About Configuring a CI/CD Pipeline
First, get your API ready. You should at least define its endpoints and download the scaffold that OMC generates for you. The scaffold represents a minimal application that you can use to make sure that your CI/CD process is working correctly before going ahead and writing and testing the code for your custom API.
When your API is ready and you can successfully push changes to the Git repository in your Developer Cloud Service project, it's time to set up CI/CD for the project.
Set Up the API in Oracle Mobile Hub
When defining the HTTP methods for your endpoints, you have the option of adding sample responses. Strictly speaking, the sample responses aren't required because you'll be writing code later that provides the real responses. However, you might want to enter some sample responses for testing the API before your custom code is ready.
Set Up the Backend
Create the backend that your API will use. The backend routes requests from your apps to the API, and provides authentication services.
Record Backend and Instance Details Information
Record information that you'll need when you configure the development tooling.
The information is used in a file called toolsConfig.json
, and is required for authentication. It allows a script to connect to Oracle Mobile Hub and deliver code artifacts to it.
Set Up a Project in Oracle Developer Cloud Service
Create a project that includes a Git repository. Later, you'll configure the project to run a build whenever you push a commit to the repository.
Create a Project
Create a project that includes a Git repository.
- Log in to the Oracle Developer Cloud Service console, and click New Project.
- On the Project Details page, enter a name for your project and click Next.
- On the Template page, select Initial Repository and click Next.
- On the Project Properties page, accept the default values and click Finish to start the provisioning process.
After the project is provisoned, your screen should be similar to the following image:
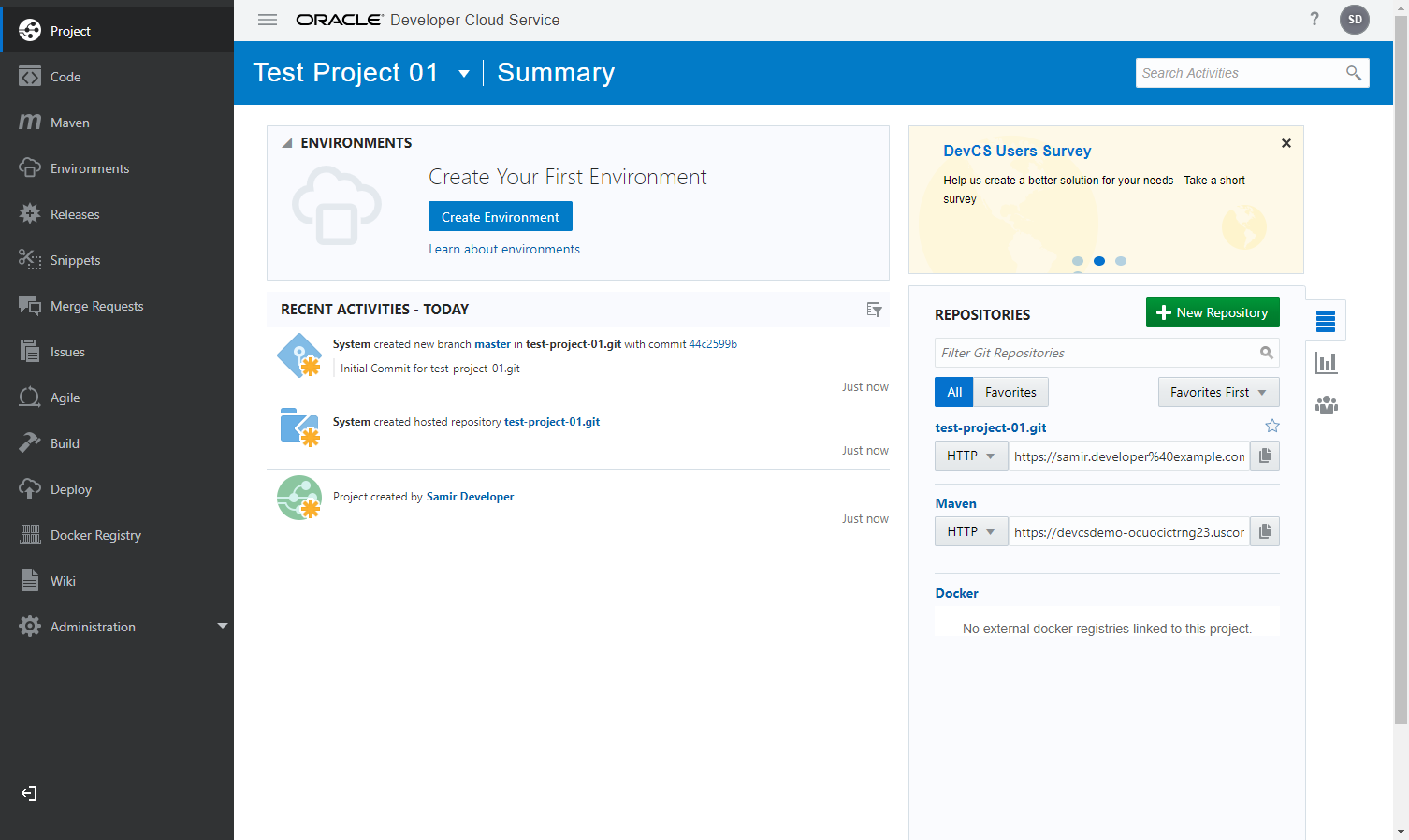
Create a New Build Job
Create a job that will run whenever you push code to the project's Git repository.
