4 REST Web Services
Web services are intended for integration to allow a system using those services to control the flow and processing of data within EICS. There are multiple types of data involved in this integration. Data that is managed by other systems and needs to get into our system, but that EICS does not manage. This includes such concepts as item, stores, and point-of-sale transaction. Data that is managed by EICS includes such ideas as inventory adjustments, transfers, deliveries, and stock counts. Some services will provide ability for external data to get into EICS, some are intended to be used real time such as approving, picking, and dispatching shipments.
REST WEB Services Security Considerations
The REST web services provided by EICS are secured using OAuth2 tokens and require SSL.
The supported OAuth2 security requires a token requested for the client_credentials grant with the EICS integration scope (for example, rgbu:siocs:integration).
Note that the scope name differs for each environment.
REST Web Service OAuth2 Requests
This section will describe how to call an EICS web service using the OAuth2 protocol. The target audience is developers who are looking to write code that calls the web service.
Using the OAuth Protocol
The OAuth protocol is relatively straightforward:
-
Get an access token from the authentication provider
-
Pass the access token along with the web service request
In this case, the authentication provider is Oracle Identity Cloud Service (IDCS). Every customer who purchases a subscription to EICS gets a subscription to IDCS as part of their purchase.
Obtaining a Token
REST APIs use OAuth2.0 for authorization.
To generate a token from IDCS, an IDCS application client will need to be created for you to use.
The Customer Administration users must create their own client credential IDCS application using the Oracle Retail Home Cloud Service. For additional details, refer to Oracle® Retail Home Administration Guide- Chapter: Oauth Application Configuration chapter – Section: Creating OAuth Client Applications.
The App name and scope that should be used for IDCS application creation should be environment specific using the format :
App Name- RGBU_SIOCS_<ENV>_EICS_INT
Scope- rgbu:siocs:integration-<ENV>
Example:
App Name- RGBU_SIOCS_STG1_ EICS_INT
Scope- rgbu:siocs:integration-STG1
You will need the following information about the IDCS application client to request a token:
-
IDCS URL
-
Client Id
-
Client Secret
-
Scope Name
Note:
the application client must be assigned the scope from the EICS IDCS cloud service in order to request the token. This assignment is performed when the application client is created.
The scope name will differ for each environment. Please ensure the correct value is used for your environment.
To generate a token, you will need to invoke the appropriate IDCS REST API. The curl command in Linux that describes the POST that will return a token is as follows:
curl -H 'Authorization: Basic <base64(clientId:clientSecret)>'
-H 'Content-Type: application/x-www-form-urlencoded;charset=UTF-8'
--request POST <IDCS URL>/oauth2/v1/token -d 'grant_type=client_credentials&scope=<EICS
Scope>'
In Windows, use double-quotes, as follows:
curl -H "Authorization: Basic <base64(clientId:clientSecret)>"
-H "Content-Type: application/x-www-form-urlencoded;charset=UTF-8"
--request POST <IDCS URL>/oauth2/v1/token -d "grant_type=client_credentials&scope=<EICS
Scope>"
This is a standard REST POST, with the following details:
-
<IDCS URL> is the IDCS URL the retailer provided
-
Include the Client Id and Client Secret as a Basic Authentication header
-
Specify the Content Type as application/x-www-form-urlencoded;charset=UTF-8
-
Specify the body as grant_type=client_credentials&scope=<EICS Scope>
The service will respond with the following JSON message:
{
"access_token": "<TOKEN>",
"token_type": "Bearer",
"expires_in": 3600
}
Note that the response will return how long the token is valid for. You should reuse the same token until it expires in order to minimize calls to IDCS to get a token.
If the token request fails, you will receive the following JSON response:
{
"error":"<error>",
"error_description":"<error description>",
"ecid":"u….."
}
The most common errors are:
-
Invalid Client. This means that the client information you send in is not correct. The error description will expand on the reason:
-
Client Authentication Failed means that the client is valid, but the client secret is incorrect.
-
Invalid OAuth Client <CLIENT> means that the client id is not valid, and the invalid client will be listed in the error message.
-
-
Invalid Request. Some part of the inbound request is not valid. The error description is usually descriptive about what the actual error condition is
Calling the EICS Web Service
To invoke the web service with an OAuth2 token, you must add an Authorization header to the request. The value of the Authorization header must be Bearer <token>, that is:
-
The word Bearer
-
A space
-
A valid token
For a REST service call, the request might look something like this:
curl -X POST -H 'Content-Type: application/json' -H 'Authorization:
Bearer <TOKEN>' -i https://CloudServiceURL --data '{PAYLOAD}'
Remember that the token will expire after a specific amount time, and to be more efficient you should always use a token so long as it's valid. It is your responsibility to make sure that you are keeping track of whether the token is still valid. Your pattern should be:
-
Check to see if you have a valid token that has not expired.
-
If not, call to IDCS and get a new token. Store it and its expiration time.
-
Send the request into the web service with the token in the Authorization header as a Bearer token.
REST WEB Services Basic Design Principles
Requests and Responses
When making requests and processing responses from REST web services it is important for the client to handle headers correctly.
The client should always use Accept for the appropriate content type when making requests.
The client should always check the response status code and Content-Type header before processing a response body.
When reading a payload from the response body, the Content-Length header must be used safely and securely along with the Content-Type.
This is important even for error responses. It is possible for errors to occur outside of the REST API layer, which may produce different content for the error. In these cases, it is common to get text or HTML content for the response body.
API Versioning
Accept-Version
The REST end points have an optional API versioning feature allowing the client to specify an API version to be accepted.
This may be used by the client to ensure that no calls may be made to a web service that uses an incorrect version number.
For example: Accept-Version: 22.1.301
If the web service does not support this API version, then the server will produce a 400 Bad Request error response.
Content-Type
application/json
The content type of both REST input and returned output is application/json.
In the case that no content is included, a content type may not be assigned.
When handling REST service responses, the client must always check the returned Content-Type and Content-Length before processing the payload.
JSON Validation
When consuming a REST service end point that requires a request payload as JSON, the client is responsible for verifying that the JSON is valid. If invalid data is sent in a request, there may be a server error processing the JSON or it may ignore some fields if the JSON is valid but does not map correctly to the API payload definition.
Always make sure that the client sends valid JSON that is designed to satisfy the API payload definition.
Synchronous vs Asynchronous
Each service API will be defined as synchronous or asynchronous. Both perform JSON validation as described above. If the API is synchronous, the remaining data validation and live updating of the data will take place immediately and the call will be rejected if any business errors occur. If the API is asynchronous, the data is set aside to be processed later and the REST service is successfully returned noting that the data has been accepted. In the case of asynchronous processing, business error and failed data recovery is monitored and the dealt with outside of the REST web service.
Online Documentation
EICS has exposed Swagger JSON files for customers to copy and use with the Swagger application as online documentation. These files can be found at:
https://<external_load_balancer>/<cust_env>/siocs-int-services/public/api/
Configured System Options In EICS
Web services apply system configuration to the request that are coming in through a web service but assumes that all in-put validation that requires user interaction to confirm has been completed by the third party system prior to accessing the service. It operates as if the user confirmed any activity. However, if a system option is a fixed restriction that does not require user interaction, and the input fails the restriction, this is always considered an error.
Examples of configurations being applied include:
Shipping inventory when inventory is less than 0 can be allowed by a user of EICS. The web services assumes that the application accessing the service did prompt the user or that their business always allows the user to this activity.
Adding a non-ranged item requires both a system configuration option to be enabled and the user to confirm the addition of the item. If the system configuration does not allow it, the web service will block the transaction and return an error (un-less processing asynchronously). If the system configuration does allow adding non-ranged items, it will automatically assume that a user confirmed this addition and processing will allow the addition of the item.
Allowing Receiver Unit Adjustments is dependent on a period of time. If a receiver unit adjustment were to come into EICS after that period of time, it would automatically be rejected, and the web service would return an error regardless of presentation or confirmation of user done by the external system.
External vs Internal Attributes
EICS web services are EICS centric and track information from an internal application point-of-view. This has ramifications on three types of data: identifiers, dates, and users.
Almost all paths and information will contain an identifier. In almost all cases, this will be an EICS internal identifier generated within our system. If external identifiers also exist for the date, they will be defined as such in the information. For example, you might encounter transferId and externalTransferId as attributes. In some cases, an API only takes an internal identifier, and you may need to use lookup APIs to retrieve an internal identifier using an external identifier as search criteria.
Timestamps are captured at the time an event occurs within EICS as part of EICS's internal tracking and state management. For example, we capture the timestamp when a shipment is created, last updated, and when it is dispatched. These timestamps occur at the time this occurred within EICS. When a REST service is called to create a transaction, such as a shipment, the create timestamp of the shipment will be the moment that service is called. If the shipment is dispatched using the web service, the dispatch date will be the moment that service is called. So if an external system dispatched a shipment two days earlier, and is just now calling the web service, it will not capture the external dispatch time. In some places, you will encounter a date that can be entered as part of the input information (for example, an externalDispatchDate, or simple a transactionTimestamp). If it is part of the input information, then it will be captured as that attribute defined in the API.
The user responsible for actions is often captured as part of transaction information with EICS. Some examples might be the user that created the data, the user the last updated it, or perhaps the user that approved it. In these cases, the user is considered an internal user as is assigned the current session user at the time the activity takes place. When accessing the REST service, the user will be fiwed as an “External User” to indicate it came from an external system, and not the user that manipulated the information in an external system. If external users are to be captured by the data, there will be independent attribute fields such as externalCreateUser that capture the identity of a user in an external system.
Dates In Content
Dates includes in JSON must be in the following format: 2022-04-19T23:59:59-05:00
Dates included as a query parameter must be in the following format: 220227152543-0700
Note:
After the format permissible length, any additional trailing characters will be ignored and the date will still be processed.Links In Content
JSON information for a data object may include links. These are self-referential APIs that defined other APIs that are available with the information. In the example below, when reading an activity lock, the following links were included that define a path to accomplish other calls. HRef lists the basic reference and the “rel” the remainder of the path. So, when you read activity lock (1), you get a delete reference that maps to /activitylock/1/delete, defining the REST path to delete that lock.
[ {
"links" : [ {
"href" : "/activitylocks/1",
"rel" : "self"
}, {
"href" : "/activitylocks/1",
"rel" : "delete"
} ],
Hypertext Transfer Protocol Status Codes
The following information documents the HTTP status codes that Oracle returns via web services calls.
Success Codes
Successful codes are returned whenever the accessing client call was made without any error in the form or content.
Code | Description |
---|---|
200 OK |
The information supplied by the customer was in a correct form. This code is returned when reading a resource or querying information about an existing resource or schema. This response code is used when the access is synchronous. |
202 Accepted |
The information supplied by the customer as in a correct form. This code is returned when access is asynchronous. |
204 No Content |
The information supplied by the customer was in a correct form. The request was successful but the API itself never provides information as a response. |
Client Failure Codes
Client failure codes indicate the client made an error in their service access and must correct their code or its content to fix the failure.
Code | Description |
---|---|
400 Bad Request |
If this code is returned, it indicates the customer made a call with invalid syntax or violated the defined properties of the input information. Detailed information may be returned that further identifies the error. |
401 Unauthorized 403 Forbidden |
If either of these codes are returned, it indicates the access to service was denied. This may occur if no OAuth2 token was provided, or the token had expired, or the identity the token was generated for did not have sufficient access. |
404 Not Found |
If this code is returned, it indicates the customer made an erroneous access call against a resource or schema that is not defined. |
405 Method Not Allowed |
If this code is returned, it indicates that the wrong HTTP method was used to make the call. Please check the API. |
406 Not Acceptable |
If this code is returned, it indicates the wrong Accept header value was used to make the call. Please check the API. |
409 Too Many Requests |
If this code is returned, it indicates that the web service has received too many service requests recently. This may indicate a cloud issue requiring support to address or the client is making too many calls too frequently and a solution may be required to avoid the issue. |
System Failure Codes
System failure codes are returned whenever the processing server encounters an unexpected or severe failure.
Code | Description |
---|---|
500 Internal Server Code |
A server error occurred that did not allow the operation to complete. |
502 Bad Gateway 503 Service Unavailable 504 Gateway Timeout |
If any of these codes are returned, it indicates an issue with the network or cloud services, which may occur due to either client or cloud networking or infrastructure issues, such as outages. |
JSON Error Element and Error Codes
If an error occurs in the form of the content, or during processing of the content, an HTTP error code will be returned along with a series of JSON Error Elements as described here.
Example Error
HTTP Response: 400 Bad Request
{
"errors": [
{
"code": 7,
"description": "Missing Attribute",
"dataElement": "storeId",
"referenceElement": "transactionId",
"referenceValue": "1236"
},
{
"code": 11,
"description": "Element Too Large",
"dataElement": "transactionId",
"dataValue": 128,
"referenceElement": "transactionId",
"referenceValue": "1236"
}
]
}
Error Attribute Definitions
Attribute | Definition |
---|---|
Code |
A numeric code indicates the issue. See Integration Error Codes table. |
Description |
The name of the error or issue. |
DataElement |
The name of an attribute or element of the JSON structure that failed. |
DataValue |
The value of the attribute or element that failed, or a piece of information about the element that failed (such as a maximum value). |
ReferenceElement |
The name of an attribute or element that will help further identify the data element. Most often the containing element one level above the failed elements (such as a transaction header). |
ReferenceValue |
The value of the attribute or element that will help further identify the data element. |
Integration Error Codes
The following table contains a listing of the error codes that can be found within returned error information.
Code | Name | Issue |
---|---|---|
1 |
Business Error |
A business processing error prevent the service from completing. |
2 |
Date Range Error |
The date range has a problem (usually indicates end date is earlier than start date in a date range. |
3 |
Duplicate Error |
Indicates duplicate element within the data is not permitted. |
4 |
Forbidden |
Access is not allowed to the service. |
5 |
Internal Server Error |
A severe error occurred with the service attempting to process the request. |
6 |
Invalid Input |
Most often this indicates that input was included that is not allowed or not needed, however it also doubles a kind of catch-all category. |
7 |
Invalid Format |
An input was in an invalid format (most often a date string in a query parameter not being in a valid date format). |
8 |
Invalid Status |
A transaction or entity is not in a valid status to proceed with the request. |
9 |
Missing Path Element |
A path element defining the path of the resource URL was not present. |
10 |
Missing Attribute |
A required attribute was missing on the input to the service. |
11 |
Not Found |
A data element in the input could not be found in the system (most often an invalid identifier). |
12 |
No Data Input |
No input exists for a service that requires input. |
13 |
No Query Input |
No query input exists at all for a query that requires at least one input. |
14 |
Element Too Large |
An input was too large (exceeded maximum count or maximum size). |
15 |
Results Too Large |
The results of the service were too large to return. |
Error Code Data Elements
The following table contains a listing of likely or possible data elements that would be matched with a code. Data element and value may not be returned in all cases.
Code | Name | Data Element | Data Value |
---|---|---|---|
1 |
Business Error |
Business exception name/key |
Data Value |
2 |
Date Range Error |
Date element name |
Value of date |
3 |
Duplicate Error |
Duplicate element name |
Duplicated Value |
4 |
Forbidden |
N/A |
- |
5 |
Internal Server Error |
N/A |
- |
6 |
Invalid Input |
Element name |
Value of element |
7 |
Invalid Format |
Element name |
Value of element |
8 |
Invalid Status |
Element name |
Status of element |
9 |
Missing Path Element |
Missing element |
- |
10 |
Missing Attribute |
Required element name |
- |
11 |
Not Found |
Element not found |
Value of element not found |
12 |
No Data Input |
Missing element |
- |
13 |
No Query Input |
N/A |
- |
14 |
Element Too Large |
Element name |
Allowed size limit |
15 |
Results Too Large |
N/A |
- |
REST Service: Activity Lock
This service allows the creation, removal, and finding of activity locks.
An activity lock is a record indicating the user, time, and a piece of information (a transaction) that should be considered "locked". All server processing validates that the accessing user has a lock on the information before updating, notifying the current user if someone else has modified the information while they were locked and preventing the stale update.
Developers should create locks on transactional information prior
to performing update calls and delete locks when the update if finished.
For example, create a lock on inventory adjustment with ID 123 with
the ActivityLock service, then use saveInventoryAdjustment
in the Inventory Adjustment service with Adjustment 123, and then
delete the activity lock using the ActivityLock service. If you do
not gain the lock, you will receive an error when attempting to save
an inventory adjustment.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/activitylocks
API Definitions
API | Description |
---|---|
Find Lock |
Search for activity lock information based on a set of criteria. |
Create Lock |
Create a user activity lock. |
Delete Lock |
Remove a user activity lock. |
Read Lock |
Retrieve complete information about an activity lock. |
API: Find Lock
Searches for locks based on input criteria. At least one input criteria should be provided.
If the number of activity locks found exceeds 10,000, a maximum limit error will be returned. Additional or more limiting search criteria will be required.
API Basics
Endpoint URL |
{base URL}/find |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Criteria |
Output |
List of activity locks |
Max Response Limit |
10,000 |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
activityType |
String (40) |
No |
A type of activity that is locked (see Additional Data Definition). |
sessionId |
String (128) |
No |
The unique identifier of the session that owns the lock. |
deviceType |
Integer (4) |
No |
The device type (see Additional Data Definition). |
userName |
String (128) |
No |
The unique identifier of the user that owns the lock. |
lockDateFrom |
Date |
No |
Start date of a range during which the activity was locked. |
lockDateTo |
Date |
No |
End date of a range during which the activity was locked. |
Example Input
{
"activityType": "3",
"sessionId": "sessionTest",
"deviceType":3
}
Output Data Definition
Attribute | Data Type | Description |
---|---|---|
lockId |
Long |
The identifier of an activity lock. |
sessionId |
String |
The identifier of the session that owns the lock. |
activityId |
String |
The identifier of the activity that is locked. |
activityType |
Integer |
The type of activity that is locked. |
deviceType |
Integer |
The device type. |
userName |
String |
The identifier of the user that owns the lock. |
lockDate |
Date |
The date the activity was locked. |
Example Output
[ {
"links" : [ {
"href" : "/activitylocks/1",
"rel" : "self"
}, {
"href" : "/activitylocks/1",
"rel" : "delete"
} ],
"lockId" : 1,
"sessionId" : "sessionTest",
"activityId" : "2",
"activityType" : 3,
"deviceType" : 3,
"userName" : "admin",
"lockDate" : "2023-01-04T08:59:41-06:00"
} ]
Additional Data Definitions
Location Type
Value | Definition |
---|---|
1 |
Bill Of Lading |
2 |
Direct Delivery Invoice |
3 |
Fulfilment Order |
4 |
Fulfilment Order Delivery |
5 |
Fulfilment Order Pick |
6 |
Fulfilment Order Reverse Pick |
7 |
Inventory Adjustment |
8 |
Inventory Adjustment Reason |
9 |
Item Basket |
10 |
Item Request |
11 |
POS Transaction Resolution |
12 |
Price Change |
13 |
Product Basket |
14 |
Product Group |
15 |
Product Group Schedule |
16 |
Replenishment Gap |
17 |
Shelf Adjustment |
18 |
Shelf Replenishment |
19 |
Shipment Reason |
20 |
Stock Count Child |
21 |
Store Order |
22 |
Ticket |
23 |
Ticket Format Basket |
24 |
Transaction Event |
25 |
Transfer |
26 |
Transfer Delivery Carton |
27 |
Transfer Shipment Carton |
28 |
Vendor Delivery Carton |
29 |
Vendor Shipment Carton |
30 |
Vendor Return |
Device Type
Value | Definition |
---|---|
1 |
Client |
2 |
Server |
3 |
Integration Service |
API: Create Lock
Used to create a new user transaction activity lock. This prevents two users from simultaneously changing the same data.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Activity Lock object |
Output |
Activity lock identifier |
Max Response Limit |
N/A |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
sessionId |
String(128) |
Yes |
The unique identifier of the session that owns the lock. |
activityId |
String(128) |
Yes |
The unique identifier of the activity that is locked. This is often a primary identifier of a transaction. |
activityType |
Integer |
Yes |
The type of activity that is locked. This is often a transaction type (see Additional Data Definition). |
Example Input
{
"activityType": 5,
"sessionId": "session01",
"activityId":"35"
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
lockId |
Long |
Yes |
The unique identifier if a new activity lock is created, or null if the lock already exists. |
Example Output
{
"lockId" : 2
}
API: Delete Lock
Used to remove a lock and indicates the activity should no longer be restricted and another user can now begin activity on that data.
It will remove the lock if it exists and perform no action if the lock does not currently exist. In either case, it returns 204 No Content.
API Basics
Endpoint URL |
{base URL}/{activityLockId}/delete |
Method |
DELETE |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Attribute | Description |
---|---|
activityLockId |
The activity lock identifier to be removed. |
API: Read Lock
Used to retrieve full information about a lock.
API Basics
Endpoint URL |
{base URL}/{activityLockId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
Activity Lock record |
Max Response Limit |
N/A |
Attribute | Description |
---|---|
activityLockId |
The activity lock identifier to be read. |
Output Data Definition
Attribute | Data Type | Description |
---|---|---|
lockId |
Long |
The identifier of an activity lock. |
sessionId |
String |
The identifier of the session that owns the lock. |
activityId |
String |
The identifier of the activity that is locked. |
activityType |
Integer |
The type of activity that is locked. |
deviceType |
Integer |
The device type. |
userName |
String |
The identifier of the user that owns the lock. |
lockDate |
Date |
The date the activity was locked. |
Example Output
{
"lockId": 4,
"sessionId": "session01",
"activityId": "37",
"activityType": 5,
"deviceType": 3,
"userName": "dev",
"lockDate": "2023-01-04T23:52:08-06:00"
REST Notification
This page captures the service APIs related to accepting notification from an external system.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/notifications
APIs
API |
Description |
findNotifications |
Find notifications |
createNotifications |
Create a series of notifications |
API: findNotifications
API is used to search for notifications.
Table 4-1 API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
Collection of notifications |
Max Response Limit |
20,000 |
Table 4-2 Query Parameters
Attribute |
Type |
Definition |
storeId |
Long(10) |
Include only records with this store identifier. |
notificationType |
Integer(4) |
Include only records with this notification type. |
transactionType |
Integer(4) |
Include only records with this transaction type. |
status |
Integer(4) |
Include only records with this status. |
createDateFrom |
Date |
Include only records with a create date on or after this date. |
createDateTo |
Date |
Include only records with a create date on or before this date. |
Attribute |
Type |
Definition |
notificationId |
Long(15) |
The unique identifier of the notification. |
notificationType |
Integer(4) |
The type of notification. See Index. |
storeId |
Long(10) |
The store identifier. |
subject |
String(400) |
The title or subject of the notification. |
message |
String(2000) |
The message text of the notification. |
status |
Integer(4) |
The current status of the notification. |
transactionType |
Integer(4) |
The type of a transaction associated to the notification. See Index. |
transactionId |
Long(15) |
The identifier of a transaction associated to the notification. |
createDate |
Date |
The date the notification was originally created. |
createUser |
String(128) |
The user that created the notification. |
API: createNotifications
Create one or more notifications within the system.
Payload |
Type |
Req |
Definition |
notifications |
Collection |
X |
The list of notifications to create. |
Table 4-3 Notification
Attribute |
Type |
Req |
Definition |
storeId |
Long(10) |
X |
The store identifier. |
notificationType |
Integer(4) |
X |
The type of notification. See Index. |
subject |
String(400) |
X |
The title or subject of the notification. |
message |
String(2000) |
X |
The message text of the notification. |
transactionType |
Integer(4) |
X |
The type of a transaction associated to the notification. See Index. |
transactionId |
Long(15) |
X |
The identifier of a transaction associated to the notification. |
createDate |
Date |
The date the notification was originally created. This will default to current date if not supplied. |
|
createUser |
String(128) |
The user that created the notification. This will default to integration user if not supplied. |
Example
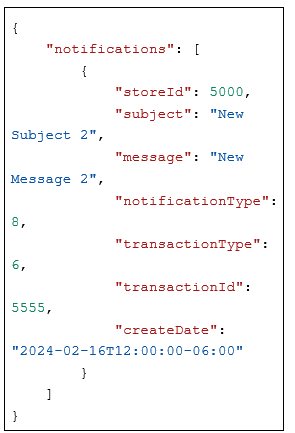
Index
Table 4-4 Notification Type
ID |
Description |
1 |
Customer Order (New) |
2 |
Customer Order Reauthorization |
3 |
Customer Order Pick Reminder |
4 |
Customer Order Pickup |
5 |
Customer Order Receipt |
6 |
Customer Order Reminder |
7 |
Fulfillment Order Reverse Pick (New) |
8 |
UIN Items on Incoming ASN Failed |
9 |
Return To Vendor Expiration Approaching |
10 |
Transfer Delivery Damaged |
11 |
Transfer Delivery Receiving Discrepancy |
12 |
Transfer Delivery Carton Misdirected |
13 |
Transfer Delivery Overdue |
14 |
Transfer Delivery UIN Discrepancy (From Finisher) |
15 |
Transfer Delivery UIN Discrepancy |
16 |
Transfer Delivery Auto-Receive Failure (From Finisher) |
17 |
Transfer Delivery Auto-Receive Failure (From Warehouse) |
18 |
Transfer Delivery Auto-Receive Failure (From Store) |
19 |
Transfer Request Approved |
20 |
Transfer Request Quantity Unavailable |
21 |
Transfer Request Expiration Approaching |
22 |
Transfer Request Submitted |
23 |
Transfer Request Rejected |
24 |
UIN Store Unexpectedly Altered |
25 |
Vendor Delivery Over Received Quantity |
26 |
Vendor Return Quantity Unavailable |
Table 4-5 Transaction Type
ID |
Description |
1 |
Customer Order |
2 |
Customer Order Pick |
3 |
Customer Order Delivery |
4 |
Customer Order Reverse Pick |
5 |
Direct Store Delivery Receiving |
6 |
Inventory Adjustment |
7 |
Item Basket |
8 |
Return To Vendor |
9 |
Return To Vendor Shipment |
10 |
Shelf Adjustment |
11 |
Shelf Replenishment |
12 |
Shelf Replenishment Gap |
13 |
Stock Count |
14 |
Store Order |
15 |
Transfer |
16 |
Transfer Shipment |
17 |
Transfer Receiving |
Table 4-6 Notification Status
ID |
Description |
1 |
Unread |
2 |
Read |
REST Service: Address
This service integrates address foundation data. Asynchronous address integration is processed through staged messages and is controlled by the MPS Work Type: DcsStore.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/addresses
API Definitions
API |
Description |
readAddress |
Read the information about a single address. |
importAddress |
Create or update the information about a single address. |
deleteAddress |
Deletes a single address. |
API: Import Address
Import a series of addresses. This allows up to 1,000 addresses before an input too large error is returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Addresses list |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
addresses |
List of details |
Yes |
A list of addresses to import. |
Address Detail Data Definition
Attribute |
Data Type |
Required |
Description |
addressId |
String(25) |
Yes |
The external identifier of the address. |
entityType |
Integer |
Yes |
Donates the type of location of the entity (see Additional Data Definition). |
entityId |
Long(10) |
Yes |
The external identifier of the entity (this will also match internal identifiers). |
addressType |
Integer |
Yes |
The type of address: (see Additional Data Definition). |
primary |
Boolean |
True if this is the primary address of the entity, false otherwise |
|
addressLine1 |
String(240) |
Yes |
The first line of the address |
addressLine2 |
String(240 |
The second line of the address |
|
addressLine3 |
String(240) |
The third line of the address |
|
city |
String(120) |
Yes |
The city of the address |
state |
String(3) |
The state of the address |
|
countryCode |
String(3) |
Yes |
The country code of the address (used by supplier) |
postalCode |
String(30) |
The postal code of the address |
|
county |
String(250) |
The county of the address |
|
companyName |
String(120) |
A company name associated with that address |
|
contactName |
String(120) |
Contact name for that address |
|
contactPhone |
String(20) |
Contact phone number for that address |
|
contactFax |
String(20) |
Contact fax number for that address |
|
contactEmail |
String(100) |
Contact email for that address |
|
firstName |
String(120) |
A first name of a contact at that address |
|
lastName |
String(120) |
A last name of the contact at that address |
|
phoneticFirstName |
String(120) |
A phonetic spelling of a first name of a contact at that address |
|
phoneticLastName |
String(120) |
A phonetic spelling of a last name of a contact at that address |
|
supplierLocation |
String(120) |
Supplier location information |
API: Delete Address
Deletes a single address.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{addressId}/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
None |
|
Output |
None |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
|
addressId |
The address identifier to be removed |
API: Read Address
Used to read a single address.
API Basics
Endpoint URL |
{base URL}/{addressId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
Address record |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
addressId |
The address identifier to be removed |
Output Data Definition
Attribute |
Data Type |
Description |
addressId |
String |
The external identifier of the address. |
entityType |
Integer |
Donates the type of location of the entity (see Additional Data Definition). |
entityId |
Long |
The external identifier of the entity (this will also match internal identifiers). |
addressType |
Integer |
The type of address: (see Additional Data Definition) |
primary |
Boolean |
True if this is the primary address of the entity, false otherwise |
addressLine1 |
String |
The first line of the address |
addressLine2 |
String |
The second line of the address |
addressLine3 |
String |
The third line of the address |
city |
String |
The city of the address |
state |
String |
The state of the address |
countryCode |
String |
The country code of the address (used by supplier) |
postalCode |
String |
The postal code of the address |
county |
String |
The county of the address |
companyName |
String |
A company name associated with that address |
contactName |
String |
Contact name for that address |
contactPhone |
String |
Contact phone number for that address |
contactFax |
String |
Contact fax number for that address |
contactEmail |
String |
Contact email for that address |
firstName |
String |
A first name of a contact at that address |
lastName |
String |
A last name of the contact at that address |
phoneticFirstName |
String |
A phonetic spelling of a first name of a contact at that address |
phoneticLastName |
String |
A phonetic spelling of a last name of a contact at that address |
supplierLocation |
String |
Supplier location information |
Additional Data Definitions
Entity Type
Value |
Definition |
1 |
Store |
2 |
Supplier |
3 |
Warehouse |
4 |
Finisher |
Address Type
Value |
Definition |
1 |
Business |
2 |
Postal |
3 |
Return |
4 |
Order |
5 |
Invoice |
6 |
Remittance |
7 |
Billing |
8 |
Delivery |
9 |
External |
REST Service Batch
This service allows an external system to schedule an adhoc batch job for execution.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/batches
API Definitions
API |
Description |
executeBatch |
Schedules the batch for immediate execution. |
findBatchJobs |
Finds all the batch jobs available to schedule. |
API: Execute Batch
Schedules the specified batch job for immediate execution.
If parameter date and/or parameter identifier are entered, they are passed as parameters to the batch job identified by the batch name.
See Batch guide for definition of data set identifiers for various batches.
API Basics
Endpoint URL |
{base URL} |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Batch information |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
batchName |
String (256) |
Yes |
The name of the batch job to execute. |
storeId |
Long(10) |
A store identifier to run the batch for. If included, it will run the batch processing for a single store. If not included, the batch will run for all stores based on functional description of the batch processing. |
|
parameterDate |
Date |
A parameter date passed to the batch job (see SIOCS adhoc batch documentation). |
|
parameterId |
String |
A parameter identifier passed to the batch job (see SIOCS adhoc batch documentation). |
API: Find Batch Jobs
Finds all the batch jobs available to schedule.
API Basics
Endpoint URL |
{base URL} |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of batch jobs |
|
Max Response Limit |
N/A |
Output Data Definition
Attribute |
Data Type |
Description |
jobName |
String |
The job name used to execute the batch. |
shortDescription |
String |
A short description of the batch job. |
longDescription |
String |
A long description of the batch job. |
jobParamHint |
String |
Some hint text for what parameter values might be. |
jobInterval |
Integer |
The execution interval of the batch job (see Additional Data Definition). |
batchType |
Integer |
The type of batch job (see Additional Data Definition). |
storeRelated |
Boolean |
Y indicates the batch job requires store level processing. |
enabled |
Boolean |
Y indicates the batch job is currently enabled and scheduled. This will not prevent batch execution via this service. |
lastExecutionTime |
String |
The timestamp of the last execution of the batch job. |
updateDate |
String |
The last time this record was updated by SIOCS. |
Additional Data Definitions
Batch Type
Value |
Definition |
1 |
Cleanup |
2 |
Operation |
3 |
System |
4 |
System Cleanup |
5 |
Data Seed |
6 |
Archive |
Batch Interval Type
Value |
Definition |
1 |
30 Minutes |
2 |
1 Hour |
3 |
2 Hours |
4 |
3 Hours |
5 |
4 Hours |
6 |
6 Hours |
7 |
8 Hours |
8 |
12 Hours |
9 |
24 Hours |
10 |
Monthly |
REST Service: Differentiator
This service integrates differentiator foundation data. Asynchronous differentiator integration is processed through staged messages and is controlled by the MPS Work Type: DcsDiff.
This service replaces the RIB flow for differentiators.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/differentiators
API Definitions
API |
Description |
importDifferentiatorTypes |
Create or update differentiator types. |
importDifferentiators |
Create or update differentiators. |
deleteDifferentiatorType |
Delete a differentiator type and all associated differentiators. |
deleteDifferentiator |
Delete a differentiator. |
API: Import Differentiator Types
Imports a differentiator type by writing a staged message and processing through DCS consumer.
If the number of records exceed 1000, an input too large error is returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/types/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Differentiator Type information |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
differenatiorTypes |
List of details |
Yes |
The differentiator types to import. |
Detail Data Definition
differenatiorTypeId |
String (10) |
Yes |
The differentiator type identifier. |
description |
String (255) |
Yes |
The differentiator type description (not translated). |
API: Import Differentiators
Imports a differentiator.
If the number of records exceed 1000, an input too large error is returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Differentiator information |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
differenatiors |
List of details |
Yes |
The differentiators to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
differenatiorId |
String (10) |
X |
The differentiator type identifier. |
differenatiorTypeId |
String (10) |
X |
The differentiator type identifier. |
description |
String (255) |
X |
The differentiator type description (not translated). |
API: Delete Differentiator Type
Deletes a differentiator type and all associated differentiators.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/types/{differenatiorTypeId}/delete |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
None |
Output |
None |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
differentiatorId |
The differentiator Id to be removed |
REST Service: Finisher
This service integrates finisher and finisher item foundation data. Asynchronous finisher integration is processed through staged messages and is controlled by the MPS Work Type: DcsPartner. Asynchronous finisher item integration is processed through staged messages and is controlled by the MPS Work Type: DcsItemLocation.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/finishers
API Definitions
API |
Description |
importFinishers |
Imports a collection of finishers. |
deleteFinisher |
Deletes a finisher. |
importItems |
Imports a collection of finisher items. |
removeItems |
Marks finisher items for deletion. |
API: Import Finishers
Imports finishers. This allows 500 finishers per service call.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of finisher import |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
finishers |
List of details |
Yes |
A list of finishers to import |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
finisherId |
Long (10) |
Yes |
The finisher identifier. |
name |
String (240) |
The finisher name. |
|
status |
Integer |
Finisher Import Status (see Additional Data Definition). |
|
currencyCode |
String (3) |
ISO currency code used by the finisher |
|
countryCode |
String (3) |
The ISO country code assigned to the finisher. |
|
languageCode |
String (6) |
The ISO language code of the finisher |
|
contactName |
String (120) |
Name of the finisher's representative contact. |
|
contactPhone |
String (20) |
Phone number of the finisher's representative contact. |
|
contactFax |
String (20) |
Fax number of the finisher's representative contact. |
|
contactTelex |
String (20) |
Telex number of the finisher's representative contact. |
|
contactEmail |
String (100) |
Email address of the finisher's representative contact. |
|
manufacturerId |
String (18) |
Manufacturer's identification number |
|
taxId |
String (18) |
Tax identifier number of the finisher. |
|
transferEntityId |
String (20) |
Identifier of the transfer entity that the finisher belongs to. |
|
paymentTerms |
String (20) |
Payment terms for the partner |
|
importCountryCode |
String (3) |
The ISO country code of the import authority. |
|
importPrimary |
Boolean |
True Indicates the code is the primary import authority of the import country. |
API: Delete Finisher
Deletes a finisher.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{finisherId}/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
None |
|
Output |
None |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
finisherId |
The finisher identifier to be removed. |
API: Import Items
Imports finisher items. This allows 5000 items per service call.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{finisherId}/items/import |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Finisher Item list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of details |
Yes |
A list of items to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The item identifier. |
status |
Integer |
Yes |
Finisher Item Import Status (see Additional Data Definition). |
API: Remove Items
Marks finisher items for later deletion. This allows 5000 items per service call.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{finisherId}/items/remove |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items list |
Output |
None |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
finisherId |
The finisher identifier to be removed. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
itemIds |
List<String> |
Yes |
A list of items to remove. |
Additional Data Definitions
Finisher Import Status
Value |
Definition |
1 |
Active |
2 |
Inactive |
Finisher Item Import Status
Value |
Definition |
1 |
Active |
2 |
Discontinued |
3 |
Inactive |
REST Service: Inventory Adjustment
This service defines operations to manage Inventory Adjustment information.
