Array Class Methods
In this section, we discuss the array class methods.
Syntax
Clone()
Description
The Clone method returns a reference to a new array, which is a copy of the given array. It copies all levels of the array, meaning, if the array contains elements which are themselves arrays (actually references to arrays), then the copy contains references to copies of the subarrays. Furthermore, if the array contains elements that are references to the same subarray, then the copy contains references to different subarrays (which of course have the same value).
Parameters
None. The array object that the clone method is executed against is the array to be cloned. Assigning the result of this method assigns a reference to the new array.
Returns
An array object copied from the original.
Example
In the following example, &AAN2 contains the three elements like &AAN, but they are distinct arrays. The last line changes only &AAN2[1][1], not &AAN[1][1].
Local Array of Array of String &AAN, &AAN2;
&AAN = CreateArray(CreateArray("A", "B"), CreateArray("C", "D"), "E");
&AAN2 = &AAN.Clone();
&AAN2[1][1] = "Z";
After the following example, &AAN contains three elements: two references to the subarray that was &AAN[2] (with elements C and D), and a reference to a subarray with element E.
&AAN[1] = &AAN[2];
After the following example, &AAN2 contains three elements: references to two different subarrays both with elements C and D, and a subarray with element E.
&AAN2 = &AAN.Clone();
Syntax
Find(value)
Description
For a one-dimensional array, the Find method returns the lowest index of an element in the array that is equal to the given value. If the value is not found in the array, it returns zero.
For a two-dimensional array, the Find method returns the lowest index of a subarray which has its first element equal to the given value. If such a subarray is not found in the array, it returns zero.
Note: This method works with arrays that have only one or two dimensions. You receive a runtime error if you try to use this method with an array that has more than two dimensions.
Parameters
Field or Control |
Definition |
---|---|
value |
The string or subarray to search for. |
Returns
An index of an element or zero.
Example
Given an array &AS containing (A, B, C, D, E), the following code sets &IND to the index of D, that is, &IND has the value 4:
Local array of string &AS;
&AS = CreateArrayRept("", 0);
&AS.Push("A");
&AS.Push("B");
&AS.Push("C");
&AS.Push("D");
&AS.Push("E");
&IND = &AS.Find("D");
Given an array of array of string &AABYNAME containing (("John", "July"), ("Jane", "June"), ("Norm", "November"), the following code sets &IND to the index of the subarray starting with "Jane", that is, &IND has the value 2:
&NAME = "Jane";
&IND = &AABYNAME.Find(&NAME);
Syntax
Get(index)
Description
Use the Get method to return the index element of an array. This method is used with the Java PeopleCode functions, instead of using subscripts (which aren't available in Java.)
Using this method is the same as using a subscript to return an item of an array. In the following example, the two lines of code are identical:
&Value = &MyArray[8];
&value = &MyArray.Get(8);
Parameters
Field or Control |
Definition |
---|---|
index |
The array element to be accessed. |
Returns
An element in an array.
Syntax
Join([separator [, arraystart, arrayend [,stringsizehint]]])
Description
The Join method converts the array that is executing the method into a string by converting each element into a string and joining these strings together, separated by separator.
Note: Join does not join two arrays together.
Each array or subarray to be joined is preceded by the string given by arraystart and followed by the string given by arrayend. If the given array is multi-dimensional, then (logically) each subarray is first joined, then the resulting strings are joined together.
Parameters
Field or Control |
Definition |
---|---|
separator |
Specifies what the elements in the resulting string should be separated with in the resulting string. Separator is set by default to a comma (","). |
arraystart |
Specifies what each array or subarray to be joined should be preceded with in the resulting string. arraystart is set by default to a left parenthesis ("("). |
arrayend |
Specifies what each array or subarray to be joined should be followed by in the resulting string. arrayend is set by default to a right parenthesis (")"). |
stringsizehint |
Specify a hint to the Join method about the resulting size of the string. This can improve performance if your application is concatenating a large number of string. See the Example section below. |
Returns
A string containing the converted elements of the array.
