Document Generator Tags
A "tag" refers to an expression that the Document Generator can replace in a document template using JSON data.
Data
The JSON data can be stored in Object Storage or specified inline in a Request.
Example - Stored in Object Storage
"data": {
"source": "OBJECT_STORAGE",
"namespace": "my_namespace",
"bucketName": "my_bucket",
"objectName": "my_folder/names.json"
}
Example - Specified inline in data.content
"data": {
"source": "INLINE",
"content": [{"name":"John"},{"name":"Monica"}]
}
Tags
Tags contain paths to some value in JSON data. For example, given this data:
{
"customer": {
"first_name": "Jack",
"last_name": "Smith"
}
}
You could use this template:
Hello {customer.first_name} {customer.last_name}!
To generate this text:
Hello Jack Smith!
{basic} Tag
Used to inject text or numbers.
Examples
Tag | Data | Result |
---|---|---|
{basic} | {"basic":"Hello!"} | Hello! |
{number} | {"number": 7} | 7 |
Formatting
{basic}
tags can be formatted. See Number Formatting for more details.
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-Basic.docx | Word-Basic.json | Word-Basic-output.docx |
{#loop}...{/loop} Tag
A {#loop}
tag is used to produce copies of a section of text vertically.
List Example
Data
{
"class_list": [
{
"name": "Sue",
"student_id": 1
},
{
"name": "Bob",
"student_id": 2
},
{
"name": "Jean",
"student_id": 3
}
]
}
Template
Class List
{#class_list}* {student_id}: {name}
{/class_list}
Result
Class List
* 1: Sue
* 2: Bob
* 3: Jean
Table Example
Data
{
"class_list": [
{
"name": "Sue",
"student_id": 1
},
{
"name": "Bob",
"student_id": 2
},
{
"name": "Jean",
"student_id": 3
}
]
}
Template
Id | Name |
---|---|
{#class_list}{student_id} | {name}{/class_list} |
Result
Id | Name |
---|---|
1 | Sue |
2 | Bob |
3 | Jean |
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-VLoop.docx | Word-VLoop.json | Word-VLoop-output.docx |
Array Operations
Filter
The filter
operation takes three arguments:
- The property to filter on
- The comparison to perform
- The value to compare against
Currently, filtering is only supported on numeric types.
To use a filter, you must specify a new list, called {#filtered}...{/filtered}
inside of the parent array doing the filtering.
Example
Data
{
"products": [
{
"price": 1,
"name": "Belt",
"category": "Accessory"
},
{
"price": 3,
"name": "Shirt",
"category": "Clothing"
},
{
"price": 2,
"name": "Hat",
"category": "Accessory"
}
]
}
Template
{#products|filter:price:>=:2}
Category | Name | Price |
---|---|---|
{#filtered}{category} | {name} | {price}{/filtered} |
{/products|filter:price:>=:2}
Result
Category | Name | Price |
---|---|---|
Clothing | Shirt | 3 |
Accessory | Hat | 2 |
Sort
The sort
operation takes two arguments:
- The name of the property to use for sorting
asc
ordesc
to sort the list inascending
ordescending
order.
To use a sort, you must specify a new list, called {#sorted}...{/sorted}
inside of the parent array being sorted.
Example
Data
{
"products": [
{
"price": 1,
"name": "Belt",
"category": "Accessory"
},
{
"price": 3,
"name": "Shirt",
"category": "Clothing"
},
{
"price": 2,
"name": "Hat",
"category": "Accessory"
}
]
}
Template
{#products|sort:price:desc}
Category | Name | Price |
---|---|---|
{#sorted}{category} | {name} | {price}{/sorted} |
{/products|sort:price:desc}
Result
Category | Name | Price |
---|---|---|
Clothing | Shirt | 3 |
Accessory | Hat | 2 |
Accessory | Belt | 1 |
Distinct
The distinct
operation takes a single argument:
- The name of the property to select distinct values of.