Service Base URL
The Cloud service base URL follows the format:
https://external_load_balancer/cust_env/siocs-int-services/api/invadjustments
APIs
API |
Description |
Find Adjustments |
Search for transactional store inventory adjustments summary headers based on input criteria. |
Read Adjustment |
Reads a transaction store inventory adjustment. |
Update Adjustment |
This API updates the details of an inventory adjustment that is currently "In Progress." |
Confirm Adjustment |
This API is used to confirm an inventory adjustment, finalizing it and processing the inventory movement. |
Cancel Adjustment |
This API is used to cancel an inventory adjustment. |
Create Adjustment |
This API is used to create a new manual inventory adjustment whose status is In Progress. |
API: Find Adjustments
API is used to search for transaction headers for inventory adjustments. No items or detailed information is returned via this service.
API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
Collection of adjustments |
Max Response Limit |
10,000 |
Query Params
Attribute |
Type |
Req |
Definition |
referenceId |
Long(12) |
No |
Return adjustments containing this reference identifier. |
status |
Integer(2) |
No |
Returns adjustments containing this status: See Index. |
adjustmentDateFrom |
Date |
No |
Returns adjustments where the adjustment date is on or after this date. |
adjustmentDateTo |
Date |
No |
Returns adjustments where the adjustment date is on or before this date. |
updateDateFrom |
Date |
No |
Returns adjustments where the adjustment date is on or after this date. |
updateDateTo |
Date |
No |
Returns adjustments where the adjustment date is on or before this date. |
itemId |
String(25) |
No |
Returns adjustments containing this item. |
reasonId |
Long(12 |
No |
Returns adjustments containing this reason code. |
Output Data Definition
Attribute |
Type |
Definition |
adjustmentId |
Long(12) |
Adjustment identifier |
storeId |
Long(10) |
Store Identifier |
referenceId |
Long(12) |
Identifier of original adjustment it was copied from |
externalId |
String(128) |
A unique identifier of the adjustment in an external system |
adjustmentDate |
Date |
The timestamp the inventory is official considered to have been adjusted |
status |
Integer(2) |
The adjustment status: See Index. |
createDate |
Date |
The date the adjustment was created in EICS |
updateDate |
Date |
The date the adjustment was last updated by EICS |
approveDate |
Date |
The date the adjustment was approved in EICS |
API: Read Adjustment
This API is used to read an inventory adjustment.
API Basics
Endpoint URL |
{base URL}/{adjustmentId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
Inventory Adjustment |
Max Response Limit |
N/A |
Path Parameter Definition
Payload |
Type |
Definition |
adjustmentId |
Long(12) |
The unique inventory adjustment identifier |
Output Data Definition
Attribute |
Type |
Definition |
adjustmentId |
Long(12) |
The unique inventory adjustment identifier |
storeId |
Long(10 |
The unique identifier of the store |
referenceId |
Long(12) |
Identifier of original adjustment it was copied from |
externalId |
String(128) |
Identifier to the adjustment in an external system |
adjustmentDate |
Date |
The timestamp the inventory is official considered to have been adjusted |
status |
Integer(2) |
The adjustment status: See Index |
comments |
String(2000) |
Comments associated to the adjustment |
createDate |
Date |
The date the adjustment was created by EICS |
createUser |
String (128) |
The user that created the adjustment in EICS |
updateDate |
Date |
The date the adjustment was last updated by EICS |
updateUser |
String (128) |
The user that last updated the adjustment in EICS |
approveDate |
Date |
The date the adjustment was approved in EICS |
approveUser |
String (128) |
The user that approved the adjustment in EICS |
externalCreateUser |
String (128) |
The non-EICS user that created the inventory adjustment in the external system |
externalUpdateUser |
String (128) |
The non-EICS user that updated the inventory adjustment in the external system |
lineItems |
Collection |
A collection of Inventory Adjustment Line Items |
Inventory Adjustment Line Item
Payload |
Type |
Definition |
lineId |
Long(12) |
The unique SIOCS identifier of the line item |
itemId |
Long(25) |
The sku level item identifier |
reasonId |
Long(12) |
The unique internal identifier of the reason code |
caseSize |
BigDecimal(10,2) |
Case size associated to the line item |
quantity |
BigDecimal(20,4) |
Quantity associated to the line item |
uins |
Collection{String} |
The UINs associated to the item quantities |
API: Update Adjustment
This API updates the details of an inventory adjustment that is currently "In Progress"
API Basics
Endpoint URL |
{base URL}/{adjustmentId} |
Method |
POST |
Successful Response |
204 OK |
Processing Type |
|
Input |
Inventory Adjustment |
Output |
N/A |
Max Response Limit |
Path Parameter Definition
Payload |
Type |
Definition |
adjustmentId |
int12 |
The unique inventory adjustment identifier. |
Input Data Definition
Attribute |
Type |
Req |
Definition |
referenceId |
Big Decimal(12) |
Identifier of original adjustment from which it was copied. |
|
externalUser |
String(128) |
A non-EICS user that created the inventory adjustment in the external system. |
|
adjustmentDate |
Date |
The timestamp the inventory is officially considered to have been adjusted. If left blank, it will default to the creation date of the record. |
|
comments |
String(2000) |
Comments associated to the adjustment. These comments will replace any previous comments. Comments will be trimmed down to 2000 characters if the entry is larger. |
|
lineItems |
Array |
X |
A collection of line items attached to the adjustment. |
Inventory Adjustment Line Item
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The SKU level item identifier. If SKU is already present, its line item will be updated. Otherwise, a new line item will be added. |
reasonId |
BigDecimal(12) |
X |
The unique identifier of the reason code associated to the adjustment. The reason code is not modifiable on an update, but it will be used if a new item is added. |
quantity |
BigDecimal(20,4) |
X |
The quantity of this line item. For UINS, the quantity must match the number of UINs. If the quantity is set to 0, the system will attempt to remove the line item from the adjustment |
caseSize |
BigDecimal(10,2) |
Case size associated to the line item |
|
uins |
array{String}(128) |
The UINs to attach to this line item. |
Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request Example:
|
API: Confirm Adjustment
This API is used to confirm an inventory adjustment, finalizing it and processing the inventory movement.
API Basics
Endpoint URL |
{base URL}/{adjustmentId}/confirm |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
Path Parameter Definition
Payload |
Type |
Definition |
adjustmentId |
Long(12) |
The unique inventory adjustment identifier |
API: Cancel Adjustment
This API is used to cancel an inventory adjustment.
API Basics
Endpoint URL |
{base URL}/{adjustmentId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
Path Parameter Definition
Payload |
Type |
Definition |
adjustmentId |
Long(12) |
The unique inventory adjustment identifier |
API: Create Adjustment
This API is used to create a new manual inventory adjustment whose status is In Progress.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Inventory Adjustment |
Output |
Inventory Adjustment Reference |
Max Response Limit |
5,000 Items |
Input Data Definition
Attribute |
Type |
Req |
Definition |
storeId |
Long(10) |
X |
Store Identifier |
referenceId |
Long(12) |
Identifier of original adjustment it was copied from |
|
externalId |
String(128) |
A unique identifier to the adjustment in an external system |
|
adjustmentDate |
Date |
The timestamp the inventory is official considered to have been adjusted |
|
externalUser |
String(128) |
A non-EICS user that created the inventory adjustment in the external system |
|
comments |
String(2000) |
Comments associated to the inventory adjustment |
|
lineItems |
Collection |
X |
collection of inventory adjustment line items |
Inventory Adjustment Line Item
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The sku level item identifier. It must be an inventoriable item. |
reasonId |
Long(12) |
X |
The unique internal identifier of the reason code. |
quantity |
BigDecimal(20,4) |
X |
Quantity associated to the line item |
caseSize |
BigDecimal(10,,2) |
Case size associated to the line item |
|
uins |
Collection{String} |
The UINs associated to the item quantities |
Output Data Definition
Attribute |
Type |
Definition |
adjustmentId |
Long(12) |
The newly created adjustment identifier |
Example Input
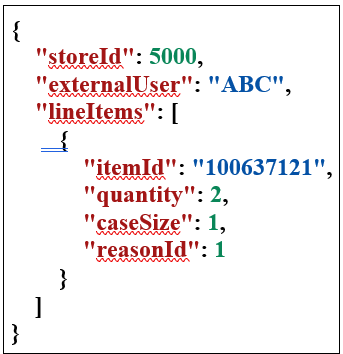
Business Data Errors
Error |
Definition |
CASE_SIZE_NOT_WHOLE |
The adjustment requires a non-decimal case size. |
EXISTING_UIN_CANNOT_BE_ADDED |
An already existing UIN cannot be added to the store. |
NON_INVENTORIABLE_ITEM |
The item cannot be adjusted because it cannot have inventory. |
NON_MANAGED_STORE |
The store does not manage its inventory. |
QUANTITY_NOT_WHOLE |
The adjustment requires a non-decimal quantity. |
Additional Date Definition
Inventory Adjustment Status
ID |
Status |
1 |
In Progress |
2 |
Completed |
3 |
Canceled |
REST Service: Item
This service integrates the item foundation data with an external application. Asynchronous item integration is processed through staged messages and is controlled by the MPS Work Types.
Note that this is item level foundational data. To lookup or access item information, use the item inquiry REST service.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/items
API Definitions
API |
Description |
importItems |
Import items into the system. |
removeItems |
Mark items for deletion at some point in the future when no records are using them. |
importHierarchies |
Imports item hierarchies into the system. |
removeHierarchies |
Marks hierarchies for deletion at some point in the future when no records are using them. |
importRelatedItems |
Imports the associations of related items. |
deleteRelatedItems |
Deletes an association of related items. |
importImageUrls |
Imports image URLs associated to the item. |
deleteImageUrls |
Delete image URLs associated to the item. |
API: Import Items
Imports items.
This flow is managed in MPS system with the following family: DcsItem.
If the input exceeds more than 100 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of Items to import |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
finishers |
List of details |
Yes |
A list of items to import |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The unique item identifier (sku number). |
transactionLevel |
Long |
Yes |
Number indicating which of the three levels transactions occur for the item. Items may only be used on transactions with inventory tracked if the transaction level and item level match. |
itemLevel |
Long |
Yes |
Number indicating which of the three levels an item resides at. Items may only be used on transactions with inventory tracked if the transaction level and item level match. |
departmentId |
Long (12) |
Yes |
The merchandise hierarchy department identifier. |
classId |
Long (12) |
The merchandise hierarchy class identifier. |
|
subclassId |
Long (12) |
The merchandise hierarchy subclass identifier. |
|
shortDescription |
String (255) |
A short description of the item. |
|
longDescription |
String (400) |
A long description of the item. |
|
differentiator1 |
String (10) |
The first differentiator identifier. |
|
differentiator2 |
String (10) |
The second differentiator identifier. |
|
differentiator3 |
String (10) |
The third differentiator identifier. |
|
differentiator4 |
String (10) |
The fourth differentiator identifier. |
|
status |
Integer |
Item Import Status (see Additional Data Definition). |
|
parentId |
String (25) |
The unique identifier of the item at the next level above this item. |
|
pack |
Boolean |
True if the item is pack, false otherwise. |
|
simplePack |
Boolean |
True if the item is a simple pack, false otherwise. |
|
sellable |
Boolean |
True if the item is sellable, false otherwise. |
|
orderable |
Boolean |
True if the item can be ordered from a supplier, false otherwise. |
|
shipAlone |
Boolean |
True if the item must be shipped in separated packaging, false otherwise. |
|
inventoriable |
Boolean |
True if the item is inventoried, false otherwise. |
|
notionalPack |
Boolean |
True indicates the inventory is held at the component level. All notional pack are marked as inventoriable in SIOCS. |
|
estimatePackInventory |
Boolean |
True if the item allows estimating pack inventory from component positions, false otherwise. |
|
primaryReferenceItem |
Boolean |
True indicates it the primary sub-translation level item. |
|
orderAsType |
Boolean |
True indicates a buyer pack is receivable at the pack level. N means at the component level. |
|
standardUom |
String (4) |
The unit of measure that inventory is tracked in. |
|
packageUom |
String (4) |
The unit of measure associated with a package size. |
|
packageSize |
Double |
The size of the product printed (will be printed on the label). |
|
eachToUomFactor |
BigDecimal |
The multiplication factor to convert 1 EA to the equivalent standard unit of measure. |
|
barcodeFormat |
String (4) |
The format of a barcode (used for Type 2 barcode items). |
|
barcodePrefix |
Long (9) |
The barcode prefix used in association with the Type 2 barcode of this item. |
|
wastageType |
Integer |
Waste Type (see Additional Data Definition). |
|
wastagePercent |
BigDecimal |
Wastage percent. |
|
wastagePercentDefault |
BigDecimal |
Default wastage percent. |
|
suggestedRetailCurrency |
String (3) |
The currency of the manufacturer suggested retail price. |
|
suggestedRetailPrice |
BigDecimal |
Manufacturer suggested retail price. |
|
brand |
String (30) |
Brand name of the brand the item belongs to. |
|
brandDescription |
String (120) |
Brand description of the brand the item belongs to. |
|
components |
List of components |
A list of components for the item (if the item is a pack) |
Component Data Definition
Attribute |
Data Type |
Required |
Description |
componentItemId |
String (25) |
Yes |
The item identifier of the component item within the pack. |
quantity |
BigDecimal |
Yes |
The quantity of component item within the pack |
API: Remove Items
Deactivate items.
This flow is managed in MPS system with the following family: DcsItem.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/remove |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Items list to remove |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of details |
Yes |
A list of item reference to update to a non-active status. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The unique item identifier |
status |
Integer |
Yes |
Item Remove Status (see Additional Data Definition). |
API: Import Hierarchies
Imports item hierarchies.
This flow is managed in MPS system with the following family: DcsHierarchy.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/hierarchies/import |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items hierarchy list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
hierarchies |
List of details |
Yes |
The hierarchies to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
departmentId |
Long (12) |
Yes |
The hierarchy department identifier. |
departmentName |
String (360) |
The hierarchy department name. |
|
classId |
Long (12) |
The hierarchy class identifier. |
|
className |
String (360) |
The hierarchy class name. |
|
subclassId |
Long (12) |
The hierarchy subclass identifier. |
|
subclassName |
String (360) |
The hierarchy subclass name. |
API: Remove Hierarchies
Deactivates item hierarchies. Once no information is associated to the item hierarchies, a cleanup batch will remove them from the database.
This flow is managed in MPS system with the following family: DcsHierarchy.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/hierarchies/remove |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items hierarchy list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
hierarchies |
List of details |
Yes |
The images to remove. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
departmentId |
Long (12) |
Yes |
The hierarchy department identifier. |
classId |
Long (12) |
The hierarchy class identifier. |
|
subclassId |
Long (12) |
The hierarchy subclass identifier |
API: Import Related Items
Imports item relationships that an item may belong to.
This flow is managed in MPS system with the following family: DcsItem.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/related/import |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items Relationship list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
relationships |
List of details |
Yes |
The relationships to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
relationshipId |
Long (20) |
Yes |
The identifier of the relationship. |
relationshipType |
Integer |
Yes |
Relationship Type (see Additional Data Definitions). |
name |
String (120) |
The name of the relationship. |
|
itemId |
String (25) |
Yes |
The item whose related records are being recorded. |
mandatory |
Boolean |
Yes |
True if the relationships are mandatory. |
relatedItems |
List of related items |
Yes |
The related items. |
Related Item Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The item that is related. |
effectiveDate |
Date |
Date at which this relationship becomes active. |
|
endDate |
Date |
Last date at which this relationship is active. |
|
priorityNumber |
Long (4) |
Number defining priority in the case of multiple substitute items. |
API: Delete Related Items
Deletes relationships between items.
This flow is managed in MPS system with the following family: DcsItem.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/related/delete |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
None |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
relationshipIds |
List<Long> |
Yes |
The relationship identifiers to remove. |
API: Import Image Urls
Import image URLs associated to the item.
This flow is managed in MPS system with the following family: DcsItem.
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/images/import |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items Image list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
images |
List of details |
Yes |
The images to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The item identifier/sku number. |
imageType |
Integer |
Yes |
Image Type (see Additional Data Definitions). |
storeId |
Long (10) |
The store identifier. This is required only if the image type is QR_CODE. |
|
imageName |
String (120) |
Yes |
The name of the image. |
imageSize |
String (6) |
The size of the image: (T) thumbnail. Other than (T), any text is accepted and there is no definition to validate against, but the text has no meaning. |
|
url |
String (1000) |
Yes |
The universal resource locator of the image. |
displaySequence |
Integer (2) |
The sequence the item should be displayed in. |
|
startDate |
Date |
The date the image becomes active. |
|
endDate |
Date |
The date the image ceases being active. |
API: Delete Image Urls
Deletes image URLs.
This flow is managed in MPS system with the following family: DcsItem
If the input exceeds more than 500 records, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/images/delete |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Items Image list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
images |
List of details |
Yes |
The images to remove. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The item identifier/sku number. |
imageName |
String (120) |
Yes |
The name of the image. |
imageType |
Integer |
Yes |
Image Type (see Additional Data Definitions). |
Additional Data Definitions
Item Status
Value |
Definition |
1 |
Active |
2 |
Discontinued |
3 |
Inactive |
4 |
Deleted |
5 |
Auto Stockable |
6 |
Non Ranged |
Item Import Status
Value |
Definition |
1 |
Active |
5 |
Auto Stockable |
6 |
Non Ranged |
Item Remove Status
Value |
Definition |
2 |
Discontinued |
3 |
Inactive |
4 |
Deleted |
Wastage Type
Value |
Definition |
1 |
Sales Wastage |
2 |
Spoilage Wastage |
Relationship Type
Value |
Definition |
1 |
Related |
2 |
Substitute |
3 |
Up-Sell |
4 |
Cross-Sell |
Image Type
Value |
Definition |
1 |
Image |
2 |
QRCode |
REST Service: Item Inquiry
This service allows the customer to retrieve information about items. These services are intended to find item themselves and do not retrieve inventory. For inventory queries, see inventory inquiry services.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/iteminquiries
API Definitions
API |
Description |
findItemBySearchScan |
Searches for summary item information based on basic item scan information such as UPC, barcode, and so on. |
findItemBySource |
Searches for summary item information based on hierarchy or source location search criteria. |
findItems |
Searches item information based on multiple items (and an optional store). |
findItemsByScan() |
Searches for item information by an unknown identifier, most likely a scan. It first searches for the item using the searchScan as a potential item, and if found will return records based on that. It then searches as a UPC, barcode, or UIN, in that order, halting if it finds potential matches. An optional store identifier can be included as well. |
findItemsByInventory |
Searches for summary information about an item based on search criteria, primarily inventory information. |
findRelatedItems() |
Search for summary information about an item based on search criteria, primarily parent item. |
findItemsByUDA() |
Search for summary information about an item based on search criteria, primarily user defined attributes (UDA). |
API: Find Item by Search Scan
Search for item information by on unknown identifier, most likely a scan. It first searches for the item using the scan itself as the potential item, and if found will return record(s). It then searches as a UPC, barcode, or UIN in that order halting if it finds potential matches.
API Basics
Endpoint URL |
{base URL}/scan/{searchScan} |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of items scanned |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Description |
searchScan |
An Item, UPC, Barcode or UIN take from a scan. |
Query Parameter Definition
Attribute |
Data Type |
Required |
Description |
storeId |
Long |
A store to verify if found item is ranged to. |
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String |
The item identifier. |
type |
Integer |
Item Type (see Additional Data Definition). |
status |
String |
Item Status (see Additional Data Definition). |
shortDescription |
String |
A short description of the item. |
longDescription |
String |
A long description of the item. |
departmentId |
Long |
The identifier of the department the item belongs to. |
classId |
Long |
The identifier of the class the item belongs to. |
subclassId |
Long |
The identifier of the subclass the item belongs to. |
storeId |
Long |
The store identifier passed in as the query parameter. |
ranged |
Boolean |
True if the item is ranged to the requested store, false otherwise. |
API: Find Item by Source
Searches for summary information about an item based on search criteria, primarily hierarchy and source location.
If the number of items found exceeds 10000, a maximum limit error will be returned. Additional or more limiting search criteria will be required.
At least one query parameter is required. If source type location is entered, then a source id for that type must also be entered.
API Basics
Endpoint URL |
{base URL}/source |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of Items summary |
|
Max Response Limit |
N/A |
Query Parameter Definition
Attribute |
Data Type |
Description |
description |
String |
Include only items with description text matching this description. |
sourceType |
Integer |
Include only items available from this source type (see Additional Data Definition). |
sourceId |
String |
Include only items available from this source identifier. |
departmentId |
Long |
Include only items associated to this merchandise hierarchy department. |
classId |
Long |
Include only items associated to this merchandise hierarchy class. |
subclassId |
Long |
Include only items associated to this merchandise hierarchy subclass. |
storeId |
Long |
Include only items ranged to this particular store. |
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String |
The item identifier. |
type |
Integer |
Item Type (see Additional Data Definition). |
status |
String |
Item Status (see Additional Data Definition). |
shortDescription |
String |
A short description of the item. |
longDescription |
String |
A long description of the item. |
departmentId |
Long |
The identifier of the department the item belongs to. |
classId |
Long |
The identifier of the class the item belongs to. |
subclassId |
Long |
The identifier of the subclass the item belongs to. |
API: Find Items
Searches for detailed information about the items using the specified input.
If the number of items found exceeds 10000, a maximum limit error will be returned. Additional or more limiting input criteria will be required.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Criteria |
Output |
List of Items |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
storeId |
Long |
Yes |
The store to retrieve item information for |
itemIds |
List<String> |
Yes |
A list of items to retrieve item information for |
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String |
The unique item identifier (sku number). |
transactionLevel |
Long |
Number indicating which of the three levels transactions occur for the item. Items may only be used on transactions with inventory tracked if the transaction level and item level match. |
itemLevel |
Long |
Number indicating which of the three levels an item resides at. Items may only be used on transactions with inventory tracked if the transaction level and item level match. |
departmentId |
Long |
The merchandise hierarchy department identifier. |
classId |
Long |
The merchandise hierarchy class identifier. |
subclassId |
Long |
The merchandise hierarchy subclass identifier. |
shortDescription |
String |
A short description of the item. |
longDescription |
String |
A long description of the item. |
differentiator1 |
String |
The first differentiator identifier. |
differentiator2 |
String |
The second differentiator identifier. |
differentiator3 |
String |
The third differentiator identifier. |
differentiator4 |
String |
The fourth differentiator identifier. |
status |
Integer |
The status (see Additional Data Definition). |
parentId |
String |
The unique identifier of the item at the next level above this item. |
pack |
Boolean |
True if the item is pack, false otherwise. |
simplePack |
Boolean |
True if the item is a simple pack, false otherwise. |
sellable |
Boolean |
True if the item is sellable, false otherwise. |
orderable |
Boolean |
True if the item can be ordered from a supplier, false otherwise. |
shipAlone |
Boolean |
True if the item must be shipped in separated packaging, false otherwise. |
inventoriable |
Boolean |
True if the item is inventoried, false otherwise. |
notionalPack |
Boolean |
True indicates the inventory for the pack is tracked at the component level. |
estimatePackInventory |
Boolean |
True if the item allows estimating pack inventory from component positions, false otherwise. |
primaryReferenceItem |
Boolean |
True indicates it the primary sub-translation level item. |
orderAsType |
Boolean |
True indicates a buyer pack is receivable at the pack level. N means at the component level. |
standardUom |
String |
The unit of measure that inventory is tracked in. |
packageUom |
String |
The unit of measure associated with a package size. |
packageSize |
Double |
The size of the product printed (will be printed on the label). |
eachToUomFactor |
BigDecimal |
The multiplication factor to convert 1 EA to the equivalent standard unit of measure. |
barcodeFormat |
String |
The format of a barcode (used for Type 2 barcode items). |
barcodePrefix |
Long |
The barcode prefix used in association with the Type 2 barcode of this item. |
wastageType |
Integer |
Type of wastage (see Additional Data Definition). |
wastagePercent |
BigDecimal |
Wastage percent. |
wastagePercentDefault |
BigDecimal |
Default wastage percent. |
suggestedRetailCurrency |
String |
The currency of the manufacturer suggested retail price. |
suggestedRetailPrice |
BigDecimal |
Manufacturer suggested retail price. |
brand |
String |
Brand name of the brand the item belongs to. |
brandDescription |
String |
Brand description of the brand the item belongs to. |
createDate |
Date |
The date the item was created in EICS. |
updateDate |
Date |
The last date the item was updated in EICS. |
(RANGED INFO)
storeId |
Long |
The store identifier. |
status |
String |
The item status. (see Additional Data Definition). |
primarySupplierId |
Long |
The unique identifier of the primary supplier of the item to this store location. |
storeControlPricing |
Boolean |
True indicates the item price can be controlled by the store. |
rfid |
Boolean |
True indicates the item is RFID tagged. |
defaultCurrencyCode |
String |
The default currency of the item's price at this store. |
purchaseType |
Long |
Purchase Type (see Additional Data Definition). |
uinType |
Integer |
UIN Type (see Additional Data Definition). |
uinCaptureTime |
Integer |
UIN Capture Time (see Additional Data Definition). |
uinLabelId |
Long |
The UIN label unique identifier. |
uinExternalCreateAllowed |
Boolean |
True if an external system can create a UIN, false otherwise. |
replenishmentMethod |
String |
The replenishment method: (SO) Store Orders (has meaning), otherwise meaningless text. |
rejectStoreOrder |
Boolean |
True indicates store orders must be on or after the next delivery date or should be rejected. |
multipleDeliveryPerDayAllowed |
Boolean |
True indicates the item allows multiple deliveries per day at the location. |
nextDeliveryDate |
Date |
The next delivery date of the time based on its replenishment type. |
Additional Data Definitions
Item Status Type
Value |
Definition |
1 |
Active |
2 |
Discontinued |
3 |
Inactive |
4 |
Deleted |
5 |
Auto Stockable |
6 |
Non Ranged |
Item Type
Value |
Definition |
1 |
Item |
2 |
Simple Pack |
3 |
Complex Pack |
4 |
Simple Breakable Pack |
5 |
Complex Breakable Pack |
Source Type
Value |
Definition |
1 |
Supplier |
2 |
Warehouse |
3 |
Finisher |
Item Purchase Type
Value |
Definition |
1 |
Consignment |
2 |
Concession |
Item UIN Type
Value |
Definition |
1 |
Serial |
2 |
AGSN |
Item UIN Capture Time Type
Value |
Definition |
1 |
Sale |
2 |
Store Receiving |
Item Wastage Type
Value |
Definition |
1 |
Sales Wastage |
2 |
Spoilage |
API: Find Items by Scan
This API searches for item information by an unknown identifier, most likely a scan. It first searches for the item using the searchScan as a potential item, and if found will return records based on that. It then searches as a UPC, barcode, or UIN, in that order, halting if it finds potential matches. An optional store identifier can be included as well.
API Basics
Endpoint URL |
{base URL}/scan |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
|
Input |
Criteria |
Output |
List of Items |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
searchScan |
String(128) |
Yes |
A scan to find matching items. |
storeId |
Long |
No |
The store to retrieve item information for. |
Output Data Definition
Table 4-7 Good Response (Code 200)
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
The short description of the item |
longDescription |
String(400) |
The long description of the item |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to |
storeId |
Integer(100) |
The store identifier (if passed in as a query parameter) |
ranged |
Boolean |
True if the item is ranged to the requested store, false otherwise. |
Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
API: Find Items by Inventory
Search for summary information about an item based on search criteria, primarily inventory information.
API Basics
Endpoint URL |
{base URL}/inventory |
|
Method |
GET |
|
Successful Response |
200 Successful |
|
Processing Type |
||
Input |
Query Parameters |
|
Output |
Inventory information |
|
Max Response Limit |
Query Parameter Definition
Attribute |
Data Type |
Description |
available |
boolean |
Include only items with positive available inventory. |
unavailable |
boolean |
Include only items with positive unavailable inventory. |
nonsellableTypeId |
integer(12) |
Include only items with this non-sellable quantity type. |
departmentId |
integer(12) |
Include only items associated to this merchandise hierarchy department. |
classId |
integer(12) |
Include only items associated to this merchandise hierarchy class. |
subclassId |
integer(12) |
Include only items associated to this merchandise hierarchy subclass. |
storeId |
integer(12) |
Include only items ranged to this particular store. |
Output Data Definition
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier. |
storeId |
Integer(10) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
A short description of the item. |
longDescription |
String(400) |
A long description of the item. |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to. |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to. |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to. |
Table 4-8 Good Response (Code 200)
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier |
storeId |
String(25) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
The short description of the item |
longDescription |
String(400) |
The long description of the item |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to |
Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
API: Find Related Items
Search for summary information about an item based on search criteria, primarily parent item.
API Basics
Endpoint URL |
{base URL}/related |
|
Method |
GET |
|
Successful Response |
200 Successful |
|
Processing Type |
||
Input |
Query Parameters |
|
Output |
Item information |
|
Max Response Limit |
Query Parameter Definition
Attribute |
Data Type |
Description |
parentItemId |
string(25) |
Include only items related to this parent item identifier. |
storeId |
integer(10) |
Include only items ranged to this particular store. |
Output Data Definition
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier. |
storeId |
Integer(10) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
A short description of the item. |
longDescription |
String(400) |
A long description of the item. |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to. |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to. |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to. |
Table 4-9 Good Response (Code 200)
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier |
storeId |
String(25) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
The short description of the item |
longDescription |
String(400) |
The long description of the item |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to |
Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
API:Find Items by UDA
Search for summary information about an item based on search criteria, primarily user defined attributes.
API Basics
Endpoint URL |
{base URL}/uda |
|
Method |
GET |
|
Successful Response |
200 Successful |
|
Processing Type |
||
Input |
Query Parameters |
|
Output |
Inventory information |
|
Max Response Limit |
Query Parameter Definition
Attribute |
Data Type |
Description |
udaId |
integer(5) |
Include only items with this user defined attribute identifier. |
udaText |
string(250) |
Include only items with this UDA text value |
udaLovId |
string(25) |
Include only items with a UDA value matching this list of values identifier. |
udaDateFrom |
date-time |
Include only items with a date UDA value on or after this date. |
udaDateTo |
date-time |
Include only items with a date UDA value on or before this date. |
storeId |
integer(12) |
Include only items ranged to this particular store. |
Output Data Definition
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier. |
storeId |
Integer(10) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
A short description of the item. |
longDescription |
String(400) |
A long description of the item. |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to. |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to. |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to. |
Table 4-10 Good Response (Code 200)
Attribute |
Data Type |
Description |
itemId |
String(25) |
The item identifier |
storeId |
String(25) |
The store identifier (if passed in a query parameter) |
type |
Integer(2) |
The item type. Valid values are< br/> 1 - Item< br/ 2> - Simple Pack< br/ 3> - Complex Pack< br/ 4> - Simple Breakable Pack < br/ 5> - Complex Breakable Pack Enum: 1 2 3 4 5 example: 1 |
status |
Integer(2) |
The status of the item Valid values are< br/> 1 - Active < br/ 2> - Discontinued < br/ 3> - Inactive < br/ 4> - Deleted < br/ 5> - Auto Stockable <br/> Non-Ranged Enum: 1 2 3 4 5 example: 1 |
shortDescription |
String(255) |
The short description of the item |
longDescription |
String(400) |
The long description of the item |
departmentId |
BigDecimal(12) |
The identifier of the department the item belongs to |
classId |
BigDecimal(12) |
The identifier of the class the item belongs to |
subclassId |
BigDecimal(12) |
The identifier of the subclass the item belongs to |
Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
REST Service: Item Inventory
This service retrieves information about item inventory.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/inventory
API Definitions
API | Description |
---|---|
Find Available Inventory |
Search for available inventory information by multiple items and multiple locations. |
Find Inventory |
Searches for standard inventory information by multiple items and multiple locations. |
Find Expanded Inventory |
Searches for expanded inventory information by multiple items at a single store. |
Find Future Inventory |
Searches for future inventory delivery information by a single item and a single store. |
Find Inventory In Buddy Stores |
Searches for inventory information at buddy stores by single input store and multiple items. |
Find Inventory In Transfer Stores |
Searches for inventory information at transfer zone stores by single input store and multiple items. |
API: Find Available Inventory
Searches for available inventory quantity about an item in requested locations. Only transaction-level items are processed, and only current available inventory is returned. The multiplied combination of items and locations within the input criteria cannot exceed 10,000. Invalid items or locations will not cause this API to fail. Inventory is returned for any item and locations found and is not returned invalid or not found items or locations.
API Basics
Endpoint URL |
{base URL}/available |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Criteria |
Output |
List of items |
Max Response Limit |
10,000 |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemIds |
List of Strings |
Yes |
A list of items to retrieve available inventory for. |
locationIds |
List of Longs |
Yes |
A list of location identifiers to retrieve available inventory for. |
locationType |
Integer |
Yes |
A location type: See Location Type |
Example Input
{
"itemIds": [
"100637156",
"100637172",
"100653105"
],
"locationIds": [
5000,
5001,
5005
],
"locationType": 1
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String |
Yes |
The item identifier. |
locationId |
Long |
Yes |
The location identifier. |
locationType |
Integer |
Yes |
The location type: See Location Type. |
availableQuantity |
BigDecimal |
Yes |
The amount of available inventory. |
unitOfMeasure |
String |
Yes |
The unit of measure of the available inventory. |
estimatedPack |
Boolean |
Yes |
True if this is an estimated pack quantity, false otherwise. |
Example Output
[
{
"itemId": "100637113",
"locationId": 5000,
"locationType": 1,
"availableQuantity": 200.0000,
"unitOfMeasure": "EA",
"estimatedPack": false
},
{
"itemId": "100637113",
"locationId": 5001,
"locationType": 1,
"availableQuantity": 200.0000,
"unitOfMeasure": "EA",
"estimatedPack": false
},
}
Additional Data Definitions
Location Type
Value | Definition |
---|---|
1 |
Store |
2 |
Warehouse |
API: Find Inventory
Query lookup of detailed inventory information about a multiple item in multiple stores. The multiplied combination of items and locations within the input criteria cannot exceed 10,000. Invalid items or locations will not cause this API to fail. Inventory is returned for any item and locations found and is not returned invalid or not found items or locations.
