4 RESTful APIs
The following RESTful APIs are available:
-
UserRestService: Users
-
SupplierRestService: Suppliers
-
SiteRestService: Sites
-
ContactRestService: Supplier/Site Contacts
-
ProductRecordRestService: Product Records
-
ProductSpecificationRestService: Product Specifications
-
TaskRestService: Users' Tasks
-
UrgentItemsRestService: Users' Urgent Items
-
ArtworkRestService: Artwork Activities
-
BusinessCategoryService: Business Categories
-
AuditRestService: Audits and Visits
-
DataPrivacyService: Data Privacy Requests
-
AttachmentRestService: Attachment Files
-
ScorecardRestService: Scorecards
-
ProjectRestService: Projects
-
ActivityRestService: Project Activities
Figure 4-1 Overview of RESTful APIs
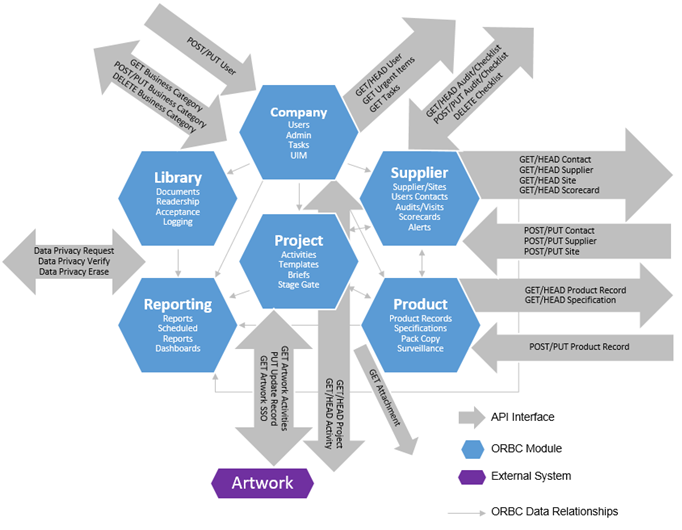
Note:
Only minimal business logic and validation is applied by the Brand Compliance REST APIs.
When creating or updating records, all values must be included. If a value or element is omitted from the XML, the field contents will be cleared when updating the record.
Generally, only the validation necessary to ensure the system's referential integrity is applied when updating records through the APIs. It is therefore possible to set the contents of some fields with values that are rejected when the user manually edits or validates the record through the UI. In some cases, it may be necessary to regress these records back to a prior status in order to edit and correct values that have been set incorrectly through the UI.
Parameters and Filtering
Various parameters can be included in calls to the APIs, generally to define what data is to be returned, but can also control how records are returned. The following table lists some commonly used parameters.
Common Parameters
Parameter | Type | Description |
---|---|---|
offset |
int |
Used with pageSize to control the paging of a returned list of records. Specifies the starting point for the retrieval of records. If not specified, zero is assumed. For example, to retrieve 150 records:
|
pageSize batchSize |
int |
Used with offset to control the paging of a returned list of records. Specifies the number of entries in each page of returned list of records. If not specified, 30 is assumed. The maximum is 100. |
isActive |
Boolean |
Used to only return records that are flagged as being active (TRUE). |
modifiedSince |
string |
Used to locate records that have been created or updated since a specific date/time. |
modifiedUntil |
string |
Used to locate records that have been updated or created until a specific date/time. |
When a list of records is returned, it may include a previousPage and nextPage element. These elements provide URI links to the previous or next pages of records respectively. A totalRecords element is included that shows the total number of records available for retrieval.
For example, using the List of Values function to retrieve a list of User records, where there are 150 records to be retrieved, with the pageSize parameter set to 50 returns the first 50 records within entity elements, along with a nextPage element containing a URI link to the next 50 records which can be used to retrieve the next 50 records. When the second page of 50 records are returned, the XML includes a nextPage URI link to the final 50 records, along with a previousPage URI link to the first 50. The following graphic illustrates this example.
Figure 4-2 Example of Retrieving a List of Users
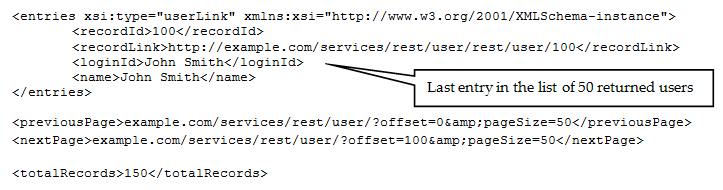
The totalRecords element shows the total number of retrievable records. The previousPage and nextPage elements are only present if there are previous and/or next pages to be retrieved.
Note:
Date/Time parameters must be provided in the YYYY-MM-DD hh:mm:ss format.
When retrieving records, the returned XML only includes elements that actually contain data; empty elements are omitted.
The details of the returned records are contained in an entities element, and repeated in an entryArray element. The entryArray element is a representation from the Java objects and can be ignored.
Where a date/time is passed in as a parameter to an endpoint, such as fromDate and toDate, validation checks for a valid date/time. If not valid, an HTTP 417 is returned, along with an XML error message detailing the exact issue with the date/time.
Error Messages
The Brand Compliance APIs use standard web services messaging protocols to notify the success or failure of a call to the service. Where an API handles a specific error condition, details of the returned message can be found in the following sections. The following table lists various generic error messages that may be returned by calls to any of the APIs.
Generic Error Messages
Element | Message | Meaning |
---|---|---|
userId |
HTTP Status 401 - Bad credentials |
User ID or password is invalid |
Password |
HTTP Status 401 - Bad credentials |
User ID or password is invalid |
Disabled |
HTTP Status 401 - User is disabled |
External System account is not enabled in Brand Compliance |
offset |
HTTP 417 IllegalStateException: Offset must be a positive integer |
Invalid offset value - must be between zero and 2,147,483,647 |
offset |
HTTP 404 Not Found |
Not numeric or an integer |
pageSize |
HTTP 417 IllegalStateException: The Page Size must be between 1 and 100 |
Invalid page size value |
HTTP 404 Not Found |
Record cannot be located due to an invalid key or ID |
|
HTTP 417 Expectation Failed |
Record cannot be located due to an invalid ID |
|
HTTP Status 500 - Internal Server Error |
Unspecified internal error occurred |
|
HTTP Status 500 - Internal Server Error |
Non-numeric value in numeric parameters |
|
modifiedSince |
HTTP 417 <errorMessage> |
Not a valid date, whereby <errorMessage> will identify the issue For example: IllegalInstantException: Cannot parse "2018-03-25 01:00:00": Illegal instant due to time zone offset transition (Europe/London) In this example, due to the clocks moving forward (in the server's time-zone), the passed in date is invalid |
modifiedUntil |
HTTP 417 <errorMessage> |
Not a valid date, whereby <errorMessage> will identify the issue (see example above) |
HTTP 400 Bad Request |
Malformed XML, or general error in content of the XML |
Wildcard Searches
The % character can be used as a wildcard filter when locating the records.
Using the % at the start of the string will search for matches where the given string ends with the text being searched for, for example, %smith could be used to search for all users with a surname of smith.
Using the % at the end of the string will look for matches where the given string begins with the text being searched for, for example, mark% could be used to search for all users with the name mark.
The following table shows which APIs and functions support the use of wildcards.
Wildcard Searches
API | Function |
---|---|
User |
List of Values |
Supplier |
List of Values |
Site |
List of Values |
Contact |
List of Values Retrieve Supplier Contact Record by Business Key Retrieve Site Contact Record by Business Key |
Product Report |
List of Values |
Product Specification |
List of Values Retrieve List with Advanced Filtering |
Business Category |
List of Values |
Task |
List of Values |
Urgent Items |
Number of Urgent Items |
Notes:
-
Wildcard searches are not case sensitive so, for example, searching a name field for John Smith or john smith will return the same matches.
-
When searching glossary parameters, be aware that they relate to the code (not the description) of the glossary entry.
-
Wildcard searches can only be applied to parameters that are string type.
UserRestService
This section describes the API for managing retailer and supplier users. The following functions are available:
-
List of Values: retrieves a list of users
-
Retrieve Record by ID: retrieves a User record using its unique identifier
-
Retrieve Record by Business Key: retrieves a User record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a User record was last updated
-
Create Record: creates a new User record
-
Update Record: updates an existing User record
List of Values
Description
Retrieves a list of users in a paged list. Use this function to retrieve a simple list of user names and IDs, or to locate User record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/user HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
name |
Optional |
string |
No |
John Smith |
jobTitle |
Optional |
string |
No |
Project Manager |
language |
Optional |
string |
No |
en_GB |
country |
Optional |
string |
No |
UK |
userRoles |
Optional |
string |
No |
PROJECT ADMINISTRATOR |
userType |
Optional |
string |
Yes |
RETAILER |
authorityProfiles |
Optional |
string |
No |
PROJECT MANAGER |
siteStatus |
Optional |
string |
Yes |
ACTIVE~INACTIVE |
supplierActive |
Optional |
string |
No |
true (otherwise will return active and inactive suppliers) |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/user/?offset=2&pageSize=20 …/services/rest/user/?siteStatus=Active …/services/rest/user/?name=Frank%
Response Details
For a successful response, XML is returned with a UserLinkList root element containing an entries element for each matched user. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
User record's internal ID |
recordLink |
string |
URI to the UserRestService Retrieve record service for this user |
loginId |
string |
Login ID / business key to the User record |
name |
string |
User's name |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
Retrieve Record by ID
Description
Retrieves a single User record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual user.
Endpoint address: /services/rest/user/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/user/105
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a userFullDTO root element containing the individual attributes of the requested User record. If an ID is not specified, a list of all users is returned (per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the User to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single User record's details using its business key (login ID). Use this function to retrieve the full details of an individual user using its Brand Compliance login ID.
Endpoint address: /services/rest/byKey/{loginId} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {loginId} parameter that determines the record to retrieve.
Example URL
…/services/rest/user/byKey/Frank Jones
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a userFullDTO root element containing the individual attributes of the requested User record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a userFullDTO root element containing the individual attributes of the requested User record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
loginId |
HTTP 404 Not found |
Invalid {loginId} - blank or not found |
loginId |
HTTP 404 Not found |
Invalid {loginId} - not found |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a User record. Use this function to determine when a user's details were last updated.
Endpoint address: /services/rest/user/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/user/105
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested User record. For example:
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
Create Record
Description
Creates a new User record. Use this function to create a new user in Brand Compliance based on data sourced from the external system.
Dependencies: If creating a supplier user, the Supplier must be present in the application and its record ID obtained. For more information, see Dependencies.
Endpoint address: /services/rest/user HTTP method: POST
Request Details
The body of the request contains a UserFullDTO to specify the details of the user to create. Compared to retrieving a user (which uses the same UserFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created User record need to be included. As a minimum, this must include the fields shown in the following table:
Supplier User Mandatory Fields
Field Name | Element Name |
---|---|
Name |
person / name |
|
person / email |
Login Id |
code |
User Role |
role / code |
Supplier Code |
person / supplier / code supplier / code |
User Type |
userType (Fixed value SUPPLIER) |
Deleted Record |
deleted (Set to false) |
Login Id Disabled |
disabled (Set to false) |
Login Permitted |
loginPermitted (Set to true) |
Language |
language / code |
Example Request XML
This example shows the minimum requirement to be able to create a supplier user.
<ns0:userFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:code>jphillips</ns0:code> <ns0:person> <ns0:email>jphillips@supplier.co.uk</ns0:email> <ns0:name>Jane Phillips</ns0:name> <ns0:supplier> <ns1:code>A0174</ns1:code> </ns0:supplier> </ns0:person> <ns0:role> <ns1:code>SUPPLIER ADMINISTRATOR</ns1:code> </ns0:role> <ns0:supplier> <ns1:code>A0174</ns1:code> </ns0:supplier> <ns0:userType>SUPPLIER</ns0:userType> <ns0:deleted>false</ns0:deleted> <ns0:disabled>false</ns0:disabled> <ns0:loginPermitted>true</ns0:loginPermitted> <ns0:restrictedAuditorAccess>false</ns0:restrictedAuditorAccess> </ns0:userFullDTO>
Note:
When creating a supplier user, the user type must be set to SUPPLIER. The supplier code must be set to match the required supplier from Brand Compliance.
Site User Mandatory Fields
Field Name | Element Name |
---|---|
Name |
person / name |
|
person / email |
Login Id |
code |
User Role |
role / code |
Supplier Code |
person / supplier / code supplier / code |
Sites |
sites / code sites/ supplierCode |
User Type |
userType (Fixed value SITE) |
Deleted Record |
deleted (Set to false) |
Login Id Disabled |
disabled (Set to false) |
Login Permitted |
loginPermitted (Set to true) |
Language |
language / code |
Example Request XML
This example shows the minimum requirement to be able to create a site user.
<ns0:userFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:code>jphillips</ns0:code> <ns0:person> <ns0:email>sphillips@supplier.co.uk</ns0:email> <ns0:name>Sean Phillips</ns0:name> <ns0:supplier> <ns1:code>WS0001</ns1:code> <ns1:id>22</ns1:id> </ns0:supplier> </ns0:person> <ns0:role> <ns1:code>SUPPLIER ADMINISTRATOR</ns1:code> </ns0:role> <ns0:sites> <ns1:code>WS0001-0001</ns1:code> <ns1:id>26</ns1:id> <ns1:supplierCode>WS0001</ns1:supplierCode> <ns1:id>22</ns1:id> </ns0:sites> <ns0:supplier> <ns1:code>WS0001</ns1:code> <ns1:id>22</ns1:id> </ns0:supplier> <ns0:userType>SITE</ns0:userType> <ns0:deleted>false</ns0:deleted> <ns0:disabled>false</ns0:disabled> <ns0:loginPermitted>true</ns0:loginPermitted> <ns0:restrictedAuditorAccess>false</ns0:restrictedAuditorAccess> </ns0:userFullDTO>
Note:
When creating a site user the user type must be set to SITE. The supplier code must be set to match the required supplier from Brand Compliance. The site codes must be set to match the required site from Brand Compliance.
Retailer User Mandatory Fields
Field Name | Element Name |
---|---|
Name |
person / name |
|
person / email |
Login Id |
code |
User Role |
role / code |
Supplier Code |
person / supplier / code supplier / code (Fixed value RETAILER) |
User Type |
userType (Fixed value RETAILER) |
Deleted Record |
deleted (Set to false) |
Login Id Disabled |
disabled (Set to false) |
Login Permitted |
loginPermitted (Set to true) |
Language |
language / code |
Example Request XML
This example shows the minimum requirement to be able to create a retailer/portal owner user.
<ns0:userFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:code>james howard</ns0:code> <ns0:person> <ns0:email>james.howard@oracle.com</ns0:email> <ns0:name>James Howard</ns0:name> <ns0:supplier> <ns1:code>RETAILER</ns1:code> </ns0:supplier> </ns0:person> <ns0:role> <ns1:code>POWER USER</ns1:code> </ns0:role> <ns0:supplier> <ns1:code>RETAILER</ns1:code> </ns0:supplier> <ns0:userType>RETAILER</ns0:userType> <ns0:deleted>false</ns0:deleted> <ns0:disabled>false</ns0:disabled> <ns0:loginPermitted>true</ns0:loginPermitted> </ns0:userFullDTO>
Note:
When creating a retailer/portal owner user, the user type must be set to RETAILER. The supplier code must be set to RETAILER.
Enabling User Accounts
Where the record is linked to another record, such as the Role in these cases, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
Set loginPermitted to true to ensure a corresponding IDCS or OCI IAM profile is automatically created for the user. If an IDCS or OCI IAM profile is not required, setting the loginPermitted attribute to false will skip the IDCS or OCI IAM profile creation.
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted, or set to true, it will exist, but will not be visible.
Where the record is linked to another record, such as the Role in these cases, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
When a new record is created in Brand Compliance, a corresponding IDCS or OCI IAM profile is automatically created to manage login authentication. If an IDCS or OCI IAM profile is not required, setting the loginPermitted attribute to false will skip the IDCS or OCI IAM profile creation.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Response Details
For a successful response, an HTTP 200 response is sent with a body containing a UserLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created User record's internal ID |
recordLink |
string |
URI to the newly created User record, for use in a GET request |
loginId |
string |
Login ID / business key to the newly created User record |
name |
string |
User's name |
Error Responses
If the supplied data does not result in a valid User (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Element | Message | Meaning |
---|---|---|
code |
ERROR: class com.micros.creations.core.domain.User.code - The condition is invalid |
Code not provided |
|
ERROR: class com.micros.creations.core.domain.User.person.email - The condition is invalid |
Email not provided |
name |
ERROR: class com.micros.creations.core.domain.User.person.name - The condition is invalid |
Name not provided |
role |
ERROR: class com.micros.creations.core.domain.User.role - A user must have an active authority profile from either roles or additional authority profiles |
Role or authority profiles not provided |
Update Record
Description
Updates an existing User record. Use this function to update a user's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/{id} HTTP method: PUT
Request Details
The body of the request contains a UserFullDTO to specify the updates to the User record. Compared to retrieving a user (which uses the same UserFullDTO type), this request is much shorter. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is similar to that for creating a user. After the call, the User record is updated to match the request.
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the User record.
Any values passed for Login Id or Email Address are ignored, as they cannot be updated once the record has been created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Process for Disabling User Accounts
A user's account can be disabled using the Update Record function as follows:
-
Call the Retrieve Record by Business Key GET function or the Retrieve Record by Id function, passing the login id or the record id respectively, to retrieve the full XML for the user.
-
Amend the returned XML as follows:
Set the account to disabled with <ns0:disabled>true<ns0:disabled> and <ns0:loginPermitted>false<ns0:loginPermitted
Remove the localeData and activeAuthorityProfiles elements.
-
Call the Update Record PUT function, passing the full amended XML to disable the account.
Response Details
If successful, an HTTP 200 response is sent with a body containing a UserLink element. The UserLink element consists of the returned elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
User record's internal ID |
recordLink |
string |
URI to the User record, for use in a GET request |
loginId |
string |
Login ID/business key to the User record |
name |
string |
User's name |
Error Responses
If the supplied data does not result in a valid user (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
SupplierRestService
This section describes the API for managing suppliers. The following functions are available:
-
List of Values: retrieves a list of suppliers
-
Retrieve Record by ID: retrieves a Supplier record using its unique identifier
-
Retrieve Record by Business Key: retrieves a Supplier record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Supplier record was last updated
-
Create Record: creates a new Supplier record
-
Update Record: updates an existing Supplier record
List of Values
Description
Retrieves a list of suppliers in a paged list. Use this function to retrieve a simple list of supplier names and IDs, or to locate Supplier record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/supplier HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
supplierStatus |
Optional |
string |
Yes |
AWAITING REGISTRATION |
supplierCode |
Optional |
string |
Yes |
A0001 |
supplierName |
Optional |
string |
Yes |
West Road Site |
supplierType |
Optional |
string |
Yes |
AGENT |
country |
Optional |
string |
Yes |
UK |
leadBusinessUnit |
Optional |
string |
Yes |
UK |
isActive |
Optional |
string |
No |
true (otherwise will return active and inactive suppliers) |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
invoicingRef |
Optional |
string |
No |
ABC123 |
Example URLs
…/services/rest/supplier/?offset=2&pageSize=20 …/services/rest/supplier/?supplierStatus=AWAITING REGISTRATION …/services/rest/supplier/?supplierName=API%
Response Details
For a successful response, XML is returned with a SupplierLinkList root element containing an entries element for each matched supplier. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Supplier record's internal ID |
recordLink |
string |
URI to the SupplierRestService Retrieve record service for this supplier |
code |
string |
Supplier code business key to the Supplier record |
name |
string |
Supplier's name |
localName |
string |
Supplier's name in business language (if used) |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
Element | Message | Meaning |
---|---|---|
supplierStatus |
HTTP 417 IllegalStateException: The Supplier Status <###> does not exist |
Invalid supplier status |
supplierStatus |
HTTP 417 IllegalStateException: The Supplier Statuses <###~###~###> do not exist |
Invalid supplier status |
supplierType |
HTTP 417 IllegalStateException: The Supplier Type <###> does not exist |
Invalid supplier type |
supplierType |
HTTP 417 IllegalStateException: The Supplier Types <###~###> do not exist |
Invalid supplier type |
country |
HTTP 417 IllegalStateException: The Country <###> does not exist |
Invalid country |
country |
HTTP 417 IllegalStateException: The Countries <###~###> do not exist |
Invalid country |
leadBusinessUnit |
HTTP 417 IllegalStateException: The Business Unit <###> does not exist |
Invalid business unit |
leadBusinessUnit |
HTTP 417 IllegalStateException: The Business Units <###~###> do not exist |
Invalid business unit |
pageSize |
Error Code: INVALIDRESTSERVICEPAGESIZE |
Page Size must be between 1 and 100 |
offset |
Error Code: INVALIDOFFSET |
Offset must be a positive integer |
supplierStatus |
Error Code: INVALIDSUPPLIERSTATUS |
Invalid Supplier Status |
supplierType |
Error Code: INVALIDSUPPLIERTYPE |
Invalid Supplier Type |
country |
Error Code: INVALIDCOUNTRY |
Invalid Country |
leadBusinessUnit |
Error Code: INVALIDBUSINESSUNIT |
Invalid Business Unit |
isActive |
Error Code: INVALIDBOOLEAN |
Invalid isActive value Permitted values (not case-sensitive) are: true; yes; 1; false; no; 0 |
Retrieve Record by ID
Description
Retrieves a single Supplier record's details using the record's internal ID (which is not visible in the UI). Use this function to retrieve the full details of an individual supplier.
Endpoint address: /services/rest/supplier/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/supplier/9
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a supplierFullDTO root element containing the individual attributes of the requested Supplier record. If an ID is not specified, a list of all suppliers is returned (per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Supplier to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Supplier record's details using its business key (supplier code). Use this function to retrieve the full details of an individual supplier using the Brand Compliance supplier code.
Endpoint address: /services/rest/supplier/byKey/{code} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {code} parameter that determines the record to retrieve.
Example URL
…/services/rest/supplier/byKey/A0901
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a supplierFullDTO root element containing the individual attributes of the requested Supplier record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a supplierFullDTO root element containing the individual attributes of the requested Supplier record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
HTTP 404 Not Found |
Invalid {code} - blank |
code |
HTTP 404 Not Found |
Invalid {code} |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Supplier record. Use this function to determine when a supplier's details were last updated.
Endpoint address: /services/rest/supplier/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/supplier/9
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Supplier record.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Responses
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
Create Record
Description
Creates a new Supplier record. Use this function to create new suppliers in Brand Compliance based on data sourced from the external system.
Dependencies: Create sites and contacts after creating the supplier. For more information, see Dependencies.
