8Integrating Siebel Business Applications with Java Applications
Integrating Siebel Business Applications with Java Applications
This chapter discusses the integration of Java applications with Siebel Business Applications. It includes the following topics:
About Siebel Business Applications and Java Applications
Many enterprises develop Java applications to meet a variety of business requirements. Typically, these applications combine existing enterprise information systems with new business functions to deliver services to a broad range of users. Oracle supports integration of its business services and business objects using the Siebel Java Data Bean. The Siebel Java Data Bean can be used for interaction with various kinds of Siebel application objects:
Business objects and business components
Business services and property sets
Integration objects
In all cases, the Java code acts as client-side proxy stub to the corresponding object on the Siebel Server. It does not implement the functionality of the object in Java.
For ease of use, the Siebel Code Generator can be used to produce Java code based on the Siebel Java Data Bean for any specific business service or integration object. This generated code has an API specific to the chosen business service or integration object.
Additionally, Siebel Business Applications support the Java EE Connector Architecture (JCA) with the Siebel Resource Adapter. The Siebel Resource Adapter supports the invocation of business services.
About the JDB Business Object API
The Java Data Bean provides an API to Siebel business objects and their business components. The API is similar in function to the API provided for other platforms, such as COM.
Example of the Business Object and Business Component Interface
Following is a code sample demonstrating use of the business object API. The sample shows how the Java Data Bean might be used to search for a Contact with a particular login name.
The first step in using the Siebel Java Data Bean is to log in to the Object Manager of the Siebel Server. The first parameter, the connection string, specifies the protocol, server name, enterprise name, and Application Object Manager name. Once logged into the Object Manager, the methods getBusObject and getBusComp are used to obtain business objects and their business components.
The code sample activates fields to allow the query to retrieve data for the specific fields, specifies the search criteria, and executes the query. If the query is successful, then the first and last name of the contact are printed to the standard output.
import com.siebel.data.*; public class ObjectInterfaceExample { public static void main(String[] args) throws SiebelException { String connectString = siebel://examplecomputer:2321/siebel/SCCObjMgr_enu"; SiebelDataBean dataBean = new SiebelDataBean(); dataBean.login(connectString, "USER", "PWD", "enu"); SiebelBusObject busObject = dataBean.getBusObject("Contact"); SiebelBusComp busComp = busObject.getBusComp("Contact"); busComp.setViewMode(3); busComp.clearToQuery(); busComp.activateField("First Name"); busComp.activateField("Last Name"); busComp.activateField("Id"); busComp.setSearchSpec("Login Name", "thomas"); busComp.executeQuery2(true,true); if (busComp.firstRecord()) { System.out.println("Contact ID: " + busComp.getFieldValue("Id")); System.out.println("First name: " + busComp.getFieldValue("First Name")); System.out.println("Last name: " + busComp.getFieldValue("Last Name")); } busComp.release(); busObject.release(); dataBean.logoff(); }
If the query results in multiple records, then the record set can be iterated as follows:
if (busComp.firstRecord()) { // obtain the fields/values from this record while (busComp.nextRecord()){ // obtain the fields/values from the next record } }
About the JDB Business Service API
Aside from the business object and business component API, the primary point of integration with the Siebel application is by using business services.
There are several ways to invoke a business service. The simplest way is using the Siebel Java Data Bean directly, as shown in the following example. Alternatively, Siebel Tools provides a Code Generator which creates, for any business service, Java classes that invoke the business service. The generated code can invoke the business service either using the Siebel Java Data Bean or using the Siebel Resource Adapter. The creation and use of generated code is described in the next topic. The Siebel Resource Adapter is part of the Java EE Connector Architecture, which is described in About the Siebel Resource Adapter.
The following is an example of invoking a business service directly using the Siebel Java Data Bean.
import com.siebel.data.SiebelDataBean; import com.siebel.data.SiebelException; import com.siebel.data.SiebelPropertySet; import com.siebel.data.SiebelService; public class BasicDataBeanTest { public static void main(String[] args) throws SiebelException { SiebelDataBean dataBean = new SiebelDataBean(); dataBean.login("siebel://examplecomputer:2321/siebel/SCCObjMgr_enu", "USER", "PWD", "enu"); SiebelService businessService = dataBean.getService("Workflow Utilities"); SiebelPropertySet input = new SiebelPropertySet(); SiebelPropertySet output = new SiebelPropertySet(); input.setValue("Please echo this"); businessService.invokeMethod("Echo", input, output); System.out.println("Output: " + output.toString()); } }
About the Siebel Code Generator
JavaBeans for invoking a particular business service can be generated using the Siebel Code Generator. These JavaBeans provide a uniform mechanism for interacting with the Siebel application from a Java or Java EE application. The JavaBean for a particular business service provides facilities for creating inputs and invoking methods. The JavaBean representing a business service can be based on either the Siebel Java Data Bean or on the Siebel Java EE Connector Architecture (JCA) Resource Adapter.
For business services whose methods have integration objects as input or output, JavaBeans representing the integration objects must be generated separately. These beans provide facilities for creating the integration objects and setting their fields.