API Basics
Endpoint URL |
{base URL}/positions |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Criteria |
Output |
List of inventory of item at stores |
Max Response Limit |
10,000 |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemIds |
List of Strings |
Yes |
A list of items to retrieve inventory for. |
storeIds |
List of Longs |
Yes |
A list of store identifiers to retrieve inventory for. |
sellingUnitOfMeasure |
Boolean |
- |
True indicates an attempt to use the selling unit of measure of the item, false indicates to use the standard unit of measure. If conversion cannot take place, it defaults back to standard unit of measure. |
Example Input
{
"itemIds": [
"100637156",
"100637172",
"100668091"
],
"storeIds": [
5000,
5001,
5002
],
"sellingUnitOfMeasure": true
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String |
Yes |
The item identifier. |
storeId |
Long |
The store identifier if the item is ranged to a store. |
|
ranged |
Boolean |
Yes |
True if the item is ranged to the store, false otherwise. |
estimated |
Boolean |
Yes |
True if the quantities are estimated, false otherwise. |
unitOfMeasure |
String |
Yes |
The unit of measure of the quantities. |
caseSize |
BigDecimal |
Yes |
The default case size of the item. |
quantityStockOnHand |
BigDecimal |
Yes |
The stock on hand quantity. |
quantityBackroom |
BigDecimal |
Yes |
The quantity located in the back room area. |
quantityShopfloor |
BigDecimal |
Yes |
The quantity located on the shop floor. |
quantityDeliveryBay |
BigDecimal |
Yes |
The quantity located in the delivery bay. |
quantityAvailable |
BigDecimal |
Yes |
The available to sell quantity. |
quantityUnavailable |
BigDecimal |
Yes |
The unavailable to sell quantity. |
quantityNonSellable |
BigDecimal |
Yes |
The total non-sellable quantity. |
quantityInTransit |
BigDecimal |
Yes |
The quantity currently in transit. |
quantityCustomerReserved |
BigDecimal |
Yes |
The quantity reserved for customer orders. |
quantityTransferReserved |
BigDecimal |
Yes |
The quantity reserved for transfers. |
quantityVendorReturn |
BigDecimal |
Yes |
The quantity reserved for vendor returns. |
nonSellableQuantities |
List of Non-Sellable Quantities |
- |
A collection containing the specific quantity in each non-sellable quantity type bucket. |
Non-Sellable Quantity Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
nonsellableTypeId |
Long |
Yes |
The non-sellable type unique identifier. |
quantity |
quantity |
Yes |
The quantity in this particular non-sellable type bucket. |
Example Output
[
{
"itemId": "100637156",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": 10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": 10.0000,
"quantityUnavailable": 0.0000,
"quantityNonSellable": 0.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000
},
{
"itemId": "100637172",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": -10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": -10.0000,
"quantityUnavailable": 20.0000,
"quantityNonSellable": 20.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000,
"nonSellableIdos": [
{
"nonsellableTypeId": 1,
"quantity": 15.0000
},
{
"nonsellableTypeId": 2,
"quantity": 5.0000
}
]
}
}
API: Find Expanded Inventory
Searches for expanded inventory information about multiple items within a single store.
API Basics
Endpoint URL |
{base URL}/{storeId}/expanded |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Criteria |
Output |
List of inventory of items |
Max Response Limit |
2,500 |
Path Parameter Definitions
Attribute | Description |
---|---|
storeId |
The store identifier of the store to process items for. |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemIds |
List of Strings |
Yes |
A list of items to retrieve expanded inventory for. |
Example Input
{
"itemIds": [
"100637156",
"100637172",
"100695081"
]
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String |
Yes |
The item identifier. |
storeId |
Long |
The store identifier if the item is ranged to a store. |
|
ranged |
Boolean |
Yes |
True if the item is ranged to the store, false otherwise. |
estimated |
Boolean |
Yes |
True if the quantities are estimated, false otherwise. |
unitOfMeasure |
String |
Yes |
The unit of measure of the quantities. |
caseSize |
BigDecimal |
Yes |
The default case size of the item. |
quantityStockOnHand |
BigDecimal |
Yes |
The stock on hand quantity. |
quantityBackroom |
BigDecimal |
Yes |
The quantity located in the back room area. |
quantityShopfloor |
BigDecimal |
Yes |
The quantity located on the shop floor. |
quantityDeliveryBay |
BigDecimal |
Yes |
The quantity located in the delivery bay. |
quantityAvailable |
BigDecimal |
Yes |
The available to sell quantity. |
quantityUnavailable |
BigDecimal |
Yes |
The unavailable to sell quantity. |
quantityNonSellable |
BigDecimal |
Yes |
The total non-sellable quantity. |
quantityInTransit |
BigDecimal |
Yes |
The quantity currently in transit. |
quantityCustomerReserved |
BigDecimal |
Yes |
The quantity reserved for customer orders. |
quantityTransferReserved |
BigDecimal |
Yes |
The quantity reserved for transfers. |
quantityVendorReturn |
BigDecimal |
Yes |
The quantity reserved for vendor returns. |
firstReceivedDate |
Date |
- |
The first date the item was received into stock. |
lastReceivedDate |
Date |
- |
The date the item last received inventory into stock. |
lastReceivedQuantity |
BigDecimal |
- |
Total amount of inventory received on the last date it was received. |
openStockCounts |
Integer |
- |
The number of stock counts open for the item at this store. |
lastStockCountType |
Integer |
- |
The type of stock count (see Additional Data Definition). |
lastStockCountApprovedDate |
Date |
- |
The date this item was last approved on a stock count at this store. |
lastStockCountTimeframe |
Integer |
- |
The stock count timeframe (see Additional Data Definition). |
uinProblemLine |
Boolean |
Yes |
True indicates it is UIN problem line item, false otherwise. |
lastRequestedQuantity |
BigDecimal |
- |
The quantity last requested for this item. |
lastUpdateDate |
Date |
Yes |
The timestamp of the last time this record was updated. |
nonSellableQuantities |
Collection of Non-Sellable Quantities |
- |
The specific quantities in each non-sellable quantity type bucket. |
Non-Sellable Quantity Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
nonsellableTypeId |
Long |
Yes |
The non-sellable type unique identifier. |
quantity |
quantity |
Yes |
The quantity in this particular non-sellable type bucket. |
Example Output
[
{
"itemId": "100637113",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 200.0000,
"quantityBackroom": 200.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": 200.0000,
"quantityUnavailable": 0.0000,
"quantityNonSellable": 0.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000,
"quantityLastReceived": 0.0000,
"quantityLastRequested": 0.0000,
"openStockCounts": 0,
"lastStockCountTimeframe": 3,
"uinProblemLine": false,
"lastUpdateDate": "2022-07-15T06:23:27-05:00"
},
{
"itemId": "100637121",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 200.0000,
"quantityBackroom": 180.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": 180.0000,
"quantityUnavailable": 20.0000,
"quantityNonSellable": 20.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000,
"quantityLastReceived": 0.0000,
"quantityLastRequested": 0.0000,
"openStockCounts": 0,
"lastStockCountTimeframe": 3,
"uinProblemLine": false,
"lastUpdateDate": "2022-07-15T06:23:27-05:00",
"nonSellableIdos": [
{
"nonsellableTypeId": 1,
"quantity": 15.0000
},
{
"nonsellableTypeId": 2,
"quantity": 5.0000
}
]
}
}
API: Find Future Inventory
Searches for future delivery records for a single store and single item.
API Basics
Endpoint URL |
{base URL}/{storeId}/{itemId}/future |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
List of delivery records |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute | Description |
---|---|
storeId |
The store identifier to retrieve future inventory for. |
itemId |
The item identifier to retrieve future inventory for. |
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String (25) |
Yes |
The item identifier. |
storeId |
Long |
Yes |
The store identifier. |
deliveries |
List of deliveryIds |
- |
A list of delivery information if it exists. |
Delivery Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
sourceLocationType |
Integer |
Yes |
Item Location Type (see Additional Data Definition). |
sourceLocationId |
Long |
Yes |
The unique identifier of the source location of the delivery. |
deliveryType |
Integer |
Yes |
Item Delivery Type (see Additional Data Definition). |
expectedDate |
Date |
Yes |
The date the inventory is expected to arrive. |
quantityInbound |
BigDecimal |
Yes |
Amount of inventory inbound on the delivery. |
quantityOrdered |
BigDecimal |
Yes |
Amount of inventory on order. |
Example Output
{
"itemId": "100637121",
"storeId": 5000,
"deliveryIdos": [
{
"sourceLocationType": 1,
"sourceLocationId": 5001,
"deliveryType": 3,
"quantityInbound": 30.0000,
"quantityOrdered": 0.0000
}
]
}
Additional Data Definitions
Item Delivery Type
Value | Definition |
---|---|
1 |
Allocation |
2 |
Purchase Order |
3 |
Transfer |
- |
- |
Item Location Type
Value | Definition |
---|---|
1 |
Store |
2 |
Supplier |
3 |
Warehouse |
4 |
Finisher |
API: Find Inventory in Buddy Stores
Searches for inventory information at buddy stores by single input store and multiple items.
API Basics
Endpoint URL |
{baseUrl}/{storeId}/associated |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
List of items |
Output |
List of inventory records |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute | Description |
---|---|
storeId |
The store identifier to find buddy stores for. |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemIds |
List of Strings |
Yes |
A list of items to retrieve inventory for. |
sellingUnitOfMeasure |
Boolean |
- |
True indicates an attempt to use the selling unit of measure of the item, false indicates to use the standard unit of measure. If conversion cannot take place, it defaults back to standard unit of measure. |
Example Input
{
"itemIds": [
"100637156",
"100637172",
"100668091"
],
"sellingUnitOfMeasure": true
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String |
Yes |
The item identifier. |
storeId |
Long |
The store identifier if the item is ranged to a store. |
|
ranged |
Boolean |
Yes |
True if the item is ranged to the store, false otherwise. |
estimated |
Boolean |
Yes |
True if the quantities are estimated, false otherwise. |
unitOfMeasure |
String |
Yes |
The unit of measure of the quantities. |
caseSize |
BigDecimal |
Yes |
The default case size of the item. |
quantityStockOnHand |
BigDecimal |
Yes |
The stock on hand quantity. |
quantityBackroom |
BigDecimal |
Yes |
The quantity located in the back room area. |
quantityShopfloor |
BigDecimal |
Yes |
The quantity located on the shop floor. |
quantityDeliveryBay |
BigDecimal |
Yes |
The quantity located in the delivery bay. |
quantityAvailable |
BigDecimal |
Yes |
The available to sell quantity. |
quantityUnavailable |
BigDecimal |
Yes |
The unavailable to sell quantity. |
quantityNonSellable |
BigDecimal |
Yes |
The total non-sellable quantity. |
quantityInTransit |
BigDecimal |
Yes |
The quantity currently in transit. |
quantityCustomerReserved |
BigDecimal |
Yes |
The quantity reserved for customer orders. |
quantityTransferReserved |
BigDecimal |
Yes |
The quantity reserved for transfers. |
quantityVendorReturn |
BigDecimal |
Yes |
The quantity reserved for vendor returns. |
nonSellableQuantities |
List of Non-Sellable Quantities |
- |
A collection containing the specific quantity in each non-sellable quantity type bucket. |
Non-Sellable Quantity Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
nonsellableTypeId |
Long |
Yes |
The non-sellable type unique identifier. |
quantity |
quantity |
Yes |
The quantity in this particular non-sellable type bucket. |
Example Output
[
{
"itemId": "100637156",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": 10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": 10.0000,
"quantityUnavailable": 0.0000,
"quantityNonSellable": 0.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000
},
{
"itemId": "100637172",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": -10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": -10.0000,
"quantityUnavailable": 20.0000,
"quantityNonSellable": 20.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000,
"nonSellableIdos": [
{
"nonsellableTypeId": 1,
"quantity": 15.0000
},
{
"nonsellableTypeId": 2,
"quantity": 5.0000
}
]
}
}
API: Find Inventory in Transfer Zone Stores
Searches for inventory at transfer zone stores by single input store and multiple items.
API Basics
Endpoint URL |
{baseUrl}/{storeId}/transferzone |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
List of items |
Output |
List of inventory records |
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute | Description |
---|---|
storeId |
The store identifier to find transfer zone stores for. |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemIds |
List of Strings |
Yes |
A list of items to retrieve inventory for. |
sellingUnitOfMeasure |
Boolean |
- |
True indicates an attempt to use the selling unit of measure of the item, false indicates to use the standard unit of measure. If conversion cannot take place, it defaults back to standard unit of measure. |
Example Input
{
"itemIds": [
"100637156",
"100637172",
"100668091"
],
"sellingUnitOfMeasure": true
}
Output Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String |
Yes |
The item identifier. |
storeId |
Long |
The store identifier if the item is ranged to a store. |
|
ranged |
Boolean |
Yes |
True if the item is ranged to the store, false otherwise. |
estimated |
Boolean |
Yes |
True if the quantities are estimated, false otherwise. |
unitOfMeasure |
String |
Yes |
The unit of measure of the quantities. |
caseSize |
BigDecimal |
Yes |
The default case size of the item. |
quantityStockOnHand |
BigDecimal |
Yes |
The stock on hand quantity. |
quantityBackroom |
BigDecimal |
Yes |
The quantity located in the back room area. |
quantityShopfloor |
BigDecimal |
Yes |
The quantity located on the shop floor. |
quantityDeliveryBay |
BigDecimal |
Yes |
The quantity located in the delivery bay. |
quantityAvailable |
BigDecimal |
Yes |
The available to sell quantity. |
quantityUnavailable |
BigDecimal |
Yes |
The unavailable to sell quantity. |
quantityNonSellable |
BigDecimal |
Yes |
The total non-sellable quantity. |
quantityInTransit |
BigDecimal |
Yes |
The quantity currently in transit. |
quantityCustomerReserved |
BigDecimal |
Yes |
The quantity reserved for customer orders. |
quantityTransferReserved |
BigDecimal |
Yes |
The quantity reserved for transfers. |
quantityVendorReturn |
BigDecimal |
Yes |
The quantity reserved for vendor returns. |
nonSellableQuantities |
List of Non-Sellable Quantities |
- |
A collection containing the specific quantity in each non-sellable quantity type bucket. |
Non-Sellable Quantity Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
nonsellableTypeId |
Long |
Yes |
The non-sellable type unique identifier. |
quantity |
quantity |
Yes |
The quantity in this particular non-sellable type bucket. |
Example Output
[
{
"itemId": "100637156",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": 10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": 10.0000,
"quantityUnavailable": 0.0000,
"quantityNonSellable": 0.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000
},
{
"itemId": "100637172",
"storeId": 5000,
"ranged": true,
"estimated": false,
"unitOfMeasure": "EA",
"caseSize": 100.00,
"quantityStockOnHand": 10.0000,
"quantityBackroom": -10.0000,
"quantityShopfloor": 0.0000,
"quantityDeliveryBay": 0.0000,
"quantityAvailable": -10.0000,
"quantityUnavailable": 20.0000,
"quantityNonSellable": 20.0000,
"quantityInTransit": 0.0000,
"quantityCustomerReserved": 0.0000,
"quantityTransferReserved": 0.0000,
"quantityVendorReturn": 0.0000,
"nonSellableIdos": [
{
"nonsellableTypeId": 1,
"quantity": 15.0000
},
{
"nonsellableTypeId": 2,
"quantity": 5.0000
}
]
}
}
REST Service: Item ISN
This rest service defines operations to manage Item ISN information.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env/siocs-int-services>/api/isns
APIs
API |
Description |
Create ISN |
Create a new ISN |
Update ISN |
Updates an existing ISN |
Delete ISN |
Delete an existing ISN |
Find ISNs |
Search for ISNs based on a set of criteria |
Read ISN Types |
Read all Item ISN types |
API: Create ISN
This API will create ISN information.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
The ISN |
Output |
The ISN including ID |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Type |
Req |
Definition |
isn |
String(128) |
X |
A scan number used to scan the item |
isnTypeId |
Long(12) |
X |
The unique identifier of the item ISN type |
itemId |
String(25) |
X |
The unique item identifier (sku number) |
uin |
String(128) |
A universal identification number |
|
externalId |
String(128) |
An identifier from an external system |
Example
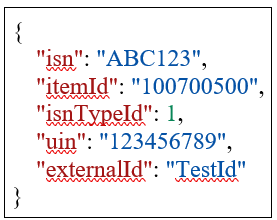
Output Data Definition
Attribute |
Type |
Definition |
isnId |
Long(12) |
The unique identifier of the ISN record |
isn |
String(128) |
This is a scan number used to scan the item |
externalId |
String(128) |
An identifier from an external system |
API: Update ISN
This API allows the ISN information to be modified. An optional value left blank will update the ISN information to blank for that value.
API Basics
Endpoint URL |
{base URL}/isnId |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
ISN Update Information |
Output |
N/A |
Max Response Limit |
N/A |
Path Data Definiton
Attribute |
Type |
Definition |
isnId |
Long(12) |
The unique identifier of the item ISN. |
Input Data Definition
Attribute |
Type |
Req |
Definition |
isnTypeId |
Long(12) |
X |
The unique identifier of the item ISN type |
itemId |
String(25) |
X |
The unique item identifier (sku number) |
uin |
String(128) |
A universal identification number |
|
externalId |
String(128) |
An identifier from an external system |
Example
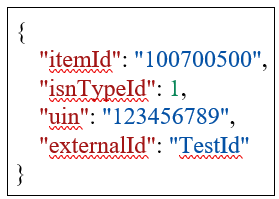
API: Delete ISN
This API deletes an item ISN.
API Basics
Endpoint URL |
{base URL}/{isnId}/delete |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
Path Data Definition
Attribute |
Type |
Definition |
isinId |
Long(12) |
The unique identifier of the item ISN. |
API: Find ISNs
This API is used to lookup or find ISNs.
API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
List of ISNs |
Max Response Limit |
10,000 |
Query Parameters
Attribute |
Type |
Definition |
isn |
String(128) |
Retrieves records containing this ISN, which could be for multiple items. |
ItemId |
String(25) |
Retrieves records associated to this unique item identifier (sku number). |
uin |
String(128) |
Retrieves records associated to this universal identification number. |
updateDateFrom |
String |
Retrieves records on or after this date. |
updateDateTo |
String |
Retrieves records on or before this date. |
Output Data Definition
Attribute |
Type |
Definition |
itemIsnId |
Long(12) |
The unique identifier of the record |
isn |
String(128) |
Retrieves records containing this ISN, which could be for multiple items. |
ItemId |
String(25) |
Retrieves records associated to this unique item identifier (sku number). |
uin |
String(128) |
Retrieves records associated to this universal identification number. |
itemIsnTypeId |
Long(12) |
The unique identifier of the item ISN type |
externalId |
String(128) |
An identifier of this ISN from an external system |
createDate |
Date |
The date this ISN record was first created |
createUser |
String(128) |
The user that created this ISN record |
updateDate |
Date |
The last date this ISN record was updated |
updateUser |
String(128) |
The user that last updated this ISN record. |
API: Read ISN Types
This API is used to lookup all the ISN types available for an ISN.
API Basics
Endpoint URL |
{base URL}/types |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
List of ISN Types |
Max Response Limit |
N/A |
Output Data Definition
Attribute |
Type |
Definition |
isnTypeId |
Long(12) |
The unique ISN type identifier |
labelKey |
String(128) |
A label for the ISN type (not translated) |
restricted |
Boolean |
Y if this represents secure data, N otherwise. |
REST Service: Item Price
This service allows for the search and retrieval of item pricing information stored within EICS.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer></cust_env>/siocs-int-services/api/prices
API Definitions
API Definitions
API | Description |
findPrices | This API can be used to search for price summary information matching filter criteria. |
FindPriceByIds | Find extended price information based on a list of potential unique price identifiers. |
API: findPrices
This API can be used to search for price summary information matching filter criteria.
At least one input criteria is required or a bad request error will be returned.
API Basics
Endpoint URL | {base URL} |
Method | GET |
Success Response | 200 OK |
Processing Type | Synchronous |
Input | Query Parameters |
Output | List of Prices |
Maximum Results Allowed | 10,000 |
Input Data Definition
Attribute | Data Type | Definition |
storeId | Long(10) | Only retrieve item price information for this store. |
effectiveDateFrom | String | Only retrieve item price information on or after this date. |
effectiveDateTo | String | Only retrieve item price information on or before this date. |
itemId | String(25) | Only retrieve item price information for this item. |
Output Data Definition
Attribute | Data Type | Definition |
priceId | Long(12) | The unique identifier of the price. |
itemId | String(25) | The unique identifier of the item. |
storeId | Long(10) | The unique identifier of the store. |
status | Integer | The status of the price. |
priceType | Integer | The type of the price. |
effectiveDate | Date | The effective date of the price. |
endDate | Date | The end date of the price. |
priceValue | BigDecimal(20,4) | The price amount. |
priceCurrency | String(3) | The price currency. |
unitOfMeasure | String(4) | The item unit of measure associated with the price. |
API: findPriceByIds
Find extended price information based on a list of potential unique price identifiers.
It will return information only for price identifiers that are found. It will not return errors or fail to process if invalid identifiers occur. It is up to the accessing information to determined prices not found using this API.
API Basics
Endpoint URL | {base URL}/find |
Method | POST |
Success Response | 200 OK |
Processing Type | Synchronous |
Input | ID List |
Output | List of Prices |
Maximum Input Allowed | 5,000 |
Input Data Definition
Attribute | Type | Definition |
priceIds | List<Long(12)> | A list of price identifiers. |
Output Data Definition
Attribute | Type | Definition |
priceId | Long(12) | The unique identifier of the price. |
itemId | String(25) | The unique identifier of the item. |
storeId | Long(10) | The unique identifier of the store. |
Status | Integer | The status of the price. |
priceType | Integer | The type of the price |
effectiveDate | Date | The effective date of the price. |
endDate | Date | The end date of the price. |
priceValue | BigDecimal(20,4) | The price amount. |
priceCurrency | String(3) | The price currency. |
unitOfMeasure | String(4) | The item unit of measure associated with the price. |
externalPriceId | Long(12) | The unique identifier of the external price or price event. |
clearanceId | Long(15) | The unique identifier of the clearance price change from the pricing engine. |
promotionId | Long(10) | The unique identifier of the promotion. |
regularPriceChangeId | Long(15) | The unique identifier of the regular price change from the pricing engine. |
resetClearanceId | Long(15) | The identifier of the clearance reset. |
storeRequested | Boolean | True indicates it is store requested, false indicates it is not store requested. |
sellingUnitPriceChange | Boolean | True indicates the selling unit retail price has changed, false indicates it has not. |
multiUnitPriceChange | Boolean | True indicates the multi-unit pricing has changed, false indicates it has not. |
multiUnitRetail | BigDecimal(20,4) | The multi-unit retail price. |
multiUnitRetailCurrency | String(3) | The currency type of the multi-unit retail price in the multi-selling unit of measure. |
multiUnits | BigDecimal(12,4) | The number of multi-units. |
multiUnitUom | String(4) | The unit of measure of the multi-unit retail price. |
promotionName | String(160 | The promotion name. |
promotionCompDtlId | Long(15) | The unique identifier of the promotion component detail from the pricing engine. |
promotionType | Integer | Promotion Component Type (See Index) |
promotionDurationType | Integer | Promotion Duration Type (See Index) |
promotionCompId | Long(10) | The unique identifier of the promotion component. |
promotionCompName | String(160) | The promotion component name. |
promotionDescription | String(640) | The promotion description. |
updateDate | Date | The date that the update took place. |
Example Input:
{ "priceIds": [ 123, 456, 789, 012 ] } |
Additional Data Definitions
Price Type
ID | Status |
1 | Permanent |
2 | Promotional |
3 | Clearance |
4 | Clearance Reset |
Price Status
ID | Status |
1 | New |
2 | Pending |
3 | Approved |
4 | Completed |
5 | Rejected |
6 | Ticket List |
7 | Extract Failed |
8 | Deleted |
99 | Default |
Promotion Component Type
ID | Status |
1 | Complex Promotion |
2 | Simple Promotion |
3 | Threshold Promotion |
4 | Credit (Finance) Promotion |
5 | Transaction Promotion |
Promotion Duration Type
ID | Status |
1 | All Day Promotion |
2 | Partial Day Promotion |
3 | Multiple Day Promotion |
REST Service: Item UDA
This service integrates user defined attribute foundation data. Asynchronous item UDA integration is processed through staged messages and is controlled by the MPS Work Types.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/udas
API Definitions
API |
Description |
importUdas |
Imports user defined attributes. |
deleteUdas |
Deletes user defined attributes. |
importItemUdas |
Imports an association between items and user defined attributes. |
deleteItemUdas |
Deletes the association between items and user defined attributes. |
readItemUdas |
Retrieves all the user defined attributes for a particular item. |
API: Import Udas
Imports user defined attributes. It is managed by DcsUda work type. If the input exceeds 500 UDAs an input too large exception will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
UDA import List |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
udas |
List of details |
Yes |
A list of user defined attributes. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
udaId |
Integer (5) |
Yes |
The user defined attribute identifier. |
type |
Integer |
Yes |
See Index: UdaType |
description |
String (120) |
Yes |
The description of the user defined attribute. |
printTicket |
Boolean |
True indicates tickets are printed for this user defined attribute. |
|
printLabel |
Boolean |
True indicates labels are printed for this user defined attribute. |
|
lovId |
String (25) |
The unique identifier of a list of values UDA. |
|
lovDescription |
String (250) |
The description of the list of values UDA. |
API: Delete Udas
Deletes user defined attributes. It is managed by DcsUda work type. If the input exceeds 500 UDAs an input too large exception will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
UDA delete list |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
udaIds |
List<Long> |
Yes |
A list of user defined attribute. |
API: Import Item Udas
Imports associations between items and user defined attributes. This is controlled by the work type: DcsItem.
If the input exceeds 500 Item UDAs an input too large exception will be returned.
A "Forbidden" response will occur if application is integrated with MFCS.
API Basics
Endpoint URL |
{base URL}/items/import |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
Item UDA list |
Output |
None |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
itemUdas |
List of details |
x |
A list of associations between an item and user defined attributes. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String |
X |
The item identifier. |
udaId |
Integer |
X |
The user defined attribute identifier. |
udaDate |
Date |
Holds the value of the user defined attribute if it is a date. |
|
udaText |
String (250) |
Holds the value of the user defined attribute if it is text. |
|
udaLovId |
String (25) |
Holds the unique numeric identifier of the user defined attribute if it is a list of values selection. |
|
|
Boolean |
Y indicates printing is done for this item and user defined attribute (which is also controlled by the UDA). |
API: Delete Item Udas
Deletes an association between item and user defined attributes. This is controlled by the work type: DcsItem.
If the input exceeds 500 Item UDAs an input too large exception will be returned.
A "Forbidden" response will occur if application is integrated with MFCS.
API Basics
Endpoint URL |
{base URL}/items/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
UDA item delete list |
|
Output |
None |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
itemUdas |
List of details |
Yes |
A list of associations between item and user defined attribute identifiers. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The item identifier. |
udaId |
Integer (5) |
Yes |
The user defined attribute identifier. |
udaDate |
Date |
Holds the value of the user defined attribute if it is a date. |
|
udaText |
String (250) |
Holds the value of the user defined attribute if it is text. |
|
udaLovId |
String (25) |
Holds the unique numeric identifier of the user defined attribute if it is a list of values selection. |
API: Find Item Udas
It will retrieve UDAs for the inputted items.
API Basics
Endpoint URL |
{base URL}/items/find |
|
Method |
POST |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
Item list |
|
Output |
UDA item list |
|
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
itemIds |
List<String> |
Yes |
A list of items to find UDAs for. |
Output Data Definition
Attribute |
Data Type |
Description |
itemId |
String |
The item identifier. |
udaId |
Integer |
The user defined attribute identifier. |
type |
String |
The user defined attribute type: (LV) List of Value, (FF) Free Form Text, DT (Date) |
description |
String |
The description of the user defined attribute. |
udaDate |
Date |
Holds the value of the user defined attribute if it is a date. |
udaText |
String |
Holds the value of the user defined attribute if it is text. |
udaLovId |
Long |
Holds the unique numeric identifier of the user defined attribute if it is a list of values selection. |
udaLoveDescription |
String |
Holds the value description of the UDA List of Values selection. |
printTicket |
Boolean |
True indicates tickets are printed for this user defined attribute. |
printLabel |
Boolean |
True indicates labels are printed for this user defined attribute. |
Additional Data Definitions
UDA Type
Value |
Definition |
1 |
Date |
2 |
Free Form Text |
3 |
List of Value |
REST Service: Item UIN
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/uins
API Definitions
API | Description |
createUin | Create a new unique identification number. |
readUin | Reads information about a Universal Identification Number. |
findUins | Find unique identification number summary information based on search criteria. |
findUinLabels | This API is used to find all the UIN labels available for a uin items. |
findUinHistory | This API is used to find UIN historical information based on search criteria. |
generateUins | This API generates new Type 2 (Auto Generated Serial Numbers) Universal Identification Numbers without changing store inventory positions. |
API: createUin
Create a new unique identification number. Note that the combination of store and item determines the administrative information about a UIN (such as UIN Type).
The newly created UIN will be in "Unconfirmed" status and its transaction type will be "UIN Web Service." To move it into inventory, use inventory adjustment or another transaction.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
UIN information |
Output |
UIN confirmation information |
Input Data Definition
Payload |
Type |
Req |
Definition |
storeId |
Long |
X |
The identifier of the store. |
itemId |
String |
X |
The identifier of the item. |
uin |
String |
X |
The universal identification number. |
Output Data Definition
Payload |
Type |
Definition |
itemUinId |
Long |
The unique identifier to the record. |
storeId |
Long |
The identifier of the store. |
itemId |
String |
The identifier of the item. |
uin |
String |
The universal identification number. |
status |
Integer |
The current status of the UIN. Valid values are in index. |
Example
{
"storeId": 5000,,
"itemId": "100700500",
"uin": "1234"
}
API: readUin
Reads information about a Universal Identification Number.
API Basics
Endpoint URL |
{base URL}/items/{itemId}/{uin} |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Path Parameters Item identifier and UIN |
Output |
UIN information |
Output Data Definition
Payload |
Type |
Definition |
itemUinId |
Long |
A unique identifier representing the record in the database. |
itemId |
String |
The identifier of the item. |
uin |
String |
The universal identification number. |
type |
Integer |
The type of UIN. Valid values are: (1) Serial Number, (2) Auto Generated Serial Number |
status |
Integer |
The current status of the UIN. See Index for valid values. |
storeId |
Long |
The store identifier |
transactionType |
Integer |
The business area that last contained the UIN, |
transactionId |
String |
The transaction id of the transaction containing the UIN. |
cartonId |
String |
The identifier of the carton containing the UIN. |
nonsellableTypeId |
Long |
A non-sellable inventory bucket the UIN was within. |
previousStatus |
Integer |
The previous status of the UIN. Valid values are in index. |
previousStoreId |
Long |
The previous store identifier associated with the previous status. |
previousTransactionType |
Integer |
The previous business area that contained the UIN for that previous status. |
previousTransactionId |
String |
The transaction id of the transaction that previously contained the UIN for that previous status. |
previousCartonId |
String |
The identifier of the carton that previously container the UIN for that previous status. |
previousNonsellableTypeId |
Long |
A non-sellabable inventory bucket the UIN was last within for that previous status. |
damaged |
Boolean |
True if the UIN is damaged, N otherwise. |
createDate |
Date |
The date the UIN was first inserted into the system. |
updateDate |
Date |
The last date the UIN was updated. |
createUser |
String |
The user that first inserted the UIN into the system. |
updateUser |
String |
The user that last updated the UIN in the system. |
API: findUins
Find unique identification number summary information based on search criteria.
API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of UINs (see ReadUIN API for Data Output) |
Maximum Results Allowed |
10,000 |
Input Data Definition
Attribute |
Type |
Definition |
storeId |
Long |
Include only UINs for this store identifier. |
itemId |
String |
Include only UINS for this item |
status |
Integer |
Include only UINs with this current status. |
updateDateFrom |
Date/String |
Include only UINs updated on or after this date. |
updateDateTo |
Date/String |
Include only UINs updated on or before this date. |
API: findUinLabels
This API is used to find all the UIN labels available for a uin items.
API Basics
Endpoint URL |
{base URL}/labels |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
List of labels |
Output Data Definition
Attribute |
Type |
Definition |
labelId |
Long |
The unique identifier of the record. |
labelCode |
String |
A unique code that defines the label. |
description |
String |
The description or label associated to the code (not translated). |
API: findUinHistory
This API is used to find UIN historical information based on search criteria.
API Basics
Endpoint URL |
{base URL}/histories |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
List of UIN history records |
Maximum Results Allowed |
10,000 |
Input Data Definition
Attribute |
Type |
Definition |
itemId |
String |
Include only UIN history for this item. |
uin |
Integer |
Include only UIN history for this UIN. |
createDateFrom |
Date/String |
Include only UIN history created on or after this date. |
createDateTo |
Date/String |
Include only UIN history created on or before this date. |
Output Data Definition
Payload |
Type |
Definition |
itemId |
String |
The identifier of the item. |
uin |
String |
The universal identification number. |
type |
Integer |
The type of UIN. Valid values are: (1) Serial Number, (2) Auto Generated Serial Number |
status |
Integer |
The current status of the UIN. Valid values are in index. |
storeId |
Long |
The store identifier |
transactionType |
Integer |
The business area that last contained the UIN, |
transactionId |
String |
The transaction id of the transaction containing the UIN. |
cartonId |
String |
The identifier of the carton containing the UIN. |
nonsellableTypeId |
Integer |
A non-sellable inventory bucket the UIN was within. |
createDate |
Date |
The date the UIN was first inserted into the system. |
updateDate |
Date |
The last date the UIN was updated. |
createUser |
String |
The user that first inserted the UIN into the system. |
updateUser |
String |
The user that last updated the UIN in the system. |
API: generateUins
This API generates new Type 2 (Auto Generated Serial Numbers) Universal Identification Numbers without changing store inventory positions.
If the UIN administrative data for the item and store used do not indicate Type 2, an error will be returned.
The new UINs generated will have a status of “Unconfirmed”.
API Basics
Endpoint URL |
{base URL}/generate |
Method |
POST |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
UIN generation information |
Output |
N/A |
Input Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String |
X |
The identifier of the item. |
storeId |
Long |
X |
The identifier of the store |
quantity |
Integer |
X |
The amount of universal identification numbers to generate. |
transactionType |
Integer |
X |
See Index: UIN Functional Area |
transactionId |
String |
A transaction reference identifier. |
Example
{
"storeId": 5000,
"itemId": "100663071",
"uin": "1234",
"quantity": 5,
"transactionType": 13
}
Additional Data Definitions
UIN Type
ID |
Description |
1 |
Serial Number |
2 |
Auto-Generated Serial Number |
UIN Status
ID |
Description |
1 |
In Stock |
2 |
Sold |
3 |
Shipped To Warehouse |
4 |
Shipped To Store |
5 |
Reserved For Shipping |
6 |
Shipped To Vendor |
7 |
Removed From Inventory |
8 |
Unavailable |
9 |
Missing |
10 |
In Receiving |
11 |
Customer Order Reserved |
12 |
Customer Order Fulfilled |
13 |
Shipped To Finisher |
99 |
Unconfirmed |
UIN Functional Area
ID |
Description |
1 |
Warehouse Delivery Receipt |
2 |
Direct Delivery Receipt |
3 |
Create Transfer |
4 |
Dispatch Transfer |
5 |
Receive Transfer |
6 |
Receipt Adjustment |
7 |
Create Return |
8 |
Dispatch Return |
9 |
Inventory Adjustment |
10 |
Stock Count |
11 |
Stock Recount |
12 |
Stock Count Authorized |
13 |
Manual |
14 |
POS Sale |
15 |
POS Return |
16 |
POS Sales Void |
17 |
POS Return Void |
18 |
UIN Web Service |
19 |
Customer Order |
20 |
Direct Delivery ASN Inbound |
21 |
Transfer ASN Inbound |
22 |
Transfer Shipment |
REST Service: Manifest
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/manifests
API Definitions
API | Description |
---|---|
Close Manifest |
Call this method to close all manifested shipments matching the input criteria. |
API: Close Manifest
Call this method to close all manifested shipments for the carrier code and carrier services. A processing message is sent to the internal message processing system and the services returns an “Accepted” response. The closing of the manifest will occur later when the message is processed.
API Basics
Endpoint URL |
{base URL}/close |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
Criteria |
|
Output |
None |
|
Max Response Limit |
- |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
carrierCode |
String (4) |
Yes |
A carrier code. |
carrierServiceCode |
String (6) |
Yes |
A carrier service code. |
trackingNumber |
List of Strings (120) |
Yes |
A list of tracking numbers associated to the contents of the manifest. |
shipDate |
Date |
- |
Indicates all items manifested prior to this date for the carrier have been shipped. |
Example Input
{
"carrierCode": "O",
"carrierServiceCode": "O",
"trackingIds": ["7861","45722"],
"shipDate":"2022-04-19T23:59:59-05:00"
}
REST Service: POS Transaction
This service retrieves information about item inventory.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/postransactions
API: Import POS Transactions
POS may integration its transaction to EICS using this web service. The service imports and process point-of-sale transactions through an asynchronous process. The service has a default limit of 1000 total items, though they may be distributed across any number of transactions. Only one store is allowed across all the transaction sent in a single requires.
The web service is optimized for speed at greater than 400 items and less than 500 items per service call. The further above or below this optimized point, processing speed will be reduced, and it may take longer for the sales to be recorded in inventory.
Since this import is asynchronous, only the form is validated prior to the data being captured and processed later. See Sales Integration for additional information about processing.
