Embedding HTML in Suitelets
The following examples illustrate how to embed HTML within Suitelet code to add custom elements to a form. Embedding HTML in Suitelets is useful for adding components that are not available through the SuiteScript N/ui/serverWidget module.
Embedding Inline HTML in a Field
In a Suitelet, you can use Form.addField(options) to add inline HTML as a field on a form. Specify the type as INLINEHTML
and use the Field.defaultValue property to set the HTML value. For more information, see N/ui/serverWidget Module.
var htmlImage = form.addField({
id: 'custpage_htmlfield',
type: serverWidget.FieldType.INLINEHTML,
label: 'HTML Image'
});
htmlImage.defaultValue = "<img src='https://<accountID>.app.netsuite.com/images/logos/netsuite-oracle.svg' alt='Oracle NetSuite logo'>";
The following screenshot shows a form with an INLINEHTML
field containing an image field.
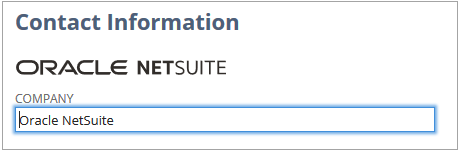
Embedding HTML from a Linked HTML Page in a Suitelet
In a Suitelet, you can use https.get(options) from the N/https module to embed HTML from a linked HTML page in the form created by the Suitelet. The Suitelet manages the data submitted by users to the HTML page. When you use a linked HTML page, you manage HTML code through strings, so it can be harder to manipulate data than it is with components created with N/serverWidget module methods. To pass data through the string containing the HTML code, your Suitelet code must change values within the string. This string becomes increasing complex as the number of values increases. For more information, see N/https Module.
The following example creates a simple volunteer sign-up sheet. It consists of two parts: the Suitelet code and the HTML. This Suitelet code uses the https.get(options) method from the N/https module to access the content from the HTML page. It uses methods from the N/record module, N/email module, and N/search module to collect, process, and respond to user-submitted data.
/**
* @NApiVersion 2.x
* @NScriptType Suitelet
*/
define(['N/https', 'N/record', 'N/email', 'N/search'],
function callbackFunction(https, record, email, search) {
function getFunction(context) {
var contentRequest = https.get({
url: "https://LinkToFormPage.html" // see the next snippet
});
var contentDocument = contentRequest.body;
var sponsorid = context.request.parameters.sponsorid;
if (sponsorid && sponsorid != "" && sponsorid != null) {
contentDocument = contentDocument.replace("{{sponsorid}}", sponsorid);
log.debug("Setting Sponsor", sponsorid)
}
var projectid = context.request.parameters.projectid;
if (projectid && projectid != "" && projectid != null) {
contentDocument = contentDocument.replace("{{projectid}}", projectid);
log.debug("Setting Project", projectid);
}
context.response.write(contentDocument);
}
function postFunction(context) {
var params = context.request.parameters;
var emailString = "First Name: {{firstname}}\nLast Name: {{lastname}}\nEmail: {{email}}\nFacebook URL: {{custentity_fb_url}}"
var contactRecord = record.create({
type: "contact",
isDynamic: true
});
for (param in params) {
if (param === "company") {
if (params[param] !== "{{sponsorid}}") {
contactRecord.setValue({
fieldId: param,
value: params[param]
});
var lkpfld = search.lookupFields({
type: "vendor",
id: params["company"],
columns: ["entityid"]
});
emailString += "\nSponsor: " + lkpfld.entityid;
}
else {
contactRecord.setValue({
fieldId: "custentity_sv_shn_isindi",
value: true
})
}
}
else {
if (param !== "project") {
contactRecord.setValue({
fieldId: param,
value: params[param]
});
var replacer = "{{" + param + "}}";
emailString = emailString.replace(replacer, params[param]);
}
}
}
var contactId = contactRecord.save({
ignoreMandatoryFields: true,
enableSourcing: true
});
log.debug("Record ID", contactId);
if (params["project"] && params["project"] !== "" && params["project"] != null && params
["project"] !== "{{projectid}}") {
var lkpfld = search.lookupFields({
type: "job",
id: params["project"],
columns: ["companyname"]
});
emailString += "\nProject Name: " + lkpfld.companyname;
var participationRec = record.create({
type: "customrecord_project_participants",
isDynamic: true
});
participationRec.setValue({
fieldId: "custrecord_participants_volunteer",
value: contactId
})
participationRec.setValue({
fieldId: "custrecord_participants_project",
value: params["project"]
})
var participationId = participationRec.save({
enableSourcing: true,
ignoreMandatoryFields: true
})
}
log.debug("Email String", emailString);
email.send({
author: -5,
recipients: 256,
subject: "New Volunteer Signed Up",
body: "A new volunteer has joined:\n\n" + emailString
});
email.send({
author: -5,
recipients: params["email"],
subject: "Thank you!",
body: "Thank you for volunteering:\n\n" + emailString
});
var contentRequest = https.get({
url: "https://LinkToFormCompletePage.html"
});
var contentDocument = contentRequest.body;
context.response.write(contentDocument);
}
function onRequestFxn(context) {
if (context.request.method === "GET") {
getFunction(context)
}
else {
postFunction(context)
}
}
return {
onRequest: onRequestFxn
};
});
The following HTML, contained in the LinkToFormPage.html file (see above snippet), creates the form that the Suitelet links to.
<form method="post" class="form-horizontal" action="https://LinkToSuitelet.js">
<table>
<tbody><tr>
<td>First Name</td>
<td class="col-md-8"><input class="form-control" id="firstname" placeholder="First Name" name="firstname" required="" type="text"></td>
</tr>
<tr>
<td>Last Name</td>
<td class="col-md-8"><input class="form-control" id="lastname" placeholder="Last Name" name="lastname" required="" type="text"></td>
</tr>
<tr>
<td>Email</td>
<td class="col-md-8"><input class="form-control" id="email" placeholder="email" name="email" required="" type="email"></td>
</tr>
<tr>
<td>Facebook URL</td>
<td class="col-md-8"><input class="form-control" id="custentity_fb_url" placeholder="Facebook" name="custentity_fb_url" required="" type="text"></td>
</tr>
<input name="company" value="{{sponsorid}}" type="hidden">
<input name="project" value="{{projectid}}" type="hidden">
</tbody></table>
<br>
<button type="submit" class="btn btn-inverse">Sign Up as a Volunteer</button>
</form>
The following screenshot illustrates the form that is created by the linked HTML page.
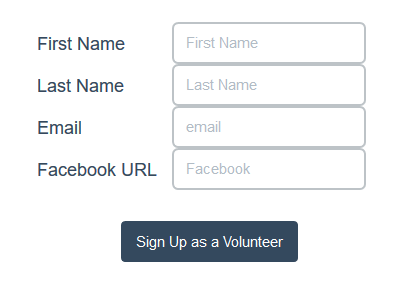