Endpoint address: /services/rest/supplier HTTP method: POST
Request Details
The body of the request contains a SupplierFullDTO to specify the detail of the supplier to create. Compared to retrieving a supplier (which uses the same SupplierFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created Supplier record need to be included. As a minimum, this must include the fields shown in the following table.
Supplier Mandatory Fields
Field Name | Element Name |
---|---|
Supplier Name |
name |
Contact Name |
supplierContactName |
|
|
Supplier Type |
supplierType/code |
Lead Business Unit |
businessUnit/code |
Billing Code |
billingCode/code |
Status |
Status |
Supplier Code Confirmed Flag |
supplierCodeConfirmed |
Deleted Record |
deleted (Set to false) |
Created On |
createdOn (set to current date using format YYYY-MM-DD or to current date and time using format YYYY-MM-DDThh:mm:ss) |
Is Active |
isActive - see below |
Potential Supplier |
potentialSupplier (Set to false) |
Example Request XML
This example shows the minimum requirement to be able to create a supplier.
<ns0:supplierFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:billingCode> <ns1:code>SMALL</ns1:code> </ns0:billingCode> <ns0:businessUnit> <ns1:code>UK</ns1:code> </ns0:businessUnit> <ns0:email>supplier.contactemail@supplier.co.uk</ns0:email> <ns0:name>Name of Supplier</ns0:name> <ns0:status>AWAITING REGISTRATION</ns0:status> <ns0:supplierContactName>Supplier Contact Name</ns0:supplierContactName> <ns0:supplierCodeConfirmed>false</ns0:supplierCodeConfirmed> <ns0:supplierType> <ns1:code>SUPPLIER_TYPE</ns1:code> </ns0:supplierType> <ns0:deleted>false</ns0:deleted> <ns0:createdOn>2020-09-29T09:00:00</ns0:CreatedOn> <ns0:isActive>false</ns0:isActive> <ns0:potentialSupplier>false</ns0:potentialSupplier> </ns0:supplierFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
The Supplier API only creates the Supplier record, just as the Site API only creates the Site record. The same goes for the User API; it only creates the User record, and similarly the Contact API only creates the Contacts records. Each Supplier record must also have at least one Site record created for it.
Supplier Contacts and their User records must be created using the respective APIs, and linked to the supplier/site with their record IDs. When a new Supplier is created through the API, the process does not automatically create the initial Supplier user.
Use of the isActive and supplierCodeConfirmed Flags
The isActive and supplierCodeConfirmed flags on the Supplier record are used to control the Registration process within the application UI.
isActive is a Boolean TRUE/FALSE value. It is always FALSE when a Supplier is created in the application UI. If set to FALSE in the API, when the supplier user first logs in to the system, the Supplier Registration wizard runs (as it does if the Supplier has been created through the application UI). Successful completion of the Registration wizard sets the isActive flag to TRUE, thus ensuring it only runs once. Therefore, if set to TRUE in the API, when the Supplier user first logs in to the system, the Registration wizard will not run.
supplierCodeConfirmed is a Boolean TRUE/FALSE value. It is always FALSE when a Supplier is created through the application UI. It is a security measure for the supplier user logging in for the first time, where they are prompted to enter their Supplier Code. The application changes the value to TRUE if there is a successful match.
Setting both isActive and supplierCodeConfirmed as FALSE will trigger the Registration wizard process when the supplier user first logs in, prompting for the entry of the Supplier Code.
Setting both isActive and supplierCodeConfirmed as TRUE indicates that the information captured during the Registration wizard process has effectively been provided, and therefore the wizard will not run.
Setting isActive as FALSE and supplierCodeConfirmed as TRUE indicates that part of the information captured during the Registration wizard process is present, but the wizard will run to allow the user to complete the data entry.
If a Supplier is created with a status of AWAITING AUTHORISATION, but with isActive set as TRUE, the Supplier status is changed to REGISTERED, thus bypassing the Registration process.
Note:
When a new supplier is created manually within the application, the system sends an email to the supplier requesting they complete the registration process. When creating a new supplier through the API, the email is not generated.
Where the record is linked to another record, such as the Supplier Type in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a SupplierLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Supplier record's internal ID |
recordLink |
string |
URI to the newly created Supplier record, for use in a GET request |
code |
string |
Supplier code business key to the newly created Supplier record |
name |
string |
Supplier's name |
localName |
string |
Supplier's name in business language (if used) |
Error Messages
If the supplied data does not result in a valid Supplier (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Element | Message | Meaning |
---|---|---|
billingCode |
ERROR: class <<hostname>>.Supplier.billingCode - The condition is invalid |
No tag provided |
billingCode |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.BillingCode using code:null |
No <ns1:code> tag |
billingCode |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>..BillingCode using code:<###> |
Invalid <ns1:code> |
businessUnit |
ERROR: class <<hostname>>..Supplier.businessUnit - The condition is invalid |
No tag provided |
businessUnit |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>..domain.BusinessUnit using code:null |
No <ns1:code> tag |
businessUnit |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>..BusinessUnit using code:<###> |
Invalid <ns1:code> |
code |
ERROR: class com.micros.creations.core.domain.Supplier.code - ??Another object exists with this value?? |
Supplier code already exists |
|
ERROR: class com.micros.creations.core.domain.Supplier.email - The condition is invalid |
No tag provided |
|
ERROR: class <<hostname>>.Supplier.email - The attribute is invalid |
Malformed email address |
supplierContactName |
ERROR: class <<hostname>>.Supplier.supplierContactName - The condition is invalid |
No tag provided |
supplierContactName |
ERROR: class <<hostname>>.Supplier.supplierContactName - The condition is invalid |
No value provided |
name (supplier name) |
ERROR: class <<hostname>>.Supplier.name - The condition is invalid |
No tag provided |
name (supplier name) |
ERROR: class <<hostname>>.Supplier.name - The condition is invalid |
No value provided |
supplierType |
ERROR: class <<hostname>>.Supplier.supplierType - The condition is invalid |
No tag provided |
supplierType |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.SupplierType using code:null |
No <ns1:code> tag |
supplierType |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.SupplierType using code:<###> |
Invalid <ns1:code> |
Update Record
Description
Updates an existing Supplier record. Use this function to update a supplier's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/supplier/{id} HTTP method: PUT
Request Details
The body of the request contains a SupplierUpdateDTO to specify the updates to the Supplier record. Compared to retrieving a supplier (which uses the SupplierFullDTO type), this request is much shorter. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is similar to that for creating a supplier, but crucially, the links to other top-level records (Sites, SiteContact, SupplierContact) are omitted. The omission of those ensures that when updating a supplier, only the supplier details need to be specified, and not the details for the related records that may not require updating (and which should be updated to calls to their respective services). After the call, the Supplier record is updated to match the request.
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the Supplier record (except for the Sites, SiteContacts, and SupplierContacts).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a SupplierLink element. The SupplierLink element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Supplier record's internal ID |
recordLink |
string |
URI to the Supplier record, for use in a GET request |
code |
string |
Supplier code business key to the Supplier record |
name |
string |
Supplier's name |
localName |
string |
Supplier's name in business language (if used) |
Error Messages
If the supplied data does not result in a valid Supplier (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Element | Message | Meaning |
---|---|---|
billingCode |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.BillingCode using code:<###> |
Invalid <ns1:code> |
businessUnit |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>domain.BusinessUnit using code:<###> |
Invalid <ns1:code> |
code |
MySQLIntegrityConstraintViolationException: Duplicate entry <###> for key 'c_code' |
Supplier code provided that already exists for another supplier |
name |
PropertyValueException: not-null property references a null or transient value: <<hostname>>.domain.Supplier.name |
No tag provided |
status |
NullPointerException: |
No status tag provided |
status |
IllegalArgumentException: Empty string is not allowed, should be null on method: <<hostname>>.domain.Supplier@1df35f3[id=<###>,code=<###>] |
No status code provided |
SiteRestService
This section describes the API for managing sites. The following functions are available:
-
List of Values: retrieves a list of sites
-
Retrieve Record by ID: retrieves a Site record using its unique identifier
-
Retrieve Record by Business Key: retrieves a Site record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Site record was last updated
-
Create Record: creates a new Site record
-
Update Record: updates an existing Site record
List of Values
Description
Retrieves a list of sites in a paged list. Use this function to retrieve a simple list of supplier names and IDs, or to locate Site record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/site HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example | Validation |
---|---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
|
pageSize |
Optional |
int |
No |
30 |
|
siteStatus |
Optional |
string |
Yes |
AWAITING REGISTRATION |
|
siteCode |
Optional |
string |
Yes |
A0001-0001 |
|
siteName |
Optional |
string |
Yes |
West Road Site |
|
siteType |
Optional |
string |
Yes |
CANNERY |
|
country |
Optional |
string |
Yes |
UK |
|
businessCategory |
Optional |
string |
Yes |
CHEESE_DAIRY |
Code in the Business Categories glossary at any level of the hierarchy. Applies the filter to Business Category and Lead Business Category (if used). Returns a result if any of the values match any of the specification's business categories. |
businessUnit |
Optional |
string |
Yes |
UK |
|
supplierName |
Optional |
string |
Yes |
ABC Ltd |
|
supplierActive |
Optional |
String |
No |
true (otherwise will return active and inactive suppliers) |
|
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
|
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
|
statusChangedFrom |
Optional |
string |
No |
2015-06-10 09:00:00 |
Both From and Until are required if either is used. Until date must be greater than or equal to the From date. |
statusChangedUntil |
Optional |
string |
No |
2015-06-10 09:00:00 |
|
statusChangedTo |
Optional |
string |
Yes |
ACTIVE |
|
leadTechnologist |
Optional |
string |
Yes |
John Smith |
The user's Login Id. |
Example URLs
…/services/rest/site/?offset=2&pageSize=20 …/services/rest/site/?siteStatus=ACTIVE …/services/rest/site/?supplierName=API%
Response Details
For a successful response, XML is returned with a SiteLinkList root element containing an entries element for each matched site. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Site record's internal ID |
recordLink |
string |
URI to the SiteRestService Retrieve record for this site |
code |
string |
Site code business key to the Site record |
name |
string |
Site's name |
localName |
string |
Site's name in business language (if used) |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
Element | Message | Meaning |
---|---|---|
siteStatus |
HTTP 417 IllegalStateException: The Supplier Status <###> does not exist |
Invalid site status |
siteStatus |
HTTP 417 IllegalStateException: The Supplier Statuses <###~###~###> do not exist |
Invalid site status |
siteType |
HTTP 417 IllegalStateException: The Supplier Type <###> does not exist |
Invalid supplier type |
siteType |
HTTP 417 IllegalStateException: The Supplier Types <###~###> do not exist |
Invalid supplier type |
country |
HTTP 417 IllegalStateException: The Country <###> does not exist |
Invalid country |
country |
HTTP 417 IllegalStateException: The Countries <###~###> do not exist |
Invalid country |
businessCategory |
HTTP 417 IllegalStateException: The Business Category <###> does not exist |
Invalid business category |
businessCategory |
HTTP 417 IllegalStateException: The Business Categories <###~###> do not exist |
Invalid business category |
businessUnit |
HTTP 417 IllegalStateException: The Business Unit <###> does not exist |
Invalid business unit |
businessUnit |
HTTP 417 IllegalStateException: The Business Units <###~###> do not exist |
Invalid business unit |
modifiedSince |
Invalid dates cause stack trace to come back to "response" |
|
modifiedUntil |
Invalid dates cause stack trace to come back to "response" |
|
pageSize |
Error Code: INVALIDRESTSERVICEPAGESIZE INVALIDPAGESIZE |
Page Size must be between 1 and 100 |
offset |
Error Code: INVALIDOFFSET |
Offset must be a positive integer |
siteStatus |
Error Code: INVALIDSITESTATUS INVALIDSITESTATUSES |
Invalid Site Status |
siteType |
Error Code: INVALIDSITETYPE |
Invalid Site Type |
country |
Error Code: INVALIDCOUNTRY |
Invalid Country |
businessCategory |
Error Code: INVALIDBUSINESSCATEGORY |
Invalid Business Category |
businessUnit |
Error Code: INVALIDBUSINESSUNIT |
Invalid Business Unit |
supplierActive |
Error Code: INVALIDBOOLEAN |
Invalid supplierActive value Permitted values (not case-sensitive) are: true; yes; 1; false; no; 0 |
leadTechnologist |
Error Code: INVALIDUSER |
Invalid Lead Technologist |
statusChangedFrom |
Error Code: INVALIDDATEFORMAT |
Invalid Status Changed From Must be a valid date/time format: Year-Month-DayOfMonth Hour:Minute:Seconds such as 2016-12-30 09:59:59 |
statusChangedUntil |
Error Code: INVALIDDATEFORMAT |
Invalid Status Changed Until Must be a valid date/time format: Year-Month-DayOfMonth Hour:Minute:Seconds such as 2016-12-30 09:59:59 |
statusChangedTo |
Error Code: INVALIDSTATUSCHANGEDTO |
Either Status Changed From or Status Changed Until must be specified if filtering on Status Changed To |
Retrieve Record by ID
Description
Retrieves a Site record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual site.
Endpoint address: /services/rest/site/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/site/87
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a siteFullDTO root element containing the individual attributes of the requested Site record. If an ID is not specified, a list of all sites is returned (per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Site to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Site record's details using its business key (supplier and codes). Use this function to retrieve the full details of an individual site using the combination of the Brand Compliance supplier and site codes.
Endpoint address: /services/rest/site/byKey/{supplierCode}/{siteCode} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {supplierCode} and {siteCode} parameters that determine the record to retrieve.
Example URL
…/services/rest/site/byKey/A0001/A0001-0001
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a siteFullDTO root element containing the individual attributes of the requested Site record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a siteFullDTO root element containing the individual attributes of the requested Site record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
supplierCode |
HTTP 404 Not Found |
Invalid {supplierCode} - blank |
siteCode |
HTTP 404 Not Found |
Invalid {siteCode} |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Site record. Use this function to determine when a site's details were last updated.
Endpoint address: /services/rest/site/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/site/87
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Site record.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
Create Record
Description
Creates a new Site record. Use this function to create new sites in Brand Compliance based on data sourced from the external system.
Dependencies: The Supplier must be present in the application and its record ID obtained. If assigning a Business Category or Product Technologist, the record must be present in the application and its record ID obtained. Create Contacts after creating the Site. For more information, see Dependencies.
Endpoint address: /services/rest/site HTTP method: POST
Request Details
The body of the request contains a SiteFullDTO element to specifying the details of the site to create. Compared to retrieving a site (which uses the same SiteFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created Site record need to be included. As a minimum, this must include the fields shown in the following table.
Site Mandatory Fields
Field Name | Element Name |
---|---|
Site Name |
name |
Site Type |
siteType/code |
Categories |
businessCategories |
Lead Product Technologist |
leadTechnicalManager |
Supplier |
supplier/code |
Site Status |
siteStatus |
Deleted Record |
deleted (Set to false) |
Example Request XML
This example shows the minimum requirement to be able to create a site against a supplier.
<ns1:siteFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:businessCategories> <ns1:code>CATEGORY2A</ns1:code> </ns0:businessCategories> <ns0:leadTechnicalManager> <ns1:code>techadmin</ns1:code> </ns0:leadTechnicalManager> <ns0:name>Site Name</ns0:name> <ns0:siteType> <ns1:code>SITE_TYPE_EXAMPLE</ns1:code> </ns0:siteType> <ns0:siteStatus> <ns1:status>ACTIVE</ns1:status> </ns0:siteStatus> <ns0:supplier> <ns1:code>WS0001</ns1:code> </ns0:supplier> <ns0:deleted>false</ns0:deleted> </ns1:siteFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
Where the record is linked to another record, such as the Site Type in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a SiteLink root element containing the site data, and a SupplierLink element, consisting of the parent supplier data. The returned elements are shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Site record's internal ID |
recordLink |
string |
URI to the newly created Site record, for use in a GET request |
code |
string |
Supplier and site code business key to the newly created Site record |
name |
string |
Site's name |
localName |
string |
Site's name in business language (if used) |
supplierLink/recordId |
long |
Newly created site's Supplier record internal ID |
supplierLink/recordLink |
string |
URI to the newly created site's Supplier record, for use in a GET request |
recordLink/code |
string |
Supplier code business key to the newly created site's Supplier record |
recordLink/name |
string |
Supplier's name |
recordLink/localName |
string |
Supplier's name in business language (if used) |
Error Messages
If the supplied data does not result in a valid Site (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Element | Message | Meaning |
---|---|---|
supplier/id |
Null Pointer Exception |
Supplier ID not provided |
supplier/id |
No row with the given identifier exists: [com.micros.creations.core.domain.Supplier#<###>] |
Supplier not found |
siteStatus |
Null Pointer Exception |
Site Status not provided |
siteStatus |
Cannot locate keyword for class com.micros.creations.core.domain.SiteStatus using status:<###> |
Site Status not found |
siteType |
ERROR: class com.micros.creations.core.domain.Site.siteType - The condition is invalid |
Site Type not provided |
siteType |
Cannot locate keyword for class com.micros.creations.core.domain.SiteType using code:<###> |
Site Type not found |
name |
class com.micros.creations.core.domain.Site.name - The condition is invalid |
Site name not provided |
leadTechnicalManager |
ERROR: class com.micros.creations.core.domain.Site.leadTechnicalManager - The condition is invalid |
Lead Technical Manager not provided |
leadTechnicalManager |
HTTP 417 IllegalStateException: Cannot locate keyword for class com.micros.creations.core.domain.User using code:<###> |
Lead Technical Manager not found |
businessCategories |
ERROR: class com.micros.creations.core.domain.Site.businessCategories - The condition is invalid |
Business Category not provided |
businessCategories |
Cannot locate keyword for class com.micros.creations.core.domain.BusinessCategory using code:<###> |
Business Category not found |
code |
Duplicate site code |
The site already exists for this supplier |
Update Record
Description
Updates an existing Site record. Use this function to update a site's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/site/{id} HTTP method: PUT
Request Details
The body of the request contains a SiteUpdateDTO to specify the updates to the Site record. Compared to retrieving a site (which uses the SiteFullDTO type), this request is much shorter. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is similar to that for creating a site, but crucially, the links to other top-level records (SiteContact) are omitted. The omission of those ensures that when updating a site, only the site details need to be specified, and not the details for the related records that may not require updating (and which should be updated with calls to their respective services). After the call, the Site record is updated to match the request
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the Site record (except for the SiteContacts).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a SiteLink root element containing the site data, and a SupplierLink element, consisting of the parent supplier data. The returned elements are shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Updated Site record's internal ID |
recordLink |
string |
URI to the updated Site record, for use in a GET request |
code |
string |
Site code business key to the updated Site record |
name |
string |
Site's name |
localName |
string |
Site's name in business language (if used) |
supplierLink/recordId |
long |
Updated site's Supplier record internal ID |
supplierLink/recordLink |
string |
URI to the updated site's Supplier record, for use in a GET request |
recordLink/code |
string |
Supplier code business key to the updated site's Supplier record |
recordLink/name |
string |
Supplier's name |
recordLink/localName |
string |
Supplier's name in business language (if used) |
Error Responses
If the supplied data does not result in a valid Site (such as a missing mandatory field), an HTTP 417 response is sent an with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
ContactRestService
This section describes the API for managing supplier and site contacts. The following functions are available:
-
List of Values: retrieves a list of contacts
-
Retrieve Record by ID: retrieves a Contact record using its unique identifier
-
Retrieve Supplier Contact Record by Business Key: retrieves a Supplier Contact record using its business key
-
Retrieve Site Contact Record by Business Key: retrieves a Site Contact record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Contact record was last updated
-
Create Record: creates a new Contact record
-
Update Record: updates an existing Contact record
List of Values
Description
Retrieves a list of contacts in a paged list. Use this function to retrieve a simple list of contact names and IDs, or to locate Contact record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/contact HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
name |
Optional |
string |
Yes |
John Smith |
supplierContactRoles |
Optional |
string |
Yes |
HEAD OF MANUFACTURING |
siteContactRoles |
Optional |
string |
Yes |
HEAD OF MANUFACTURING |
country |
Optional |
string |
Yes |
UK |
selectedSites |
Optional |
string |
No |
A0001-0001 |
supplierCode |
Optional |
string |
Yes |
A0001 |
supplierName |
Optional |
string |
Yes |
ABC Ltd. |
siteStatus |
Optional |
string |
Yes |
ACTIVE~INACTIVE |
supplierActive |
Optional |
string |
No |
true (otherwise will return active and inactive suppliers) |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/contact/?offset=2&pageSize=20 …/services/rest/contact/?siteStatus=ACTIVE …/services/rest/contact/?supplierName=API%
Response Details
For a successful response, XML is returned with a ContactLinkList root element containing an entries element for each matched contact. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Contact record's internal ID |
recordLink |
string |
URI to the ContactRestService Retrieve record service for this contact |
|
string |
Contact's email address |
name |
string |
Contact's name |
siteContact |
Boolean |
Indicates if a contact for the site (true), else false |
supplierContact |
Boolean |
Indicates if a contact for the supplier (true), else false |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
Element | Message | Meaning |
---|---|---|
supplierContactRoles |
HTTP 417 IllegalStateException: The Supplier Contact Role <###> does not exist |
Invalid supplier contact role |
supplierContactRoles |
HTTP 417 IllegalStateException: The Supplier Contact Roles <###~###> do not exist |
Invalid supplier contact role |
siteContactRoles |
HTTP 417 IllegalStateException: The Site Contact Role <###> does not exist |
Invalid site contact role |
siteContactRoles |
HTTP 417 IllegalStateException: The Site Contact Roles <###~###> do not exist |
Invalid site contact role |
country |
HTTP 417 IllegalStateException: The Country <###> does not exist |
Invalid country code |
country |
HTTP 417 IllegalStateException: The Countries <###~###> do not exist |
Invalid country code |
siteStatus |
HTTP 417 IllegalStateException: The Supplier Status <###> does not exist |
Invalid supplier status code |
pageSize |
Error Code: INVALIDRESTSERVICEPAGESIZE INVALIDPAGESIZE |
Page Size must be between 1 and 100 |
offset |
Error Code: INVALIDOFFSET |
Offset must be a positive integer |
supplierContactRole |
Error Code: INVALIDCONTACTROLE |
Invalid Supplier Contact Role |
siteContactRole |
Error Code: INVALIDCONTACTROLE |
Invalid Site Contact Role |
country |
Error Code: INVALIDCOUNTRY |
Invalid Country |
selectedSite |
Error Code: INVALIDSITE |
Invalid Site |
supplierCode |
Error Code: INVALIDSUPPLIER |
Invalid Supplier |
siteStatus |
Error Code: INVALIDSITESTATUS |
Invalid Site Status |
supplierActive |
Error Code: INVALIDBOOLEAN |
Invalid supplierActive value Permitted values (not case-sensitive) are: true; yes; 1; false; no; 0 |
Retrieve Record by ID
Description
Retrieves a single Contact record's details using the record's unique ID. Use this function to retrieve the full details of an individual supplier or site contact.
