5 Oracle Key Vault Client SDK Program Structure
The Oracle Key Vault client SDK program structure covers areas such as the program flow, types, environment, connection, and session.
- About the Oracle Key Vault Client SDK Program Structure
The program structure of an Oracle Key Vault SDK program describes the approach the Oracle Key Vault client will take to write an endpoint SDK program. - Oracle Key Vault Program Flow
The Oracle Key Vault general program flow includes creating the environment handle, followed by the connection and session, then termination and release. - Oracle Key Vault Program Environment
The Oracle Key Vault program environment is the region or block in the endpoint where the Oracle Key Vault functions are executed. - Oracle Key Vault Program Connection
The Oracle Key Vault program connection has two types of connections: explicit and intrinsic. - Oracle Key Vault Program Session
The Oracle Key Vault program session are of two types: Oracle Key Vault session and Oracle Key Vault call session.
Parent topic: Introduction to the Oracle Key Vault Client SDK
5.1 About the Oracle Key Vault Client SDK Program Structure
The program structure of an Oracle Key Vault SDK program describes the approach the Oracle Key Vault client will take to write an endpoint SDK program.
The Oracle Key Vault SDK itself is written using certain assumptions and the Oracle Key Vault client must write the endpoint program code in a manner that is consistent with those assumptions.
The Oracle Key Vault client SDK is available for C and Java platforms. The Oracle Key Vault SDK follows the data types, calling conventions, syntax and semantics of the programming language.
The endpoint SDK program can be compiled and linked in the same manner as any other C or Java application. There is no need for any separate pre-processing or post compilations steps.
For an endpoint program to communicate with the Oracle Key Vault server, the endpoint SDK program must follow the program flow, link Oracle Key Vault client SDK library and account for the characteristics of the Oracle Key Vault program.
Parent topic: Oracle Key Vault Client SDK Program Structure
5.2 Oracle Key Vault Program Flow
The Oracle Key Vault general program flow includes creating the environment handle, followed by the connection and session, then termination and release.
Oracle Key Vault program flows are categorized by their structure and how much KMIP detail is involved to write them: basic and advanced.
- Basic Program Flow
A basic program works seamlessly with KMIP programs. - Advanced Program Flow
The advanced program flow can be categorized as an Oracle Key Vault program with batching and detailed Oracle Key Vault program.
Parent topic: Oracle Key Vault Client SDK Program Structure
5.2.1 Basic Program Flow
A basic program works seamlessly with KMIP programs.
- It consists of Oracle Key Vault client SDK functions that perform broad KMIP operations such as creating, activating, retrieving, and registering keys or credentials with Oracle Key Vault server.
- The client need not be aware of the intricate KMIP details when using the functions.
- It contains functions to help the client focus on functionality such as creating and rotating a key, rather than handling details of the KMIP specification, setting up connections with Oracle Key Vault, and other operations.
- It is use-case driven.
- It is KMIP agnostic. Very little KMIP knowledge is required to make use of these functions.
- It can be deployed quickly.
- It integrates easily.
The disadvantage of a basic program is that not every KMIP detail is supported.
- Create the Oracle Key Vault environment handle.
- Optionally, configure the trace information.
- Configure the connection information.
- Optionally, set up the Oracle Key Vault program session.
- Create Oracle Key Vault operations or function arguments, if required.
- Execute the Oracle Key Vault operations or functions.
- Interpret and free up the Oracle Key Vault operations or function results if necessary.
- Terminate the Oracle Key Vault program session if it had been established earlier.
- Release the Oracle Key Vault environment handle.