Configure the SCM Polling Trigger
You can set up a poll that checks for changes to the Git repository on a schedule that you define. If changes are detected, a build is started.
If you're the only developer who pushes code to the repository, then an immediate build is probably appropriate. However, when several people will be pushing code, you can set up a polling trigger that periodically checks for changes to the repository before starting a build.
To set up the SCM polling trigger, you use the crontab format to set a polling schedule. If you're not familiar with the crontab format, you can use the graphical interface to create the schedule.
The default schedule is every 30 minutes, represented by 0/30 * * * *
. To set the schedule to run at 30 minutes past the hour every hour, use 30 * * * *
.
Set Up the Build Parameters
Set up a string parameter and a password parameter to hold the user name and password for your Oracle Mobile Hub account.
Keep track of the names of these parameters because you'll need them later when you configure the developer tooling.
Configure the Unix Shell Builder
The shell builder is a Bash script that runs on the build system whenever a build is triggered. It installs dependencies then delivers your code to Oracle Mobile Hub.
The script runs against the source from the Git repository that you set up when you created the project in Oracle Developer Cloud Service. Note that if the script fails at any point, it exits with an error and no code is deployed.
Download the JavaScript Scaffold
After you've created the API skeleton, the system creates a scaffold for the API code which you can download in a zip file.
The scaffold contains the initial structure and files for the implementation of the API, which you'll use as a starting point for your customizations. You need to extract the files and place them in your working directory.
- In Oracle Mobile Hub, open the API that you want the scaffold for.
- In the API navigation panel, click Implementation.
- Click JavaScript Scaffold, which downloads the scaffold file to your computer.
- Extract
package.json
,toolsConfig.json
, and the.js
file for your API to your working directory. It's the same directory that was created when you cloned the Git repository, and contains the.git
directory.
Install and Configure Development Tooling
The tools are part of a download that also contains an Oracle Mobile Hub API that you can use for testing and debugging your custom API implementations on your development system. The API is not required for using the CI/CD pipeline. See the readme inside the omce-tools
directory for instructions on using the API, which is called OracleMobileAPI.
The tools support two versions of Node.js: 6.10.0 and 8.9.x. In this procedure it's assumed that you're using version 8.9.4 or greater.
To install and configure the tooling:
- Go to https://www.oracle.com/technetwork/topics/cloud/downloads/amce-downloads-4478270.html and download Custom Code Test Tools version 18.3.1 or later. Note that the file names on this page still follow the legacy product names, but the code that they contain is up to date with the latest release. Similarly for the names of the tools inside the download.
- Open the file that you downloaded and extract the contents of the
omce-tools/omce-tools
directory to your working directory, the one that has your.git
directory in it. For example, if your working directory istest-project
, then extract the contents ofomce-tools/omce-tools
totest-project/omce-tools
. - Install the Node configuration: Change to the
omce-tools/node-configurations/8.9
directory and runnpm install
. - Install omce-tools on your system: Change to the
omce-tools
directory and runnpm install -g
. - Create an environment variable called NODE_PATH and set it to
node-configurations/8.9/node_modules
. - Make sure the tools are installed correctly. In the
omce-tools
directory, runnode omce-test --version
. The result should be 18.3.1. - Update
toolsConfig.json
. When you downloaded the scaffold, it included thetoolsConfig.json
configuration file. Use the information that you recorded from the backend to replace the placeholder text intoolsConfig.json
.