Usage
Usage examples on Github
Example JavaScript usage (@gvt/graphviz
also contains TypeScript definitions if you are using TypeScript). For styles, please notice that you can use JavaScript expressions to filter the vertex/edges giving a condition.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello'
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World'
}
},
{
id: 3,
properties: {
name: 'Some Name'
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${properties.name}'
},
'vertex[properties.label === "blue"]': {
color: 'blue'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
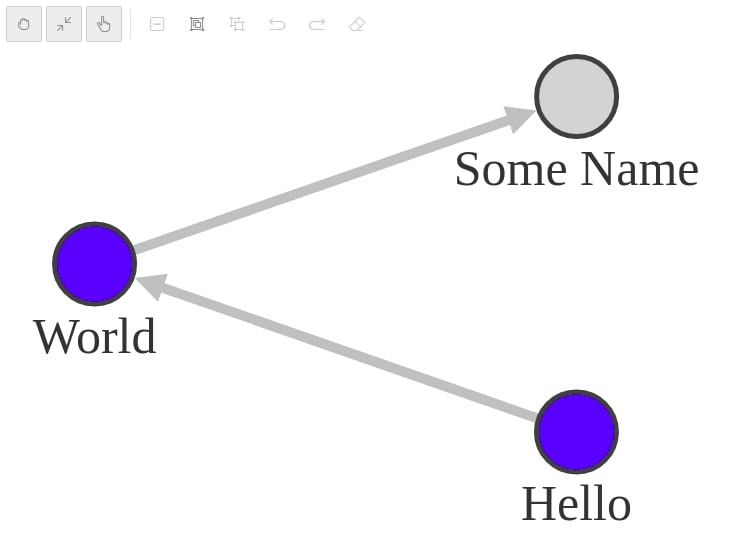
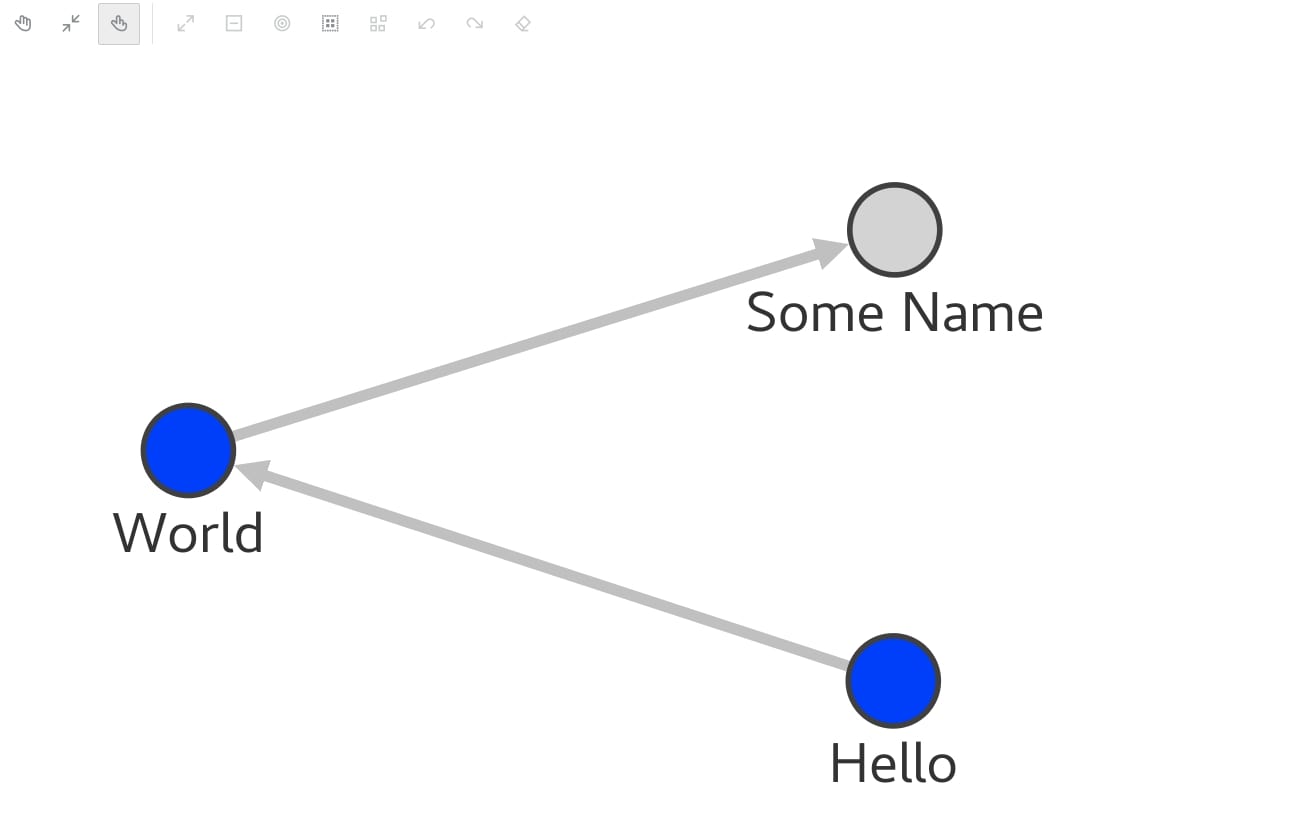
The children attribute allows for the user to to create children nodes that appear on the circumference of the nodes they are indicated. Styles can be applied like they would be applied to any node.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello'
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World'
}
},
{
id: 3,
properties: {
name: 'Some Name'
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${properties.name}',
// This would add two children to every vertex, any name can be assigned to these children nodes.
children: {
firstChild: {
size: '4',
color: 'red',
