PeopleCode Built-in Functions and Language Constructs: T
The PeopleCode built-In functions and language constructs beginning with the letter T are listed in alphabetical order within this topic.
Syntax
Tan(angle)
Description
Use the Tan function to calculate the tangent of the given angle (opposite / adjacent).
Parameters
Field or Control |
Definition |
---|---|
angle |
A value in radians. |
Note: In theory, values of angle such that angle mod pi
= pi/2 are not valid for this function, because inputs approaching
such values produce results that tend toward infinity. In practice,
however, no computer system can represent such values exactly. Thus,
for example, the statement Tan(Radians(90))
produces a number close to the largest value PeopleCode can represent,
rather than an error.
Returns
A real number.
Example
The following example returns the tangent of an angle measuring 1.2 radians:
&MY_RESULT = Tan(1.2);
Description
Use the Then keyword in an if block. See If for more information.
Syntax
throw expression
Description
Use the throw statement to throw an exception. This can be used to create your own exceptions, instead of using ones generated by the system.
Parameters
Field or Control |
Definition |
---|---|
expression |
Specify the exception object that you want to throw. This can either be an already defined and created exception object, or one that you create with the Throw statement. |
Returns
None.
Example
Local Exception &ex;
Function t1(&i As integer) Returns number
Local number &res = &i / 0;
End-Function;
Function t2
throw CreateException(2, 160, "'%1' doesn't support property or method '%2'", "SomeClass", "SomeMethod");
End-Function;
try
/* This will cause a divide by 0 leading to an exception */
/* This code will never be caught since t1(2) will resume execution */
/* in the catch block below. It is here to show how an exception can */
/* be thrown directly bythe PeopleCode itself. */
t2();
Local number &res = t1(2);
catch Exception &caught
MessageBox(0, "", 0, 0, "Caught exception: " | &caught.ToString());
end-try;
Syntax
Time(n)
Description
Use the Time function to derive a Time value from a Number value. Use it to assign values to Time fields and variables, since Time values cannot be directly represented as constants.
Parameters
Field or Control |
Definition |
---|---|
n |
A Number in the form HHMMSS[.SSSSSS], representing a time to a precision of up to .000001 second, based on a 24-hour clock. |
Returns
Returns a Time value based on the number n.
Example
The example sets &START_TIME to 12:34:56.123456:
&START_TIME = Time(123456.123456);
Syntax
Time3(hours, mins, secs)
Description
Use the Time3 function to derive a Time value from three supplied numbers. It can be used to assign values to Time fields and variables, since Time values cannot be directly represented as constants.
Parameters
Field or Control |
Definition |
---|---|
hours |
A Number in the form HH between 00 and 23, representing hours on a 24-hour clock. |
mins |
A Number in the form MM between 00 and 59, representing minutes of the hour. |
secs |
A Number in the form SS[.SSSSSS], between 00 and 59.999999, representing seconds. |
Returns
Returns a Time value based equal to the sum of the three input values representing hours, minutes, and seconds, to a precision of .000001 second.
Example
The example sets &START_TIME to 11.14.09.300000:
&START_TIME = Time3(11,14,9.3);
Syntax
TimePart(datetime_val)
Description
Use the TimePart function to derive the time component of a DateTime value.
Parameters
Field or Control |
Definition |
---|---|
datetime_val |
A DateTime value from which to extract the time component. |
Returns
Returns a Time value.
Example
The example set &T to 15.34.35.000000:
&DT = DateTimeValue("12/13/1993 3:34:35 PM");
&T = TimePart(&DT);
Syntax
TimeToTimeZone(OldTime, SourceTimeZone, DestinationTimeZone)
Description
Use the TimeToTimeZone function to convert a time field from the time specified by SourceTimeZone to the time specified by DestinationTimeZone.
Considerations Using this Function
This function should generally be used in PeopleCode, not for displaying time. If you take a time value, convert it from base time to client time, then try to display this time, depending on the user settings, when the time is displayed the system might try to do a second conversion on an already converted time. This function could be used as follows: suppose a user wanted to check to make sure a time was in a range of times on a certain day, in a certain timezone. If the times were between 12 AM and 12PM in EST, these resolve to 9 PM and 9AM PST, respectively. The start value is after the end value, which makes it difficult to make a comparison. This function could be used to do the conversion for the comparison, in temporary fields, and not displayed at all.
Parameters
Field or Control |
Definition |
---|---|
OldTime |
Specify the time value to be converted. |
SourceTimeZone |
Specify the time zone that OldTime is in. Values are: timezone - a time zone abbreviation or a field reference to be used for converting OldTime. Local - use the local time zone for converting OldTime. Base - use the base time zone for converting OldTime. |
DestinationTimeZone |
Specify the time zone that you want to convert OldTime to. Values are: timezone - a time zone abbreviation or a field reference to be used for converting OldTime. Local - use the local time zone for converting OldTime. Base - use the base time zone for converting OldTime. |
Returns
A converted time value.
Example
The following example TESTTM is a time field with a value 01/01/99 10:00:00. This example converts TESTTM from Eastern Standard Time (EST) to Pacific Standard Time (PST).
&NEWTIME = TimeToTimeZone(TESTTM, "EST", "PST");
&NEWTIME is a time variable with a value of 7:00:00AM.
Syntax
TimeValue(time_str)
Description
Use the TimeValue function to calculate a Time value based on an input string. This function can be used to assign a value to a Time variable or field using a string constant, since a Time value cannot be represented with a constant.
Parameters
Field or Control |
Definition |
---|---|
time_str |
A string representing the time. It can either be in the form HH:MM:SS.SSSSSS, based on a 24-hour clock, or in the form HH:MM:SS indicator, where indicatoris either AM or PM. |
Returns
Returns a Time value based on time_str.
Example
The example sets &START_TIME to 12.13.00.000000:
&START_TIME = TimeValue("12:13:00 PM");
Syntax
TimeZoneOffset(DateTime {[, timezone | "Base" | "Local"]})
Description
Use the TimeZoneOffset function to generate a time offset for datetime. The offset represents the relative time difference to GMT. If no other parameters are specified with datetime, the server's base time zone is used.
Parameters
Field or Control |
Definition |
---|---|
datetime |
Specify the DateTime value to be used for generating the offset. |
timezone | Local | Base |
Specify a value to be used with datetime. The values are: timezone - a time zone abbreviation or a field reference to be used with datetime. Local - use the local time zone with datetime. Base - use the base time zone with datetime. |
Returns
An offset string of the following format:
Shh:mm
where
Field or Control |
Definition |
---|---|
S |
is + or -, with + meaning East of Greenwich |
hh |
is the hours of offset |
mm |
is the minutes of offset |
Description
Use the To keyword in a for loop. See For for more information.
