Develop the Chatbot Skill
Use open questions combined with an active listening approach to create an intelligent conversation with the bot user.
You use Intents to determine what a user wants to do. For a single-purpose skill, it's possible to not define any Intents. In that case, the flow starts with a state that takes care of some initial preparations and introductions. It then transitions directly to the state that starts the process of getting information from the user.
You can use Custom Components to handle calculations and processes that are difficult or impossible to do within the dialog flow.
Create Intelligent Intents
Intents help the bot determine what the user wants to do.
Each Intent has a list of sample utterances that you provide. You create the sample utterances and then train the bot. The bot uses NLP to create a machine learning model based on the utterances that you created. When a user enters their free-form text, the bot uses this model to interpret the input and match it with one or more Intents. In your BotML, you define which state the flow should go to depending on which Intent matches the user's utterance.
You can also design your bot to have a single intent. In this case, you don't need to create any Intents. Instead, the dialog flow goes directly to the first state. The first state should handle preliminary set up and housekeeping before making the transition to the state that asks the first open question. The sample chatbot skill uses this approach.
Design Effective Entities
Use Entities to extract specific pieces of information from user input.
You can use a custom Entity of type Composite Bag to hold a variety of specialized Entities. The Entities might be specialized for detecting color, brand names, and the like.
For example, a chatbot skill that specializes in helping users find a used car might have a Composite Bag called UsedCar. The Composite Bag could contain many Entities. The overall goal would be to extract information such as color, brand, age, price range, and other relevant data.
In the dialog flow, you would use the System.Text component to display a prompt and assign the user response to a variable. The variable type is string. Then use the System.MatchEntity component to detect which Entities have values and which do not.
The following snippet demonstrates the technique.
context:
variables:
used_car: "string"
car_info: "CarInfo" # Composite Bag Entity
states:
getInput:
component: "System.Text"
properties:
prompt: "Tell me a bit about the car that you're looking for."
variable: "used_car"
transitions: {}
matchInput:
component: "System.MatchEntity"
properties:
sourceVariable: "used_car"
variable: "car_info"
transitions:
actions:
match: "startCarHooks"
nomatch: "checkRetry"
The user enters some free-form text that describes the car that they're looking for. That input is assigned to the used_car variable. The system then examines the variable's content and picks out the Entities that are inside the Composite Bag.
In the preceding example, if one or more Entities match the user response, the startCarHooks state is called. The startCarHooks state initiates a series of states that you can use to demonstrate active listening, based on the Entity values in the Composite Bag. For example, if the user states that they're looking for an electric car but they don't say anything else, the bot might provide the following response: "An electric car is a good choice these days. Can you provide more detail about the kind of electric car you want?"
If no Entities match, the checkRetry state is called. The checkRetryState initiates a series of states that obtain the missing data from the user.
The sample code uses a more sophisticated version of the technique.
Create Custom Components
Use the Oracle Bots Node SDK to create custom compnonents.
Use a custom component in the following cases:
- You want to connect to a backend system such as a database.
- You need to do complicated computations that are difficult and time consuming to develop and test using Freemarker Template Language in the dialog flow.
- You don't want to learn Freemarker Template Language.
The custom component code can be in one of three places, as shown in the following diagram.
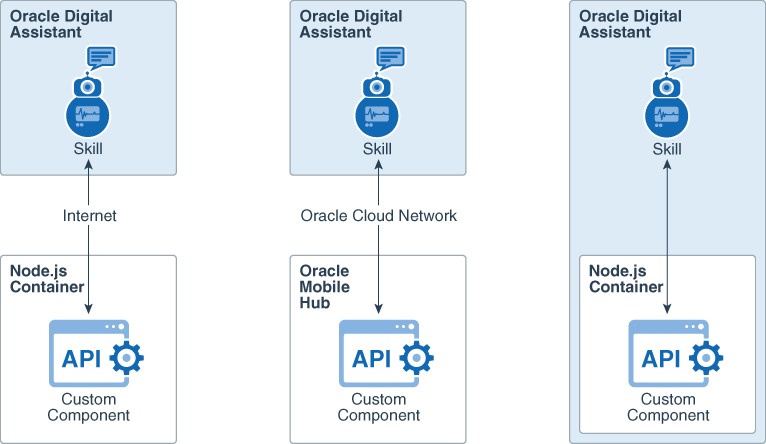
Description of the illustration bot_hosting_options.png
The first option shows the Node.js container that's hosted on a third-party service. Communications between the skill bot and the custom component take place over the public Internet. Third-party services include Oracle Cloud Infrastructure and your own infrastructure.
The second option shows the Node.js container that's hosted on Oracle Mobile Hub. Communications between the skill and the custom component take place over the Oracle Cloud Network, within and between data centers.
The third option shows the Node.js container that's embedded in the Oracle Digital Assistant instance.
Develop the Dialog Flow
Design the dialog flow to ensure that the information that you need is collected from the user.
You can do this by examining the response to an open question. If the response is not adequate, rephrase the question and try again.
You should also think about the user journey. That is, think about their experience as they interact with the bot. For a used car sales skill, your user journey might look like the following outline:
- Greeting/onboarding. Introduce the skill bot's persona and explain a bit about the process.
- Prompt for vehicle wishes. For example "What kind of car would you like to drive home today?"
- If the response is ambiguous or incomplete, repeat the prompt, but use a different phrasing. For example, "An electric car is a good choice these days. Do you have any other preferences, such as brand, color, or range?"
- Show interest in the person and reflect parts of their response back to them to show that the bot is listening. For example ask "What will you be using the vehicle for?" Then if the response is something like "drive to work" then reply "I hope your commute isn't too long, but I'm sure we can find something comfortable for you."
- Prompt for additional information such as price range or method of payment, and respond accordingly.
- Display a list of vehicles that fit the criteria and end with a call to action. For example, "Here are some cars that fit your criteria. Select one or more to arrange a test drive."
You can examine the sample code to understand how to implement active listening aspects of the outline into the BotML code.