POS Transaction ID and Sales Audit
In order for the sales audit process to match and audit a POS transaction that was previously recieved, the transactionId attribute of the POS Transaction must use the same data elements and format as the sales audit file. The sales audit file concatenates the THEAD file line id value, plus a dash separator, plus the THEAD transaction number clume value. For example, 111-222. The transactionId attribute of the POS transaction must match these values and format.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
Asynchronous |
Input |
List of transactions |
Output |
None |
Max Input Limit |
- |
Input Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
Transactions |
List of PosTransactions |
Yes |
A list of transactions to process. |
Pos Transaction Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
storeId |
Long |
Yes |
The unique identifier of the store that is the source of the transaction. This attribute is used to synchronize with sale audit. |
transactionId |
String (128) |
Yes |
A unique identifier of the point-of-sale transaction. This attribute is used to synchronize with sale audit. |
transactionTimestamp |
Date |
Yes |
The date and time of the transaction. |
custOrderId |
String (128) |
- |
An external customer order identifier. This attribute is required for customer order related point-of-sale transactions. |
CustomerOrderComm |
String (512) |
- |
A comment associated to the customer order. |
externalUser |
String (128) |
- |
User information from the external point-of-sale system. |
items |
List of Items |
Yes |
A collection of items belonging to the transaction. |
Pos Transaction Item Data Definition
Attribute | Data Type | Required | Description |
---|---|---|---|
itemId |
String (25) |
Yes |
The transaction-level SKU number of the item. |
quantity |
BigDecimal |
Yes |
The quantity of the item transacted. |
unitOfMeasure |
String (4) |
Yes |
Unit of measure of the quantity. |
uin |
String (128) |
- |
The unique identifier number (serial number). If not empty, the quantity will be overwritten with a quantity of one. |
epc |
String (256) |
- |
A complete SGTIN-96 EPC of the item. |
reasonCode |
Integer |
- |
A reason code associated to the line item. This reason code represents the inventory movement reason. If the field is left blank, the appropriate inventory movement reason code will be defaulted based on the type of sale. This field is required when non-sellable sub-level inventory tracking is active in the system. |
dropShip |
Boolean |
- |
True if this item is a drop ship, false if it is not. Drop ship sales do not impact stock positions. |
fulfillOrderId |
String (128) |
- |
If the transaction is associated to a customer order, this is the external fulfillment order identifier. |
fulfillmentOrderLineNumber |
Long |
- |
If the transaction is associated to a customer order, this is the line number of the order that this item transaction aligns with. |
reservationType |
Integer |
- |
If the transaction is a customer order, this is the type of reservation. See Reservation Type. |
transactionCode |
Integer |
Yes |
A code that indicates the transaction event that took place on the item. See Transaction Codes. |
comments |
String (512) |
- |
Comments associated to the line item. |
Example Input
{
"transactions":
[
{
"storeId": 5000,
"transactionId": 1236,
"transactionTimestamp": "2022-04-19T23:59:59-05:00",
"externalUser": "ABC",
"custOrderId": "1111",
"items":
[
{
"itemId": 5678,
"transactionCode": 5,
"reservationType": 1,
"fulfillOrderId": "2222",
"dropShip": false
}
]
}
]
}
Possible Business Exception Codes
In addition to the normal REST error codes, the following business data element may be returned when a business error occurs.
Business Exception Data Definition
Name | Definition |
---|---|
DUPLICATE_TRANSACTION |
One or more the transactions or transaction items are not unique. This will cause the entire request to be rejected. |
Additional Data Definitions
Reservation Type
Value | Definition |
---|---|
1 |
Web Order |
2 |
Special Order |
3 |
Pickup or Delivery |
4 |
Layaway |
5 |
On Hold |
Transaction Code
Value | Definition |
---|---|
1 |
Sale |
2 |
Return |
3 |
Void Sale |
4 |
Void Return |
5 |
Order New |
6 |
Order Fulfill |
7 |
Order Cancel |
REST Product Group
This service allows for the integration of product group with external systems.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/productgroups
APIs
API |
Description |
findProductGroups |
Searches for product group summary headers based on input criteria. |
readProductGroup |
Reads the details about a specific product group. |
createProductGroup |
Creates a new product group including its components. |
updateProductGroup |
Modifies an existing product group including its components. |
cancelProductGroup |
Cancels an existing product group. |
API: findProductGroup
This API is used to search for a product group summary using input criteria.
Table 4-11 API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
Collection of product groups |
Max Response Limit |
5,000 |
Table 4-12 Query Parameters
Query |
Type |
Definition |
storeId |
Long(10) |
Returns only product groups that match this store (which includes all store product groups). |
type |
Integer(3) |
Returns only product groups that match this product group type. See Index for types. |
description |
String(100) |
Returns only products groups that contain this text in its description. |
itemId |
String(25) |
Returns only product groups that contain this individual sku number in its single items list. |
departmentId |
Long(12) |
Returns only product groups that contain this hierarchy department identifier. |
classId |
Long(12) |
Returns only product groups that contain this hierarchy class identifier. |
subclassId |
Long(12) |
Returns only product groups that contain this hierarchy subclass identifier. |
updateDateFrom |
String |
Returns only product groups updated on or after this date. |
updateDateTo |
String |
Returns only product groups updated on or before this date. |
Table 4-13 Output Data Definitions
Payload |
Type |
Definition |
productGroupId |
Long(12) |
The unique product group identifier. |
description |
String(100) |
A description of the product group. |
storeId |
Long(10) |
The store identifier or blank if the product group is for all stores. |
type |
Integer(3) |
Indicates the type of product group. See Index. |
status |
Integer(1) |
Indicates the status of the product group. See Index. |
updateDate |
Date |
The date the product group was last updated. |
API: readProductGroup
This API is used to read a product group.
Table 4-14 API Basics
Endpoint URL |
{base URL}/{productGroupId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the product group. |
Output |
Collection of product groups |
Max Response Limit |
5,000 |
Table 4-15 Output Data Definition
Payload |
Type |
Definition |
productGroupId |
Long(12) |
The unique identifier of the product group. |
description |
String(100) |
A description of the product group. |
storeId |
Long(10) |
The unique identifier of a store associated to the product group. This will be blank/null if the product group is for all stores. |
type |
Integer(3) |
Indicates the type of product group. See Index. |
status |
Integer(1) |
Indicates the status of the product group. See Index. |
unitOfMeasureMode |
Integer(4) |
Unit of measure type for pick list product groups. See Index. |
allItemGroup |
Boolean |
True indicates the product group is for all items. If set to true, then hierarchies and items will not be present. |
updateDate |
Date |
The date the product group was last updated. |
stockCountDetail |
Object |
Details about a stock count product group (used for all three stock count types). This will only be populated if the product group type is for a stock count. |
replenishmentDetail |
Object |
Details about a replenishment product group. This will only be populated if the product group type is for replenishment. |
storeOrderDetail |
Object |
Details about a store order product group. This will only be populated if the product group type is for store orders. |
autoAdjustmentDetail |
Object |
Details about an auto inventory adjustment product group. This will only be populated if the product group type is for auto adjustments. |
autoTicketPrintDetail |
Object |
Details about an auto ticket print product group. This will only be populated if the product group type is for auto ticket print. |
hierarchies |
List<Object> |
A list of merchandise item hierarchies that belong to the group. |
itemIds |
List<String(25)> |
A list of item SKU numbers associated to the product group. |
Table 4-16 Stock Count Detail
Payload |
Type |
Definition |
countingMethod |
Integer |
The method of counting a stock count: See Index (StockCountingMethod) |
breakdownType |
Integer |
The method of breaking a stock count down into child counts: See Index (StockCountBreakdownType) |
varianceCount |
Integer(8) |
The number of units in standard unit of measure considered discrepant on a stock count. |
variancePercent |
BigDecimal(12,4) |
The percentage of units in standard unit of measure considered discrepant on a stock count. |
varianceValue |
BigDecimal(12,4) |
The value of units considered discrepant on a stock count. This will only be populated for unit and amount stock count product group type. |
performRecount |
Boolean |
True indicates the stock count should be recounted if discrepancies are found. |
autoAuthorize |
Boolean |
True indicates the stock count should be automatically authorized after count. |
activeItems |
Boolean |
True indicates active items should be included on the stock count. |
inactiveItems |
Boolean |
True indicates inactive items should be included on the stock count. |
discontinuedItems |
Boolean |
True indicates discontinued items should be included on the stock count. |
deletedItems |
Boolean |
True indicates deleted items should be included on the stock count. |
zeroStockOnHand |
Boolean |
True indicates items with 0 stock on hand should be included on the stock count. |
positiveStockOnHand |
Boolean |
True indicates items with positive stock on hand should be included on the stock count. |
negativeStockOnHand |
Boolean |
True indicates items with negative stock on hand should be included on the stock count. |
problemLineNegativeAvailable |
Boolean |
True indicates to include items with negative available quantities. This will only be populated if the product group type is for a problem line stock count. |
problemLinePickLessSuggested |
Boolean |
True indicates to include pick lists less than suggested amount. This will only be populated if the product group type is for a problem line stock count. |
problemLineReplenishLessSuggested |
Boolean |
True indicates to include shelf replenishments that are less than the suggested amount. This will only be populated if the product group type is for a problem line stock count. |
problemLineUinDiscrepancy |
Boolean |
True indicates UIN discrepancies should be included in the stock count. This will only be populated if the product group type is for a problem line stock count. |
Table 4-17 Replenishment Detail
Payload |
Type |
Definition |
autoReplenishment |
Boolean |
True indicates that the product group is eligible for automatic replenishment through a scheduled batch run. |
differentiatorMode |
Integer(3) |
The display type of the diffentiator. See Index. |
Table 4-18 Store Order Detail
Payload |
Type |
Definition |
daysBeforeDelivery |
Integer(3) |
The date calculated from the creation date that the user wants the store order delivery by. |
storeOrderAddItems |
Boolean |
True indicates items can be added to the store order after it has been generated by the system. |
storeOrderItemsOnly |
Boolean |
Y indicates the store order can only include items that are marked as store order replenishment. |
restrictSupplierId |
Long(12) |
Limits the creation of a store order from this product group to only this supplier. |
restrictWarehouseId |
Long(12) |
Limits the creation of a store order from this product group to only this warehouse. |
restrictHierarchyId |
Long(12) |
Limits the creation of a store order from this product group to only this merchandise hierarchy. |
restrictAreaId |
Long(12) |
Limits the creation of a store order from this product group to only this store sequence area. |
Table 4-19 Auto Adjustment Detail
Payload |
Type |
Definition |
adjustmentQuantity |
BigDecimal(20,4) |
The quantity that should be automatically adjusted by this product group. |
adjustmentPercent |
BigDecimal(20,4) |
The percentage of quantity that should be automatically adjusted by this product group. |
adjustmentReasonCode |
Long(12) |
The unique identifier of a reason code associated to this product group. |
updateToZero |
Boolean |
Update stock to zero when generating adjustment with this product group. |
Table 4-20 Auto Ticket Print Detail
Payload |
Type |
Definition |
refreshTicketQuantity |
Boolean |
True if the ticket quantity should be refreshed prior to printing. |
Table 4-21 Item Hierarchy Detail
Payload |
Type |
Definition |
departmentId |
Long(12) |
The department identifier |
classId |
Long(12) |
The class identifier |
subclassId |
Long(12) |
The subclass identifier |
API: createProductGroup
This API is used to create a new product group that will be in progress status.
Table 4-22 API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Product Group |
Output |
Product Group Status |
Max Response Limit |
5,000 |
Table 4-23 Input Data Definition
Payload |
Type |
Req |
Definition |
description |
String(100) |
X |
A description of the product group. |
type |
Integer(3) |
X |
Indicates the type of product group. See Index. |
storeId |
Long(10) |
The unique identifier of a store associated to the product group. This will be blank/null if the product group is for all stores. |
|
allItems |
Boolean |
True if the product group is for all items, false otherwise. If all items, any hierarchies and items included will be ignored. |
|
unitOfMeasureMode |
Integer |
Unit of measure type for pick lists. See Index.. |
|
autoAdjustmentDetail |
Object |
Details about an auto inventory adjustment product group. This is only processed and is required if the type is auto inventory adjustment type. |
|
autoTicketPrintDetail |
Object |
Details about an auto ticket print product group. This is only processed and is required if the type is auto ticket print. |
|
replenishmentDetail |
Object |
Details about a replenishment product group. This is only processed and is required if the type is a shelf replenishment type.. |
|
storeOrderDetail |
Object |
Details about a store order product group. This is only processed and is required if the type is the store order type. |
|
unitCountDetail |
Object |
Details about a unit stock count product group. This is only processed and is required if the type is a stock count type. |
|
unitAmountCountDetail |
Object |
Details about a unit and amount stock count product group. This is only processed and is required if the type is a stock count type. |
|
problemLineCountDetail |
Object |
Details about a problem line stock count product group. This is only processed and is required if the type is a stock count type. |
|
hierarchies |
List<Object> |
A list of up to 5,000 unique merchandise item hierarchies that belong to the group (avoid duplicate or overlapping hierarchies). |
|
itemIds |
List<String(25)> |
A list of up to 5,000 unique item SKU numbers on the product group. |
Table 4-24 Unit Count Detail
Payload |
Type |
Req |
Definition |
countingMethod |
Integer |
X |
The method of counting a stock count: See Index (StockCountingMethod) |
breakdownType |
Integer |
X |
The method of breaking a stock count down into child counts: See Index (StockCountBreakdownType). Must be "Location" for guided counts. |
varianceCount |
Integer(8) |
The number of units in standard unit of measure considered discrepant on a stock count. Variance count and percent cannot both be empty. |
|
variancePercent |
BigDecimal(12,4) |
The percentage of units in standard unit of measure considered discrepant on a stock count. Variance count and percent cannot both be empty. |
|
performRecount |
Boolean |
True indicates the stock count should be recounted if discrepancies are found. This cannot be set to true if the counting method is "Third Party. |
|
autoAuthorize |
Boolean |
True indicates the stock count should be automatically authorized after count. |
|
activeItems |
Boolean |
True indicates active items should be included on the stock count. At least one item status choice is required as true. |
|
inactiveItems |
Boolean |
True indicates inactive items should be included on the stock count. At least one item status choice is required as true. |
|
discontinuedItems |
Boolean |
True indicates discontinued items should be included on the stock count. At least one item status choice is required as true. |
|
deletedItems |
Boolean |
True indicates deleted items should be included on the stock count. At least one item status choice is required as true. |
|
zeroStockOnHand |
Boolean |
True indicates items with 0 stock on hand should be included on the stock count. At least one stock choice is required as true. |
|
positiveStockOnHand |
Boolean |
True indicates items with positive stock on hand should be included on the stock count. At least one stock choice is required as true. |
|
negativeStockOnHand |
Boolean |
True indicates items with negative stock on hand should be included on the stock count. At least one stock choice is required as true. |
Table 4-25 Unit and Amount Count Detail
Payload |
Type |
Req |
Definition |
countingMethod |
Integer |
X |
The method of counting a stock count: See Index (StockCountingMethod) |
breakdownType |
Integer |
X |
The method of breaking a stock count down into child counts: See Index (StockCountBreakdownType). Must be "Location" for guided counts. |
varianceCount |
BigDecimal(12,4) |
The number of units in standard unit of measure considered discrepant on a stock count. Count, percent, and value cannot all be empty. |
|
variancePercent |
BigDecimal(12,4) |
The percentage of units in standard unit of measure considered discrepant on a stock count. Count, percent, and value cannot all be empty. |
|
varianceValue |
BigDecimal(12,4) |
The value of units considered discrepant on a stock count. Count, percent, and value cannot all be empty. |
|
performRecount |
Boolean |
True indicates the stock count should be recounted if discrepancies are found. This cannot be set to true if the counting method is "Third Party". |
|
autoAuthorize |
Boolean |
True indicates the stock count should be automatically authorized after count. |
|
zeroStockOnHand |
Boolean |
True indicates items with 0 stock on hand should be included on the stock count. |
Table 4-26 Problem Line Count Detail
Payload |
Type |
Req |
Definition |
countingMethod |
Integer |
X |
The method of counting a stock count: See Index (StockCountingMethod). |
breakdownType |
Integer |
X |
The method of breaking a stock count down into child counts: See Index (StockCountBreakdownType). Must be "Location" for guided counts. |
autoReplenishment |
Boolean |
True indicates that the product group is eligible for automatic replenishment through a scheduled batch run. |
|
differentiatorMode |
Integer(3) |
The display type of the differentiator. See Index. |
|
varianceCount |
BigDecimal(12,4) |
The number of units in standard unit of measure considered discrepant on a stock count. Variance count and percent cannot both be empty. |
|
variancePercent |
BigDecimal(12,4) |
The percentage of units in standard unit of measure considered discrepant on a stock count. Variance count and percent cannot both be empty |
|
performRecount |
Boolean |
True indicates the stock count should be recounted if discrepancies are found. This cannot be set to true if the counting method is "Third Party." |
|
autoAuthorize |
Boolean |
True indicates the stock count should be automatically authorized after count. |
|
negativeStockOnHand |
Boolean |
True indicates items with negative stock on hand should be included on the stock count. |
|
negativeAvailable |
Boolean |
True indicates to include items with negative available quantities. |
|
pickLessSuggested |
Boolean |
True indicates to include pick lists less than suggested amount. |
|
replenishLessSuggested |
Boolean |
True indicates to include shelf replenishments that are less than the suggested amount. |
|
uinDiscrepancy |
Boolean |
True indicates UIN discrepancies should be included in the stock count. This will only be populated if the product group type is for a problem line stock count. |
Table 4-27 Store Order Detail
Payload |
Type |
Req |
Definition |
daysBeforeDelivery |
Integer(3) |
The date calculated from the creation date that the user wants the store order delivery by. |
|
storeOrderAddItems |
Boolean |
True indicates items can be added to the store order after it has been generated by the system. |
|
storeOrderItemsOnly |
Boolean |
Y indicates can only include items that are marked as store order replenishment. |
|
restrictSupplierId |
Long(12) |
Limits the creation of a store order from this product group to only this supplier. |
|
restrictWarehouseId |
Long(12) |
Limits the creation of a store order from this product group to only this warehouse. |
|
restrictHierarchyId |
Long(12) |
Limits the creation of a store order from this product group to only this merchandise hierarchy. |
|
restrictAreaId |
Long(12) |
Limits the creation of a store order from this product group to only this store sequence area. |
Table 4-28 Auto Adjustment Detail
Payload |
Type |
Req |
Definition |
adjustmentQuantity |
BigDecimal(20,4) |
The quantity that should be automatically adjusted by this product group. Either quantity or percent is required. |
|
adjustmentPercent |
BigDecimal(20,4) |
The percentage of quantity that should be automatically adjusted by this product group. Either quantity or percent is required. |
|
adjustmentReasonCode |
Long(12) |
X |
The unique identifier of a reason code associated to this product group. |
updateToZero |
Boolean |
Update stock to zero when generating adjustment with this product group. |
Table 4-29 Auto Ticket Print Detail
Payload |
Type |
Req |
Definition |
refreshTicketQuantity |
Boolean |
True if the ticket quantity should be refreshed prior to printing. |
Table 4-30 Item Hierarchy Detail
Payload |
Type |
Req |
Definition |
departmentId |
Long(12) |
X |
The department identifier |
classId |
Long(12) |
The class identifier |
|
subclassId |
Long(12) |
The subclass identifier |
Table 4-31 Output Data Definition
Attribute |
Type |
Definition |
productGroupId |
Long(12) |
The product group identifier. |
status |
Integer(1) |
Indicates the status of the product group: (0) Active, (1) Canceled |
Figure 4-1 Example input unitCountDetail
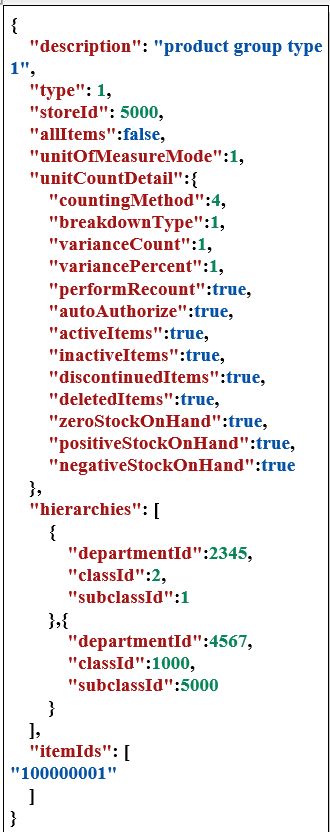
Figure 4-2 Example input unitAmountCountDetail:
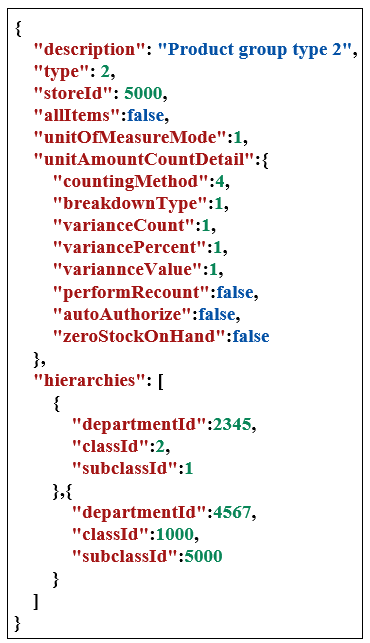
Figure 4-3 Example input problemLineCountDetail:
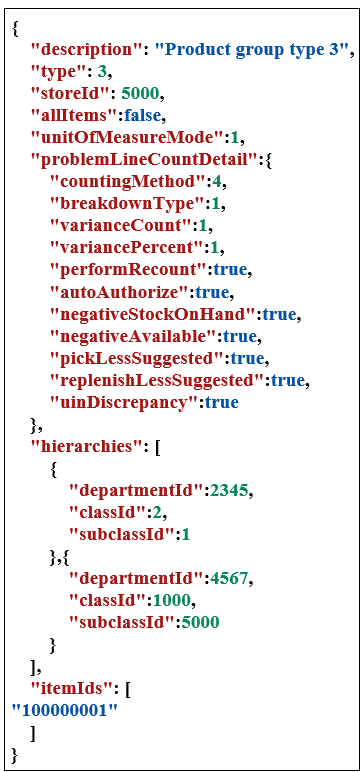
Figure 4-4 Example input autoAdjustmentDetail:
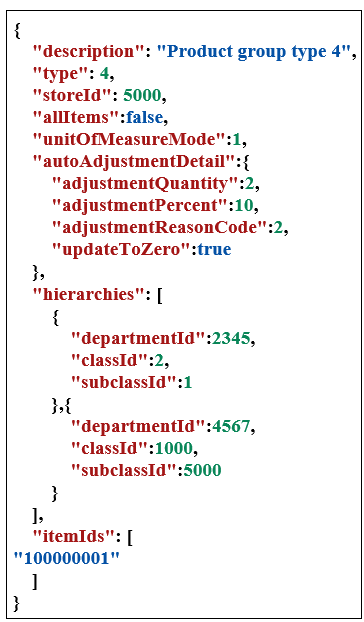
Figure 4-5 Example input autoTicketPrintDetail:
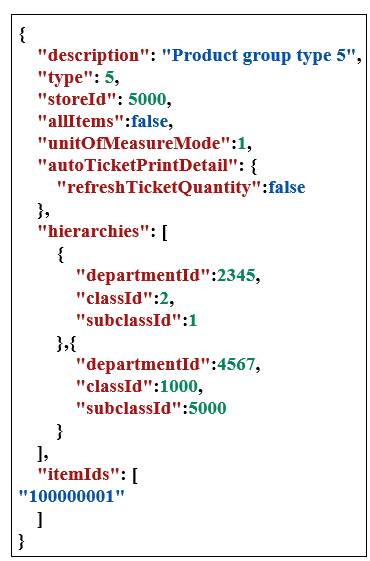
Figure 4-6 Example input replenishmentDetail:
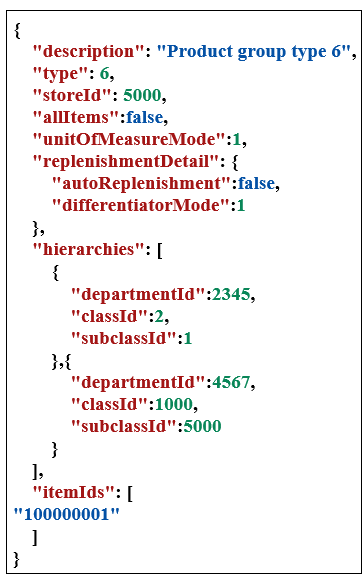
Figure 4-7 Example input storeOrderDetail:
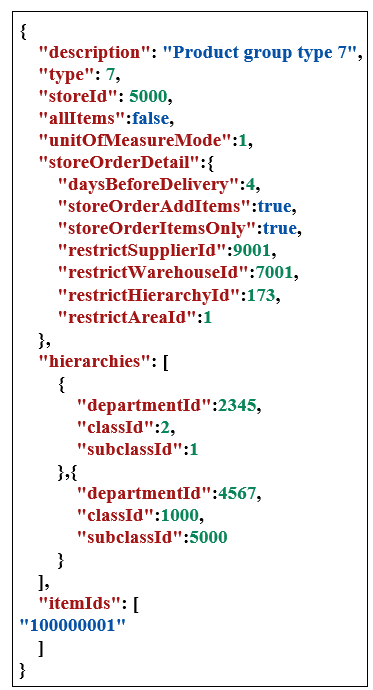
API: updateProductGroup
This API is used to modify a product group.
See API createProductGroup for the data definition of the product group type data objects.
API Basics
Table 4-32 API Basics
Endpoint URL |
{base URL}/{productGroupId} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the product group |
Input |
Product Group |
Output |
Product Group Status |
Input Data Definition
Payload |
Type |
Req |
Definition |
storeId |
Long(10) |
The unique identifier of a store associated to the product group. This will be blank/null if the product group is for all stores. If this is altered for a unit and amount group already assigned to schedules, it may return a validate error that it can no longer be altered. |
|
description |
String(100) |
X |
A description of the product group. |
allItems |
Boolean |
True if the product group is for all items, false otherwise. If all items, any hierarchies and items included will be ignored. Unit of measure type for pick lists. See Index. |
|
unitOfMeasureMode |
Integer |
Unit of measure type for pick lists. See Index. |
|
autoAdjustmentDetail |
ProductGroupAdjustmentIdo |
Details about an auto inventory adjustment product group. This is only processed and is required if the type is auto inventory adjustment type. |
|
autoTicketPrintDetail |
ProductGroupTicketPrintIdo |
Details about an auto ticket print product group. This is only processed and is required if the type is auto ticket print. |
|
replenishmentDetail |
ProductGroupReplenishmentIdo |
Details about a replenishment product group. This is only processed and is required if the type is a shelf replenishment type. |
|
storeOrderDetail |
ProductGroupStoreOrderIdo |
Details about a store order product group. This is only processed and is required if the type is the store order type. |
|
unitCountDetail |
ProductGroupUnitCountIdo |
Details about a unit stock count product group. This is only processed and is required if the type is a stock count type. |
|
unitAmountCountDetail |
ProductGroupUnitAmountCountIdo |
Details about a unit and amount stock count product group. This is only processed and is required if the type is a stock count type. |
|
problemLineCountDetail |
ProductGroupProblemLineCountIdo |
Details about a problem line stock count product group. This is only processed and is required if the type is a stock count type. |
|
hierarchies |
ProductGroupHierarchyIdo |
A list of up to 5,000 unique merchandise item hierarchies that belong to the group (avoid duplicates or overlapping hierarchies). |
|
itemIds |
List<String(25)> |
A list of up to 5,000 unique item SKU numbers on the product group. |
Figure 4-8 Example Input unitCountDetail:
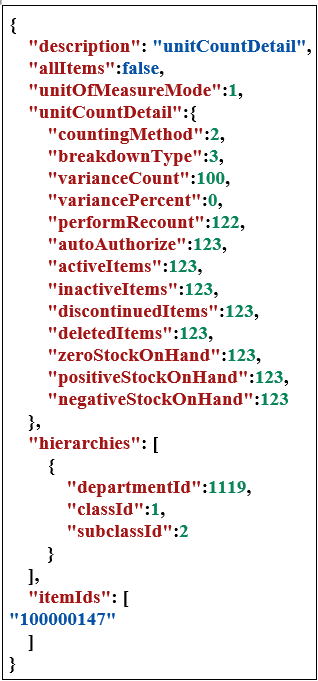
Figure 4-9 Example Input unitAmountCountDetail:
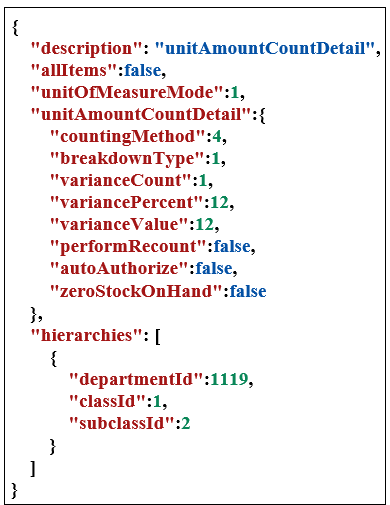
Figure 4-10 Example Input problemLineCountDetail:
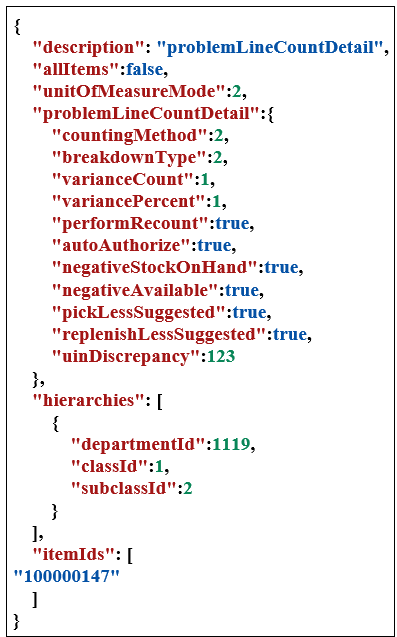
Figure 4-11 Example Input autoAdjustmentDetail:
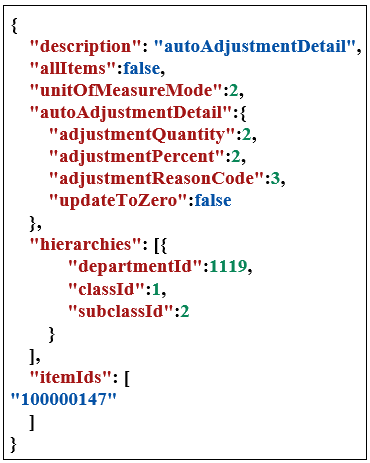
Figure 4-12 Example Input autoTicketPrintDetail:
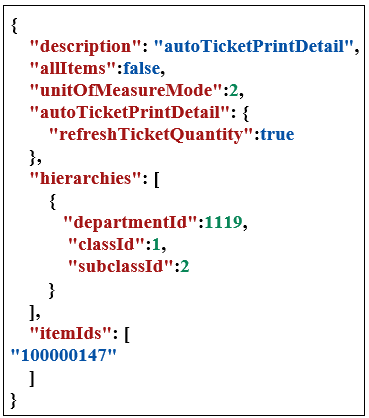
Figure 4-13 Example Input replenishmentDetail:
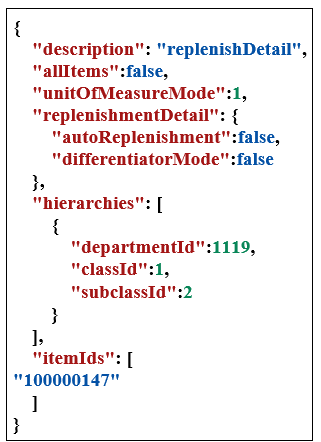
Figure 4-14 Example Input storeOrderDetail:
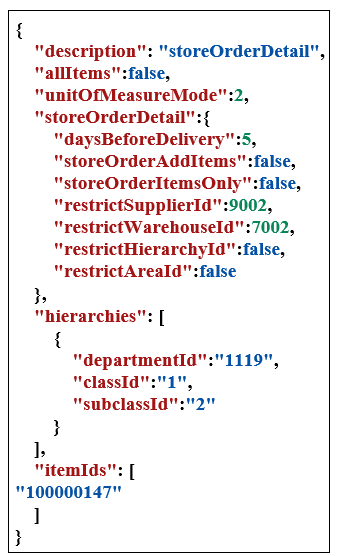
API: cancelProductGroup
Cancels the product group.
Table 4-33 API Basics
Endpoint URL |
{base URL}/{productGroupId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the product group |
Index
Table 4-34 Product Group Type
ID |
Description |
1 |
Stock Count: Unit |
2 |
Stock Count: Unit and Amount |
3 |
Stock Count: Problem Line |
4 |
Auto Inventory Adjustment |
5 |
Auto Ticket Print |
6 |
Shelf Replenishment |
7 |
Store Order |
Table 4-35 Product Group Status
ID |
Description |
1 |
Active |
2 |
Canceled |
Table 4-36 Unit of Measure Mode
ID |
Description |
1 |
Standard |
2 |
Cases |
3 |
Preferred |
Table 4-37 Shelf Replenishment Differentiator Mode
ID |
Description |
1 |
Differentiator 1 |
2 |
Differentiator 2 |
3 |
Differentiator 3 |
4 |
Differentiator 4 |
Table 4-38 Stock Counting Method
ID |
Description |
1 |
Guided |
2 |
Unguided |
3 |
Third Party |
4 |
Auto |
Table 4-39 Stock Count Breakdown Type
ID |
Description |
1 |
Department |
2 |
Class |
3 |
Subclass |
4 |
Location |
5 |
None |
Rest Service: Security
This service provides an end point for the management of security information within SIOCS.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api
API Definitions
Table 4-40 Security API Definitions
API |
Description |
Import Users |
Imports a collection of user security information changes and processes them synchronously. This allows up to 2,000 users. |
Delete Users |
Deletes security information for the specified users within SIOCS. |
API: Import Users
Imports a collection of user security information changes and processes them synchronously. This allows up to 2,000 users.
Table 4-41 API Basics
Endpoint URL |
/security/import/users |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
synchronous |
Input |
Query parameters |
Output |
N/A |
Max Response |
2000 |
Table 4-42 Input Data Definition
Parameter |
Format |
Required |
Definition |
users |
array |
No |
A list of security changes to import. See below: |
Security Changes to Import |
|||
userName |
string(text128) |
Yes |
The user name of the user. |
addedStores |
array[Integer](int10) |
No |
The stores to be added to the user. |
removedStores |
array[Integer](int10 |
No |
The stores to be removed from the user. |
addedRoles |
array |
No |
The roles to be assigned to the user: roleName — The name of the role, string(text128), required storeId — The identifier of the store for a specific store assignment, or no value for assignment applying to all allowed stores., BigDecimal(int10), optional startDate — The date the role assignment should start to be allowed., date-time, optional endDate — The date the role assignment should stop being allowed., date-time, optional |
removedRoles |
array |
No |
The role assignments to be removed from the user: roleName — The name of the role, string(text128), required storeId — The identifier of the store for a specific store assignment, or no value for assignment applying to all allowed stores., BigDecimal(int10), optional |
Example Input ImportUsers
{
"users": [
{
"userName": "abc",
"addedStores":[1000, 2000],
"removedStores":[1001, 2001],
"addedRoles": [
{
"roleName": "role_a",
"storeId": 3000,
"endDate": "2032-11-19T23:59:59-05:00"
},
{
"roleName": "role_c",
"startDate": "2022-11-19T23:59:59-05:00"
},
{
"roleName": "role_e",
}
],
"removedRoles": [
{
"roleName": "role_c",
"storeId": 3001
},
{
"roleName": "role_d"
}
]
}
]
}
Table 4-43 Responses
Code |
Description |
202 |
Accepted |
400 |
Bad Request Example:
|
API: Delete Users
Deletes security information for the specified users within SIOCS.
Table 4-44 API Basics
Endpoint URL |
/security/users/delete |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
|
Input |
Query parameters |
Output |
N/A |
Max Response |
Table 4-45 Query Parameter Definitions
Property |
Format |
Required |
Definition |
userNames |
string(text128) |
Yes |
A list of user names to delete information for. Example:
|
Table 4-46 Responses
Code |
Description |
202 |
Accepted |
400 |
Bad Request Example:
|
REST Service: Reason Code
This service allows and external system to retrieve available reason codes. Reason codes are attached to inventory adjustments or shipments.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/ reasoncodes
API Definitions
API | Description |
---|---|
Find Adjustment Reason Codes |
Finds reason codes available for inventory adjustments. |
Find Shipment Reason Codes |
Finds reason codes available for shipments. |
API: Find Adjustment Reason Codes
This API is used to find reason codes available for inventory adjustments.
