35.66 SDO_UTIL.TILE_GEOMETRY
Format
SDO_UTIL.TILE_GEOMETRY( geom IN SDO_GEOMETRY, x_axis_min IN NUMBER, x_axis_max IN NUMBER, y_axis_min IN NUMBER, y_axis_max IN NUMBER, tile_resolution IN NUMBER, resolution_factor IN NUMBER := 0, perform_intersection IN VARCHAR2 := 'TRUE', compute_percent IN VARCHAR2 := 'FALSE', geodetic_tolerance IN NUMBER := .05) RETURN mdsys.tile_geom_table_type DETERMINISTIC PIPELINED PARALLEL_ENABLE;
Description
Tiles a geometry based on the specified tile resolution and resolution factor.
Returns MDSYS.TILE_GEOM_TABLE_TYPE
, which is a table of
MDSYS.TILE_GEOM_TYPE
objects.
Parameters
- geom
-
Geometry to tile.
- x_axis_min
-
Minimum value along the x-axis for tiling domain. (See the Usage Notes for more information.)
- x_axis_max
-
Maximum value along the x-axis for tiling domain. (See the Usage Notes for more information.)
- y_axis_min
-
Minimum value along the y-axis for tiling domain. (See the Usage Notes for more information.)
- y_axis_max
-
Maximum value along the y-axis for tiling domain. (See the Usage Notes for more information.)
- tile_resolution
-
Tile size value. (See the Usage Notes for more information.)
- resolution_factor
-
A value factor applied to the
tile_resolution
parameter.Default value is 0. (See the Usage Notes for more information.)
- perform_intersection
-
A string value of
TRUE
(the default) clips boundary tiles to the geometry boundary.A string value of
FALSE
returns full tiles along the geometry boundary. - compute_percent
-
The default string value is
FALSE
.A string value of
TRUE
computes the value between 0 and 1. To compute this valueperform_intersection
must also be set toTRUE
. - geodetic_tolerance
-
Default is
0.05
.This parameter is only used if the geometry to tile is longitude/latitude. The default value can be overridden with a value smaller than 0.05.
Usage Notes
SDO_UTIL.TILE_GEOMETRY
function can be used to:
- Tile geometries, for example, farm plots or land parcels
- Tile geometries with the tiles that coincide with the cells of a raster.
For raster cell sized tiles, use the extent of the raster as the tiling domain, and set the
tile_resolution
to the raster resolution.
This function returns a table of type
MDSYS.TILE_GEOM_TABLE_TYPE
, which is defined as follows:
CREATE OR REPLACE TYPE mdsys.tile_geom_table_type AS TABLE OF tile_geom_type;
The object type MDSYS.TILE_GEOM_TYPE
, used in the preceding
code, is defined as follows:
CREATE OR REPLACE TYPE mdsys.tile_geom_type AS OBJECT (
tile_id NUMBER,
status CHAR,
percent NUMBER,
tile_center SDO_GEOMETRY,
geom SDO_GEOMETRY);
MDSYS.TILE_GEOM_TYPE
are:
tile_id
: A unique number assigned to each tile, beginning with 1.status
: The value can be either:'I'
: for interior tile'B'
: for boundary tile on the geometry
percent
: Percent of tile area coincident with geometry area. Value between 0 and 1. Interior tiles will always return 1.tile_center
: Center point of the tile.geom
: A geometry tile. If the parameterperform_intersection
isTRUE
, boundary tiles are clipped to the geometry boundary.