Figure 5-1 Basic Oracle Key Vault Program Flowchart
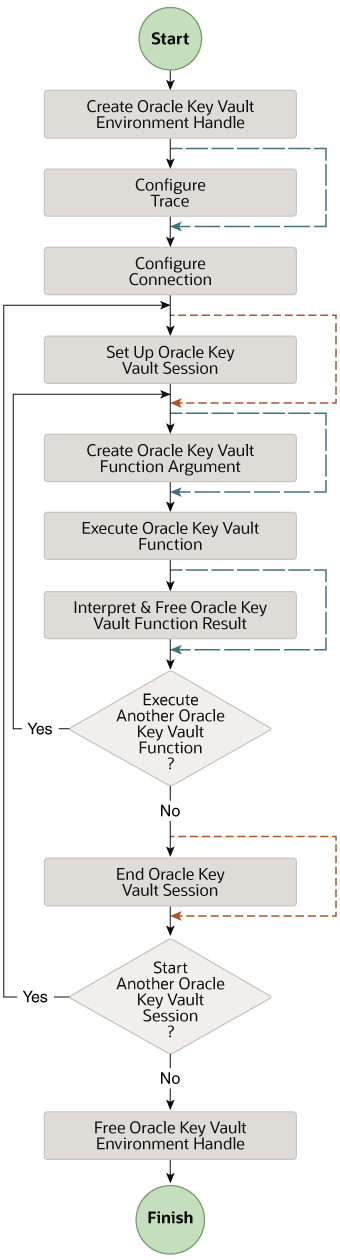
Description of "Figure 5-1 Basic Oracle Key Vault Program Flowchart"
Example 5-1 Basic Oracle Key Vault Program
... OKVEnv *env = okvEnvCreate(OKV_MEMORY_FUNCTIONS); okvEnvSetConfig(env, (oratext *)config_file, (oratext *) connection_pwd); okvEnvSetTrace(env, (oratext *)trace_dir, OKV_TRACE_DEBUG); okvConnect(env); okvCreateKey(env, ..., unique_id, &unique_id_len); printf("unique identifier %s", unique_id); okvActivate(env, unique_id); okvDisconnect(env); okvDestroy(env, unique_id); okvEnvFree(&env); ...
Parent topic: Oracle Key Vault Program Flow
5.2.2 Advanced Program Flow
The advanced program flow can be categorized as an Oracle Key Vault program with batching and detailed Oracle Key Vault program.
- Oracle Key Vault Program with Batching
Many KMIP operations such as locating and activating objects can be batched by using theokvBatchCreate
API. - Detailed Oracle Key Vault Program
The detailed Oracle Key Vault program can cover most of the use cases that are allowed by the KMIP protocol.
Parent topic: Oracle Key Vault Program Flow
5.2.2.1 Oracle Key Vault Program with Batching
Many KMIP operations such as locating and activating objects can be batched
by using the okvBatchCreate
API.
Batching two or more KMIP operations means that the KMIP request packets for both the operations will be aggregated and sent to the Oracle Key Vault server together in one super or batched KMIP packet. This reduces round trips for the information exchanged between the client SDK and the Oracle Key Vault server.
- Create the Oracle Key Vault environment handle.
- Optionally, configure the trace information.
- Configure the connection information.
- Optionally, set up the Oracle Key Vault program session.
- Start batching.
- Create the Oracle Key Vault KMIP TTLV function arguments, if required.
- Create the Oracle Key Vault functions.
- Execute the batched operations. This will execute all the Oracle Key
Vault functions and possibly process the data returned from the KMIP
server.
- Interpret any Oracle Key Vault function results, if required.
- End batching.
- Terminate the Oracle Key Vault program session if it was established earlier.
- Release the Oracle Key Vault environment handle.
The Oracle Key Vault program flow with batching is same as basic program flow except for the Oracle Key Vault function execution part and is as shown in the figure below.
Figure 5-2 Advanced Oracle Key Vault Program with Batching Flowchart
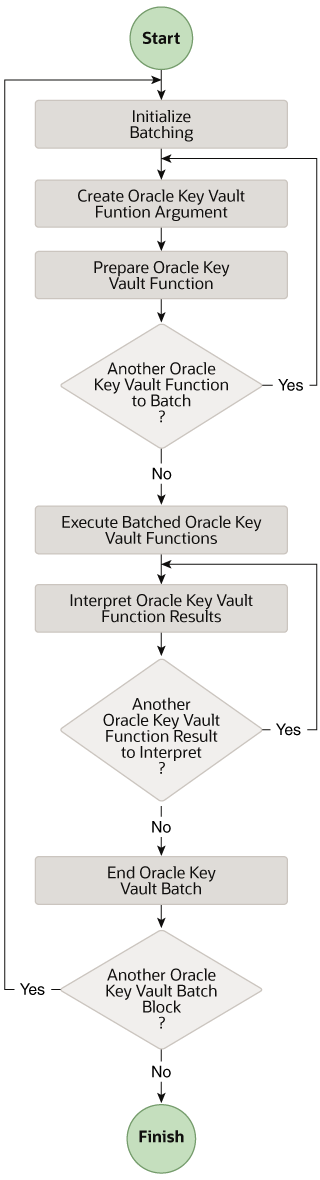
Description of "Figure 5-2 Advanced Oracle Key Vault Program with Batching Flowchart"
Example 5-2 Advanced Oracle Key Vault Program With Batching
... OKVEnv *env = okvEnvCreate(OKV_MEMORY_FUNCTIONS); okvEnvSetConfig(env, (oratext *)config_file, (oratext *) connection_pwd); okvEnvSetTrace(env, (oratext *)trace_dir, OKV_TRACE_DEBUG); okvBatchCreate(env); okvCreateKey(env, ..., unique_id, &unique_id_len); okvActivate(env, (oratext *)NULL); okvDestroy(env, (oratext *)NULL); okvBatchExecute(env); printf("unique identifier %s", unique_id); okvBatchFree(env); okvEnvFree(&env); ...