The business services most commonly used for integration are EAI Siebel Adapter and various ASI business services based on the data sync service. The methods of these business services typically have inputs and outputs that are property sets of a special type called integration objects. Siebel Java integration provides special support for working with integration objects.
The following Siebel Code Generator topics are also discussed:
Invoking the Siebel Code Generator
This topic describes how to invoke the Siebel Code Generator to create JavaBeans for either a Siebel business service or a Siebel integration object.
To invoke the Siebel Code Generator
Start Siebel Tools.
Note: For information about how to use Siebel Tools, see Using Siebel Tools.Select Business Service or Integration Object in the Object Explorer.
Note: If Integration Object is not present, then add it by checking Integration Object on the Object Explorer tab of the Development Tools Options window opened by selecting View, then Options.Select the desired business service or integration object.
For example, at the first section of the Integration Object list, there is a set of three buttons: Synchronize, Generate Schema, and Generate Code.
Click Generate Code.
Complete the Code Generator wizard:
Leave the business service as is. There is only one available, the Siebel Code Generator.
Select either Java(JDB) (Java Data Bean) or Java(JCA) (Java EE Connector Architecture/Siebel Resource Adapter) for the Supported Language.
Browse to select an existing folder as the output folder. Your Java code for the selected business services or integration objects is stored in subdirectories there, as explained next.
Click Finish.
The code is generated and the wizard closes, returning you to the Business Service or Integration Object form.
Code Generated for a Business Service
The code generated for a business service includes a class representing the business service itself as well as classes representing inputs and outputs of its methods. These classes are described in detail in this topic.
ASI business services based on the data sync service have integration objects as part of the input or output of their methods. The JavaBeans representing these integration objects must be generated separately from the business service.
The classes for a given business service reside in a package in one of the following:
com.siebel.service.jdb.business service name or
com.siebel.service.jca.business service name
Depending on whether the beans are based on the Java Data Bean or the Siebel JCA Resource Adapter. For example, generated JDB code for the EAI Siebel Adapter resides in the package com.siebel.service.jdb.eaisiebeladapter.
The Code Generator creates the standard Java directory structure reflecting the package structure. As shown in the following image, a subfolder named com
is created in the folder specified during the generation process. The com
folder contains a folder named siebel
, which in turn contains a folder named service
. Under the service
is a folder named jdb
(or jca
), containing a folder named for the business service. This last folder contains the classes for the business service. Each class is defined in its own file. The folder created under jdb
(or jca
) for every business service generated contains several Java files.
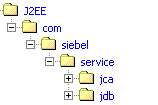
One Java class is generated to represent the business service itself. The name of the class is the name of the business service with all special characters replaced by underscores (_) and BusServAdapter appended to the end. For example, the class representing EAI Siebel Adapter is EAI_Siebel_AdapterBusServAdapter.
The Java class has one method for each method of the business service. Its name is the name of the method with m prefixed. For code based on the Java Data Bean, the class is a subclass of com.siebel.integration.adapter.SiebelJDBAdapterBase. For code based on the Siebel Resource Adapter, the class is a subclass of com.siebel.integration.adapter.SiebelJCAAdapterBase.
Additionally, for each method of the business service defined in Siebel Tools, one Java class is created for the method's input and one for the method's output. The name of the class is the name of the method with Input or Output appended. The class encapsulates all input (or output) arguments for the method. Each argument is represented as a field whose name is that of the argument with f prefixed. For each field, public set and get methods are provided Java methods for reading and writing their values.
For example, the business service CC XML Converter, which has two methods, PropSetToXML and XMLToPropSet, generates the following four classes:
CC_XML_Converter BusServiceAdapter
PropSetToXMLInput
PropSetToXMLOutput
XMLToPropSetInput
The first class, CC_XML_Converter BusServiceAdapter, represents the business service as a whole; it has methods mPropSetToXML and mXMLToPropSet. The other three classes represent the input or output parameters of the two methods. (Notice there is no class XMLToPropSetOutput because that method has no outputs.) Those three classes each have methods to read and write the individual parameters, as well as methods to convert to and from a com.siebel.data.SiebelPropertySet.
About Methods of Java Classes Generated for a Business Service
The tables in the following topics describe the methods that are present in the generated Java code for every business service. Generic names (for example, GenericService and GenericMethod) are substituted for the actual names of the business service, methods, and arguments.
Methods for Java class com.siebel.service.jdb.GenericServiceBusServAdapter
The following table lists the methods in the Java class com.siebel.service.jdb.GenericServiceBusServAdapter generated for an example business service, GenericService, that has the business service method GenericMethod.