Syntax
TotalRowCount(scrollpath)
Where scrollpath is:
[RECORD.level1_recname, level1_row, [RECORD.level2_recname, level2_row, ] RECORD.target_recname
To prevent ambiguous references, you can use SCROLL. scrollname, where scrollname is the same as the scroll level’s primary record name.
Description
Use the TotalRowCount function to calculate the number of rows (including rows marked as deleted) in a specified scroll area of a page.
Note: This function remains for backward compatibility only. Use the RowCount rowset property instead.
Rows that have been marked as deleted remain accessible to PeopleCode until the database has been updated; that is, all the way through SavePostChange.
TotalRowCount is used to calculate the upper limit of a For loop if you want the loop to go through rows in the scroll that have been marked as deleted. If the logic of the loop does not need to execute on deleted rows, use ActiveRowCount instead.
Parameters
Field or Control |
Definition |
---|---|
scrollpath |
A construction that specifies a scroll level in the component buffer. |
Returns
Returns a Number equal to the total rows (including rows marked as deleted) in the target scroll.
Example
The example uses TotalRowCount to calculate the limiting value on a For loop, which loops through all the rows in the scroll area:
&ROW_COUNT = TotalRowCount(RECORD.BUS_EXPENSE_PER, CurrentRowNumber(1),
RECORD.BUS_EXPENSE_DTL);
for &I = 1 to &ROW_COUNT
/* do something with row &I that has to be done to deleted as well as active rows */
end-for;
Syntax
Transfer(new_instance, MenuName.MENU_NAME, BarName.BAR_NAME, ItemName.MENU_ITEM_NAME, Page.COMPONENT_ITEM_NAME, action [, keylist])
In which keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
Or in which keylist is a list of field references in the form:
&RecordObject1 [, &RecordObject2]. . .
Description
Use the Transfer function to exit the current context and transfer the user to another page. Transfer can either start a new instance of the component processor and transfer to the new page there, or close the old page and transfer to the new one in the same instance of the component processor.
Note: The Transfer function cannot be used with an Internet script or an Application Engine program.
Transfer is more powerful than the simpler TransferPage, which permits a transfer only within the current component in the current instance of the component processor. However, any variables declared as component do not remain defined after using the Transfer function, whether you’re transferring within the same component or not.
You can use Transfer from a secondary page (either with or without using a pop-up menu) only if you’re transferring to a separate instance of a component. You cannot use Transfer from a secondary page if you’re not transferring to a separate instance of a component.
If you provide a valid search key for the new page in the optional keylist, the new page opens directly, using the values provided from keylist as search key values. A valid key means that enough information is provided to uniquely identify a row: not all of the key values need to be provided. If no key is provided, or if the key is invalid, or if not enough information is provided to identify a unique row, the search dialog box displays, enabling the end user to search for a row.
Note: If Force Search Processing is specified in Application Designer for the component, the search dialog box always displays, whether the keylist is provided or not.
If MENU_NAME+BAR_NAME+MENU_ITEM_NAME+COMPONENT_ITEM_NAME is an invalid combination, an error message displays explaining that there were invalid transfer parameters.
In the COMPONENT_ITEM_NAME parameter, make sure to pass the component item name for the page, not the page name.
Image: Determining the component item name
The component item name is specified in the component definition, in the Item Name column on the row corresponding to the specific page, as shown here. In this example, the PERSONAL_DATA page name appears twice: once with an item name of PERSONAL_DATA_1, and once with the item name of PERSONAL_DATA_2.
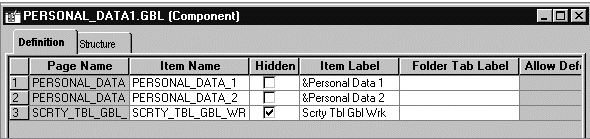
Differences Between Transfer and TransferExact
When you do a transfer, the first thing the system checks is whether all the key field values for the target component are provided.
If all the keys aren't provided, the search page is displayed. In this scenario, TransferExact and Transfer are the same.
If all the keys are provided, a Select is done against the search record for that component using those keys.
If you use the Transfer function, a LIKE operator is used in the Where clause of that Select for each key.
If you use the TransferExact function, the equals operator is used in the Where clause for each key. Using equals allows the database to take full advantage of key indexes for maximum performance.
See TransferExact.
Restrictions on Use With a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use With SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Considerations for the Transfer Function and Catching Exceptions
Using the Transfer function inside a try-catch block does not catch PeopleCode exceptions thrown in the new component. Starting a new component starts a brand new PeopleCode evaluation context. Exceptions are only caught for exceptions thrown within the current component.
In the following code example, the catch statement only catches exceptions thrown in the code prior to the DoModal, but not any exceptions that are thrown within the new component:
/* Set up transaction */
If %CompIntfcName = "" Then
try
&oTrans = &g_ERMS_TransactionCollection.GetTransactionByName(RB_EM_WRK.DESCR);
&sSearchPage = &oTrans.SearchPage;
&sSearchRecord = &oTrans.SearchRecord;
&sSearchTitle = &oTrans.GetSearchPageTitle();
If Not All(&sSearchPage, &sSearchRecord, &sSearchTitle) Then
Error (MsgGetText(17834, 8081, "Message Not Found"));
End-If;
&c_ERMS_SearchTransaction = &oTrans;
/* Attempt to transfer to hidden search page with configurable filter */
&nModalReturn = DoModal(@("Page." | &sSearchPage), &sSearchTitle, - 1, - 1);
catch Exception &e
Error (MsgGetText(17834, 8082, "Message Not Found"));
end-try;
Parameters
Field or Control |
Definition |
---|---|
new_instance |
Set this parameter to True to start a new application instance, or to False to use the current window and replace the page already displayed. |
MENU_NAME |
The name of the menu where the page is located prefixed with the reserved word MenuName. |
BAR_NAME |
The name of the menu bar where the page is located, prefixed with the reserved word BarName. |
MENU_ITEM_NAME |
The name of the menu item where the page is located, prefixed with the reserved word ItemName. |
COMPONENT_ITEM_NAME |
The component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. This parameter must be prefixed with the keyword Page. |
action |
Uses a single-character code as in %Action. Valid actions are "A" (add), "U" (update), "L" (update/display all), "C" (correction), and "E" (data entry). |
[keylist ] |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, or if Force Search Processing is selected for the component, the search dialog box appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
Returns
None.