children: {
size: '2'
}
},
secondChild: {
size: '2',
color: 'green',
border: {
'width': 1,
'color': 'black'
}
}
}
},
'vertex[properties.label === "blue"]': {
color: 'blue'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
Interpolation has two use cases, it can be applied to the size or color of the vertices or edges. Additionally, interpolation has three different types, linear interpolation, discrete interpolation and color interpolation.
The normal interpolation can be used to define the size of nodes or edges within a range using a property as the value to interpolate in said range.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
// The label is changed to see the size of the node on it.
label: '${interpolate("properties.age", 1, 20)}',
// The size will be defined by the interpolation of properties.age in the range of 1 -> 20.
size: '${interpolate("properties.age", 1, 20)}'
},
'vertex[properties.label === "blue"]': {
color: 'blue'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
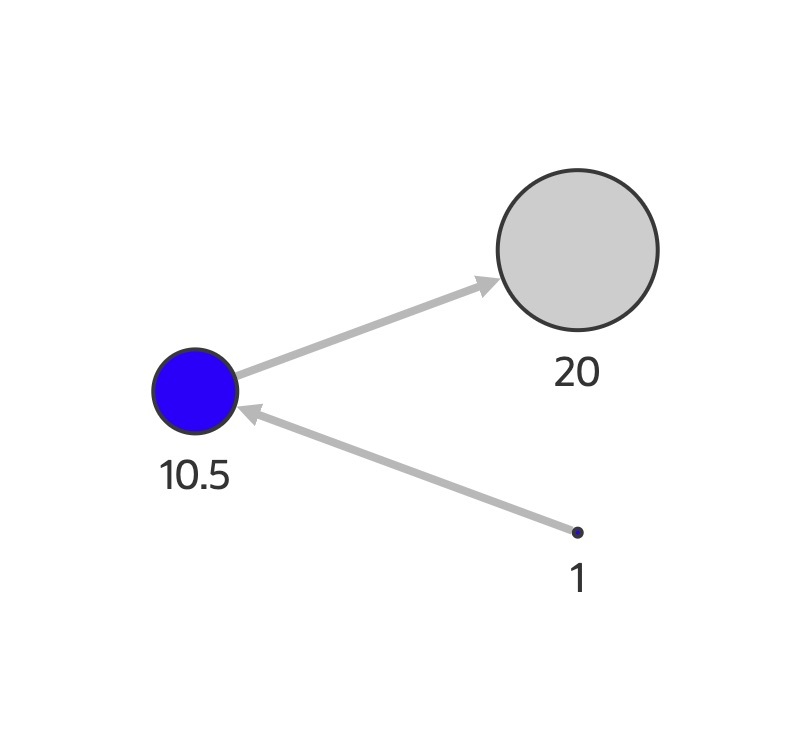
Additionally, multiple values can be used in the interpolation instead of just using one range.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
// The label is changed to see the size of the node on it.