Parent topic: Advanced Program Flow
5.2.2.2 Detailed Oracle Key Vault Program
The detailed Oracle Key Vault program can cover most of the use cases that are allowed by the KMIP protocol.
A detailed Oracle Key Vault program consists of a set of Oracle Key Vault Client SDK functions that allow the client to write programs that are an actual representation of the KMIP packets being transported to the server. This requires the client to know KMIP protocol in some details.
The basic SDK program covers most of the common use cases. More complex operations or use cases can be done with a detailed Oracle Key Vault program.
The program structure is mostly the same as the basic Oracle Key Vault program structure. However, there is an additional operation handle that is used to define the KMIP operation.
The Oracle Key Vault detailed program flow can also make use of batching the multiple KMIP operations.
- Create the Oracle Key Vault environment handle.
- Optionally, configure the trace information.
- Configure the connection information.
- Optionally, configure the Oracle Key Vault program session.
- Create an Oracle Key Vault operation array for multiple KMIP operations.
- Build a KMIP request message for the Oracle Key Vault operations.
- Execute the Oracle Key Vault operations, possibly in batch mode.
- Unwrap the KMIP response message for the Oracle Key Vault operations.
- Free up the Oracle Key Vault operation array.
- Terminate the Oracle Key Vault program session if you had established it earlier.
- Optionally, configure the Oracle Key Vault program session.
- Release the Oracle Key Vault environment handle.
The detailed Oracle Key Vault program flow is same as basic program flow except for the Oracle Key Vault function execution part and is as shown in the figure below.
Figure 5-3 Detailed Oracle Key Vault Program Flowchart
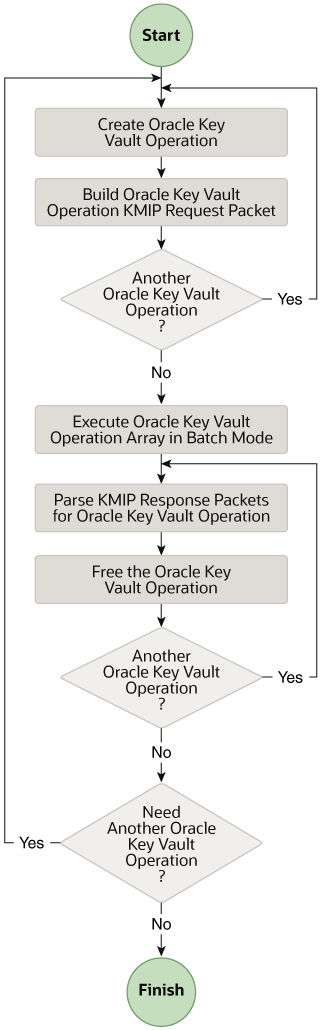
Description of "Figure 5-3 Detailed Oracle Key Vault Program Flowchart"
Example 5-3 Advanced Oracle Key Vault Detailed Program
... OKVEnv *env = okvEnvCreate(OKV_MEMORY_FUNCTIONS); /* Allocate Operation array for two KMIP operations */ OKVOps *ops[2]; ub4 alg = CRYPTO_ALG_AES; okvEnvSetConfig(env, (oratext *)config_file, (oratext *) connection_pwd); okvEnvSetTrace(env, (oratext *)trace_file, OKV_TRACE_DEBUG); /* First OKV KMIP Operation */ ops[0] = okvOpsCreate(env, OKVOpCreate); req = okvTTLVGetRequest(env, ops[0]); okvTTLVAddToObject(env, req, OKVDEF_TAG_OBJ_TYPE, ...); template = okvTTLVAddToObject(env, req, OKVDEF_TAG_TEMPLATE_ATTR_ST, ..); attr = okvTTLVAddToObject(env, template, OKVDEF_TAG_ATTR_ST, ...); okvTTLVAddToObject(env, attr, OKVDEF_TAG_ATTR_NAME, "Cryptographic Algorithm" ...); okvTTLVAddToObject(env, attr, OKVDEF_TAG_ATTR_INDEX, &ind, ..); okvTTLVAddToObject(env, attr, OKVDEF_TAG_ATTR_VALUE, &alg, ..); ... so on for crypto algorithm len and crypto mask attributes ... /* Second OKV KMIP Operation */ ops[1] = okvOpsCreate(env, OKVOpActivate); req = okvTTLVGetRequest(env, ops[1]); /* Note KMIP ID for activation is implicit since detailed operations are always batched. */ /* Execute OKV KMIP Operations in Batch mode */ okvOpsExecuteOp(env, ops, 2); /* Parse the result of the first OKV KMIP Operation */ resp = okvTTLVGetResponse(env, ops[0]); tagid = okvTTLVGetChild(env, resp, 1, OKVDEF_TAG_ID, 0, &ctag_id_len); ctag_id = okvTTLVGetValue(tagid); ctag_id[ctag_id_len] = 0; printf("\nCreated Key %s", ctag_id); /* Parse the result of the second OKV KMIP Operation */ resp = okvTTLVGetResponse(env, ops[1]); tagid = okvTTLVGetChild(env, resp, 0, OKVDEF_TAG_ID, 0, &atag_id_len); atag_id = okvTTLVGetValue(tagid); atag_id[atag_id_len] = 0; printf("\nActivated Key %s", atag_id); /* Free the two operations */ okvOpsFree(env, &ops[0]); okvOpsFree(env, &ops[1]); okvEnvFree(&env); ...