Example
The example starts a new instance of the component processor and transfers to a new page in Update mode. The data in the new page is selected by matching the EMPLID field from the old page.
Transfer( True, MenuName.ADMINISTER_PERSONNEL, BarName.USE, ItemName.PERSONAL_DATA, Page.PERSONAL_DATA_1, "U");
The following example is used with workflow.
Local Record &WF_WL_DEFN_VW, &MYREC, &PSSTEPDEFN;
If All(WF_WORKLIST_VW.BUSPROCNAME) Then
&BPNAME = FetchValue(WF_WORKLIST_VW.BUSPROCNAME, CurrentRowNumber());
&WLNAME = FetchValue(WF_WORKLIST_VW.WORKLISTNAME, CurrentRowNumber());
&INSTANCEID = FetchValue(WF_WORKLIST_VW.INSTANCEID, CurrentRowNumber());
&WF_WL_DEFN_VW = CreateRecord(RECORD.WF_WL_DEFN_VW);
&PSSTEPDEFN = CreateRecord(RECORD.PSSTEPDEFN);
SQLExec("select %List(SELECT_LIST, :1) from %Table(:1) where Busprocname = :2 and Worklistname = :3", &WF_WL_DEFN_VW, &BPNAME, &WLNAME, &WF_WL_DEFN_VW);
SQLExec("select %List(SELECT_LIST, :1) from %Table(:1) where Activityname = :2 and Stepno = 1 and Pathno = 1", &PSSTEPDEFN, &WF_WL_DEFN_VW.ACTIVITYNAME.Value, &PSSTEPDEFN);
Evaluate &PSSTEPDEFN.DFLTACTION.Value
When = 0
&ACTION = "A";
When = 1
&ACTION = "U";
When-Other
&ACTION = "U";
End-Evaluate;
&MYREC = CreateRecord(@("RECORD." | &WF_WL_DEFN_VW.WLRECNAME.Value));
SQLExec("Select %List(SELECT_LIST, :1) from %Table(:1) where Busprocname = :2 and Worklistname = :3 and Instanceid = :4", &MYREC, &BPNAME, &WLNAME, &INSTANCEID, &MYREC);
Transfer( True, @("MenuName." | &PSSTEPDEFN.MENUNAME.Value), @("BarName." | &PSSTEPDEFN.BARNAME.Value), @("ItemName." | &PSSTEPDEFN.ITEMNAME.Value), @("Page." | &PSSTEPDEFN.PAGEITEMNAME.Value), &ACTION, &MYREC);
End-If;
Syntax
TransferExact(new_instance, MenuName.MENU_NAME, BarName.BAR_NAME, ItemName.MENU_ITEM_NAME, Page.COMPONENT_ITEM_NAME, action [, keylist] [, AutoSearch])
where keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
OR
&RecordObject1 [, &RecordObject2]. . .
Description
Use the TransferExact function to exit the current context and transfer the end user to another page using an exact match of all keys in the optional key list. TransferExact can either start a new instance of the component processor and transfer to the new page there, or close the old page and transfer to the new one in the same instance of the component processor.
Note: The TransferExact function cannot be used with an internet script or an application engine program.
TransferExact is more powerful than the simpler TransferPage, which permits a transfer only within the current component in the current instance of the component processor. However, any variables declared as Component do not remain defined after using the TransferExact function, whether you’re transferring within the same component or not.
You can use TransferExact from a secondary page (either with or without using a pop-up menu) only if you’re transferring to a separate instance of a component. You cannot use TransferExact from a secondary page if you’re not transferring to a separate instance of a component.
If you provide a valid search key for the new page in the optional keylist, the new page opens directly, using the values provided from keylist as search key values. A valid key means that enough information is provided to uniquely identify a row: not all of the key values need to be provided. If no key is provided, or if the key is invalid, or if not enough information is provided to identify a unique row, the search dialog box displays, enabling the end user to search for a row.
Note: If Force Search Processing is specified in Application Designer for the component, the search dialog box always displays, whether the keylist is provided or not.
If MENU_NAME+BAR_NAME+MENU_ITEM_NAME+COMPONENT_ITEM_NAME is an invalid combination, an error message displays explaining that there were invalid transfer parameters.
In the COMPONENT_ITEM_NAME parameter, make sure to pass the component item name for the page, not the page name.
Image: Determining the component item name
The component item name is specified in the component definition, in the Item Name column on the row corresponding to the specific page, as shown here. In this example, the PERSONAL_DATA page name appears twice: once with an item name of PERSONAL_DATA_1, and once with the item name of PERSONAL_DATA_2.
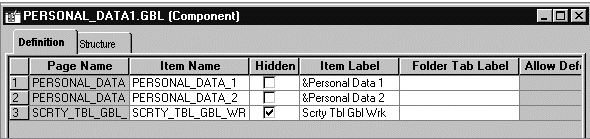
Differences Between Transfer and TransferExact
When you do a transfer, the first thing the system checks is whether all the key field values for the target component are provided.
If all the keys aren't provided, the search page is displayed. In this scenario, TransferExact and Transfer are the same.
If all the keys are provided, a Select is done against the search record for that component using those keys.
If you use the Transfer function, a LIKE operator is used in the Where clause of that Select for each key.
If you use the TransferExact fuction, the equals operator is used in the Where clause for each key. Using equals allows the database to take full advantage of key indexes for maximum performance.
See Transfer.
Restrictions on Use With a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use With SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Considerations Using Exceptions and the TransferExact Function
Using the TransferExact function inside a try block to transfer a user to a page in another component does not catch PeopleCode exceptions thrown in the new component. Starting a new component starts a brand new PeopleCode evaluation context. Catches are only caught for exceptions thrown within the current component.
In the following code example, the catch statement only catches exceptions thrown in the code prior to using the DoModal function, but not any exceptions that are thrown within the new component.
