4 Implementing a Channelized Connectivity Enablement Scenario
This chapter describes implementing a channelized connectivity enablement scenario using various Oracle Communications Unified Inventory Management (UIM) application program interfaces (APIs). You can use this information to gain a better understanding of how the UIM APIs can be used to implement any channelized connectivity enablement scenario.
About the Channelized Connectivity Enablement Scenario
Figure 4-1 shows the process flow for a channelized connectivity enablement scenario:
Figure 4-1 Process Flow for a Generic Channelized Connectivity Scenario
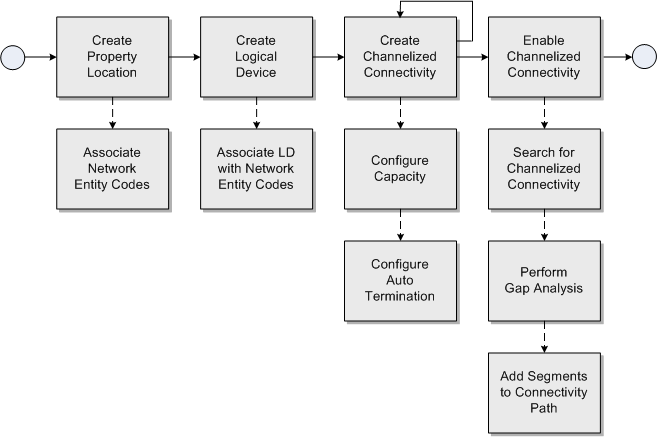
Description of "Figure 4-1 Process Flow for a Generic Channelized Connectivity Scenario"
This process flow begins with creating a property location and associating network entity codes with the property location. The network entity codes are used in subsequent steps in the process flow, such as associating them with logical devices.
The process flow continues with creating logical devices with device interfaces that can terminate on the bearer channelized connectivity, and associating logical devices with the network entity codes previously created. This involves creating logical device search criteria to find the required logical device specification.
Next is creating channelized connectivity, which represents bearer channelized connectivity between two network entity codes that define attributes of technology, rate code, and channelized connectivity function.
The process flow continues by configuring the capacity for the channelized connectivity to channelize it, and by optionally terminating them on the device interfaces of logical devices previously created. This is called auto termination of device interfaces because it also terminates the sub-device interfaces down the hierarchy to the channels when the channelized connectivity is terminated automatically. This represents the bearer channelized connectivity that will be used in enablement in subsequent steps of the process flow.
The process flow continues with creating channelized connectivity to represent the rider between two network entity codes that define attributes of technology, rate code, and channelized connectivity function. For a channelized connectivity entity to be enabled by a channel, its rate code must match or be compatible with the rate code of the channel.
Next is enabling channelized connectivity, which can be manually done by searching for and adding the bearer channelized connectivity's channel. This involves creating channelized connectivity search criteria to search for the bearer channelized connectivity and selecting the appropriate channel. Enablement can also be done by adding bearer channelized connectivity through gap analysis to the rider that involves creating path analysis criteria to search for the bearer channelized connectivity between a source/intermediate/target property locations or logical devices.
Now that you have a high-level understanding of the channelized connectivity enablement scenario process flow, each part of the process flow is further described in the following sections. Each section includes information about the specific UIM APIs used to perform each step. Example code is also included for each step.
Creating a Property Location and Associating Network Entity Codes
This section describes the UIM API methods used to create a property location and to associate network entity codes with the property location.
Table 4-1, Table 4-2, and example code provide information about using the API methods to create a property location and to associate network entity codes to the property location.