label: '${interpolate("properties.age", 1, 20, 40)}',
// The size will be defined by the interpolation of properties.age using the values of 1, 20, 40.
size: '${interpolate("properties.age", 1, 20, 40)}'
},
'vertex[properties.label === "blue"]': {
color: 'blue'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
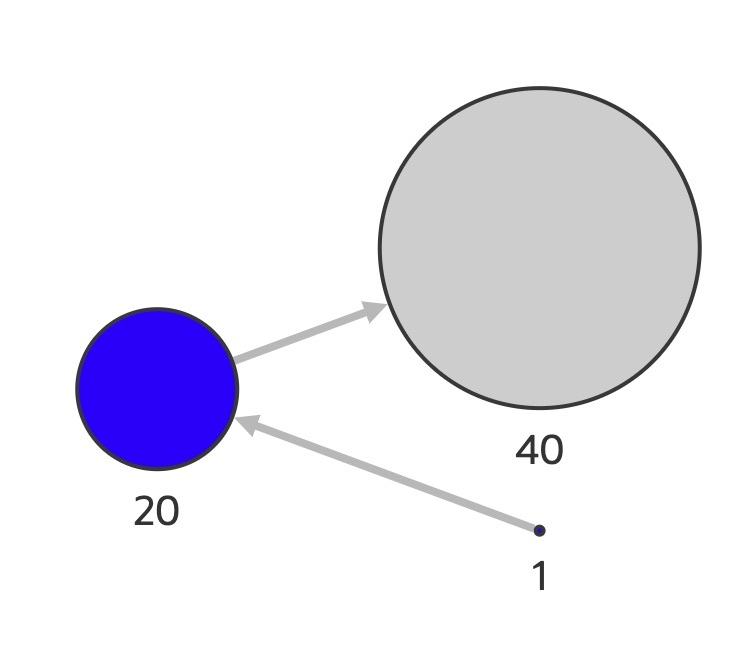
Discrete interpolation can be used to define the size of nodes or edges within a range using a property as the value to interpolate in said range. Except that contrary to the normal interpolation, the resulting values while using discrete interpolation can only be exactly the start or ending value of the range, if the property being used lies in the first half between the min and max values, it will be rounded down, and otherwise will be rounded up. Discrete interpolation can also be used for colors.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
// The label is changed to see the size of the node on it.
label: '${interpolate.discrete("properties.age", 1, 20)}',
// The size will be defined by the interpolation of properties.age in the range of 1 -> 20.
// In this example since the node with age 20 is exactly in the middle, it will be rounded up to 20.