Endpoint address: /services/rest/contact/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/contact/405
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a contactAndPersonDTO root element containing the individual attributes of the requested Contact record and the person it associates to.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Contact to return with id:<###> |
Invalid {id} - not found |
Retrieve Supplier Contact Record by Business Key
Description
Retrieves a single Supplier Contact record's details using its business key (supplier code, contact name, and email address). Use this function to retrieve the full details of an individual supplier contact using the combination of the Brand Compliance supplier codes, name of the contact, and the contact's email address.
Endpoint address: /services/rest/contact/byKey/supplier/{supplierCode}/{name}/{email} HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
supplierCode |
Mandatory |
string |
No |
A0001 |
name |
Mandatory |
string |
No |
John Doe |
|
Mandatory |
string |
No |
john.doe@email.com |
Example URL
…/services/rest/contact/byKey/supplier/A0001/John Doe/john.doe@email.com
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a contactAndPersonDTO root element containing the individual attributes of the requested Contact record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a contactAndPersonDTO root element containing the individual attributes of the requested Contact record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Responses
In the document cannot be located, an HTTP 404 response is sent.
Retrieve Site Contact Record by Business Key
Description
Retrieves a single Site Contact record's details using its business key (supplier code, site code, contact name, and contact email). Use this function to retrieve the full details of an individual site contact using the combination of the Brand Compliance supplier and site codes, name of the contact, and the contact's email address.
Endpoint address: /services/rest/contact/byKey/site/{supplierCode}/{siteCode}/{name}/{email} HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
supplierCode |
Mandatory |
string |
No |
A0001 |
siteCode |
Mandatory |
string |
No |
A0001-0001 |
name |
Mandatory |
string |
No |
John Doe |
|
Mandatory |
string |
No |
john.doe@email.com |
Example URL
…/services/rest/contact/byKey/site/A0001/A0001-0001/ John Doe/john.doe@email.com
Response Details
For a successful response, XML is returned with a contactAndPersonDTO root element containing the individual attributes of the requested Contact record and the person it associates to.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Responses
In the document cannot be located, an HTTP 404 response is sent.
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Contact record. Use this function to determine when a supplier or site contact's details were last updated.
Endpoint address: /services/rest/contact/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/contact/405
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header to show the last modification date and time of the last update of the requested Contact record.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
Create Record
Description
Creates a new Contact record (and the associated Person record). Use this function to create new supplier or site contacts in Brand Compliance based on data sourced from the external system.
Dependencies: The Supplier (and Site, if a site contact) must be present in the application and its record ID obtained. Omit the Site element if not a site contact. The User/Person must be present in the application and its record ID obtained. For more information, see Dependencies.
Endpoint address: /services/rest/contact HTTP method: POST
Request Details
The body of the request contains a ContactAndPersonDTO to specify the details of the contact to create. Compared to the retrieving a contact (which uses the same ContactAndPersonDTO type), this request is much shorter. Only the attributes that are to be populated on the created Contact record need to be included. As a minimum, this must include the fields shown in the following table.
Contact Mandatory Fields
Field Name | Element Name |
---|---|
Name |
contactFullDTO/person/name personFullDTO/name |
User Id |
contactFullDTO/person/id |
Supplier |
contactFullDTO/person/supplier/id contactFullDTO/company/code personFullDTO/supplier/code personFullDTO/supplier/id |
|
contactFullDTO/person/email personFullDTO/email |
Phone |
contactFullDTO/contactDetails/phoneNumber |
Supplier Contact Roles |
contactFullDTO/supplierContactRole/code |
Contact Type |
contactFullDTO/dtype |
Site Contact Flag |
contactFullDTO/siteContact |
Supplier Contact Flag |
contactFullDTO/supplierContact |
Deleted Record |
deleted (Set to false) |
Example Request XML
This example shows the minimum requirement to be able to create a contact against a supplier.
<ns0:ContactAndPersonDTO xmlns:ns4="http://www.oracle.com/orbcmcs/service/rest/model" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full" xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple"> <ns0:contactFullDTO> <ns0:contactDetails> <ns0:phoneNumber>01234 56789</ns0:phoneNumber> </ns0:contactDetails> <ns0:dtype>SupplierContact</ns0:dtype> <ns0:person> <ns1:email>jphillips@example.com</ns1:email> <ns1:name>Jane Phillips</ns1:name> <ns1:supplier> <ns1:code>A0001</ns1:code> </ns1:supplier> </ns0:person> <ns0:siteContact>false</ns0:siteContact> <ns0:supplierContact>true</ns0:supplierContact> <ns0:supplierContactRole> <ns1:code>ACCOUNT MANAGER</ns1:code> </ns0:supplierContactRole> <ns0:company> <ns1:code>A0001</ns1:code> </ns0:company> </ns0:contactFullDTO> <ns0:personFullDTO> <ns0:email>jphillips@example.com</ns0:email> <ns0:name>Jane Phillips</ns0:name> <ns0:supplier> <ns1:code>A0001</ns1:code> </ns0:supplier> </ns0:personFullDTO> <ns0:deleted>false</ns0:deleted> </ns0:ContactAndPersonDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
If a contact has multiple supplier or site contact roles, they should be separate entries as follows:
<ns0:supplierContactRole> <ns1:code>ACCOUNT MANAGER</ns1:code> </ns0:supplierContactRole> <ns0:supplierContactRole> <ns1:code>AUDITS AND VISITS CONTACT</ns1:code> </ns0:supplierContactRole> <ns0:supplierContactRole> <ns1:code>EMERGENCY CONTACT</ns1:code> </ns0:supplierContactRole>
Where the record is linked to another record, such as the Contact Role in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a ContactLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Contact record's internal ID |
recordLink |
string |
URI to the newly created Contact record, for use in a GET request |
|
string |
Email address of the newly created contact |
name |
string |
Contact's name |
siteContact |
Boolean |
Indicates if a contact for the site (true), else false |
supplierContact |
Boolean |
Indicates if a contact for the supplier (true), else false |
Error Messages
Element | Message | Meaning |
---|---|---|
phoneNumber |
phoneNumber - The condition is invalid |
Missing tag |
dtype |
IllegalArgumentException: Invalid value for dtype:<###> |
Invalid value SupplierContact; SiteContact |
name & email |
HTTP 417 IllegalStateException: Cannot locate Person record with name: <###> and email: <###> |
A valid user must be found with matching name and email address |
company |
HTTP 417 IllegalStateException: Cannot locate keyword for class com.micros.creations.core.domain.Company using code:<###> |
Invalid company/supplier code |
Update Record
Description
Updates an existing Contact record. Use this function to update a supplier or site contact's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/contact/{id} HTTP method: PUT
Request Details
The body of the request contains a ContactAndPersonDTO element to specify the updates to the Contact record. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is the same as that for creating a contact. After the call, the Contact record is updated to match the request.
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the Contact record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a ContactLink element. The ContactLink element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Contact record's internal ID |
recordLink |
string |
URI to the Contact record, for use in a GET request |
|
string |
Contact's email address |
name |
string |
Contact's name |
siteContact |
Boolean |
Indicates if a contact for the site (true), else false |
supplierContact |
Boolean |
Indicates if a contact for the supplier (true), else false |
Error Messages
If the supplied data does not result in a valid Contact (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
ProductRecordRestService
This section describes the API for managing Product records. The following functions are available:
-
List of Values: retrieves a list of Product records
-
Retrieve Record by ID: retrieves a Product record using its unique identifier
-
Retrieve Record by Business Key: retrieves a Product record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Product record was last updated
-
Create Record: creates a new Product record
-
Update Record: updates an existing Product record
List of Values
Description
Retrieves a list of Product records in a paged list. Use this function to retrieve a simple list of product titles and IDs, or to locate Product record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/productRecord HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
specType |
Optional |
string |
Yes |
FOOD~CNF |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
productRecordCode |
Optional |
integer |
No |
123456 |
altProductNumber |
Optional |
string |
No |
ABC001 |
division |
Optional |
string |
Yes |
DIV_A~DIV_B Must be the code of an entry in the Divisions glossary |
Example URLs
…/services/rest/productRecord/?offset=2&pageSize=20 …/services/rest/productRecord/?specType=FOOD
Response Details
For a successful response, XML is returned with a ProductRecordLinkList root element containing an entries element for each matched Product Record. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Product record's internal ID |
recordLink |
string |
URI to the ProductRecordRestService Retrieve record service for this Product record |
code |
string |
Code business key to the Product record |
title |
string |
Product's title |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
Element | Message | Meaning |
---|---|---|
specType |
HTTP 417 IllegalStateException: The Spec Type <###> does not exist |
Invalid specification type |
modifiedSince |
Invalid dates cause stack trace to come back to "response" |
|
modifiedUntil |
Invalid dates cause stack trace to come back to "response" |
|
pageSize |
Error Code: INVALIDPAGESIZE |
Page Size must be between 1 and 100 |
offset |
Error Code: INVALIDOFFSET |
Offset must be a positive integer |
specificationVersion |
Error Code: INVALIDSPECNOMANDATORYVERSION |
Specification Number is mandatory if filtering on Version |
specificationType |
Error Code: INVALIDSPECIFICATIONTYPE |
Invalid Specification Type |
Retrieve Record by ID
Description
Retrieves a single Product record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual Product Record.
Endpoint address: /services/rest/productRecord/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/productRecord/99
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a productRecordFullDTO root element containing the individual attributes of the requested Product record. If an ID is not specified, a list of all Product records is returned (per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 3 - Product for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Product Record to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Product record's details using its business key (code). Use this function to retrieve the full details of an individual Product record using its Brand Compliance product code.
The Product record's business key is the product code that is visible in the UI. This an individual number assigned by Brand Compliance. It is not the product's traded unit identifier such as a SKU, that is, the product number, which is also visible in the UI.
Endpoint address: /services/rest/productRecord/byKey/{code} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {code} parameter that determines the record to retrieve.
Example URL
…/services/rest/productRecord/byKey/31
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a productRecordFullDTO root element containing the individual attributes of the requested Product record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a productRecordFullDTO root element containing the individual attributes of the requested Product record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 3 - Product for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
HTTP 404 Not Found |
Invalid {code} - blank |
code |
HTTP 404 Not Found |
Invalid {code} - not found |
code |
NumberFormatException: For input string: <###> |
Invalid {code} - not numeric |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Product record. Use this function to determine when a Product record's details were last updated.
Endpoint address: /services/rest/productRecord/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/productRecord/99
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header to showing the date and time of the last update of the requested Product record.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
Create Record
Description
Creates a new Product record. Use this function to create new Product records in Brand Compliance based on data sourced from the external system.
Dependencies: If linked to a supplier or site, the Supplier/Site must be present in the application and its record ID obtained. If assigning a Business Category, Product Technologist, or Other Contact, the record must be present in the application and its record ID obtained. Product Technologist and Other Contacts may be omitted to default to TBC if the TBC user is present in the application. The status must be Active in order to be linked to a specification; for the status to be Active, the Product record must be linked to a supplier. For more information, see Dependencies.
Endpoint address: /services/rest/productRecord HTTP method: POST
Request Details
The body of the request contains a ProductRecordFullDTO to specify the Product record to create. Compared to retrieving a Product record (which uses the same ProductRecordFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created Product record need to be included. As a minimum, this must include the fields shown in the following table.
Product Record Mandatory Fields
Field Name | Element Name |
---|---|
Product Title |
title |
Status |
productRecordStatus |
Variant Name |
variantName |
Quantity |
quantity |
Specification Type |
specType - see below |
Specification Type Format |
specTypeFormat - see below |
Technologist |
technologist |
Other Contacts |
productRecordOtherUser |
Supplier Details |
supplier |
Site Details |
site |
Deleted Record |
deleted (Set to false) |
Language |
labelI18NKey |
Note:
To ensure a valid unique business key code is automatically assigned to the record being created, a value of zero must be passed in the <code> element of the XML when submitting the POST request.
Example Request XML
This example shows the minimum requirement to be able to create a Product record.
<ns0:productRecordFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:code>1290</ns0:code> <ns0:productCovered> <ns0:quantity>Small</ns0:quantity> <ns0:retailerProductNumber>ABC123</ns0:retailerProductNumber> <ns0:variantName>Small White Rolls</ns0:variantName> </ns0:productCovered> <ns0:productRecordOtherUser> <ns0:role> <ns1:code>BUYER</ns1:code> </ns0:role> <ns0:user> <ns1:code>TBC</ns1:code> </ns0:user> <ns0:labelI18NKey>contacts.buyer</ns0:labelI18NKey> </ns0:productRecordOtherUser> <ns0:productRecordOtherUser> <ns0:role> <ns1:code>PRODUCT DEVELOPMENT MANAGER</ns1:code> </ns0:role> <ns0:user> <ns1:code>TBC</ns1:code> </ns0:user> <ns0:labelI18NKey>contacts.productDevelopmentManager</ns0:ns0:labelI18NKey> </ns0:productRecordOtherUser> <ns0:specTypeFormat> <ns1:code>FOODUK</ns1:code> <ns1:specType>FOOD</ns1:specType> </ns0:specTypeFormat> <ns0:technologist> <ns1:code>PRODUCT TECHNOLOGIST</ns1:code> </ns0:technologist> <ns0:title>White Bread Rolls</ns0:title> <ns0:productRecordStatus> <ns1:status>DRAFT</ns1:status> </ns0:productRecordStatus> <ns0:deleted>false</ns0:deleted> </ns0:productRecordFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
The productCoverage element holds the product's title, quantity, and code regardless of whether the portal is operating in multi variant mode where a single Product record may represent multiple variants (such as sizes) of the product, or in single variant mode, where the Product record represents just a single variant of the product. With single variant mode, there will only ever be a single productCoverage element.
Single Variant Mode
<productCovered> <productTitle/> <variantName/> <quantity/> <retailerProductNumber/> </productCovered>
Multi Variant Mode
<productCovered> <productTitle/> <variantName/> <quantity/> <retailerProductNumber/> </productCovered> <productCovered> <productTitle/> <variantName/> <quantity/> <retailerProductNumber/> </productCovered>
If a specification type has more than one format, such as a Food specification having separate formats for Food - UK and Food - US, the specTypeFormat/code element is used to specify the specification format and specType is used to identify the specification type, for example:
<ns0:specTypeFormat> <ns1:code>FOODUK</ns1:code> <ns1:specType>FOOD</ns1:specType> </ns0:specTypeFormat>
The code of the specification type/format must be used rather than the description.
Where the record is linked to another record, such as the Technologist in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
Note:
The API does not apply status-based validation as is applied using the Brand Compliance UI when manually progressing a Product record.
It is therefore possible, using the API, to create a Product record at Active status without the supplier or site being populated. In this case, it will not be possible to link Product Specifications to the Product record until a valid supplier and site has been assigned manually.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 3 - Product for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a ProductRecordLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Product record's internal ID |
recordLink |
string |
URI to the newly created Product record, for use in a GET request |
code |
string |
Code business key to the newly created Product record |
title |
string |
Product's title |
Error Messages
If the supplied data does not result in a valid Product record (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Element | Message | Meaning |
---|---|---|
productCoverage |
ERROR: class <<hostname>>.ProductRecord.productCovered - The condition is invalid |
No tag provided |
code |
ERROR: class <<hostname>>.ProductRecord.code - A Product Record's code is mandatory |
No tag provided |
code |
ERROR: class <<hostname>>.ProductRecord.code - A Product Record's code is mandatory |
No value provided |
code |
ERROR: class <<hostname>>.ProductRecord.code - A Product Record's code must be unique:<###> |
Code already exists |
code |
HTTP Status 400 - Bad request |
Code is not numeric |
title |
DtoValidationException: title is mandatory |
No tag provided No value provided |
productRecordStatus |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.PoductRecordStstus using status:<###> |
No tag provided No value provided Status not found |
variantName |
ERROR: class <<hostname>>.ProductRecord.productCovered[###].variantName - Variant name is mandatory |
No tag provided No value provided |
quantity |
ERROR: class <<hostname>>.ProductRecord.productCovered[###].quantity - Quantity name is mandatory |
No tag provided No value provided |
specType |
ObjectNotFoundException: No row with the given identifier exists:[<<hostname>>.SpecTypeFormat#<###>] |
No value provided Specification type not found |
technologist |
MySQLIntegrityConstraintViolationException: Cannot add or update a child row: a foreign key constraint fails ('<###>'.'t_prdrcd',CONSTRAINT '<###>' FOREIGN KEY ('fk_technologist') REFERENCES 't_user' ('c_id')) |
No tag provided No value provided |
technologist |
ObjectNotFoundException: No row with the given identifier exists: [com.micros.creations.core.domain.User#<###>] |
Technologist not found |
productRecordOtherUser/user |
DtoValidationException: ProductRecordOtherUser has no valid user for role:<###> ProductRecordOtherUser Roles are mandatory for the following missing Roles: [<###>] |
No tag provided User not found |
productRecordOtherUser/user |
ObjectNotFoundException: No row with the given identifier exists:[<<hostname>>.Usert#<###>] |
No value provided |
productRecordOtherUser/role |
DtoValidationException: ProductRecordOtherUser has no valid user for role:<###> ProductRecordOtherUser Roles are mandatory for the following missing Roles: [<###>] |
No tag provided User not found |
productRecordOtherUser/role |
ObjectNotFoundException: No row with the given identifier exists:[<<hostname>>.Role#<###>] |
No value provided |
supplier |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.Company using code:<###> |
No tag provided |
supplier |
MySQLIntegrityConstraintViolationException: Cannot add or update a child row: a foreign key constraint fails ('<###>'.'t_prdrcd',CONSTRAINT '<###>' FOREIGN KEY ('fk_supplier') REFERENCES 't_company' ('c_id')) |
No value provided Supplier not found |
site |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.Site using id:<###>, code:<###>, supplierCode:<###> and supplierId:<###> |
No tag provided No value provided Supplier not found |
countryWhereSold |
HTTP 417 IllegalStateException: Cannot locate keyword for class <<hostname>>.CountryWhereSold using code:<###> and spec type:<###> |
No value provided |
countryWhereSold |
ObjectNotFoundException: No row with the given identifier exists:[<<hostname>>.CountryWhereSold#<###>] |
Country not found |
ERROR: class <<hostname>>.ProductRecord.barcode[<###> - Bar Code must be either 8 or 13 characters long |
Invalid code length |
|
ERROR: class<<hostname>>.ProductRecord.shippingCaseCode[<###> - Shipping Case Code must be either 14 digits long |
Invalid code length |
Update Record
Description
Updates an existing Product record. Use this function to update a Product record's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/productRecord/{id} HTTP method: PUT
Request Details
The body of the request contains a ProductRecordFullDTO to specify the updates to the Product record. Compared to retrieving a Product record (which uses the same ProductRecordFullDTO type), this request is much shorter. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is the same as that for creating a Product Record. After the call, the Product Record is updated to match the request.
Note:
When updating records, all values must be included. If a value is or element is omitted from the request, the field contents will be cleared on the Product record.