Table 4-1 Creating a Property Location
Topic | Information |
---|---|
Name |
LocationManager.createPropertyLocation (Collection<PropertyLocation> locations) |
Description |
Creates the Property Location instances with the given inputs. User has to specify one mandatory Primary address as input with which a property Location has to be created. Every property location also has a property address associated with it. |
Pre-Condition |
The locations parameter needs to be prepared with necessary attributes |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
|
Table 4-2 Associating Network Entity Codes with a Property Location
Topic | Information |
---|---|
Name |
LocationManager.associateNetworkEntityCodeToNetworkLocation (List<NetworkEntityCode> entitycodes, PropertyLocation location) |
Description |
This method is called during the association or creation of the network entity code in the context of property location. |
Pre-Condition |
The location parameter already exists. |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
|
Example 4-1 Creating a Property Location and Associating Network Entity Codes with the Property Location
Finder finder = PersistenceHelper.makeFinder(); PropertyLocation propertyLocation = locationManager.makePropertyLocation(); PropertyAddress propertyAddress = locationManager.makePropertyAddress(); LocationManager locationManager = PersistenceHelper.makeLocationManager(); //Set all necessary attributes needed for Property Address and Property Location propertyAddress.setStreetAddress((String)paramMap.get("streetAddress")); propertyAddress.setCity((String)paramMap.get("city")); propertyAddress.setState((String)paramMap.get("state")); propertyAddress.setCountry((String)paramMap.get("country")); propertyAddress.setIsValidated(Boolean.valueOf ((String)paramMap.get("isValidated"))); propertyAddress.setIsNonValidatedAddressAccepted(true); propertyAddress.setIsPrimaryAddress(true); Set<PropertyAddress> addressSet = new HashSet<PropertyAddress>(1); addressSet.add(propertyAddress); propertyLocation.setPropertyAddresses(addressSet); propertyLocation.setNetworkLocationCode("PLANO"); propertyLocation.setLatitude("34"); propertyLocation.setLongitude("54"); Collection<PropertyLocation> list = new ArrayList<PropertyLocation>(1); list.add(propertyLocation); List<PropertyLocation> propLocobjects = locationManager. createPropertyLocation(list); networkLocation = propLocobjects.get(0); List<NetworkEntityCode> networkEntityCodes = new ArrayList<NetworkEntityCode>(); NetworkEntityCode nec = locationManager.makeNetworkEntityCode(); nec.setName(necStr); networkEntityCodes.add(nec); if (!Utils.isEmpty(networkEntityCodes)) { locationManager.associateNetworkEntityCodeToNetworkLocation (networkEntityCodes,networkLocation); }
Creating a Logical Device and Associating LD Interfaces with Network Entity Codes
This section describes the UIM API methods used to create a logical device with default logical device interfaces, and to associate the logical device interfaces with the previously created network entity codes.
Table 4-3 and example code provide information about using the API method to create a logical device with default logical device interfaces.
Table 4-3 Creating a Logical Device
Topic | Information |
---|---|
Name |
LogicalDeviceManager.createLogicalDevice (Collection<LogicalDevice> logicalDevices) |
Description |
Creates logical device entities and their provided device interfaces and sub-device interfaces based on the specification. |
Pre-Condition |
Logical device specification with device interfaces is defined and exists already. |
Internal Logic |
Device interfaces can also provide other device interfaces. The number of device interfaces to be created will be determined by the minimum value defined in the specification relationships. The input logical device entities should be sparsely populated with the specification, hard attributes and characteristics. The provided device interfaces will be derived based on the specification. Characteristics will be defaulted based on the specification. The id of the device interfaces will be generated. If required characteristics exist for a provided device interface that are not defaulted, then the logical device will still be created. |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-2 Creating a Logical Device with Default Logical Device Interfaces
Finder finder = PersistenceHelper.makeFinder(); LogicalDeviceManager ldMgr = PersistenceHelper.makeLogicalDeviceManager(); Collection<Specification> specs = finder.findByName(Specification.class,"ldSpecName"); LogicalDeviceSpecification ldSpec = (LogicalDeviceSpecification)specs.iterator().next(); LogicalDevice ld = ldMgr.makeLogicalDevice(); ld.setName("ldName"); ld.setId("ldId"); ld.setSpecification(ldSpec); List<LogicalDevice> ldList = new ArrayList<LogicalDevice>(); ldList.add(ld); ldMgr.createLogicalDevice(ldList);
The following table and example code provide information about using the API method to associate a logical device with a network entity code.