Method | Description |
---|---|
GenericServiceBusServAdapter() |
Constructor that uses the default properties file, siebel.properties. |
GenericServiceBusServAdapter(SiebelDataBean) |
Constructor that reuses the resources of an existing SiebelDataBean. |
GenericServiceBusServAdapter(String) |
Constructor taking the name of the properties file to use. |
GenericServiceBusServAdapter(String, String, String) |
Constructor taking the username, password, and connect string. |
GenericServiceBusServAdapter(String, String, String, String) |
Constructor taking the username, password, connect string, and language. |
GenericMethod(GenericMethodInput) |
Invokes the specified business service method. |
Methods for Java class com.siebel.service.jdb.GenericMethodInput
The following table lists the methods in the Java class com.siebel.service.jdb.GenericMethodInput generated for an example business service method, GenericMethod.
Method | Description |
---|---|
GenericMethodInput() |
Constructor. |
GenericMethodInput(SiebelPropertySet) |
Constructor that sets its fields from the given property set. |
fromPropertySet(SiebelPropertySet) |
Copies field values from the given property set. |
toPropertySet() |
Returns a SiebelPropertySet with the properties and values corresponding to the fields of this object. |
getfGenericArgument() |
Returns the value of business service method argument. |
setfGenericArgument(String) |
Sets the value of a business service method argument. |
Methods for Java class com.siebel.service.jdb.GenericMethodOutput Methods
The following table lists the methods in the Java class com.siebel.service.jdb.GenericMethodOutput generated for an example business service method, GenericMethod.
Method | Description |
---|---|
GenericMethodOutput() |
Constructor. |
GenericMethodOutput(SiebelPropertySet) |
Constructor that sets its fields from the given property set. |
fromPropertySet(SiebelPropertySet) |
Copies field values from the given property set. |
toPropertySet() |
Returns a SiebelPropertySet with the properties and values corresponding to the fields of this object. |
getfGenericArgument () |
Returns the value of business service method argument. |
setfGenericArgument () |
Sets the value of a business service method argument. |
About the Code Generated for an Integration Object
Integration objects are special kinds of property sets that are the input and output of business services based on the data sync service. JavaBeans based on integration objects are designed to be used with those business services or with the EAI Siebel Adapter and can be used to query, delete, upsert, and synchronize information in the Siebel Server's database.
The integration object, and each of its components, has its own Java class, stored in the package com.siebel.local.IntegrationObjectName. The class for the integration object has IO appended to the end, and the class for an integration component has IC appended. The Code Generator creates the standard Java directory structure reflecting the package structure. In the selected folder, a subfolder named com
is created, containing a subfolder siebel
, containing a subfolder local
, which contains one subfolder for each integration object that was generated. The Java files are stored in the lowest directory. This structure is shown in the following image. One folder is created under local
for each integration object that is generated. The folder that is created contains all the Java files for that integration object.
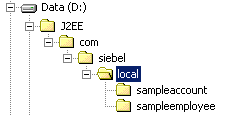
For example, the integration object Sample Account; which has five components Account, Account Attachment, Account_Organization, Business Address, and Contact; generates the following six classes:
Sample_AcccountIO
AccountIC
Account_AttachmentIC
Account_OrganizationIC
Business_AddressIC
ContactIC
The first class, suffixed with IO, represents the entire integration object. It has methods to construct the object, to read and write fields, to add integration object components, and to convert to and from a SiebelPropertySet. The other five classes, suffixed with IC, represent the individual integration object components and provide methods that are for constructing the component to read and write fields and to convert to and from a SiebelPropertySet.
Methods of Java Classes Generated for an Integration Object
The following table describes the methods that are present in the generated Java code for every integration object, using the example integration object GenericIntObj.
Object | Description |
---|---|
addfintObjInst(SiebelHierarchy) |
Adds an integration object component object to the integration object. |
clone |
Returns a copy of the integration object. |
equals(Object) |
Determines whether integration object has the same data as the integration object passed. |
fromPropertySet(SiebelPropertySet) |
Copies the data from the given property set to the integration object. |
getfIntObjectFormat |
Returns a String containing the format of the integration object. |
getfIntObjectName |
Returns the integration object name property. |
getfintObjInst |
Returns a Vector representation of the integration object. |
getfMessageId |
Returns the MessageId property of the integration object. |
getfMessageType |
Returns the MessageType property of the integration object. |
getfOutputIntObjectName |
Returns the OutputIntObjectName property of the integration object. |
Generic_ObjectIO() |
Default constructor. |
Generic_ObjectIO(SiebelPropertySet ps) |
Creates an integration object (and its hierarchy) based on a property set. |
setfIntObjectFormat |
Sets the IntObjectFormat property of the integration object. |
setfIntObjectName |
Sets the IntObjectName property of the integration object. |
setfMessageId |
Sets the MessageId property of the integration object. |
setfMessageType |
Sets the MessageType property of the integration object. |
setfOutputIntObjectName |
Sets the OutputIntObjectName property of the integration object. |
toPropertySet |
Returns a SiebelPropertySet representation of the integration object. |
Methods of Java Classes Generated for an Integration Object Component
The following table describes the methods that are present in the generated Java code for every integration object component, using an example integration object component, GenericIntComp, that has the child components GenericIntCompChild and field GenericField.