Example
Data
{
"products": [
{
"price": 1,
"name": "Belt",
"category": "Accessory"
},
{
"price": 3,
"name": "Shirt",
"category": "Clothing"
},
{
"price": 2,
"name": "Hat",
"category": "Accessory"
}
]
}
Template
Categories:
{#products|distinct:category}
* {category}
{/products|distinct:category}
Result
Categories:
* Accessory
* Clothing
Break
The break
operator groups items together based on a shared property. It takes one argument:
- The name of the property to group items on.
To use a break, you must specify a new list, called {#break}...{/break}
inside of the parent array being broken into groups.
Example
In this example note that the "Belt" and "Hat" items shared a category ("Accessory"), but they are not grouped in the array ("Belt" is the first item in the array and "Hat" is the last item).
Once the break
operator has been applied, the items are grouped together in the output.
Data
{
"products": [
{
"price": 1,
"name": "Belt",
"category": "Accessory"
},
{
"price": 3,
"name": "Shirt",
"category": "Clothing"
},
{
"price": 2,
"name": "Hat",
"category": "Accessory"
}
]
}
Template
{#products|break:category}
{#break}* Category:{category}, Product:{name}
{/break}
{/products|break:category}
Result
* Category:Clothing, Product:Shirt
* Category:Accessory, Product:Belt
* Category:Accessory, Product:Hat
Group
Splits an array into sub arrays of the given size. A single argument is required:
- An integer specifying the group size.
To use a group, you must specify a new list, called {#group}...{/group}
inside of the parent array being broken into groups.
If the array does not split perfectly into groups, the last group will be of smaller size. For example, if you have an array of 5 items, and you use group:2
on it, then two groups of two items and one
group of one item will be created.
Example
Data
{
"products": [
{
"price": 1,
"name": "Belt",
"category": "Accessory"
},
{
"price": 3,
"name": "Shirt",
"category": "Clothing"
},
{
"price": 2,
"name": "Hat",
"category": "Accessory"
}
]
}
Template
{#products|group:2}Items
{#group}* {name}
{/group}
{/products|group:2}
Result
Items
* Belt
* Shirt
Items
* Hat
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-VLoop-Arrays.docx | Word-VLoop-Arrays.json | Word-VLoop-Arrays-output.docx |
{:loop}...{/loop} Tag
A {:loop}
tag is used to produce copies of a section of text horizontally.
Table Example
Data
{
"weekdays": [
{
"name": "Monday"
},
{
"name": "Tuesday"
},
{
"name": "Wednesday"
},
{
"name": "Thursday"
},
{
"name": "Friday"
}
]
}
Template
Days | {:weekdays}{name}{/weekdays} |
---|---|
Result
Days | Monday | Tuesday | Wednesday | Thursday | Friday |
---|---|---|---|---|---|
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-HLoop.docx | Word-HLoop.json | Word-HLoop-output.docx |
Aggregators
Sum
The Sum Aggregator is used to calculate the sum of an array.
Example
Data
{
"items": [
{
"name": "ball",
"price": 1
},
{
"name": "stick",
"price": 2
},
{
"name": "cup",
"price": 3
}
]
}
Template
Total Price: {items|sum:price}
Result
Total Price: 6
Average
The Average Aggregator is used to calculate the average of an array.
Example
Data
{
"items": [
{
"name": "ball",
"price": 1
},
{
"name": "stick",
"price": 2
},
{
"name": "cup",
"price": 3
}
]
}
Template
Average Price: {items|avg:price}
Result
Average Price: 2
Max
The Max Aggregator is used to find the maximum value of an array.
Example
Data
{
"items": [
{
"name": "ball",
"price": 1
},
{
"name": "stick",
"price": 2
},
{
"name": "cup",
"price": 3
}
]
}
Template
Max Price: {items|max:price}
Result
Average Price: 3
Min
The Min Aggregator is used to find the minimum value of an array.