Table 4-4 Associating a Logical Device with a Network Entity Code
Topic | Information |
---|---|
Name |
LogicalDeviceManager.updateLogicalDevice (Collection<LogicalDevice> logicalDevices) |
Description |
This method is intended to update the hard attributes and characteristics of a logical device. |
Pre-Condition |
Logical device exists already. The location of a logical device can only be changed if it does not have any active consumers or interconnections on the logical device or any of its device interfaces. |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-3 Associating a Logical Device with a Network Entity Code
Finder finder = PersistenceHelper.makeFinder(); LogicalDeviceManager ldMgr = PersistenceHelper.makeLogicalDeviceManager(); LocationManager locationManager = PersistenceHelper.makeLocationManager(); // find an existing logical device LogicalDevice ld = finder.findById(LogicalDevice.class, "ldId").iterator().next(); // find an existing property location that has network entity code PropertyLocation pls = (PropertyLocation)locationManager.findNetworkEntityLocation("PLANO"); ld.setPropertyLocation(pls); NetworkEntityCodeSearchCriteria criteria = locationManager.makeNetworkEntityCodeSearchCriteria(); criteria.setPropertyLocation(pls); //find network entity code matching "001" List<NetworkEntityCode> networkEntityCodes = locationManager.findNetworkEntityCodes(criteria); NetworkEntityCode networkEntCd = null; if (!Utils.isEmpty(networkEntityCodes)) { String networkEntityCod= "001"; for (NetworkEntityCode nec : networkEntityCodes) { if ((pls.getNetworkLocationCode() + "." + networkEntityCode).equals nec.getNetworkLocationEntityCode())) { networkEntCd = nec; } } } ld.setNetworkEntityCode(networkEntCd); networkEntCd.setLogicalDevice(ld); List<LogicalDevice> ldList = new ArrayList<LogicalDevice>(); ldList.add(ld); ldMgr.updateLogicalDevice(ldList);
Creating Channelized Connectivity
This section describes the UIM API methods used to:
Create Channelized Connectivity
Table 4-5 and example code provide information about using the API method to create channelized connectivity. (You use the same API method to create the bearer channelized connectivity and the rider channelized connectivity.)
Table 4-5 Creating Channelized Connectivity
Topic | Information |
---|---|
Name |
ConnectivityManager.createConnectivity(N connectivity, String aNetworkLocationEntityCode, String zNetworkLocationEntityCode, int quantity, boolean contiguousSerialAllocation) |
Description |
This method will create channelized connectivity. Valid A Location and Z Location must be set on the channelized connectivity instance. |
Pre-Condition |
Two property locations to represent A and Z side of the channelized connectivity already exists. ora_uim_basetechnologies is already installed. |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-4 Creating Channelized Connectivity
String rateCode = "STM1; String function = "SM01"; String aLocation = "DALLAS"; String zLocation = "PLANO"; String aEntityCode = "DALLAS.001"; String zEntityCode = "PLANO.001"; int qtyInt = 1; boolean isContiguos = "true"; TDMConnectivityManager manager = (TDMConnectivityManager)PersistenceHelper.makeConnectivityManager (TDMConnectivity.class); Finder finder = PersistenceHelper.makeFinder(); NetworkConnectivity c = manager.makeTDMFacility(); NetworkConnectivity nc = (NetworkConnectivity)c; String technology = finder.findByName(Technology.class, "SDH").iterator().next(); nc.setTechnology(technology); finder.reset(); String rateCode = finder.findByName(RateCode.class, "STM1").iterator().next(); nc.setRateCode(rateCode); finder.reset(); String function = finder.findByName(ConnectivityFunction.class,"SM01").iterator().next(); nc.setConnectivityFunction(function); String aLocationCode = aLocation; if(!Utils.isEmpty(aEntityCode)){ aLocationCode = aLocation+"."+aEntityCode;} String zLocationCode = zLocation; if(!Utils.isEmpty(zEntityCode)){ zLocationCode = zLocation+"."+zEntityCode;} int tempQty = qtyInt; while(tempQty >0) { if(tempQty > 99){ qtyInt = 99;} else{ qtyInt = tempQty;} Collection<TDMConnectivity> createdConnectivities = manager.createConnectivity(c, aLocationCode, zLocationCode, qtyInt, isContiguos); }
Configure Capacity on the Channelized Connectivity
Table 4-6 and example code provide information about using the API method to configure capacity on the channelized connectivity.
