Extend Attachments
Use an order management extension to create, read, update, or delete an attachment.
Overview
Use a text or XML attachment file. Don't use a binary file.
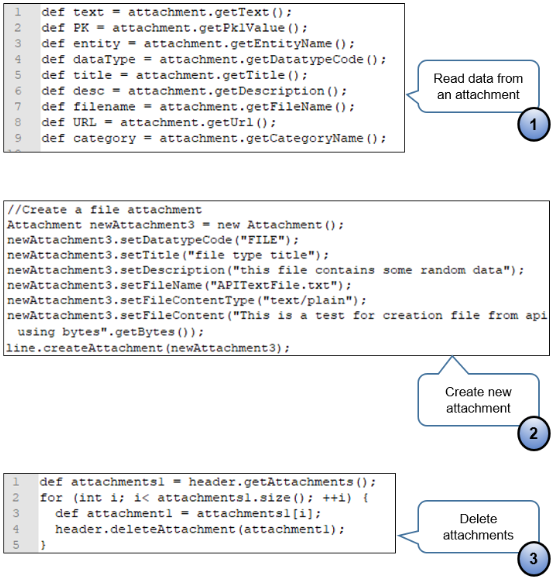
Note
-
Use get methods to get attachment details from an attachment that already exists, such as getText to get all the text that the attachment contains, use getPklValue to get the sales order or order line that references the attachment, getFileName to get the name of the attachment file name, and so on. Here's some of the methods you can use.
-
getText
-
getPklValue
-
getEntityName
-
getDataTypeCode
-
getTitle
-
getDescription
-
getFileName
-
getUrl
-
getCategoryName
-
-
Use the createAttachment method to create an attachment, and use
set
methods to specify how to create it. You can specify a new attachment file of type File, Text, or URL. For example, use setFileName to specify the name of the attachment file. Here are some of the methods you can use.-
setDataTypeCode
-
setTitle
-
setDescription
-
setFileName
-
setFileContentType
-
setFileContent
-
-
Use the deleteAttachment method to delete an attachment. In this example, the code uses a
for
statement to iterate through all the attachments that a sales order contains and deletes all of them.
Get Attachments from Order Headers
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import oracle.apps.scm.doo.common.extensions.Attachment;
import oracle.jbo.domain.BlobDomain;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("PMC TEST")) return;
List < Message > messages = new ArrayList < Message > ();
//messages.add(new Message( Message.MessageType.ERROR, "HeaderId: " + header.getAttribute("HeaderId")));
/////////////////Read header attachments
def attachments = header.getAttachments();
for (int i = 0; i < attachments.size(); ++i) {
def attachment = attachments[i];
messages.add(new Message(Message.MessageType.ERROR, "Pk1value:" + attachment.getPk1Value()));
messages.add(new Message(Message.MessageType.ERROR, "Entityname:" + attachment.getEntityName()));
messages.add(new Message(Message.MessageType.ERROR, "DatatypeCode:" + attachment.getDatatypeCode()));
messages.add(new Message(Message.MessageType.ERROR, "Title:" + attachment.getTitle()));
messages.add(new Message(Message.MessageType.ERROR, "Description:" + attachment.getDescription()));
messages.add(new Message(Message.MessageType.ERROR, "FileName:" + attachment.getFileName()));
messages.add(new Message(Message.MessageType.ERROR, "File Content Type:" + attachment.getFileContentType()));
def blobDomainData = attachment.getFileContent();
messages.add(new Message(Message.MessageType.ERROR, "File Content:" + blobDomainData.toString()));
messages.add(new Message(Message.MessageType.ERROR, "Url:" + attachment.getUrl()));
messages.add(new Message(Message.MessageType.ERROR, "Text:" + attachment.getText()));
}
ValidationException ex = new ValidationException(messages);
throw ex;
Get Attachments from Order Lines
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("PMC TEST")) return;
List < Message > messages = new ArrayList < Message > ();
//messages.add(new Message( Message.MessageType.ERROR, "Enter Attachment On Save"));
//messages.add(new Message( Message.MessageType.ERROR, "CustomerPONumber: " + poNumber));
Long headerId = header.getAttribute("HeaderId");
messages.add(new Message(Message.MessageType.ERROR, "HeaderId: " + headerId));
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
def attachments = line.getAttachments();
for (int i = 0; i < attachments.size(); ++i) {
def attachment = attachments[i];
messages.add(new Message(Message.MessageType.ERROR, "Pk1value:" + attachment.getPk1Value()));
messages.add(new Message(Message.MessageType.ERROR, "Entityname:" + attachment.getEntityName()));
messages.add(new Message(Message.MessageType.ERROR, "DatatypeCode:" + attachment.getDatatypeCode()));
messages.add(new Message(Message.MessageType.ERROR, "Text:" + attachment.getText()));
messages.add(new Message(Message.MessageType.ERROR, "Title:" + attachment.getTitle()));
messages.add(new Message(Message.MessageType.ERROR, "Description:" + attachment.getDescription()));
messages.add(new Message(Message.MessageType.ERROR, "FileName:" + attachment.getFileName()));
messages.add(new Message(Message.MessageType.ERROR, "Url:" + attachment.getUrl()));
messages.add(new Message(Message.MessageType.ERROR, "Url:" + attachment.getCategoryName()));
}
}
ValidationException ex = new ValidationException(messages);
throw ex;
Add Attachment to Order Header
The example adds an attachment to an order header.
