA-REC BY USER-MESSAGE.
. . .
If the AUTOTRAN parameter is set to
Y, you do not need to call the
TPBEGIN, and
TPCOMMIT or
TPABORT transaction routines explicitly. As a result, you can avoid the overhead of testing for transaction level. In addition, you can set the
TRANTIME parameter to specify the time-out interval: the amount of time that may elapse after a transaction for a service begins, and before it is rolled back if not completed.
For example, suppose you are revising the OPEN_ACCT service shown in the preceding code listing. Currently,
OPEN_ACCT defines the transaction explicitly and then tests for its existence. To reduce the overhead introduced by these tasks, you can eliminate them from the code. Therefore, you need to require that whenever
OPEN_ACCT is called, it is called in transaction mode. To specify this requirement, enable the
AUTOTRAN and
TRANTIME system parameters in the configuration file.
•
|
TRANTIME configuration parameter in Setting Up an Oracle Tuxedo Application.
|