4 Working with Event Handlers
This topic describes event handlers in JavaFX applications. Learn how event handlers can be used to process the events generated by keyboard actions, mouse actions, scroll actions, and other user interactions with your application.
Event handlers enable you to handle events during the event bubbling phase. A node can have one or more handlers for handling an event. A single handler can be used for more than one node and more than one event type. If an event handler for a child node does not consume the event, an event handler for a parent node enables the parent node to act on the event after a child node processes it and to provide common event processing for multiple child nodes.
Registering and Removing an Event Handler
To process an event during the event bubbling phase, a node must register an event handler. An event handler is an implementation of the EventHandler
interface. The handle()
method of this interface provides the code that is executed when the event that is associated with the handler is received by the node that registered the handler.
To register a handler, use the addEventHandler()
method. This method takes the event type and the handler as arguments. In Example 4-1, the first handler is added to a single node and processes a specific event type. A second handler for handling input events is defined and registered by two different nodes. The same handler is also registered for two different types of events.
Example 4-1 Register a Handler
// Register an event handler for a single node and a specific event type node.addEventHandler(DragEvent.DRAG_ENTERED, new EventHandler<DragEvent>() { public void handle(DragEvent) { ... }; }); // Define an event handler EventHandler handler = new EventHandler(<InputEvent>() { public void handle(InputEvent event) { System.out.println("Handling event " + event.getEventType()); event.consume(); } // Register the same handler for two different nodes myNode1.addEventHandler(DragEvent.DRAG_EXITED, handler); myNode2.addEventHandler(DragEvent.DRAG_EXITED, handler); // Register the handler for another event type myNode1.addEventHandler(MouseEvent.MOUSE_DRAGGED, handler);
Note that an event handler that is defined for one type of event can also be used for any subtypes of that event. See Event Types for information on the hierarchy of event types.
When you no longer want an event handler to process events for a node or for an event type, remove the handler using the removeEventHandler()
method. This method takes the event type and the handler as arguments. In Example 4-2, the handler defined in Example 4-1 is removed from the DragEvent.DRAG_EXITED
event for myNode1
. The handler is still executed by myNode2
and by myNode1
for the MouseEvent.MOUSE_DRAGGED
event.
Example 4-2 Remove a Handler
// Remove an event handler myNode1.removeEventHandler(DragEvent.DRAG_EXITED, handler);
Tip:
To remove an event handler that was registered by a convenience method, pass null to the convenience method, for example, node1.setOnMouseDragged(null)
.
Using Event Handlers
Event handlers are typically used on a the leaf nodes or on a branch node of the event dispatch chain and are called during the event bubbling phase of event handling. Use a handler on a branch node to perform actions such as defining a default response for all child nodes.
To see an example of how handlers can be used, download the KeyboardExample.zip
file. Extract the NetBeans project and open it in the NetBeans IDE. The following sections describe the handlers that are used by this example.
Keyboard Example
The Keyboard example demonstrates the following uses of handlers:
-
Registering a single handler for two different event types
-
Providing common event processing for child nodes in a parent node
Figure 4-1 is the screen that is shown when the Keyboard Example is started. The user interface consists of four letters, each in its own square, which represent the corresponding keyboard key. The first key on the screen is highlighted, which indicates that it has the focus. Use the left and right arrow keys on the keyboard to move the focus to a different key on the screen.
Figure 4-1 Initial Screen for Keyboard Example
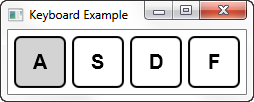
Description of "Figure 4-1 Initial Screen for Keyboard Example"
When the Enter key is pressed, the key on the screen with the focus changes to red. When the Enter key is released, the key on the screen returns to its previous color. When the key for a letter that matches one of the keys on the screen is pressed, the matching key on the screen changes to red, and returns to its previous color when the key is released. When a key that does not match any key on the screen is pressed, nothing happens. Figure 4-2 shows the screen when the A key has focus and the D key on the keyboard is pressed.
Handlers for the Keyboard Example
In the Keyboard example, internally each key shown on the screen is represented by a key node. All key nodes are contained in a single keyboard node. Each key node has a handler that receives key events when the key has focus. The handler responds to the key-pressed and key-released events for the Enter key by changing the color of the key on the screen. The event is then consumed so that the keyboard node, which is the parent node, does not receive the event.
Example 4-3 shows the installEventHandler()
method that defines the handler for the key nodes.
Example 4-3 Handler for the Key Nodes
private void installEventHandler(final Node keyNode) { // handler for enter key press / release events, other keys are // handled by the parent (keyboard) node handler final EventHandler<KeyEvent> keyEventHandler = new EventHandler<KeyEvent>() { public void handle(final KeyEvent keyEvent) { if (keyEvent.getCode() == KeyCode.ENTER) { setPressed(keyEvent.getEventType() == KeyEvent.KEY_PRESSED); keyEvent.consume(); } } }; keyNode.setOnKeyPressed(keyEventHandler); keyNode.setOnKeyReleased(keyEventHandler); }
The keyboard node has two handlers that handle key events that are not consumed by a key node handler. The first handler changes the color of the key node that matches the key pressed. The second handler responds to the left and right arrow keys and moves the focus.
Example 4-4 shows the installEventHandler()
method that defines the handlers for the keyboard node.
Example 4-4 Handlers for the Keyboard Node
private void installEventHandler(final Parent keyboardNode) { // handler for key pressed / released events not handled by // key nodes final EventHandler<KeyEvent> keyEventHandler = new EventHandler<KeyEvent>() { public void handle(final KeyEvent keyEvent) { final Key key = lookupKey(keyEvent.getCode()); if (key != null) { key.setPressed(keyEvent.getEventType() == KeyEvent.KEY_PRESSED); keyEvent.consume(); } } }; keyboardNode.setOnKeyPressed(keyEventHandler); keyboardNode.setOnKeyReleased(keyEventHandler); keyboardNode.addEventHandler(KeyEvent.KEY_PRESSED, new EventHandler<KeyEvent>() { public void handle( final KeyEvent keyEvent) { handleFocusTraversal( keyboardNode, keyEvent); } }); }
The two handlers for the key-pressed event are considered peer handlers. Therefore, even though each handler consumes the event, the other handler is still invoked.