Example
The following example:
Local array of array of number &AAN;
&AAN = CreateArray(CreateArray(1, 2), CreateArray(3, 4), 5);
&STR = &AAN.Join(", ");
produces in &STR the string:
((1, 2), (3, 4), 5)
The following example makes use of the stringsizehint parameter. The following application class passes the resulting string size hint in the Value property.
class StringBuffer
method StringBuffer(&InitialValue As string, &MaxSize As integer);
method Append(&New As string);
method Reset();
property string Value get set;
property integer Length readonly;
private
instance array of string &Pieces;
instance integer &MaxLength;
end-class;
method StringBuffer
/+ &InitialValue as String, +/
/+ &MaxSize as Integer +/
&Pieces = CreateArray(&InitialValue);
&MaxLength = &MaxSize;
&Length = 0;
end-method;
method Reset
&Pieces = CreateArrayRept("", 0);
&Length = 0;
end-method;
method Append
/+ &New as String +/
Local integer &TempLength = &Length + Len(&New);
If &Length > &MaxLength Then
throw CreateException(0, 0, "Maximum size of StringBuffer exceeded(" | &MaxLength | ")");
End-If;
&Length = &TempLength;
&Pieces.Push(&New);
end-method;
get Value
/+ Returns String +/
Local string &Temp = &Pieces.Join("", "", "", &Length);
/* collapse array now */
&Pieces = CreateArrayRept("", 0);
&Pieces.Push(&Temp); /* start out with this combo string */
Return &Temp;
end-get;
set Value
/+ &NewValue as String +/
/* Ditch our current value */
%This.Reset();
&Pieces.Push(&NewValue);
end-set;
The following code concatenates strings.
While &file.ReadLine(&line)
&S.Append(&line);
&S.Append(&separator);
Syntax
Next(&index)
Description
The Next method increments the given index variable. It returns true if and only if the resulting index variable refers to an existing element of the array. Next is typically used in the condition of a WHILE clause to process a series of array elements up to the end of the array.
&index must be a variable of type integer, or of type Any initialized to an integer, as Next attempts to update it.
If you want to start from the first element of the array, start Next with an index variable with the value zero. The first thing Next does is to increment the value by one.
Parameters
Field or Control |
Definition |
---|---|
&index |
The array element where processing should start. &index must be a variable of type integer, or of type Any initialized to an integer, as Next attempts to update it. |
Returns
True if the resulting index refers to an existing element of the array, False otherwise.
Example
Next can be used in a While loop to iterate through an array in the following manner:
&INDEX = 0;
While &A.Next(&INDEX)
/* Process &A[&INDEX] */
End-While;
In the following code example, &BOTH is a two-dimensional array. This example writes the data from each subarray in &BOTH into a different file.
&I = 0;
While &BOTH.Next(&I)
&J = 1;
&STRING1 = &BOTH[&I][&J];
&MYFILE1.writeline(&STRING1);
&J = &J + 1;
&STRING2 = &BOTH[&I][&J];
&MYFILE2.writeline(&STRING2);
End-While;
Syntax
Pop()
Description
The Pop method removes the last element from the array and returns its value.
Parameters
None.
Returns
The value of the last element of the array. If the last element is a subarray, the subarray is returned.
Example
The Pop method can be used with the Push method to use an array as a stack. To put values on the end of the array, use Push. To take the values back off the end of the array, use Pop.
Suppose we have a two-dimensional array &SUBPARTS which gives the subparts of each part of some assemblies. Each row (subarray) of &SUBPARTS starts with the name of the part, and then has the names of the subparts. Assuming there are no "loops" in this data, the following code puts all the subparts, subsubparts, and so on, of the part given by &PNAME into the array &ALLSUBPARTS, in "depth first order" (that is, subpart1, subparts of subpart1, …, subpart2, subparts of subpart2, …). We stack the indexes into &SUBPARTS when we want to go down to the subsubparts of the current subpart.