When a Product Specification is initially linked to the Product record, any values in the product title, barcode, shipping case code, and quantity fields are carried through to the Specification. If subsequent changes are made to the barcode or shipping case code using the Product Record API, the changes will not be automatically cascaded to the Specification, however, if the Specification is at draft status it may be manually delinked and relinked to the Product record in order to refresh these values.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 3 - Product for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a ProductRecordLink element. The ProductRecordLink element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Product record's internal ID |
recordLink |
string |
URI to the Product record, for use in a GET request |
code |
string |
Code business key to the Product record |
title |
string |
Product's title |
Error Responses
If the supplied data does not result in a valid Product record (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
ProductSpecificationRestService
This section describes the API for managing Product Specifications. The following functions are available:
-
List of Values: retrieves a list of Product Specifications
-
Retrieve Record by ID: retrieves a Product Specification using its unique identifier
-
Retrieve Record by Business Key: retrieves a Product Specification using its business key
-
Retrieve List with Advanced Filtering: retrieves a Product Specification using additional filtering options
-
Check Record Modification Timestamp: retrieves the timestamp when a Product Specification was last updated
-
Create Record: creates a new Product Specification record
-
Update Record: updates an existing Product Specification record
List of Values
Description
Retrieves a list of Product Specifications in a paged list. Use this function to retrieve a simple list of product titles and IDs, or to locate Product Specification IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/specification HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example | Validation |
---|---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
|
pageSize |
Optional |
int |
No |
30 |
|
specType |
Optional |
string |
Yes |
FOOD~CNF |
|
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
|
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
|
specKey |
Optional |
string |
Yes |
12345-1 |
Can be used to return one or more specifications by specifying the specification number and version. The - character is used to separate the spec number and versions. For example, to return a single specification: 1234-1; to return three specifications: 12345-1~12345-2~98765-1 Validation ensures that each pair of values contains a digit, otherwise the message Invalid Specification key, must contain a numeric specification number and specification version is returned. So 1234-1 would be valid, 1234-one or 1234- would be invalid. |
productTechnologist |
Optional |
string |
Yes |
John Smith |
The name of the product technologist. Validation ensures that each value is a valid user name, otherwise the message Invalid Product Technologist is returned. |
packCopyLocale |
Optional |
string |
No |
en_GB |
Limits the retuned specifications to those where their pack copy language is the language specified. Validation ensures that each value is a valid Locale code, otherwise the message Invalid Pack Copy Locale is returned. So pt_BR would be valid; Brazil would be invalid. |
status |
Optional |
string |
Yes |
RETAILER_ DRAFT |
Available options: SUPPLIER_DRAFT RETAILER_DRAFT COLLABORATIVE_DRAFT GATE_STEP PART_PACK_COPY_SENT PACK_COPY_SENT PACK_COPY_READY READY_FOR_AUTHORISATION SUPPLIER_AUTHORISED ACTIVE OFF_RANGE DE_LISTED SUPERSEDED NOT_PROGRESSED APPROVE_FOR_LABELLING PRODUCE_DRAFT PRODUCE_PACK_COPY PRODUCE_APPROVED PRODUCE_ARCHIVED |
title |
Optional |
string |
Yes |
12345 |
Applies the filter to the Product Title in the specification's Header section to return specifications that contain the specified value in the Product Title or Product Title in Business Language. |
supplierName |
Optional |
string |
Yes |
ABC Supplies Ltd. |
Applies the filter to the Supplier Name (in the Supplier Information table for Produce) to return specifications that contain the specified value in the Supplier Name or Supplier Name in Business Language. |
siteName |
Optional |
string |
Yes |
ABC London |
Applies the filter to the Site Name in the Primary Sites table (in the Supplier Information table for Produce) to return specifications that contain the specified value in the Site Name or Site Name in Business Language. |
specNumber |
Optional |
integer |
No |
12 |
The specification number. Mandatory if Version filter is used. |
version |
Optional |
integer |
No |
1 |
Used in association with SpecNumber. Must be at least 1. |
brand |
Optional |
string |
Yes |
BRANDA |
The Code for the required Brand. |
businessArea |
Optional |
string |
Yes |
UK |
The Code for the required Business Area. |
language |
Optional |
string |
Yes |
en_GB |
The Code for the required pack copy language, such as, en_GB. Specifying a preferred language will return only values in that language. If not specified, the base language is assumed. |
supplierCode |
Optional |
string |
Yes |
A0001 |
Filters on the Primary Sites supplier code for Produce Specifications or the Supplier code for all other types of Specification. |
siteCode |
Optional |
string |
Yes |
A0001-001 |
Filters on the code of the Sites in the Primary Sites table of the Product Specification. Use the full site code incorporating the Supplier Code, such as A0001-001. |
businessCategory |
Optional |
string |
Yes |
CHEESE_DAIRY |
The Code for the required Business Category. Searches all levels of Business Category and Lead Business Category path. Returns a result if any of the values match any of the specification's business categories. |
productNumber |
Optional |
string |
Yes |
12345 |
Filters on the Product Number column of the Product Coverage table. |
countryWhereSold |
Optional |
string |
Yes |
FOOD_COUNTRY_WHERE_SOLD |
The Code for the required Country Where Sold. |
productCoverage |
Optional |
string |
Yes |
Chicken (500g) |
Filters on the Product Name column of the Product Coverage table. Note: The source of the Product Coverage data is the Product Specification, regardless of the specification type and specification status. |
specificationSectionType |
Optional |
string |
Yes |
RECIPE_AND_RAW_MATERIALS |
Available values: ADVANCED_PACKAGING ALLERGY_AND_DIETARY_ADVICE BATCH_CODING CLAIMS_SUBSTANTIATION COMPONENTS COUNTER_TICKET FINISHED_PRODUCT_STANDARDS FNF_STORAGE MAIN_DETAILS NUTRITION OTHER_LABELLING_COPY PACKAGING POST_LAUNCH_INFORMATION PROCESS_CONTROLS PRODUCT_APPROVAL_REQUIREMENTS PRODUCT_CHARACTER_COMP PRODUCT_REQUIREMENTS RECIPE_AND_RAW_MATERIALS STORAGE |
productRecordCode |
Optional |
integer |
No |
123456 |
|
altProductNumber |
Optional |
string |
No |
ABC001 |
|
division |
Optional |
string |
Yes |
DIV_A~DIV_B |
Must be the code of an entry in the Divisions glossary. |
statusChangedFrom |
Optional |
string |
No |
2018-06-10 09:00:00 |
Date the specification status changed. |
statusChangedUntil |
Optional |
string |
No |
2018-06-10 09:00:00 |
Date the specification status changed. Date must be either the same as, or after the statusChangedFrom parameter. |
statusChangedTo |
Optional |
string |
Yes |
ACTIVE |
Must have a statusChangedFrom and/or status changedTo entry, if used. Specification status codes Allowable values: SUPPLIER DRAFT RETAILER_DRAFT COLLABORATIVE_DRAFT GATE-STEP PART_PACK_COPY_SENT PACK_COPY_SENT PACK_COPY_READY READY_FOR_AUTHORISATION SUPPLIER_AUTHORISED ACTIVE OFF_RANGE DE-LISTED SUPERSEDED NOT_PROGRESSED APPROVE_FOR_LABELLING PRODUCE_DRAFT PRODUCE_PACK_COPY PRODUCE_APPROVED PRODUCE_ARCHIVED |
softDelete |
Optional |
string |
No |
true This also includes specifications that have been soft deleted. Otherwise soft deleted specifications are excluded. Soft deleted specifications are those that show in the Deleted Specifications list view within Brand Compliance. |
Note:
The status filter applies to the specification's current status. The statusChanged filters are used together to retrieve specifications that changed to the statuses specified in statusChangedTo within the date range specified in statusChangedFrom and statusChangedUntil.
This could also be used with status to, for example, retrieve all the specifications that are currently Active, and changed from Collaborative Draft or Gate Step within a particular date range.
Example URLs
…/services/rest/specification/?offset=2&pageSize=20 …/services/rest/specification/?specType=FOOD
Response Details
For a successful response, XML is returned with a ProductSpecificationLinkList root element containing an entries element for each matched Product Specification. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Product Specification's internal ID |
recordLink |
string |
URI to the ProductSpecificationRestService Retrieve record service for this specification |
specNumber |
string |
Specification number business key to the Product Specification record |
specVersion |
int |
Specification version business key to the Product Specification record |
title |
string |
Product Specification's title |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
Element | Message | Meaning |
---|---|---|
<errorCode>NOFILTER <errorMessage>No Filter Specified, please try again |
No parameter provided |
|
pageSize |
<errorCode>INVALIDBATCHSIZE <errorMessage>The Batch Size must be between 1 and 100 |
Invalid batch size No batch size provided No batch size tag |
specStatus |
<errorCode>INVALIDSPECSTATUS <errorMessage>The Status <###> does not exist |
Spec Status not found Spec Status is blank |
specType |
<errorCode>INVALIDSPECTYPE <errorMessage>The Spec Type <###> does not exist |
Spec Type not found Spec Type is blank |
countryWhereSold |
<errorCode>INVALIDCOUNTRYWHERESOLD <errorMessage>The Country Where Sold >###> does not exist |
Country not found Country is blank |
language |
<errorCode>INVALIDLANGUAGE <errorMessage>The Language <###> does not exist |
Language not found Language is blank |
sectionType |
<errorCode>INVALIDSECTIONTYPE <errorMessage>The Section Type does not exist |
Section type not found Section type is blank |
fromDate |
HTTP 400 Bad Request |
Invalid date/time format |
toDate |
HTTP 400 Bad Request |
Invalid date/time format |
Retrieve Record by ID
Description
Retrieves a single Product Specification's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual specification.
Endpoint address: /services/rest/specification/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve. Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example | Validation |
---|---|---|---|---|---|
specificationSectionType |
Optional |
string |
Yes |
STORAGE |
Allowable Values: ADVANCED_PACKAGING ALLERGY_AND_DIETARY_ADVICE BATCH_CODING CLAIMS_SUBSTANTIATION COMPONENTS COUNTER_TICKET FINISHED_PRODUCT_STANDARDS FNF_STORAGE MAIN_DETAILS NUTRITION OTHER_LABELLING_COPY PACKAGING POST_LAUNCH_INFORMATION PROCESS_CONTROLS PRODUCT_APPROVAL_REQUIREMENTS PRODUCT_CHARACTER_COMP PRODUCT_REQUIREMENTS RECIPE_AND_RAW_MATERIALS STORAGE This filter should behave like the equivalent filter in the existing Specifications SOAP API - only returning data from the Main Details section plus the specified sections. |
locale |
Optional |
string |
Yes |
en_GB~fr |
Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/specification/97
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a productSpecificationFullDTO root element containing the individual attributes of the requested specification. If an ID is not specified, a list of all specifications is returned (per the List of Values function).
Note:
The XML structure caters for nutrient targets in the Nutrition section.
The foodStandardCategory element provides the category description details; a specificationFoodStandardCategoryDetail element per nutrient in the category provides the details of the nutrients and whether the target was achieved, for each.
The foodStandardCategory and specificationFoodStandardCategoryDetail elements are both children to the specificationSectionFoodNutritionSection element.
The response includes the following elements, which are sourced from the Product Record associated with the specification: Business Category, Lead Business Category, Product Coverage table (Product Name, Product Number, Quantity, Alt. Product Number, and Division).
If the locale parameter is used, fields that can hold a separate on-pack value in the specification's pack copy language have those values returned in a packCopyLocaleData element. If no value is present, the element is omitted. For example, if the API is called with the locale parameter set to the portal's base language and the specification's pack copy language is French, the XML returned for the FoP Product Type field would be:
<frontOfPackProductType> <code>ANZ_FULL_PER_PACK_SOLID_FOOD</code> <packCopyLocaleData> <description>Full Table Per Pack Solid Food</description> <id>32</id> <locale>fr</locale> <referenceIntakeText> DI*</referenceIntakeText> </packCopyLocaleData> <localeData> <description>Full Table Per Pack Solid Food</description> <id>31</id> <referenceIntakeText> DI*</referenceIntakeText> </localeData> ... </frontOfPackProductType>
Change History:
The date and time of a specification's status change is included as a timestamp value within the statusHistory element. This can be used to determine the age distribution of specifications. For example:
<ns1:statusHistory> <ns1:comments>...</ns1/comments <ns1:localData> <ns1:statusFrom>Deleted</ns1:statusFrom> <ns1:statusTo>Retailer Draft</ns1:statusTo> <ns1:id>160544</ns1:id> </ns1:localData> <ns1:id>3682</ns1:id> <ns1:createdOn>2018-12-20T12:23:43.000Z</ns1:createdOn> <ns1:updatedOn>2018-12-20T12:23:43.000Z</ns1:updatedOn> </ns1:statusHistory> <ns1:statusHistory> <ns1:localData> <ns1:statusTo>Retailer Draft</ns1:statusTo> <ns1:statusFrom>Deleted</ns1:statusFrom> <ns1:id>160531</ns1:id> </ns1:localData> <ns1:id>3681</ns1:id> <ns1:createdOn>2018-12-20T12:22:52.000Z</ns1:createdOn> <ns1:updatedOn>2018-12-20T12:22:52.000Z</ns1:updatedOn> </ns1:statusHistory>
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 4 - Product (Food Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 5 - Product (CNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 6 - Product (FNF Specification), and Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 7 - Product (BWS Specification) for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Product Specification to return with id:<###> |
Invalid {id} - not found |
specificationSectionType |
Error Code: INVALIDSPECIFICATIONSECTIONTYPE |
Invalid Specification Section Type |
Retrieve Record by Business Key
Description
Retrieves a single Product Specification's details using its business key (specification number and version). Use this function to retrieve the full details of an individual specification using its Brand Compliance specification number and version.
Endpoint address: /services/rest/specification/byKey/{specNumber}/{specVersion} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve. Parameters are passed as URI parameters.
Example URL
…/services/rest/specification/byKey/104/1
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return a productSpecificationFullDTO root element containing the individual attributes of the requested specification.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a productSpecificationFullDTO root element containing the individual attributes of the requested specification.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 4 - Product (Food Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 5 - Product (CNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 6 - Product (FNF Specification), and Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 7 - Product (BWS Specification) for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
specNumber |
HTTP 404 Not Found |
Invalid {specNumber} - blank |
specNumber |
HTTP 404 Not Found |
Invalid {specNumber} - not found |
specVersion |
HTTP 404 Not Found |
Invalid {specVersion} - blank |
specVersion |
HTTP 404 Not Found |
Invalid {specVersion} - not found |
specificationSectionType |
Error Code: INVALIDSPECIFICATIONSECTIONTYPE |
Invalid Specification Section Type |
Retrieve List with Advanced Filtering
Description
Retrieves a list of Product Specifications in a paged list using advanced filtering. Use this function to retrieve specifications based on the values of specific fields.
Endpoint address: /services/rest/specification/advanced HTTP method: POST
Request Details
Parameters are passed in the request in XML format.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example | Validation |
---|---|---|---|---|---|
offset |
Mandatory |
int |
No |
60 |
>0 |
batchsize |
Mandatory |
int |
No |
30 |
>0 and <=100 |
specType |
Optional |
string |
Yes |
FOOD |
FOOD FNF CNF PRODUCE BWS |
specStatus |
Optional |
string |
Yes |
RETAILER_ DRAFT |
SUPPLIER_DRAFT RETAILER_DRAFT COLLABORATIVE_DRAFT GATE_STEP PART_PACK_COPY_SENT PACK_COPY_SENT PACK_COPY_READY READY_FOR_AUTHORISATION SUPPLIER_AUTHORISED ACTIVE OFF_RANGE DE_LISTED SUPERSEDED NOT_PROGRESSED APPROVE_FOR_LABELLING PRODUCE_DRAFT PRODUCE_PACK_COPY PRODUCE_APPROVED PRODUCE_ARCHIVED |
productNumber |
Optional |
string |
Yes |
12345 |
Filters on the Product Number column of the Product Coverage table. |
language |
Optional |
string |
Yes |
en_GB |
The Code for the required pack copy language, such as, en_GB. Specifying a preferred language will return only values in that language. If not specified, the base language is assumed. |
specificationSectionType |
Optional |
string |
Yes |
RECIPE_AND_RAW_MATERIALS |
Available values: ADVANCED_PACKAGING ALLERGY_AND_DIETARY_ADVICE BATCH_CODING CLAIMS_SUBSTANTIATION COMPONENTS COUNTER_TICKET FINISHED_PRODUCT_STANDARDS FNF_STORAGE MAIN_DETAILS NUTRITION OTHER_LABELLING_COPY PACKAGING POST_LAUNCH_INFORMATION PROCESS_CONTROLS PRODUCT_APPROVAL_REQUIREMENTS PRODUCT_CHARACTER_COMP PRODUCT_REQUIREMENTS RECIPE_AND_RAW_MATERIALS STORAGE |
countryWhereSold |
Optional |
string |
Yes |
FOOD_COUNTRY_WHERE_SOLD |
The Code for the required Country Where Sold. |
Product Coverage |
Optional |
string |
Yes |
Chicken (500g) |
Filters on the Product Name column of the Product Coverage table. |
supplier |
Optional |
string |
Yes |
A0001 |
Filters on the Primary Sites supplier code for Produce Specifications or the Supplier code for all other types of Specification. |
site |
Optional |
string |
Yes |
A0001-001 |
Filters on the code of the Sites in the Primary Sites table of the Product Specification. Use the full site code incorporating the Supplier Code, such as A0001-001. |
fromDate |
Optional |
string |
No |
2013-06-10T09:00:00 |
Can be used in conjunction with toDate to form a date range or can be specified individually. |
toDate |
Optional |
string |
No |
2013-06-10T09:00:00 |
Can be used in conjunction with fromDate to form a date range or can be specified individually. |
For example, to retrieve the Main Details and Nutrition sections for active Food specifications where the product number is ABC001 from a specific supplier/site, the request would be:
<request> <batchsize>100</batchsize> <offset>0</offset> <specStatus>ACTIVE</specStatus> <specType>FOOD</specType> <countryWhereSold>UK</countryWhereSold> <productNumber>ABC001</productNumber> <language>en_GB</language> <sectionType>MAIN_DETAILS</sectionType> <sectionType>NUTRITION</sectionType> <supplier>A0001</supplier> <site>A0001-0001</site> <fromDate>2016-01-01T01:00:00</fromDate> <toDate>2016-10-01T01:00:00</toDate> </request>
If no filtering is required, omit the parameter from the call. For example, if all specification types are to be included, omit the specType element (rather than including it with no value specified).
If multiple values for a parameter are to be included, repeat the parameter in the call. For example, if Main Details and Nutrition section types are to be returned, include two specificationSectionType elements in the call, one for MAIN_DETAILS and one for NUTRITION.
Searching with either fromDate or toDate specified, as well as one or more specStatus, filters the specifications on the Status Change History. Use this to retrieve specifications that changed to a specific status during a date range.
Searching with either fromDate or toDate specified without specStatus filters on the specification's last amended date.
When filtering with site codes, the following rules apply:
-
For non-unique site codes, for example passing a site code filter which finds more than one site with the same code: specifications from all sites with the site code are returned.
-
For non-unique site codes with a uniquely identifying supplier code, for example passing a site code and a supplier code: specifications from sites with site codes linked to the supplier code are returned.
-
For unknown site codes, for example passing a site code which does not find any matching sites: no specifications are returned.
-
For combination of unique, non-unique, and unknown site codes, for example passing multiple different site codes, which find a mix of more than one site with the same code, a single matching site, and no matching site: specifications from all sites with the relevant site codes are returned.
-
If no filters, for example when not passing in any site code data: specifications are returned based upon the other parameters.
Response Details
For a successful response, XML is returned with a GetProductSpecificationServiceResponse root element containing details of the specifications that match the selection criteria.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 4 - Product (Food Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 5 - Product (CNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 6 - Product (FNF Specification), and Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 7 - Product (BWS Specification) for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
<errorCode>NOFILTER <errorMessage>No Filter Specified, please try again |
No parameter provided |
|
batchSize |
<errorCode>INVALIDBATCHSIZE <errorMessage>The Batch Size must be between 1 and 100 |
Invalid batch size No batch size provided No batch size tag |
specStatus |
<errorCode>INVALIDSPECSTATUS<errorMessage>The Status <###> does not exist |
Spec Status not found Spec Status is blank |
specType |
<errorCode>INVALIDSPECTYPE <errorMessage>The Spec Type <###> does not exist |
Spec Type not found Spec Type is blank |
countryWhereSold |
<errorCode>INVALIDCOUNTRYWHERESOLD <errorMessage>The Country Where Sold >###> does not exist |
Country not found Country is blank |
language |
<errorCode>INVALIDLANGUAGE <errorMessage>The Language <###> does not exist |
Language not found Language is blank |
sectionType |
<errorCode>INVALIDSECTIONTYPE <errorMessage>The Section Type does not exist |
Section type not found Section type is blank |
fromDate |
HTTP 400 Bad Request |
Invalid date/time format |
toDate |
HTTP 400 Bad Request |
Invalid date/time format |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Product Specification. Use this function to determine when a specification's details were last updated.
Endpoint address: /services/rest/specification/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/specification/97
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested specification.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Product Specification to return with id:<###> |
Invalid {id} - not found |
Create Record
Description
Creates a new Product Specification record. Use this function to create a new specification in Brand Compliance based on data sourced from the external system.
Dependencies: If linking to a Product Record, the active Product Record must be present and in the application and its record ID obtained; the Supplier and Site (and associated Users, Contacts, and Business Categories) must be present in the application and the record IDs obtained. For more information, see Dependencies.
Endpoint address: /services/rest/specification HTTP method: POST
Request Details
The body of the request contains a ProductSpecificationFullDTO to specify the detail of the specification to create. Compared to retrieving a specification (which uses the same ProductSpecificationFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created specification need to be included. As a minimum, this must include the fields shown in the following table.
Product Specification Mandatory Fields
Field Name | Element Name |
---|---|
Specification Name |
title |
Legislation |
legislation |
Pack Copy Language |
packCopyLanguage / code |
Specification Type |
specTypeFormat / code |
Specification Number (unique) |
specNumber |
Version |
specVersion |
Status |
productSpecificationStatus / status |
Multi-pack Specification |
isMultipack |
Main Details Section (container) |
specificationSectionDetail/specificationSectionFoodMainDetailsSection |
Deleted Record |
deleted (Set to false) |
Note:
These fields represent the minimum necessary to create a specification. Various other fields will be mandatory in order to then progress the specification through its workflow.
The Main Details Section "container" is necessary to create the specification's Main Details section. For specification types other than Food, the specificationSectionFoodMainDetailsSection will be alternatively named accordingly.
To ensure a valid unique business key code is automatically assigned to the record being created, a value of zero must be passed in the <code> element of the XML when submitting the POST request.
Assigning the Supplier and Contacts
As described in Dependencies above, if the specification is to be linked to a supplier and contact users, the necessary record IDs must be obtained and populated when creating the specification (or updated after creation).
When a Product Record is created through the application UI and is linked to a Product Specification, the system automatically assigns the supplier, site, and contacts to the specification. When created through the API, these links must be formed by providing the appropriate record IDs.
For a specification to be assigned to a supplier and site, it must also be linked to a Product Record. A Supplier must exist and have at least one Site assigned to it; a Product Record must exist and have the Supplier and Site assigned to it. The record IDs of the Supplier, Site, and Product Records must be obtained using the GET method of the respective APIs, and inserted in the XML message when creating or updating the Product Specification.
For users to be assigned to a specification as contacts, the record IDs of the Contacts and Users must be obtained using the GET method of the respective APIs and inserted into the XML message (per contact type) when creating or updating the Product Specification.
The same applies for other values that may be derived from the Product Record, such as Business Category and Country Where Sold.
Retailer Contacts do not appear in the Specification API’s XML; they are derived in the UI from the linked Product Records.
Example Request XML
This example shows the minimum requirement to be able to create a specification.
<ns0:productSpecificationFullDTO xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full" xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple"> <ns0:isMultipack>false</ns0:isMultipack> <ns0:legislation>MODULE_TYPE_EU</ns0:legislation> <ns0:packCopyLanguage> <ns1:code>en_GB</ns1:code> </ns0:packCopyLanguage> <ns0:specNumber>8925</ns0:specNumber> <ns0:specTypeFormat> <ns1:code>FOODUK</ns1:code> </ns0:specTypeFormat> <ns0:specVersion>1</ns0:specVersion> <ns0:specificationSectionDetail> <ns0:specificationSectionFoodMainDetailsSection> </ns0:specificationSectionFoodMainDetailsSection> </ns0:specificationSectionDetail> <ns0:title>API Test Specification</ns0:title> <ns0:productSpecificationStatus> <ns1:status>RETAILER_DRAFT</ns1:status> </ns0:productSpecificationStatus> </ns0:productSpecificationFullDTO> </ns0:productSpecificationStatus> <ns0:deleted>false</ns0:deleted> </ns0:productSpecificationFullDTO>
Set the deleted element to false to ensure the specification can be seen in the UI. If omitted, or set to true, the specification will not be visible.
Where the record is linked to another record, such as the Specification Type in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 4 - Product (Food Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 5 - Product (CNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 6 - Product (FNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 7 - Product (BWS Specification), and Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 8 - Product (Produce Specification) for details of their mapping to the fields within the Brand Compliance UI.
Response Details
For a successful response, an HTTP 200 response is sent with a body containing a ProductSpecificationLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Product Specification's internal ID |
recordLink |
string |
URI to the newly created Product Specification record, for use in a GET request |
specNumber |
long |
Specification Number business key to the newly created Product Specification record |
specVersion |
int |
Specification Version business key to the newly created Product Specification record |
title |
string |
Product Specification's title |
Error Responses
If the supplied data does not result in a valid Product Specification (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Update Record
Description
Updates an existing Product Specification. Use this function to update a Product Specification's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/specification/{id} HTTP method: PUT
Request Details
The body of the request contains a ProductSpecificationFullDTO element to specify the updates to the specification. Compared to retrieving a specification (which uses the same ProductSpecificationFullDTO type), this request is much shorter. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
The request content is similar to that for creating a specification. After the call, the Product Specification record is updated to match the request.