size: '${interpolate.discrete("properties.age", 1, 20)}'
},
'vertex[properties.label === "blue"]': {
color: 'blue'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
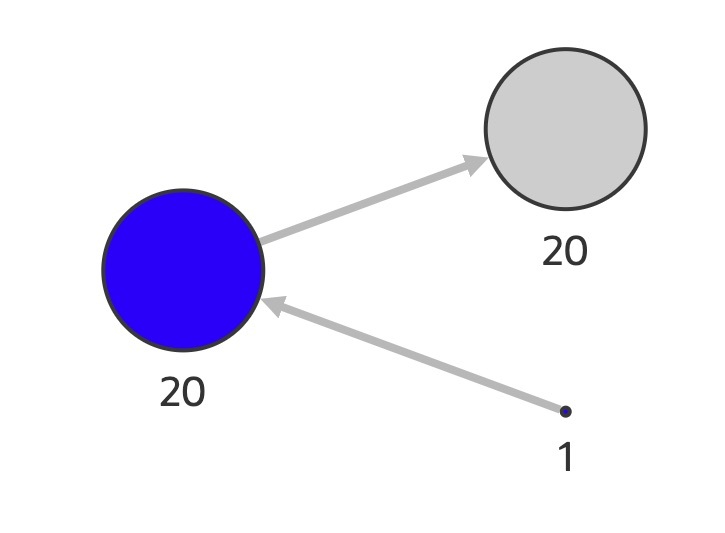
Discrete interpolation can also be used using colors. All that is required is to define the colors that want to be discretely interpolated.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${interpolate.discrete("properties.age", "black", "white")}',
color: '${interpolate.discrete("properties.age", "black", "white")}'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
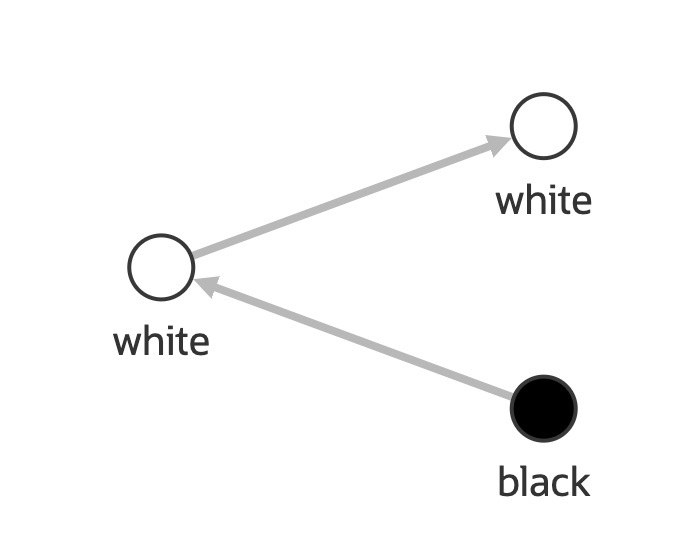
Colors can also be linearly interpolated using the interpolate.color function. All that is required is to send in the colors between which the user wants to interpolate the desired property.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${properties.age}',
color: '${interpolate.color("properties.age", "black", "white")}'
}
};
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles }
});
|
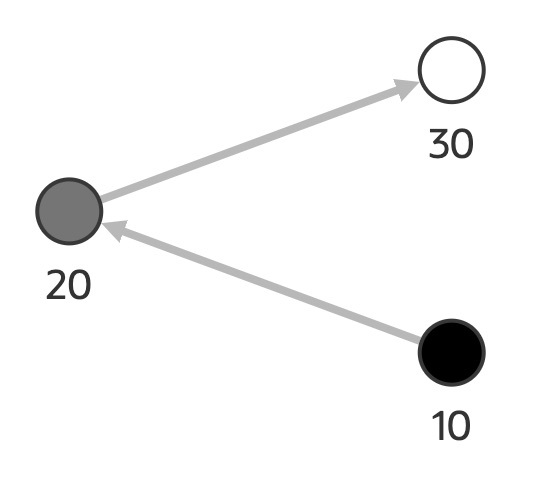
Filters can be applied on any attribute of the graph that can be found inside the properties from vertices or edges. Once the property or properties have been defined, a condition is verified and the vertices or edges are filtered accordingly on wether or not the properties return true for the given coditions.
The supported operators on which the properties are verified are =
, >
, <
, >=
, <=
, !=
and ~
which verifies if there is a match of the value on the property.