Parent topic: Advanced Program Flow
5.3 Oracle Key Vault Program Environment
The Oracle Key Vault program environment is the region or block in the endpoint where the Oracle Key Vault functions are executed.
The Oracle Key Vault program environment begins with the initialization of the Oracle Key Vault environment handle and ends with the freeing of this handle. The initialization and freeing of the Oracle Key Vault environment handle can be done in different endpoint program functions so the Oracle Key Vault environment exists across functions in the endpoint program.
The Oracle Key Vault program environment is limited to a process or thread only and, as such, the Oracle Key Vault environment will exist within a process or a thread only. The Oracle Key Vault environment handle must not be used in the threads or processes forked after the Oracle Key Vault environment is initialized. The forked processes or threads can have their own Oracle Key Vault environments.
Parent topic: Oracle Key Vault Client SDK Program Structure
5.4 Oracle Key Vault Program Connection
The Oracle Key Vault program connection has two types of connections: explicit and intrinsic.
- Explicit connections: You must configure the connection that connects to the Oracle Key Vault server explicitly, perform a few Oracle Key Vault client SDK operations, and then disconnect from the Oracle Key Vault server.
- Intrinsic connections: You must configure the connection and then execute the Oracle Key Vault client SDK function. This function sets up the connections and then ends when the task is complete.
The difference between the two approaches is the number of times the connection between endpoint SDK Program and Oracle Key Vault server has to be setup. The connections are SSL connections and could prove to be costly for performance intensive applications.
There is a two-minute time-out on the Oracle Key Vault server connections if there is no activity on the connection. For explicit connections, the connection is reset automatically if it is disconnected.
Parent topic: Oracle Key Vault Client SDK Program Structure
5.5 Oracle Key Vault Program Session
The Oracle Key Vault program session are of two types: Oracle Key Vault session and Oracle Key Vault call session.
An Oracle Key Vault program session exists from the time the endpoint program explicitly connects to the Oracle Key Vault server, to the time it explicitly disconnects from the Oracle Key Vault server. This is described as the first case in the previous section (explicit connections). There can be multiple Oracle Key Vault function calls during an Oracle Key Vault session, that is, between the time the connection is setup and is disconnected.
The second case described in the previous section (intrinsic connections) sets up the connection only for the duration of one Oracle Key Vault client SDK function. This temporary internal session is called Oracle Key Vault program call session or Oracle Key Vault call session.
In general, if there are a number of KMIP operations to be executed together in time and program space, then it is better to set up the Oracle Key Vault session. If there are just one or two operations to be executed, and if they are batched, then there is no need to set up the Oracle Key Vault session explicitly.
Oracle Key Vault program sessions exist within an Oracle Key Vault program environment. More than one Oracle Key Vault session can exist within an Oracle Key Vault program environment in a serial fashion. One Oracle Key Vault session cannot be embedded in another Oracle Key Vault session. Since the scope of an Oracle Key Vault environment is a single process or a thread, the Oracle Key Vault session is also limited to a process or thread.
An Oracle Key Vault session by definition encompasses both batched and non-batched Oracle Key Vault functions. While batching saves the round trips to the Oracle Key Vault server, an Oracle Key Vault session reduces the need to repeatedly set up a connection with the Oracle Key Vault server.
Parent topic: Oracle Key Vault Client SDK Program Structure