/* Set up transaction */
If %CompIntfcName = "" Then
try
&oTrans = &g_ERMS_TransactionCollection.GetTransactionByName(RB_EM_WRK.DESCR);
&sSearchPage = &oTrans.SearchPage;
&sSearchRecord = &oTrans.SearchRecord;
&sSearchTitle = &oTrans.GetSearchPageTitle();
If Not All(&sSearchPage, &sSearchRecord, &sSearchTitle) Then
Error (MsgGetText(17834, 8081, "Message Not Found"));
End-If;
&c_ERMS_SearchTransaction = &oTrans;
/* Attempt to transfer to hidden search page with configurable filter */
&nModalReturn = DoModal(@("Page." | &sSearchPage), &sSearchTitle, - 1, - 1);
catch Exception &e
Error (MsgGetText(17834, 8082, "Message Not Found"));
end-try;
Parameters
Field or Control |
Definition |
---|---|
new_instance |
Set this parameter to True to start a new application instance, or to False to use the current window and replace the page already displayed. |
MENU_NAME |
The name of the menu where the page is located prefixed with the reserved word MenuName. |
BAR_NAME |
The name of the menu bar where the page is located, prefixed with the reserved word BarName. |
MENU_ITEM_NAME |
The name of the menu item where the page is located, prefixed with the reserved word ItemName. |
COMPONENT_ITEM_NAME |
The component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. This parameter must be prefixed with the keyword Page. |
Action |
Uses a single-character code as in %Action. Valid actions are "A" ( add), "U" (update), "L" (update/display all), "C" (correction), and "E" (data entry). |
Keylist |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, or if Force Search Processing is selected for the component, the search dialog box appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
AutoSearch |
Specify whether an automatic search on the target search page is executed after the transfer. This means the search results are already shown without the end user having to click the Search button. This parameter takes a Boolean value: True, do an automatic search. The default value is False (that is, the user has to click the Search button). |
Returns
None.
Example
The example starts a new instance of the component processor and transfers to a new page in Update mode. The data in the new page is selected by matching the EMPLID field from the old page.
TransferExact(true, MenuName.ADMINISTER_PERSONNEL, BarName.USE, ItemName.PERSONAL_DATA, Page.PERSONAL_DATA_1, "U");
Using the following PeopleCode program:
&MYREC = CreateRecord(RECORD.QEOPC_9A2FIELDS);
&MYREC.QE_TITLE.Value = "KEY";
Transfer(False, MenuName.QE_PEOPLECODE_PAGES, BarName.USE, ItemName.QEPC9PROPSTESTS, Page.QEOPC_9A2FIELDS, "U", &MYREC);
The following SQL is produced:
SELECT DISTINCT TOP 301 QE_TITLE, QEPC_ALTSRCH FROM PS_QEOPC_9A2FIELDS
WHERE QE_TITLE LIKE 'KEY%' ORDER BY QE_TITLE
If you change the Transfer to TransferExact:
&MYREC = CreateRecord(RECORD.QEOPC_9A2FIELDS);
&MYREC.QE_TITLE.Value = "KEY";
TransferExact(False, MenuName.QE_PEOPLECODE_PAGES, BarName.USE, ItemName.QEPC9PROPSTESTS, Page.QEOPC_9A2FIELDS, "U", &MYREC);
The following SQL is produced:
SELECT DISTINCT TOP 301 QE_TITLE, QEPC_ALTSRCH FROM PS_QEOPC_9A2FIELDS
WHERE QE_TITLE=:1 ORDER BY QE_TITLE
Syntax
TransferModeless(MenuName.MENU_NAME, BarName.BAR_NAME, ItemName.MENU_ITEM_NAME, Page.COMPONENT_ITEM_NAME, action [, keylist] [, AutoSearch])
In which keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
Or in which keylist is a list of field references in the form:
&RecordObject1 [, &RecordObject2]. . .
Description
Use the TransferModeless function to open a new page in a modeless window on top of the parent window. Only one modeless window can be opened per browser session. Similar to the new_instance parameter of the Transfer function, TransferModeless instantiates a separate instance of the component processor so that the parent window and secondary window are completely independent PeopleCode contexts.
Note: TransferModeless is currently not supported for transfers from or to fluid components. In addition, TransferModeless function cannot be used with an Internet script or an Application Engine program.
TransferModeless is more powerful than the simpler TransferPage, which permits a transfer only within the current component in the current instance of the component processor. However, any variables declared as component do not remain defined after using the TransferModeless function, whether you’re transferring within the same component or not.
You can use TransferModeless from a secondary page (either with or without using a pop-up menu) only if you’re transferring to a separate instance of a component. You cannot use TransferModeless from a secondary page if you’re not transferring to a separate instance of a component.
If you provide a valid search key for the new page in the optional keylist, the new page opens directly, using the values provided from keylist as search key values. A valid key means that enough information is provided to uniquely identify a row: not all of the key values need to be provided. If no key is provided, or if the key is invalid, or if not enough information is provided to identify a unique row, the search dialog box displays, enabling the end user to search for a row.
Note: If Force Search Processing is specified in Application Designer for the component, the search dialog box always displays, whether the keylist is provided or not.
If MENU_NAME+BAR_NAME+MENU_ITEM_NAME+COMPONENT_ITEM_NAME is an invalid combination, an error message displays explaining that there were invalid transfer parameters.
In the COMPONENT_ITEM_NAME parameter, make sure to pass the component item name for the page, not the page name.
Image: Determining the component item name
The component item name is specified in the component definition, in the Item Name column on the row corresponding to the specific page, as shown here. In this example, the PERSONAL_DATA page name appears twice: once with an item name of PERSONAL_DATA_1, and once with the item name of PERSONAL_DATA_2.
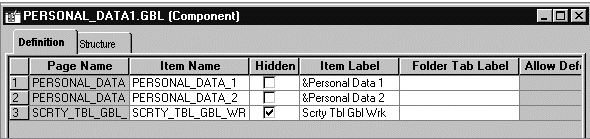
Modeless Windows
In addition to modal secondary windows, you can create a modeless window. A modeless window is different from a modal window launched by any of the DoModal* functions in that its state is separate from that of the parent, launching component. When a modeless window is launched from a classic component using the TransferModeless function, the modeless window does not mask the parent window, which allows the user to update the modeless and parent window from the same browser session at the same time. However, when a modeless window is launched from a fluid component using either the ViewContentURLModeless or ViewURLModeless functions, the parent window is masked and cannot be accessed until the modeless window is dismissed. In this respect and from the user’s perspective, a modeless window launched from a fluid component operates similar to a modal window; but programmatically, its state is separate from that of the parent, launching component.
Note: While the title bar of a modeless window includes an X (or cancel) button, it cannot include any custom buttons.
Important! Only one active child modeless window can be open at one time. Therefore, after opening a modeless child window from the parent, you cannot open a second modeless child window from that modeless window. However, you can open a modal window from that modeless window.
Restrictions on Use With a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use With SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Considerations for the TransferModeless Function and Catching Exceptions
Using the TransferModeless function inside a try-catch block does not catch PeopleCode exceptions thrown in the new component. Starting a new component starts a brand new PeopleCode evaluation context. Exceptions are only caught for exceptions thrown within the current component.