x_axis_min
, x_axis_max
,
y_axis_min
and y_axis_max
used in the
SDO_UTIL.TILE_GEOMETRY
function, represent the tiling domain. The tiling
domain is an extent that contains all the geometries that are required to tile. For example,
for longitude/latitude geometries, a tiling domain can be specified using the following
parameter values:
x_axis_min
:-180
x_axis_max
:180
y_axis_min
:-90
y_axis_max
:90
The tile_resolution
and resolution_factor
parameters influence the tile size as highlighted in the following:
- The desired tile size value is determined by the
tile_resolution
parameter. For example, for a value 5, tiles will be 5x5 or a factor of 5x5 if the parameterresolution_factor
is not 0. resolution_factor
, when specified, applies a factor to thetile_resolution
parameter, to tile a geometry with either smaller or larger tiles thantile_resolution
.The following table describes the tile size as determined by the
resolution_factor
values:Resolution Factor Value Tile Size 0 (the default) No factor is applied. Tiles size are driven by the tile_resolution
parameter.See Example-1 for more information.
> 0 Tiles generated will be a factor smaller relative to the tile_resolution
parameter. For example, for the followingresolution_factor
values:- 1: tile size is 1/4 smaller than
tile_resolution
- 2: tile size is 1/16 smaller than
tile_resolution
- 3: tile size is 1/64 smaller than
tile_resolution
See Example-2 for more information.
< 0 Tiles generated will be a factor larger relative to the tile_resolution
parameter. For example, for the followingresolution_factor
values:- -1: tile size is 4 times larger than
tile_resolution
- -2: tile size is 16 times larger than
tile_resolution
- -3: tile size is 64 times larger than
tile_resolution
See Example-3 for more information.
However, when generating tiles, smaller tiles will be fully contained by larger tiles. Also, tiles generated with different resolutions, larger or smaller, will always be aligned.
- 1: tile size is 1/4 smaller than
Examples
The following examples tile geometries with the tiles that coincide with the
cells of a raster. Also, the raster extent is projected (not longitude, latitude), so the
domain extent is set to the extent of the raster. The parameter,
perform_intersection => 'TRUE'
in the examples, causes clipping of the
boundary tiles to the boundary of the farm.
Example 1
This example tiles a farm geometry with tiles that are equal to the size of a raster cell. The resolution of the raster is 1000, so tiles will be 1000x1000
WITH part0 AS (SELECT b.tile_id, b.status, b.percent, b.tile_center, b.geom FROM farm_plots a, TABLE (sdo_util.tile_geometry(geom => a.geom, x_axis_min => 272039.5, x_axis_max => 275188.5, y_axis_min => 370575.5, y_axis_max => 380165.5, tile_resolution => 1000, resolution_factor => 0, perform_intersection => 'TRUE', compute_percent => 'TRUE', geodetic_tolerance => NULL)) b WHERE a.id = -1) SELECT tile_id, geom FROM part0 ORDER BY tile_id;
The following figure depicts the resulting output:
Figure 35-2 Tile size same as
tile_resolution
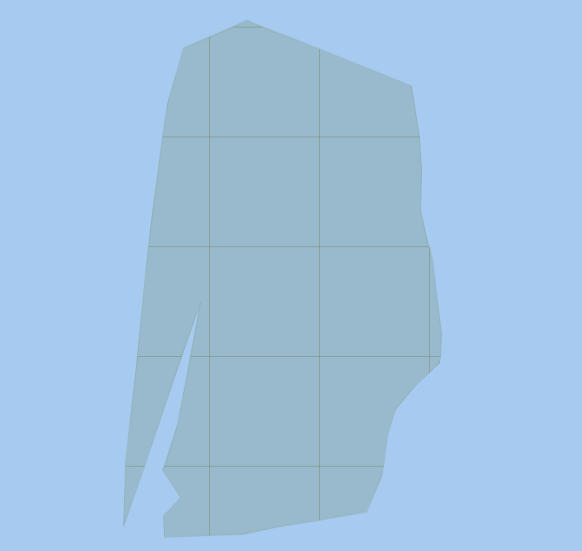
Description of "Figure 35-2 Tile size same as tile_resolution"
Example 2
The following example tiles a farm geometry with tiles that are 1/4 times
smaller than 1000 x 1000, because resolution_factor => 1
is
specified.