Local array of array of string &SUBPARTS;
Local array of string &ALLSUBPARTS;
Local array of array of number &STACK;
Local array of number &CUR;
Local string &SUBNAME;
/* Set the ALLSUBPARTS array to an empty array of string. */
&ALLSUBPARTS = CreateArrayRept("dummy", 0);
/* Start with the part name. */
&STACK = CreateArray(CreateArray(&SUBPARTS.Find(&PNAME), 2));
While &STACK.Len > 0
&CUR = &STACK.Pop();
If &CUR[1] <> 0 And
&CUR[2] <= &SUBPARTS[&CUR[1]].Len Then
/* There is a subpart here. Add it. */
&SUBNAME = &SUBPARTS[&CUR[1], &CUR[2]];
&ALLSUBPARTS.Push(&SUBNAME);
/* Tour its fellow subparts later. */
&STACK.Push(CreateArray(&CUR[1], &CUR[2] + 1));
/* Now tour its subsubparts. */
&STACK.Push(CreateArray(&SUBPARTS.Find(&SUBNAME), 2));
End-If;
End-While;
Syntax
Push(paramlist)
Where paramlist is an arbitrary-length list of values in the form:
value1 [, value2] ...
Description
The Push method adds the values in paramlist onto the end of the array executing the method. If a value is not the correct dimension, it is flattened or promoted to the correct dimension first, then the resulting values are added to the end of the array.
Considerations Using Arrays With Object References
This method only adds an element to the end of an array. It does not clone or otherwise deep-copy the parameters. For example, if you are adding a reference to an object, Push just adds a reference to the object at the end of the array. This is similar to an assignment. It is not making a copy of the object. The following code snippet only puts a reference to the same record onto the end of the array.
While &SQL.Fetch(&Rec);
&MYARRAY.Push(&Rec);
...
End-While;
Even though the array is growing, all the elements point to the same record. You have only as many standalone record objects as you create. The following code snippet creates new standalone records, so each element in the array points to a new object:
local Record &FetchedRec = CreateRecord(Record.PERSONAL_DATA);
While &SQL.Fetch(&FetchedRec)
&MYARRAY.Push(&FetchedRec);
&FetchedRec = CreateRecord(Record.PERSONAL_DATA);
End-While;
Parameters
Field or Control |
Definition |
---|---|
paramlist |
An arbitrary-length list of values, separated by commas. |
Returns
None.
Example
The following example loads an array with data from a database table.
Local array of record &MYARRAY;
Local SQL &SQL;
&I = 1;
&SQL = CreateSQL("Select(:1) from %Table(:1) where EMPLID like ‘8%’", &REC);
While &SQL.Fetch(&REC);
&MYARRAY.Push(CreateRecord(RECORD.PERSONAL_DATA));
&I = &I + 1;
&REC.CopyFieldsTo(&MYARRAY[&I]);
End-While;
Syntax
Replace(start, length[, paramlist])
Where paramlist is an arbitrary-length list of values in the form:
value1 [, value2] ...
Description
Replace replaces the length elements starting at start with the given values, if any. If length is zero, the insertion takes place before the element indicated by start. Otherwise, the replacement starts with start and continues up to and including length, replacing the existing values with paramlist.
If a negative number is used for start, it indicates the starting position relative to the last element in the array, such that −1 indicates the position just after the end of the array. To insert at the end of the array (equivalent to the Push method), use a start of −1 and a length of 0.
If a negative number is used for length, it indicates a length measuring downward to lower indexes. Both flattening and promotion can be applied to change the dimension of the supplied parameters to match the elements of the given array.
Similar to how the built-in function Replace is used to update a string, the Replace method is a general way to update an array, and can cause the array to grow or shrink.
See Using Flattening and Promotion.
Using Replace to Remove an Element
You can use the Replace method to remove an element from an array. Just specify the item you want replaces, with length equal to one.
The following example removes the item from &Index:
&Array.Replace(&Index, 1);
Parameters
Field or Control |
Definition |
---|---|
start |
Specifies where to start replacing the given elements in the array. If a negative number is used for start, it indicates the starting position relative to the last element in the array. |
length |
Specifies the number of elements in the array to be replaced. |
paramlist |
Specifies values to be used to replace existing values in the array. This parameter is optional. |
Returns
None.
Example
For example, given the following array:
Local array of string &AS;
&AS = CreateArray("AA", "BB", "CC");
After executing the next code, the array &AN will contain four elements, ZZ, YY, BB, CC:
&AS.Replace(1, 1, "ZZ", "YY");
After executing the next code, the array &AN will contain three elements, ZZ, MM, CC:
&AS.Replace(2, 2, "MM");
After executing the next code, the array &AN will contain three elements, ZZ, OO, CC.