In the following code example, the catch statement only catches exceptions thrown in the code prior to the DoModal, but not any exceptions that are thrown within the new component:
/* Set up transaction */
If %CompIntfcName = "" Then
try
&oTrans = &g_ERMS_TransactionCollection.GetTransactionByName(RB_EM_WRK.DESCR);
&sSearchPage = &oTrans.SearchPage;
&sSearchRecord = &oTrans.SearchRecord;
&sSearchTitle = &oTrans.GetSearchPageTitle();
If Not All(&sSearchPage, &sSearchRecord, &sSearchTitle) Then
Error (MsgGetText(17834, 8081, "Message Not Found"));
End-If;
&c_ERMS_SearchTransaction = &oTrans;
/* Attempt to transfer to hidden search page with configurable filter */
&nModalReturn = DoModal(@("Page." | &sSearchPage), &sSearchTitle, - 1, - 1);
catch Exception &e
Error (MsgGetText(17834, 8082, "Message Not Found"));
end-try;
Parameters
Field or Control |
Definition |
---|---|
MENU_NAME |
The name of the menu where the page is located prefixed with the reserved word MenuName. |
BAR_NAME |
The name of the menu bar where the page is located, prefixed with the reserved word BarName. |
MENU_ITEM_NAME |
The name of the menu item where the page is located, prefixed with the reserved word ItemName. |
COMPONENT_ITEM_NAME |
The component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. This parameter must be prefixed with the keyword Page. |
Action |
Uses a single-character code as in %Action. Valid actions are "A" ( add), "U" (update), "L" (update/display all), "C" (correction), and "E" (data entry). |
Keylist |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, or if Force Search Processing is selected for the component, the search dialog box appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
AutoSearch |
Specify whether an automatic search on the target search page is executed after the transfer. This means the search results are already shown without the end user having to click the Search button. This parameter takes a Boolean value: True, do an automatic search. The default value is False (that is, the user has to click the Search button). |
Returns
None.
Syntax
TransferNode(new_instance, NODE.nodename, MENUNAME.menuname, MARKET.marketname, COMPONENT.componentname, PAGE.component_item_name, action [, keylist])
where keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
OR
&RecordObject1 [, &RecordObject2]. . .
Description
Use the TransferNode function to transfer the user to a page in another Node, but within the same portal.
TransferNode can either start a new instance of the application and transfer to the new page, or close the old page and transfer to the new one in the same instance of the component processor.
Component scoped and Global scoped variables are not available if the new page is in a different node.
Entering null values ("") for the node opens the new component within the current node or portal.
If you want to transfer the end user to another portal, use the TransferPortal function.
If you provide a valid search key for the new page in the optional fieldlist, the new page opens directly, using the values provided from fieldlist as search key values. If no key is provided, or if the key is invalid, the search dialog displays, allowing the end user to search for a row.
Note: If Force Search Processing is specified in Application Designer for the component, the search dialog always displays, whether the keylist is provided or not.
If TransferNode is called in a RowInit PeopleCode program, the PeopleCode program is terminated. However, the component processor continues with its RowInit processing, calling RowInit on the other fields. The actual transfer won't happen until after that completes. You may want to place any TransferPage functions in the Activate event for the page, or later in the Component Processor event flow.
See Understanding Component Definitions.
Restrictions on Use with a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use with SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Considerations Using Exceptions and the TransferNode Function
Using the TransferNode function inside a try blockdoes not catch PeopleCode exceptions thrown in the new component. Starting a new component starts a brand new PeopleCode evaluation context. Catches are only caught for exceptions thrown within the current component.
In the following code example, the catch statement only catches exceptions thrown in the code prior to using the DoModal function, but not any exceptions that are thrown within the new component.
/* Set up transaction */
If %CompIntfcName = "" Then
try
&oTrans = &g_ERMS_TransactionCollection.GetTransactionByName(RB_EM_WRK.DESCR);
&sSearchPage = &oTrans.SearchPage;
&sSearchRecord = &oTrans.SearchRecord;
&sSearchTitle = &oTrans.GetSearchPageTitle();
If Not All(&sSearchPage, &sSearchRecord, &sSearchTitle) Then
Error (MsgGetText(17834, 8081, "Message Not Found"));
End-If;
&c_ERMS_SearchTransaction = &oTrans;
/* Attempt to transfer to hidden search page with configurable filter */
&nModalReturn = DoModal(@("Page." | &sSearchPage), &sSearchTitle, - 1, - 1);
catch Exception &e
Error (MsgGetText(17834, 8082, "Message Not Found"));
end-try;
Parameters
Field or Control |
Definition |
---|---|
new_instance |
Set this parameter to True to start a new application instance, or to False to use the current window and replace the page already displayed. |
nodename |
Specify the name of the node that contains the content, prefixed with the reserved word NODE. You can also use a string, such as %Node, for this value. |
menuname |
Specify the name of the menu containing the content, prefixed with the reserved word MENUNAME. You can also use a string, such as %Menu, for this value. |
marketname |
Specify the name of the market of the component, prefixed with the reserved word MARKET. You can also use a string, such as %Market, for this value. |
component_item_name |
Specify the component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. If you specify a page, it must be prefixed with the keyword PAGE. You can also specify a null ("") for this parameter. |
action |
Specify a single-character code. Valid actions are:
You can also specify a null ("") for this parameter. |
keylist |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, of if Force Search Processing has been selected, the search dialog appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
Returns
A Boolean value: True if function completed successfully, False otherwise.
Syntax
TransferPanel([PANEL.panel_name])
Description
Use the TransferPanel function to transfer control to the panel indicated by PANEL. panel_name_name within, or to the panel set with the SetNextPage function.
Note: The TransferPanel function is supported for compatibility with previous releases of PeopleTools. New applications should use the TransferPage function instead.
Related Links
Syntax
TransferPage([Page.page_name_name])
Description
Use the TransferPage function to transfer control to the page indicated by Page.page__name or to the page set with the SetNextPage function. The page that you transfer to must be in the current component or menu. To transfer to a page outside the current component or menu, or to start a separate instance of the component processor prior to transfer into, use the Transfer function.
Note: If the visibility of the current page is set to False in a PeopleCode program, then you must invoke the TransferPage function to transfer control to a visible page.
See SetNextPage, Transfer.
Note: You can’t use TransferPage from a secondary page.
Any variable declared as a Component variable will still be defined after using a TransferPage function.
Considerations Using TransferPage
The following are important considerations when using the TransferPage function:
TransferPage always terminates the current PeopleCode program.
TransferPage is always processed after all events are completed.