API Basics
Endpoint URL |
{base URL} /adjustments |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of inventory adjustment reasons |
|
Max Response Limit |
N/A |
Output Data Definition
Attribute |
Data Type |
Description |
|
reasonId |
Long |
The unique identifier of the inventory adjustment reason code. |
|
reasonCode |
Integer |
Unique reason code associated to external systems. |
|
description |
String |
A description of the inventory reason code. |
|
dispositionCode |
Integer |
The inventory disposition associated to the code. |
|
dispositionDescription |
String |
A description of the inventory disposition associated to the code (not translated). |
|
fromNonSellableType |
Long |
From unavailable sub-bucket (indicates the sub-bucket of disposition of stock movement) |
|
fromNonSellableTypeDescription |
String |
The description of the from unavailable sub-bucket (not translated). |
|
toNonSellableType |
Long |
To unavailable sub-bucket (indicates the sub-bucket of disposition of stock movement) |
|
toNonSellableTypeDescription |
String |
The description of the to unavailable sub-bucket (not translated). |
|
systemRequired |
Boolean |
True indicates the reason code is required for the system to function. A system required reason code cannot be deactivated. |
|
displayable |
Boolean |
True indicates the reason code can be used by a transactional inventory adjustment created by an entity other than EICS internal service. |
|
publish |
Boolean |
- |
True indicating inventory movements with this reason code should be published to external systems |
Example Input
[
{
"reasonId": 1,
"reasonCode": 1,
"description": “invAdjReason.1",
"dispositionCode": 4,
"dispositionDescription": "inventoryDisposition.ATS-DIST",
"systemRequired": true,
"displayable": false,
"publish": true
},
{
"reasonId": 2,
"reasonCode": 81,
"description": "invAdjReason.81",
"dispositionCode": 4,
"dispositionDescription": "inventoryDisposition.ATS-DIST",
"systemRequired": false,
"displayable": true,
"publish": true
}
]
API: Find Shipment Reason Codes
This API is used to find the reason codes available for shipments.
API Basics
Endpoint URL |
{base URL} /shipments |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of reasons codes |
|
Max Response Limit |
N/A |
Output Data Definition
Attribute |
Data Type |
Description |
reasonId |
Long |
The unique identifier of the inventory adjustment reason code. |
reasonCode |
String |
Unique reason code associated to external systems. |
description |
String |
A description of the inventory reason code (not translated). |
type |
Integer |
The Shipment Reason Code Type: See the Shipment Reason Code Type. |
useAvailable |
Boolean |
True if it should use available inventory, false otherwise. |
nonSellableTypeId |
Long |
An identifier of associated unavailable sub-bucket (indicates the sub-bucket of disposition of stock movement) |
Example Input
[
{
"reasonId": 1,
"reasonCode": "F",
"description": "shipmentReason.4.F",
"type": 4,
"useAvailable": true
},
{
“reasonId": 2,
"reasonCode": "O",
"description": "shipmentReason.4.O",
"type": 4,
"useAvailable": true
},
{
"reasonId": 3,
"reasonCode": "U",
"description": "shipmentReason.4.U",
"type": 4,
"useAvailable": false,
"nonSellableTypeId": 1
}
]
Additional Data Definitions
Shipment Reason Code Type
Value |
Definition |
1 |
Store |
2 |
Supplier |
3 |
Warehouse |
4 |
Finisher |
5 |
Customer |
Rest Shipping
This service integrates various shipping support information with an external application. This is primarily various lookups of shipping data such as carriers and package sizes to use within shipping transactions.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/shipping
APIs
Table 4-47 APIs
API |
Description |
findCarriers |
Finds all the carriers available for shipping. |
findCarrierServices |
Finds all the carrier services available for shipping. |
findCartonSizes |
Finds the available sizes of cartons for shipping. |
findWeightUoms |
Finds all the various units of measurement of the carton measurements. |
findMotives |
Finds all the motives available for a shipping type. |
API: findCarriers
This API is used to find all the carriers available for shipping.
Table 4-48 API Basics
Endpoint URL |
/carriers |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Output |
Collection of carriers |
Table 4-49 Output Data Definition
Attribute |
Type |
Definition |
carrierId |
Long(10) |
The unique identifier of the carrier. |
code |
String(4) |
A unique character code for the carrier. |
description |
String(128) |
A description or name of the carrier. |
manifestType |
Integer(1) |
The carrier delivery manifest type (see Additional Data Definitions) |
API: findCarrierServices
This API is used to find all the carrier services available for shipping.
Table 4-50 API Basics
Endpoint URL |
/carrierservices |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Output |
Collection of carriers |
Table 4-51 Output Data Definition
Attribute |
Type |
Definition |
carrierServiceId |
Long(10) |
The unique identifier of the carrier service. |
code |
String(6) |
A unique character code for the carrier service. |
description |
String(128) |
A description or name of the carrier service. |
averageDeliveryDays |
Integer(4) |
The average number of days it takes to deliver using this service. |
carrierId |
Long(10) |
The unique identifier of the carrier this service is used with. |
defaultService |
Boolean |
True is this is the default service type for the carrier, false otherwise. |
weightRequired |
Boolean |
True is weight is required for this carrier service, false otherwise. |
cartonSizeRequired |
Boolean |
True if dimensions/size is required for this carrier service, false otherwise. |
API: findCartonSizes
This API is used to find all the carton services available for shipping at the particular store.
Table 4-52 API Basics
Endpoint URL |
/cartonsizes/{storeId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The store identifier of the store to retrieve carton sizes for. |
Output |
Collection of carton sizes |
Table 4-53 Output Data Definition
Attribute |
Type |
Definition |
cartonSizeId |
Long(12) |
A unique identifier of this particular carton size definition. |
storeId |
Long(10) |
The store identifier the carton size is used at. |
description |
String(120) |
A description of the carton size. |
height |
BigDecimal(12,4) |
The height of the carton in units of measure. |
width |
BigDecimal(12,4) |
The width of the carton in units of measure. |
length |
BigDecimal(12,4) |
The length of the carton in units of measure. |
unitOfMeasure |
String(4) |
The unit of measure of the height, width, and length. |
API: findWeightUoms
This API is used to find all the weight unit of measures available for shipping.
Table 4-54 API Basics
Endpoint URL |
/weightuoms |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Output |
Collection of shipping weight units of measure |
Attribute |
Type |
Definition |
uom |
String(4) |
The unit of measure |
description |
String(120) |
The unit of measure description |
API: findMotives
This API is used to find all the motives available for a shipping type. See index for available shipment types.
Table 4-55 API Basics
Endpoint URL |
/motives/(shipmentType} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The shipment type to retrieve motives for (see Additional Data Definitions) |
Output |
Collection of carton sizes |
Table 4-56 Output Data Definition
Attribute |
Type |
Definition |
id |
Long(18) |
The unique identifier of the shipping motive |
code |
String(6) |
The external code of the shipping motive |
description |
String(120) |
A description of the motive |
sequence |
Long |
The sequence in which the motive should appear in a list |
REST Service: Stock Count
The stock count services handle tasks related to a stock count.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/stockcounts
API Definitions
API | Description |
---|---|
Snapshot Count |
Snapshots a stock count capturing the current stock on hand quantities. |
API: Snapshot Stock Count
Executes a snapshot of the stock count capturing the current stock on hand quantity of each item on the count. The process of doing a snapshot first determines whether the stock count needs a snapshot and only snapshots those stock counts or stock count children that need a snapshot. If the stock count or stock count child does not need a snapshot, the service is considered successful.
API Basics
Endpoint URL |
/{stockCountId}/snapshot |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Path Parameter Definitions
Attribute | Definition |
---|---|
stockCountId |
The internal identifier of the stock count header. |
REST Service: Store
This service integrates the store foundation data with an external application. Store integration is controlled by the MPS Work Type: DcsStore.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/stores
API Definitions
API | Description |
---|---|
Import Stores |
Imports a collection of stores. |
Delete Store |
Deactivate a single store. |
Read Store |
Read store information based on an identifier (or link) |
Find Stores |
Lookup store information based on a collection of store identifiers. |
Find Associated Stores |
Lookup store associated to the specified input store. |
Find Auto Receive Stores |
Lookup stores that are allowed to auto receive from the specified input store. |
Find Transfer Zone Stores |
Lookup stores that are in the same transfer zone as the specified input store. |
Find Adjustment Reason Codes |
Finds reason codes available for inventory adjustments. |
Find Shipment Reason Codes |
Finds reason codes available for shipments. |
API: Import Stores
Imports a collection of stores. This allows 1,000 stores per input call. All imported stores will be inventory-holding regular stores.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of Store to Import |
|
Output |
None |
|
Max Response Limit |
1000 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
stores |
List of stores to import |
Yes |
A collection of up to 1000 stores to import. |
Stores Data Definition
Attribute |
Data Type |
Required |
Description |
storeId |
Long(10) |
Yes |
The store identifier |
storeName |
String(150) |
Yes |
The name of the store. |
languageCode |
String(3) |
- |
The language code of the store. |
countryCode |
String(3) |
- |
The country code of the store. |
currencyCode |
String(3) |
- |
The currency code of the store. |
timezone |
String(80) |
- |
The timezone of the store. |
transferZoneId |
String(128) |
Yes |
The transfer zone identifier of the store. |
organizationUnitId |
String(15) |
- |
The organization unit identifier of the store. |
managedStore |
Boolean |
- |
True indicates that EICS manages the inventory, false indicates it does not. |
customerOrdering |
Boolean |
- |
True indicates this store can take customer orders, false indicates it does not. |
Example Output
{
"stores": [
{
"storeId": 5002,
"storeName": "Leamington Spa",
"languageCode": "EN",
"countryCode": "US",
"currencyCode": "USD",
"timezone": "America/Los_Angeles",
"transferZoneId": "1000",
"organizationUnitId": "1111",
"managedStore": true,
"allowsCustomerOrders": false
},
{
"storeId": 5003,
"storeName": "Leamington Spa",
"languageCode": "EN",
"countryCode": "US",
"currencyCode": "USD",
"timezone": "America/Los_Angeles",
"transferZoneId": "1000",
"organizationUnitId": "1111",
"managedStore": true,
"allowsCustomerOrders": false
}
]
}
API: Delete Store
Delete a store. Prior to placing the request to delete into the MPS queue, it validates that the store exists and that the store contains no items ranged to it.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{storeId}/delete |
||
Method |
POST |
||
Successful Response |
202 Accepted |
||
Processing Type |
Asynchronous |
||
Input |
None |
||
Output |
None |
||
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
API: Read Store
Retrieve information about a store based on a single unique store identifier or link.
API Basics
Endpoint URL |
{base URL}/{storeId} |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
Store |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Output Data Definition
Attribute |
Data Type |
Description |
storeId |
Long |
The store identifier |
storeName |
String |
The name of the store. |
languageCode |
String |
The language code of the store |
countryCode |
String |
The country code of the store. |
currencyCode |
String |
The currency code of the store |
timezone |
String |
The timezone of the store |
transferZoneId |
String |
The transfer zone identifier of the store |
organizationUnitId |
String |
The organization unit identifier of the store. |
managedStore |
boolean |
True indicates that EICS manages the inventory, false indicates it does not. |
customerOrdering |
boolean |
True indicates this store can take customer orders, false indicates it does not. |
Example Output
{
"links": [
{
"href": "/stores/5000",
"rel": "self"
},
{
"href": "/stores/5000/delete",
"rel": "delete"
}
],
"storeId": 5000,
"storeName": "Solihull",
"languageCode": "EN",
"countryCode": "US",
"currencyCode": "USD",
"timezone": "America/Chicago",
"transferZoneId": "1000",
"organizationUnitId": "1111",
"managedStore": true,
"allowsCustomerOrders": false
}
API: Find Stores
Find stores based on a list of potential unique store identifiers. It allows a maximum of 1500 store identifiers.
API Basics
Endpoint URL |
{base URL}/find |
|
Method |
POST |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
List of stores ids |
|
Output |
List of stores |
|
Max Response Limit |
1500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
stores |
List of stores Ids |
Yes |
A collection of up to 1500 stores to read. |
Example Input
{
"storeIds": [
5000,
5001
]
}
Output Data Definition
Attribute |
Data Type |
Description |
Stores |
List of Stores |
A collection containing the store information |
Stores Data Definition
Attribute |
Data Type |
Required |
Description |
|
storeId |
Long |
Yes |
The store identifier |
|
storeName |
String |
The name of the store. |
||
languageCode |
String |
The language code of the store |
||
countryCode |
String |
The country code of the store. |
||
currencyCode |
String |
The currency code of the store |
||
timezone |
String |
The timezone of the store |
||
transferZoneId |
String |
The transfer zone identifier of the store |
||
organizationUnitId |
String |
The organization unit identifier of the store. |
||
managedStore |
boolean |
True indicates that EICS manages the inventory, false indicates it does not. |
||
customerOrdering |
boolean |
True indicates this store can take customer orders, false indicates it does not. |
Example Output
[
{
"links": [
{
"href": "/stores/5000",
"rel": "self"
},
{
"href": "/stores/5000/delete",
"rel": "delete"
}
],
"storeId": 5000,
"storeName": "Solihull",
"languageCode": "EN",
"countryCode": "US",
"currencyCode": "USD",
"timezone": "America/Chicago",
"transferZoneId": "1000",
"organizationUnitId": "1111",
"managedStore": true,
"allowsCustomerOrders": false
},
{
"links": [
{
"href": "/stores/5001",
"rel": "self"
},
{
"href": "/stores/5001/delete",
"rel": "delete"
}
],
"storeId": 5001,
"storeName": "Nottingham",
"languageCode": "EN",
"countryCode": "US",
"currencyCode": "USD",
"timezone": "America/New_York",
"transferZoneId": "1000",
"organizationUnitId": "1111",
"managedStore": true,
"allowsCustomerOrders": false
}
]
API: Find Associated Stores
Find potential associated stores (buddy stores) to the specified input store.
API Basics
Endpoint URL |
{base URL}/{storeId}/associated |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of stores |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Output Data Definition
Attribute |
Data Type |
Required |
Description |
Stores |
List of Stores Ids |
Yes |
A collection containing the store Ids |
Stores Data Definition
Attribute |
Data Type |
Required |
Description |
storeId |
Long |
Yes |
The internal identifier of the store. |
Example Output
[
{
"links": [
{
"href": "/stores/5001",
"rel": "self"
},
{
"href": "/stores/5001/delete",
"rel": "delete"
}
],
"storeId": 5001
},
{
"links": [
{
"href": "/stores/5002",
"rel": "self"
},
{
"href": "/stores/5002/delete",
"rel": "delete"
}
],
"storeId": 5002
}
]
API: Find Auto Receive Stores
Find stores that are allowed to auto receive from the specified input store.
API Basics
Endpoint URL |
{base URL}/{storeId}/autoreceive |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of stores |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Example Output
[
{
"links": [
{
"href": "/stores/5001",
"rel": "self"
},
{
"href": "/stores/5001/delete",
"rel": "delete"
}
],
"storeId": 5001
},
{
"links": [
{
"href": "/stores/5002",
"rel": "self"
},
{
"href": "/stores/5002/delete",
"rel": "delete"
}
],
"storeId": 5002
}
]
API: Find Transfer Zone Stores
Find stores that are available within the transfer zone of the input store.
API Basics
Endpoint URL |
{base URL}/{storeId}/transferzone |
|
Method |
GET |
|
Successful Response |
200 OK |
|
Processing Type |
Synchronous |
|
Input |
None |
|
Output |
List of stores |
|
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Output Data Definition
Attribute |
Data Type |
Required |
Description |
Stores |
List of Stores Ids |
Yes |
A collection containing the store Ids |
Stores Data Definition
Attribute |
Data Type |
Require d |
Description |
storeId |
Long |
Yes |
The internal identifier of the store. |
Example Output
[
{
"links": [
{
"href": "/stores/5001",
"rel": "self"
},
{
"href": "/stores/5001/delete",
"rel": "delete"
}
],
"storeId": 5001
},
{
"links": [
{
"href": "/stores/5002",
"rel": "self"
},
{
"href": "/stores/5002/delete",
"rel": "delete"
}
],
"storeId": 5002
}
]
REST Service: Store Item
This service integrates the store item foundation data with an external application. Store integration is controlled by the MPS Work Type: DcsItemLocation.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/storeitems
API Definitions
API |
Description |
Import Store Items |
Imports items at a store location. |
Remove Store Items |
Deactivate items at a store location. |
Import Replenishment Items |
Imports replenishment item information. |
Remove Replenishment Items |
Deactivate replenishment item information. |
API: Import Store Items
Imports store items into the system.
If more than 10,000 items are included in a single call, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of store Items to Import |
|
Output |
None |
|
Max Response Limit |
10,000 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of stores items to import |
Yes |
A collection of up to 10,000 stores items to import. |
Stores Items Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String(25) |
Yes |
The unique item identifier (sku number). |
shortDescription |
String(255) |
- |
A short description of the item at this store. |
longDescirption |
String(400) |
- |
A long description of the item at this store. |
status |
String |
Yes |
See Index (Item Status) |
primarySupplierId |
Long(10) |
- |
The unique identifier of the primary supplier of the item to this store location. |
storeControlPricing |
Boolean |
- |
True indicates the item price can be controlled by the store. |
rfid |
Boolean |
- |
True indicates the item is RFID tagged. |
defaultCurrencyCode |
String(3) |
- |
The default currency of the item's price at this store. |
purchaseType |
Long |
- |
See Index (Purchase Type) |
uinType |
Integer |
- |
See Index (UIN Type) |
uinCaptureTime |
Integer |
- |
See Index (UIN Capture Time) |
uinLabelCode |
Long |
- |
The UIN label unique identifier. |
uinExternalCreateAllowed |
Boolean |
- |
True if an external system can create a UIN, false otherwise. |
Example Input
{
"items": [
{
"itemId": "100637121",
"defaultCurrencyCode": "USB",
"longDescription": "TestDescriptionAA",
"primarySupplierId": 1,
"purchaseType": 1,
"rfid": true,
"shortDescription": "TestShortAA",
"status": 1,
"storeControlPricing": false,
"uinCaptureTime": 1,
"uinExternalCreateAllowed": true,
"uinLabelCode": "SN",
"uinType": 1
}
]
}
Additional Data Definitions
Item Status
Value |
Definition |
1 |
Active |
2 |
Discontinued |
3 |
Inactive |
4 |
Auto Stockable |
Purchase Type
Value |
Definition |
1 |
Normal Merchandise |
2 |
Consignment Stock |
3 |
Concession Items |
UIN Capture Time
Value |
Definition |
1 |
Sale |
2 |
Store Receiving |
UIN Type
Value |
Definition |
1 |
Serial Number |
2 |
Auto-Generated Serial Number |
API: Remove Store Items
This will mark items as no longer usable. When all data is cleared out of transactions that reference this data, later batch jobs will eventually delete the material.
If more than 1000 items are included in a single call, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{storeId}/remove |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
None |
|
Output |
None |
|
Max Input Limit |
1000 |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of items to remove |
Yes |
A collection of up to 1000 items to remove. |
Example Input
{
"itemIds": [
"100637121",
"100637113"
]
}
API: Import Replenishment Items
Imports item replenishment information for an item at a store location.
If more than 1000 items are included in a single call, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{storeId}/replenish/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
None |
|
Output |
List of Items Replenishment to import |
|
Max Input Limit |
1000 |
Path Parameter Definitions
Attribute |
Definition |
storeId |
The internal identifier of the store. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of Item Replenishment Import |
Yes |
The item replenishment information to import. |
Item Replenishment Import Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String (25) |
Yes |
The unique item identifier (sku number). |
replenishmentMethod |
String(6) |
A code representing the replenishment method. (SO indicates Store Order). |
|
rejectStoreOrder |
Boolean |
True indicates store orders must be on or after the next delivery date or should be rejected. |
|
multipleDeliveryPerDayAllowed |
Boolean |
True indicates the item allows multiple deliveries per day at the location. |
|
nextDeliveryDate |
Date |
The next delivery date of the time based on its replenishment type. |
Example Input
{
"items": [
{
"itemId": "100637121",
"replenishmentMethod": "AB",
"rejectStoreOrder": false,
"multipleDeliveryPerDayAllowed": false,
"nextDeliveryDate": "2022-11-19T23:59:59-05:00"
}
]
}
API: Remove Replenishment Items
Clears the replenishment item information from within the store item setting the replenishment properties to empty, null, or default flag settings.
If more than 1000 items are included in a single call, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/replenish/remove |
|
Method |
POST |
|
Successful Response |
200 OK |
|
Processing Type |
Asynchronous |
|
Input |
List of item ids |
|
Output |
None |
|
Max Input Limit |
1000 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
Items |
List of item Ids |
Yes |
A collection of up to 1000 items to read. |
Example Input
{
"itemIds": [
"100637121",
"100637113"
]
REST Service: Store Order
This service allows query and approval of store orders.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/storeorders
APIs
API |
Description |
Find Orders |
Finds store order header records based on search criteria |
Read Order |
Reads a store order |
Approve Order |
Approves the store order and notifies external system |
Cancel Order |
Cancels the store order and notifies external system |
API: Read Order
API Basics
Endpoint URL |
{base URL}/{storeOrderId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
The store order |
Max Response Limit |
NA |
Output Data Definition
Attribute |
Type |
Definition |
storeOrderId |
Long(12) |
The unique identifier of the record. |
storeId |
Long(10) |
The unique identifier of the store. |
externalTransferId |
String(128) |
An external transfer identifier that this store order is associated to. |
externalPurchaseOrderId |
String(128) |
An external purchase order identifier that this store order is associated to. |
externalReference |
String(128) |
A reference to the store order in an external system. |
parentReference |
Long(12) |
Reference to a parent transfer or order. |
description |
String(2000) |
A description of the store order or cause of the store order. |
status |
Integer(2) |
The status: See Index |
origin |
Integer(2) |
The origin: See Index |
requestedDeliveryDate |
Date |
The date that the store requests the delivery arrive by. |
autoApproveDate |
Date |
The date the record was automatically approved in EICS. |
addItemsAllowed |
Boolean |
Y indicates new items can be added to the store order. |
replenishmentItemsOnly |
Boolean |
Y indicates only store order replenishment items can be added to the store order. |
contextId |
Long(18) |
A context identifier associated to the store order. |
deliverySlotId |
Long(15) |
The unique identifier of the delivery time. |
productGroupId |
Long(12) |
The unique identifier of a product group associated to the order. |
productGroupDescription |
String(250) |
The description of the product group associated to the order. |
restrictToSupplierId |
Long(12) |
Allow only items on the store order that are associated to this supplier. |
restrictToWarehouseId |
Long(12) |
Allow only items on the store order that are associated to this warehouse. |
restrictToHierarchyId |
Long(12) |
Allow only items on the store order that belong to this merchandise hierarchy. |
restrictToAreaId |
Long(12) |
Allow only items on the store order that are associated to this store sequence area. |
createDate |
Date |
The date the record was created in EICS. |
createUser |
String(128) |
The user that created the record in EICS. |
externalCreateDate |
Date |
The date the store order was created in an external system. |
updateDate |
Date |
The date the record was last updated in EICS. |
approvedDate |
Date |
The date the record was approved in EICS. |
approvedUser |
String(128) |
The user that approved the record in EICS. |
lineItems |
Collection |
A collection of Store Order Line Items |
Store Order Line Item
Column |
Type |
Definition |
lineId |
Long(12) |
The unique identifier of the record. |
itemId |
String(25) |
The unique identifier of the item. |
approvedQuantity |
BigDecimal(20,4) |
The quantity of item ordered. |
suggestedQuantity |
BigDecimal(20,4) |
The quantity of item ordered from the external system. |
caseSize |
BigDecimal(10,2) |
The case size of the item ordered. |
unitCostCurrency |
String(3) |
The currency of the unit cost. |
unitCostAmount |
BigDecimal(12,4) |
The value of the unit cost. |
deliverySlotId |
Long(14) |
The unique identifier of the delivery time. |
API Find Orders
API is used to find lightweight transaction headers for store orders.
API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of store order headers |
Max Response Limit |
5,000 |
Query Parameters
Attribute |
Type |
Definition |
storeId |
Long(12) |
Include only records for this store. |
externalReference |
String(128) |
Include only records with this external transfer identifier. |
externalTransferId |
String(128) |
Include only records with this external transfer identifier. |
externalPurchaseOrderId |
String(128) |
Include only records with this external transfer identifier. |
status |
Integer |
Include only records with this status. See StockOrderCriteriaStatus Index. |
itemId |
String(25) |
Include only records with this item on them. |
updateDateFrom |
Date |
Include records with a last update date on or after this date. |
updateDateTo |
Date |
Include records with a first update date on or before this date. |
Output Data Definition
Column |
Type |
Definition |
storeOrderId |
Long(12) |
The unique identifier of the record. |
storeId |
Long(10) |
The unique identifier of the store. |
externalTransferId |
String(128) |
An external transfer identifier that this store order is associated to. |
externalPurchaseOrderId |
String(128) |
An external purchase order identifier that this store order is associated to. |
externalReference |
String(128) |
A reference to the store order in an external system. |
description |
String(2000) |
A description of the store order or cause of the store order. |
status |
Integer(2) |
The status: See Index |
requestedDeliveryDate |
Date |
The date that the store requests the delivery arrive by. |
updateDate |
Date |
The date the record was last updated in EICS. |
API: Approve Order
This operation will approve the store order and send notifications and updates of the approval to an external system.
API Basics
Endpoint URL |
{base URL}/{storeOrderId}/approve |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
The store order |
Max Response Limit |
N/A |
REST Service: Store Sequencing
This service defines operations to manage Store Sequence information.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/sequences
APIs
API |
Description |
Find Sequence Areas |
Finds sequence area header based on search criteria. |
Read Sequence Area |
Reads the details of a sequence area. |
Create Sequence Area |
Create a sequence area along with all its details. |
Update Sequence Area |
Replace the entire sequence area including all its details (a complete sequence area must be sent). |
Delete Sequence Area |
Delete a sequenced area and all its details. |
Create Sequence Item |
Create a single sequence item in a sequence area. |
Update Sequence Item |
Updates a single sequence item from a sequence area. |
Delete Sequence Item |
Removes a single sequence item from a sequence area. |
API: Find Sequence Areas
This API is used to search for sequence area headers. No items or detailed information is returned by this API. At least one criteria is required in order to search.
API Basics
Table 4-57 API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
Collection of Sequence Areas |
Max Response Limit |
15,000 |
Query Parameters
Table 4-58 Query Parameters
Attribute |
Type |
Definition |
storeId |
Long(12) |
Include only records for this store. |
areaType |
Integer(9) |
Area Type (see Index). |
departmentId |
Long(12) |
The unique identifier of a department associated to the area. |
classId |
Long(12) |
The unique identifier of a class within the department associated to the area. |
itemId |
String(25) |
Include only records with this item on them. |
Output Data Definition
Table 4-59 Output Data Definition
Attribute |
Type |
Definition |
sequenceAreaId |
Long(10) |
The unique identifier of the sequence area. |
storeId |
Long(10) |
The unique identifier of the store. |
description |
String(255) |
A description of this store sequence. |
areaType |
Integer(9) |
Area Type (see Index). |
departmentId |
Long(12) |
The unique identifier of a department associated to the area. |
classId |
Long(12) |
The unique identifier of a class within the department associated to the area. |
sequenceOrder |
Long(20 |
The order of this store sequence compared to other store sequences at the store. |
unsequenced |
Boolean |
True indicates that this is a default sequence area that contains all the items that have not been sequenced within a different area. |
API: Read Sequence Area
This API is used to read an entire sequence area including its details.
API Basics
Table 4-60 API Basics
Endpoint URL |
{base URL}/{sequenceAreaId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
N/A |
Output |
Sequence Area |
Max Response Limit |
N/A |
Path Parameter Definition
Table 4-61 Path Parameter Definition
Attribute |
Type |
Definition |
sequenceAreaId |
Long(10) |
The unique identifier of the sequence area. |
Output Data Definition
Table 4-62 Output Data Definition
Attribute |
Type |
Definition |
sequenceAreaId |
Long(10) |
The unique identifier of the sequence area. |
storeId |
Long(10) |
The unique identifier of the store. |
description |
String(255) |
A description of this store sequence. |
areaType |
Integer(9) |
Area Type (see Index). |
departmentId |
Long(12) |
The unique identifier of a department associated to the area. |
classId |
Long(12) |
The unique identifier of a class within the department associated to the area. |
sequenceOrder |
Long(20 |
The order of this store sequence compared to other store sequences at the store. |
unsequenced |
Boolean |
True indicates that this is a default sequence area that contains all the items that have not been sequenced within a different area. |
Items |
Collection |
Contains all the items in the area (see Store Sequence Item) |
Store Sequence Item
Table 4-63 Store Sequence Item
Payload |
Type |
Definition |
sequenceItemId |
Long(12) |
The unique area item identifier. |
itemId |
String(25) |
The unique identifier of the item |
sequenceOrder |
Long(20) |
The order of the item within its sequence. |
capacity |
BigDecimal(11,2) |
The amount of the item that can be contained in the area for that item at the unit of measure. |
width |
Long(12) |
The number of items that can fit across the width of the shelf. |
uomMode |
Integer(2) |
The mode of display of the unit of measure of the item. |
primaryLocation |
Boolean |
Y indicates this is the primary sequence for the item. |
ticketQuantity |
BigDecimal(11,2) |
The quantity of tickets that need to be printed for the item inventory area. |
ticketFormatId |
Long(10) |
The unique identifier of the ticket format used to print the tickets. |
API: Create Sequence Area
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Sequence Area Create Info |
Output |
Sequence Area Reference Info |
Max Response Limit |
1,000 items in the area |
Input Data Definition
Attribute |
Type |
Req |
Definition |
storeId |
Long(10) |
X |
The unique identifier of the store. |
description |
String(255) |
A description of this store sequence. |
|
areaType |
Integer(9) |
X |
Area Type (see Index). |
departmentId |
Long(12) |
The unique identifier of a department associated to the area. |
|
classId |
Long(12) |
The unique identifier of a class within the department associated to the area. |
|
sequenceOrder |
Long(20) |
X |
The order of this store sequence compared to other store sequences at the store. The sequence order must be unique for the set of areas within the store. |
items |
Collections |
X |
All the items that belong to this sequence area (see Sequence Area Item Create) |
Store Sequence Area Item Create
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the item |
sequenceOrder |
Long(20) |
X |
The order of the item within its sequence. |
capacity |
BigDecimal(11,2) |
X |
The amount of the item that can be contained in the area for that item at the unit of measure. |
width |
Long(12) |
The number of items that can fit across the width of the shelf. |
|
uomMode |
Integer(2) |
X |
The mode of display of the unit of measure of the item. |
primaryLocation |
Boolean |
Y indicates this is the primary sequence for the item. |
|
ticketQuantity |
BigDecimal(11,2) |
The quantity of tickets that need to be printed for the item inventory area. |
|
ticketFormatId |
Long(10) |
The unique identifier of the ticket format used to print the tickets. |
Output Data Definition
Attribute |
Type |
Definition |
sequenceAreaId |
Long(12) |
The newly created adjustment identifier. |
storeId |
Long(10) |
The store identifier. |
Example
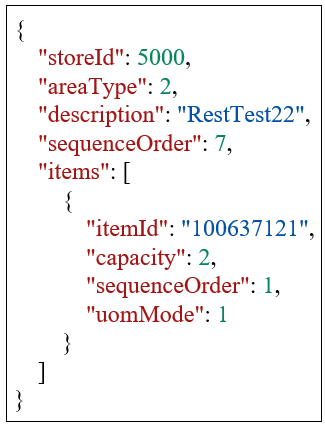
API: Update Sequence Area
This API is used to update a sequence area within a store.
This API will replace the entire sequence area with the new data passed to the API. Only the area identifier and store identifier will be preserved from previous data.
API Basics
Table 4-64 API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
Sequence Area Update Info |
Output |
N/A |
Max Response Limit |
1,000 items in the area |
Path Parameter Definition
Table 4-65 Path Parameter Definition
Attribute |
Type |
Definition |
sequenceItemId |
Long(12) |
The unique area item identifier |
Input Data Definition
Table 4-66 Input Data Definition
Attribute |
Type |
Req |
Definition |
description |
String(255) |
A description of this store sequence. |
|
areaType |
Integer(9) |
X |
Area Type (see Index). |
departmentId |
Long(12) |
The unique identifier of a department associated to the area. |
|
classId |
Long(12) |
The unique identifier of a class within the department associated to the area. |
|
sequenceOrder |
Long(20) |
X |
The order of this store sequence compared to other store sequences at the store. The sequence order must be unique for the set of areas within the store. |
items |
Collections |
X |
All the items that belong to this sequence area (see Sequence Area Item Update) |
Store Sequence Area Item Update
Table 4-67 Store Sequence Area Item Update
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the item |
sequenceOrder |
Long(20) |
X |
The order of the item within its sequence. |
capacity |
BigDecimal(11,2) |
X |
The amount of the item that can be contained in the area for that item at the unit of measure. |
width |
Long(12) |
The number of items that can fit across the width of the shelf. |
|
uomMode |
Integer(2) |
X |
The mode of display of the unit of measure of the item. |
primaryLocation |
Boolean |
Y indicates this is the primary sequence for the item. |
|
ticketQuantity |
BigDecimal(11,2) |
The quantity of tickets that need to be printed for the item inventory area. |
|
ticketFormatId |
Long(10) |
The unique identifier of the ticket format used to print the tickets. |
Example
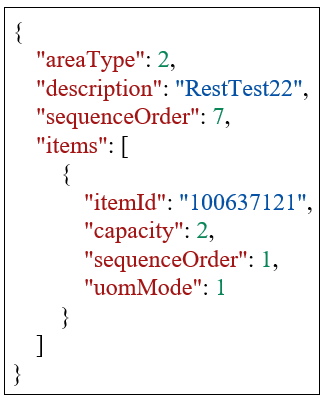
API: Delete Sequence Area
This API is used to delete a sequence area and all its items. This will not delete a sequence area currently being used by a sequenced stock count.
API Basics
Table 4-68 API Basics
Endpoint URL |
{base URL} |
Method |
DELETE |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
API: Create Sequence Item
This API is used to create a new sequence item within a sequence area.
API Basics
Table 4-69 API Basics
Endpoint URL |
{base URL}/{sequenceAreaId}/items |
Method |
POST |
Successful Response |
200 No Content |
Processing Type |
Synchronous |
Input |
Store Sequence Item Create |
Output |
Store Sequence Item |
Max Response Limit |
N/A |
Input Data Definition
Table 4-70 Input Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the item |
sequenceOrder |
Long(20) |
X |
The order of the item within its sequence. |
capacity |
BigDecimal(11,2) |
X |
The amount of the item that can be contained in the area for that item at the unit of measure. |
width |
Long(12) |
The number of items that can fit across the width of the shelf. |
|
uomMode |
Integer(2) |
X |
The mode of display of the unit of measure of the item. (see Index) |
primaryLocation |
Boolean |
Y indicates this is the primary sequence for the item. |
|
ticketQuantity |
BigDecimal(11,2) |
The quantity of tickets that need to be printed for the item inventory area. |
|
ticketFormatId |
Long(10) |
The unique identifier of the ticket format used to print the tickets. |
Example
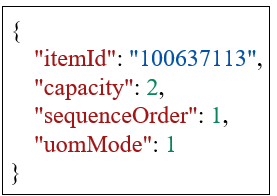
API: Update Sequence Item
This API is used to update a sequence item within a sequence area.
API Basics
Table 4-71 API Basics
Endpoint URL |
{base URL}/{sequenceAreaId}/items/{itemId} |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
Path Parameter Definition
Table 4-72 Path Parameter Definition
Attribute |
Type |
Definition |
sequencerAreaId |
Long(12) |
The unique sequence area identifier. |
itemId |
String(25) |
The item to be deleted from the sequence. |
Input Data Definition
Table 4-73 Input Data Definition
Payload |
Type |
Req |
Definition |
sequenceOrder |
Long(20) |
X |
The order of the item within its sequence. |
capacity |
BigDecimal(11,2) |
X |
The amount of the item that can be contained in the area for that item at the unit of measure. |
width |
Long(12) |
The number of items that can fit across the width of the shelf. |
|
uomMode |
Integer(2) |
X |
The mode of display of the unit of measure of the item. |
primaryLocation |
Boolean |
Y indicates this is the primary sequence for the item. |
|
ticketQuantity |
BigDecimal(11,2) |
The quantity of tickets that need to be printed for the item inventory area. |
|
ticketFormatId |
Long(10) |
The unique identifier of the ticket format used to print the tickets. |
Example
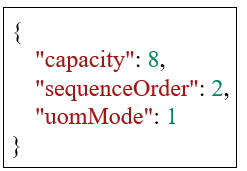
API: Delete Sequence Item
This API is used to delete a sequence item from an area.