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import oracle.apps.scm.doo.common.extensions.Attachment;
import oracle.jbo.domain.BlobDomain;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("WriteAttachmentsHeaderOnEndSubmit_run_extension")) return;
List < Message > messages = new ArrayList < Message > ();
//messages.add(new Message( Message.MessageType.ERROR, "HeaderId: " + header.getAttribute("HeaderId")));
////create header attachments
//Create TEXT attachment
Attachment newAttachment1 = new Attachment();
newAttachment1.setDatatypeCode("TEXT");
newAttachment1.setTitle("This is a text type title on submit");
newAttachment1.setText("this is some text");
newAttachment1.setDescription("this is some description");
header.createAttachment(newAttachment1);
//Create URL attachment
Attachment newAttachment2 = new Attachment();
newAttachment2.setDatatypeCode("WEB_PAGE");
newAttachment2.setTitle("This URL points to google server on submit");
newAttachment2.setUrl("http://www.google.com/");
newAttachment2.setDescription("Used for searching stuffs");
header.createAttachment(newAttachment2);
//Create a file attachment
Attachment newAttachment3 = new Attachment();
newAttachment3.setDatatypeCode("FILE");
newAttachment3.setTitle("file type title");
newAttachment3.setDescription("this file contains some random data on submit");
newAttachment3.setFileName("APITextFile.txt");
//newAttachment3.setFileContentType("application/text");
newAttachment3.setFileContentType("text/plain");
//newAttachment3.setFileContent(new BlobDomain("This is a test creation using Bytes".getBytes()));
newAttachment3.setFileContent("This is a test for creation file from api using bytes".getBytes());
header.createAttachment(newAttachment3);
/*delete all header attachments
//messages.add(new Message( Message.MessageType.ERROR, "Delete all header attachments"));
def attachments1 = header.getAttachments();
for (int i; i< attachments1.size(); ++i) {
def attachment1 = attachments1[i];
//header.deleteAttachment(attachment1);
}*/
ValidationException ex = new ValidationException(messages);
throw ex;
Delete Attachment from Order Header
The example deletes an attachment from the order header.
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import oracle.apps.scm.doo.common.extensions.Attachment;
import oracle.jbo.domain.BlobDomain;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("DeleteAttachmentsHeaderOnSubmit_run_extension")) return;
//delete all header attachments
//messages.add(new Message( Message.MessageType.ERROR, "Delete all header attachments"));
def attachments1 = header.getAttachments();
for (int i; i < attachments1.size(); ++i) {
//throw new ValidationException("An order with the Purchase Order ddddd Number " + attachments1 + " already exists.");
def attachment1 = attachments1[i];
header.deleteAttachment(attachment1);
}
Add Attachment to Order Line
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import oracle.apps.scm.doo.common.extensions.Attachment;
import oracle.jbo.domain.BlobDomain;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("WriteAttachmentsLineOnEndSubmitOnly_run_extension")) return;
List < Message > messages = new ArrayList < Message > ();
//messages.add(new Message( Message.MessageType.ERROR, "HeaderId: " + header.getAttribute("HeaderId")));
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
/////////////////create header attachments
//Create TEXT attachment
Attachment newAttachment1 = new Attachment();
newAttachment1.setDatatypeCode("TEXT");
newAttachment1.setTitle("This is a text type title");
newAttachment1.setText("this is some text");
newAttachment1.setDescription("this is some description");
line.createAttachment(newAttachment1);
//Create URL attachment
Attachment newAttachment2 = new Attachment();
newAttachment2.setDatatypeCode("WEB_PAGE");
newAttachment2.setTitle("This URL points to google server");
newAttachment2.setUrl("http://www.google.com/");
newAttachment2.setDescription("Used for searching stuffs");
line.createAttachment(newAttachment2);
//Create a file attachment
Attachment newAttachment3 = new Attachment();
newAttachment3.setDatatypeCode("FILE");
newAttachment3.setTitle("file type title");
newAttachment3.setDescription("this file contains some random data");
newAttachment3.setFileName("APITextFile.txt");
//newAttachment3.setFileContentType("application/text");
newAttachment3.setFileContentType("text/plain");
//newAttachment3.setFileContent(new BlobDomain("This is a test creation using Bytes".getBytes()));
newAttachment3.setFileContent("This is a test for creation file from api using bytes".getBytes());
line.createAttachment(newAttachment3);
}
/*delete all header attachments
//messages.add(new Message( Message.MessageType.ERROR, "Delete all header attachments"));
def attachments1 = header.getAttachments();
for (int i; i< attachments1.size(); ++i) {
def attachment1 = attachments1[i];
//header.deleteAttachment(attachment1);
}*/
ValidationException ex = new ValidationException(messages);
throw ex;
Delete Attachment from Order Line
import oracle.apps.scm.doo.common.extensions.ValidationException;
import oracle.apps.scm.doo.common.extensions.Message;
import oracle.apps.scm.doo.common.extensions.Attachment;
import oracle.jbo.domain.BlobDomain;
String poNumber = header.getAttribute("CustomerPONumber");
if (poNumber == null) return;
if (!poNumber.startsWith("DeleteAttachmentsLineOnSubmitOnly_run_extension")) return;
List < Message > messages = new ArrayList < Message > ();
//messages.add(new Message( Message.MessageType.ERROR, "HeaderId: " + header.getAttribute("HeaderId")));
def lines = header.getAttribute("Lines");
while (lines.hasNext()) {
def line = lines.next();
//delete all attachments from the order header
//messages.add(new Message( Message.MessageType.ERROR, "Delete all header attachments"));
def attachments1 = line.getAttachments();
for (int i; i < attachments1.size(); ++i) {
def attachment1 = attachments1[i];
line.deleteAttachment(attachment1);
}
}