Given these considerations, here are some scenarios for how TransferPage executes:
When called in RowInit: The current RowInit PeopleCode program is terminated, but RowInit processing continues. In addition, RowInit PeopleCode programs run for the rest of the fields in the row. Then TransferPage is processed.
When called in FieldEdit: The FieldEdit PeopleCode program is terminated. The FieldChange program for that field still runs. Then TransferPage is processed.
When called in SavePreChange: The SavePreChange program for that field is terminated. SavePreChange runs for the rest of the fields on that page. Then SavePostChange run for all the fields. Then TransferPage is processed.
When called in FieldChange in deferred mode: In deferred mode, changed fields are processed in order. The FieldChange program is terminated. Then any subsequent fields in the page order are processed with the normal FieldEdit-Field Change logic. Once that has finished, the TransferPage is processed.
When TransferPage is processed, any PeopleCode associated with the Activate event for the page being transferred to runs. This always occurs at the end, after all field processing.
If TransferPage is called multiple times during field processing, all the calls are processed at the end, in the same order the calls were made. The Activate event executes each time. The final active page is the one that was transferred to by the last call.
Restrictions on Use With a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use With SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Parameters
Field or Control |
Definition |
---|---|
page_name |
A String equal to the name of the page you are transferring to, as set in the page definition, prefixed with the reserved word Page. The page must be in the same component as the page you are transferring from. |
Returns
Optionally returns a Boolean value indicating whether the function executed successfully.
Example
The following examples both perform the same function, which is to transfer to the JOB_DATA_4 page:
TransferPage(Page.JOB_DATA_4);
or
SetNextPage(Page.JOB_DATA_4);
TransferPage( );
Syntax
TransferPortal(new_instance, PORTAL.portalname, NODE.nodename, MENUNAME.menuname, MARKET.marketname, COMPONENT.componentname, PAGE.component_item_name, action [, keylist])
where keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
OR
&RecordObject1 [, &RecordObject2]. . .
Description
Use the TransferPortal function to transfer the user to a page in another Node in a different portal.
TransferPortal can either start a new instance of the application and transfer to the new page, or close the old page and transfer to the new one in the same instance of the component processor.
Component scoped and Global scoped variables are not available after this function.
If you want to transfer the end user to another node within the same portal, use the TransferNode function.
If you provide a valid search key for the new page in the optional fieldlist, the new page opens directly, using the values provided from fieldlist as search key values. If no key is provided, or if the key is invalid, the search dialog displays, allowing the end user to search for a row.
Note: If Force Search Processing is specified in Application Designer for the component, the search dialog always displays, whether the keylist is provided or not.
If TransferPortal is called in a RowInit PeopleCode program, the PeopleCode program is terminated. However, the component processor continues with its RowInit processing, calling RowInit on the other fields. The actual transfer won't happen until after that completes. You may want to place any TransferPortal functions in the Activate event for the page, or later in the Component Processor flow.
See Understanding Component Definitions.
Restrictions on Use with a Component Interface
This function is ignored (has no effect) when used by a PeopleCode program that’s been called by a Component Interface.
Restrictions on Use with SearchInit Event
You can't use this function in a SearchInit PeopleCode program.
Restrictions on Use with Different Releases
You cannot use this function to transfer a user from a PeopleTools 8.42 portal to any base PeopleTools 8.1x portal that overwrites the expired cookie value when login occurs.
The TransferPortal function is currently supported to transfer users to pages in other nodes to base PeopleTools 8.18 portals, including all PeopleTools 8.18 versions and patches.
Considerations Using Exceptions and the TransferPortal Function
Using the TransferPortal function inside a try block does not catch PeopleCode exceptions thrown in the new component. Starting a new component starts a brand new PeopleCode evaluation context. Catches are only caught for exceptions thrown within the current component.
In the following code example, the catch statement only catches exceptions thrown in the code prior to using the DoModal function, but not any exceptions that are thrown within the new component.
/* Set up transaction */
If %CompIntfcName = "" Then
try
&oTrans = &g_ERMS_TransactionCollection.GetTransactionByName(RB_EM_WRK.DESCR);
&sSearchPage = &oTrans.SearchPage;
&sSearchRecord = &oTrans.SearchRecord;
&sSearchTitle = &oTrans.GetSearchPageTitle();
If Not All(&sSearchPage, &sSearchRecord, &sSearchTitle) Then
Error (MsgGetText(17834, 8081, "Message Not Found"));
End-If;
&c_ERMS_SearchTransaction = &oTrans;
/* Attempt to transfer to hidden search page with configurable filter */
&nModalReturn = DoModal(@("Page." | &sSearchPage), &sSearchTitle, - 1, - 1);
catch Exception &e
Error (MsgGetText(17834, 8082, "Message Not Found"));
end-try;
Parameters
Field or Control |
Definition |
---|---|
new_instance |
Set this parameter to True to start a new application instance, or to False to use the current window and replace the page already displayed. |
PortalName |
Specify the name of the portal that you want to transfer to, prefixed with the reserved word Portal. |
nodename |
Specify the name of the node that contains the content, prefixed with the reserved word NODE. You can also use a string, such as %Node, for this value. |
menuname |
Specify the name of the menu containing the content, prefixed with the reserved word MENUNAME. You can also use a string, such as %Menu, for this value. |
marketname |
Specify the name of the market of the component, prefixed with the reserved word MARKET. You can also use a string, such as %Market, for this value. |
component_item_name |
Specify the component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. If you specify a page, it must be prefixed with the keyword PAGE. You can also specify a null ("") for this parameter. |
action |
Specify a single-character code. Valid actions are:
You can also specify a null ("") for this parameter. |
keylist |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, of if Force Search Processing has been selected, the search dialog appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
Returns
A Boolean value: True if function completed successfully, False otherwise.
Syntax
TransferTop(new_window, MenuName.MENU_NAME, BarName.BAR_NAME, ItemName.MENU_ITEM_NAME, Page.COMPONENT_ITEM_NAME, action [, keylist])
In which keylist is a list of field references in the form:
[recordname.]field1 [, [recordname.]field2]. . .
Or in which keylist is a list of field references in the form:
&RecordObject1 [, &RecordObject2]. . .
Description
Use the TransferTop function to exit the current context and transfer the user to another page by always replacing the current window. Use the TransferTop function as an alternative to the Transfer function to ensure that when the source component is being displayed within a fluid wrapper (that is, the source component is being displayed within a fluid activity guide, a master/detail component, an interactive grouplet, or a modeless window) that the entire current window is replaced with the new content.
Unlike Transfer, TransferTop ignores the new_window parameter. TransferTop always exits the current context and completely replaces the current window.