WITH part0 AS (SELECT b.tile_id, b.status, b.percent, b.tile_center, b.geom FROM farm_plots a, TABLE (sdo_util.tile_geometry(geom => a.geom, x_axis_min => 272039.5, x_axis_max => 275188.5, y_axis_min => 370575.5, y_axis_max => 380165.5, tile_resolution => 1000, resolution_factor => 1, perform_intersection => 'TRUE', compute_percent => 'TRUE', geodetic_tolerance => NULL)) b WHERE a.id = -1) SELECT tile_id, geom FROM part0 ORDER BY tile_id;
The following figure depicts the resulting output:
Figure 35-3 Tile size smaller than
tile_resolution
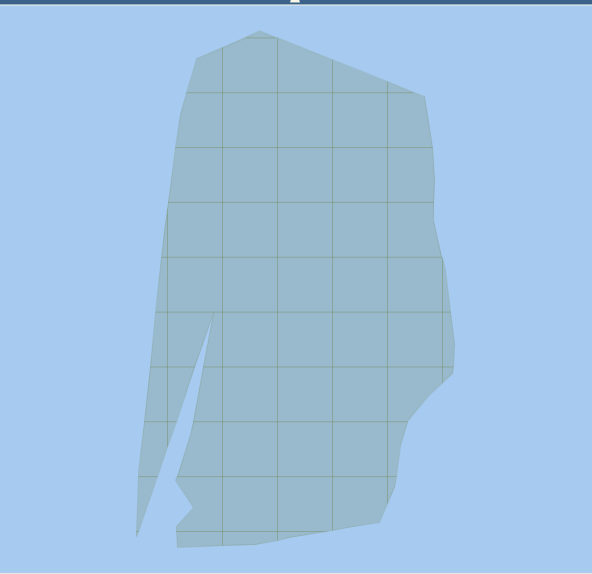
Description of "Figure 35-3 Tile size smaller than tile_resolution"
Example 3
The following example tiles a farm geometry with tiles that are 4 times greater
than 1000 x 1000, because resolution_factor => -1
is specified.
WITH part0 AS (SELECT b.tile_id, b.status, b.percent, b.tile_center, b.geom FROM farm_plots a, TABLE (sdo_util.tile_geometry(geom => a.geom, x_axis_min => 272039.5, x_axis_max => 275188.5, y_axis_min => 370575.5, y_axis_max => 380165.5, tile_resolution => 1000, resolution_factor => -1, perform_intersection => 'TRUE', compute_percent => 'TRUE', geodetic_tolerance => NULL)) b WHERE a.id = -1) SELECT tile_id, geom FROM part0 ORDER BY tile_id;
The following figure depicts the resulting output:
Figure 35-4 Tile size greater than
tile_resolution
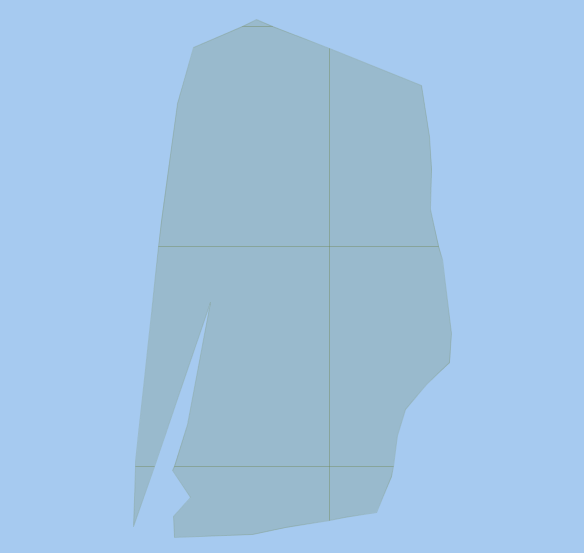
Description of "Figure 35-4 Tile size greater than tile_resolution"
Parent topic: SDO_UTIL Package (Utility)