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the Product Specification record.
The fields in the Product Coverage table (variant name, quantity, and product number) may be updated regardless of the status of the specification. If the specification is at a draft status, the Product Coverage parent element is <ns0:productCovered> (the Product Record); otherwise, it is <ns0:specificationProductCovered> (a snapshot of the data held on the Product Specification).
Cascade update of Product/Article Number:
Where there is a one-to-one relationship, it is possible for the Retailer Product Number (also known as the Article number) in the Product Coverage table to be automatically updated in the Specification when it is updated in the related Product Record (or vice versa for Produce Specifications). This is of use where the product number is assigned to the Product Record, typically from an external system, after the Specification has been created. Use of the feature is controlled by system parameters.
In order for the cascade update to occur, the user must form a unique key using the Alternative Product Number and Division fields in the Product Coverage table. The cascade only updates the Retailer Product Number; it does not add or remove rows in the Product Coverage table; if the Alternative Product Number or Division change, a new version of the Product Record must be created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 4 - Product (Food Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 6 - Product (FNF Specification), Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 7 - Product (BWS Specification), and Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 8 - Product (Produce Specification) for details of their mapping to the fields within the Brand Compliance UI.
Advanced Packaging Section
When maintaining the Advanced Packaging section using the UI, when a row is added to the Packaging Component table, if its Material or Level is configured to require a recycling advice icon, a corresponding row is automatically added to the Recycling Advice table to capture the details for that Component and Material/Level Component (on clicking the Refresh Packaging Data button). The parent/child relationship is formed automatically by the system, where the child Recycling Advice row references its parent Packaging Component row’s Global Id. When updating Advanced Packaging section using the API, the global id relationship must also be maintained.
When adding to the Packaging Components table, there are two options:
-
Omit the global id, to have the system allocate it. For this method, it is not possible to add to the Recycling Advice table at the same time. It requires a secondary update after retrieving the globalId the system has assigned.
-
Specify the global id on the Packaging Components record. This method allows entries to be added to the Packaging Component and Recycling Advice at the same time, but requires a globalId to be supplied in the XML.
Further details are as follows:
-
The basic structure of the XML that represents the Packaging Components and Recycling Advice tables (the example below is for Food, but applies to all specification types):
<specificationSectionFoodAdvancedPackagingSection> <advancedPackagingComponentDetails> - contains <globalId> to uniquely identify the row <advancedPackagingRecyclingAdviceDetails> <advancedPackagingComponentDetail> - contains <entityGlobalId> to reference the parent <globalId>
-
When adding a child
advancedPackagingComponentDetail
record, it is not possible to create a childadvancedPackagingRecyclingAdviceDetails
record at the same time, unless<globalId>
is populated for the<advancedPackagingComponentDetails>
record. -
Validation will prevent the generation of an
advancedPackagingRecyclingAdviceDetails
record when its parent (advancedPackagingComponentDetail
) global id is not specified, and will return code #417, with an appropriate error message instead. -
When
globalId
in the<advancedPackagingComponentDetails>
is omitted, the system will automatically assign a global id to the<advancedPackagingComponentDetails>
, as previously. -
When generating an
advancedPackagingRecyclingAdviceDetails
record, the system automatically controls the creation of its child<advancedPackagingComponentDetail>
reference data, from the Parent<advancedPackagingComponentDetails> <globalId>
(thereby maintaining the same parent/child relationship as if the process was initiated through the UI). -
If the global id of an
advancedPackagingComponentDetails
record is modified, the childadvancedPackagingRecyclingAdviceDetails
records will be automatically updated accordingly, therefore always maintaining the correct reference to the parent entity. -
The
advancedPackagingRecyclingAdviceDetails
records’ child<advancedPackagingComponentDetail>
reference data is now read only.
An outline example of the process is as follows:
-
Assuming the Advanced Packaging Section exists, with empty
advancedPackagingComponentDetails
andadvancedPackagingComponentDetail
(the bare minimal data), retrieve the specification XML.<ns1:productSpecificationFullDTO> <ns1:specificationSectionDetail> <ns1:specificationSectionFoodAdvancedPackagingSection>
-
Add
advancedPackagingComponentDetails
.<ns1:productSpecificationFullDTO> <ns1:specificationSectionDetail> <ns1:specificationSectionFoodAdvancedPackagingSection> <ns1:advancedPackagingComponentDetails> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> </ns1:advancedPackagingComponentDetails>
-
Submit the update.
-
Re-retrieve the specification XML.
<ns1:productSpecificationFullDTO> <ns1:specificationSectionDetail> <ns1:specificationSectionFoodAdvancedPackagingSection> <ns1:advancedPackagingComponentDetails> <ns1:globalId>fbe43b25-ae66-492e-a596-cbe8eabacdaa</ns1:globalId> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> <ns1:id>791</ns1:id> </ns1:advancedPackagingComponentDetails>
-
Add
advancedPackagingRecyclingAdviceDetails
.<ns1:productSpecificationFullDTO> <ns1:specificationSectionDetail> <ns1:specificationSectionFoodAdvancedPackagingSection> <ns1:advancedPackagingComponentDetails> <ns1:advancedPackagingRecyclingAdviceDetails> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> <ns1:advancedPackagingRecyclingAdviceDetails> <ns1:globalId>fbe43b25-ae66-492e-a596-cbe8eabacdaa</ns1:globalId> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> <ns1:id>791</ns1:id> </ns1:advancedPackagingComponentDetails>
-
Re-retrieve the specification XML. This will automatically add
advancedPackagingComponentDetail
.<ns1:productSpecificationFullDTO> <ns1:specificationSectionDetail> <ns1:specificationSectionFoodAdvancedPackagingSection> <ns1:advancedPackagingComponentDetails> <ns1:advancedPackagingRecyclingAdviceDetails> <ns1:advancedPackagingComponentDetail> <ns1:entityGlobalId>fbe43b25-ae66-492e-a596-cbe8eabacdaa</ns1:entityGlobalId> <ns1:entityReferenceClass>com.micros.creations.core.domain.AdvancedPackagingComponentDetail</ns1:entityReferenceClass> <ns1:id>735</ns1:id> </ns1:advancedPackagingComponentDetail> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> <ns1:id>545</ns1:id> </ns1:advancedPackagingRecyclingAdviceDetails> <ns1:globalId>fbe43b25-ae66-492e-a596-cbe8eabacdaa</ns1:globalId> <ns1:specType>FOOD</ns1:specType> <ns1:specTypeText>Food</ns1:specTypeText> <ns1:id>791</ns1:id> </ns1:advancedPackagingComponentDetails>
Opening the specification in the UI, the Advanced Packaging section shows the Packaging Component table now has a single row (with data as ‘-’), and also the Recycling Advice table has a single row (again with data as ‘-’). To define the relevant columns of data for these tables, it is of course necessary to define the appropriate XML data accordingly.
Response Details
If successful, an HTTP 200 response is sent with a body containing a ProductSpecificationLink element. The ProductSpecificationLink element consists of the returned elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Product Specification's internal ID |
recordLink |
string |
URI to the Product Specification record, for use in a GET request |
code |
string |
Code business key to the Product Specification |
specNumber |
long |
Specification Number business key to theProduct Specification record |
specVersion |
int |
Specification Version business key to the Product Specification record |
title |
string |
Specification's title |
Error Responses
If the supplied data does not result in a valid Product Specification (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating an Internal Error Id. The request should not be reattempted with the same content.
TaskRestService
This section describes the API for managing user tasks. The following function is available:
-
List of Tasks: Retrieves a list of user tasks
List of Tasks
Description
Retrieves a list of tasks for a user in the given language. Use this function to retrieve a list of a specific user's entries in their Brand Compliance Task Manager app. Parameters are used to specify the name of the user, and the language of the returned task details.
Endpoint address: /services/rest/task HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/ Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
userId |
Mandatory |
string |
No |
John Smith |
language |
Mandatory |
string |
No |
en_GB |
Example URL
…/services/task/?language=en_GB
The userId parameter is the login ID of the user for which the tasks list is to be retrieved. The language parameter is the code of the language record/locale in which to retrieve the task details.
Response Details
For a successful response, XML is returned with a TaskDTOList root element containing a tasks element for each matched task. The root element consists of the elements in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
message |
string |
Name of the task, in the language specified |
messageId |
string |
A language-agnostic identifier for the task |
myCreationsLink |
string |
A URI to the Brand Compliance system, which will open the list of items for that task |
taskItemCount |
int |
The number of items in the task |
Error Messages
Element | Message | Meaning |
---|---|---|
userId |
userId required |
User not provided |
userId |
Cannot find user with login id <###> |
User not found |
language |
language required |
Language not provided |
language |
Invalid locale format: <###> |
Language not found |
UrgentItemsRestService
This section describes the API for retrieving a count of a user's urgent items. The following function is available:
-
Number of Urgent Items: retrieves the number of urgent items pending for a user
Number of Urgent Items
Description
Retrieves a count of the number of Urgent Item tasks for a user. Use this function to determine the number of pending urgent tasks a specific user has in their Brand Compliance Urgent Item Manager app. A parameter is passed to specify the name of the user.
Endpoint address: /services/rest/urgentItems HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/ Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
userId |
Mandatory |
string |
No |
John Smith |
The userId parameter is the login ID of the user for which the number of pending urgent items are to be retrieved.
Response Details
For a successful response, XML is returned with an UrgentItemsModel root element containing an itemCount element that specifies the number of Urgent Items.
Error Messages
Element | Message | Meaning |
---|---|---|
userId |
User not provided: userId required |
User not provided |
userId |
Cannot find user with login id <###> |
User not found |
ArtworkRestService
This section describes the API for Artwork integration. The following functions are available:
-
Started Activities: retrieves a list of Artwork Activities that have started
-
Update Record: updates existing Artwork Activities
Started Activities
Description
Retrieves a list of Project Activities that have their useMyArtwork flag set, and their status has changed to Started within the specified date range. This function is used for the integration of Brand Compliance with an external Artwork Management system.
Endpoint address: /services/rest/artwork/started HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
0 |
pageSize |
Optional |
int |
No |
30 |
fromDate |
Mandatory |
string |
No |
2014-12-30 23:59:59 |
toDate |
Mandatory |
string |
No |
2015-12-30 23:59:59 |
Response Details
For a successful response, XML is returned with a CreateArtworkRequestList root element containing an entries element for each matching Artwork project activity. A totalRecords element identifies the number of records returned. Separate activityDetails and project elements group the returned activity and project data. The elements consist of the elements in the following tables.
Returned Elements - activityDetails
Element | Type | Description |
---|---|---|
activityName |
string |
Project activity's title |
activityRecordId |
long |
Project activity record's internal ID |
actualStartDate |
string |
Actual start date of the project activity |
checkpointOnly |
Boolean |
Is the project activity a checkpoint only type |
criticalPath |
Boolean |
Is the project activity a critical path type |
duration |
int |
Duration of the project activity |
isAutoGate |
string |
Project's type of auto gate |
isGate |
Boolean |
Is the project activity a gate type |
isKey |
Boolean |
Is the project activity a key type |
projectId |
long |
Project record's internal ID |
projectTitle |
string |
Project's title |
proposedEndDate |
string |
Proposed end date of the project activity |
proposedStartDate |
string |
Proposed start date of the project activity |
responsibleUserRoles |
string |
Role names of the responsible users |
sequenceNumber |
string |
Project activity's sequence number |
statusCode |
string |
Code of the project activity's status |
subStatusCode |
string |
Code of the project activity's sub-status |
Returned Elements - projects
Element | Type | Description |
---|---|---|
brand |
string |
Name of the project's brand |
categories |
string |
Names of the project's categories (the lowest level of the selected category - may be multiples) |
masterProject |
Boolean |
If the project is a master type |
newCategoryComments |
string |
Category comments |
packaging |
string |
Packaging details |
productRangeInformation |
string |
Project’s product range details |
projectConcept |
string |
Project's business key ID |
projectId |
string |
Project's business key ID |
projectManager |
string |
Name of the project's project manager |
projectRecordId |
long |
Project record's internal ID |
projectTitle |
string |
Project's title |
projectType |
string |
Type of project |
proposedProducts |
string |
Project's proposed products |
specType |
string |
Type of project's product specification |
status |
string |
Status of the project |
subBrand |
string |
Name of the project's sub-brand |
supplier |
string |
Name of the supplier associated to the project |
site |
string |
Name of the site associated with the project |
targetConsumer |
string |
Project's target consumer details |
teamDetails/role |
string |
Name of the team role |
teamDetails/users |
string |
Name of the team users |
templateFolder |
string |
Name of the project template folder |
templateType |
string |
Type of the project's template |
templateUsed |
string |
Name of the project's template |
Error Messages
Element | Message | Meaning |
---|---|---|
fromDate |
fromDate parameter required |
From date not provided |
toDate |
toDate parameter required |
To date not provided |
fromDate |
fromDate and toDate parameters are required |
From and To dates not provided |
toDate |
From date must be before or equal to To date |
From date is after To date |
fromDate |
HTTP 417 <errorMessage> |
Not a valid date, whereby <errorMessage> will identify the issue For example: IllegalInstantException: Cannot parse "2018-03-25 01:00:00": Illegal instant due to time zone offset transition (Europe/London) In this example, due to the clocks In this example, due to the clocks moving forward (in the server's time-zone), the passed in date is invalid |
toDate |
HTTP 417 <errorMessage> |
Not a valid date, whereby <errorMessage> will identify the issue (see example above) |
Update Record
Description
Updates the sub-status of existing Artwork Project Activities. This function is used for the integration of Brand Compliance with an external Artwork Management system, to update the status of an Artwork activity from the external system.
Endpoint address: /services/rest/artwork/update HTTP method: POST
Request Details
The body of the request contains an activityUpdateRequest element consisting of the elements shown in the following table.
Request Elements
Element | Type | Description |
---|---|---|
activityName |
string |
Project activity's name |
activityRecordId |
long |
Project activity record's internal ID |
projectId |
string |
ID business key to the Project record |
projectRecordId |
long |
Project record's internal ID |
subStatusCode |
string |
Code business key to the activity's sub-status |
After the call, the Project Activity record's sub-status is updated to match the request.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 9 - Project for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing an ArtworkActivityLink element. The ArtworkActivityLink element consists of the elements shown in the following table.
Returned Elements
If successful, an HTTP 200 response is sent.
Element | Type | Description |
---|---|---|
recordId |
long |
Project activity's internal ID |
recordLink |
string |
URI to the Product Activity record, for use in a GET request |
code |
string |
Code business key to the Project Activity record |
title |
string |
Project activity's title |
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
The following conditions may prevent a successful update:
-
An invalid project record id or activity record id.
-
The activity sub-statuses not being configured in Brand Compliance (including the general sub-statuses COMPLETED, MESSAGE SENT, MESSAGE ACCEPTED, and MESSAGE FAILED).
-
The activity's template not being flagged as an artwork type, or having the permitted activity sub-statuses assigned, prior to the activity being created (a snapshot of the template settings is stored on the Activity record on creation).
BusinessCategoryService
This section describes the API for managing Business Categories. The following functions are available:
-
List of Values: retrieves a list of categories
-
Retrieve Record by ID: retrieves a Business Category record using its unique identifier
-
Check Record Modification Timestamp: retrieves the timestamp when a Business Category record was last updated
-
Create Record: creates a new Business Category record
-
Update Record: updates an existing Business Category record
-
Delete Record: deletes an existing Business Category record
List of Values
Description
Retrieves a list of categories in a paged list. Use this function to retrieve a simple list of categories and IDs, or to locate Business Category record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/businessCategory HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
code |
Optional |
string |
Yes |
POTATOES_LOOSE |
parentCode |
Optional |
string |
Yes |
POTATOES |
entityDescription |
Optional |
string |
Yes |
Loose Potatoes |
specificationType |
Optional |
string |
Yes |
FOOD~PRODUCE |
topLevelCategory |
Optional |
Boolean |
No |
TRUE |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/businessCategory/?offset=2&pageSize=20 …/services/rest/businessCategory/?code=POTATOES_LOOSE …/services/rest/businessCategory/?entityDescription=Potato%
The code parameter will attempt to find a single category with a matching code. The parentCode parameter will find any categories whose parent has the given code.
The entityDescription matches any category whose description (irrespective of language) matches the value given; this is just the description of the category in question and does not include those of parents.
The specificationType parameter is a tilde (~) separated list of types.
Note:
Including a value in the specificationType filter will exclude any categories for which no specification type has been selected.
The topLevelCategory parameter determines whether only top-level categories (those with no parent) will be included in the results.
Response Details
For a successful response, XML is returned with a BusinessCategoryLinkList root element containing an entries element for each matched category. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Business Category record's internal ID |
recordLink |
string |
URI to the BusinessCategoryService Retrieve record service for this category |
code |
string |
Code business key to the Business Category record |
entityDescription |
string |
Category's name |
path |
string |
Category's full path (including parent levels - separated by '/') |
Error Messages
Element | Message | Meaning |
---|---|---|
specificationType |
<###> is not a valid Specification Type |
Specification type not found |
pageSize |
Error Code: INVALIDPAGESIZE |
Page Size must be between 1 and 100 |
offset |
Error Code: INVALIDOFFSET |
Offset must be a positive integer |
code |
Error Code: INVALIDBUSINESSCATEGORY |
Invalid Business Category |
parentCode |
Error Code: INVALIDBUSINESSCATEGORY |
Invalid Business Category |
specificationType |
Error Code: INVALIDSPECIFICATIONTYPE |
Invalid SpecificationType |
topLevelCategory |
Error Code: INVALIDBOOLEAN |
Invalid topLevelCategory value Permitted values (not case-sensitive) are: true; yes; 1; false; no; 0 |
Retrieve Record by ID
Description
Retrieves a single Business Category record's details using the record's unique ID. Use this function to retrieve the full details of an individual category.
Endpoint address: /services/rest/businessCategory/{id} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/businessCategory/105
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a BusinessCategoryFullDTO root element containing the individual attributes of the requested Business Category record. If an ID is not specified, a list of all categories is returned (per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
Invalid record id |
Invalid {id} - not found |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Business Category record. Use this function to determine when a category's details were last updated.
Endpoint address: /services/rest/businessCategory/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/businessCategory/105
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Business Category record. For example:
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
Create Record
Description
Creates a new Business Category record. Use this function to create a new category in Brand Compliance based on data sourced from the external system.
Dependencies: If a lower-level category, the parent Business Category must be present in the application and its record ID obtained. For more information, see Dependencies.
Endpoint address: /services/rest/businessCategory HTTP method: POST
Request Details
The body of the request contains a BusinessCategoryFullDTO to specify the details of the category to create. Compared to retrieving a user (which uses the same BusinessCategoryFullDTO type), this request is much shorter. Only the attributes that are to be populated on the created Business Category record need to be included. As a minimum, this must include the fields shown in the following table:
Business Category Mandatory Fields
Field Name | Element Name |
---|---|
Code |
code |
Description |
description |
Parent Code |
parentCode |
Parent or Child level |
topLevelCategory |
Deleted Record |
deleted (Set to false) |
Path |
path (The category's full path descriptions, concatenated and separated by /) |
Note:
If creating a top level category, topLevelCategory must be set to true; if creating a lower level category, topLevelCategory must be set to false, and parentCode becomes mandatory (the parent category's code).
Example Request XML
<ns0:businessCategoryFullDTO xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full" xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple"> <ns0:code>X1</ns0:code> <ns0:localeData> <ns0:description>Category X - Level 1</ns0:description> <ns0:path>Category X - Level 1</ns0:path> </ns0:localeData> <ns0:topLevelCategory>true</ns0:topLevelCategory> <ns0:deleted>false</ns0:deleted> </ns0:businessCategoryFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
The following example adds a second level category to the above top-level parent category:
<ns0:businessCategoryFullDTO xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full" xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple"> <ns0:code>X2</ns0:code> <ns0:localeData> <ns0:description>Category X - Level 2</ns0:description> <ns0:path>Category X - Level 1 / Category X - Level 2</ns0:path> </ns0:localeData> <ns0:parentCode>X1</ns0:parentCode> <ns0:topLevelCategory>false</ns0:topLevelCategory> <ns0:deleted>false</ns0:deleted> </ns0:businessCategoryFullDTO>
The value of the code element must be unique.
The description and path elements are locale-dependent, so are contained within a localeData element, with a locale element supported language code, for example:
<ns0:localeData> <ns0:description>Cheese</ns0:description> <ns0:locale>en_GB</ns0:locale> </ns0:localeData> <ns0:localeData> <ns0:description>Fromage</ns0:description> <ns0:locale>fr</ns0:locale> </ns0:localeData>
The hierarchy of category levels is maintained through the parent code, linking a category to its immediate parent. All levels except the top level must therefore have a valid parent code specified.
The number of levels in the hierarchy is defined in the Brand Compliance Admin area.
Categories may be assigned to specific product specifications, or available for use by all; if specified, the specification type must be match those assigned to its parent categories.
Where the record is linked to another record, such as the Locale in this case, the business key must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
Response Details
For a successful response, an HTTP 200 response is sent with a body containing a BusinessCategoryLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Business Category record's internal ID |
recordLink |
string |
URI to the newly created Business Category record, for use in a GET request |
code |
string |
Code ID business key to the newly created Business Category record |
entityDescription |
string |
Category's description |
Error Responses
If the supplied data does not result in a valid Business Category (such as a missing mandatory field), an HTTP 417 response is sent with an XML body message stating the validation errors. The request should not be reattempted with the same content.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
Code {0} has already been used |
Codes must be unique |
parent |
The Parent Code cannot be blank when the Top Level Category flag is false |
A parent code must be specified unless the category is a top level category |
topLevelCategory |
The Top Level Category flag cannot be true when the Parent Code has a value |
A category cannot be a top level category and have a parent |
description |
Description must be provided |
Descriptions are mandatory |
code |
The parent Business Category with code {0} cannot be found |
The parent category must exist |
parent |
A new Business Category cannot be added to the Business Category with the code{0} because it will fall outside of the Business Category Hierarchy |
Categories can only be added within the bounds of the Business Category Hierarchy as defined in Brand Compliance's Admin/System Control/Business Category Configuration |
specificationType |
{0} is not a valid Specification Type(s) |
The specification type or types must be valid |
specificationType |
The Specification Types must be a subset of the parent Business Category's Specification Types: {0} |
This message is issued when no specification types are specified on a child, but there are specification types assigned to the parent It lists the specification types selected on the parent |
specificationType |
The Business Category Specification Types {0} are not a subset of the parent Business Category Specification Types {1} |
This message is issued when the category's specification types have not all be selected on the parent The child's specification types are listed in {0} and the parent's specification types are listed in {1} |
Update Record
Description
Updates an existing Business Category record. Use this function to update a category's details in Brand Compliance based on data sourced from the external system, or to move a category to appear beneath a different parent category.