&AS.Replace( - 2, - 1, "OO");
Image: &AS expanded in PeopleCode debugger
The following image is an example of &AS expanded in PeopleCode debugger.

Syntax
Reverse()
Description
The Reverse method reverses the order of the elements in the array.
If the array is composed of subarrays, the Reverse method reverses only the elements in the super-array, it doesn’t reverse all the elements in the subarrays. For example, the following:
&AN = CreateArray(CreateArray(1, 2), CreateArray(3, 4), CreateArray(5, 6)).reverse();
results in &AN containing:
((5,6), (3,4), (1,2)
Parameters
None.
Returns
None.
Example
Suppose you had the following array.
Local Array of Sting &AS;
&AS = CreateArray("R", "O", "S", "E");
If you executed the Reverse method on this array, the elements would be ESOR.
Syntax
Set(index)
Description
Use the Set method to set the value of the index element of an array. This method is used with the Java PeopleCode functions, instead of using subscripts (which aren't available in Java.)
Using this method is the same as using a subscript to reference an item of an array. In the following example, the two lines of code are identical:
&MyArray[8] = &MyValue;
&MyArray.Set(8) = &MyValue;
Parameters
Field or Control |
Definition |
---|---|
index |
The array element to be accessed. |
Returns
None.
Syntax
Shift()
Description
Use the Shift method to remove the first element from the array and return it. Any following elements are "shifted" to an index of one less than they had.
Parameters
None.
Returns
Returns the value of the first element of the array. If the first element is a subarray, the subarray is returned.
Example
For &I = 1 to &ARRAY.Len;
&ITEM = &ARRAY.Shift;
/* do processing */
End-For;
Syntax
Sort(order)
Description
The Sort method rearranges the elements of the array executing the method into an order.
The type of sort done by this function, that is, whether it is a linguistic or binary sort, is determined by the Sort Order Option on the PeopleTools Options page.
If the array is one-dimensional, the elements are arranged in either ascending or descending order.
The type of the first element is used to determine the kind of comparison to be made. Any attempt to sort an array whose elements are not all of the same type results in an error.
If order is "A", the order is ascending; if it is "D", the order is descending. The comparison between elements is the same one as if done using the PeopleCode comparison operators (<, >, =, and so on.)
Note: If you execute this method on a server, the string sorting order is determined by the character set and localization of the server.
If the array is two-dimensional, the subarrays are arranged in order by the first element of each subarray. Sorting an array whose subarrays have different types of first elements will result in an error. The comparison is done by using the PeopleCode comparison operators (<, >, =, and so on.)
Note: This method works with arrays that have only one or two dimensions. You receive a runtime error if you try to use this method with an array that has more than two dimensions.
Parameters
Field or Control |
Definition |
---|---|
order |
Specifies whether the array should be sorted in ascending or descending order. Values for order are: |
Value |
Description |
---|---|
A |
Ascending |
D |
Descending |
Returns
None.
Example
The following example changes the order of the elements in array &A to be ("Frank", "Harry", "John").
&A = CreateArray("John", "Frank", "Harry");
&A.Sort();
&A = CreateArray(CreateArray("John", 1952), CreateArray("Frank", 1957), CreateArray("Harry", 1928));
Image: &A expanded in PeopleCode debugger
The following example changes the order of the elements in array &A to be (("Frank", 1957), ("Harry", 1928), ("John", 1952)).