Object Component | Description |
---|---|
addfGenericIntCompChildIC(GenericIntCompChildIC) |
Adds to the integration object component the given child integration object component. |
clone |
Returns a copy of the integration object. |
equals(Object) |
Determines whether the integration object component has the same data as the passed integration object component. |
fromPropertySet(SiebelPropertySet) |
Populates the integration object component based upon the contents of a property set. |
getfGenericIntCompChildIC |
Returns a Vector containing all child integration object components of type ChildIntObjComp associated with the integration object component. |
getfGenericField() |
Returns the value of the field GenericField. |
GenericIntCompIC() |
Default constructor. |
GenericIntCompIC(SiebelPropertySet) |
Creates an integration object component from a property set. |
setfGenericField(val) |
Sets the value of the field GenericField. |
toPropertySet |
Returns a property set representation of the integration object component. |
About Running the Java Data Bean
Two Siebel .jar files are needed to compile and run a Java application that uses the Java Data Bean:
Siebel.jar
SiebelJI_lang.jar (lang is the installed language pack; for example, SiebelJI_enu.jar for English or SiebelJI_jpn.jar for Japanese.)
These jar files are provided with the standard Siebel installation under the directory INSTALLED_DIR\classes
.
Documentation of individual classes is provided in the form of javadoc (Siebel_JavaDoc.jar), which is installed when installation option Siebel Java Integrator (a component of the Siebel Tools or the Siebel Server installer) is chosen. This .jar file contains the up-to-date javadoc for the Siebel Java Data Bean, Siebel Resource Adapter, and dependent classes.
Connect String and Credentials for the SiebelDataBean
When using the SiebelDataBean directly, without any generated code, three arguments must be passed to the login method. A fourth argument, language code, is optional.
connect string
Siebel username
Siebel password
language code (default is enu)
The connect string has the following form:
siebel://SiebelServerName:SCBPort/EnterpriseName/XXXObjMgr_lang
For example:
siebel://examplecomputer:2321/mysiebelenterprise/SCCObjMgr_enu
When using generated code, these parameters can be taken from the siebel.properties file, which must be in the classpath of the Java Virtual Machine (JVM). These properties are read from siebel.properties at the time an instance of the generated business service class is created using that explicitly specifies siebel.properties, for example:
Siebel_AccountBusServAdapter svc = new Siebel_AccountBusServAdapter("siebel.properties");
They can be overridden by calling the methods setConnectString, setUserName, setPassword, and setLanguage any time prior to calling initialize() or invoking a business service method (such as GenericMethod in Methods for Java class com.siebel.service.jdb.GenericServiceBusServAdapter). This is the behavior when the default (no-argument) constructor of the generated Java class is used.
Alternatively, the generated class provides the following four constructors with arguments:
One String argument: the name of the property file to be used.
Three String arguments: the connect string, username, and password. No properties file is used.
Four String arguments: the connect string, username, password, and language. No properties file is used.
SiebelDataBean argument: the SiebelDataBean passed already has parameters assigned and its login method executed.
Connection Parameters for the SiebelDataBean
Regardless of how the SiebelDataBean is invoked, certain parameters of the connection can be set using the properties file. These are siebel.conmgr.txtimeout, siebel.conmgr.poolsize, siebel.conmgr.sesstimeout, siebel.conmgr.retry, and siebel.conmgr.jce.
Other connection parameters can also be specified in the properties file, but they are used only in conjunction with generated code (subclasses of com.siebel.integration.adapter.SiebelJDBAdapterBase or SiebelJCAAdapterBase).
Property | Description |
---|---|
siebel.conmgr.txtimeout |
The number of milliseconds to wait after sending a request to the Siebel Server. Must be a positive integer; other values are ignored. The default value is 600000 milliseconds (10 minutes); the maximum value is 2,147,483,647 ms (approximately 25 days). |
siebel.conmgr.poolsize |
For each Application Object Manager process, a pool of open connections is maintained and shared by all users of that process. This parameter specifies the maximum number of connections that are stored in the pool. Its value must be a positive integer less than 500; other values are ignored. The default is 2. |
siebel.conmgr.sesstimeout |
The number of seconds the Siebel Server waits before disconnecting an idle client session. Its value must be a nonnegative integer. The default is 2700 seconds (45 minutes); the maximum value is 2,147,483,647 s (approximately 68 years). |
siebel.conmgr.jce |
Determines whether encryption of transmissions is done using Java Cryptography Extension (JCE) or RSA (if the connection uses encryption). 1 indicates JCE; 0 indicates RSA. The default is 0. |
siebel.conmgr.retry |
The number of attempts to be made at establishing a connection (opening a session) before giving up. Must be a positive integer. The default is 3. |
siebel.conmgr.virtualhosts |
A listing of virtual servers representing a group of like servers that perform the same function, for example, call center functions. An incoming login for the call center virtual server tries servers from the list in a round-robin fashion. An example of such a list follows: VirtualServer1=sid1:host:port,sid2:host:port...; VirtualServer2=... where VirtualServer1, VirtualServer2, and so on, are assigned lists of real Siebel Servers with host names and port numbers (of the local SCBroker component). |
siebel.connection.string |
The Siebel connect string. For information about the syntax of the connect string, see Siebel Object Interfaces Reference. |
siebel.loglevel |
The level of messages to be logged. Must be a positive integer less than 6. Other values are ignored or throw an exception. 0 causes only FATAL messages to be logged; 1 ERROR; 2 WARN; 3 INFO; 4 DETAIL; 5 DEBUG. The default is 0.