Example
Data
{
"items": [
{
"name": "ball",
"price": 1
},
{
"name": "stick",
"price": 2
},
{
"name": "cup",
"price": 3
}
]
}
Template
Minimum Price: {items|min:price}
Result
Minimum Price: 1
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-Aggregators.docx | Word-Aggregators.json | Word-Aggregators-output.docx |
{%image} Tag
An {%image}
tag is used to inject an image into a document. Images can be provided from an OCI Object Storage Bucket, or from a URL. Images must be provided as an object, for example:
{
"my_image": {
"source": "OBJECT_STORAGE",
"objectName": "image.png",
"namespace": "object_storage_namespace",
"bucketName": "my_bucket_name",
"mediaType": "image/png"
}
}
The specific schema for each source type is described below. Additionally, the following properties may be (optionally) included in the image object to control formatting of the image:
- width: A
string
of digits, followed bypx
. E.G.200px
. Sets the width of the image. - height: A
string
of digits, followed bypx
. E.G.200px
. Sets the height of the image. - alt_text: A
string
. This will be set as the alternative text of the image.
Default size of inserted images:
- Smaller images: If an image is smaller than the page size, the original size is preserved.
- Larger images: If an image is larger than the page size, it is resized to fit within the page width and height. The aspect ratio of the image is maintained.
Supported Formats
Document Generator supports the following image formats:
- PNG
- JPG
- GIF
- BMP
Schemas
OCI Object Storage
source
: must be set toOBJECT_STORAGE
objectName
: The path and name of the filenamespace
: The namespace of your object storage bucketbucketName
: The name of the bucket that contains the filemediaType
: The Media Type (MIME) of the image
Example
Data
{
"my_image": {
"source": "OBJECT_STORAGE",
"objectName": "image.png",
"namespace": "object_storage_namespace",
"bucketName": "my_bucket_name",
"mediaType": "image/png",
"width": "400px",
"height": "200px"
}
}
Template
{%my_image}
Result
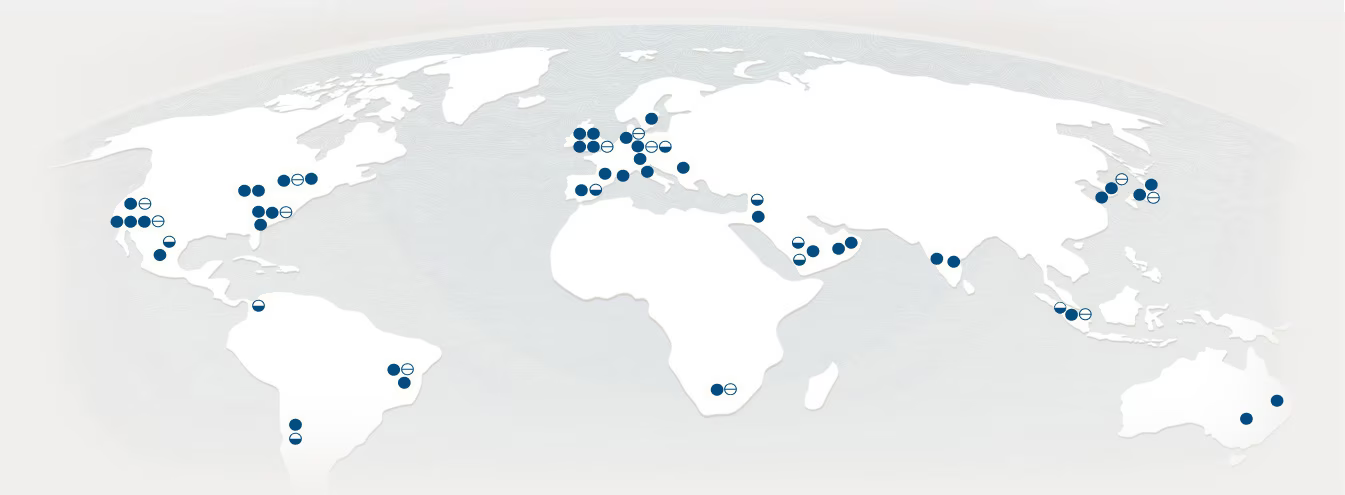
URL
source
: must be set toURL
url
: the image URL instring
format
Note: to use images from the Internet, Document Generator needs outbound access to the Internet. For example, if Document Generator is running in a private subnet in OCI, you could set up a NAT Gateway to allow Document Generator to connect to the Internet.