Table 4-6 Configuring Capacity on the Channelized Connectivity
Topic | Information |
---|---|
Name |
SignalTerminationPointManager.applyCapacityConfiguration (MultiplexedFacility connectivity, List<RateCode> orderedRateCodes, String signalAddress) |
Description |
This method configures a connectivity to the required rate code level and also creates channels at those levels. |
Pre-Condition |
Not applicable |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Also call TDMConnectivityManager.createAndAutoTerminateChannels(M multiplexedFacility, boolean doValidation) to ensure terminations are also adjusted accordingly. |
Example 4-5 Configuring Capacity on the Channelized Connectivity
Finder finder = PersistenceHelper.makeFinder(); String connectivityIdentifier = "ALLNTXC01 / FRSCTXC01 / STM1 / SM01 / 1"; String sourceRateCode = "OM80"; String destinitionRateCode = "OM32"; RateCode sourceRC = finder.findByName(RateCode.class, sourceRateCode).iterator().next(); RateCode destinitionRC = finder.findByName(RateCode.class, destinitionRateCode).iterator().next(); TDMConnectivityManager mgr = (TDMConnectivityManager)PersistenceHelper.makeConnectivityManager (TDMFacility.class); TDMConnectivitySearchCriteria criteria = mgr.makeTDMSearchCriteria(); CriteriaItem item = criteria.makeCriteriaItem(); item.setName("connectivityIdentifier"); item.setValue("connectivityIdentifier); item.setOperator(CriteriaOperator.EQUALS); criteria.setConnectivityIdentifier(item); TDMFacility tdm = mgr.findTDMConnectivities(criteria).iterator().next(); SignalTerminationPointManager stpMgr = PersistenceHelper.makeSignalTerminationPointManager(); List<RateCode> orderedRateCodes = new ArrayList<RateCode>(); if (sourceRC != null){ orderedRateCodes.add(sourceRC);} if (destinitionRC != null){ orderedRateCodes.add(destinitionRC);} stpMgr.applyCapacityConfiguration(tdm, orderedRateCodes, ""); mgr.createAndAutoTerminateChannels(tdm, true);
Configure Auto Termination on the Channelized Connectivity
Table 4-7 and example code provide information about using the API method to configure auto-termination on the channelized connectivity.
Table 4-7 Auto-terminating the Channelized Connectivity
Topic | Information |
---|---|
Name |
ConnectivityManager.assignDeviceInterface(E connectivity, DeviceInterface di, ConnectivityEndpoint endpoint) |
Description |
This method terminates the channelized connectivity with the device interface at the given end point. Also auto-terminates the channels on the sub-device interfaces. |
Pre-Condition |
Ensure the capacity is configured at the required level on the channelized connectivity and the sub-device interfaces are created beforehand until that level. |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-6 Auto-Terminating the Channelized Connectivity
Finder finder = PersistenceHelper.makeFinder(); String tdmName = "DS3_TDM_Tail"; String diId = "DS3-1-1"; ConnectivityEndpoint endPoint = ConnectivityEndpoint.A_ENDPOINT; DeviceInterface di = finder.findById(DeviceInterface.class, diId).iterator().next(); finder.reset(); TDMFacility tdm = finder.findByName(TDMFacility.class, tdmName).iterator().next(); TDMConnectivityManager manager = (TDMConnectivityManager) PersistenceHelper.makeConnectivityManager(TDMConnectivity.class); tdm = (TDMFacility) manager.assignDeviceInterface(tdm, di, endPoint);
Enabling Channelized Connectivity
This section describes the UIM API methods used to enable channelized connectivity by:
Manually Enabling Channelized Connectivity
Table 4-8 and example code provide information about using the API method to manually enable channelized connectivity by manually searching for the channelized connectivity and adding segments to the connectivity path.