Endpoint address: /services/rest/businessCategory/{id} HTTP method: PUT
Request Details
The body of the request contains a BusinessCategoryUpdateDTO to specify the updates to the Business Category record.
The request content is similar to that for creating a category, but does not include the child categories link. If children are to be managed, then a separate call is required for each child. As a minimum, the values specified as mandatory for the Create Record function (see above) must be included.
After the call, the Business Category record is updated to match the request.
Note:
When updating records, all values must be included. If a value or element is omitted from the request, the field contents will be cleared on the Business Category record.
The id element is used to locate the category to update.
The hierarchy of category levels is maintained through the parent code, linking a category to its immediate parent. All levels except the top level must therefore have a valid parent code specified.
The number of levels in the hierarchy is defined in the Brand Compliance Admin area.
Categories may be assigned to specific product specifications, or available for use by all; if specified, the specification type must be match those assigned to its parent categories.
Categories may be moved to the same level within another part of the hierarchy. When a category is moved, its children are automatically moved.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 1 - Framework for details of their mapping to the fields within the Brand Compliance UI.
The body of the request contains a BusinessCategoryUpdateDTO element to specify how the category should appear after the update.
Response Details
If successful, an HTTP 200 response is sent with a body containing a BusinessCategoryLink element. The BusinessCategoryLink element consists of the returned elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Business Category record's internal ID |
recordLink |
string |
URI to the Business Category record, for use in a GET request |
code |
string |
Code ID business key to the Business Category record |
entityDescription |
string |
Category's description |
path |
string |
Category's full path (including parent levels - separated by '/') |
Error Responses
If the supplied data does not result in a valid Business Category (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Error Messages
Element | Message | Meaning |
---|---|---|
recordId |
Invalid record id |
The ID used to call the PUT process does not relate to an existing record |
parent |
The Parent Code cannot be blank when the Top Level Category flag is false |
A parent code must be specified unless the category is a top level category |
topLevelCategory |
The Top Level Category flag cannot be true when the Parent Code has a value |
A category cannot be a top level category and have a parent |
code |
The parent Business Category with code {0} cannot be found |
The parent category must exist |
code |
A new Business Category cannot be added to the Business Category with the code{0} because it will fall outside of the Business Category Hierarchy |
Categories can only be added within the bounds of the Business Category Hierarchy as defined in Brand Compliance's Admin/System Control/Business Category Configuration |
parent |
Business Category cannot be moved from a parent at level {0} to a parent at level {1} |
Categories can only be moved between parent categories at the same level in the hierarchy |
specificationType |
{0} is not a valid Specification Type(s) |
The specification type(s) must be valid |
specificationType |
The Specification Types must be a subset of the parent Business Category's Specification Types: {0} |
This message is issued when no specification types are specified on a child but there are specification types assigned to the parent It lists the specification types selected on the parent |
specificationType |
The Business Category Specification Types {0} are not a subset of the parent Business Category Specification Types {1} |
This message is issued when the category's specification types have not all be selected on the parent The child's specification types are listed in {0} and the parent's specification types are listed in {1} |
Delete Record
Description
Deletes an existing Business Category record, along with any associated child categories. Use this function to remove a single category or a category and all its lower level categories.
Endpoint address: /services/rest/businessCategory/{id} HTTP method: DELETE
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to delete.
Response Details
If the Business Category is deleted successfully, an HTTP 200 response is sent.
Error Responses
If a Business Category cannot be deleted due to it being referenced by another record (or a child of the category being referenced by another record), an HTTP 417 response is sent with an ErrorMessage/Message XML body containing the message: "Could not delete: with a URI link to the category."
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Could not delete: {URI} |
Category is referenced by another record |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
Invalid record id for deletion |
Invalid {id} - not found |
AuditRestService
This section describes the API for managing audit and visits. The following functions are available:
-
List of Values (Audit): retrieves a list of audits and visits
-
Retrieve Record by ID (Audit): retrieves an Audit or Visit record using its unique identifier
-
Retrieve Record by Business Key (Audit): retrieves an Audit or Visit record using its business key
-
Check Record Modification Timestamp (Audit): retrieves the timestamp when an Audit or Visit record was last updated
-
Create Record (Audit): creates a new Audit or Visit record
-
Update Record (Audit): updates an existing Audit or Visit record
-
List of Values (Checklist): retrieves a list of checklists
-
Retrieve Record by ID (Checklist): retrieves a Checklist record using its unique identifier
-
Retrieve Record by Business Key (Checklist): retrieves a Checklist record using its business key
-
Create Record (Checklist): creates a new Checklist record
-
Update Record (Checklist): updates an existing Checklist record
-
Delete Record (Checklist): deletes an existing Checklist record
List of Values (Audit)
Description
Retrieves a list of Audits or Visits in a paged list. Use this function to locate Audit or Visit record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/audit HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
status |
Optional |
string |
Yes |
Scheduled~In Progress |
code |
Optional |
string |
No |
2001 |
Example URL
…/services/rest/audit/?offset=2&pageSize=20
Response Details
For a successful response, XML is returned with an AuditVisitLinkList root element containing an entries element for each matched audit or visit. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Audit/Visit record's internal ID |
recordLink |
string |
URI to the AuditRestService Retrieve record service for this audit/visit |
code |
string |
Audit/Visit's code business key |
dueDate |
string |
Audit/Visit's due date |
siteCode |
string |
Audit/Visit's site code |
supplierCode |
string |
Audit/Visit's supplier code |
templateCode |
string |
Audit/Visit's template code |
Error Messages
Element | Message | Meaning |
---|---|---|
pageSize |
Error Code: INVALIDPAGESIZE |
Page Size must be between 1 and 100 |
Retrieve Record by ID (Audit)
Description
Retrieves a single Audit or Visit record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual audit or visit.
Endpoint address: /services/rest/audit/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/audit/105
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of an AuditFullDTO root element containing the individual attributes of the requested Audit or Visit record. If an ID is not specified, a list of all audits/visits is returned (per the List of Values function).
File Structure:
The main elements of the auditFullDTO root element:
-
auditTemplateSnapshot and Template - the settings from the associated template.
-
nonConformances - If the audit/visit has any non conformances/issues raised against it, they appear within a nonConformances element - a separate element for each.
-
supplier and site - details of the supplier and site that the audit/visit is related to.
-
udfData - the contents of any user-defined custom fields associated to the Audit/Visit record. nonConformances may also contain udfData elements, if the Non Conformance record has user-defined custom fields configured.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Audit to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key (Audit)
Description
Retrieves a single Audit or Visit record's details using its business key (code). Use this function to retrieve the full details of an individual audit or visit using its key code.
Endpoint address: /services/rest/audit/byKey/{code} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {code} parameter that determines the record to retrieve.
Example URL
…/services/rest/audit/byKey/110
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return an AuditFullDTO Element Message containing the individual attributes of the requested Audit or Visit record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a AuditFullDTO root element containing the individual attributes of the requested Audit or Visit record.
File Structure:
The main elements of the auditFullDTO root element:
-
auditTemplateSnapshot and Template - the settings from the associated template.
-
nonConformances - If the audit/visit has any non conformances/issues raised against it, they appear within a nonConformances element - a separate element for each.
-
supplier and site - details of the supplier and site that the audit/visit is related to.
-
udfData - the contents of any user-defined custom fields associated to the Audit/Visit record. nonConformances may also contain udfData elements, if the Non Conformance record has user-defined custom fields configured.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
HTTP 404 Not Found |
Invalid {code} - blank or not found |
code |
HTTP 404 Not Found |
Invalid {code} - not found |
Check Record Modification Timestamp (Audit)
Description
Retrieves the last modification date and time of an Audit or Visit record. Use this function to determine when the audit or visit was last updated.
Endpoint address: /services/rest/audit/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/audit/105
Response
If successful, an HTTP 200 response is sent containing the Last-Modified header to show the last modification date and time of the last update of the requested Audit or Visit record.
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
Create Record (Audit)
Description
Creates a new Audit or Visit record. Use this function to create a new audit or visit in Brand Compliance based on data sourced from the external system.
Dependencies: The Supplier and Site must be present in the application and its record ID obtained. If assigning a Business Category, the ID of the Business Category record must be present in the application and its record ID obtained. If assigning a Product Technologist or People Present, the users must be present in the application and the record IDs of the User record obtained. If non conformances/issues are to be included, and they are associated to a specific user of the application, the record IDs of the User record must be obtained. An audit/visit must be associated to an audit/visit template. For more information, see Dependencies.
Endpoint address: /services/rest/audit HTTP method: POST
Request Details
The body of the request contains an AuditFullDTO element to specify the detail of the audit or visit to create, which is based on the AuditFullDTO element returned when retrieving an audit/visit. As a minimum, the fields shown in the following table must be populated.
Audit/Visit Mandatory Fields
Field Name | Element Name |
---|---|
Audit/Visit Template |
template / id (or code) auditTemplateSnapshot |
Audit/Visit Code |
code |
Lead Technologist |
leadTechnicalManager / code |
Supplier |
supplier / id |
Site |
site / id |
Due Date |
dueDate |
Record Type |
recordType |
Status |
status / id |
From Date |
fromDate |
To Date |
toDate |
People Present |
peoplePresent / name |
Deleted Record |
deleted (Set to false) |
Note:
If the status is Scheduled, fromDate, toDate, and peoplePresent are not mandatory.
To ensure a valid unique business key code is automatically assigned to the record being created, a value of zero must be passed in the <code> element of the XML when submitting the POST request.
People Present Table:
This table is used to identify the people present during the audit or visit. The options for completing the table depend on the settings in the audit/visit's template:
-
The table must contain at least one entry (whether an audit or a visit).
-
If a visit or an internal audit, the entry should be a user of the system who has a Technologist role and is set as an approved auditor. The template may have been set to just allow specific auditors.
-
If a third-party audit, the entry should be a Certification Body. The template may have been set to just allow specific auditors.
-
Rows of free text may be added to the table.
File Structure:
The main elements of the AuditFullDTO root element are shown in the following table:
Summary of AuditFullDTO Elements
Element Name | Description |
---|---|
announced costChargedToSupplier auditTemplateSnapshot |
Values from the audit/visit template, required to control the record's behavior when it is progressed within Brand Compliance. |
code |
The audit/visit's business key. |
dueDate fromDate toDate leadTechnical Manager recordType |
Values that may be mandatory for the audit/visit to be created. |
nonConformances |
Container for the non conformance data - a separate element for each non conformance/issue. |
supplier |
ID of the supplier that the audit/visit is associated to. |
site |
ID of the site that the audit/visit is associated to. |
status |
The audit/visit's status. |
template |
ID of the audit/visit template that the audit/visit is associated to. |
Example Request XML
This example shows the minimum requirement to be able to create an In Progress audit or visit.
<ns0:auditFullDTO xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full" xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple"> <ns0:announced>true</ns0:announced> <ns0:costChargedToSupplier>false</ns0:costChargedToSupplier> <ns0:auditTemplateSnapshot> <ns0:allowWorkingOffline>false</ns0:allowWorkingOffline> <ns0:allowableScoreOptions> <ns1:code>AMBER</ns1:code> </ns0:allowableScoreOptions> <ns0:allowableScoreOptions> <ns1:code>GREEN</ns1:code> </ns0:allowableScoreOptions> <ns0:auditType>INTERNAL</ns0:auditType> <ns0:auditVisibility>OPTIONAL</ns0:auditVisibility> <ns0:autoSchedule>false</ns0:autoSchedule> <ns0:buyerInformationRequired>true</ns0:buyerInformationRequired> <ns0:categoriesRequired>2</ns0:categoriesRequired> <ns0:categoryRequired>false</ns0:categoryRequired> <ns0:certificationBodiesSelectable>ALL</ns0:certificationBodiesSelectable> <ns0:closureDeadline>56</ns0:closureDeadline> <ns0:closureDeadlineNoOfDays>0</ns0:closureDeadlineNoOfDays> <ns0:commentsNotVisibleToOrganisationRequired>true </ns0:commentsNotVisibleToOrganisationRequired> <ns0:costChargedToSupplier>false</ns0:costChargedToSupplier> <ns0:defaultFrequency>12</ns0:defaultFrequency> <ns0:defaultIssueCompletion>28</ns0:defaultIssueCompletion> <ns0:extendedDeadline>56</ns0:extendedDeadline> <ns0:extendedDeadlineNoOfDays>0</ns0:extendedDeadlineNoOfDays> <ns0:internalAuditorsSelectable>ALL</ns0:internalAuditorsSelectable> <ns0:isActive>true</ns0:isActive> <ns0:localeData> <ns0:name>Checklist Audit</ns0:name> <ns0:id>49</ns0:id> </ns0:localeData> <ns0:mayExternalManagersEditConfig>MAY_NOT_EDIT </ns0:mayExternalManagersEditConfig> <ns0:nonConformanceCreationMethod>VIA_CHECKLISTS </ns0:nonConformanceCreationMethod> <ns0:organisationType>SITE</ns0:organisationType> <ns0:recordType>AUDIT</ns0:recordType> <ns0:scoreAudit>true</ns0:scoreAudit> <ns0:scoreRequired>true</ns0:scoreRequired> <ns0:stampOrgWithLastAuditDate>true</ns0:stampOrgWithLastAuditDate> <ns0:supplierGenerated>false</ns0:supplierGenerated> <ns0:useAuditVisitStandards>false</ns0:useAuditVisitStandards> <ns0:id>6</ns0:id> </ns0:auditTemplateSnapshot> <ns0:dueDate>2017-04-27</ns0:dueDate> <ns0:fromDate>2017-04-27</ns0:fromDate> <ns0:leadTechnicalManager> <ns1:code>PROD-TECH</ns1:code> <ns1:disabled>false</ns1:disabled> <ns1:externalAuthenticationUser>false</ns1:externalAuthenticationUser> <ns1:timeZone>Europe/London</ns1:timeZone> <ns1:userType>RETAILER</ns1:userType> <ns1:id>12</ns1:id> </ns0:leadTechnicalManager> <ns0:nonConformances> <ns0:description><p>Test</p></ns0:description> <ns0:reference>AR01</ns0:reference> <ns0:status> <ns1:status>OPEN</ns1:status> </ns0:status> <ns0:type> <ns1:code>MAJOR</ns1:code> </ns0:type> <ns0:code>AR01-11</ns0:code> </ns0:nonConformances> <ns0:recordType>AUDIT</ns0:recordType> <ns0:site> <ns1:id>27</ns1:id> </ns0:site> <ns0:status> <ns1:status>IN PROGRESS</ns1:status> </ns0:status> <ns0:supplier> <ns1:id>21</ns1:id> </ns0:supplier> <ns0:template> <ns1:id>8</ns1:id> </ns0:template> <ns0:toDate>2017-04-27</ns0:toDate> <ns0:code>AR1</ns0:code> <ns0:deleted>false</ns0:deleted> </ns0:auditFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
Where the record is linked to another record, such as the Template, the business key or ID must be provided (not the description) in order to form the link between the records. In general, the business key will be the code attribute of the linked record. The supplier and site elements can contain just the ID of the respective record links.
A unique code for the audit/visit must be assigned.
Templates:
The audit/visit is linked to its template using the template and auditTemplateSnapshot elements. The template element can contain just the code or ID of the associated Template record.
The auditTemplateSnapshot element must be completed to provide the parameters that control the audit/visits' behavior as it progresses through its Brand Compliance workflow. If scoring is used, the allowable options can be specified using the code or ID of the glossary record; if none are specified, all options will be available when the user selects the score.
Non Conformances:
If the audit/visit is to be created with non conformances/issues already raised against it, they can be added within a nonConformances element - a separate element for each. A unique code must be assigned to each. The status can be set using its code or ID.
For further information about the process for creating audit, non conformances and checklists, see Dependencies.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing a AuditVisitLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Newly created Audit/Visit record's internal ID |
recordLink |
string |
URI to the newly created Audit/Visit record, for use in a GET request |
code |
string |
Audit/Visit's code business key |
dueDate |
string |
Audit/Visit's due date |
siteCode |
string |
Audit/Visit's site code |
supplierCode |
string |
Audit/Visit's supplier code |
templateCode |
string |
Audit/Visit's template code |
Error Responses
If the supplied data does not result in a valid Audit or Visit (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Error Messages
Element | Message | Meaning |
---|---|---|
peoplePresent |
WARNING: class com.micros.creations.core.domain.Audit.peoplePresent - There must be at least one entry in the People Present table |
People Present not provided |
recordType |
IllegalArgumentException: No enum constant com.micros.creations.core.type.AuditRecordType. <###> |
Record Type not found |
supplier/id code site/id code supplierId supplierCode |
HTTP 417 IllegalStateException: Cannot locate keyword for class com.micros.creations.core.domain.Site using id: <###>, code:<###>, supplierCode:<###> and supplierId:<###> |
Site Id not found Site Code not found Supplier Id not found Supplier Code not found |
template |
EntityNotFoundException: Unable to find com.micros.creations.core.domain.AuditTemplate with id <###> |
Template not found |
fromDate |
ERROR: class com.micros.creations.core.domain.Audit.fromDate - The condition is invalid |
From Date not provided |
code |
ERROR: class com.micros.creations.core.domain.Audit.code - The condition is invalid |
Audit/Visit code not provided |
code |
ERROR: class com.micros.creations.core.domain.Audit.code - Another object exists with this value |
Audit/Visit code already exists |
Update Record (Audit)
Description
Updates an existing Audit or Visit record. Use this function to update an audit or visit's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/audit/{id} HTTP method: PUT
Request Details
The body of the request contains an AuditFullDTO element to specify the updates to the Audit or Visit record, which is based on the AuditFullDTO type returned when retrieving an audit/visit.
Note:
The request content is similar to that for creating an audit or visit.
As a minimum, the values specified as mandatory for the Create Record function (see above) must be included. However, when updating records, all values must be included.
If a value or element is omitted from the request, the field contents will be cleared on the Audit/Visit record.
If the audit/visit has any non conformances/issues raised against it, they appear within a nonConformances element - a separate element for each. The non conformances/issues can be updated or created when updating the audit/visit. If creating a new Non Conformance record, assign a unique code and omit the id element.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing an AuditVisitLink element. The AuditVisitLink element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Audit/Visit record's internal ID |
recordLink |
string |
URI to the Audit/Visit record, for use in a GET request |
code |
string |
Audit/Visit's code business key |
dueDate |
string |
Audit/Visit's due date |
siteCode |
string |
Audit/Visit's site code |
supplierCode |
string |
Audit/Visit's supplier code |
templateCode |
string |
Audit/Visit's template code |
Error Responses
If the supplied data does not result in a valid Audit or Visit (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
List of Values (Checklist)
Description
Retrieves a list of Checklists in a paged list. Use this function to locate Checklist record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/audit/{auditId}/checklist HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
auditId |
Optional |
long |
No |
105 |
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
Example URL
…/services/rest/audit/105/checklist/?offset=2&pageSize=20
Response Details
For a successful response, XML is returned with an AuditChecklistLinkList root element containing an entries element for each matched audit or visit. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Checklist record's internal ID |
recordLink |
string |
URI to the AuditRestService Retrieve record service for this checklist |
parentId |
string |
The parent Audit/Visit's internal ID |
auditCode |
string |
The parent Audit/Visit's code business key |
globalId |
string |
Checklist's code business key |
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
Retrieve Record by ID (Checklist)
Description
Retrieves a single Checklist record's details using the record's internal unique ID (which is not visible in the UI) and its parent audit/visit's internal unique Id. Use this function to retrieve the full details of an individual audit or visit.
Endpoint address: /services/rest/audit/{auditId}/checklist/{id} HTTP method: GET
Request Details
The URL contains the {auditId} parameter that identifies the parent Audit/Visit record, and the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/audit/105/checklist/7
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of an auditChecklistFullDTO root element containing the individual attributes of the requested Checklist record. If a Checklist ID is not specified, a list of all the audit/visit's checklists is returned (as per the List of Values function).
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
auditId |
HTTP 404 Not found |
Invalid {auditId} - not numeric |
auditId |
HTTP 417 IllegalStateException: Cannot find the Audit to return with id:<###> |
Invalid {auditId} - not found |
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Audit to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key (Checklist)
Description
Retrieves a single Checklist record's details using its business key (code) and its parent audit/visit's code. Use this function to retrieve the full details of an individual checklist using its key code.
Endpoint address: /services/rest/audit/byKey/{auditCode}/checklist/{checklistCode} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {auditCode} parameter that identifies the parent Audit/Visit record, and the {checklistCode} parameter that determines the record to retrieve.
Example URL
…/services/rest/audit/byKey/110/checklist/7374be1e-2a6c-11e7-8fda-0021f60dd918
Response Details
If successful, an HTTP 301 redirect response is sent containing a header Location with the URI of the document requested.
The returned URI represents the call to be made to the Retrieve Record by ID function (with the id substituted with the relevant value), which will return an auditChecklistFullDTO root element containing the individual attributes of the requested Checklist record.
Note:
If your method of calling the API is configured to automatically follow HTTP 301 redirects, the call may automatically return a auditChecklistFullDTO root element containing the individual attributes of the requested Checklist record.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
auditCode |
HTTP 404 Not Found |
Invalid {auditCode} - blank or not found |
checklistCode |
HTTP 404 Not Found |
Invalid {checklistCode} - blank or not found |
Create Record (Checklist)
Description
Creates a new Checklist record. Use this function to create a new checklist in Brand Compliance based on data sourced from the external system.
Dependencies: To create an audit with a checklist, the parent Audit record must be created using the Create Record (Audit/Visit) function, before creating the associated Checklist records.
Therefore, when creating the checklist, the parent Audit/Visit record must be present in the application and its record ID obtained. A checklist's questions and the permitted answers are determined by the checklist template that has been assigned to the audit/visit's template. The IDs of the Checklist Template record must also be provided when creating a template. For more information, see Dependencies.
Endpoint address: /services/rest/audit{auditId}/checklist HTTP method: POST
Request Details
The body of the request contains an auditChecklistFullDTO element to specify the detail of the checklist to create, which is based on the auditChecklistFullDTO element returned when retrieving a checklist. As a minimum, the fields shown in the following table must be populated.
Checklist Mandatory Fields
Field Name | Element Name |
---|---|
Audit/Visit Id |
audit / id See note 1. |
Audit/Visit Code |
audit /code See note 1. |
Checklist Template |
localeData / id |
Checklist Comments Allowed |
commentsAllowed |
Questionnaire Questions |
questionnaire / questionnaireQuestion / questionDetails |
Question Answers |
questionnaire / questionnaireQuestion / questionnaireAnswer questionnaire / questionnaireQuestion / quesAvailAnsFull |
Deleted Record |
deleted (Set to false) |
Notes:
-
Either code or ID may be specified to identify the audit/visit.
