Post Quantities Completed for Work Order Operations
You can use a REST API to post quantities completed for the last work order operation. You can scan the work order number and the last operation number, and then post the quantity for the last operation.
Let's discuss these scenarios:
-
Get dispatch state quantities for one work order operation.
-
Post quantities completed for one work order operation.
Get Dispatch State Quantities for One Work Order Operation
You create a solution that allows John Smith, a discrete manufacturer, to use a mobile client to connect to your partner application, and then use a REST API. An application on the client scans work order number M1-1208 and operation 20. The client then gets the quantity in different dispatch states for operation 20.
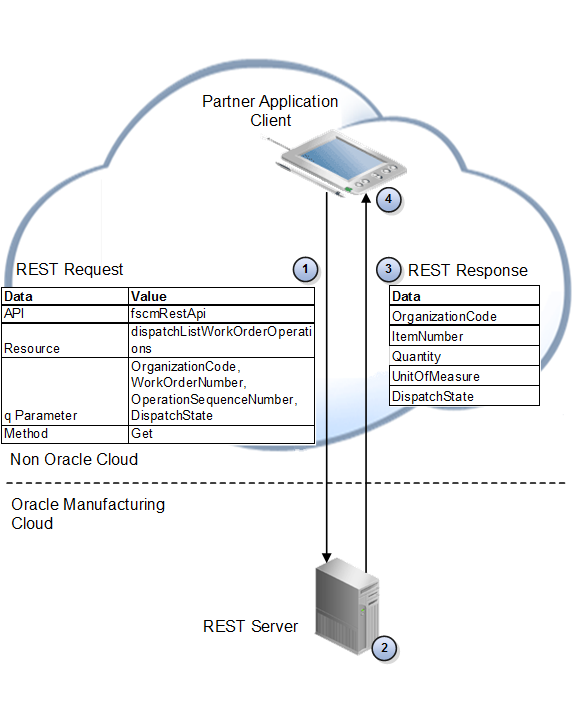
Here's a typical application processing flow for the scenarios:
-
The client captures the work order number and operation sequence number. You send a REST API request from the client to the server.
-
The response payload includes details that describe the results of the request.
-
Your partner application extracts the values for the following attributes from the response payload, and then displays them in the client.
Note:
Some resources contain only a few attributes. Others might contain several hundred attributes. This table includes only some of the attributes from the dispatchListWorkOrderOperations resource.
Attribute
Description
OrganizationCode
Value that uniquely identifies the material transaction number. For example, 22386581.
ItemNumber
A flag that indicates whether the transaction succeeded or failed.
Quantity
Quantity that is in the READY dispatch state. For example, 100.
UnitOfMeasure
Unit of Measure of the item. For example, Ea.
DispatchState
Current dispatch status of an operation, such as Ready, Completed, Scrapped, or Rejected.
Example URL
Use this resource URL format to get the quantity that is in dispatch state READY for organization code M1, work order number M1-1208, and work order operation sequence number 20.
GET
curl -u username:password "https://servername/fscmRestApi/resources/version/dispatchListWorkOrderOperations?q=OrganizationCode=M1;WorkOrderNumber=M1-1208;OperationSequenceNumber=20;DispatchState=READY"
Example Response
Here's an example of the response body in JSON format.
{ "items": [{ "OrganizationId": 207, "OrganizationCode": "M1", "OrganizationName": "Tall Manufacturing", "WorkAreaId": 300100073218239, "WorkAreaCode": "JISOP_WA2", "WorkAreaName": "JISOP_WA2", "WorkCenterId": 300100073218246, "WorkCenterCode": "JISOP_WC2M1_MAC", "WorkCenterName": "JISOP_WC2M1_MAC", "WorkCenterDescription": "Work Center for Machine Resources - SOP", "WorkOrderId": 300100084274447, "WorkOrderNumber": "M1-1208", "WorkOrderDescription": "Work Order for 5.5" mobile", "InventoryItemId": 100100039578388, "ItemNumber": "MI-1234", "ItemDescription": "Mobile 5.5" ", "OperationId": 300100084274449, "OperationSequenceNumber": 20, "OperationName": "JI3K_OP2", "OperationDescription": "Test", "DispatchState": "READY", "Quantity": 100, "UnitOfMeasure": "EA", "PlannedStartDate": "2016-10-10T10:08:00-07:00", "PlannedCompletionDate": "2016-10-10T10:08:00-07:00", "WorkOrderPriority": null, "PurchaseOrderId": null, "PurchaseOrder": null, "PurchaseOrderLineId": null, "PurchaseOrderLine": null, "CustomerId": null, "CustomerName": null, } ] }
Post Quantities Completed for One Work Order Operation
An operator on the shop floor records the completed quantity, rejected quantity, and scrap quantity in the client for one of operation 20, and then posts the operation transaction in Oracle Manufacturing Cloud. The client displays the Ready quantity. Assume that 96 Ea are good, 1 Ea must be scrapped, and 3 Ea are rejected and a decision is pending.