Table 4-8 Manually Enabling Channelized Connectivity
Topic | Information |
---|---|
Name |
ConnectivityManager.addSegmentsToConnectivityPath(E connectivityTrail, PipeConfigurationItem connectivityPath, PipeConfigurationItem gapItem, List<Pipe> bearerList) throws ValidationException |
Description |
The connectivityTrail parameter is the channelized connectivity that will be enabled. The connectivityPath parameter is the PipeConfigurationItem of the path. The gapItem parameter is the PipeConfigurationItem of the gap that will be resolved. The bearerList parameter contains other connectivities to be added for enablement. See Oracle Communications Information Model Reference for information on PipeConfigurationItem. |
Pre-Condition |
Not applicable |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-7 Manually Enabling Channelized Connectivity by Searching for the Connectivity and Adding Segments to the Connectivity Path
String trailName = "EDINBURGH.002 / LONDON.001 / VC12 / VC12 / 1"; //We want to add connectivities to first path int pathIndex = "0"; //Assuming there are other connectivities already added to this path int gapIndex = "0"; PersistenceHelper.makeBusinessInteractionManager().switchContext ((String)null, null); Finder finder = PersistenceHelper.makeFinder(); Connectivity connectivityTrail = finder.findByName(Connectivity.class, trailName).iterator().next(); List<String> bearers = new ArrayList<String>(); bearers.add("EDINBURGH.001 / EDINBURGH.002 / STM4 / SM04 / 139 / 1-1-1-2"); bearers.add("EDINBURGH.001 / MACHESTER.001 / STM4 / SM04 / 139 / 1-1-1-2"); bearers.add("LONDON.001 / MACHESTER.001 / STM4 / SM04 / 139 / 1-1-1-2"); List<Pipe> bearerList = new ArrayList<Pipe>(bearers.size()); for (String bearerName : bearers) { finder.reset(); Pipe connectivity = finder.findByName (TDMFacility.class, bearerName).iterator().next(); bearerList.add(connectivity); } PipeConfigurationVersion designVersion = ConnectivityUtils.getInProgressDesignVersion((Pipe)connectivityTrail); List<PipeConfigurationItem> allPaths = PipeHelper.getAllTransportItems(designVersion); PipeConfigurationItem connectivityPath = allPaths.get(pathIndex); PipeConfigurationItem gapItem = connectivityPath.getChildConfigItems().get(gapIndex); ConnectivityManager manager = PersistenceHelper.makeConnectivityManager(); manager.addSegmentsToConnectivityPath (connectivityTrail, connectivityPath, gapItem, bearerList);
Performing Gap Analysis
Table 4-9 and example code provide information about using the API method to perform gap analysis.