Note: The siebel.loglevel parameter is used only in conjunction with the generated code for the SiebelJCAAdapterBase subclass.
|
siebel.logfile |
The name of a file to which logging is directed. Strings that cause a FileNotFoundException cause an error to be logged and are ignored. The default is to print to the JVM’s standard output.
Note: The siebel.logfile parameter is used only in conjunction with the generated code for the SiebelJCAAdapterBase subclass.
|
siebel.user.name |
The Siebel username to be used for logging in to the Application Object Manager. |
siebel.user.password |
The Siebel password to be used for logging in to the Application Object Manager. |
siebel.user.language |
The language code indicating the natural language to be used for messages and other strings. Default is enu. |
siebel.jdb.classname |
The name of a subclass of com.siebel.data.SiebelDataBean to use instead of SiebelDataBean. Strings that do not specify a valid class or specify a class that is not a subclass of SiebelDataBean cause an error log to be logged and SiebelDataBean to be used instead. |
Here is a sample siebel.properties file:
siebel.connection.string = siebel://examplecomputer:2321/siebel/EAIObjMgr_enu siebel.user.name = User1 siebel.user.password = password siebel.user.language = enu siebel.user.encrypted = false siebel.conmgr.txtimeout = 300000 siebel.conmgr.poolsize = 5 siebel.conmgr.sesstimeout = 3600 siebel.conmgr.retry = 5 siebel.conmgr.jce = 1 siebel.loglevel = 0
Examples Using Generated Code for Integration Objects
The following code examples use the code generation facilities provided in Siebel Tools. For more information, see About the Siebel Code Generator, for both business services and integration objects. By using the code generation facilities, many of the complexities of the Siebel property sets and business service interfaces have been abstracted, providing a standards-based JavaBean interface.
Siebel Account Business Service Example
The following is a code sample invoking the QueryByExample method of the Siebel Account business service. In addition to the generated code for Siebel Account (resident in com.siebel.service.jdb.siebelaccount), the sample uses the generated code for the Account Interface integration object (resident in com.siebel.local.accountinterface).
The code invokes the QueryByExample method of the Siebel Account business service. The parameter to this method is formed from an instance of the Account Interface integration object, which serves as the example, essentially specifying a search criterion of all accounts that start with the letters Ai. The output integration object is converted to a Vector and iterated through to print the names of matching accounts.
import com.siebel.data.SiebelDataBean; import com.siebel.data.SiebelException; import com.siebel.service.jdb.siebelaccount.Siebel_AccountBusServAdapter; import com.siebel.service.jdb.siebelaccount.QueryByExampleInput; import com.siebel.service.jdb.siebelaccount.QueryByExampleOutput; import com.siebel.local.accountinterface.Account_InterfaceIO; import com.siebel.local.accountinterface.AccountIC; public class JDBSiebelAccount { public static void main(String[] args) throws SiebelException { Siebel_AccountBusServAdapter svc = new Siebel_AccountBusServAdapter("USER", "PWD","siebel://examplecomputer:2321/siebel/SCCObjMgr_enu","enu"); // Create the example-accounts starting with "Ai": AccountIC acctIC = new AccountIC(); Account_InterfaceIO acctIO = new Account_InterfaceIO(); acctIO.addfintObjInst(acctIC); acctIC.setfName("Ai*"); QueryByExampleInput qbeIn = new QueryByExampleInput(); qbeIn.setfSiebelMessage(acctIO); // Call QueryByExample QueryByExampleOutput qbeOut = svc.mQueryByExample(qbeIn); acctIO = new Account_InterfaceIO(qbeOut.getfSiebelMessage().toPropertySet()); Vector ioc = acctIO.getfintObjInst(); // print the name of each account returned: if (!ioc.isEmpty()) { for(int i=0; i < ioc.size(); i++) { acctIC = (AccountIC) ioc.get(i); System.out.println(acctIC.getfName()); } } }
EAI Siebel Adapter Business Service Example
The following example uses the generated code for the EAI Siebel Adapter business service. An instance is instantiated using the constructor that takes an instance of SiebelDataBean. The QueryPage method is called; its output is actually an Account Interface integration object, but the object returned is not strongly typed and instead is used to construct an Account Interface instance. The generated code for Account Interface is also needed for this example.
