HDR RIM Service Hook
RIM Services raise workflow business events to which applications can subscribe. You can write subscription classes to listen to such events. This provides a loose coupling with the RIM Service that lets you integrate or disintegrate unique functionality by subscribing or unsubscribing to business events.
With this hook, you can code a Subscription Class subscribed to a business event; you are expected to include custom functionality in this class. The following sequence figure 10.4.1 illustrates the interaction between an application persisting data and a custom Subscription class:
Figure 10-2
Figure 9-3 Interaction between App Persisting Data and Custom Subscription Class
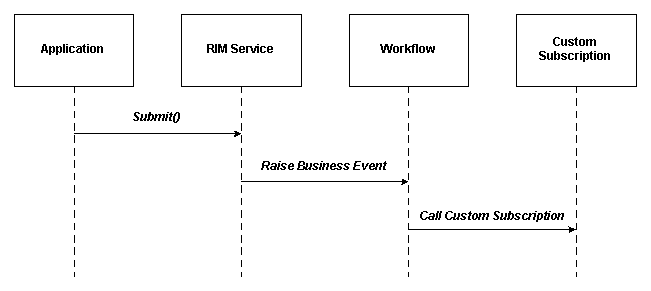
The following sections describe the RIM Service Event and Subscription, and include a Subscription Code Example:
Event and Subscription
The RIM Service raises event oracle.hsgbu.hdr.hl7.persist.event.ControlActSubmissionPost on submit. It passes trigger event and control act identifiers of the persisted data to the event in key-value pairs:
Keys
Key |
Description |
TRG_EVNT |
Trigger event of persisted message |
CONTROL_ACT_ID |
Control act identifier root of persisted message |
CONTROL_ACT_EXT |
Control act identifier extension of persisted message. |
Example 9-7 Event and Subscription
When RIM Service raises the event oracle.hsgbu.hdr.hl7.persist.event.ControlActSubmissionPost, workflow calls the onBusinessEvent method of the customer subscription class. All of the forgoing parameters are bundled in the BusinessEvent object and passed to the subscription class. The Subscription class can read those parameters in its onBusinessEvent method as illustrated in the following example:
public void onBusinessEvent(Subscription eo, BusinessEvent event, WorkflowContext wfCxt) throws BusinessEventException { try { String TriggerEvent = event.getStringProperty("TRG_EVNT"); String ControlActId_Root = event.getStringProperty("CONTROL_ACT_ID"); String ControlActId_Extension = event.getStringProperty("CONTROL_ACT_EXT"); ... } catch (Exception e) { e.printStackTrace(); } }
Subscription Code Sample
The RIM Service receives an HL7 message, persists and resends the message to all organizations registered for that trigger event.
The functionality to resend the message to all organizations registered for the trigger event can be implemented in a subscription class to be executed when the RIM Service persists that message.
Example 9-8 Subscription Code Example
package oracle.hsgbu.hdr.sample.scenario; import java.io.IOException; import java.io.InputStream; import java.io.PrintWriter; import java.io.StringWriter; import java.util.Properties; import oracle.hsgbu.hdr.fwk.base.common.ETSException; import oracle.hsgbu.hdr.fwk.serviceLocator.common.ServiceLocator; import oracle.hsgbu.hdr.hl7.domain.NullFlavor; import oracle.hsgbu.hdr.hl7.factories.DataTypeFactory; import oracle.hsgbu.hdr.hl7.types.II; import oracle.hsgbu.hdr.hl7.types.ST; import oracle.hsgbu.hdr.hl7.types.UID; import oracle.hsgbu.hdr.message.omprocessor.ControlActRequest; import oracle.hsgbu.hdr.message.omprocessor.OMPHelper; import oracle.hsgbu.hdr.message.omprocessor.OMPService; import oracle.hsgbu.hdr.security.Responsibility; import oracle.hsgbu.hdr.security.SessionContext; import oracle.hsgbu.hdr.security.SessionService; import oracle.apps.fnd.wf.bes.BusinessEvent; import oracle.apps.fnd.wf.bes.BusinessEventException; import oracle.apps.fnd.wf.bes.SubscriptionInterface; import oracle.apps.fnd.wf.bes.server.Subscription; import oracle.apps.fnd.wf.common.WorkflowContext; public class WorkflowListenerSubscription implements SubscriptionInterface { protected ServiceLocator mServiceLocator = null; public void onBusinessEvent(Subscription eo, BusinessEvent event, WorkflowContext wfCxt) throws BusinessEventException { try { // Get OMPService OMPService mOMPService = getServiceLocator().getOMPService(); // Read parameres passed by RIM Service persistence. String triggerEvent = event.getStringProperty("TRG_EVNT"); String controlActId_Root = event.getStringProperty("CONTROL_ACT_ID"); String controlActId_Ext = event.getStringProperty("CONTROL_ACT_EXT"); DataTypeFactory mDataTypeFactory = DataTypeFactory.getInstance(getServiceLocator()); UID uidVal = mDataTypeFactory.newUID(controlActId_Root); ST extVal = mDataTypeFactory.newST(controlActId_Ext); II controlActId = mDataTypeFactory.newII(uidVal, extVal, mDataTypeFactory.nullBL(NullFlavor.NI)); // Create ControlActRequest OMPHelper ompHelper = new OMPHelper(); ControlActRequest ompCACTRequest = ompHelper.newControlActRequest(); ompCACTRequest.setControlActId(controlActId); ompCACTRequest.setTriggerEvent(triggerEvent); // Generate message mOMPService.generateMessage(ompCACTRequest);r } catch (Exception e) { throw new BusinessEventException(e.getMessage()); } } protected ServiceLocator getServiceLocator() throws ETSException,IOException { if (mServiceLocator != null) { return mServiceLocator; } ClassLoader loader = Thread.currentThread().getContextClassLoader(); Properties props = new Properties(); props.load(loader.getResourceAsStream("jndi.properties")); //Specify Client mode: Local or Remote props.setProperty(ServiceLocator.CLIENT_MODE, ServiceLocator.REMOTE); ServiceLocator mServiceLocator = ServiceLocator.getInstance(props); mServiceLocator.login("sysadmin","sysadmin"); return mServiceLocator; } }
See also:
- Oracle Workflow Administrator's Guide
- Oracle Workflow API Reference
- Oracle Workflow Developer's Guide
- Oracle Workflow User's Guide