Specify Functions
Use functions in event actions and conditions to perform common operations with strings, values, and arrays. For example, use a function to add two values, concatenate two strings, or sum items in table rows.
-
You can specify parameters for selected functions. The parameter value can be a constant, data definition value, control value, other function, or connector data value.
-
Some functions support selecting arrays of data or repeatable controls.
-
You can nest functions, such as concatenate multiple strings or the results of multiple rows. You might select a concatenate function and concatenate a data value, connector data value, or control value with another value such as a constant.
Note that the event window where you specify functions has an embedded Help that gives details on each function. Choose a category in the Category column, the Function column on the right displays the related functions of the chosen category. Select a function to view details about that function with examples in the area below.
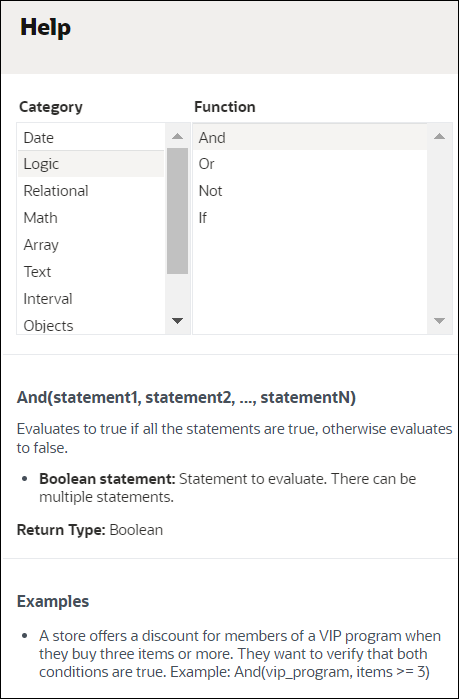
Description of the illustration embedded-help.png
Function Category | Function Name | Parameters | Description |
---|---|---|---|
Date | Current Date | None |
Returns the current date in the format yyyy-mm-dd. For example: 1998-06-12. |
Date | Current Time | None |
Returns the current time in 24-hour time using the format T00:00:00. For example: T21:07:28. |
Date | Current Date Time | None |
Returns the current date and time in the format yyyy-mm-dd T00:00:00. For example: 1977-06-16 T21:07:28. |
Date | Add Seconds | (Date, Number) |
Adds a specified number of seconds to a specified time. AddSeconds(originalTime, secondsToAdd)
|
Date | Add Minutes | (Date, Number) |
Adds a specified number of minutes to a specified time. AddMinutes(originalTime, minutesToAdd)
|
Date | Add Hours | (Date, Number) |
Adds a specified number of hours to a specified time. AddHours(originalTime, hoursToAdd)
|
Date | Add Days | (Date, Number) |
Adds a specified number of days to a specified date. AddDays(originalDate, daysToAdd)
|
Date | Add Months | (Date, Number) |
Adds a specified number of months to a specified date. AddMonths(originalDate, monthsToAdd)
|
Date | Add Years | (Date, Number) |
Adds a specified number of years to a specified date. AddYears(originalDate, yearsToAdd)
|
Logic | And | (statement1, statement2,…, statementN) |
Evaluates to true if all the statements are true, otherwise evaluates to false. And(statement1, statement2, ..., statementN)
|
Logic | Or | (statement1, statement2,…, statementN) |
Evaluates to true if any of the statements is true, otherwise evaluates to false. Or(statement1, statement2, ..., statementN)
|
Logic | Not | (Condition) |
Negates the value of the specified statement. Not(statement)
|
Logic | Inline If | (Condition, ValueIfTrue, ValueIfFalse) |
Inline if condition. If the condition is true, it returns the first value. If the condition is false, it returns the second value. If(condition, valueIfTrue, valueIfFalse)
|
Relational | Equal (=) | (Input1, Input2) |
Returns true if the two values are the same, otherwise returns false. =(input1, input2)
|
Relational | Greater than (>) | (Input1, Input2) |
Returns true if the first statement is greater than the second statement, otherwise returns false. >(input1, input2)
|
Relational | Less than (<) | (Input1, Input2) |
Returns true if the first statement is less than the second statement, otherwise returns false. <(input1, input2)
|
Relational | Greater than or equal to (>=) | (Input1, Input2) |
Returns true if the first statement is greater than or equal to the second statement, otherwise returns false. >=(input1, input2)
|
Relational | Less than or equal to (<=) | (Input1, Input2) |
Returns true if the first statement is less than or equal to the second statement, otherwise returns false. <=(input1, input2)
|
Math | Sum ( + ) | (Number,Number) |
Adds two values. +(value1, value2)
|
Math | Summation | ([Numbers]) |
Adds the values of multiple numbers. Summation(numbers)
|
Math | Subtract ( - ) | (Number,Number) |
Subtracts two values. -(value1, value2)
|
Math | Multiply ( * ) | ([Number]) |
Multiplies two or more values. *(factors)
|
Math | Divide ( / ) | (Number, Number) |
Divides a value. The result has a scale of 10. For example: 355/113 = 3.1415929203) /(dividend, divisor)
|
Math | Integer Division | (Number, Number) |
Divides numbers and truncates the result (for example, 5/2=2, -5/2=-2). |
Math | Modulo (%) | (Number, Number) |
Finds the remainder after division of one number by another. |