Example
Data
{
"my_image": {
"source": "URL",
"url": "https://www.oracle.com/.../.jpg"
}
}
Template
{%my_image}
Result
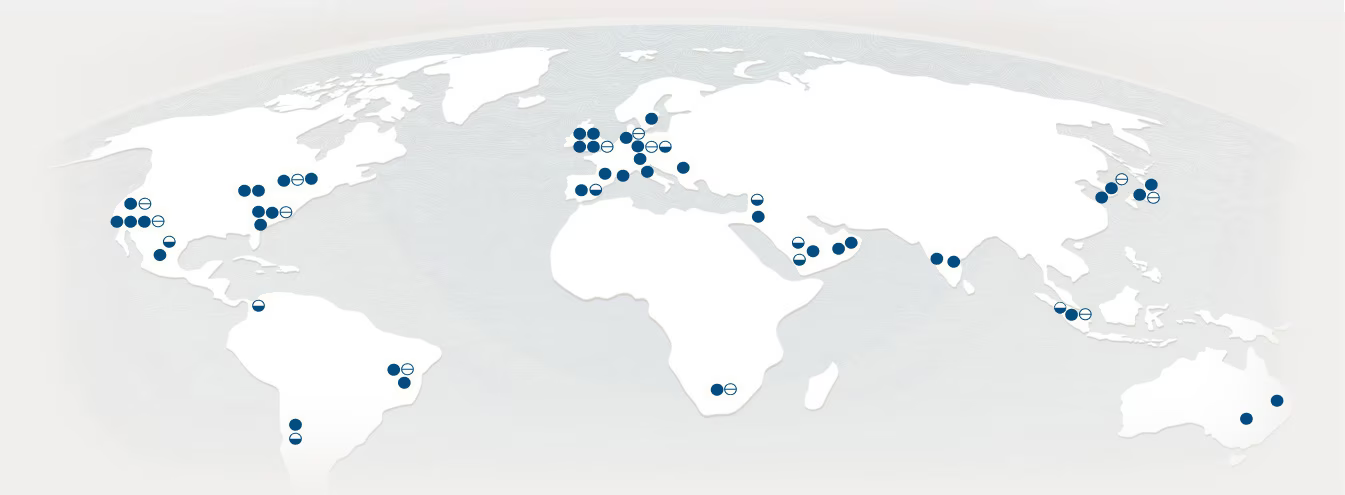
Data URL
Document Generator also supports the ability to pass images inside of Data URLs. The image must be Base64 encoded.
source
: must be set toURL
url
: the image URL instring
format
Example
Data
{
"my_image": {
"source": "URL",
"url": "data:image/png;base64,iVBORw0KG...go"
}
}
Template
{%my_image}
Result
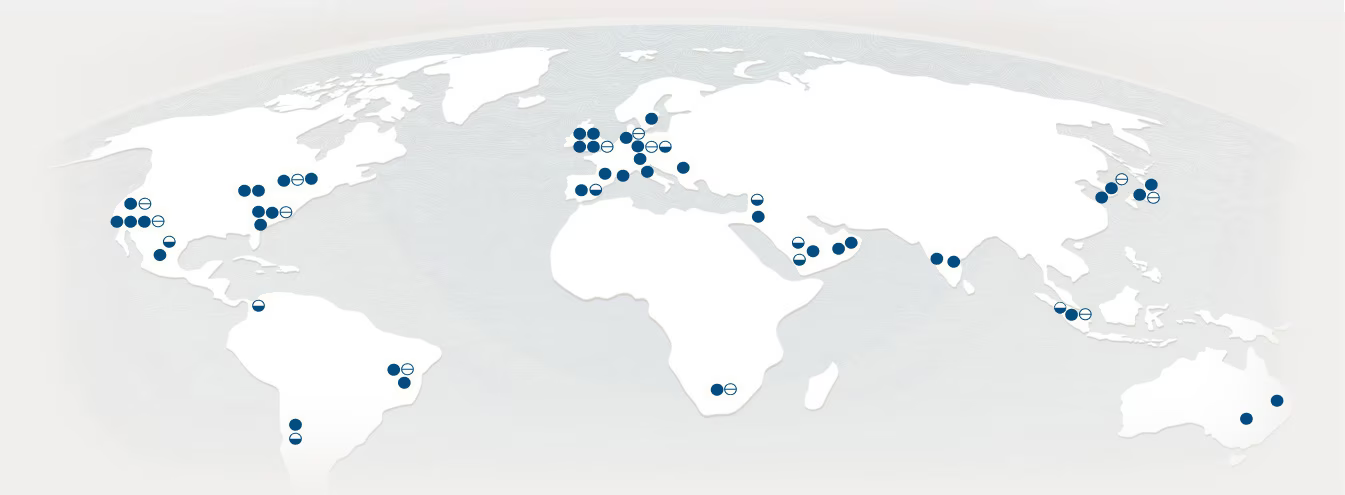
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-Images.docx | Word-Images.json | Word-Images-output.docx |
{?pagebreak} and {?columnbreak} Tags
A pagebreak tag is used to introduce a page break. There are four variations of these tags:
{?pagebreak}
and{?page}
both create page breaks.{?columnbreak}
and{?column}
both create column breaks.