Table 4-9 Performing Gap Analysis
Topic | Information |
---|---|
Name |
List<PathResultSet> findPaths(PipeSpecification enabledPipe, PathAnalysisCriteria criteria) throws ValidationException |
Description |
The enabledPipe parameter is the channelized connectivity to be enabled. The criteria parameter is used in performing gap analysis. |
Pre-Condition |
Ensure the channelize connectivities that you are expecting the results are already created, terminated, and their capacity is configured. |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-8 Performing Gap Analysis
String sourceLocationCode = "EDINBURGH.002"; String intermediateLocationCode = "MACHESTER.001"; String targetLocationCode = "LONDON.001"; String rateCodeName = "VC12"; LocationManager locationManager = PersistenceHelper.makeLocationManager(); TopologyObject sourceNode = (TopologyObject)locationManager.findNetworkEntityLocation(sourceLocationCode); TopologyObject targetNode = (TopologyObject)locationManager.findNetworkEntityLocation(targetLocationCode); TopologyObject intermediateNode = null; if(!Utils.isEmpty(intermediateLocationCode)){ intermediateNode = (TopologyObject)locationManager.findNetworkEntityLocation (intermediateLocationCode); } if(sourceNode == null || targetNode == null || (!Utils.isEmpty(intermediateLocationCode) && intermediateNode == null)){ throw new IllegalArgumentException("Invalid source/intermediate/target"); } RateCode rateCode = null; CapacityManager capacityManager = PersistenceHelper.makeCapacityManager(); RateCodeSearchCriteria rateCodeSC = capacityManager.makeRateCodeSearchCriteria(); CriteriaItem rateCodeNameItem = rateCodeSC.makeCriteriaItem(); rateCodeNameItem.setName(rateCodeName); rateCodeNameItem.setOperator(CriteriaOperator.EQUALS); rateCodeNameItem.setValue(rateCodeName); rateCodeSC.setName(rateCodeNameItem); List<RateCode> rateCodes = capacityManager.findRateCode(rateCodeSC); if (!Utils.isEmpty(rateCodes)) { rateCode = rateCodes.get(0); } if(rateCode == null){ throw new IllegalArgumentException("Invalid rateCode"); } PathAnalysisCriteria criteria = new PathAnalysisCriteria(); criteria.setSourceNode(sourceNode); criteria.setIntermediateNode(intermediateNode); criteria.setTargetNode(targetNode); criteria.setRateCode(rateCode); criteria.setGapAnalysis(true); PathAnalysisManager pathAnalysisManager = PersistenceHelper.makePathAnalysisManager(); List<PathResultSet> paths = pathAnalysisManager.findPaths(criteria);
Adding Segments To Connectivity Path Based on the Gap Analysis Results
Table 4-10 and example code provide information about using the API method to add segments to the connectivity path based on the gap analysis results.
Table 4-10 Adding Segments to Connectivity Path Based on Gap Analysis Results
Topic | Information |
---|---|
Name |
ConnectivityManager.addSegmentsToConnectivityPath (E connectivityTrail, PipeConfigurationItem connectivityPath, PipeConfigurationItem gapItem, PathResultSet path) throws ValidationException; |
Description |
The connectivityTrail parameter is the channelized connectivity that will be enabled. The connectivityPath parameter is the PipeConfigurationItem representing the path to which the segments have to be added. The gapItem parameter is the PipeConfigurationItem of the gap that will be resolved. The path parameter is the results returned from gap analysis. (You can pass the results retrieved in the previous example. For example, paths.get(0)). See Oracle Communications Information Model Reference for information on PipeConfigurationItem. |
Pre-Condition |
Not applicable |
Internal Logic |
Not applicable |
Post-Condition |
Not applicable |
Extensions |
Not applicable |
Tips |
Not applicable |
Example 4-9 Adding Segments to Connectivity Path Based on Gap Analysis Results
String trailName = "EDINBURGH.002 / LONDON.001 / VC12 / VC12 / 1"; //We want to add connectivities to first path int pathIndex = "0"; //Assuming there are other connectivities already added to this path int gapIndex = "0"; PersistenceHelper.makeBusinessInteractionManager().switchContext ((String)null, null); Finder finder = PersistenceHelper.makeFinder(); Connectivity connectivityTrail = finder.findByName(Connectivity.class, trailName).iterator().next(); PipeConfigurationVersion designVersion = ConnectivityUtils.getInProgressDesignVersion((Pipe)connectivityTrail); List<PipeConfigurationItem> allPaths = PipeHelper.getAllTransportItems(designVersion); PipeConfigurationItem connectivityPath = allPaths.get(pathIndex); PipeConfigurationItem gapItem = connectivityPath.getChildConfigItems().get(gapIndex); ConnectivityManager manager = PersistenceHelper.makeConnectivityManager(); /*Here paths are the path returned by gap analysis. Assuming the first one is the list is selected*/ manager.addSegmentsToConnectivityPath (connectivityTrail, connectivityPath, gapItem, paths.get(0));