import com.siebel.data.SiebelDataBean; import com.siebel.data.SiebelException; import com.siebel.local.accountinterface.Account_InterfaceIO; import com.siebel.local.accountinterface.AccountIC; import com.siebel.service.jdb.eaisiebeladapter.EAI_Siebel_AdapterBusServAdapter; import com.siebel.service.jdb.eaisiebeladapter.QueryPageInput; import com.siebel.service.jdb.eaisiebeladapter.QueryPageOutput; public class DataBeanDemo { public static void main(String[] args) throws SiebelException { SiebelDataBean m_dataBean = new SiebelDataBean(); String conn = "siebel://examplecomputer:2321/siebel/SCCObjMgr_enu"; m_dataBean.login(conn, "USER", "PWD", "enu"); // Construct the EAI Siebel Adapter, using the data bean EAI_Siebel_AdapterBusServAdapter svc = new EAI_Siebel_AdapterBusServAdapter(m_dataBean); svc.initialize(); try { // Set values of the arguments to the QueryPage method. QueryPageInput qpInput = new QueryPageInput(); qpInput.setfPageSize(Integer.toString(10)); // Return 10 records. qpInput.setfOutputIntObjectName("Account Interface"); qpInput.setfStartRowNum(Integer.toString(0)); // Start at record 0. QueryPageOutput qpOutput = svc.mQueryPage(qpInput); // Construct the integration object using the QueryPage output Account_InterfaceIO acctIO = new Account_InterfaceIO(qpOutput.getfSiebelMessage().toPropertySet()); // Convert the results to a vector for processing Vector ioc = acctIO.getfintObjInst(); // Print name of each account if (!ioc.isEmpty()) { for (int i = 0; i < ioc.size(); i++) { AccountIC acctIC = ((AccountIC) ioc.get(i)); System.out.println(acctIC.getfName()); } } } catch (SiebelException e) {} finally { m_dataBean.logoff(); } } }
About the Siebel Resource Adapter
The Siebel Resource Adapter is for use within the Java EE Connector Architecture (JCA) by Java EE-based applications (EJBs, JSPs, servlets) that are deployed on containers. JCA provides clients with a standard interface to multiple enterprise information services such as the Siebel application.
The Siebel Resource Adapter implements system-level contracts that allow a standard Java EE application server to perform services such as pooling connections and managing security. This is referred to as operation within a managed environment.
The Java EE Connection Architecture also provides for operation in a nonmanaged environment, where the client need not be deployed in a Java EE container, but instead uses the adapter directly. In this case, the client takes responsibility for services such as managing security.
The Siebel Resource Adapter has transaction support level NoTransaction. This means that the Siebel Resource Adapter does not support local or JTA transactions. For more information about JCA, see:
http://jcp.org/en/jsr/detail?id=322
The following Siebel Resource Adapter topics are also discussed:
Using the Resource Adapter
When deploying the Siebel Resource Adapter to a Java EE application server (for example, Oracle Application Server, Oracle WebLogic Server, or IBM WebSphere MQ), you must make sure that the necessary Siebel JAR files are included. The Siebel JAR files that must be added to the classpath are:
SiebelJI.jar
SiebelJI_lang.jar (lang is the installed language pack; for example, SiebelJI_enu.jar for English or SiebelJI_jpn.jar for Japanese.)
The resource adapter archive, or RAR file, might also be required for deployment. Refer to the documentation of the Java EE application server for more information about deploying a JCA adapter on the server.
The following topics contain code samples for both managed and nonmanaged environments.
About the Connect String and Credentials for the Java Connector
The Java Connector Architecture allows for credentials to be supplied using either Container-Managed Sign-on or Application-Managed Sign-On.
With Container-Managed Sign-On, the application server's container identifies the principal and passes it to the JCA adapter in the form of a JAAS Subject. Application servers provide their own system of users and roles; such a user must be mapped to Siebel user and password for the purpose of the JCA adapter. Application servers allow the specification of such mappings. With Container-Managed Sign-On, the Siebel connect string and language must be specified in the deployment descriptor of the adapter (ra.xml). If a Siebel user name and password are present in the descriptor, then they are used by the application server only to create an initial connection to the Siebel application when the application server is started, which is not necessary.
With Application-Managed Sign-On, the client application must provide the credentials and connect string. This is done just as for the Java Data Bean, as described in About Running the Java Data Bean, by either supplying them in siebel.properties or setting them programmatically using setUserName, setPassword, setConnectString, and setLanguage. If any of these parameters are supplied using Application-Managed Sign-On, then supply all four of them in that manner.
Managed Code Sample Using the Siebel Resource Adapter
The following is a code sample using the Siebel Resource Adapter in a managed environment. The sample is a servlet that makes a simple invocation to a business service using the generated JCA code. (For more information about generating code, see About the Siebel Code Generator.)