File Structure:
The following diagram shows the structure of the auditChecklistFullDTO request file structure:
Figure 4-3 RESTful Checklist Request
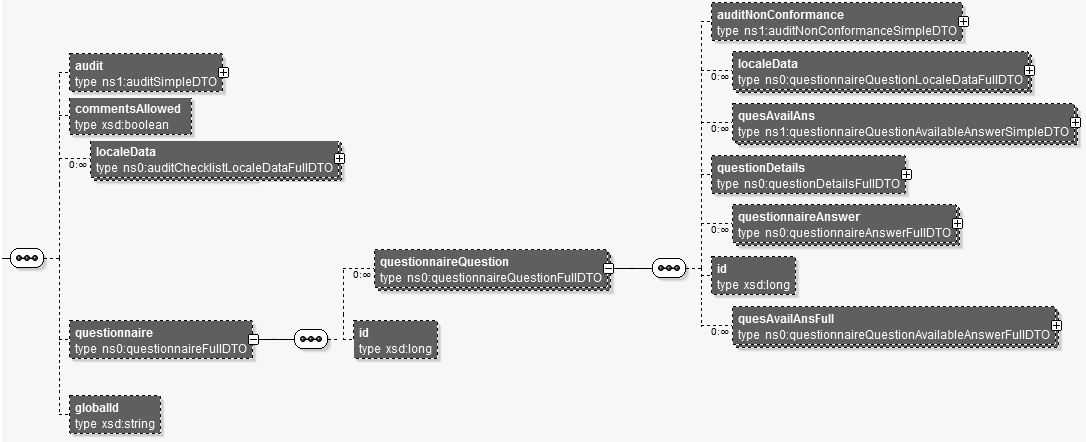
Summary of AuditChecklistFullDTO Elements
Element Name | Description |
---|---|
audit |
Identifies the parent audit record by code or id. |
commentsAllowed |
A value from the checklist template, required to control the record's behavior when it is progressed within Brand Compliance. |
localeName |
Identifies the checklist template by name and id. |
questionnaire |
Groups the questions and their answers. |
questionnaireQuestion |
Groups the checklist questions and their answers - repeated for each question in the checklist. reference is the question reference. |
questionDetails |
Individual questions. question is the question text. answerComment is the answer's comments (if configured). answerFieldType is code of answer type (MULTI_SELECTION, TEXT, or SINGLE_SELECTION). commentAllowed is whether comments are allowed. |
questionnaireAnswer |
Groups the answer details. |
questionnaireAvailableAnswer |
Answers for the individual questions. code is the question's unique key. commentMandatory is whether comments are mandatory or not. generateNonConformance is whether an answer will generate an issue. |
quesAvailAnsFull |
The available answers - repeated for each answer. code is the question's unique key. commentMandatory is whether comments are mandatory or not. id and keywordTypeEnum are the keys to the Issues glossary. |
Process for Creating Checklist Audits
The general process for creating checklist audits:
-
Create the Audit/Visit. While it is possible to create a checklist audit using the Create Audit function, it is recommended that they be created within the Brand Compliance application.
A method of creating a new audit:
-
Retrieve an existing audit using a GET function.
-
Omit the id and statusChangeHistory elements from the retrieved file.
-
Omit localData elements from status and allowableAuditStandards.
-
Assign a new unique code.
-
Link to the Supplier, Site, and Audit Template records using the relevant IDs.
-
Change other content to reflect the new audit.
-
Submit using the POST function.
-
If non conformances are to be created at the time of creating the audit/visit, include them as nonConformances elements.
Note:
The API does not automatically create a non conformance based on the answer of a checklist question. Non conformances must be assigned when either creating or updating the Audit/Visit record.
-
-
Retrieve the code or ID of the newly created Audit, and its template detail using a GET function.
-
Create the checklist using the POST function (repeat for each checklist):
-
Identify the parent audit/visit by setting the code or id within audit element.
-
Can retrieve an existing checklist using a GET function, in which case the id and questionnaireQuestionAvailableAnswer elements must be omitted. Also omit any id elements within the questionnaire element, except those that relate to the auditNonConformance element.
-
Example Request XML
This example shows the minimum requirement to be able to create an audit checklist. This example contains three questions, each with the available answers of Yes, No, or Not Applicable.
Figure 4-4 Checklist Questions
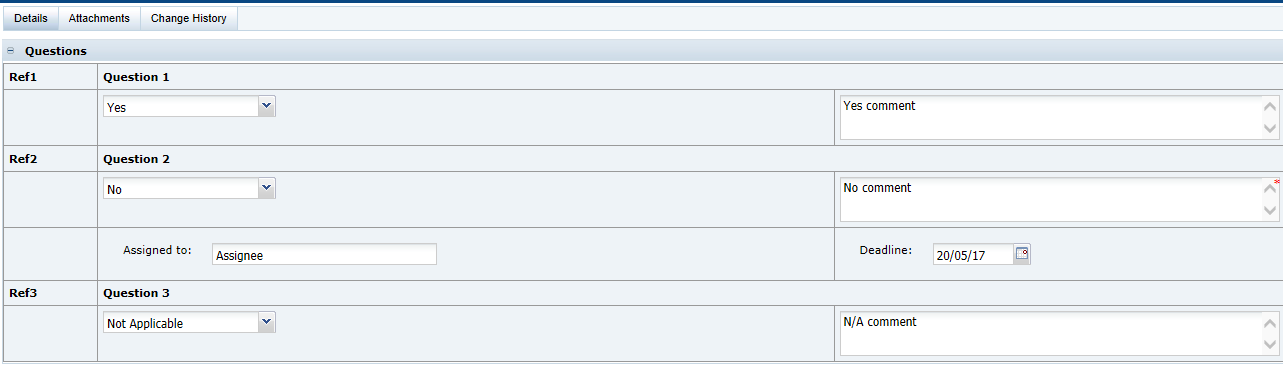
<ns0:auditChecklistFullDTO xmlns:ns1="http://www.micros.com/creations/core/domain/dto/v1p0/simple" xmlns:ns0="http://www.micros.com/creations/core/domain/dto/v1p0/full"> <ns0:audit> <ns1:code>AUD09</ns1:code> <ns1:id>132</ns1:id> </ns0:audit> <ns0:commentsAllowed>false</ns0:commentsAllowed> <ns0:localeData> <ns0:name> <![CDATA[ API Test Template ]]> </ns0:name> <ns0:id>563</ns0:id> </ns0:localeData> <ns0:questionnaire> <ns0:questionnaireQuestion> <ns0:localeData> <ns0:reference> <![CDATA[Ref1]]> </ns0:reference> </ns0:localeData> <ns0:questionDetails> <ns0:answerComment> <![CDATA[Yes comment]]> </ns0:answerComment> <ns0:answerFieldType>SINGLE_SELECTION</ns0:answerFieldType> <ns0:commentAllowed>true</ns0:commentAllowed> <ns0:localeData> <ns0:question> <![CDATA[Question 1]]> </ns0:question> </ns0:localeData> </ns0:questionDetails> <ns0:questionnaireAnswer> <ns0:questionnaireAvailableAnswer> <ns1:code>QQAA7236</ns1:code> <ns1:commentMandatory>false</ns1:commentMandatory> <ns1:generateNonConformance>false </ns1:generateNonConformance> <ns1:localeData> <ns0:answer>Yes</ns0:answer> </ns1:localeData> </ns0:questionnaireAvailableAnswer> <ns0:questionnaireQuestion> <ns1:localeData> <ns0:reference> <![CDATA[Ref1]]> </ns0:reference> </ns1:localeData> </ns0:questionnaireQuestion> </ns0:questionnaireAnswer> <ns0:quesAvailAnsFull> <ns0:code>QQAA7236</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Yes</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7237</ns0:code> <ns0:commentMandatory>true</ns0:commentMandatory> <ns0:generateNonConformance>true</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>No</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7238</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Not Applicable</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> </ns0:questionnaireQuestion> <ns0:questionnaireQuestion> <ns0:localeData> <ns0:reference> <![CDATA[Ref2]]> </ns0:reference> </ns0:localeData> <ns0:questionDetails> <ns0:answerComment> <![CDATA[No comment]]> </ns0:answerComment> <ns0:answerFieldType>SINGLE_SELECTION</ns0:answerFieldType> <ns0:commentAllowed>true</ns0:commentAllowed> <ns0:localeData> <ns0:question> <![CDATA[Question 2]]> </ns0:question> </ns0:localeData> </ns0:questionDetails> <ns0:questionnaireAnswer> <ns0:questionnaireAvailableAnswer> <ns1:code>QQAA7240</ns1:code> <ns1:commentMandatory>true</ns1:commentMandatory> <ns1:generateNonConformance>true</ns1:generateNonConformance> <ns1:localeData> <ns0:answer>No</ns0:answer> </ns1:localeData> </ns0:questionnaireAvailableAnswer> <ns0:questionnaireQuestion> <ns1:localeData> <ns0:reference> <![CDATA[Ref2]]> </ns0:reference> </ns1:localeData> </ns0:questionnaireQuestion> </ns0:questionnaireAnswer> <ns0:quesAvailAnsFull> <ns0:code>QQAA7239</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Yes</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7240</ns0:code> <ns0:commentMandatory>true</ns0:commentMandatory> <ns0:generateNonConformance>true</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>No</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7241</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Not Applicable</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> </ns0:questionnaireQuestion> <ns0:questionnaireQuestion> <ns0:localeData> <ns0:reference> <![CDATA[Ref3]]> </ns0:reference> </ns0:localeData> <ns0:questionDetails> <ns0:answerComment> <![CDATA[N/A comment]]> </ns0:answerComment> <ns0:answerFieldType>SINGLE_SELECTION</ns0:answerFieldType> <ns0:commentAllowed>true</ns0:commentAllowed> <ns0:localeData> <ns0:question> <![CDATA[Question 3]]> </ns0:question> </ns0:localeData> </ns0:questionDetails> <ns0:questionnaireAnswer> <ns0:questionnaireAvailableAnswer> <ns1:code>QQAA7244</ns1:code> <ns1:commentMandatory>false</ns1:commentMandatory> <ns1:generateNonConformance>false</ns1:generateNonConformance> <ns1:localeData> <ns0:answer>Not Applicable</ns0:answer> </ns1:localeData> </ns0:questionnaireAvailableAnswer> <ns0:questionnaireQuestion> <ns1:localeData> <ns0:reference> <![CDATA[Ref3]]> </ns0:reference> </ns1:localeData> </ns0:questionnaireQuestion> </ns0:questionnaireAnswer> <ns0:quesAvailAnsFull> <ns0:code>QQAA7242</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Yes</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7243</ns0:code> <ns0:commentMandatory>true</ns0:commentMandatory> <ns0:generateNonConformance>true</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>No</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> <ns0:quesAvailAnsFull> <ns0:code>QQAA7244</ns0:code> <ns0:commentMandatory>false</ns0:commentMandatory> <ns0:generateNonConformance>false</ns0:generateNonConformance> <ns0:localeData> <ns0:answer>Not Applicable</ns0:answer> </ns0:localeData> </ns0:quesAvailAnsFull> </ns0:questionnaireQuestion> </ns0:questionnaire> <ns0:globalId>7470e250-36cc-4dea-a0f1-eb6040185865</ns0:globalId> <ns0:deleted>false</ns0:deleted> </ns0:auditChecklistFullDTO>
Set the deleted attribute to false to ensure the record can be seen in the UI. If omitted or set to true, it will exist, but will not be visible.
For further information about the process for creating audit, non conformances, and checklists, see Dependencies.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing an AuditChecklistLink root element. The root element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Checklist record's internal ID |
recordLink |
string |
URI to the newly created Checklist record, for use in a GET request |
parentId |
long |
The parent Audit/Visit's internal ID |
auditCode |
string |
The parent Audit/Visit's code business key |
globalId |
string |
Checklist's code business key |
Error Responses
If the supplied data does not result in a valid Checklist (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Error Messages
Element | Message | Meaning |
---|---|---|
audit / id audit / code |
HTTP 417 IllegalStateException: The checklists audit cannot be located |
Audit/Visit id/code not found |
id |
HTTP 417 IllegalStateException: Cannot find matching relation to update on <###> looking for <###> |
Invalid link to related record |
Update Record (Checklist)
Description
Updates an existing Checklist record. Use this function to update a checklist's details in Brand Compliance based on data sourced from the external system.
Endpoint address: /services/rest/audit/{auditId}/checklist/{id} HTTP method: PUT
Request Details
The body of the request contains an auditChecklistFullDTO element to specify the updates to the Audit or Visit record, which is based on the auditChecklistFullDTO type which is returned when retrieving an audit/visit.
Note:
The request content is similar to that for creating a checklist.
As a minimum, the values specified as mandatory for the Create Record function (see above) must be included. However, when updating records, all values must be included.
If a value or element is omitted from the request, the field contents will be cleared on the Checklist record.
The link to the parent Audit/Visit record is defined by the code or ID within the audit element.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Response Details
If successful, an HTTP 200 response is sent with a body containing an auditChecklistLink element. The auditChecklistLink element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Checklist record's internal ID |
recordLink |
string |
URI to the Checklist record, for use in a GET request |
parentId |
long |
The parent Audit/Visit's internal ID |
auditCode |
string |
The parent Audit/Visit's code business key |
globalId |
string |
Checklist's code business key |
Error Messages
If the supplied data does not result in a valid Audit or Visit (such as a missing mandatory field), an HTTP 417 response is sent with an ErrorMessage/Message XML body message stating the validation errors. The request should not be reattempted with the same content.
Delete Record (Checklist)
Deletes an existing Checklist record. Use this function to delete a checklist that has been created in error from the external system
Endpoint address: /services/rest/audit/{auditId}/checklist/{id} HTTP method: DELETE
Request Details
There are no request parameters, but the URL contains the {auditId} parameter that identifies the parent Audit/Visit record, and the {id} parameter that determines the record to delete.
Note:
It is not possible to manually delete checklists from an audit/visit within the application; this delete function has been provided solely for the deletion of checklists that have been created in error by the API.
To delete a checklist, the external system must be granted access to the AUDIT_CHECKLIST_DELETE endpoint.
Example URL
…/services/rest/audit/105/checklist/7
Response Details
If successful, an HTTP 200 response is sent.
Error Messages
Element | Message | Meaning |
---|---|---|
auditId |
HTTP 404 Not Found |
Invalid {auditId} - not numeric |
auditId |
HTTP 417 IllegalStateException: Cannot find the Audit to return with id:<###> |
Invalid {auditId} - not found |
id |
HTTP 404 Not Found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Audit Checklist to return with id:<###> |
Invalid {id} - not found |
DataPrivacyService
This section describes the API for executing Data Privacy requests. The following functions are available:
-
Right to Access: retrieves personal data from the system relating to the name of an individual
-
Right to be Forgotten: erases personal data from the system for an individual
Although minimal personal data is held in Brand Compliance, it does include the names and contact details of retailer/portal owner, supplier, and third-party users. The Data Privacy API provides a means for the retailer/portal owner to:
-
Request details of personal data relating to an individual be held within the system.
-
Request that the personal data relating to the individual be removed from the system.
For additional detail on using the Data Privacy API, including examples, see Appendix: Using the Data Privacy API.
Right to Access
Description
Retrieves a set of personal data that relates to the name of an individual. Use this function to perform Right to Access requests for the following scenarios:
-
An employee of the retailer/portal owner requests an electronic copy of their personal data that is held within the system.
-
An employee of one of the retailer/portal owner's suppliers requests an electronic copy of their personal data that is held within the system.
-
An employee of the retailer/portal owner's partner organizations requests an electronic copy of their personal data that is held within the system.
The API's access function allows the name of the individual to be passed, with an XML message being returned containing any personal data found for that name. The XML message is a structured text format, which is both machine and human readable.
Figure 4-5 Right to Access Process
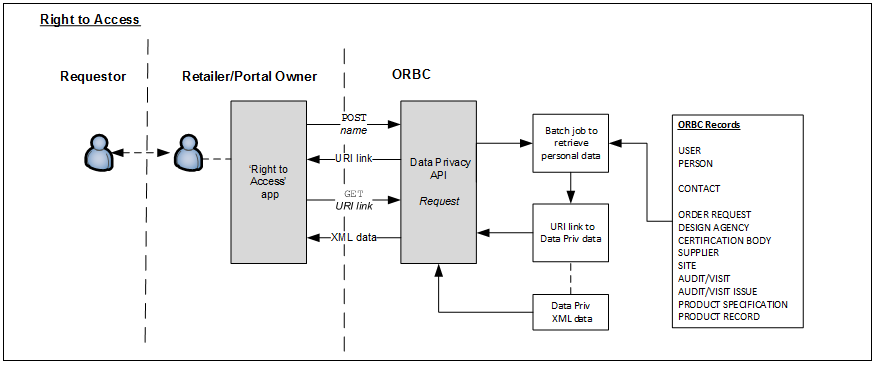
The steps for completing a Right to Access request are as follows (for further details, see Appendix: Using the Data Privacy API):
-
Submit a POST call, passing the name of the individual.
A batch job is submitted within Brand Compliance to search the system for personal data for the presence of the name (for which records and fields are searched, see Appendix: Using the Data Privacy API). On completion, a Data Privacy record containing the retrieved personal data is created within Brand Compliance.
A link to the Data Privacy record is returned as the response to the POST call.
-
On receipt of the response to the POST call, submit a GET call, passing the returned URI link.
Note:
If the GET call is submitted before the batch job has finished compiling the personal data, just a header will be returned, and the GET call should be retried.
A message containing the personal data is returned. The XML data is structured within a dataprivRequestDTO root element (for details of the message structure, see Appendix: Using the Data Privacy API).
Just the personal data fields and values to identify the record are returned, not the full record contents.
Addresses may contain multiple localeData elements for the language translation of countries.
XML elements will only be present if they contain data.
-
Assess whether the returned data relates to the individual who made the request, editing and/or reformatting it if necessary, before passing it to the requestor.
Endpoint address: /services/rest/datapriv/access HTTP methods: POST, GET
Request Details
For the POST method, a name parameter is passed in the payload.
For the GET method, an id parameter is passed as a URI parameter.
URI Parameters
Example URL (POST method): …/services/rest/access/
Example URL (GET method): …/services/rest/access/?id=16
Response Details
For a successful response to the POST method, a link to the Data Privacy record is returned.
For a successful response to the GET method, XML is returned with a dataprivRequestDTO root element containing an element for each record where the personal data has been found.
Error Responses
In the event that an error occurs, an HTTP 500 response is sent.
Right to be Forgotten
Description
Erases a set of personal data that relates to an individual. Use this function to perform Right to be Forgotten requests for the following scenarios:
-
An employee of the retailer/portal owner requests their personal data be erased from the system.
-
An employee of one of the retailer/portal owner's suppliers requests their personal data be erased from the system.
-
An employee of a partner organizations requests their personal data be erased from the system.
-
The retailer/portal owner initiates the purging of an inactive user's personal data from the system.
An erase function allows the XML message returned from a Right to Access request to be used to erase the data.
Figure 4-6 Right to be Forgotten Process
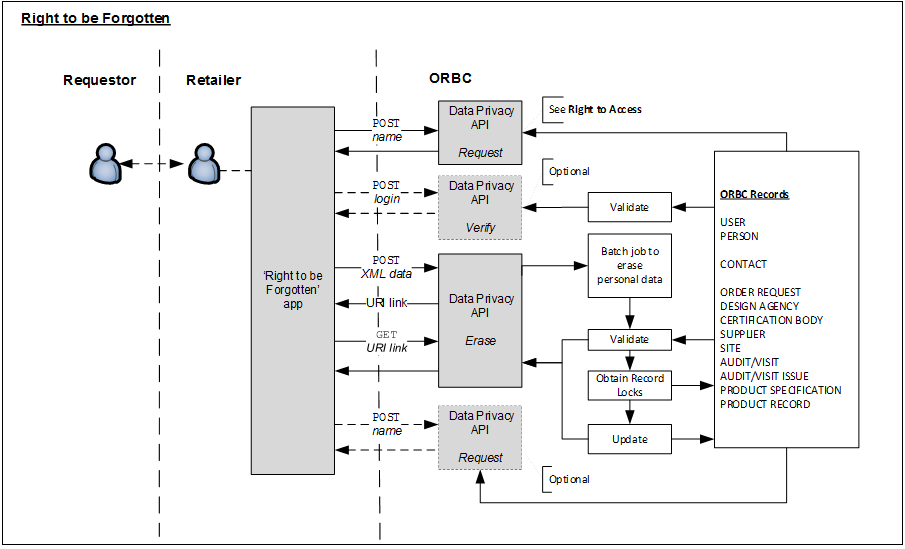
The steps for completing a Right to be Forgotten request are as follows:
-
The Right to Access service must be used to retrieve the personal data for the individual. For details, see Right to Access
. -
View, and if necessary, edit the XML returned from the Right to Access request.
As there may be any number of users with the same name, it is necessary to decide which data represents the individual who made the request, removing any that is not relevant (for further information on editing the XML message, see Appendix: Using the Data Privacy API).
-
Optionally submit a POST call to the verify function, passing the login id of the individual (obtained from the XML message returned in Step 2).
This step is used to check whether the erasure of data for the individual will be permitted, before actually submitting the request. The erase will not be permitted if the individual is responsible for the completion of a supplier site's Scorecard.
XML is returned with a dataprivVerifyDTO root element containing a status and a message element. If the call is successful the message will contain Success, otherwise it will contain Failure and the reason for the failure will be present in the message element.
-
Submit a POST call to the erase function, passing the XML retrieved from the Right to Access request (with any edits having been applied).
A batch job is submitted to validate the request, verify that the erasure is permitted (that is, the individual is not responsible for the completion of a Scorecard), and obtain locks on the records to be updated.
-
If the validation fails, or a lock cannot be obtained, the process is terminated and an XML is returned with a dataprivErasureDTO root element containing a message element that contains the reason for the failure, and the status element set to Failure.
If no exceptions are encountered, a Data Privacy record containing the personal data to erase is created and a link to the record is returned as the response to the POST call.
-
On receipt of the response to the POST call, submit a GET call to the erase function, using the returned URI link.
The update processing replaces the data passed in the XML message with 30 characters of randomly-generated text. Email addresses are changed to x@example.com, where x is 15 characters of randomly-generated text*. GPS coordinate numbers and country selections are blanked.
Some fields have a specific length, in which case the 30 characters of obfuscated text is overridden:
-
Name 50 characters
-
Login 20 characters
-
Address lines 20 characters
-
Post Code 5 characters
* Email addresses that are stored as free text are fully obfuscated, rather than set to x@example.com.