You can use TransferTop from a secondary page only if you’re transferring to a separate instance of a component. You cannot use TransferTop from a secondary page if you’re not transferring to a separate instance of a component.
If you provide a valid search key for the new page in the optional keylist, the new page opens directly, using the values provided from keylist as search key values. A valid key means that enough information is provided to uniquely identify a row: not all of the key values need to be provided. If no key is provided, or if the key is invalid, or if not enough information is provided to identify a unique row, the search page could be displayed (depending on the component), enabling the user to search for a row.
If MENU_NAME+BAR_NAME+MENU_ITEM_NAME+COMPONENT_ITEM_NAME is an invalid combination, an error message displays explaining that there were invalid transfer parameters.
In the COMPONENT_ITEM_NAME parameter, make sure to pass the component item name for the page, not the page name.
Image: Determining the component item name
The component item name is specified in the component definition, in the Item Name column on the row corresponding to the specific page, as shown here. In this example, the PERSONAL_DATA page name appears twice: once with an item name of PERSONAL_DATA_1, and once with the item name of PERSONAL_DATA_2.
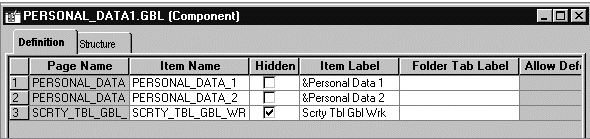
Restrictions on Using TransferTop
TransferTop cannot be used with an Internet script or an Application Engine program.
TransferTop is ignored (has no effect) when used by a PeopleCode program that’s been called by a component interface.
You can't use TransferTop in a SearchInit PeopleCode program.
Parameters
Field or Control |
Definition |
---|---|
new_window |
Note: This parameter is ignored and has no effect. |
MENU_NAME |
The name of the menu where the page is located prefixed with the reserved word MenuName. |
BAR_NAME |
The name of the menu bar where the page is located prefixed with the reserved word BarName. |
MENU_ITEM_NAME |
The name of the menu item where the page is located prefixed with the reserved word ItemName. |
COMPONENT_ITEM_NAME |
The component item name of the page to be displayed on top of the component when it displays. The component item name is specified in the component definition. This parameter must be prefixed with the keyword Page. |
action |
Uses a single-character code as in %Action. Valid actions are "A" (add), "U" (update), "L" (update/display all), "C" (correction), and "E" (data entry). |
[keylist] |
An optional list of field specifications used to select a unique row at level zero in the page you are transferring to, by matching keys in the page you are transferring from. It can also be an already instantiated record object. If a record object is specified, any field of that record object that is also a field of the search record for the destination component is added to keylist. The keys in the fieldlist must uniquely identify a row in the "to" page search record. If a unique row is not identified, or if Force Search Processing is selected for the component, the search dialog box appears. If the keylist parameter is not supplied then the destination component's search key must be found as part of the source components level 0 record buffer. |
Returns
None.
Example
The example starts does not start a new instance of the component processor, but transfers to a new page in Update mode.
TransferTop( False, MenuName.QE_FLUID_CR, BarName.USE, ItemName.QE_CBACK_F1, Page.QE_CBACK_F1, "U");
Syntax
Transform({XmlString | &XmlDoc} AE_Program_Name, Initial_Node_Name, Initial_Message__Name, Initial_Message_Version, Result_Node_Name, Result_Message_Name, Result_Message_Version)
Description
Use the Transform function to modify one transaction, as specified by the Initial parameters, to another transaction, specified by the Result parameters, using an Application Engine program. This is used with Integration Broker.
Generally using this function implies that you're transforming a message that you're not actually sending or receiving at the current time. By using this method, and specifying the two transactions, it's as if you're defining a relationship, without having to use the relationship component.
Note: This function does not work on the OS/390 and z/OS batch servers.
Considerations Using the Transform Functions
The Transform function uses an existing Application Engine program to do transformations. This enables you to break up the flow of Integration Broker and do transformations when you need to. If you wish to reuse your Application Engine programs, you can invoke them by using this function.
The TransformEx function does not use an Application Engine program to do a transformation. Instead, it does an Extensible Stylesheet Language Transformation (XSLT.) This enables you to dynamically do transformations outside of Integration Broker, such as, performing transformations on pagelets in a portal every time a page is accessed.
The TransformExCache function also does XSLT transformations without using an Application Engine program, outside of Integration Broker. Use TransformExCache when you have a large volume of similar transformations to be done. Caching technology is used with this function. You may see an increase in performance, as well as an increase in memory consumption, using this function.
Parameters
Field or Control |
Definition |
---|---|
XmlString | &XmlDoc |
Specify an already populated XmlDoc object, an XML string, or other text that you want transformed. |
AE_Program_Name |
Specify the name of the Application Engine program that you want to use for the transformation. |
Initial_Node_Name |
Specify the name of the initial node as a string. |
Initial_Message_Name |
Specify the name of the initial message. |
Initial_Message_Version |
Specify the version of the initial message that you want to use. |
Result_Node_Name |
Specify the result, where you want the transformed message to go to. |
Result_Message_Name |
Specify the name of the result message, the one to use for the output. |
Result_Message_Version |
Specify the version of the result message to be used. |
Returns
An XmlDoc object containing the resulting XML from the transformation. Null is never returned. If you do not want to display an error to the user, place this function inside a try-catch statement.
Syntax
TransformEx(XmlString, XsltString)
Description
Use the TransformEx function to do an XSLT transformation of the specified XML string.
This function also strips off any encoding information located within the XML Declaration.
The input, output, and XSL string must all be well-formed XML. If the output is HTML, it is actually XHTML (which is well-formed XML.)
Note: This function does not work on the OS/390 and z/OS batch servers.
Considerations Using the Transform Functions
The Transform function uses an existing Application Engine program to do transformations. This enables you to break up the flow of Integration Broker and do transformations when you need to. If you wish to reuse your Application Engine programs, you can invoke them by using this function.
The TransformEx function does not use an Application Engine program to do a transformation. Instead, it does an Extensible Stylesheet Language Transformation (XSLT.) This enables you to dynamically do transformations outside of Integration Broker, such as, performing transformations on pagelets in a portal every time a page is accessed.
The TransformExCache function also does XSLT transformations without using an Application Engine program, outside of Integration Broker. Use TransformExCache when you have a large volume of similar transformations to be done. Caching technology is used with this function. You may see an increase in performance, as well as an increase in memory consumption, using this function.
Parameters
Field or Control |
Definition |
---|---|
XmlString |
Specify the XML string that you want transformed. |
XsltString |
Specify the XSLT string you wish to use to transform the XML string. |
Returns
The output of the transformation as a string if successful, NULL otherwise.