Let's say the user wants to create a filter that turns blue all vertices that have a label with value "blue" on their properties.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${properties.name}'
}
};
const settings = {
filters: [
{
// This will filter vertices
target: 'vertex',
component: 'vertex',
// This defines the properties of the filter
properties: {
// The title for the filter that will show in the legend
legendTitle: ['Filter by label'],
// The colors to apply to the nodes that are filtered
colors: ['blue']
},
// The conditions on which the filter will be applied
conditions: {
operator: 'and',
conditions:[
// This condition will verify if the label on a vertex is equals to 'blue'
{
property: 'label',
operator: '=',
value: 'blue'
}
]
}
}
]
}
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles, settings }
});
|
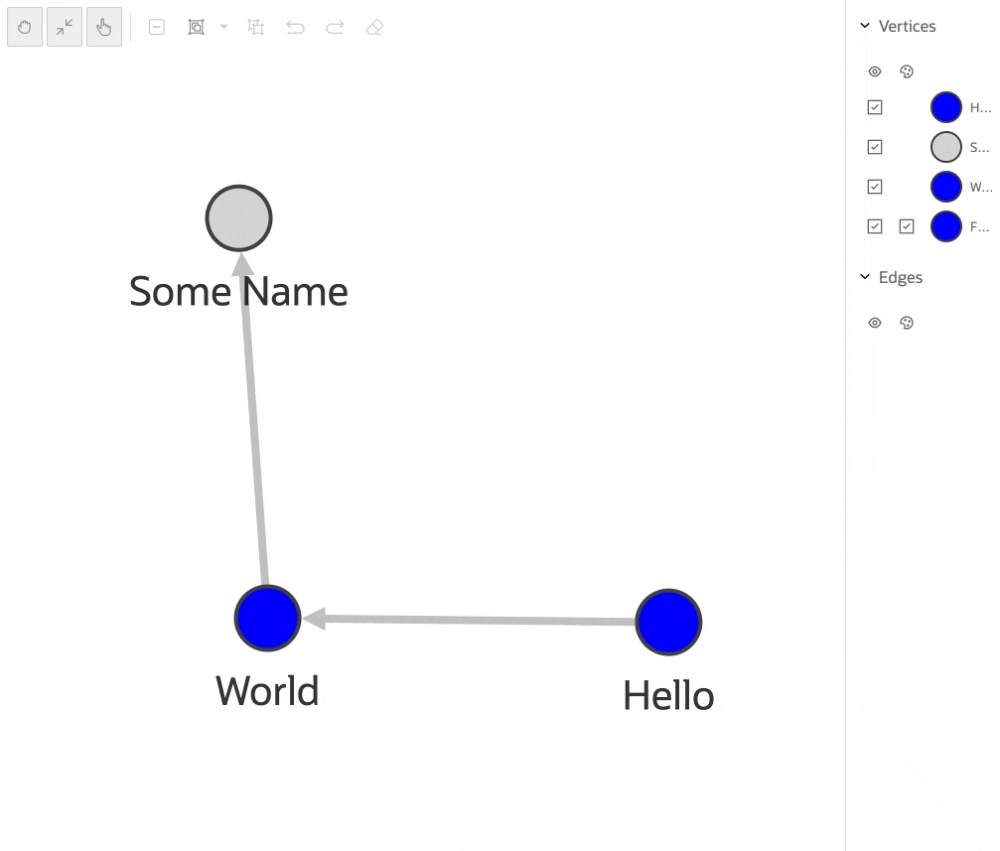
Filters can also be applied to modify the size of the nodes. Additionally, multiple conditions can be used at a time and they can be set up using the and
and or
operators such that the filter only applies if all the conditions are met or any of the conditions are met respectively.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84 | // This import is not necessary if you are using Oracle JET.