The JCA ConnectionFactory is obtained through JNDI. Credentials are obtained at run time from the JAAS Subject passed to the servlet. The connect string and language are obtained from the deployment descriptor (ra.xml). Other connection parameters are obtained from the siebel.properties file.
import javax.naming.*; import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import com.siebel.integration.jca.cci.SiebelConnectionFactory; import com.siebel.service.jca.eaifiletransport.*; public class ManagedConnectionServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException,ServletException { PrintWriter reply = response.getWriter(); try { // Specify siebel.properties in the constructor. EAI_File_TransportBusServAdapter bs = new EAI_File_TransportBusServAdapter("siebel.properties"?); InitialContext jndi = new InitialContext(); SiebelConnectionFactory scf = (SiebelConnectionFactory)jndi.lookup("siebelJCA"); bs.setConnectionFactory(scf); // Username and password obtained from JAAS Subject passed by server at runtime. // Connect string and language obtained from deployment descriptor, ra.xml. ReceiveInput input = new ReceiveInput(); input.setfCharSetConversion("UTF-8"); input.setfFileName("D:\\helloWorld.txt"); ReceiveOutput output = bs.mReceive(input); reply.println(output.getf_Value_()); } catch (Exception e) { reply.println("Exception:" + e.getMessage()); } } }
Nonmanaged Code Sample Using the Siebel Resource Adapter
The following is a code sample using the Siebel Resource Adapter in a nonmanaged environment. The sample performs the same function as the Managed sample; it is a servlet that makes a simple invocation to a business service using the generated JCA code. (For more information about generating code, see About the Siebel Code Generator.)
The JCA ConnectionFactory is created directly. The username, password, connect string, and language are obtained from siebel.properties or set programmatically. Other connection parameters are obtained from the siebel.properties file.
import java.io.*; import javax.servlet.*; import javax.servlet.http.*; import com.siebel.integration.jca.cci.notx.SiebelNoTxConnectionFactory; import com.siebel.service.jca.eaifiletransport.*; public class BookshelfNonManagedConnectionSample extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { PrintWriter reply = response.getWriter(); try { EAI_File_TransportBusServAdapter bs = new EAI_File_TransportBusServAdapter(“siebel.properties�?); bs.setConnectionFactory(new SiebelNoTxConnectionFactory()); // Username, password, connect string, and language are read from // siebel.properties, which must be in the classpath of the servlet // and be specified in the constructor. // Alternatively, they can be set here programmatically: // bs.setUserName("USER"); // bs.setPassword("PWD"); // bs.setConnectString("siebel://examplecomputer:2321/siebel/ SCCObjMgr_enu"); ReceiveInput input = new ReceiveInput(); input.setfCharSetConversion("UTF-8"); input.setfFileName("D:\\helloWorld.txt"); ReceiveOutput output = bs.mReceive(input); reply.println(output.getf_Value_()); } catch (Exception e) { reply.println("Exception:" + e.getMessage()); } } }
About JCA Logging
The following improvements have been made to JCA logging in Oracle’s Siebel CRM version 8.0 and later:
Appending JCA logs to one file, which is found in the working directory of the JVM.
Previously, each JCA thread would overwrite the same log file over and over again. Now all JCA threads log into one file. When the log file size exceeds 100 MB, it is renamed and a new one is started. For example, test.log is renamed to test_1166581351656.log, where the value is the number of milliseconds since 1970.
Proper logging of call stacks for LOG_DEBUG.
Previously, JCA log events in the LOG_DEBUG level (level 5) logged the call stack, but the call stack was often incomplete and cryptic. Now the call stack is a complete Java call stack.
Logging of thread names.
Previously, the JCA logs did not include the thread name. Now that all threads log to one file, each line contains the thread name. An example of a line in the log file is:
[SIEBEL INFO] Thread[Servlet.Engine.Transports : 4,5,main] [2010-11-04 15:58:38.058] [SiebelManagedConnection(2137125295)] Cleaning up 0 handles on SiebelManagedConnection(2137125295)
New logging in LOG_DETAIL (level 4):
When a listener thread is created (logs the host and port):
[SIEBEL DETAIL] Thread[Thread-1482,5,main] [2010-11-04 16:12:10.139] [] creating socket for listening thread: host=xyz port=9312
When the main thread sends a request to the Siebel Server (logs the packet number):
[SIEBEL DETAIL] Thread[Thread-1482,5,main] [2010-11-04 16:12:56.521] [] set tx=2813 [SIEBEL DETAIL] Thread[Thread-1482,5,main] [2010-11-04 16:12:56.521] [] wait=1 tx=2813
When the main thread receives a response:
[SIEBEL DETAIL] Thread[Thread-1482,5,main] [2010-11-04 16:12:56.580] [] end loop tx=2813 isDone
Before the listener thread reads a packet (logs the number of bytes in the packet):
[SIEBEL DETAIL] Thread[Thread-54,5,Listener Threads] [2010-11-04 16:12:56.575] [] about to read to bytes: len=1800
As the listener thread reads the packet (logs the packet number and number of bytes read thus far):
[SIEBEL DETAIL] Thread[Thread-54,5,Listener Threads] [2010-11-04 16:12:56.575] [] read some bytes: tx=2813 len=1800 read=1800
Logging call stacks when opening and closing a connection to the Siebel Server.