Example
try
&outStr = TransformEx(&inXML, &inXSLT);
catch Exception &E
MessageBox(0, "", 0, 0, "Caught exception: " | &E.ToString());
end-try;
Syntax
TransformExCache(&XmlDoc, FilePath, XsltKey)
Description
Use the TransformExCache function to do an Extensible Stylesheet Language Transformation (XSLT) transformation of the specified XmlDoc object.
The file specified by FilePath must be in well-formed XML.
Note: This function does not work on the OS/390 and z/OS batch servers.
Considerations Using the Transform Functions
The Transform function uses an existing Application Engine program to do transformations. This enables you to break up the flow of Integration Broker and do transformations when you need to. If you wish to reuse your Application Engine programs, you can invoke them by using this function.
The TransformEx function does not use an Application Engine program to do a transformation. Instead, it does an Extensible Stylesheet Language Transformation (XSLT.) This enables you to dynamically do transformations outside of Integration Broker, such as, performing transformations on pagelets in a portal every time a page is accessed.
The TransformExCache function also does XSLT transformations without using an Application Engine program, outside of Integration Broker. Use TransformExCache when you have a large volume of similar transformations to be done. Caching technology is used with this function. You may see an increase in performance, as well as an increase in memory consumption, using this function.
Parameters
Field or Control |
Definition |
---|---|
&XmlDoc |
Specify an already instantiated and populated XmlDoc object that you want transformed. |
FilePath |
Specify an XSLT file. You must specify an absolute path to the file, including the file extension. |
XsltKey |
Specify a key to uniquely name the compiled and cached XSLT in the data buffers. This key is used both to create the item in memory as well as retrieve it. This parameter takes a string value, up to 30 characters. |
Returns
An XmlDoc object containing the resulting XML from the transformation. Null is never returned. If you do not want to display an error to the user, place this function inside a try-catch statement.
Example
Local XmlDoc &inXMLdoc = CreateXmlDoc("");
Local Boolean &ret = &inXMLdoc.ParseXmlFromURL("c:\temp\in.xml");
Local XmlDoc &outDoc = TransformExCache(&inXMLdoc, "c:\temp\in.xsl", "INBOUND");
Syntax
TreeDetailInNode(setID, tree, effdt, detail_value, node)
Description
Use the TreeDetailInNode function to determine whether a specific record field value is a descendant of a specified node in a specified tree.
Note: This function is not compatible with the PeopleSoft Pure Internet Architecture. However, this function is still available for use with the PeopleSoft Tree Manager Windows client, available in the 8.1 product line.
An equivalent PeopleCode tree class method or built-in function for PeopleSoft Pure Internet Architecture does not exist, however, you may achieve this same functionality using the tree classes.
Parameters
Field or Control |
Definition |
---|---|
setID |
SetID for the appropriate business unit. This parameter is required. If there is no SetID, you can pass a NULL string ("", not a blank) and a blank will be used. |
tree |
The tree name that contains the detail_value. |
effdt |
Effective date to be used in the search. You must use a valid date. |
detail_value |
The recordname.fieldname containing the value you are looking for. |
node |
The "owning" tree node name. |
Returns
Returns a Boolean value, True if detail_value is a descendant of node in tree.
Example
This example sets the value of &APPR_RULE_SET to the value at the APPR_RULE_LN record and APPR_RULE_SET fieldname, on the tree ACCOUNT.
&APPR_RULE_SET = TreeDetailInNode("SALES", "ACCOUNT", %Date, APPR_RULE_LN.APPR_RULE_SET, "test");
Syntax
TriggerBusinessEvent(BUSPROCESS.bus_proc_name, BUSACTIVITY.activity_name, BUSEVENT.bus_event_name)
Description
Use the TriggerBusinessEvent funciton to trigger a business event and the workflow routings associated with that event. This function should only be used in Workflow PeopleCode. You can edit Workflow PeopleCode via the Event Definition dialog while you are defining a workflow event.
Parameters
Field or Control |
Definition |
---|---|
bus_proc_name |
A string consisting of the name of the business process, as defined in the Business Process Designer, prefixed with the reserved word BUSPROCESS. |
activity_name |
A string consisting of the name of the business activity, as defined in the Business Process Designer, prefixed with the reserved word BUSACTIVITY. |
bus_event_name |
A string consisting of the name of the business event, as defined in the Business Process Designer, prefixed with the reserved word BUSEVENT. |
Returns
Returns a Boolean value: True if successful, false otherwise. The return value is not optional.
Note: You must check the return from TriggerBusinessEvent to see if you have an error. If you have an error, all of the updates up to that TriggerBusinessEvent process are rolled back. However, if you don’t halt execution, even if you have an error, all updates after the TriggerBusinessEvent process are committed. This could result in your database information being out of synch.
Example
The following example triggers the Deny Purchase Request event in the Manager Approval activity of the Purchase Requisition business process:
&SUCCESS = TriggerBusinessEvent(BUSPROCESS."Purchase Requisition", BUSACTIVITY."Manager Approval", BUSEVENT."Deny Purchase Request");
Syntax
Truncate(dec, digits)
Description
Use the Truncate function to truncate a decimal number dec to a specified precision.
Parameters
Field or Control |
Definition |
---|---|
digits |
A Number value that sets the precision of the truncation (that is, the number of digits to leave on the right side of the decimal point). |
Returns
Returns a Number value equal to dec truncated to a digits precision.
Example
The example sets the value of &NUM to 9, 9.99, -9, then 0.
&NUM = Truncate(9.9999, 0);
&NUM = Truncate(9.9999, 2);
&NUM = Truncate(-9.9999, 0);
&NUM = Truncate(0.001, 0);
Syntax
try Protected StatementList catchQualifiedID &ID StatementList end-try
Description
Use the try statement as part of a try-catch block to trap exceptions thrown either by the system or by using the CreateException function.
Parameters
Field or Control |
Definition |
---|---|
Protected StatementList |
Specify the statements that are protected by the try-catch block. |
catch QualifiedID &ID |
Specify the catch statement at the end of the list of statements you want to protect. |
QualifiedID |
Specify what class of exception you are catching—that is, Exception or the name of a class extending the Exception class. |
&ID |
Specify a variable to be set with the caught exception. |
StatementList |
Specify the steps to be taken once the exception is caught. |
Returns
None.
Example
try
&res = 15.3 / 7 * 22.1;
catch Exception &c1
MessageBox(0, "", 0, 0, "Caught exception: " | &c1.ToString());
end-try;