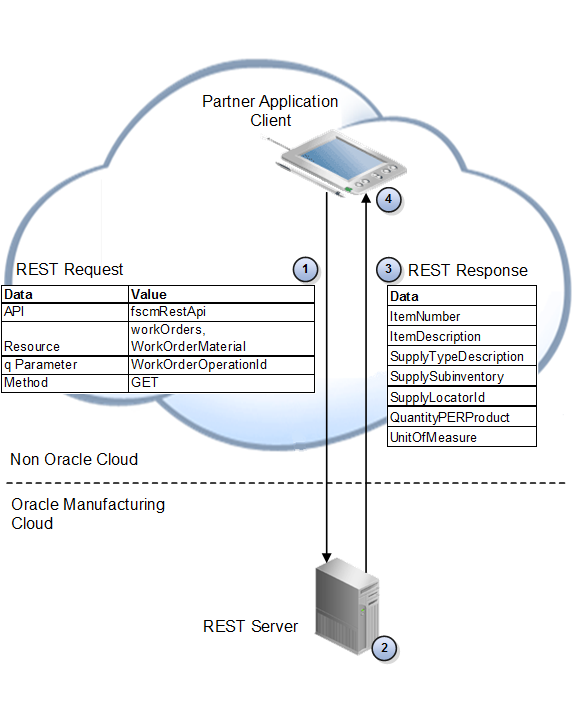
Here's a typical application processing flow for the scenarios:
-
The client captures the quantity to complete and to put away into inventory, the quantity to reject, and the quantity to scrap. You send a REST API request from the client to the server.
-
The response payload includes details that describe the results of the request.
-
Your partner application extracts the values for the following attributes from the response payload, and then displays them in the client.
Attribute
Description
WoOperationTransactionId
Value that uniquely identifies the material transaction number. For example, 22386581.
ErrorsExistFlag
A flag that indicates whether the transaction succeeded or failed.
Example URL
Use this resource URL format to post an operation transaction for assembly MI-1234, work order M1-1208, and operation sequence number 20.
POST
curl -u username:password -X POST -H "Content-Type:application/vnd.oracle.adf.resourceitem+json" -d 'request payload' "https://servername/fscmRestApi/resources/version/operationTransactions"
Example Request
Here's an example of the request body in JSON format.
{ "BatchCode" : MVU098, "SourceSystemCode" : "Client", "OperationTransactionDetail" : [ { "OperationTransactionsInput" : "HeaderNumber" : "HN_001", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "OperationSequence" : 20, "FromDispatchState" : "READY", "ToDispatchState" : "COMPLETE", "TransactionQuantity" : 96, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : null, "CompletionLocator" : null, "TransactionDate" : null, "ReasonCode" : null, "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null } { "OperationTransactionsInput" : "HeaderNumber" : "HN_002", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "OperationSequence" : 20, "FromDispatchState" : "READY", "ToDispatchState" : "REJECT", "TransactionQuantity" : 3, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : null, "CompletionLocator" : null, "TransactionDate" : null, "ReasonCode" : null, "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null } { "OperationTransactionsInput" : "HeaderNumber" : "HN_003", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "OperationSequence" : 20, "FromDispatchState" : "READY", "ToDispatchState" : "SCRAP", "TransactionQuantity" : 1, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : null, "CompletionLocator" : null, "TransactionDate" : null, "ReasonCode" : "Scrapped as part of Impact Testing", "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null }] }
Example Response
Here's an example of the response body in JSON format.
{ "SourceSystemType" : null, "SourceSystemCode" : "Client", "ErrorsExistFlag" : "false", "OperationTransactionDetail" : [ { "WoOperationTransactionId" : 29627, "SourceSystemCode" : "client", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "WoOperationSequenceNumber" : 20, "TransactionDate" : "2016-10-31T23:30:50-07:00", "FromDispatchState" : "READY", "ToDispatchState" : "COMPLETE", "TransactionQuantity" : 96, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : "ABCSUB01", "ReasonCode" : null, "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null, "CompletionLocator" : "ABC1.1.1", "ErrorMessages" : "", "ErrorMessageNames" : "", "InspectionEventId" : null } { "WoOperationTransactionId" : 29627, "SourceSystemCode" : "client", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "WoOperationSequenceNumber" : 20, "TransactionDate" : "2016-10-31T23:30:50-07:00", "FromDispatchState" : "READY", "ToDispatchState" : "REJECT", "TransactionQuantity" : 3, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : null, "ReasonCode" : null, "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null, "CompletionLocator" : null, "ErrorMessages" : "", "ErrorMessageNames" : "", "InspectionEventId" : null } { "WoOperationTransactionId" : 29627, "SourceSystemCode" : "client", "OrganizationCode" : "M1", "WorkOrderNumber" : "M1-1208", "WoOperationSequenceNumber" : 20, "TransactionDate" : "2016-10-31T23:30:50-07:00", "FromDispatchState" : "READY", "ToDispatchState" : "SCRAP", "TransactionQuantity" : 1, "TransactionUnitOfMeasure" : "Ea", "ComplSubinventoryCode" : null, "ReasonCode" : "Scrapped as part of Impact Testing", "TransactionNote" : null, "PONumber" : null, "POLineNumber" : null, "SoldToLegalEntityName" : null, "CompletionLocator" : null, "ErrorMessages" : "", "ErrorMessageNames" : "", "InspectionEventId" : null }] }