Previously, the JCA logs for LOG_INFO (level 3) logged the opening and closing of a connection, but did not log the call stack. Now the call stack is logged, for example:
[SIEBEL INFO] Thread[Servlet.Engine.Transports : 2,5,main] [2010-11-05 07:53:26.078] [SiebelConnection(507473761)] Opening a new connection to Siebel ... java.lang.Throwable at com.siebel.integration.util.a.trace(Unknown Source) at com.siebel.integration.util.SiebelTrace.trace(Unknown Source) at com.siebel.integration.jca.cci.SiebelConnection.a(Unknown Source) at com.siebel.integration.jca.cci.SiebelConnection.initialize(Unknown Source) at com.siebel.integration.jca.cci.SiebelConnection.<init>(Unknown Source) at com.siebel.integration.jca.cci.notx.SiebelNoTxConnection.<init>(Unknown Source) at com.siebel.integration.jca.spi.notx.SiebelNoTxManagedConnectionFactory.createManagedConnection(Unknown Source) at com.ibm.ejs.j2c.poolmanager.FreePool.createManagedConnectionWithMCWrapper(FreePool.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.FreePool.createOrWaitForConnection(FreePool.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.PoolManager.reserve(PoolManager.java(Compiled Code)) at com.ibm.ejs.j2c.ConnectionManager.allocateMCWrapper(ConnectionManager.java(Compiled Code)) at com.ibm.ejs.j2c.ConnectionManager.allocateConnection(ConnectionManager.java(Compiled Code)) at com.siebel.integration.jca.cci.SiebelConnectionFactory.getConnection(Unknown Source) at com.siebel.integration.adapter.SiebelJCAAdapterBase.invoke(SiebelJCAAdapterBase.java(Compiled Code)) ... [SIEBEL INFO] Thread[Servlet.Engine.Transports : 2,5,main] [2010-11-05 07:53:26.243] [SiebelConnection(507473761)] Opened a new connection to Siebel (Siebel session : siebel.tcpip.none.none://myserver.example.com:2321/esblp01/SCCObjMgr_enu/!10.6373.3ba70.465c2246) [SIEBEL INFO] Thread[Thread-56,5,main] [2010-11-05 07:54:38.484] [SiebelConnection(974516018)] Closing the connection java.lang.Throwable at com.siebel.integration.util.a.trace(Unknown Source) at com.siebel.integration.util.SiebelTrace.trace(Unknown Source) at com.siebel.integration.jca.cci.SiebelConnection.a(Unknown Source) at com.siebel.integration.jca.cci.SiebelConnection.close(Unknown Source) at com.siebel.integration.jca.spi.SiebelManagedConnection.destroy(Unknown Source) at com.ibm.ejs.j2c.MCWrapper.destroy(MCWrapper.java:1380) at com.ibm.ejs.j2c.poolmanager.FreePool.cleanupAndDestroyMCWrapper(FreePool.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.PoolManager.reclaimConnections(PoolManager.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.PoolManager.executeTask(PoolManager.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.TaskTimer.executeTask(TaskTimer.java(Compiled Code)) at com.ibm.ejs.j2c.poolmanager.TaskTimer.run(TaskTimer.java:113)
Logging execution of a request in LOG_INFO (level 3).
Previously, execution of a request was logged in LOG_DEBUG. Now the request is logged in LOG_INFO with no call stack, for example:
[SIEBEL INFO] Thread[Servlet.Engine.Transports : 2,5,main] [2010-11-05 07:53:26.244] [SiebelConnection(507473761)] Executing com.siebel.integration.jca.client.SiebelInteractionSpec@1b6bef7c
Mapping a JCA Thread to a Siebel Server Task and Log File
From the JCA logging information, you can find the Siebel Server task and log file, which can be useful in diagnosing threads that use large amounts of CPU time.
To map a JCA thread to a Siebel Server task and log file
Examine the JCA log file to find the high-CPU thread, for example:
[SIEBEL INFO] Thread[Servlet.Engine.Transports : 2,5,main] [2010-11-05 07:53:26.243] [SiebelConnection(507473761)] Opened a new connection to Siebel (Siebel session : siebel.tcpip.none.none://myserver.example.com:2321/esblp01/ SCCObjMgr_enu/!10.6373.3ba70.465c2246)
The Siebel session URL takes the following form:
siebel[.transport][.encryption][.compression]://host[:port]/EnterpriseServer/ AppObjMgr_lang/!AppObjMgrID.ProcessID.TaskID.timestamp
where the Application Object Manager ID, process ID, task ID, and timestamp are represented by hexadecimal numbers.
Use the Siebel session URL to find the following parameters, converting hexadecimal numbers to decimal:
Parameter Example Host
myserver.example.com
Siebel Enterprise Server
esblp01
Application Object Manager_lang
SCCObjMgr_enu
Application Object Manager ID
10 (16 decimal)
Task ID
3ba70 (244336 decimal)
Find the corresponding Siebel Server log file, which is in the
SIEBEL_SERVER_ROOT/log
directory:Windows:
AppObjMgr_lang_AppObjMgrID_taskID.log
For example:
SCCObjMgr_enu_0016_244336.log
UNIX:
AppObjMgr_lang_taskID.log
For example:
SCCObjMgr_enu_244336.log