API Basics
Table 4-74 API Basics
Endpoint URL |
{base URL}/{sequenceAreaId}/items/{itemId} |
Method |
DELETE |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
N/A |
Output |
N/A |
Max Response Limit |
N/A |
Path Parameter Definition
Table 4-75 Path Parameter Definition
Attribute |
Type |
Definition |
sequencerAreaId |
Long(12) |
The unique sequence area identifier. |
itemId |
String(25) |
The item to be deleted from the sequence. |
REST Service: Supplier
This service integrates supplier and supplier item foundation data.
Asynchronous supplier integration is processed through staged messages and is controlled by the MPS Work Type: DcsSupplier.
Asynchronous supplier item integration is processed through staged messages and is controlled by the MPS Work Type: DcsSupplierItem.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/suppliers
API Definitions
API |
Description |
Import Suppliers |
Imports supplier. |
Delete Supplier |
Deletes a supplier. |
Import Items |
Imports items for a supplier. |
Delete Items |
Deletes items from a supplier. |
Import Item UOMs |
Imports units of measure for a supplier item. |
Delete Item UOMs |
Deletes units of measure from a supplier item. |
Import Item Countries |
Imports countries for a supplier item. |
Delete Item Countries |
Deletes countries from a supplier item. |
Import Item Dimensions |
Imports items dimensions for a supplier item country. |
Delete Item Dimensions |
Deletes dimensions from a supplier item country. |
Import Item Manufacturers |
Imports manufacturers for a supplier item country. |
Delete Item Manufacturers |
Deletes manufacturers from a supplier item country. |
API: Import Suppliers
Imports a list of suppliers.
If the import contains more than 500 suppliers, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/import |
||
Method |
POST |
||
Successful Response |
202 Accepted |
||
Processing Type |
Asynchronous |
||
Input |
List of suppliers to Import |
||
Output |
None |
||
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
suppliers |
List of details |
Yes |
A collection of up to 500 suppliers to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
name |
String (240) |
- |
The supplier's name. |
status |
Integer |
Yes |
The supplier’s status: See Supplier Status |
dunsNumber |
String (9) |
- |
The nine-digit number assigned and maintained by Dun and Bradstreet. |
taxId |
String (18) |
- |
The tax identification number of the supplier. |
parentId |
Long (128) |
- |
The parent supplier's identifier. |
countryCode |
String (3) |
- |
The 2-3 character ISO code of the country. |
languageCode |
String (3) |
- |
The 2-3 character ISO code of the language. |
currencyCode |
String (3) |
- |
The 2-3 character ISO code of the currency. |
returnAllowed |
Boolean |
- |
True is return is allowed to the supplier, N otherwise. |
authorizationRequired |
Boolean |
- |
True indicates an authorization number is required when merchandise is return to this supplier. |
orderCreateAllowed |
Boolean |
- |
True indicates at a purchase order can be created for the supplier when processing deliveries from that supplier. |
vendorCheck |
Boolean |
- |
True indicates that orders from this supplier requires vendor control. |
vendorCheckPercent |
BigDecimal |
- |
Indicates the percentage of items per receipt that will be marked for vendor checking. |
quantityLevel |
Integer |
Yes |
The Quantity Level: See Quantity Level |
deliveryDiscrepancy |
Integer |
Yes |
The delivery discrepancy: See Supplier Delivery Discrepancy Type |
organizationUnitIds |
List<Long> |
Yes |
A complete list of organization unit identifiers for the supplier. |
Example Input
{
"suppliers": [
{
"supplierId": 5000,
"status": 1,
"quantityLevel": 1,
"deliveryDiscrepancy": 1,
"organizationUnitIds": [ 1 ]
},
{
"supplierId": 5001,
"status": 1,
"returnAllowed": false,
"quantityLevel": 1,
"deliveryDiscrepancy": 1,
"organizationUnitIds": [ 1 ]
}
]
}
Additional Data Definitions
Supplier Delivery Discrepancy Type
Value |
Definition |
1 |
Allow Any Discrepancy |
2 |
Allow Overage But Not Short Receipts |
3 |
Discrepancy Not Allowed |
Supplier Status
Value |
Definition |
1 |
Active |
2 |
Inactive |
Quantity Level
Value |
Definition |
1 |
Eaches |
2 |
Cases |
API: Delete Supplier
Deletes a supplier.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/{supplierId}/delete |
||
Method |
POST |
||
Successful Response |
202 Accepted |
||
Processing Type |
Asynchronous |
||
Input |
None |
||
Output |
None |
||
Max Response Limit |
1000 |
Path Parameter Definitions
Attribute |
Definition |
supplierId |
The internal identifier of the supplier. |
API: Import Items
Imports supplier items. Later during asynchronous processing, it validates that both the supplier and item exist before inserting new records.
If the import contains more than 500 supplier items, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/items |
|||||
Method |
POST |
|||||
Successful Response |
202 Accepted |
|||||
Processing Type |
Asynchronous |
|||||
Input |
List of Supplier Items to import |
|||||
Output |
None |
|||||
Max Response Limit |
500 |
Path Parameter Definitions
Attribute |
Definition |
|
supplierId |
The internal identifier of the supplier. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
|||
items |
List of details |
Yes |
The supplier item information to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
vendorProductNumber |
String (256) |
- |
Vendor product number. |
primary |
Boolean |
- |
True indicates this supplier is the primary supplier for the item at all locations. |
Example Input
{
"items": [
{
"supplierId": 5000,
"itemId": "163715121",
"vendorProductNumber": "abc"
},
{
"supplierId": 5001,
"itemId": "163715121",
"vendorProductNumber": "def"
}
]
}
API: Delete Items
Deletes supplier items.
If the delete contains more than 500 supplier items, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/items/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List of details |
Yes |
The supplier item information to delete. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (15) |
Yes |
The item identifier. |
Example Input
{
"items":
[
{
"supplierId":"9002",
"itemId": "100637130"
},
{
"supplierId":"9002",
"itemId": "100637148"
}
]
}
API: Import Item UOMs
Imports supplier item unit of measure information.
If the import contains more than 500 supplier item units of measure, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/uoms |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items UOMs to import |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
uoms |
List of details |
Yes |
The UOMs to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
unitOfMeasure |
String (4) |
Yes |
The unit of measure. |
Value |
BigDecimal |
Yes |
Equivalent item/supplier shipping carton value in unit of measure |
Example Input
{
"uoms": [
{
"supplierId": 5000,
"itemId": "163715121",
"unitOfMeasure": "KG",
"value": "1.0"
},
{
"supplierId": 5001,
"itemId": "163715121",
"unitOfMeasure": "KG",
"value": "1.0"
}
]
}
API: Delete Item UOMs
Deletes supplier item unit of measure information.
If the delete contains more than 500 supplier item units of measure, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/uoms/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items UOMs to delete |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
uoms |
List of details |
Yes |
The UOMs to delete. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
unitOfMeasure |
String (4) |
Yes |
The unit of measure. |
Example Input
{
"uoms":
[
{
"supplierId":"9002",
"itemId": "100637130",
"unitOfMeasure":"EA"
},
{
"supplierId":"9002",
"itemId": "100637148",
"unitOfMeasure":"EA"
}
]
API: Import Item Countries
Imports supplier item country information. If the imported supplier item country is for the primary supplier of the item, the item's case size will be updated on the item master to reflect the imported case size.
If the import contains more than 500 supplier item countries, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/countries |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items country to import |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
countries |
List of details |
Yes |
The countries to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item. |
caseSize |
BigDecimal |
Yes |
Number of items with a case from the supplier. |
unitCostCurrency |
String (3) |
- |
The unit cost currency for that item and supplier in that country. |
unitCostValue |
BigDecimal |
- |
The unit cost of the item and supplier in that country. |
Example Input
{
"countries": [
{
"supplierId": 5100,
"itemId": "163715121",
"countryCode": "US",
"caseSize":7
},
{
"supplierId": 5200,
"itemId": "163715121",
"countryCode": "US",
"caseSize":8
}
]
}
API: Delete Item Countries
Deletes supplier item country information.
If the delete contains more than 500 supplier item countries, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/countries/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items country to remove |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
countries |
List of details |
Yes |
The countries to remove. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item. |
Example Input
{
"countries":
[
{
"supplierId":"9002",
"itemId": "100637130",
"countryCode":"US"
}
]
}
API: Import Item Dimensions
Imports supplier item country dimension information.
If the import contains more than 500 supplier item dimensions, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/dimensions |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items dimensions to import |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
dimensions |
List of details |
Yes |
The dimensions to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item. |
dimensionName |
String (6) |
Yes |
Dimension name |
presentationMethod |
String (6) |
- |
Describes the packaging method |
Length |
BigDecimal |
- |
The length in dimension unit of measure. |
Width |
BigDecimal |
- |
The width in dimension unit of measure. |
Height |
BigDecimal |
- |
The height in dimension unit of measure. |
dimensionUom |
String (4) |
- |
The unit of measure of the dimensions. |
Weight |
BigDecimal |
- |
The weight of the object in weight unit of measure. |
netWeight |
BigDecimal |
- |
The net weight of the goods without packaging in weight unit of measure. |
weightUom |
String (4) |
- |
The weight unit of measure. |
liquidVolume |
BigDecimal |
- |
The liquid value or capacity of the object. |
liquidVolumeUom |
String (4) |
- |
The liquid volume unit of measure. |
statisticalCube |
BigDecimal |
- |
A statistical value of the object’s dimensions used for shipment loading purposes. |
Example Input
{
"dimensions": [
{
"supplierId": 5100,
"itemId": "163715121",
"countryCode": "US",
"dimensionName": "Hello"
},
{
"supplierId": 7100,
"itemId": "163715121",
"countryCode": "US",
"dimensionName": "Bye"
}
]
}
API: Delete Item Dimensions
Deletes supplier item country dimension information.
If the delete contains more than 500 supplier items, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/dimensions/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items dimensions to remove |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
Dimensions |
List of details |
Yes |
The dimensions to remove. |
Supplier Items Dimensions Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item |
dimensionName |
String (6) |
Yes |
Dimension name |
Example Input
{
"dimensions":
[
{
"supplierId":"9002",
"itemId": "100637130",
"countryCode":"US",
"dimensionName":"Dimen1"
},
{
"supplierId":"9002",
"itemId": "100637148",
"countryCode":"US",
"dimensionName":"Dimen2"
}
]
}
API: Import Item Manufacturers
Import supplier item country manufacturer information.
If the import contains more than 500 supplier item manufacturers, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/manufacturers |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items manufacturer to import |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
manufacturers |
List of details |
Yes |
The manufacturers to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item. |
primary |
Boolean |
- |
True indicates it is primary country of manufacture. |
Example Input
{
"manufacturers": [
{
"supplierId": 5100,
"itemId": "163715121",
"countryCode": "US",
"primary": true
},
{
"supplierId": 7100,
"itemId": "163715121",
"countryCode": "US",
"primary": true
}
]
}
API: Delete Item Manufacturers
Deletes supplier item country manufacturer information.
If the delete contains more than 500 supplier item manufacturers, an input too large error will be returned.
A "Forbidden" response will occur if application is integrated with MFCS or RMS.
API Basics
Endpoint URL |
{base URL}/manufacturers/delete |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous |
|
Input |
List of supplier items manufacturers to remove |
|
Output |
None |
|
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
manufacturers |
List of details |
Yes |
The manufacturers to remove. |
Detail Data Definition
Attribute |
Data Type |
Required |
Description |
supplierId |
Long (10) |
Yes |
The supplier identifier. |
itemId |
String (25) |
Yes |
The item identifier. |
countryCode |
String (3) |
Yes |
The ISO country code of the supplier that produces the item. |
Example Input
{
"manufacturers":
[
{
"supplierId":"9002",
"itemId": "100637130",
"countryCode":"US"
},
{
"supplierId":"9002",
"itemId": "100637148",
"countryCode":"US"
}
]
}
REST Service: Ticket
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/tickets
API Definitions
API |
Description |
readTicket |
This API reads a current actively ticket by its identifier. |
findTickets |
API is used to find summarized ticket headers for a set of criteria. |
readArchivedTicket |
This API reads a previously printed and archived ticket by its identifier. |
findArchivedTickets |
API is used to find archived ticket summaries. |
createTickets |
Creates new tickets within the system. |
updateTickets |
Updates current tickets within the system. |
printTickets |
Prints the requested tickets. |
findTicketFormats |
Reads all the available ticket formats. |
findTicketPrinters |
Finds the printers available to print tickets. |
API: readTicket
This API reads a current actively ticket by its identifier.
API Basics
Endpoint URL |
{base URL}/{ticketId} |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
The ticket identifier |
Output |
The ticket |
Output Data Definition
Column |
Type |
Definition |
ticketId |
Long(12) |
The unique ticket identifier. |
itemId |
String(25) |
The item identifier. |
storeId |
Long(10) |
The store identifier. |
originType |
Integer(2) |
The origin type (See Index) |
ticketFormatId |
Long(12) |
The identifier of the ticket format used for printing. |
ticketFormatDescription |
String(100) |
The description of the ticket format associated to the ticket. |
ticketFormatType |
Integer(4) |
The type of the ticket format associated to the ticket. |
ticketFormatReference |
String(255) |
The format reference used to print the ticket. |
ticketFormatZplId |
Long(12) |
The ZPL format file identifier. |
ticketCount |
Integer(3) |
The number of instances of this ticket to print. |
ticketSequence |
Integer(3) |
The sequence number of a ticket within a ticket grouping. |
printQuantity |
BigDecimal(12,4) |
The quantity to be printed on the ticket. |
printDate |
Date |
The date the ticket should be printed. |
groupId |
Long(12) |
An internal EICS grouping identifier that groups the tickets. |
groupIdExternal |
String(128) |
An external system grouping identifier that groups the tickets. |
autoPrint |
Boolean |
True if the ticket should automatically print, false if the ticket requires manual printing. |
autoRefeshQuantity |
Boolean |
True indicates the ticket count gets refreshed with SOH at the time of printing, false it prints as is. |
countryOfManufacture |
String(3) |
A two letter country code denoting the country of manufacture for the item. |
shortDescription |
String(255) |
The short description of the item. |
longDescription |
String(400) |
The long description of the item. |
shortDescriptionLanguage |
String(255) |
The short description of the item in the language of the store. |
longDescriptionLanguage |
String(400) |
The long description of the item in the language of the store. |
differentiatorType1 |
String(10) |
The description of the differentiator type for differentiator 1. |
differentiatorType2 |
String(10) |
The description of the differentiator type for differentiator 2. |
differentiatorType3 |
String(10) |
The description of the differentiator type for differentiator 3. |
differentiatorType4 |
String(10) |
The description of the differentiator type for differentiator 4. |
differentiatorDescription1 |
String(255) |
The description of differentiator 1 of the item. |
differentiatorDescription2 |
String(255) |
The description of differentiator 2 of the item. |
differentiatorDescription3 |
String(255) |
The description of differentiator 3 of the item. |
differentiatorDescription4 |
String(255) |
The description of differentiator 4 of the item. |
departmentId |
Long(12) |
The department identifier of the item. |
departmentName |
String(360) |
The department name of the item. |
classId |
Long(12) |
The class identifier of the item. |
className |
String(360) |
The class name of the item. |
subclassId |
Long(12) |
The subclass identifier of the item. |
subclassName |
String(360) |
The subclass name of the item. |
primaryUpc |
String(25) |
The primary Unique Product Code for the item. |
priceCurrency |
String(3) |
The currency code of the ticket price. |
priceAmount |
BigDecimal(12,4) |
The amount of the ticket price. |
priceType |
Integer(3) |
The type of the ticket price. (See Index) |
priceUom |
String(4) |
The unit of measure of the ticket price. |
priceActiveDate |
Date |
The date the ticket price became active. |
priceExpireDate |
Date |
The date the ticket price expires. |
mutliUnitPriceCurrency |
String(3) |
The currency code of the ticket's multi-unit price. |
multiUnitPriceAmount |
BigDecimal(12,4) |
The amount of the ticket's multi-unit price. |
multiUnitPriceUom |
String(4) |
The unit of measure of the ticket's multi-unit price. |
multiUnitQuantity |
BigDecimal(12,4) |
The multi-unit quantity associated to the price. |
overridePriceCurrency |
String(3) |
The override price currency code. |
overridePriceAmount |
BigDecimal(20,4) |
The override price amount. |
previousPriceCurrency |
String(3) |
The currency code of the previous price. |
previousPriceAmount |
BigDecimal(12,4) |
The amount of the previous price. |
previousPriceType |
Integer(3) |
The price type of the previous price. |
lowestMonthlyPriceCurrency |
String(3) |
The currency code of the lowest monthly price. |
lowestMonthlyPriceAmount |
BigDecimal(12,4) |
The amount of the lowest monthly price. |
lowestMonthlyPriceType |
Integer(3) |
The price type of the lowest monthly price. |
printedDate |
Date |
The date that the ticket was printed. |
printedUser |
String(128) |
The user that printed the ticket. |
createDate |
Date |
The date the ticket was created. |
createUser |
String(128) |
The user that created the ticket. |
updateDate |
Date |
The date the ticket was last updated. |
updateUser |
String(128) |
The user that last updated the ticket. |
udas |
Collection |
A group of associated user defined attributes. |
UDA
Column |
Type |
Definition |
name |
String |
The name of the user defined attribute. |
value |
String |
The value of the user defined attributes. |
API: findTickets
API is used to find summarized ticket headers for a set of criteria.
If maximum results are exceeded, additional or more limiting input criteria will be required.
API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of Ticket Headers |
Maximum Results Allowed |
5,000 |
Input Data Definition
Attribute |
Type |
Definition |
itemId |
String(25) |
Include only records with this item identifier. |
storeId |
Long(10) |
Include only records with this store identifier. |
ticketFormatId |
Long(12) |
Include only records with this ticket format identifier. |
groupId |
Long(12) |
Include only records with this EICS group identifier. |
groupIdExternal |
String(128) |
Include only records with this external system group identifier. |
printDateFrom |
String |
Include only records on or after this scheduled print date and time. |
printDateTo |
String |
Include only records on or before this scheduled print date and time. |
updateDateFrom |
String |
Include only records on or after this scheduled print date and time. |
updateDateTo |
String |
Include only records on or before this scheduled print date and time. |
Output Data Definition
Column |
Type |
Definition |
ticketId |
Long(12) |
The unique ticket identifier. |
itemId |
String(25) |
The item identifier. |
storeId |
Long(10) |
The store identifier. |
originType |
Integer(2) |
The origin type (See Index) |
ticketFormatId |
Long(12) |
The identifier of the ticket format used for printing. |
ticketSequence |
Integer(3) |
The sequence number of a ticket within a ticket grouping. |
groupId |
Long(12) |
An internal EICS grouping identifier that groups the tickets. |
groupIdExternal |
String(128) |
An external system grouping identifier that groups the tickets. |
autoPrint |
Boolean |
True if the ticket should automatically print, false if the ticket requires manual printing. |
printDate |
Date |
The date the ticket should be printed. |
printQuantity |
BigDecimal(12,4) |
The quantity to be printed on the ticket. |
updateDate |
Date |
The date the ticket was last updated. |
API: readArchivedTicket
This API reads a previously printed and archived ticket by its identifier.
API Basics
Endpoint URL |
{base URL}/archives/{ticketId} |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
The ticket identifier |
Output |
The ticket details |
Output Data Definition
See readTicket() for the output data definition of this API.
API: findArchivedTickets
API is used to find archived ticket summaries.
If the number of tickets found exceeds the limit, additional or more limiting input criteria will be required.
API Basics
Endpoint URL |
{base URL}/archives |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of Ticket Headers |
Maximum Results Allowed |
5,000 |
Input Data Definition
Attribute |
Type |
Definition |
itemId |
String(25) |
Include only records with this item identifier. |
storeId |
Long(10) |
Include only records with this store identifier. |
ticketFormatId |
Long(12) |
Include only records with this ticket format identifier. |
groupId |
Long(12) |
Include only records with this EICS group identifier. |
groupIdExternal |
String(128) |
Include only records with this external system group identifier. |
printedDateFrom |
String |
Include only records that were printed on or after this date and time. |
printedDateTo |
String |
Include only records that were printed on or before this date and time. |
Output Data Definition
Column |
Type |
Definition |
ticketId |
Long(12) |
The unique ticket identifier. |
itemId |
String(25) |
The item identifier. |
storeId |
Long(10) |
The store identifier. |
originType |
Integer(2) |
The origin type (See Index) |
ticketFormatId |
Long(12) |
The identifier of the ticket format used for printing. |
ticketSequence |
Integer(3) |
The sequence number of a ticket within a ticket grouping. |
groupId |
Long(12) |
An internal EICS grouping identifier that groups the tickets. |
groupIdExternal |
String(128) |
An external system grouping identifier that groups the tickets. |
printedDate |
Date |
The date the ticket was printed. |
printQuantity |
BigDecimal(12,4) |
The quantity printed on the ticket. |
priceCurrency |
String(3) |
The currency of the price of the ticket. |
priceAmount |
BigDecimal(12,4) |
The amount of the price of the ticket. |
API: createTickets
Creates new tickets within the system.
API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Ticket group |
Output |
List of ticket identifying information |
Maximum Input Allowed |
2,000 tickets within the group |
Input Data Definition
Payload |
Type |
Req |
Definition |
storeId |
Long(10) |
X |
The store identifier. |
groupIdExternal |
String(128) |
An external system grouping identifier that groups the tickets. |
|
printDate |
Date |
X |
The date the ticket should be printed. |
autoPrint |
Boolean |
True if the ticket should automatically print, false if the ticket requires manual printing. Defaults to false. |
|
autoRefeshQuantity |
Boolean |
True indicates the ticket count gets refreshed with SOH at the time of printing, false it prints as is. Defaults to false. |
|
tickets |
Collection |
X |
The tickets to create. |
Ticket
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The item identifier. |
originType |
Integer(2) |
X |
The origin type (See Index) |
ticketFormatId |
Long(12) |
X |
The identifier of the ticket format used for printing. |
ticketCount |
Integer(3) |
X |
The number of instances of this ticket to print. |
ticketSequence |
Integer(3) |
X |
The sequence number of a ticket within a ticket grouping. |
printQuantity |
BigDecimal(12,4) |
X |
The quantity to be printed on the ticket. |
overridePriceCurrency |
String(3) |
The override price currency code. Required if an amount is entered. |
|
overridePriceAmount |
BigDecimal(20,4) |
The override price amount. |
|
countryOfManufacture |
String(3) |
A two letter country code denoting the country of manufacture for the item. |
Output Data Definition
Payload |
Type |
Definition |
ticketId |
Long(12) |
The unique ticket identifier. |
storeId |
Long(10) |
The store identifier. |
ticketFormatId |
Long(12) |
The identifier of the ticket format used for printing. |
Example Input
{
"storeId": 5000,
"groupIdExternal": "123456",
"printDate": "2023-01-10T23:59:59-05:00",
"autoPrint": false,
"autoRefreshQuantity": false,
"tickets": [
{
"itemId": "100637121",
"originType": 1,
"ticketFormatId": 1,
"ticketCount": 2,
"ticketSequence": 3
},
{
"itemId": "100637113",
"originType": 2,
"ticketFormatId": 2,
"ticketCount": 4,
"ticketSequence": 5
}
]
}
API: Update Tickets
Updates current tickets within the system.
If a field that is not required contains an empty or null value, the ticket will be updated to that empty or null value.
API Basics
Endpoint URL |
{base URL}/update |
Method |
POST |
Success Response |
204 No Content |
Processing Type |
Synchronous |
Processing Type |
Synchronous |
Input |
Tickets |
Output |
N/A |
Maximum Input Allowed |
2,000 tickets |
Input Data Definition
Payload |
Type |
Req |
Definition |
ticketId |
Long(12) |
X |
The unique ticket identifier. |
originType |
Integer(2) |
X |
The origin type (See Index) |
ticketCount |
Integer(3) |
X |
The number of instances of this ticket to print. |
ticketSequence |
Integer(3) |
X |
The sequence number of a ticket within a ticket grouping. |
printQuantity |
BigDecimal(12,4) |
X |
The quantity to be printed on the ticket. |
printDate |
Date |
X |
The date the ticket should be printed. |
autoPrint |
Boolean |
True if the ticket should automatically print, false if the ticket requires manual printing. |
|
autoRefeshQuantity |
Boolean |
True indicates the ticket count gets refreshed with SOH at the time of printing, false it prints as is. |
|
overridePriceCurrency |
String(3) |
The override price currency code. Required if an amount is entered. |
|
overridePriceAmount |
BigDecimal(20,4) |
The override price amount. |
|
countryOfManufacture |
String(3) |
A two letter country code denoting the country of manufacture for the item. |
Example Input
{
"tickets": [
{
"ticketId": 8,
"originType": 2,
"printDate": "2023-01-10T23:59:59-05:00",
"ticketCount": 22,
"ticketSequence": 33
},
{
"ticketId": 9,
"originType": 1,
"printDate": "2023-01-10T23:59:59-05:00",
"ticketCount": 44,
"ticketSequence": 55
}
]
}
API: printTickets
Prints the requested tickets. This can print both current tickets and previously printed tickets.
It is assumed that the calling system will have verified or retrieved the ticket ids prior. The ticket ids and archived ticket ids will not be validated.
This service operation will simply print any tickets that are found that match identifiers passed in and ignore any identifiers for which tickets are not found.
Depending on printing configuration with the system, the printing may occur synchronous and real-time or the tickets may be staged and sent asynchronously to another system after a short delay.
API Basics
Endpoint URL |
{base URL}/print |
Method |
POST |
Success Response |
204 No Content |
Processing Type |
Synchronous/Asynchronous |
Input |
Ticket identifiers |
Output |
N/A |
Maximum Input Allowed |
100 total ticket ids |
Input Data Definition
Payload |
Type |
Req |
Definition |
printerId |
Long |
X |
The identifier of the printer to print the tickets on. |
refreshQuantity |
Boolean |
If true, the quantities of all the tickets will be refreshed prior to printing. If false, they will not. |
|
ticketIds |
List<Long> |
A list of ticket identifiers of the tickets to print. If this is null or empty, then archived ticket ids must contain a value. Not validated. |
|
archivedTicketIds |
List<Long> |
A list of archived ticket identifiers of tickets to print. If this is null or empty, then ticket ids must contain a value. Not validated. |
API: findTicketFormats
Reads all the available ticket formats.
API Basics
Endpoint URL |
{base URL}/formats |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
List of ticket formats |
Input Data Definition
Column |
Type |
Definition |
storeId |
Long(10) |
Include only ticket formats available at this store. |
Output Data Definition
Column |
Type |
Definition |
formatId |
Long(12) |
The unique format identifier. |
storeId |
Long(10) |
The store identifier. |
description |
String(100) |
A description of the ticket (possibly from ticket format). |
type |
Integer(4) |
The type of ticket format. (See Index) |
zplTemplateId |
Long(12) |
The unique identifier to the ZPL template to be used for printing. |
formatReference |
String(255) |
A reference to the format content to use for this format. |
defaultFormat |
Boolean |
True if this is the default form for the type, False otherwise. |
defaultPrinterId |
Long(6) |
Teh default printer identifier for the format. |
createDate |
Date |
The date the format was created. |
createUser |
String(128) |
The user that created the format. |
updateDate |
Date |
The date the format was last updated. |
updateUser |
String(128) |
The user that last updated the format. |
API: findTicketPrinters
Finds the printers available to print tickets.
API Basics
Endpoint URL |
{base URL}/printers |
Method |
GET |
Success Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query parameters |
Output |
List of printers |
Query Params
Column |
Type |
Definition |
storeId |
Long(10) |
Include only ticket printers available at this store. |
Output Data Definition
Column |
Type |
Definition |
printerId |
Long(6) |
The unique identifier of the printer. |
storeId |
Long(10) |
The identifier of the store the printer is assigned to. |
printerType |
Integer(3) |
The print type of the printer (see Index). |
printerDescription |
String(200) |
A description of the printer. |
printerUri |
String(300) |
The URI address of the printer. |
printerName |
String(128) |
The logical name of the printer. |
defaultManifest |
Boolean |
True if the printer is the default printer at the store for manifest printing. |
defaultPreshipment |
Boolean |
True if the printer is the default printer at the store for preshipment printing. |
Additional Data Definitions
Ticket Format Type
ID |
Description |
1 |
Item Basket |
2 |
Shelf Label |
Price Type
ID |
Description |
1 |
Permanent |
2 |
Promotional |
3 |
Clearance |
4 |
Clearance Reset |
Printer Type
ID |
Description |
1 |
Item Ticket |
2 |
Shelf Label |
3 |
Postscript |
Ticket Origin Type
ID |
Description |
1 |
External |
2 |
Price Change |
3 |
Foundation |
4 |
Manual |
5 |
Promotional Price Change |
6 |
Clearance Price Change |
7 |
Permanent Price Change |
8 |
Reset Price Change |
REST Service: Transfer
This service allows the creation, modification, and cancellation of transfers from an external application. This does not include allocations.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/transfers
API Definitions
API |
Description |
readTransfer |
Reads the full detail of a transfer. |
findTransfers |
Finds transfers headers based on a set of input criteria to search for. |
findTransferContexts |
Retrieves all available transfer contexts for creating a new transfer. |
requestTransfer |
Moves the status of a new transfer request to requested, notifying the sending location of the transfer. |
cancelRequest |
Moves the status of a new transfer request to canceled if the request is still new or a work in progress. |
rejectRequest |
Rejects the requested transfer as long as it is still only requested or request in progress. |
approveRequest |
Moves the transfer to approved status and does inventory shifts if the transfer is "Requested" or "Request In Progress" and goes into "Approved" |
cancelTransfer |
Moves the status a transfer to canceled, but only if it is in the "Transfer In Progress" status. |
closeTransfer |
Closes out a transfer as long as the transfer is open and not yet in shipping status. |
API: Read Transfer
Read a Transfer.
Table 4-78 API Basics
Endpoint URL |
/{transferId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
Transfer |
Table 4-79 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
Table 4-80 Output Data Definition
Attribute |
Type |
Definition |
transferId |
Long(15) |
The unique identifier of the transfer. |
externalTransferId |
String(128) |
An external identifier supplied from an external system. |
distroNumber |
String(128) |
A distribution number (used for integration with other systems). It is another type of external identifier. |
sourceLocationType |
Integer |
The location type of the source of the transfer. See Additional Data Definitions. |
sourceLocationId |
Long(10) |
The identifier of the source location of the transfer. |
destinationLocationType |
Integer |
The location type of the destination of the transfer. See Additional Data Definitions. |
destinationLocationId |
Long(10) |
The identifier of the destination location of the transfer. |
status |
Integer |
The current status of the transfer. See Additional Data Definitions |
originType |
Integer |
The origin type of the transfer. See Additional Data Definitions |
contextTypeId |
Long(18) |
Unique identifier of a context associated to the transfer. |
contextTypeCode |
Long(4) |
An external code of the context. |
contextValue |
String(25) |
A value or some information related to the context associated to the transfer. |
customerOrderNumber |
String(128) |
External system identifier of the customer order. |
fulfillmentOrderNumber |
String(128) |
External system identifier of the fulfillment order. |
allowPartialDelivery |
Boolean |
True indicates that the partial delivery is allowed for the transfer, false indicates it is not. |
useAvailable |
Boolean |
True indicates the transfer must use available stock, false indicates it uses unavailable stock. |
authorizationCode |
String(12) |
An authorization code assigned to the transfer. |
notAfterDate |
Date |
Date after which the transfer is no longer valid. |
createDate |
Date |
The date this record was created. |
createUser |
String(128) |
The user that created this record. |
updateDate |
Date |
The date this record was last updated. |
updateUser |
String(128) |
The user that last updated this record. |
requestDate |
Date |
The date the transfer was requested. |
requestUser |
String(128) |
The user that requested the transfer. |
approvalDate |
Date |
The date the transfer was approved. |
approvalUser |
String(12) |
The user that approved the transfer. |
importId |
String(128) |
The identifier from an original data seed import. |
lineItems |
Collection |
A collection of transfer line items. |
Table 4-81 Transfer Line Item Data Definition
Column |
Type |
Definition |
lineId |
Long(15) |
The unique internal identifier of the transfer line item. |
itemId |
String(25) |
The item identifier. |
caseSize |
BigDecimal(10,2) |
The case size associated to this line item. |
quantityRequested |
BigDecimal(20,4) |
The quantity that was requested. |
quantityApproved |
BigDecimal(20,4) |
The quantity that was approved. |
quantityShipping |
BigDecimal(20,4) |
The quantity that is currently in shipping. |
quantityShipped |
BigDecimal(20,4) |
The quantity that has currently shipped. |
quantityReceived |
BigDecimal(20,4) |
The quantity that has been received into stock. |
quantityDamaged |
BigDecimal(20,4) |
The quantity that has been received as damaged. |
preferredUom |
String(4) |
The preferred unit of measure of the transfer line item. |
API: Find Transfer
This API is used to find transaction headers for transfers. If the number of transfer found exceeds 10,000, a maximum limit error will be returned. Additional or more limiting input criteria will be required.
Table 4-82 API Basics
Endpoint URL |
|
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of Transfer Headers |
Table 4-83 Query Parameter Definitions
Attribute |
Type |
Definition |
externalTransferId |
String(128) |
Include only records with this external transfer identifier. |
sourceLocationId |
Long(10) |
Include only records with this source location identifier. |
sourceLocationType |
Integer |
Include only records with this source location type. See Additional Data Definitions |
destinationLocationId |
Long(10) |
Include only records with this destination location identifier. |
destinationLocationType |
Integer |
Include only records with this destination location type. See Additional Data Definitions. |
status |
Integer |
Include only records with this status. See Additional Data Definitions. |
itemId |
String(25) |
Include only records with this item on them. |
updateDateFrom |
Date |
Include records with a last update date on or after this date. |
updateDateTo |
Date |
Include records with a first update date on or before this date. |
Attribute |
Type |
Definition |
transferId |
Long(15) |
The unique identifier of the transfer. |
externalTransferId |
String(128) |
An external identifier supplied from an external system. |
sourceLocationType |
Integer |
The type of the source location of the transfer. See Additional Data Definitions. |
sourceLocationId |
Long(10) |
The identifier of the source location of the transfer. |
destinationLocationType |
Integer |
The type of the destination location of the transfer. See Additional Data Definitions. |
destinationLocationId |
Long(10 |
The identifier of the destination location of the transfer. |
status |
Integer |
The current status of the transfer. See Additional Data Definitions. |
originType |
Integer |
The origin type of the transfer. See Additional Data Definitions. |
customerOrderNumber |
String(128) |
The external customer order number. |
fulfillmentOrderNumber |
String(128) |
The external fulfillment order number. |
notAfterDate |
Date |
Date after which the transfer is no longer valid. |
createDate |
Date |
The date this record was created. |
updateDate |
Date |
The date this record was last updated. |
requestDate |
Date |
The date the transfer was requested. |
approvalDate |
Date |
The date the transfer was approved. |
API: Request Transfer
This API finalizes the request of the transfer and begins the approval process.
Table 4-84 API Basics
Endpoint URL |
/{transferId}/requests |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-85 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Release Request
This API finalizes the request of the transfer and begins the approval process.
Table 4-86 API Basics
Endpoint URL |
/{transferId}/release |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-87 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Approve Request
Approves a transfer request for shipment moving it to the shipping stage.
Table 4-88 API Basics
Endpoint URL |
/{transferId}/requests/approve |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-89 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Reject Request
Rejects the transfer request closing out the transfer. This is done when the approver of a request decides not to fulfill it.
Table 4-90 API Basics
Endpoint URL |
/{transferId}/reject |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-91 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Cancel Request
Cancels a transfer request closing out the transfer. This is done when the requester decides the transfer is not needed prior to finishing their request.