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-Breaks.docx | Word-Breaks.json | Word-Breaks-output.docx |
{*hyperlink} Tag
A {*hyperlink}
tag is used to insert a clickable hyperlink (including email addresses) into a document.
A hyperlink must be provided as an object with the following properties:
url
: a URL instring
formaturl_text
(optional): astring
to be displayed instead of the URL
Example
Data
{
"link": {
"url": "https://www.oracle.com/",
"url_text": "Click me!"
}
}
Template
Follow the link: {*link}
Result
Follow the link: Click me!
Sample documents
MS Word Template | JSON Data | Output |
---|---|---|
Word-Hyperlinks.docx | Word-Hyperlinks.json | Word-Hyperlinks-output.docx |
Number Formatting
If a value being supplied to a {basic}
tag is a number, Document Generator supports the ability to format it.
A formatter is specified as follows: {input_number|format:[format]:[separators]:[currency_symbol]}
where:
[format]
is a supported number format[separators]
(optional) is a 2 character string. The first character indicates the decimal separator, the second character indicates the comma separator[currency_symbol]
(optional) the currency symbol to apply ifL
is present in[format]
Number formats must be specified in one of the following Oracle Number Formats:
FML999G999G999G999G990D00
FML999G999G999G999G990
999G999G999G999G990D00
999G999G999G999G990D0000
999G999G999G999G999G999G990
999G999G999G999G990D00MI
999G999G999G999G990D00MI
S999G999G999G999G990D00
999G999G999G999G990D00S
S999G999G999G999G990D00
999G999G999G999G990D00PR
FML999G999G999G999G990PR
999G999G999G999G990D00PR
Examples
The following table is given to share examples of these formats, given a number:
Template | unit_price: 1234.5 | unit_price: -1234.5 |
---|---|---|
{unit_price|format:"FML999G999G999G999G990D00":"<>":"$"} | $1>234<50 | -$1>234<50 |
{unit_price|format:"999G999G999G999G999G999G990"} | 1,234 | -1,234 |
{unit_price|format:"999G999G999G999G990D00MI"} | 1,234.50 | 1,234.50- |
{unit_price|format:"S999G999G999G999G990D00"} | +1,234.50 | -1,234.50 |
{unit_price|format:"999G999G999G999G990D00PR"} | 1,234.50 | <1,234.50> |
{unit_price|format:"FML999G999G999G999G990PR"} | $1,234 | <$1,234> |
Tag - Advanced Examples
Loop in Loop
In this example, we have 2 levels of loops. One for the movies and another for the actors.
MS Word Template | JSON Data | Output |
---|---|---|
Word-LoopInLoop.docx | Word-LoopInLoop.json | Word-LoopInLoop-output.docx |
Aggregation and Formatting combination
In this example, the Total of the Invoice uses both Aggregation (sum) and Number Formatting.
MS Word Template | JSON Data | Output |
---|---|---|
Word-AggregationAndFormat.docx | Word-AggregationAndFormat.json | Word-AggregationAndFormat-output.docx |