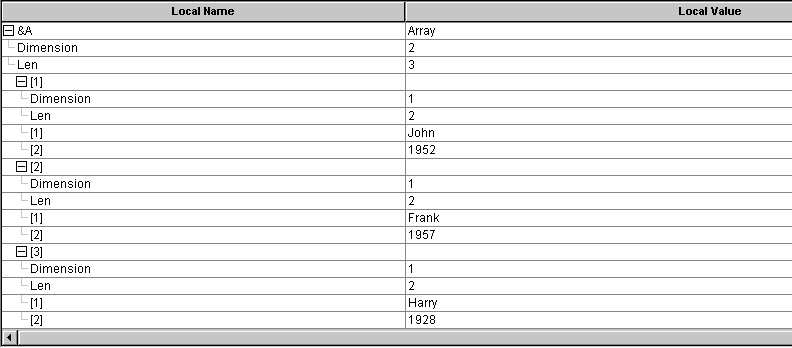
&A.Sort("A");
Image: &A expanded in PeopleCode debugger, showing code results
The following image is an example of &A expanded in PeopleCode debugger, showing code results.
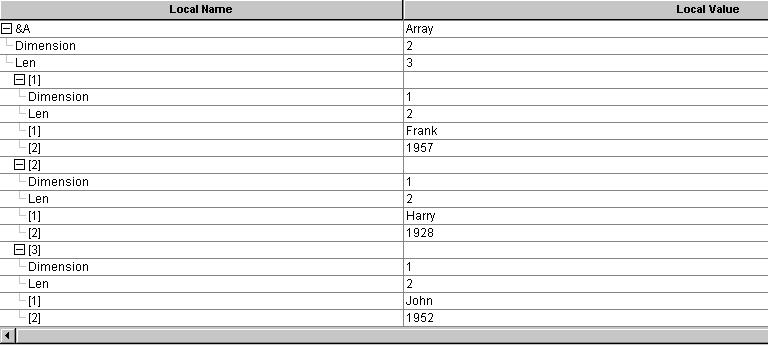
Syntax
Subarray(start, length)
Description
The Subarray method creates a new array from an existing one, taking the elements from start for a total of length. If length if omitted, all elements from start to the end of the array are used.
If the array is multi-dimensional, the subarrays of the created array are references to the same subarrays from the existing array. This means if you make changes to the original subarrays, the referenced subarrays are also changed. To make distinct subarrays, use the Clone method.
Parameters
Field or Control |
Definition |
---|---|
start |
Specifies where in the array to begin the subarray. |
length |
Specifies the number of elements in the array to be part of the subarray. |
Returns
An array object.
Example
To make a distinct array from a multi-dimensional array, use the following:
&A = &AAN.Subarray(1, 2).Clone();
Syntax
Substitute(old_val, new_val)
Description
The Substitute method replaces every occurrence of a value found in an array with a new value. To replace an element that occurs in a specific location in an array, use Replace.
If the array is one-dimensional, Substitute replaces every occurrence of the old_val in the array with new_val.
If the array is two-dimensional, Substitute replaces every subarray whose first element is equal to old_val, with the subarray given by new_val.
Note: This method works with arrays that have only one or two dimensions. You receive a runtime error if you try to use this method with an array that has more than two dimensions.
Parameters
Field or Control |
Definition |
---|---|
old_val |
Specifies the existing value in the array to be replaced. |
new_val |
Specifies the value with which to replace occurrences of old_val. |
Returns
None
Example
The following example changes the array &A to be ("John", "Jane", "Hamilton" ).
&A = CreateArray();
&A[1] = "John";
&A[2] = "Jane";
&A[3] = "Henry";
&A.Substitute("Henry", "Hamilton");
The following example changes the array &A to be (("John", 1952), ("Jane", 1957), ("Hamilton", 1971), ("Frank", 1961)).
&A = CreateArray(CreateArray("John", 1952), CreateArray("Jane", 1957), CreateArray("Henry", 1928), CreateArray("Frank", 1961));
&A.Substitute("Henry", CreateArray("Hamilton", 1971));
Syntax
Unshift(paramlist)
Where paramlist is an arbitrary-length list of values in the form:
value1 [, value2] ...
Description
The Unshift method adds the given elements to the start of the array. Any following elements are moved up to indexes that are larger by the number of values moved. Flattening and Promotion are used to change the dimension of the supplied parameters to be one less than that of the given array.
Parameters
Field or Control |
Definition |
---|---|
paramlist |
Specifies values to be added to the start of the array. |
Returns
None.
Example
The following code changes &A to be ("x", "Y", "a", "B", "c").
&A = CreateArray("a", "B", "c");
&A.Unshift("x", "Y");