Table 4-92 API Basics
Endpoint URL |
/{transferId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-93 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Approve Transfer
Approves a transfer for shipment, moving it to the shipping stage.
Table 4-94 API Basics
Endpoint URL |
/{transferId}/approve |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-95 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Cancel Transfer
Cancels a transfer that has been approved.
Table 4-96 API Basics
Endpoint URL |
/{transferId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-97 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Close Transfer
Closes a transfer that has been approved or approved and partially shipped.
Table 4-98 API Basics
Endpoint URL |
/{transferId}/close |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-99 Path Parameter Definitions
Parameter |
Definition |
transferId |
The internal identifier of the transfer header. |
API: Find Transfer Contexts
Reads all the available contexts for a transfer.
Table 4-100 API Basics
Endpoint URL |
/contexts |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
List of Transfer Contexts |
Table 4-101 Output Data Definition
Attribute |
Type |
Definition |
contextId |
Long(18) |
The unique identifier of the context. |
code |
String(6) |
A short code associated to the context |
description |
String(120) |
A description of the context. |
sequence |
Long |
A sorting sequence for the context. |
Additional Data Definitions
Table 4-102 Transfer Status
Value |
Definition |
1 |
New Request |
2 |
Requested |
3 |
Request In Progress |
4 |
Rejected Request |
5 |
Canceled Request |
6 |
Transfer In Progress |
7 |
Approved |
8 |
In Shipping |
9 |
Completed Transfer |
10 |
Canceled Transfer |
Table 4-103 Transfer Location Type
Value |
Definition |
1 |
Store |
2 |
Warehouse |
3 |
Finisher |
Table 4-104 Transfer Origin Type
Value |
Definition |
1 |
External |
2 |
Internal |
3 |
Adhoc |
Table 4-105 Transfer Status For Query Parameters
Value |
Definition |
1 |
New Request |
2 |
Requested |
3 |
Request In Progress |
4 |
Rejected Request |
5 |
Canceled Request |
6 |
Transfer In Progress |
7 |
Approved |
8 |
In Shipping |
9 |
Completed Transfer |
10 |
Canceled Transfer |
99 |
Active |
REST Service: Transfer Shipment
This service allows the creation, modification, and cancellation of transfer shipments from an external application.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/tsfshipments
API Definitions
API |
Definition |
readShipment |
Reads the transfer shipment. This does not include containers or items. |
findShipments |
Finds transfer shipment header information based on a set of criteria. |
submitShipment |
Submits the transfer shipment. |
cancelSubmitShipment |
Cancels the submission of a transfer shipment. |
dispatchShipment |
Dispatches a transfer shipment. |
cancelShipment |
Cancels a transfer shipment. |
confirmCarton |
Confirms a container for shipment. |
cancelCarton |
Cancels a container, removing it from the shipment. |
openCarton |
Opens a confirmed container so that it can be modified again. |
createShipment |
Creates a shipment. |
updateShipment |
Updates shipment header information. |
createCarton |
Adds a carton to the shipment. |
updateCarton |
Updates a carton on the shipment. |
API: Read Shipment
Read a transfer shipment.
Table 4-106 API Basics
Endpoint URL |
/{shipmentId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
Transfer Shipment |
Table 4-107 Path Parameter Definitions
Parameter |
Definition |
shipmentId |
The internal identifier of the transfer shipment header. |
Table 4-108 Output Data Definition
Column |
Type |
Definition |
shipmentId |
Long(15) |
The unique internal identifier of the transfer shipment. |
storeId |
Long(10) |
The unique store identifier that is the source of the shipment. |
destinationType |
Integer(2) |
The location type of the destination. See Additional Data Definitions-Destination Type. |
destinationId |
Long(10) |
The unique identifier of the destination. |
asn |
String(128) |
The advance shipment notification number. |
status |
Integer(2) |
The current status of the shipment. See Additional Data Definitions: Status |
authorizationCode |
String(12) |
An authorization code associated to the shipment. |
billOfLadingId |
Long(12) |
The unique identifier of the bill of lading. |
adhocDocumentId |
Long(15) |
An adhoc transfer document identifier associated to the shipment. |
billOfLadingId |
Long(15) |
The bill of lading number. |
alternateAddress |
String(2000) |
An alternate destination address. |
carrierRole |
Integer(2) |
The type of carrier for the shipment. See Additional Data Definitions |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company. |
motive |
String(120) |
A motive for the shipment. |
taxId |
String(18) |
The tax identifier of the supplier it is being shipped to. |
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
weight |
BigDecimal(12,4) |
The weight of the shipment. |
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
requestedPickupDate |
Date |
The requested pickup date. |
fiscalDocumentRequestId |
Long(20) |
The identifier of the request for a fiscal document. |
fiscalDocumentReferenceId |
Long(20) |
The unique identifier of the fiscal document. |
fiscalDocumentNumber |
String(255) |
The fiscal document number. |
fiscalDocumentStatus |
Integer(4) |
The status of the fiscal document. |
fiscalDocumentRejectReason |
String(255) |
A reason the fiscal document was rejected. |
fiscalDocumentUrl |
String(255) |
A URL to the fiscal document. |
createUser |
String(128) |
The user that created the shipment record. |
createDate |
Date |
The date the shipment record was created. |
updateUser |
String(128) |
The user that last updated the shipment. |
updateDate |
Date |
The last date the shipment was updated. |
submitUser |
String(128) |
The user that submitted the shipment record. |
submitDate |
Date |
The date the shipment was submitted within EICS. |
dispatchUser |
String(128) |
The user that dispatched the shipment. |
dispatchDate |
Date |
The date the shipment was dispatched within EICS. |
cartons |
Collection |
A collection of cartons on the shipment. |
Table 4-109 Output Data Definition (Cartons)
Column |
Type |
Definition |
cartonId |
Long(10) |
The unique identifier of the record. |
externalCartonId |
String(128) |
A container identifier from an external system. |
status |
Integer(4) |
The current status of the container. |
dimensionId |
Long(10) |
The shipment container dimension identifier. |
weight |
BigDecimal(12,4) |
The weight of the container. |
weightUom |
String(4) |
The unit of measure of the weight of the container. |
trackingNumber |
String(128) |
A tracking number for the container. |
useAvailableInventory |
Boolean |
True indicates use only available inventory, False indicates use non-available inventory. |
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
customerOrderRelated |
Integer(4) |
The customer order related value (see Additional Data Definitions). |
createUser |
String(128) |
The user that created the container in EICS. |
createDate |
Date |
The date the container was created in EICS. |
updateUser |
String(128) |
The user that last updated the container in EICS. |
updateDate |
date |
The date the container was last updated in EICS. |
approvalUser |
String(128) |
The user that approved the container in EICS. |
approvalDate |
Date |
The date the container was approved in EICS. |
lineItems |
Collection |
The line items in the container. |
Table 4-110 Output Data Definition (Line Item)
Column |
Type |
Definition |
lineId |
Long(15) |
The unique identifier of the record. |
itemId |
String(25) |
The unique identifier of the item. |
caseSize |
BigDecimal(10,2) |
The case size of this line item. |
quantity |
BigDecimal(20,4) |
The quantity being shipped. |
transferId |
Long(12) |
The unique identifier of the associated transfer. |
externalTransferId |
Long(15) |
The external system identifier of the associated transfer. |
customerOrderNumber |
String(128) |
The customer order number if line item is for a customer order. |
fulfillmentOrderNumber |
String(128) |
The fulfillment order number if line item is for a customer order. |
preferredUom |
String(4) |
The preferred unit of measure for the shipment quantity. |
shipmentReasonId |
Long(15) |
A unique identifier of a shipment reason associated to this item. |
uins |
List<String> |
A list of UINs that are shipped. The number of UINs must match the quantity attribute. |
Example
Figure 4-15 Example
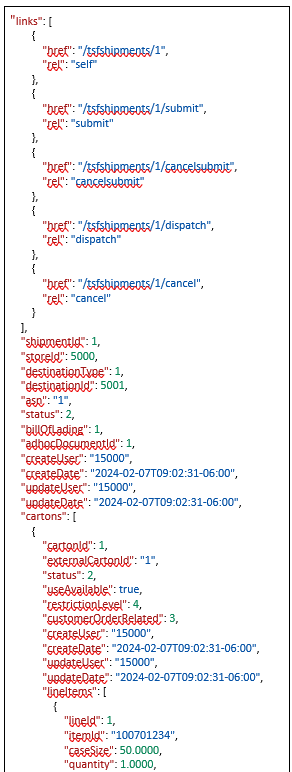
Figure 4-16 Example, continued
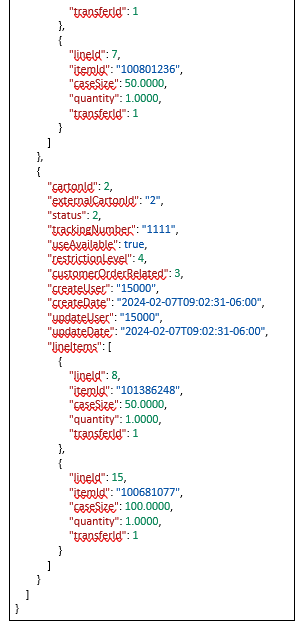
API: Find Transfer Shipment
Search for shipments based on a set of criteria.
If more than 10,000 shipments are found a "Results Too Large" response is returned and search criteria will need to be more restrictive.
Table 4-111 API Basics
Endpoint URL |
|
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of Transfer Shipments Headers |
Table 4-112 Query Parameter Definitions
Attribute |
Type |
Definition |
storeId |
Long(10) |
Include only transfer shipments from this store. |
destinationType |
Integer(2) |
Include only transfer shipments to this destination type. |
destinationId |
Long(10) |
Include only transfer shipments to this destination identifier. |
asn |
String(128) |
Include only transfer shipments with this ASN. |
status |
Integer(2) |
Include only transfer shipments in this status. |
updateDateFrom |
String |
Include only transfer shipments last updated on or after this date. |
updateDateTo |
String |
Include only transfer shipments last updated on or before this date. |
Table 4-113 Output Data Definition
Column |
Type |
Definition |
shipmentId |
Long(15) |
The unique internal identifier of the transfer shipment. |
storeId |
Long(10) |
The unique store identifier that is the source of the shipment. |
destinationType |
Integer(2) |
The location type of the destination. See Additional Data Definitions: Destination Type. |
destinationId |
Long(10) |
The unique identifier of the destination location. |
asn |
String(128) |
The advance shipment notification number. |
status |
Integer(4) |
The current status of the shipment. See Additional Data Definitions: Status |
createDate |
Date |
The date the shipment record was created. |
updateDate |
Date |
The last date the shipment was updated. |
submitDate |
Date |
The date the shipment was submitted within EICS. |
dispatchDate |
Date |
The date the shipment was dispatched within EICS. |
API: Submit Shipment
Submits the transfer shipment moving it to a noneditable state while it is waiting to be dispatched.
Table 4-114 API Basics
Endpoint URL |
/{shipmentId}/submit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-115 Path Parameter Definitions
Parameter |
Definition |
shipmentId |
The internal identifier of the transfer shipment header. |
API: Cancel Submit Shipment
Cancels the submissions of the transfer shipment return it to an in progress and editable state.
Table 4-116 API Basics
Endpoint URL |
/{shipmentId}/cancelsubmit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-117 Path Parameter Definitions
Parameter |
Definition |
shipmentId |
The internal identifier of the transfer shipment header. |
API: Dispatch Shipment
Dispatches the transfer shipment updating all the inventory positions and closing the shipment.
Table 4-118 API Basics
Endpoint URL |
/{shipmentId}/dispatch |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-119 Path Parameter Definitions
Parameter |
Definition |
shipmentId |
The internal identifier of the transfer shipment header. |
API: Cancel Shipment
Cancels the transfer shipment reversing any reserved inventory currently picked.
Table 4-120 API Basics
Endpoint URL |
/{shipmentId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-121 Path Parameter Definitions
Parameter |
Definition |
shipmentId |
The internal identifier of the transfer shipment header. |
API: Confirm Carton
Confirms a transfer shipment container, completing the container and making it non-editable and awaiting dispatch.
Table 4-122 API Basics
Endpoint URL |
cartons/{cartonId}/confirm |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-123 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the transfer shipment carton. |
API: Cancel Carton
Cancels a transfer shipment container.
Table 4-124 API Basics
Endpoint URL |
cartons/{cartonId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-125 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the transfer shipment carton. |
API: Open Carton
Opens a transfer shipment container.
Table 4-126 API Basics
Endpoint URL |
cartons/{cartonId}/open |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-127 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the transfer shipment carton. |
API: createShipment
This API is used to create a new transfer shipment whose status is "In Progress."
Note:
Container item/reason combination cannot be duplicated within the container.Table 4-128 API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Transfer Shipment |
Output |
Transfer Shipment Status |
Maximum Input Limit |
1,000 overall line items on shipment |
Table 4-129 Input Data Definition
Attribute |
Type |
Req |
Definition |
storeId |
Long(10 |
X |
The identifier of the store shipping the goods. |
destinationType |
Integer(2) |
X |
The location type of the destination (see Index). |
destinationId |
Long(10) |
X |
The location identifier of the destination |
asn |
String(15) |
The advance shipping number from an external system. |
|
authorizationCode |
String(12) |
A vendor authorization code. It may be required for some suppliers. |
|
displayCartons |
Boolean |
True if cartons should be displayed, otherwise false. |
|
addressType |
Integer |
The type of return address. See Index. |
|
carrierRole |
Integer(2) |
X |
The type of carrier for the shipment. See Index |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
|
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
|
alternateAddress |
String(2000) |
An alternate destination address. |
|
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
|
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company. |
|
motiveId |
Long(18) |
The unique identifier of a bill of lading motive. |
|
taxId |
taxId |
The tax identifier of the supplier it is being shipped to. |
|
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
|
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
|
weight |
BigDecimal(12,4) |
The weight of the shipment. |
|
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
|
requestedPickupDate |
Date |
The requested pickup date. |
|
cartons |
Collection |
X |
A group of cartons to create along with the shipment. |
notes |
Collections of Strings |
A collection of up to 100 notes. |
Table 4-130 Shipment Carton Data Definition
Payload |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A container identifier from an external system. |
|
trackingNumber |
String(128) |
The tracking number of the container. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
Long(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
useAvailableInventory |
Boolean |
True indicates available inventory will be used, false indicates unavailable inventory should be used. |
|
lineItems |
Collection |
X |
A collection of line items to create along with the carton. |
Table 4-131 Shipment Lien Item Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the SKU item. |
transferId |
Long(12) |
X |
The unique identifier of a transfer this item is being shipped for. |
reasonId |
Long(15) |
The unique identifier of the shipment reason associated to this line item. It is not allowed for available inventory and required for unavailable inventory. |
|
quantity |
BigDecimal(12,4) |
X |
The quantity to ship. |
caseSize |
BigDecimal(10,2) |
The case size of the item for this particular shipment. |
|
uins |
Collecton<String> |
A collection of UINs to ship. This number of UINs must match the quantity. |
Table 4-132 Output Data Definition
Attribute |
Type |
Definition |
shipmentId |
Long(15) |
The unique identifier of the shipment. |
storeId |
Long(10) |
The store identifier of the store shipping the goods. |
asn |
String(15) |
The Advancing Shipping Number. |
status |
Integer(2) |
The shipment status. See Index. |
Figure 4-17 Example Code
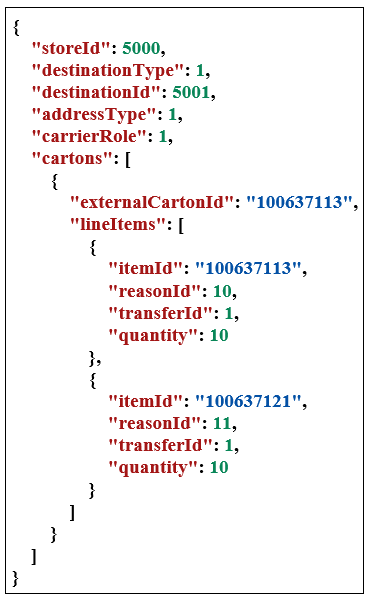
API: updateShipment
This API is used to update to the header portion of a shipment as well as its bill of lading information.
The shipment header cannot be updated while containers/cartons are currently confirmed for shipping.
Table 4-133 API Basics
Endpoint URL |
{base URL}/{shipmentId} |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the transfer shipment |
Input |
Transfer Shipment |
Table 4-134 Input Data Definition
Column |
Type |
Definition |
authorizationCode |
String(12) |
An authorization code associated to the shipment. |
displayCartons |
Boolean |
True if cartons should be displayed, otherwise false. |
addressType |
Integer(2) |
The type of return address. See Index. |
carrierRole |
Integer(2) |
The type of carrier for the shipment. See Index. |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
alternateAddress |
String(2000) |
An alternate destination address. |
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company |
motiveId |
Long(18) |
The unique identifier of a bill of lading motive. |
taxId |
String(18) |
The tax identifier of the supplier it is being shipped to. |
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
weight |
BigDecimal(12,4) |
The weight of the shipment. |
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
requestedPickupDate |
Date |
The requested pickup date. |
notes |
Collection(String) |
A collection of up to 100 notes to add to the notes associated to the shipment. |
Figure 4-18 Code Example
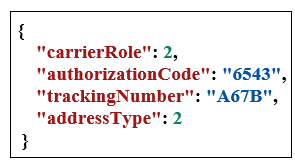
API: createCarton
This API is used to add a new carton/container to a "New" or "In Progress" shipment.
Container item/reason combination cannot be duplicated within the container.
Table 4-135 API Basics
Endpoint URL |
{base URL}/{shipmentId}/cartons |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the transfer shipment |
Input |
Transfer Shipment Carton |
Output |
Transfer Shipment Carton Status |
Maximum Input Limit |
1,000 overall line items on carton |
Table 4-136 Input Data Definition
Column |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A carton identifier or barcode label from an external system. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
BigDecimal(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
trackingNumber |
String(128) |
A tracking number for the container. |
|
useAvailableInventory |
Boolean |
True indicates use only available inventory, False indicates use non-available inventory. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
lineItems |
Collection |
X |
The line items in the container. |
Table 4-137 Shipment Line Item Data Definition
Column |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the item. |
caseSize |
BigDecimal(10,2) |
The case size of this line item. |
|
quantity |
BigDecimal(20,4) |
X |
The quantity to be shipped. |
transferId |
Long(12) |
X |
The unique identifier of the associated transfer. |
reasonId |
Long(15) |
A unique identifier of a shipment reason associated to this item. It is not allowed for available inventory and required for unavailable inventory. |
|
uins |
List<String> |
A list of UINs to be shipped. This must match the quantity. |
Table 4-138 Output Data Definition
Attribute |
Type |
Definition |
shipmentId |
Long(15) |
The unique identifier of the shipment. |
cartonId |
Long(15) |
The unique identifier of the new carton. |
externalId |
String(128) |
The external identifier or barcode label of the container. |
status |
Integer(2) |
The carton status. See Index. |
Figure 4-19 Code Example
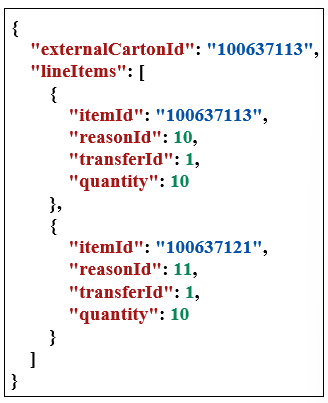
API: updateCarton
This API is used to update an existing carton that is in "New" or "In Progress" shipment.
Table 4-139 API Basics
Endpoint URL |
{base URL}/cartons/{cartonId} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the carton |
Input |
Transfer Shipment Carton |
Output |
Transfer Shipment Carton Status |
Maximum Input Limit |
1,000 overall line items on carton |
Table 4-140 Input Data Definition
Payload |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A container identifier from an external system. |
|
trackingNumber |
String(128) |
The tracking number of the container. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
Long(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
lineItems |
Collection |
A collection of up to 1,000 line items to update or add within the carton. See TransferShipmentUpdateCartontemIdo. |
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the SKU item. |
transferId |
Long(12) |
X |
The unique identifier of the associated transfer. |
reasonId |
Long(15) |
The unique identifier of the shipment reason associated to this line item. It is not allowed for available inventory and required for unavailable inventory. |
|
quantity |
BigDecimal(12,4) |
X |
The quantity shipped. Reducing this to 0 will remove the line item from the shipment. |
caseSize |
BigDecimal(10,2) |
The case size of the item for this particular shipment. |
|
uins |
Collection<String> |
The UINs associated to the item quantities. The number of UINS must match the quantity shipped. |
Figure 4-20 Code Example
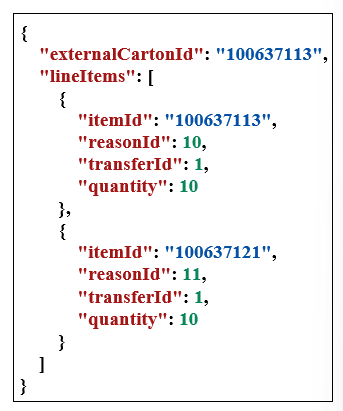
REST Service: Translations
This page captures the service APIs related to retrieving translations. It allows the translation of such things as labels and item descriptions.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/translations
API Definitions
API |
Description |
Find Locales |
Finds all locales that can be used to translation a series of text keys. |
Find Translations |
Finds the translations for a series of text keys, translating into text for the locale if it is available. |
Find Item Descriptions |
Finds the translations for item descriptions, translating it to the text of the locale if it is available. |
API: Find Locales
Finds all locales available for use in translation.
API Basics
Endpoint URL |
{base URL}/locales |
||
Method |
GET |
||
Successful Response |
200 OK |
||
Processing Type |
Synchronous |
||
Input |
Criteria |
||
Output |
List of locales |
||
Max Response Limit |
N/A |
Output Data Definition
Attribute |
Data Type |
Description |
|
localeId |
Long |
The SIOCS internal unique identifier of the record. |
|
language |
String |
A code representing a language (ISO 639 alpha-2 or alpha-3 language code). |
|
country |
String |
A code representing a country of the language (ISO 3166 alpha-2 country code or UN M.49 numeric-3 area code. ) |
|
variant |
String |
A code representing a variant of the country of the language (an arbitrary value indication the variant). |
|
description |
String |
A description of the locale. |
Example Output
[
{
"localeId": 1,
"language": "en",
"description": "English"
},
{
"localeId": 2,
"language": "de",
"description": "German"
},
{
"localeId": 3,
"language": "fr",
"description": "French"
}
]
API: Find Translations
Searches for translations for text keys and a given locale.
API Basics
Endpoint URL |
{base URL}/find |
||
Method |
POST |
||
Successful Response |
200 OK |
||
Processing Type |
Synchronous |
||
Input |
Criteria |
||
Output |
A map of translation key and its translation |
||
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
localeId |
Long(12) |
Yes |
Unique identifier of the Locale to translate keys for (see find Locales). |
keys |
List<String(600)> |
Yes |
A list of text keys to attempt to translate. |
Example Input
{
"localeId": 1,
"keys": [
"invAdjReason.1",
"invAdjReason.2"
]
}
Output Data Definition
Attribute |
Data Type |
Description |
|
values |
Map<String, String> |
A map where the key is the translation key and the value is the translation value. |
Example Output
{
"invAdjReason.2": "Shrinkage",
"invAdjReason.1": "Wastage"
}
API: Find Items Descriptions
Finds the translations for item descriptions, translating it to the text of the locale if it is available.
API Basics
Endpoint URL |
{base URL}/items |
||
Method |
POST |
||
Successful Response |
200 OK |
||
Processing Type |
Synchronous |
||
Input |
Criteria |
||
Output |
A list of translation items |
||
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
LocaleId |
Long (12) |
Yes |
Unique identifier of the Locale to translate descriptions for (see findLocales). |
itemIds |
List<String(25)> |
Yes |
A list of items to get descriptions for within the locale. |
Example Input
{
"localeId": 1,
"itemIds": [
"100637121",
"100637113"
]
}
Output Data Definition
Attribute |
Data Type |
Required |
Description |
itemId |
String |
The item identifier. |
|
shortDescription |
String |
The short description in the locale's text if available. |
|
longDescription |
String |
The long description in the locale's text if available. |
Example Output
[
{
"itemId": "100637121",
"shortDescription": "translation value for 100637121",
"longDescription": "translation value for 100637121"
},
{
"itemId": "100637113",
"shortDescription": "translation value for 100637113",
"longDescription": "translation value for 100637113"
}
]
REST Service: Vendor Delivery
This service allows the import and handling of direct store deliveries from vendors/suppliers.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/dsds
API Definitions
API |
Description |
readDelivery |
Read the full details of a vendor delivery. |
findDeliveries |
Find vendor delivery headers based on input search criteria. |
receiveDelivery |
Receives the expected quantities of a vendor delivery so that it is ready to be confirmed. |
confirmDelivery |
Confirm the receipt of a vendor delivery updating inventory positions with the receipt information. |
rejectDelivery |
Rejects the vendor delivery and do not allow it to be received. |
cancelDelivery |
Cancel a vendor delivery. |
submitCarton |
Moves the status of the carton to submitted and prevents further updates. The carton must still be confirmed. No inventory positions are updated via this operation. |
cancelSubmitCarton |
Opens a submitted carton for further updates, moving the status back to in-progress. |
confirmCarton |
Confirms the receipt a vendor delivery carton. |
cancelCarton |
Cancels a vendor delivery carton. |
openCarton |
Re-opens a completed carton after receipt allowing it to be received a second time (possibly adjusting quantities). |
API: Read Delivery
Retrieves a vendor delivery.
Table 4-144 API Basics
Endpoint URL |
/{deliveryId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
None |
Output |
Transfer Shipment |
Table 4-145 Path Parameter Definitions
Parameter |
Definition |
deliveryId |
The internal identifier of the vendor delivery header. |
Table 4-146 Output Data Definition
Column |
Type |
Definition |
deliveryId |
Long(12) |
The unique identifier of the delivery record. |
storeId |
Long(10) |
The unique identifier of the store receiving the inventory. |
supplierId |
Long(10) |
The unique identifier of the supplier shipping the inventory. |
status |
Integer(4) |
The current status of the delivery. See Vendor Delivery Status. |
originType |
Integer(2) |
The origin type of the delivery. See Vendor Delivery Origin Type. |
purchaseOrderId |
Long(12) |
The purchase order that the delivery is associated to. |
receiptNumber |
Long |
The global receipt number of the delivery used to identifier the receipt across applications through integration. |
asn |
String(128) |
The advanced shipping notification of the delivery. |
invoiceNumber |
String(128) |
A unique identifier of an invoice associated to this delivery. |
invoiceCurrency |
String |
A currency code of the invoice cost. |
invoiceAmount |
BigDecimal |
The value of the invoice cost. |
customerOrderId |
String(128) |
A customer order identifier (from an external system) associated to the delivery. |
fulfillmentOrderId |
String(128) |
A fulfillment order identifier (from an external system) associated to the delivery. |
billOfLadingId |
String(128) |
An external identifier of a bill of lading record. |
carrierName |
String(128) |
The name of the carrier. |
carrierType |
Integer(2) |
The type of the carrier. See Carrier Type. |
carrierCode |
String(4) |
Unique code that identifies the carrier. |
countryCode |
String(3) |
A country code. |
sourceAddress |
String(1000) |
The address of the source shipping location sending the delivery to the store. |
licensePlate |
String(128) |
The license plate of the delivery vehicle. |
freightId |
String(128) |
A freight identifier associated to the delivery. |
fiscalDocumentRequestId |
Long(20) |
The identifier of the request for a fiscal document. |
fiscalDocumentReferenceId |
Long(20) |
The unique identifier of the fiscal document. |
fiscalDocumentNumber |
String(255) |
The fiscal document number. |
createDate |
Date |
The date the delivery record was created. |
updateDate |
Date |
The date the delivery record was last updated. |
expectedDate |
Date |
The expected date of the delivery. |
invoiceDate |
Date |
The date of the delivery invoice. |
receivedDate |
Date |
The date the delivery was received. |
createUser |
String(128) |
The user that created the delivery .record. |
updateUser |
String(128) |
The user who last updated the delivery record. |
receivedUser |
String(128) |
The user who received the delivery record. |
cartons |
Collection |
A list of cartons. |
Table 4-147 Open Data Definition (Carton)
Column |
Type |
Definition |
cartonId |
Long(12) |
The unique identifier of the carton record. |
externalCartonId |
String(128) |
An external identifier of the carton. |
referenceId |
String(128) |
A reference identifier to the carton. |
status |
Integer(4) |
The current status of the carton (See Additional Data Definitions Vendor Delivery Carton Status) |
damagedReason |
String(128) |
A reason for the carton damage that took place. |
serialCode |
Long(18) |
A serial code for the carton. |
trackingNumber |
String(128) |
The tracking number of the carton. |
damageRemaining |
Boolean |
indicates all remaining quantities should be damaged on final receipt. |
uinRequired |
Boolean |
True if a UIN item exists within the carton, otherwise false. |
receiveAtShopFloor |
Boolean |
True if receive the inventory at shop floor, otherwise false. |
qualityControl |
Boolean |
True indicates that the carton requires detailed receiving. |
externalCreate |
Boolean |
True indicates the carton was externally created, false indicates it was created by EICS. |
adjusted |
Boolean |
True indicates the carton is adjusted, otherwise false. |
customerOrderRelated |
Integer(4) |
Customer Order Related Type (See Additional Data Definitions) |
createUser |
String(128) |
The user who created the carton. |
updateUser |
String(128) |
The user who last updated the carton. |
receivedUser |
String(128) |
The user who received the carton. |
createDate |
Date |
The date the carton was created. |
updateDate |
Date |
The date the carton was last updated. |
receivedDate |
Date |
The date the carton was received. |
lineItems |
Collection |
The line items associated with the container. |
Table 4-148 Output Data Definition (Line Item)
Column |
Type |
Definition |
lineId |
Long(12) |
The unique identifier of the line item record. |
itemId |
String(25) |
The unique identifier of the item. |
caseSize |
BigDecimal(10,2) |
A number of units in the case that this item was shipped with. |
quantityExpected |
BigDecimal(20,4) |
The total number of units expected on the delivery. |
quantityReceived |
BigDecimal(20,4) |
The total number of units received on the delivery. |
quantityDamaged |
BigDecimal(20,4) |
The total number of units received as damaged on the delivery. |
quantityReceivedOverage |
BigDecimal(20,4) |
Amount of received inventory over expected quantities. |
quantityDamagedOvarage |
BigDecimal(20,4) |
Amount of received damage inventory over expected quantities. |
quantityPreviouslyReceived |
BigDecimal(20,4) |
Units previously received (captured at time container is re-opened after receipt) |
quantityPreviouslyDamaged |
BigDecimal(20,4) |
Units previously received as damaged (captured at time container is re-opened after receipt) |
unitCostCurrency |
String(3) |
The unit cost currency of this item delivery. |
unitCostAmount |
BigDecimal(12,4) |
The unit cost value of this item delivery. |
unitCostOverrideCurrency |
String(3) |
The override unit cost currency of this item delivery. |
unitCostOverrideAmount |
BigDecimal(12,4) |
The override unit cost value of this item delivery. |
purchaseOrderId |
Long (12) |
The internal unique identifier of the purchase order of this particular item delivery. |
purchaseOrderNumber |
String(128) |
The external purchase order number of this particular item delivery. |
customerOrderNumber |
String(128) |
The unique external customer order identifier of this particular item delivery. |
fulfillmentOrderNumber |
String(128) |
The unique external fulfillment order identifier of this particular item delivery. |
vendorProductNumber |
String(256) |
The vendor product number of the item. |
uins |
Collection<String> |
A list of UINs associated to the line item. |
API: Find Vendor Delivery
API is used to find transaction headers for vendor deliveries.
If more than 10,000 deliveries are found, a "results too large" error will be returned. Limit the results with further search criteria.
Table 4-149 API Basics
Endpoint URL |
|
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of Vendor Shipments |
Table 4-150 Query Parameter Definitions
Attribute |
Type |
Definition |
storeId |
Long(10) |
Include only records where the delivery is to this store. |
asn |
String(128) |
Include only records for this advanced shipping notification. |
originType |
Integer(2) |
Include only records for this origin type. (See Additional Data Definitions: Vendor Delivery Origin Type). |
status |
Integer(4) |
Include only records where the delivery is in this status. (See Additional Data Definitions: Vendor Delivery Criteria Status) |
customerOrderNumber |
String(128) |
Include only records for this customer order number. |
fulfillmentOrderNumber |
String(128) |
Include only records for this fulfilment order number. |
invoiceNumber |
String(128) |
Include only records for this invoice number. |
supplierId |
Long(10) |
Include only records for this supplier identifier. |
purchaseOrderNumber |
String(12) |
Include only records where a line item is associated to this external purchase order number. |
updateDateFrom |
String |
Include only records with a last update date on or after this date. |
updateDateto |
String |
Include only records with a first update date on or before this date. |
Table 4-151 Output Data Definition
Column |
Type |
Definition |
deliveryId |
Long(12) |
The unique identifier of the delivery record. |
storeId |
Long(10) |
The unique identifier of the store receiving the inventory. |
supplierId |
Long(10) |
The unique identifier of the supplier shipping the inventory. |
status |
Integer(4) |
The current status of the delivery. See Vendor Delivery Status. |
originType |
Integer(2) |
The origin type of the delivery. See Vendor Delivery Origin Type. |
purchaseOrderId |
Long(12) |
The purchase order that the delivery is associated to. |
receiptNumber |
Long |
The global receipt number of the delivery used to identifier the receipt across applications through integration. |
asn |
String(128) |
The advanced shipping notification of the delivery. |
invoiceId |
String(128) |
A unique identifier of an invoice associated to this delivery. |
customerOrderId |
String(128) |
A customer order identifier associated to the delivery. |
fulfillmentOrderId |
String(128) |
A fulfillment order identifier (from an external system) associated to the delivery. |
createDate |
Date |
The date the delivery record was created. |
updateDate |
Date |
The date the delivery record was last updated. |
expectedDate |
Date |
The expected date of the delivery. |
invoiceDate |
Date |
The date of the delivery invoice. |
receivedDate |
Date |
The date the delivery was received. |
API: Receive Delivery
Updates the received quantities to quantities that were expected so that it is ready to be confirmed. It puts the delivery in the "In Progress" status.
Table 4-152 API Basics
Endpoint URL |
/{deliveryId}/receive |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-153 Path Parameter Definitions
Parameter |
Definition |
deliveryId |
The internal identifier of the vendor delivery header. |
API: Confirm Delivery
Confirms the delivery and receives the goods into inventory.
Table 4-154 API Basics
Endpoint URL |
/{deliveryId}/confirm |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-155 Path Parameter Definitions
Parameter |
Definition |
deliveryId |
The internal identifier of the vendor delivery header. |
API: Reject Delivery
Rejects the delivery without receiving goods, placing it in rejected status.
Table 4-156 API Basics
Endpoint URL |
/{deliveryId}/reject |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-157 Path Parameter Definitions
Parameter |
Definition |
deliveryId |
The internal identifier of the vendor delivery header. |
API: Cancel Delivery
Cancels the delivery without receiving the goods and places it in canceled status.
Table 4-158 API Basics
Endpoint URL |
/{deliveryId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-159 Path Parameter Definitions
Parameter |
Definition |
deliveryId |
The internal identifier of the vendor delivery header. |
API: Submit Carton
Moves the status of the carton to submitted and prevents further updates. The carton may still be confirmed. No inventory positions are updated via this operation.
Table 4-160 API Basics
Endpoint URL |
cartons/{cartonId}/submit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-161 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the vendor delivery carton. |
API: Cancel Submit Carton
Opens a submitted carton for further updates, moving the status to in-progress.
Table 4-162 API Basics
Endpoint URL |
cartons/{cartonId}/cancelsubmit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-163 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the vendor delivery carton. |
API: Confirm Carton
Confirms the final receipt of a vendor delivery carton.
Table 4-164 API Basics
Endpoint URL |
cartons/{cartonId}/confirm |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-165 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the vendor delivery carton. |
API: Cancel Carton
Cancels a vendor delivery carton.
Table 4-166 API Basics
Endpoint URL |
cartons/{cartonId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-167 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the vendor delivery carton. |
API: Open Carton
Re-open a completed carton after receipt allowing it to be received again.