Array | Min | ([Number]) |
Returns the minimum value in a set of numbers. Min(number1, number2, ..., numberN)
|
Array | Max | ([Number]) |
Returns the maximum value in a set of numbers. Max(number1, number2, ..., numberN)
|
Array | Count | ([Any]) |
Counts the number of elements in one or more arrays. Count(array1, array2, ..., arrayN)
|
Array | Avg | ([Number]) |
Calculates the average value of the elements in one or more arrays. Avg(array1, array2, ..., arrayN)
|
Array | Concat | ([Any]) |
Concatenates the specified arrays by appending them at the end of the first array. ConcatArrays(array1, array2, ..., arrayN)
|
Array | Index Of | ([Array], Element) |
Returns the index of an element in an array. For example, IndexOf ([1,2,3], 1) returns 0. Returns -1 if the element specified is not found in the array. |
Array | At Index | ([Any], Index) |
Finds the value at a particular index of an array or string. |
Text | Concat | ([String]) |
Concatenates multiple strings into one single string. Concat(array1, array2, ..., arrayN)
|
Text | Split | (String, String) |
Splits a string into multiple parts based on a separator. The separators delimit the different parts in the string, and aren't part of the result. Split(text, separator)
|
Text | Join | ([String], String) |
Combines multiple strings into one using a separator between the different strings. Join(strings, separator)
|
Text | To Lowercase | (String) |
Converts all the characters in a string to lowercase. ToLowercase(text)
|
Text | To Uppercase | (String or Array, Element) |
Converts all the characters in a string to uppercase. ToUppercase(text)
|
Text | Length | (String) | Returns the number of characters in a string. |
Text | Trim | (String) | Removes leading or trailing spaces in a string. |
Text | Contains | (String or Array, Element) | Checks if a string or array (within a control) contains a specific element and returns a Boolean value. |
Text | Replace | (String, String, String) |
Replaces a text string. Uses three parameters, where:
For example, REPLACE("Hello World!", "Hello", "World") returns "World World!" Note: In regular expressions there are 12 characters with special meanings: backslash \, caret ^, dollar sign $, period or dot ., vertical bar or pipe symbol |, question mark ?, asterisk or star *, plus sign +, opening parenthesis (, the closing parenthesis ), opening square bracket [, and opening curly brace {. If you want to use any of them as regular characters, you will need to escape them with backslash \. |
Text | Matches | (String, String) |
Checks if two strings or expressions match and returns a Boolean value. |
Text | Substring | (String, StartingIndex, Length) |
Returns a substring of a string, beginning at the specified starting index up to the length indicated. For example, Substring ('apple', 0, 3) returns app. |
Interval |
Duration in Minutes |
(Date, Date) |
Calculates the number of minutes between two dates. DurationinMinutes(date1, date2)
|
Interval |
Duration in Hours |
(Date, Date) |
Calculates the number of hours between two dates. DurationinHours(date1, date2)
|
Interval |
Duration in Days |
(Date, Date) |
Calculates the number of days between two dates. DurationinDays(date1, date2).
|
Interval |
Duration in Months |
(Date, Date) |
Calculates the number of months between two dates. DurationinMonths(date1, date2)
|
Interval |
Duration in Years |
(Date, Date) |
Calculates the number of years between two dates. DurationinYears(date1, date2)
|
Objects |
Convert to JSON |
Any |
Converts an object into a String using the JSON format. ConverttoJSON(objectToConvert)
|
Objects |
Parse from JSON |
(String) |
Parses a String that contains content in JSON format and returns an Object. ParsefromJSON(JSONtoParse)
|
Objects |
Get Property |
(Object, String) |
Gets a specified property from an Object. GetProperty(object, property)
|
Current User |
Current user's ID |
None |
Gets the ID for the current logged in user. |
Current User |
Current user's first name |
None |
Gets the first name for the current logged in user. |
Current User |
Current user's middle name |
None |
Gets the middle name for the current logged in user. |
Current User |
Current user's last name |
None |
Gets the last name for the current logged in user. |
Current User |
Current user's email |
None |
Gets the email for the current logged in user. |
Other |
Current UUID |
None |
Generates a universally unique identifier. |
Other |
Current browser language |
None |
Gets the locale that the user's browser is using. |
Other |
Get application name |
None |
Returns the name of the process application. |