import '@ovis/graph/alta.css';
import Visualization from '@gvt/graphviz';
const vertices = [
{
id: 1,
properties: {
label: 'blue',
name: 'Hello',
age: 10
}
},
{
id: 2,
properties: {
label: 'blue',
name: 'World',
age: 20
}
},
{
id: 3,
properties: {
name: 'Some Name',
age: 30
}
}
];
const edges = [
{
id: 1,
source: 1,
target: 2
},
{
id: 2,
source: 2,
target: 3
}
];
const styles = {
vertex: {
label: '${properties.name}'
}
};
const settings = {
filters: [
{
target: 'vertex',
component: 'vertex',
properties: {
legendTitle: ['Filter by name'],
sizes: [15]
},
conditions: {
// Two conditions are being used and both conditions must be met to aplly the filter since the 'and' operator is being used
operator: 'and',
conditions:[
// This condition will verify that the name contains the letter o
{
property: 'name',
operator: '~',
value: 'o'
},
// This condition will verify that the name contains the letter l
{
property: 'name',
operator: '~',
value: 'l'
}
]
}
}
]
}
new GraphVisualization({
target: document.body,
props: { data: { vertices, edges }, styles, settings }
});
|
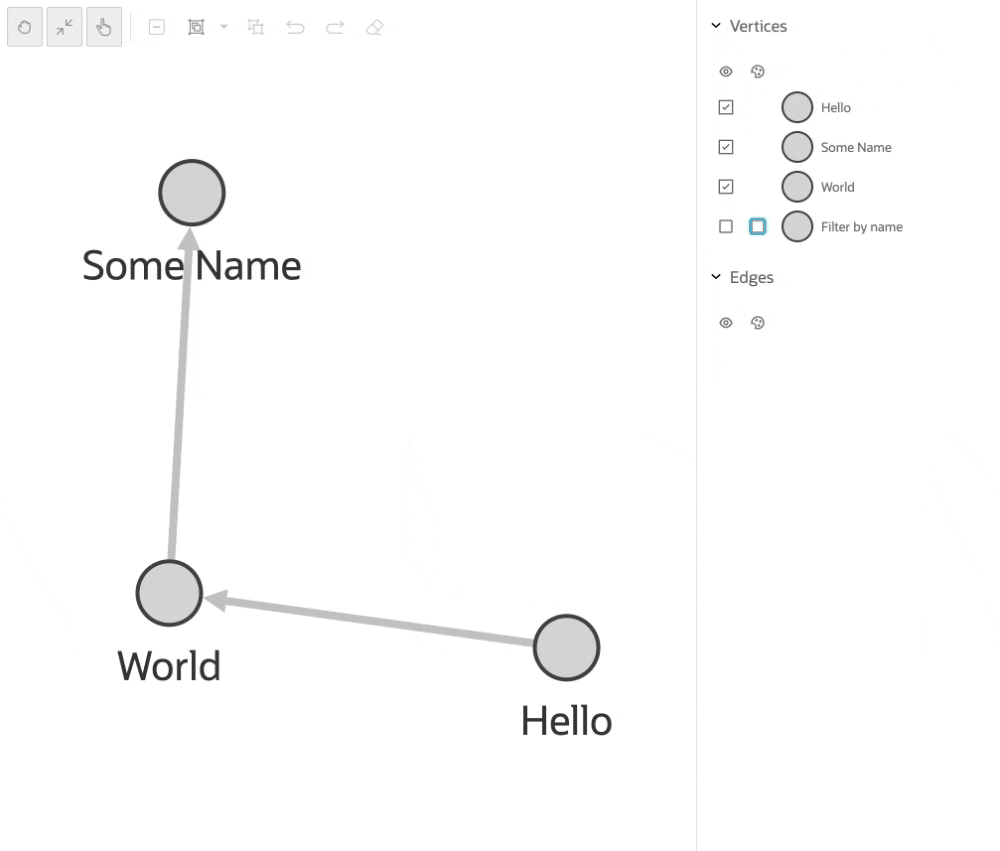