Table 4-168 API Basics
Endpoint URL |
cartons/{cartonId}/open |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Input |
None |
Output |
None |
Table 4-169 Path Parameter Definitions
Parameter |
Definition |
cartonId |
The internal identifier of the vendor delivery carton. |
Additional Data Definitions
Table 4-170 Vendor Delivery Carton Status
Value |
Status |
1 |
New |
2 |
In Progress |
3 |
Submitted |
4 |
Received |
5 |
Damaged |
6 |
Missing |
7 |
Canceled |
Table 4-171 Customer Order Related Type
Value |
Status |
1 |
Yes |
2 |
Mix |
3 |
No |
Table 4-172 Vendor Delivery Status
Value |
Status |
1 |
New |
2 |
In Progress |
3 |
Received |
4 |
Canceled |
5 |
Rejected |
Table 4-173 Vendor Delivery Criteria Status
Value |
Status |
1 |
New |
2 |
In Progress |
3 |
Received |
4 |
Canceled |
5 |
Rejected |
99 |
Active |
Table 4-174 Vendor Delivery Origin Type
Value |
Status |
1 |
Advanced Shipping Notification |
2 |
Purchase Order |
3 |
Dex-Nex |
4 |
Manual/On the Fly |
Table 4-175 Vendor Delivery Create Origin Type
Value |
Status |
2 |
Purchase Order |
3 |
Dex-Nex |
4 |
Manual/On the Fly |
Table 4-176 Carrier Type
Value |
Status |
1 |
Corporate |
2 |
Third Party |
Rest Service: Vendor Return
A return request is a return to vendor that comes into the store inventory system from an external system. A return request is a made for items to be returned to the supplier from the store. The items and quantities requested to be returned and reasons (for RTV) will be listed. Updating the return allows the approved quantity to be assigned. Once the request has been approved, the items are shipped to the supplier.
The service allows for the integration of return requests with an external system.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api
API Definitions
Table 4-177 Vendor Return API Definitions
API |
Description |
approveReturn |
Approves a vendor return for shipment. Use the Update Return operation to assign and persist desired quantities before approval. |
closeReturn |
Closes out the vendor return. |
findReturn |
Finds up to 10,000 return headers for the input criteria. |
importReturn |
Imports a vendor return request managed by an external system. |
importReturnDelete |
This will place a delete notification in the system (in the MPS work queue with DcsRtv work type) for asynchronous processing. |
readReturn |
Retrieves all the details about a vendor return. |
updateReturn |
Update vendor return approved quantities prior to approving the vendor return. |
API: Approve Return
Approves a vendor return for shipment. Use the Update Return operation to assign and persist desired quantities before approval.
Table 4-178 API Basics
Endpoint URL |
/vendorreturns/{returnId}/approve |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
|
Input |
Path parameter |
Output |
None |
Table 4-179 Path Parameter Definitions
Parameter |
Definition |
returnId |
The unique identifier of the vendor return. |
Table 4-180 Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request Example:
|
API: Close Return
Closes out the vendor return..
Table 4-181 API Basics
Endpoint URL |
/vendorreturns/{returnId}/close |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
|
Input |
Path parameter |
Output |
None |
Table 4-182 Path Parameter Definitions
Parameter |
Definition |
returnId |
The unique identifier of the vendor return. |
Table 4-183 Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request Example:
|
API: Find Return
Finds up to 10,000 return headers for the input criteria.
Table 4-184 API Basics
Endpoint URL |
/vendorreturns |
Method |
Get |
Successful Response |
200 Successful |
Processing Type |
|
Input |
Query parameters |
Output |
List of returns |
Max Response |
10,000 |
Table 4-185 Query Parameter Definitions
Name |
Format |
Description |
storeId |
number(int10) |
Include only records from this store. |
supplierId |
number(int10) |
Include only returns to this supplier. |
externalReturnId |
string(text128) |
Include only returns for this vendor return. |
status |
integer(int4) |
Include only returns with this status. Valid values are: <br/> 1 - Requested <br/> 2 - Request In Progress <br/> 3 - RTV In Progress <br/> 4 - Approved <br/> 5 - In Shipping <Br/> 6 - Completed <br/> 7 - Rejected <br/> 8 - Canceled Request <br/> 9 - Canceled RTV <br/> 99 - Active |
itemId |
string(text25) |
Include only returns that contain this item. |
shipmentReasonId |
number(int15) |
Include only returns that contain this shipment reason. |
updateDateFrom |
string(date-time) |
Include only returns with an update date on or after this date. |
updateDateTo |
string(date-time |
Include only returns with an update date on or before this date. |
Table 4-186 Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
API: Import Return
Imports a vendor return request managed by an external system. Processing assumes that any notification to other systems about the creation of this information will be managed by the external system. The imported return is processed asynchronous through our MPS sub-system and is controlled by the DcsRtv work type. If more than 1,000 items are included in the return, a "input too large" error will be returned. This operation will return a forbidden error code if the system is integrated with Oracle merchandising.
Table 4-187 API Basics
Endpoint URL |
/vendorreturns/imports |
Method |
POST |
Successful Response |
202 Accepted |
Processing Type |
asynchronous |
Input |
Query parameters |
Output |
N/A |
Max Response |
1000 |
Table 4-188 Query Parameter Definitions
Parameter |
Format |
Required |
Definition |
externalReturnId |
string(text128) |
Yes |
A unique identifer of the vendor return from an external system. This identifier is used to determine if the import will be created or updated. |
storeId |
number(int10) |
Yes |
The identifier of the store returning the inventory. This value is ignored if the return already exists. |
supplierId |
number(int10) |
Yes |
The identifier of the supplier the goods are being shipped to. This value is ignored if the return already exists. |
notAfterDate |
string(date-time) |
No |
A date after which the return should not be shipped. If not included, this value will be defaulted through system configuration. |
authorizationCode |
string(text12) |
No |
An authorization number that may be required for the return. |
addressLine1 |
string(text240) |
No |
The first line of the destination address. |
addressLine2 |
string(text240) |
No |
The second line of the destination address. |
addressLine3 |
string(text240) |
No |
The third line of the destination address. |
addressCity |
string(text120 |
No |
The city of the destination address. |
addressState |
string(text3) |
No |
The state of the destination address. |
addressCountry |
string(text3) |
No |
The country code of the destination address. |
addressPostalCode |
string(text30) |
No |
The postal code of the destination address. |
comment |
string(text2000) |
No |
A comment to associate to the vendor return request. |
createDate |
string(date-time) |
Yes |
The date the return was created. |
lineItems |
Array |
Yes |
Vendor Return Import Line Items itemId — the unique identifier of the SKU item. format: string(text25), required reasonCode — a reason code associated to the item return. format:string(text6), required quantity — the quantity requested to return. format: BigDecimal(decimal (20,4)), required sequence — An external sequence number for the item on the return. format: BigDecimal(int15), optional |
Table 4-189 Responses
Code |
Description |
202 |
Accepted |
400 |
Bad Request Example:
|
API: Import Return Delete
This will place a delete notification in the system (in the MPS work queue with DcsRtv work type) for asynchronous processing. When processed, it will cancel the vendor return if it qualifies for cancellation (it is not already in progress). This operation will return a forbidden error code if the system is integrated with Oracle merchandising.
Table 4-190 API Basics
Endpoint URL |
/vendorreturns/imports |
Method |
DELETE |
Successful Response |
202 Accepted |
Processing Type |
asynchronous |
Input |
Path parameters |
Output |
N/A |
Max Response |
1000 |
Table 4-191 Path Parameters
Parameter |
Format |
Required |
Definition |
externalReturnId |
string(text128) |
Yes |
A unique identifer of the vendor return from an external system. |
Table 4-192 Responses
Code |
Description |
202 |
Accepted |
400 |
Bad Request Example:
|
API: Read Return
Retrieves all the details about a vendor return.
Table 4-193 API Basics
Endpoint URL |
/vendorreturns/{returnId} |
Method |
Get |
Successful Response |
200 Successful |
Processing Type |
|
Input |
Path parameters |
Output |
return information |
Max Response |
10,000 |
Table 4-194 Path Parameters
Name |
Format |
Required |
Description |
returnId |
number(int12) |
Yes |
The unique identifier of the vendor return |
Table 4-195 Responses
Code |
Description |
200 |
Successful Example:
|
400 |
Bad Request Example:
|
API: Update Return
Update vendor return approved quantities prior to approving the vendor return.
Table 4-196 API Basics
Endpoint URL |
/vendorreturns/{returnId}/ |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
|
Input |
Path parameter, Request |
Output |
N/A |
Table 4-197 Path Parameter
Parameter |
Format |
Required |
Definition |
returnId |
number(int12) |
Yes |
The unique identifier of the vendor return. |
Request
in the Request body, enter the vendor return details to update.
{
"authorizationCode": "JID112",
"lineItems": [
{
"itemId": "10045600",
"shipmentReasonId": 82236,
"quantity": 100
}
]
}
Table 4-198 Responses
Code |
Description |
204 |
No Content |
400 |
Bad Request Example:
|
REST Vendor Shipment
This service allows the creation, modification, and cancellation of vendor return shipments and vendor return shipment cartons from an external application.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer></cust_env>/siocs-int-services/api/rtvshipments
APIs
API |
Description |
readShipment |
Reads an entire return-to-vendor shipment. |
findShipment |
Finds vendor return shipments based on input criteria. |
submitShipment |
Submits the vendor shipment. |
cancelSubmitShipment |
Cancels the submission of a vendor shipment. |
dispatchShipment |
Dispatches a vendor shipment. |
cancelShipment |
Cancels a vendor shipment. This will close the associated vendor return. |
confirmCarton |
Confirms a vendor shipment carton. |
cancelCarton |
Cancels a vendor shipment carton. |
openCarton |
Opens a previously confirmed vendor shipment carton prior to dispatch. |
createShipment |
Creates a new vendor shipment along with cartons and line items. |
updateShipment |
Updates vendor shipment header information. |
createCarton |
Adds a carton to an existing vendor shipment. |
updateCarton |
Updates vendor shipment carton information. |
API: readShipment
Reads a Vendor return shipment.
Table 4-199 API Basics
Endpoint URL |
{base URL}/{shipmentId} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment |
Output |
The vendor shipment |
Table 4-200 Input Data Definition
Column |
Type |
Definition |
shipmentId |
Long(15) |
The unique identifier of the record. |
storeId |
Long(10) |
The unique identifier of the store. |
supplierId |
Long(10) |
The unique identifier of the supplier. |
vendorReturnId |
Long(15) |
The unique identifier of the vendor return. |
vendorReturnExternalId |
String(128) |
A unique identifier from an external system. |
status |
Integer(2) |
The shipment status. See Index. |
notAfterDate |
Date |
A date after which the shipment should not be shipped. |
authorizationCode |
String(12) |
A vendor authorization code |
contextId |
Long(18) |
An identifier of a context for the shipment. |
contextValue |
String(25) |
A context value of the context |
cartons |
List<Object> |
A collection of cartons for the shipment |
ADDRESS |
||
destinationAddressLine1 |
String(240) |
The first line of the destination address. |
destinationAddressLine2 |
String(240) |
The second line of the destination address. |
destinationAddressLine3 |
String(240) |
The third line of the destination address. |
destinationAddressCity |
String(120) |
The city of the destination address. |
destinationAddressState |
String(3) |
The state of the destination address. |
destinationAddressCountry |
String(3) |
The country code of the destination address (used by supplier). |
destinationAddressPostalCode |
String(30) |
The postal code of the destination address. |
BILL OF LADING |
||
billOfLadingId |
Long(15) |
The bill of lading number. |
alternateAddress |
String(2000) |
An alternate destination address. |
carrierRole |
Integer(2) |
The type of carrier for the shipment. See Index |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company. |
motive |
String(120) |
A motive for the shipment. |
taxId |
String(18) |
The tax identifier of the supplier it is being shipped to. |
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
weight |
BigDecimal(12,4) |
The weight of the shipment. |
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
requestedPickupDate |
Date |
The requested pickup date. |
FISCAL DOCUMENT |
||
fiscalDocumentRequestId |
Long(20) |
The identifier of the request for a fiscal document. |
fiscalDocumentReferenceId |
Long(20) |
The unique identifier of the fiscal document. |
fiscalDocumentNumber |
String(255) |
The fiscal document number. |
fiscalDocumentStatus |
Integer(4) |
The status of the fiscal document. |
fiscalDocumentRejectReason |
String(255) |
A reason the fiscal document was rejected. |
fiscalDocumentUrl |
String(255) |
A URL to the fiscal document. |
Dates |
||
createUser |
String(128) |
The user that created the shipment in EICS. |
createDate |
Date |
The date the shipment was created in EICS. |
submitUser |
String(128) |
The user that submitted the shipment in EICS. |
submitDate |
Date |
The date the shipment was submitted in EICS. |
dispatchUser |
String(128) |
The user that dispatched the shipment in EICS. |
dispatchDate |
Date |
The date the shipment was dispatched in EICS. |
updateUser |
String(128) |
The user that last updated the shipment in EICS. |
updateDate |
Date |
The date the shipment was last updated in EICS. |
Table 4-201 Shipment Carton Data Definition
Column |
Type |
Definition |
cartonId |
Long(15) |
The unique identifier of the record. |
externalCartonId |
String(128) |
A container identifier from an external system. |
status |
Long(2) |
The status of the carton. See Index |
cartonDimensionId |
Long(10) |
The shipment container dimension identifier. |
weight |
BigDecimal(12,4) |
The weight of the container. |
weightUom |
String(4) |
The unit of measure of the weight of the container. |
trackingNumber |
String(128) |
The tracking number of the container. |
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. See Index. |
createUser |
String(128) |
The user that created the container in EICS. |
createDate |
Date |
The date the container was created in EICS. |
updateUser |
String(128) |
The user that last updated the container in EICS. |
updateDate |
Date |
The user that last updated the container in EICS. |
approvalUser |
String(128) |
The user that approved the container in EICS. |
approvalDate |
Date |
The date the container was approved in EICS. |
lineItems |
Collection |
A collection of line items. |
Table 4-202 Shipment Line Item Data Definition
Column |
Type |
Definition |
lineId |
Long(15) |
The unique identifier of the line item record. |
itemId |
String(25) |
The unique identifier of the item. |
shipmentReasonId |
Long(15) |
The unique identifier of the shipment reason. |
caseSize |
BigDecimal(10,2) |
The case size of this record. |
quantity |
BigDecimal(20,4) |
The quantity shipped. |
uins |
Collection<String> |
The UINs being shipped. |
API: findShipments
This API is used to find transaction headers for vendor shipments.
Table 4-203 API Basics
Endpoint URL |
{base URL} |
Method |
GET |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
Query Parameters |
Output |
List of vendor shipment headers |
Maximum Response Limit |
10,000 |
(Query Parameters Table Missing)
Column |
Type |
Definition |
storeId |
Long(10) |
Include only shipments from this store. |
supplierId |
Long(10) |
Include only shipments to this supplier. |
vendorReturnId |
Long(15) |
Include only shipments for this vendor return (by internal id). |
vendorReturnExternalId |
String(128) |
Include only shipments for this vendor return (by external id). |
status |
Integer(2) |
Include only shipments for this status. |
itemId |
String(25) |
Include only shipments that contain this item. |
updateDateFrom |
String(128) |
Include only shipments that were last updated on or after this date. |
updateDateTo |
String(128) |
Include only shipments that were last updated on or before this date. |
shipmentId |
Long(15) |
The unique identifier of the shipment. |
storeId |
Long(10) |
The unique identifier of the store. |
supplierId |
Long(10) |
The unique identifier of the supplier. |
vendorReturnId |
Long(15) |
The unique identifier of the vendor return. |
vendorReturnExternalId |
String(128) |
A unique identifier of the vendor return from an external system. |
status |
Integer(2) |
The shipment status. See Index. |
notAfterDate |
Date |
A date after which the shipment should not be shipped. |
submitDate |
Date |
The date the shipment was submitted in EICS. |
dispatchDate |
Date |
The date the shipment was dispatched in EICS. |
updateDate |
Date |
The date the shipment was last updated in EICS. |
API: submitShipment
This API submits the vendor shipment. This places the vendor shipment into a submitted status awaiting review and eventual dispatch. Fiscal document data capture can occur as part of this process.
Table 4-204 API Basics
Endpoint URL |
{base URL}/{shipmentId}/submit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment |
Input |
Optional shipping information |
Column |
Type |
Definition |
vehicleNumber |
String(25) |
The vehicle license plate or identifying number. |
vehicleStateOrCountry |
String(25) |
The vehicles state or country. |
driverName |
String(30) |
The name of the driver. |
driverLicenseNumber |
String(30) |
The driver's license number. |
Figure 4-21 Example Input
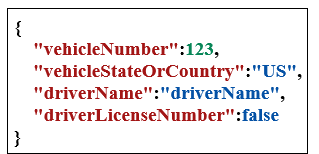
API: cancelSubmitShipment
This API cancels the submission of a vendor shipment, moving it back to ‘in progress’ status.
Table 4-205 API Basics
Endpoint URL |
{base URL}/{shipmentId}/cancelsubmit |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment |
API: dispatchShipment
This API ispatches the vendor shipment to the destination, closing the shipment and updating inventory appropriately.
Table 4-206 API Basics
Endpoint URL |
{base URL}/{shipmentId}/dispatch |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment |
API: cancelShipment
This API cancels the vendor shipment. This will close the associated vendor return.
Table 4-207 API Basics
Endpoint URL |
{base URL}/{shipmentId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment |
API: confirmCarton
This API confirms a vendor shipment carton. This indicates the carton is ready for dispatch and sets it so it can no longer be modified.
Table 4-208 API Basics
Endpoint URL |
{base URL}/cartons/{cartontId}/confirm |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment carton |
API: cancelCarton
This API cancels a vendor shipment carton, effectively removing its contents from the shipment.
Table 4-209 API Basics
Endpoint URL |
{base URL}/cartons/{cartontId}/cancel |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment carton |
API: openCarton
This API opens a previously confirmed vendor shipment carton so that it can be modified again.
Table 4-210 API Basics
Endpoint URL |
{base URL}/cartons/{cartontId}/open |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The identifier of the shipment carton |
API: createShipment
This API is used to create a new vendor shipment whose status is "In Progress."
Table 4-211 API Basics
Endpoint URL |
{base URL} |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Input |
The vendor shipment |
Output |
The vendor shipment status |
Maximum Input Limit |
1,000 items on the shipment |
Table 4-212 Input Data Definition
Attribute |
Type |
Req |
Definition |
storeId |
Long(10) |
X |
The unique identifier of the store. |
supplierId |
Long(10) |
X |
The unique identifier of the supplier. |
vendorReturnId |
Long(15) |
The unique identifier of a return to vendor request associated to this shipment. |
|
authorizationCode |
String(12) |
A vendor authorization code. It may be required for some suppliers. |
|
notAfterDate |
Date |
A date after which the shipment should not be shipped. |
|
contextId |
Long(18) |
An identifier that associates a context to the shipment. |
|
contextValue |
String(25) |
A context value for the context. |
|
addressType |
Integer |
The type of return address. See Index. |
|
carrierRole |
Integer(2) |
X |
The type of carrier for the shipment. See Index. |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
|
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
|
alternateAddress |
String(2000) |
An alternate destination address. |
|
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
|
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company. |
|
motiveId |
Long(18) |
The unique identifier of a bill of lading motive. |
|
taxId |
String(18) |
The tax identifier of the supplier it is being shipped to. |
|
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
|
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
|
weight |
BigDecimal(12,4) |
The weight of the shipment. |
|
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
|
requestedPickupDate |
Date |
The requested pickup date. |
|
cartons |
Collection |
X |
A collection of cartons to create along with the shipment. |
notes |
Collections of Strings() |
A collection of up to 100 notes. |
Table 4-213 Shipment Carton Data Definition
Payload |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A container identifier from an external system. |
|
trackingNumber |
String(128) |
The tracking number of the container. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
Long(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
lineItems |
Collection |
X |
A collection of line items to create along with the carton. |
Table 4-214 Shipment Line Item Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the SKU item. The combination of item identifier and reason identifier make a unique line. |
reasonId |
Long(15) |
X |
The unique identifier of the shipment reason associated to this line item. The combination of item identifier and reason identifier make a unique line. |
quantity |
BigDecimal(12,4) |
X |
The quantity shipped. |
caseSize |
BigDecimal(10,2) |
The case size of the item for this particular shipment. |
|
uins |
Collection |
A list of UINs being shipped on the line item. The number of UINs must match the quantity. |
Table 4-215 Output Data Definition
Attribute |
Type |
Definition |
shipmentId |
Long(15) |
The unique identifier of the shipment. |
returnId |
Long(15) |
The unique identifier of the associated vendor return. |
status |
Integer(2) |
The shipment status. See Index. |
Figure 4-22 Example Input
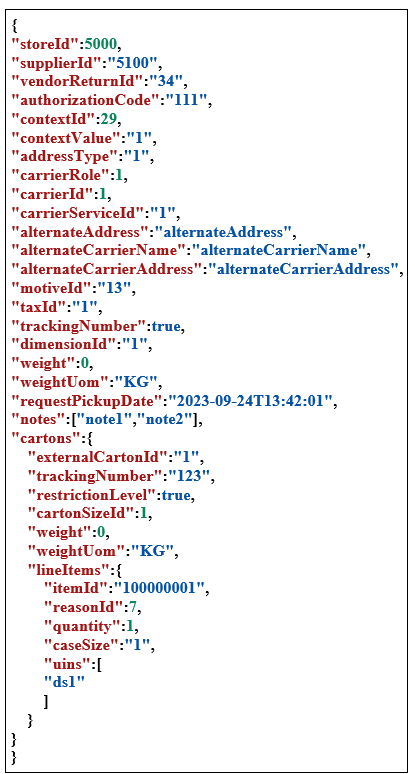
API: updateShipment
This API is used to update to the header portion of a shipment as well as its bill of lading information. See carton related operations for creating or updating cartons on a shipment.
Table 4-216 API Basics
Endpoint URL |
{base URL}/{shipmentId} |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The vendor shipment identifier |
Input |
Vendor shipment header information |
Table 4-217 Input Data Definitions
Attribute |
Type |
Req |
Definition |
authorizationCode |
String(12) |
A vendor authorization code. It may be required for some suppliers. |
|
notAfterDate |
Date |
A date after which the shipment should not be shipped. |
|
contextId |
Long(18) |
An identifier that associates a context to the shipment. |
|
contextValue |
String(25) |
A context value for the context. |
|
addressType |
Integer |
The type of return address. See Index. |
|
carrierRole |
Integer(2) |
X |
The type of carrier for the shipment. See Index. |
carrierId |
Long(10) |
A unique identifier of a carrier for the shipment. |
|
carrierServiceId |
Long(10) |
A unique identifier of a carrier service for the shipment. |
|
alternateAddress |
String(2000) |
An alternate destination address. |
|
alternateCarrierName |
String(240) |
The name of a third-party shipping company. |
|
alternateCarrierAddress |
String(2000) |
The address of a third-party shipping company. |
|
motiveId |
Long(18) |
The unique identifier of a bill of lading motive. |
|
taxId |
String(18) |
The tax identifier of the supplier it is being shipped to. |
|
trackingNumber |
String(128) |
A tracking number associated to the shipment. |
|
dimensionId |
Long(12) |
The identifier of a dimension associated to the shipment. |
|
weight |
BigDecimal(12,4) |
The weight of the shipment. |
|
weightUom |
String(4) |
The unit of measure of the weight of the shipment. |
|
requestedPickupDate |
Date |
The requested pickup date. |
|
notes |
Collections of Strings() |
A collection of up to 100 notes to be added to the list of notes. |
Figure 4-23 Example Input
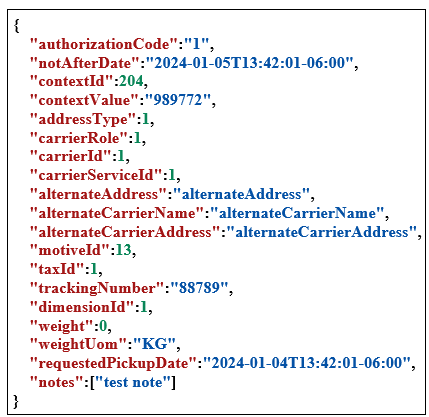
API: createCarton
This API is used to add a new carton/container to a "New" or "In Progress" shipment.
Table 4-218 API Basics
Endpoint URL |
{base URL}/{shipmentId}/cartons |
Method |
POST |
Successful Response |
200 OK |
Processing Type |
Synchronous |
Path Parameter |
The vendor shipment identifier |
Input |
Vendor shipment carton |
Output |
Vendor shipment carton status |
Maximum Input Limit |
Up to 1,000 items on the carton |
Table 4-219 Input Data Definition
Payload |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A container identifier from an external system. |
|
trackingNumber |
String(128) |
The tracking number of the container. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
Long(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
lineItems |
Collection |
X |
A collection of line items to create with the carton. |
Table 4-220 Shipment Line Item Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the SKU item. The combination of item identifier and reason identifier make a unique line. |
reasonId |
Long(15) |
X |
The unique identifier of the shipment reason associated to this line item. The combination of item identifier and reason identifier make a unique line. |
quantity |
BigDecimal(12,4) |
X |
The quantity shipped. |
caseSize |
BigDecimal(10,2) |
The case size of the item for this particular shipment. |
|
uins |
Collection<String> |
The UINs associated to the item quantities. Only included for items that are serial number UIN type. AGSN items will be blank. The number of UINS must match the quantity shipped. |
Table 4-221 Output Data Definition
Attribute |
Type |
Definition |
shipmentId |
Long(15) |
The unique identifier of the shipment. |
cartonId |
Long(15) |
The unique identifier of the new carton. |
status |
Integer(2) |
The carton status. See Index. |
Figure 4-24 Example Input
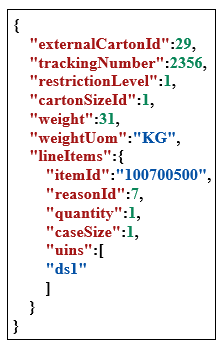
API: updateCarton
This API is used to update an existing carton that is in "New" or "In Progress" on a shipment that is "New" or "In Progress."
Table 4-222 API Basics
Endpoint URL |
{base URL}/cartons/{cartonId} |
Method |
POST |
Successful Response |
204 No Content |
Processing Type |
Synchronous |
Path Parameter |
The carton identifier |
Input |
Vendor shipment carton |
Maximum Input Limit |
Up to 1,000 items on the carton |
Table 4-223 Input Data Definition
Payload |
Type |
Req |
Definition |
externalCartonId |
String(128) |
A container identifier from an external system. |
|
trackingNumber |
String(128) |
The tracking number of the container. |
|
restrictionLevel |
Integer(4) |
A hierarchy restriction level for items in the container. |
|
cartonSizeId |
Long(10) |
The shipment container dimension identifier. |
|
weight |
Long(12,4) |
The weight of the container. |
|
weightUom |
String(4) |
The unit of measure of the weight of the container. |
|
lineItems |
Collection |
A collection of line items to update or add within the carton. |
Table 4-224 Shipment Line Item Data Definition
Payload |
Type |
Req |
Definition |
itemId |
String(25) |
X |
The unique identifier of the SKU item. The combination of item identifier and reason identifier make a unique line. |
reasonId |
Long(15) |
X |
The unique identifier of the shipment reason associated to this line item. The combination of item identifier and reason identifier make a unique line. |
quantity |
BigDecimal(12,4) |
X |
The quantity shipped. Setting the quantity to 0 will attempt to cancel or remove the line item. |
caseSize |
BigDecimal(10,2) |
The case size of the item for this particular shipment. |
|
uins |
Collection<String> |
The UINs associated to the item quantities. The number of UINS must match the quantity shipped. |
Figure 4-25 Example Input
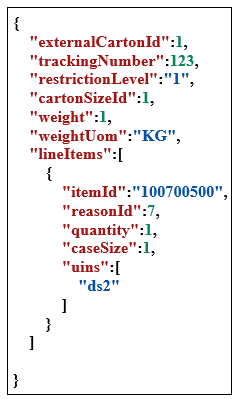
Index
Table 4-225 Vendor Shipment Status
ID |
Description |
1 |
New |
2 |
In Progress |
3 |
Submitted |
4 |
Shipped |
5 |
Canceled |
Table 4-226 Vendor Shipment CriteriaStatus
ID |
Description |
1 |
New |
2 |
In Progress |
3 |
Submitted |
4 |
Shipped |
5 |
Canceled |
99 |
Active |
Table 4-227 Vendor Shipment Carton Status
ID |
Description |
1 |
New |
2 |
In Progress |
3 |
Submitted |
4 |
Shipped |
5 |
Canceled |
Table 4-228 Shipment Carrier Role
ID |
Description |
1 |
Sender |
2 |
Receiver |
3 |
Third Party |
Table 4-229 Merchandise Hierarchy Restriction Level
ID |
Description |
1 |
Department |
2 |
Class |
3 |
Subclass |
4 |
None |
Table 4-230 Fiscal Document Status
ID |
Description |
1 |
Approved |
2 |
Submitted |
3 |
Rejected |
4 |
Canceled |
Table 4-231 Address Type
Type |
Description |
1 |
Business |
2 |
Postal |
3 |
Return |
4 |
Order |
5 |
Invoice |
6 |
Remittance |
7 |
Billing |
8 |
Delivery |
9 |
External |
REST Service: Warehouse
This service integrates warehouse and warehouse item foundation data as well as warehouse item inventory adjustments.
Asynchronous warehouse integration is processed through staged messages and is controlled by the MPS Work Type: DcsWarehouse.
Service Base URL
The Cloud service base URL follows the format:
https://<external_load_balancer>/<cust_env>/siocs-int-services/api/warehouses
API Definitions
API |
Description |
Import Warehouses |
Imports a collection of warehouses into the system. |
Delete Warehouse |
Deletes a warehouse from the system. |
Import Items |
Imports a collection of warehouse items. |
Delete Items |
Deletes warehouse items from the system. |
Import Adjustments |
Imports a collection of warehouse items adjustments that took place. |
Import Inventory |
Imports a collection of warehouse item inventory to update the inventory positions. |
API: Import Warehouses
This will import warehouses through foundation warehouse processing.
If more than 500 warehouses are sent in a single call, an input too large error will be returned.
API Basics
Endpoint URL |
{base URL}/import |
|||
Method |
POST |
|||
Successful Response |
202 Accepted |
|||
Processing Type |
Asynchronous |
|||
Input |
List of warehouses to import |
|||
Output |
None |
|||
Max Response Limit |
500 |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
warehouses |
List of details |
Yes |
A list of warehouses to import. |
Detail Data Definition
Attribute |
Data Type |
Required |
Size |
Description |
warehouseId |
Long (10) |
Yes |
The warehouse identifier. |
|
name |
String (150) |
Yes |
150 |
The name of the warehouse. |
organizationUnit |
String (15) |
15 |
The organization the warehouse belongs to. |
|
countryCode |
String (3) |
3 |
The ISO country code of the warehouse. |
|
currencyCode |
String (40) |
3 |
The ISO currency code of the warehouse. |
Example Output
{
"warehouses": [
{
"warehouseId": 64,
"name": "DownTownWarehouse-1",
"organizationUnit": "70001",
"countryCode": "IN",
"currencyCode": "INR"
},
{
"warehouseId": 65,
"name": "CitynWarehouse-1",
"organizationUnit": "70001",
"countryCode": "IN",
"currencyCode": "INR"
}
]
}
]
API: Delete Warehouse
Deletes a warehouse. The warehouse will not be deleted if any items remain ranged to the warehouse.
API Basics
Endpoint URL |
{base URL}{warehouseId}/delete |
|||
Method |
POST |
|||
Successful Response |
202 Accepted |
|||
Processing Type |
Synchronous |
|||
Input |
None |
|||
Output |
None |
|||
Max Response Limit |
N/A |
Path Parameter Definitions
Attribute |
Definition |
warehouseId |
The internal identifier of the warehouse. |
API: Import Items
API Basics
Imports a collection of warehouse items.
If more than 5000 items are sent in a single call, an input too large error will be returned.
Endpoint URL |
{base URL}/{warehouseId}/items/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous (High Volume) |
|
Input |
A list of warehouse items to import |
|
Output |
None |
|
Max Response Limit |
5000 |
Path Parameter Definitions
Attribute |
Definition |
warehouseId |
The internal identifier of the warehouse. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
|
items |
List of details |
Yes |
A list of warehouse items to import. |
Detail Import Data Definition
Attribute |
Data Type |
Required |
Description |
|
itemId |
String (25) |
Yes |
The item identifier. |
|
standardUom |
String (4) |
Yes |
The standard unit of measure of the item. |
|
status |
Integer |
Yes |
The status (See Index: Warehouse Item Import Status) |
|
clearInventory |
Boolean |
Yes |
True indicates that the inventory positions should all be set to zero. |
Example Input
{
"items": [
{
"itemId": "100000147",
"standardUom": "EA",
"status": 1,
"clearInventory": true
},
{
"itemId": "100000148",
"standardUom": "KG",
"status": 2,
"clearInventory": false
}
]
}
Additional Data Definitions
Warehouse Import Item Status
Value |
Definition |
1 |
ACTIVE |
2 |
DISCONTINUED |
3 |
INACTIVE |
API: Delete Items
Marks warehouse items for later deletion.
If more than 5000 items are sent in a single call, an input too large error will be returned.
API Basics
Endpoint URL |
{base URL}{warehouseId}/delete |
|||
Method |
POST |
|||
Successful Response |
202 Accepted |
|||
Processing Type |
Asynchronous |
|||
Input |
List of item ids to delete |
|||
Output |
None |
|||
Max Response Limit |
5000 |
Path Parameter Definitions
Attribute |
Definition |
warehouseId |
The internal identifier of the warehouse. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
items |
List<String(25)> |
Yes |
A collection of up to 5000 items to remove. |
Example Input
{
"itemIds": [ "100000301", "100000147" ]
}
API: Import Adjustments
API: Import Adjustments
A list of warehouse adjustments is processed, inventory is updated for the warehouse items, and then the adjustments are discarded.
They are not persisted anywhere and this process does not produce a transaction history record.
If more than 5000 items are sent in a single call, an input too large error will be returned.
API Basics
Endpoint URL |
{base URL} {warehouseId}/adjustments/import |
|
Method |
POST |
|
Successful Response |
202 Accepted |
|
Processing Type |
Asynchronous (High Volume) |
|
Input |
A list of warehouse adjustments to import |
|
Output |
None |
|
Max Response Limit |
5000 |
Path Parameter Definitions
Attribute |
Definition |
warehouseId |
The internal identifier of the warehouse. |
Input Data Definition
Attribute |
Data Type |
Required |
Description |
|
adjustments |
List of details |
Yes |
A list of adjustments that occurred for that warehouse. |
Detail Import Data Definition
Attribute |
Data Type |
Required |
Description |
|
itemId |
String (25) |
Yes |
The unique item identifier. |
|
quantity |
BigDecimal |
Yes |
The quantity to be adjusted. |
|
reasonCode |
Integer |
Yes |
The unique reason code of an inventory adjustment reason code. |
Example Input
{
"adjustments": [
{
"itemId": "100000147",
"quantity": 100,
"reasonCode": 182
},
{
"itemId": "100000024",
"quantity": 50,
"reasonCode": 183
}
]
}
API: Import Inventory
This operation updates the inventory positions of a warehouse.
API Basics
Endpoint URL |
{base URL}/{warehouseId}/inventory/import |
Method |
POST |
Successful Response |
200 Accepted |
Processing Type |
Asynchronous |
Input |
List of items with their inventory |
Max Input |
10,000 items |
Output |
N/A |
Max Response Limit |
N/A |
Input Data Definition
Attribute |
Type |
Definition |
Items |
List of Warehouse Inventory |
A list of items to overwrite inventory for at the warehouse. |
Warehouse Inventory Ido
Attribute |
Type |
Definition |
itemId |
String(25) |
The unique item identifier. |
quantityTotal |
BigDecimal(12,4) |
The total quantity of the item in inventory. |
quantityReserved |
BigDecimal(12,4) |
he quantity reserved for outgoing shipping. |
quantityUnavailable |
BigDecimal(12,4) |
The quantity unavailable for usage. |
quantityInTransit |
BigDecimal(12,4) |
The quantity in transit to the warehouse. |
Example Input
{
"items
{
"itemId": "100000147",
"quantityTotal”: 100,
"quantityReserved”: 8
},
{
"itemId": "100000024",
"quantityTotal”: 50,
"quantityInTransit”: 183
}
]
}