Only certain fields are permitted to be erased (for details, see Appendix: Using the Data Privacy API).
When updating a user account, the account is set to deactivated, and the password is set to expired.
Note:
If the GET call is submitted before the batch job has finished compiling the personal data, just a header will be returned, and the GET call should be retried.
The erasure of data is not reversible.
-
-
Optionally submit another Right to Access request to check that the data has been erased as expected.
-
Inform the requestor of the outcome of their request.
The Right to be Forgotten facility may also be used for purging user accounts that are no longer active.
Endpoint address: /services/rest/datapriv/erase HTTP methods: POST (verify and erase), GET (erase)
Request Details
For the verify POST method, a loginId parameter is passed in the payload.
For the erase POST method, an XML message is passed in the payload.
For the erase GET method, an id parameter is passed as a URI parameter.
URI Parameters
Example URL (verify POST method): …/services/rest/verify/
Example URL (erase POST method): …/services/rest/erase/
Example URL (erase GET method): …/services/rest/erase/?id=32
Response Details
For a successful response to the verify POST method, XML is returned with a dataprivVerifyDTO root element containing a status of Success.
For a successful response to the erase POST method, a link to the Data Privacy record is returned.
For a successful response to the erase GET method, an HTTP 200 response is sent with a dataprivErasureDTO root element containing a status of Success.
Error Responses
For a failure response to the verify method, XML is returned with a dataprivVerifyDTO root element containing a status of Failure, with the reason in the message element.
For a failure response to the erase method, XML is returned with a dataprivErasureDTO root element containing a status of Failure, with the reason in the message element.
Error Messages
Element | Message | Meaning |
---|---|---|
user |
Only one User can be erased at a time |
More than one User was passed in to the erase function. The XML must only relate to a single user. |
person |
Only one Person can be erased at a time |
More than one Person was passed in to the erase function. The XML must only relate to a single person. |
id |
The User is linked to Person with ID: %id but the Person included has ID: %id |
The wrong Person has been included. %id is the id taken from the XML. |
id |
The Contacts are linked to Person with ID: %id but the Person included has ID: %id |
The wrong Person has been included. %id is the id taken from the XML. |
id |
The Contact with ID %id is linked to a different User than the one being erased |
The Contacts passed do not match the user being erased. %id is replaced with the id from the XML. |
person |
All Contacts being erased must be linked to the same Person |
Where no User is being erased, all Contacts being erased must be for the same person. |
person |
Please include Person with ID: %id |
If User or Contacts are passed, the matching Person must also be included. |
id |
Cannot find entity of type: %rec and ID: %id |
An id is passed that is not found. %rec is the internal name of the record, such as ProductRecord. %id is the id taken from the XML. |
id |
Entity of type: %rec and ID: %id is currently locked, please try again later |
One of the records to be erased is currently locked. %rec is the internal name of the record, such as ProductRecord. %id is the id taken from the XML. Retry the request until the record becomes available. |
id |
Entity of type: %rec with id: %id has been edited during the erase process, please verify data |
One of the records updated has been edited between up front validation and the processing of that particular record. %rec is the internal name of the record, such as ProductRecord. %id is the id taken from the XML. |
user |
The user cannot be erased because they are responsible for the approval of a Scorecard |
The user is named as being responsible for completing a scorecard, so they cannot be erased. |
login id |
Cannot find User with login ID: %id |
No User account found for the passed login id. %id is the login id. |
The erase has failed due to unexpected internal error |
Other unexpected condition has occurred. |
AttachmentRestService
This section describes the API for retrieving the file attachments from certain records. The following function is available:
-
Retrieve Report Attachment: retrieves attachment files that have been generated by the system as report outputs
-
Retrieve Attachment: retrieves files that have been attached to records
The scorecardFullDTO, projectFullDTO and projectActivityFullDTO elements contain an attachments element which will includes a globalId element for each of the record's file attachments. The Retrieve Attachment service can be used to retrieve the actual attachment files by passing gloablId as a parameter.
The following describes the steps for retrieving file attachments:
Figure 4-7 Steps for Retrieving Attachments
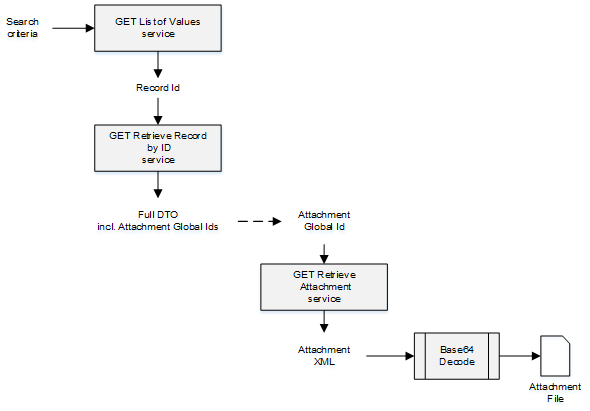
-
Call the List of Values service for the record with appropriate filters to locate the records for which attachments are to be retrieved. Record ids are returned for any records that match search criteria.
-
Call the Retrieve Record by ID service for each record id returned from the List of Values call. The record's full DTO element is returned, containing the record's data, including an attachments element which has a globalId element for each of the record's file attachments.
-
Call the Retrieve Attachment service passing the globalId as a parameter. The attachment file's data is returned as a Base 64 text string. Use a suitable decoder to generate the actual attachment file.
Retrieve Report Attachment
Description
Retrieves an attachment file that has been generated as the output of a report. The file types will be PDF, Excel, RTF, or HTML. When a report is generated, the outputs are stored in the Report Outputs area, grouped within a hierarchical folder structure.
The API provides a means of automatically locating reports for further analysis of the data within an external system. The ID of the attachment to be retrieved is sent to the external system's designated email address.
For additional detail on using the Report Attachment API, see Appendix: Using the Report Attachment API.
Endpoint address: /services/rest/attachment/report/{globalId} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {globalId} parameter that determines the record to retrieve.
Example URL
…/services/rest/attachment/report/f25eebdd-e7c5-4bb2-b61c-f35171438386
Response Details
For a successful response, XML is returned with a data element which contains the actual attachment as a Base64 encoded text string. The string must then be processed by a Base64 decoder to convert it to the actual report file.
Error Messages
Element | Message | Meaning |
---|---|---|
globalId |
HTTP 404 Not found |
Invalid {globalId} - not numeric |
globalId |
HTTP 417 IllegalStateException: Cannot find the Attachment to return with id:<###> |
Invalid {globalId} - not found |
Retrieve Attachment
Description
Retrieves a file that has been attached to a record. The files types will typically be documents or images.
Endpoint address: /services/rest/attachment/{globalId} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {globalId} parameter that determines the record to retrieve.
Example URL
…/services/rest/attachment/RTxy92Ha4lsQE6Bjc-18t1wJ7smbYoCH
Response Details
For a successful response, XML is returned with a data element which contains the actual attachment as a Base64 encoded text string. The string must then be processed by a Base64 decoder to convert it to the actual attachment file.
Error Messages
Element | Message | Meaning |
---|---|---|
globalId |
HTTP 404 Not found |
Invalid {globalId} - not numeric |
globalId |
HTTP 417 IllegalStateException: Cannot find the Attachment to return with id:<###> |
Invalid {globalId} - not found |
ScorecardRestService
This section describes the API for managing scorecards. The following functions are available:
-
List of Values: retrieves a list of scorecards
-
Retrieve Record by ID: retrieves a Scorecard record using its unique identifier
-
Retrieve Record by Business Key: retrieves a Scorecard record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Scorecard record was last updated
List of Values
Description
Retrieves a list of scorecards in a paged list. Use this function to locate Scorecard record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/scorecard HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
status |
Optional |
string |
Yes |
IN PROGRESS~COMPLETE |
siteStatus |
Optional |
string |
Yes |
AWAITING REGISTRATION |
siteType |
Optional |
string |
Yes |
PRIMARY |
siteCode |
Optional |
string |
Yes |
A0001-0001 |
siteName |
Optional |
string |
Yes |
West Road Site |
siteNameBusiness Language |
Optional |
string |
Yes |
West Road Site |
supplierCode |
Optional |
string |
Yes |
A0001 |
supplierName |
Optional |
string |
Yes |
ABC Ltd |
supplierNameBusiness Language |
Optional |
string |
Yes |
ABC Ltd |
businessCategory |
Optional |
string |
Yes |
POTATOES_LOOSE |
templateCode |
Optional |
string |
Yes |
ETHICAL_SCORECARD |
dueDate |
Optional |
string |
Yes |
2015-05-19 |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/scorecard/?offset=2&pageSize=20 …/services/rest/scorecard/?status=IN PROGRESS~COMPLETE …/services/rest/scorecard/?businessCategory=POTATOES_LOOSE
Response Details
For a successful response, XML is returned with a ScorecardLinkList root element containing an entries element for each matched scorecard. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Scorecard record's internal ID |
recordLink |
string |
URI to the ScorecardRestService Retrieve record service for this scorecard |
scorecardCode |
string |
Scorecard's code business key |
siteCode |
string |
Scorecard's site code |
supplierCode |
string |
Scorecard's supplier code |
templateCode |
string |
Scorecard's template code |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
Retrieve Record by ID
Description
Retrieves a single Scorecard record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual scorecard.
Endpoint address: /services/rest/scorecard/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/scorecard/54
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a scorecardFullDTO root element containing the individual attributes of the requested Scorecard record. If an ID is not specified, a list of all scorecards is returned (per the List of Values function).
File Structure
The main elements of the scorecardFullDTO root element:
-
scorecardTemplateSnapshot and Template - the settings from the associated template.
-
scorecardQuestions - the scorecard's questions and their answers - a separate element for each.
-
supplier and site - details of the supplier and site that the scorecard is related to.
-
udfData - the contents of any user-defined custom fields associated to the Scorecard record.
-
attachments - a child element containing the ids of any file attachments the scorecard has.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Scorecard to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Scorecard record's details using its business key (code). Use this function to retrieve the full details of an individual scorecard using its key code.
Endpoint address: /services/rest/scorecard/byKey/{code} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {code} parameter that determines the record to retrieve.
Example URL
…/services/rest/scorecard/byKey/184
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a scorecardFullDTO root element containing the individual attributes of the requested Scorecard record.
File Structure
The main elements of the scorecardFullDTO root element:
-
scorecardTemplateSnapshot and Template - the settings from the associated template.
-
scorecardQuestions - the scorecard's questions and their answers - a separate element for each.
-
supplier and site - details of the supplier and site that the scorecard is related to.
-
udfData - the contents of any user-defined custom fields associated to the Scorecard record.
-
attachments - a child element containing the ids of any file attachments the scorecard has.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 2 - Supplier for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
HTTP 404 Not found |
Invalid {code} - blank or not found |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Scorecard record. Use this function to determine when a scorecard was last updated.
Endpoint address: /services/rest/scorecard/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/scorecard/54
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Scorecard record. For example:
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
ProjectRestService
This section describes the API for managing projects. The following functions are available:
-
List of Values: retrieves a list of projects
-
Retrieve Record by ID: retrieves a Project record using its unique identifier
-
Retrieve Record by Business Key: retrieves a Project record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when a Project record was last updated
List of Values
Description
Retrieves a list of projects in a paged list. Use this function to locate Project record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/project HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
projectStatus |
Optional |
string |
Yes |
IN PROGRESS~COMPLETED |
templateCode |
Optional |
string |
Yes |
NEW_BAKERY_RANGE |
masterProject |
Optional |
Boolean |
No |
TRUE |
parentProject |
Optional |
string |
Yes |
NEW_LINE_DEVELOPMENT |
projectType |
Optional |
string |
Yes |
CHANGE OF SUPPLIER |
projectName |
Optional |
string |
Yes |
New Potato Product Launch |
projectManagerName |
Optional |
string |
Yes |
John Smith |
businessCategory |
Optional |
string |
Yes |
POTATOES_LOOSE |
specificationType |
Optional |
string |
Yes |
FOOD |
siteStatus |
Optional |
string |
Yes |
AWAITING REGISTRATION |
siteType |
Optional |
string |
Yes |
PRIMARY |
siteCode |
Optional |
string |
Yes |
A0001-0001 |
siteName |
Optional |
string |
Yes |
West Road Site |
siteNameBusiness Language |
Optional |
string |
Yes |
West Road Site |
supplierCode |
Optional |
string |
Yes |
A0001 |
supplierName |
Optional |
string |
Yes |
ABC Ltd |
supplierNameBusiness Language |
Optional |
string |
Yes |
ABC Ltd |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/user/?offset=2&pageSize=20 …/services/rest/user/?projectStatus=IN PROGRESS~COMPLETED …/services/rest/user/?templateCode=NEW_LINE_DEVELOPMENT
Response Details
For a successful response, XML is returned with a ProjectLinkList root element containing an entries element for each matched project. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Project record's internal ID |
recordLink |
string |
URI to the ProjectRestService Retrieve record service for this project |
projectId |
string |
Project's business key |
projectName |
string |
Project's name |
parentProjectId |
string |
Parent project's business key |
parentProjectName |
string |
Parent project's name |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
Retrieve Record by ID
Description
Retrieves a single Project record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual project.
Endpoint address: /services/rest/project/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/project/20
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a projectFullDTO root element containing the individual attributes of the requested Project record. If an ID is not specified, a list of all projects is returned (per the List of Values function).
File Structure
The main elements of the projectFullDTO root element:
-
projectTemplateSnapshot and Template - the settings from the associated template.
-
briefs - the associated project briefs; a separate element for each.
-
teams - the users and their roles associated to the project.
-
linkedRecords - the associated linked records; a separate element for each.
-
udfData - the contents of any user-defined custom fields associated to the Project record.
-
attachments - a child element containing the ids of any file attachments the project has.
Projects and their activities can be nested within a hierarchy. In the absence of a function to extract the full hierarchy with a single GET call, to retrieve the hierarchy of a project a number of successive calls is required. The Parent Project field is a Boolean value which will be blank for projects that have no hierarchy, or are at the top level of the hierarchy. Use the id or code of the Parent Project to traverse the levels. Use the Activities endpoints to retrieve the project's activity details. Master projects will not have a parent project id or a project name.
If a project is linked to a record such as a Product Specification or Audit, the linkedRecords element contains a list of the linked records, containing the id and business key of the record (audit code, product record code, spec number, supplier code, or site code), its type, name and status, and the date it was created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 9 - Project for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Project to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Project record's details using its business key (code). Use this function to retrieve the full details of an individual project using its key code.
Endpoint address: /services/rest/project/byKey/{code} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {code} parameter that determines the record to retrieve.
Example URL
…/services/rest/project/byKey/943
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a projectFullDTO root element containing the individual attributes of the requested Project record.
File Structure
The main elements of the projectFullDTO root element:
-
projectTemplateSnapshot and Template - the settings from the associated template.
-
teams - the users and their roles associated to the project.
-
briefs - the associated project briefs; a separate element for each.
-
linkedRecords - the associated linked records; a separate element for each.
-
udfData - the contents of any user-defined custom fields associated to the Project record.
-
attachments - a child element containing the ids of any file attachments the project has.
Projects and their activities can be nested within a hierarchy. In the absence of a function to extract the full hierarchy with a single GET call, to retrieve the hierarchy of a project a number of successive calls is required. The Parent Project field is a Boolean value which will be blank for projects that have no hierarchy, or are at the top level of the hierarchy. Use the id or code of the Parent Project to traverse the levels. Use the Activities endpoints to retrieve the project's activity details. Master projects will not have a parent project id or a project name.
If a project is linked to a record such as a Product Specification or Audit, the linkedRecords element contains a list of the linked records, containing the id and business key of the record (audit code, product record code, spec number, supplier code, or site code), its type, name and status, and the date it was created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 9 - Project for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
code |
HTTP 404 Not found |
Invalid {code} - blank or not found |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of a Project record. Use this function to determine when the project was last updated.
Endpoint address: /services/rest/project/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/project/20
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Project record. For example:
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
ActivityRestService
This section describes the API for managing project activities. The following functions are available:
-
List of Values: retrieves a list of activities
-
Retrieve Record by ID: retrieves an Activity record using its unique identifier
-
Retrieve Record by Business Key: retrieves an Activity record using its business key
-
Check Record Modification Timestamp: retrieves the timestamp when an Activity record was last updated
List of Values
Description
Retrieves a list of activities in a paged list. Use this function to locate Acitivity record IDs prior to a retrieve or update operation. Parameters are available to apply specific selection criteria for filtering the returned records.
Endpoint address: /services/rest/activity HTTP method: GET
Request Details
Parameters are passed as URI parameters.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
offset |
Optional |
int |
No |
60 |
pageSize |
Optional |
int |
No |
30 |
projectId |
Optional |
string |
Yes |
PR0000007 |
projectName |
Optional |
string |
Yes |
New Potato Product Launch |
activityName |
Optional |
string |
Yes |
Artwork |
activityCode |
Optional |
string |
Yes |
762 |
activityStatus |
Optional |
string |
Yes |
STARTED~COMPLETED |
activitySubStatus |
Optional |
string |
Yes |
MESSAGE SENT |
activityType |
Optional |
string |
Yes |
PRODUCT_ARTWORK |
activityTemplateCode |
Optional |
string |
Yes |
ARTWORK |
linkedRecord |
Optional |
string |
Yes |
SUPPLIER Note: Wildcard search not supported. Options are: SCORECARD SUPPLIER SITE AUDIT PRODUCT RECORD PRODUCT SPECIFICATION |
personResponsibleName |
Optional |
string |
Yes |
John Smith |
proposedStartDate |
Optional |
string |
No |
2015-05-19 |
proposedEndDate |
Optional |
string |
No |
2015-05-19 |
actualStartDate |
Optional |
string |
No |
2015-05-19 |
actualEndDate |
Optional |
string |
No |
2015-05-19 |
sequenceNo |
Optional |
string |
Yes |
1.0 |
modifiedSince |
Optional |
string |
No |
2015-05-19 13:30:39 |
modifiedUntil |
Optional |
string |
No |
2015-05-19 13:30:39 |
Example URLs
…/services/rest/user/?offset=2&pageSize=20 …/services/rest/user/?activityStatus=STARTED~COMPLETED
Response Details
For a successful response, XML is returned with a ProjectActivityLinkList root element containing an entries element for each matched project. The entries element consists of the elements shown in the following table.
Returned Elements
Element | Type | Description |
---|---|---|
recordId |
long |
Project Activity record's internal ID |
recordLink |
string |
URI to the AcitvityRestService Retrieve record service for this activity |
activityCode |
string |
Project Activity's business key |
projectRecordId |
string |
The parent Project's internal ID |
projectId |
string |
The parent Project's business key |
The returned XML also contains a totalRecords element, which states the total number of retrievable records that match the filter parameters.
Error Messages
In the event that an error occurs, an HTTP 500 response is sent.
Retrieve Record by ID
Description
Retrieves a single Activity record's details using the record's internal unique ID (which is not visible in the UI). Use this function to retrieve the full details of an individual project activity.
Endpoint address: /services/rest/activity/{id} HTTP method: GET
Request Details
The URL contains the {id} parameter that determines the record to retrieve.
URI Parameters
Parameter Name | Mandatory/Optional | Value Type | Multiple Value Separator (~) Supported? | Example |
---|---|---|---|---|
locale |
Optional |
string |
Yes |
en_GB~fr Locale country code. If used, fields that have language translations have a localeData element returned for the specified locales if data is present. |
Example URL
…/services/rest/activity/5
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a projectActivityFullDTO root element containing the individual attributes of the requested Activity record.
File Structure
The main elements of the projectActivityFullDTO root element:
-
activityTemplateSnapshot and Template - the settings from the associated template.
-
briefs - the associated project briefs; a separate element for each.
-
linkedRecords - the associated linked records; a separate element for each.
-
udfData - the contents of any user-defined custom fields associated to the Activity record.
-
attachments - a child element containing the ids of any file attachments the activity has.
If an activity is linked to a record such as a Product Specification or Audit, the linkedRecords element contains a list of the linked records, containing the id and business key of the record (audit code, product record code, spec number, supplier code or site code), its type, name and status, and the date it was created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 9 - Project for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 404 Not found |
Invalid {id} - not numeric |
id |
HTTP 417 IllegalStateException: Cannot find the Activity to return with id:<###> |
Invalid {id} - not found |
Retrieve Record by Business Key
Description
Retrieves a single Activity record's details using its business key (code) and its parent project's code. Use this function to retrieve the full details of an individual activity using its key code.
Endpoint address: /services/rest/activity/byKey/{activityCode} HTTP method: GET
Request Details
There are no request parameters, but the URL contains the {activityCode} parameter that determines the record to retrieve.
Example URL
…/services/rest/activity/byKey/762
Response Details
For a successful response, an HTTP 301 message is returned, which is automatically acted upon to retrieve XML consisting of a projectActivityFullDTO root element containing the individual attributes of the requested Activity record.
File Structure
The main elements of the projectActivityFullDTO root element:
-
activityTemplateSnapshot and Template - the settings from the associated template.
-
briefs - the associated project briefs; a separate element for each.
-
linkedRecords - the associated linked records; a separate element for each.
-
udfData - the contents of any user-defined custom fields associated to the Activity record.
-
attachments - a child element containing the ids of any file attachments the activity has.
If an activity is linked to a record such as a Product Specification or Audit, the linkedRecords element contains a list of the linked records, containing the id and business key of the record (audit code, product record code, spec number, supplier code, or site code), its type, name and status, and the date it was created.
See the associated WADL for a full list of the attributes, and the Oracle Retail Brand Compliance Management Cloud Service Data Dictionary, Volume 9 - Project for details of their mapping to the fields within the Brand Compliance UI.
Error Messages
Element | Message | Meaning |
---|---|---|
activityCode |
HTTP 404 Not found |
Invalid {activityCode} - blank or not found |
Check Record Modification Timestamp
Description
Retrieves the last modification date and time of an Activity record. Use this function to determine when the project activity was last updated.
Endpoint address: /services/rest/activity/{id} HTTP method: HEAD
Request Details
There are no request parameters, but the URL contains the {id} parameter that determines the record to retrieve.
Example URL
…/services/rest/activity/5
Response Details
If successful, an HTTP 200 response is sent containing the Last-Modified header showing the date and time of the last update of the requested Activity record. For example:
HTTP/1.1 200 OK Date: Wed, 13 Jul 2016 07:52:14 GMT Last-Modified: Fri, 08 Jul 2016 06:44:46 GMT Content-Type: application/xml Content-Length: 0
Error Messages
Element | Message | Meaning |
---|---|---|
id |
HTTP 417 Expectation Failed |
Invalid {id} - not found |
id |
HTTP 404 Not found |
Invalid {id} - not numeric |