Adding a Record With Other Deposit, Cash Back Lines
In some cases, you might want to create a deposit record using the Other Deposits and Cash Back sublists. The Other Deposits sublist is used to debit one or more accounts named in the sublist lines. By contrast, the Cash Back list is generally used to credit accounts.
Each sublist has an amount field, but these fields are different. That is, in the Other Deposits sublist, a positive amount field debits the account identified by the line. In the Cash Back sublist, the amount field credits the account identified by the line.
For example, in the illustration below, the Undeposited Funds account is debited $5,000 and the Advances Paid account is debited $8,000.
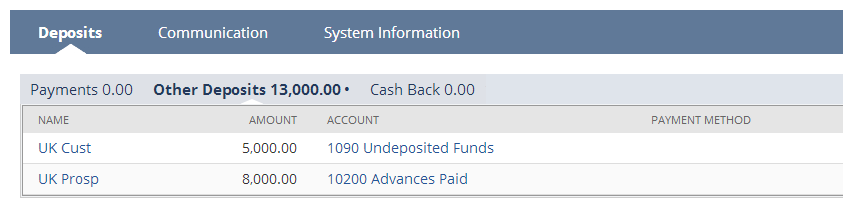
In the illustration below, the Other Receivables account is credited $150.
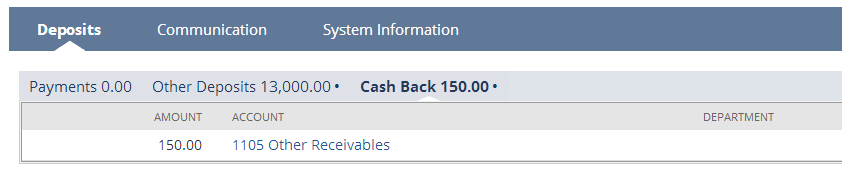
In this example, the net sum of the lines is a positive amount ($12,850), which means the record can be added without error. (When the net value of all sublist lines is negative, the record cannot be added.)
The recipient of the funds is identified by the account body field. For example, in the screenshot below, the recipient is the UK Bank GBP account.
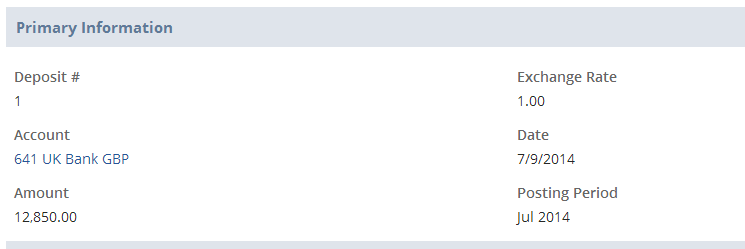
The following code sample shows how you would create this record.
C#
private void addSecondDeposit()
{
// Create object.
Deposit myDeposit = new Deposit();
// Set an external Id.
myDeposit.externalId = "805A";
// Identify the account that will receive the deposit.
RecordRef myAccount = new RecordRef();
myAccount.internalId = "6";
myDeposit.account = myAccount;
// Create an instance of the Other Deposits sublist that can take up to two lines.
myDeposit.otherList = new DepositOtherList();
myDeposit.otherList.depositOther = new DepositOther[2];
// Populate the lines.
RecordRef myEntity = new RecordRef();
myEntity.type = RecordType.customer;
myEntity.typeSpecified = true;
myEntity.internalId = "1766";
RecordRef myOtherDepositAccount = new RecordRef();
myOtherDepositAccount.internalId = "12";
myDeposit.otherList.depositOther[0] = new DepositOther();
myDeposit.otherList.depositOther[0].entity = myEntity;
myDeposit.otherList.depositOther[0].amount = 5000;
myDeposit.otherList.depositOther[0].amountSpecified = true;
myDeposit.otherList.depositOther[0].account = myOtherDepositAccount;
RecordRef myEntity2 = new RecordRef();
myEntity2.type = RecordType.customer;
myEntity2.typeSpecified = true;
myEntity2.internalId = "1974";
RecordRef myOtherDepositAccount2 = new RecordRef();
myOtherDepositAccount2.internalId = "244";
myDeposit.otherList.depositOther[1] = new DepositOther();
myDeposit.otherList.depositOther[1].entity = myEntity2;
myDeposit.otherList.depositOther[1].amount = 8000;
myDeposit.otherList.depositOther[1].amountSpecified = true;
myDeposit.otherList.depositOther[1].account = myOtherDepositAccount2;
// Create an instance of the Cash Back sublist.
myDeposit.cashBackList = new DepositCashBackList();
myDeposit.cashBackList.depositCashBack = new DepositCashBack[1];
// Populate the sublist.
RecordRef myCashBackAccount = new RecordRef();
myCashBackAccount.internalId = "9";
myDeposit.cashBackList.depositCashBack[0] = new DepositCashBack();
myDeposit.cashBackList.depositCashBack[0].amount = 150;
myDeposit.cashBackList.depositCashBack[0].amountSpecified = true;
myDeposit.cashBackList.depositCashBack[0].account = myCashBackAccount;
// Execute the operation.
_service.add(myDeposit);
}
SOAP Request
<soap:Body>
<add xmlns="urn:messages_2017_1.platform.webservices.netsuite.com">
<record xmlns:q1="urn:bank_2017_1.transactions.webservices.netsuite.com" xsi:type="q1:Deposit" externalId="805A">
<q1:account internalId="6" />
<q1:otherList>
<q1:depositOther>
<q1:entity internalId="1766" type="customer" />
<q1:amount>5000</q1:amount>
<q1:account internalId="12" />
</q1:depositOther>
<q1:depositOther>
<q1:entity internalId="1974" type="customer" />
<q1:amount>8000</q1:amount>
<q1:account internalId="244" />
</q1:depositOther>
</q1:otherList>
<q1:cashBackList>
<q1:depositCashBack>
<q1:amount>150</q1:amount>
<q1:account internalId="9" />
</q1:depositCashBack>
</q1:cashBackList>
</record>
</add>
</soap:Body>
SOAP Response
<soapenv:Body>
<addResponse xmlns="urn:messages_2017_1.platform.webservices.netsuite.com">
<writeResponse>
<platformCore:status isSuccess="true" xmlns:platformCore="urn:core_2017_1.platform.webservices.netsuite.com"/>
<baseRef internalId="7952" externalId="805A" type="deposit" xsi:type="platformCore:RecordRef" xmlns:platformCore="urn:core_2017_1.platform.webservices.netsuite.com"/>
</writeResponse>
</addResponse>
</soapenv:Body>
Related Topics
- Deposit
- Deposit Supported Operations
- Deposit Code Samples
- Adding a Record With Payment Lines
- Updating the Deposit Record
- Common Errors With Deposit Records
- SOAP Web Services Standard Operations
- add
- SuiteTalk SOAP Web Services Platform Overview
- Deposits
- Making Deposits
- Viewing the Deposits List
- General Ledger Impact of Transactions
- Bank Transaction GL Impact